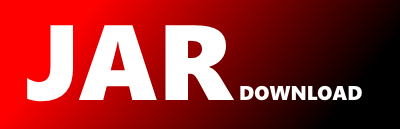
target.apidocs.com.google.api.services.bigquery.model.MaterializedViewDefinition.html Maven / Gradle / Ivy
MaterializedViewDefinition (BigQuery API v2-rev20240905-2.0.0)
com.google.api.services.bigquery.model
Class MaterializedViewDefinition
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.bigquery.model.MaterializedViewDefinition
-
public final class MaterializedViewDefinition
extends com.google.api.client.json.GenericJson
Definition and configuration of a materialized view.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
MaterializedViewDefinition()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
MaterializedViewDefinition
clone()
byte[]
decodeMaxStaleness()
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
MaterializedViewDefinition
encodeMaxStaleness(byte[] maxStaleness)
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
Boolean
getAllowNonIncrementalDefinition()
Optional.
Boolean
getEnableRefresh()
Optional.
Long
getLastRefreshTime()
Output only.
String
getMaxStaleness()
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
String
getQuery()
Required.
Long
getRefreshIntervalMs()
Optional.
MaterializedViewDefinition
set(String fieldName,
Object value)
MaterializedViewDefinition
setAllowNonIncrementalDefinition(Boolean allowNonIncrementalDefinition)
Optional.
MaterializedViewDefinition
setEnableRefresh(Boolean enableRefresh)
Optional.
MaterializedViewDefinition
setLastRefreshTime(Long lastRefreshTime)
Output only.
MaterializedViewDefinition
setMaxStaleness(String maxStaleness)
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
MaterializedViewDefinition
setQuery(String query)
Required.
MaterializedViewDefinition
setRefreshIntervalMs(Long refreshIntervalMs)
Optional.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAllowNonIncrementalDefinition
public Boolean getAllowNonIncrementalDefinition()
Optional. This option declares the intention to construct a materialized view that isn't
refreshed incrementally.
- Returns:
- value or
null
for none
-
setAllowNonIncrementalDefinition
public MaterializedViewDefinition setAllowNonIncrementalDefinition(Boolean allowNonIncrementalDefinition)
Optional. This option declares the intention to construct a materialized view that isn't
refreshed incrementally.
- Parameters:
allowNonIncrementalDefinition
- allowNonIncrementalDefinition or null
for none
-
getEnableRefresh
public Boolean getEnableRefresh()
Optional. Enable automatic refresh of the materialized view when the base table is updated. The
default value is "true".
- Returns:
- value or
null
for none
-
setEnableRefresh
public MaterializedViewDefinition setEnableRefresh(Boolean enableRefresh)
Optional. Enable automatic refresh of the materialized view when the base table is updated. The
default value is "true".
- Parameters:
enableRefresh
- enableRefresh or null
for none
-
getLastRefreshTime
public Long getLastRefreshTime()
Output only. The time when this materialized view was last refreshed, in milliseconds since the
epoch.
- Returns:
- value or
null
for none
-
setLastRefreshTime
public MaterializedViewDefinition setLastRefreshTime(Long lastRefreshTime)
Output only. The time when this materialized view was last refreshed, in milliseconds since the
epoch.
- Parameters:
lastRefreshTime
- lastRefreshTime or null
for none
-
getMaxStaleness
public String getMaxStaleness()
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
- Returns:
- value or
null
for none
- See Also:
decodeMaxStaleness()
-
decodeMaxStaleness
public byte[] decodeMaxStaleness()
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
- Returns:
- Base64 decoded value or
null
for none
- Since:
- 1.14
- See Also:
getMaxStaleness()
-
setMaxStaleness
public MaterializedViewDefinition setMaxStaleness(String maxStaleness)
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
- Parameters:
maxStaleness
- maxStaleness or null
for none
- See Also:
#encodeMaxStaleness()
-
encodeMaxStaleness
public MaterializedViewDefinition encodeMaxStaleness(byte[] maxStaleness)
[Optional] Max staleness of data that could be returned when materizlized view is queried
(formatted as Google SQL Interval type).
- Since:
- 1.14
- See Also:
The value is encoded Base64 or {@code null} for none.
-
getQuery
public String getQuery()
Required. A query whose results are persisted.
- Returns:
- value or
null
for none
-
setQuery
public MaterializedViewDefinition setQuery(String query)
Required. A query whose results are persisted.
- Parameters:
query
- query or null
for none
-
getRefreshIntervalMs
public Long getRefreshIntervalMs()
Optional. The maximum frequency at which this materialized view will be refreshed. The default
value is "1800000" (30 minutes).
- Returns:
- value or
null
for none
-
setRefreshIntervalMs
public MaterializedViewDefinition setRefreshIntervalMs(Long refreshIntervalMs)
Optional. The maximum frequency at which this materialized view will be refreshed. The default
value is "1800000" (30 minutes).
- Parameters:
refreshIntervalMs
- refreshIntervalMs or null
for none
-
set
public MaterializedViewDefinition set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public MaterializedViewDefinition clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy