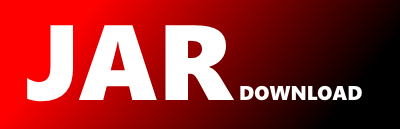
com.google.api.services.bigquery.model.JobStatistics2 Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-05-04 17:28:03 UTC)
* on 2018-06-15 at 19:42:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.bigquery.model;
/**
* Model definition for JobStatistics2.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class JobStatistics2 extends com.google.api.client.json.GenericJson {
/**
* [Output-only] Billing tier for the job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer billingTier;
/**
* [Output-only] Whether the query result was fetched from the query cache.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean cacheHit;
/**
* [Output-only, Experimental] The DDL operation performed, possibly dependent on the pre-
* existence of the DDL target. Possible values (new values might be added in the future):
* "CREATE": The query created the DDL target. "SKIP": No-op. Example cases: the query is CREATE
* TABLE IF NOT EXISTS while the table already exists, or the query is DROP TABLE IF EXISTS while
* the table does not exist. "REPLACE": The query replaced the DDL target. Example case: the query
* is CREATE OR REPLACE TABLE, and the table already exists. "DROP": The query deleted the DDL
* target.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ddlOperationPerformed;
/**
* [Output-only, Experimental] The DDL target table. Present only for CREATE/DROP TABLE/VIEW
* queries.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TableReference ddlTargetTable;
/**
* [Output-only] The original estimate of bytes processed for the job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long estimatedBytesProcessed;
/**
* [Output-only] The number of rows affected by a DML statement. Present only for DML statements
* INSERT, UPDATE or DELETE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numDmlAffectedRows;
/**
* [Output-only] Describes execution plan for the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List queryPlan;
static {
// hack to force ProGuard to consider ExplainQueryStage used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ExplainQueryStage.class);
}
/**
* [Output-only] Referenced tables for the job. Queries that reference more than 50 tables will
* not have a complete list.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List referencedTables;
/**
* [Output-only] Job resource usage breakdown by reservation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List reservationUsage;
static {
// hack to force ProGuard to consider ReservationUsage used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ReservationUsage.class);
}
/**
* [Output-only] The schema of the results. Present only for successful dry run of non-legacy SQL
* queries.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TableSchema schema;
/**
* [Output-only, Experimental] The type of query statement, if valid. Possible values (new values
* might be added in the future): "SELECT": SELECT query. "INSERT": INSERT query; see
* https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language
* "UPDATE": UPDATE query; see https://cloud.google.com/bigquery/docs/reference/standard-sql/data-
* manipulation-language "DELETE": DELETE query; see
* https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language
* "MERGE": MERGE query; see https://cloud.google.com/bigquery/docs/reference/standard-sql/data-
* manipulation-language "CREATE_TABLE": CREATE [OR REPLACE] TABLE without AS SELECT.
* "CREATE_TABLE_AS_SELECT": CREATE [OR REPLACE] TABLE ... AS SELECT ... "DROP_TABLE": DROP TABLE
* query. "CREATE_VIEW": CREATE [OR REPLACE] VIEW ... AS SELECT ... "DROP_VIEW": DROP VIEW query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String statementType;
/**
* [Output-only] [Experimental] Describes a timeline of job execution.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List timeline;
/**
* [Output-only] Total bytes billed for the job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalBytesBilled;
/**
* [Output-only] Total bytes processed for the job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalBytesProcessed;
/**
* [Output-only] Total number of partitions processed from all partitioned tables referenced in
* the job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalPartitionsProcessed;
/**
* [Output-only] Slot-milliseconds for the job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalSlotMs;
/**
* [Output-only, Experimental] Standard SQL only: list of undeclared query parameters detected
* during a dry run validation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List undeclaredQueryParameters;
/**
* [Output-only] Billing tier for the job.
* @return value or {@code null} for none
*/
public java.lang.Integer getBillingTier() {
return billingTier;
}
/**
* [Output-only] Billing tier for the job.
* @param billingTier billingTier or {@code null} for none
*/
public JobStatistics2 setBillingTier(java.lang.Integer billingTier) {
this.billingTier = billingTier;
return this;
}
/**
* [Output-only] Whether the query result was fetched from the query cache.
* @return value or {@code null} for none
*/
public java.lang.Boolean getCacheHit() {
return cacheHit;
}
/**
* [Output-only] Whether the query result was fetched from the query cache.
* @param cacheHit cacheHit or {@code null} for none
*/
public JobStatistics2 setCacheHit(java.lang.Boolean cacheHit) {
this.cacheHit = cacheHit;
return this;
}
/**
* [Output-only, Experimental] The DDL operation performed, possibly dependent on the pre-
* existence of the DDL target. Possible values (new values might be added in the future):
* "CREATE": The query created the DDL target. "SKIP": No-op. Example cases: the query is CREATE
* TABLE IF NOT EXISTS while the table already exists, or the query is DROP TABLE IF EXISTS while
* the table does not exist. "REPLACE": The query replaced the DDL target. Example case: the query
* is CREATE OR REPLACE TABLE, and the table already exists. "DROP": The query deleted the DDL
* target.
* @return value or {@code null} for none
*/
public java.lang.String getDdlOperationPerformed() {
return ddlOperationPerformed;
}
/**
* [Output-only, Experimental] The DDL operation performed, possibly dependent on the pre-
* existence of the DDL target. Possible values (new values might be added in the future):
* "CREATE": The query created the DDL target. "SKIP": No-op. Example cases: the query is CREATE
* TABLE IF NOT EXISTS while the table already exists, or the query is DROP TABLE IF EXISTS while
* the table does not exist. "REPLACE": The query replaced the DDL target. Example case: the query
* is CREATE OR REPLACE TABLE, and the table already exists. "DROP": The query deleted the DDL
* target.
* @param ddlOperationPerformed ddlOperationPerformed or {@code null} for none
*/
public JobStatistics2 setDdlOperationPerformed(java.lang.String ddlOperationPerformed) {
this.ddlOperationPerformed = ddlOperationPerformed;
return this;
}
/**
* [Output-only, Experimental] The DDL target table. Present only for CREATE/DROP TABLE/VIEW
* queries.
* @return value or {@code null} for none
*/
public TableReference getDdlTargetTable() {
return ddlTargetTable;
}
/**
* [Output-only, Experimental] The DDL target table. Present only for CREATE/DROP TABLE/VIEW
* queries.
* @param ddlTargetTable ddlTargetTable or {@code null} for none
*/
public JobStatistics2 setDdlTargetTable(TableReference ddlTargetTable) {
this.ddlTargetTable = ddlTargetTable;
return this;
}
/**
* [Output-only] The original estimate of bytes processed for the job.
* @return value or {@code null} for none
*/
public java.lang.Long getEstimatedBytesProcessed() {
return estimatedBytesProcessed;
}
/**
* [Output-only] The original estimate of bytes processed for the job.
* @param estimatedBytesProcessed estimatedBytesProcessed or {@code null} for none
*/
public JobStatistics2 setEstimatedBytesProcessed(java.lang.Long estimatedBytesProcessed) {
this.estimatedBytesProcessed = estimatedBytesProcessed;
return this;
}
/**
* [Output-only] The number of rows affected by a DML statement. Present only for DML statements
* INSERT, UPDATE or DELETE.
* @return value or {@code null} for none
*/
public java.lang.Long getNumDmlAffectedRows() {
return numDmlAffectedRows;
}
/**
* [Output-only] The number of rows affected by a DML statement. Present only for DML statements
* INSERT, UPDATE or DELETE.
* @param numDmlAffectedRows numDmlAffectedRows or {@code null} for none
*/
public JobStatistics2 setNumDmlAffectedRows(java.lang.Long numDmlAffectedRows) {
this.numDmlAffectedRows = numDmlAffectedRows;
return this;
}
/**
* [Output-only] Describes execution plan for the query.
* @return value or {@code null} for none
*/
public java.util.List getQueryPlan() {
return queryPlan;
}
/**
* [Output-only] Describes execution plan for the query.
* @param queryPlan queryPlan or {@code null} for none
*/
public JobStatistics2 setQueryPlan(java.util.List queryPlan) {
this.queryPlan = queryPlan;
return this;
}
/**
* [Output-only] Referenced tables for the job. Queries that reference more than 50 tables will
* not have a complete list.
* @return value or {@code null} for none
*/
public java.util.List getReferencedTables() {
return referencedTables;
}
/**
* [Output-only] Referenced tables for the job. Queries that reference more than 50 tables will
* not have a complete list.
* @param referencedTables referencedTables or {@code null} for none
*/
public JobStatistics2 setReferencedTables(java.util.List referencedTables) {
this.referencedTables = referencedTables;
return this;
}
/**
* [Output-only] Job resource usage breakdown by reservation.
* @return value or {@code null} for none
*/
public java.util.List getReservationUsage() {
return reservationUsage;
}
/**
* [Output-only] Job resource usage breakdown by reservation.
* @param reservationUsage reservationUsage or {@code null} for none
*/
public JobStatistics2 setReservationUsage(java.util.List reservationUsage) {
this.reservationUsage = reservationUsage;
return this;
}
/**
* [Output-only] The schema of the results. Present only for successful dry run of non-legacy SQL
* queries.
* @return value or {@code null} for none
*/
public TableSchema getSchema() {
return schema;
}
/**
* [Output-only] The schema of the results. Present only for successful dry run of non-legacy SQL
* queries.
* @param schema schema or {@code null} for none
*/
public JobStatistics2 setSchema(TableSchema schema) {
this.schema = schema;
return this;
}
/**
* [Output-only, Experimental] The type of query statement, if valid. Possible values (new values
* might be added in the future): "SELECT": SELECT query. "INSERT": INSERT query; see
* https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language
* "UPDATE": UPDATE query; see https://cloud.google.com/bigquery/docs/reference/standard-sql/data-
* manipulation-language "DELETE": DELETE query; see
* https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language
* "MERGE": MERGE query; see https://cloud.google.com/bigquery/docs/reference/standard-sql/data-
* manipulation-language "CREATE_TABLE": CREATE [OR REPLACE] TABLE without AS SELECT.
* "CREATE_TABLE_AS_SELECT": CREATE [OR REPLACE] TABLE ... AS SELECT ... "DROP_TABLE": DROP TABLE
* query. "CREATE_VIEW": CREATE [OR REPLACE] VIEW ... AS SELECT ... "DROP_VIEW": DROP VIEW query.
* @return value or {@code null} for none
*/
public java.lang.String getStatementType() {
return statementType;
}
/**
* [Output-only, Experimental] The type of query statement, if valid. Possible values (new values
* might be added in the future): "SELECT": SELECT query. "INSERT": INSERT query; see
* https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language
* "UPDATE": UPDATE query; see https://cloud.google.com/bigquery/docs/reference/standard-sql/data-
* manipulation-language "DELETE": DELETE query; see
* https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language
* "MERGE": MERGE query; see https://cloud.google.com/bigquery/docs/reference/standard-sql/data-
* manipulation-language "CREATE_TABLE": CREATE [OR REPLACE] TABLE without AS SELECT.
* "CREATE_TABLE_AS_SELECT": CREATE [OR REPLACE] TABLE ... AS SELECT ... "DROP_TABLE": DROP TABLE
* query. "CREATE_VIEW": CREATE [OR REPLACE] VIEW ... AS SELECT ... "DROP_VIEW": DROP VIEW query.
* @param statementType statementType or {@code null} for none
*/
public JobStatistics2 setStatementType(java.lang.String statementType) {
this.statementType = statementType;
return this;
}
/**
* [Output-only] [Experimental] Describes a timeline of job execution.
* @return value or {@code null} for none
*/
public java.util.List getTimeline() {
return timeline;
}
/**
* [Output-only] [Experimental] Describes a timeline of job execution.
* @param timeline timeline or {@code null} for none
*/
public JobStatistics2 setTimeline(java.util.List timeline) {
this.timeline = timeline;
return this;
}
/**
* [Output-only] Total bytes billed for the job.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalBytesBilled() {
return totalBytesBilled;
}
/**
* [Output-only] Total bytes billed for the job.
* @param totalBytesBilled totalBytesBilled or {@code null} for none
*/
public JobStatistics2 setTotalBytesBilled(java.lang.Long totalBytesBilled) {
this.totalBytesBilled = totalBytesBilled;
return this;
}
/**
* [Output-only] Total bytes processed for the job.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalBytesProcessed() {
return totalBytesProcessed;
}
/**
* [Output-only] Total bytes processed for the job.
* @param totalBytesProcessed totalBytesProcessed or {@code null} for none
*/
public JobStatistics2 setTotalBytesProcessed(java.lang.Long totalBytesProcessed) {
this.totalBytesProcessed = totalBytesProcessed;
return this;
}
/**
* [Output-only] Total number of partitions processed from all partitioned tables referenced in
* the job.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalPartitionsProcessed() {
return totalPartitionsProcessed;
}
/**
* [Output-only] Total number of partitions processed from all partitioned tables referenced in
* the job.
* @param totalPartitionsProcessed totalPartitionsProcessed or {@code null} for none
*/
public JobStatistics2 setTotalPartitionsProcessed(java.lang.Long totalPartitionsProcessed) {
this.totalPartitionsProcessed = totalPartitionsProcessed;
return this;
}
/**
* [Output-only] Slot-milliseconds for the job.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalSlotMs() {
return totalSlotMs;
}
/**
* [Output-only] Slot-milliseconds for the job.
* @param totalSlotMs totalSlotMs or {@code null} for none
*/
public JobStatistics2 setTotalSlotMs(java.lang.Long totalSlotMs) {
this.totalSlotMs = totalSlotMs;
return this;
}
/**
* [Output-only, Experimental] Standard SQL only: list of undeclared query parameters detected
* during a dry run validation.
* @return value or {@code null} for none
*/
public java.util.List getUndeclaredQueryParameters() {
return undeclaredQueryParameters;
}
/**
* [Output-only, Experimental] Standard SQL only: list of undeclared query parameters detected
* during a dry run validation.
* @param undeclaredQueryParameters undeclaredQueryParameters or {@code null} for none
*/
public JobStatistics2 setUndeclaredQueryParameters(java.util.List undeclaredQueryParameters) {
this.undeclaredQueryParameters = undeclaredQueryParameters;
return this;
}
@Override
public JobStatistics2 set(String fieldName, Object value) {
return (JobStatistics2) super.set(fieldName, value);
}
@Override
public JobStatistics2 clone() {
return (JobStatistics2) super.clone();
}
/**
* Model definition for JobStatistics2ReservationUsage.
*/
public static final class ReservationUsage extends com.google.api.client.json.GenericJson {
/**
* [Output-only] Reservation name or "unreserved" for on-demand resources usage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* [Output-only] Slot-milliseconds the job spent in the given reservation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long slotMs;
/**
* [Output-only] Reservation name or "unreserved" for on-demand resources usage.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* [Output-only] Reservation name or "unreserved" for on-demand resources usage.
* @param name name or {@code null} for none
*/
public ReservationUsage setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* [Output-only] Slot-milliseconds the job spent in the given reservation.
* @return value or {@code null} for none
*/
public java.lang.Long getSlotMs() {
return slotMs;
}
/**
* [Output-only] Slot-milliseconds the job spent in the given reservation.
* @param slotMs slotMs or {@code null} for none
*/
public ReservationUsage setSlotMs(java.lang.Long slotMs) {
this.slotMs = slotMs;
return this;
}
@Override
public ReservationUsage set(String fieldName, Object value) {
return (ReservationUsage) super.set(fieldName, value);
}
@Override
public ReservationUsage clone() {
return (ReservationUsage) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy