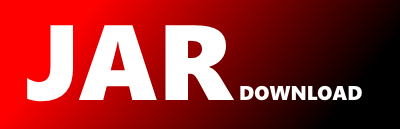
com.google.api.services.bigqueryreservation.v1.BigQueryReservation Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.bigqueryreservation.v1;
/**
* Service definition for BigQueryReservation (v1).
*
*
* A service to modify your BigQuery flat-rate reservations.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link BigQueryReservationRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class BigQueryReservation extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the BigQuery Reservation API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://bigqueryreservation.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://bigqueryreservation.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public BigQueryReservation(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
BigQueryReservation(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code BigQueryReservation bigqueryreservation = new BigQueryReservation(...);}
* {@code BigQueryReservation.Projects.List request = bigqueryreservation.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code BigQueryReservation bigqueryreservation = new BigQueryReservation(...);}
* {@code BigQueryReservation.Locations.List request = bigqueryreservation.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Retrieves a BI reservation.
*
* Create a request for the method "locations.getBiReservation".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link GetBiReservation#execute()} method to invoke the remote
* operation.
*
* @param name Required. Name of the requested reservation, for example:
* `projects/{project_id}/locations/{location_id}/biReservation`
* @return the request
*/
public GetBiReservation getBiReservation(java.lang.String name) throws java.io.IOException {
GetBiReservation result = new GetBiReservation(name);
initialize(result);
return result;
}
public class GetBiReservation extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/biReservation$");
/**
* Retrieves a BI reservation.
*
* Create a request for the method "locations.getBiReservation".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link GetBiReservation#execute()} method to invoke the
* remote operation. {@link GetBiReservation#initialize(com.google.api.client.googleapis.servi
* ces.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. Name of the requested reservation, for example:
* `projects/{project_id}/locations/{location_id}/biReservation`
* @since 1.13
*/
protected GetBiReservation(java.lang.String name) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.BiReservation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/biReservation$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetBiReservation set$Xgafv(java.lang.String $Xgafv) {
return (GetBiReservation) super.set$Xgafv($Xgafv);
}
@Override
public GetBiReservation setAccessToken(java.lang.String accessToken) {
return (GetBiReservation) super.setAccessToken(accessToken);
}
@Override
public GetBiReservation setAlt(java.lang.String alt) {
return (GetBiReservation) super.setAlt(alt);
}
@Override
public GetBiReservation setCallback(java.lang.String callback) {
return (GetBiReservation) super.setCallback(callback);
}
@Override
public GetBiReservation setFields(java.lang.String fields) {
return (GetBiReservation) super.setFields(fields);
}
@Override
public GetBiReservation setKey(java.lang.String key) {
return (GetBiReservation) super.setKey(key);
}
@Override
public GetBiReservation setOauthToken(java.lang.String oauthToken) {
return (GetBiReservation) super.setOauthToken(oauthToken);
}
@Override
public GetBiReservation setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetBiReservation) super.setPrettyPrint(prettyPrint);
}
@Override
public GetBiReservation setQuotaUser(java.lang.String quotaUser) {
return (GetBiReservation) super.setQuotaUser(quotaUser);
}
@Override
public GetBiReservation setUploadType(java.lang.String uploadType) {
return (GetBiReservation) super.setUploadType(uploadType);
}
@Override
public GetBiReservation setUploadProtocol(java.lang.String uploadProtocol) {
return (GetBiReservation) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the requested reservation, for example:
* `projects/{project_id}/locations/{location_id}/biReservation`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the requested reservation, for example:
`projects/{project_id}/locations/{location_id}/biReservation`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the requested reservation, for example:
* `projects/{project_id}/locations/{location_id}/biReservation`
*/
public GetBiReservation setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/biReservation$");
}
this.name = name;
return this;
}
@Override
public GetBiReservation set(String parameterName, Object value) {
return (GetBiReservation) super.set(parameterName, value);
}
}
/**
* Looks up assignments for a specified resource for a particular region. If the request is about a
* project: 1. Assignments created on the project will be returned if they exist. 2. Otherwise
* assignments created on the closest ancestor will be returned. 3. Assignments for different
* JobTypes will all be returned. The same logic applies if the request is about a folder. If the
* request is about an organization, then assignments created on the organization will be returned
* (organization doesn't have ancestors). Comparing to ListAssignments, there are some behavior
* differences: 1. permission on the assignee will be verified in this API. 2. Hierarchy lookup
* (project->folder->organization) happens in this API. 3. Parent here is `projects/locations`,
* instead of `projects/locationsreservations`.
*
* Create a request for the method "locations.searchAllAssignments".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link SearchAllAssignments#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The resource name with location (project name could be the wildcard '-'), e.g.:
* `projects/-/locations/US`.
* @return the request
*/
public SearchAllAssignments searchAllAssignments(java.lang.String parent) throws java.io.IOException {
SearchAllAssignments result = new SearchAllAssignments(parent);
initialize(result);
return result;
}
public class SearchAllAssignments extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}:searchAllAssignments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Looks up assignments for a specified resource for a particular region. If the request is about
* a project: 1. Assignments created on the project will be returned if they exist. 2. Otherwise
* assignments created on the closest ancestor will be returned. 3. Assignments for different
* JobTypes will all be returned. The same logic applies if the request is about a folder. If the
* request is about an organization, then assignments created on the organization will be returned
* (organization doesn't have ancestors). Comparing to ListAssignments, there are some behavior
* differences: 1. permission on the assignee will be verified in this API. 2. Hierarchy lookup
* (project->folder->organization) happens in this API. 3. Parent here is `projects/locations`,
* instead of `projects/locationsreservations`.
*
* Create a request for the method "locations.searchAllAssignments".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link SearchAllAssignments#execute()} method to invoke the
* remote operation. {@link SearchAllAssignments#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent Required. The resource name with location (project name could be the wildcard '-'), e.g.:
* `projects/-/locations/US`.
* @since 1.13
*/
protected SearchAllAssignments(java.lang.String parent) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.SearchAllAssignmentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchAllAssignments set$Xgafv(java.lang.String $Xgafv) {
return (SearchAllAssignments) super.set$Xgafv($Xgafv);
}
@Override
public SearchAllAssignments setAccessToken(java.lang.String accessToken) {
return (SearchAllAssignments) super.setAccessToken(accessToken);
}
@Override
public SearchAllAssignments setAlt(java.lang.String alt) {
return (SearchAllAssignments) super.setAlt(alt);
}
@Override
public SearchAllAssignments setCallback(java.lang.String callback) {
return (SearchAllAssignments) super.setCallback(callback);
}
@Override
public SearchAllAssignments setFields(java.lang.String fields) {
return (SearchAllAssignments) super.setFields(fields);
}
@Override
public SearchAllAssignments setKey(java.lang.String key) {
return (SearchAllAssignments) super.setKey(key);
}
@Override
public SearchAllAssignments setOauthToken(java.lang.String oauthToken) {
return (SearchAllAssignments) super.setOauthToken(oauthToken);
}
@Override
public SearchAllAssignments setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchAllAssignments) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchAllAssignments setQuotaUser(java.lang.String quotaUser) {
return (SearchAllAssignments) super.setQuotaUser(quotaUser);
}
@Override
public SearchAllAssignments setUploadType(java.lang.String uploadType) {
return (SearchAllAssignments) super.setUploadType(uploadType);
}
@Override
public SearchAllAssignments setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchAllAssignments) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name with location (project name could be the wildcard '-'), e.g.:
* `projects/-/locations/US`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name with location (project name could be the wildcard '-'), e.g.:
`projects/-/locations/US`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name with location (project name could be the wildcard '-'), e.g.:
* `projects/-/locations/US`.
*/
public SearchAllAssignments setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of items to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of items to return per page. */
public SearchAllAssignments setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous List request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous List request, if any. */
public SearchAllAssignments setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Please specify resource name as assignee in the query. Examples: *
* `assignee=projects/myproject` * `assignee=folders/123` * `assignee=organizations/456`
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Please specify resource name as assignee in the query. Examples: * `assignee=projects/myproject` *
`assignee=folders/123` * `assignee=organizations/456`
*/
public java.lang.String getQuery() {
return query;
}
/**
* Please specify resource name as assignee in the query. Examples: *
* `assignee=projects/myproject` * `assignee=folders/123` * `assignee=organizations/456`
*/
public SearchAllAssignments setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public SearchAllAssignments set(String parameterName, Object value) {
return (SearchAllAssignments) super.set(parameterName, value);
}
}
/**
* Deprecated: Looks up assignments for a specified resource for a particular region. If the request
* is about a project: 1. Assignments created on the project will be returned if they exist. 2.
* Otherwise assignments created on the closest ancestor will be returned. 3. Assignments for
* different JobTypes will all be returned. The same logic applies if the request is about a folder.
* If the request is about an organization, then assignments created on the organization will be
* returned (organization doesn't have ancestors). Comparing to ListAssignments, there are some
* behavior differences: 1. permission on the assignee will be verified in this API. 2. Hierarchy
* lookup (project->folder->organization) happens in this API. 3. Parent here is
* `projects/locations`, instead of `projects/locationsreservations`. **Note** "-" cannot be used
* for projects nor locations.
*
* Create a request for the method "locations.searchAssignments".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link SearchAssignments#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The resource name of the admin project(containing project and location), e.g.:
* `projects/myproject/locations/US`.
* @return the request
*/
public SearchAssignments searchAssignments(java.lang.String parent) throws java.io.IOException {
SearchAssignments result = new SearchAssignments(parent);
initialize(result);
return result;
}
public class SearchAssignments extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}:searchAssignments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Deprecated: Looks up assignments for a specified resource for a particular region. If the
* request is about a project: 1. Assignments created on the project will be returned if they
* exist. 2. Otherwise assignments created on the closest ancestor will be returned. 3.
* Assignments for different JobTypes will all be returned. The same logic applies if the request
* is about a folder. If the request is about an organization, then assignments created on the
* organization will be returned (organization doesn't have ancestors). Comparing to
* ListAssignments, there are some behavior differences: 1. permission on the assignee will be
* verified in this API. 2. Hierarchy lookup (project->folder->organization) happens in this API.
* 3. Parent here is `projects/locations`, instead of `projects/locationsreservations`. **Note**
* "-" cannot be used for projects nor locations.
*
* Create a request for the method "locations.searchAssignments".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link SearchAssignments#execute()} method to invoke the
* remote operation. {@link SearchAssignments#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The resource name of the admin project(containing project and location), e.g.:
* `projects/myproject/locations/US`.
* @since 1.13
*/
protected SearchAssignments(java.lang.String parent) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.SearchAssignmentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchAssignments set$Xgafv(java.lang.String $Xgafv) {
return (SearchAssignments) super.set$Xgafv($Xgafv);
}
@Override
public SearchAssignments setAccessToken(java.lang.String accessToken) {
return (SearchAssignments) super.setAccessToken(accessToken);
}
@Override
public SearchAssignments setAlt(java.lang.String alt) {
return (SearchAssignments) super.setAlt(alt);
}
@Override
public SearchAssignments setCallback(java.lang.String callback) {
return (SearchAssignments) super.setCallback(callback);
}
@Override
public SearchAssignments setFields(java.lang.String fields) {
return (SearchAssignments) super.setFields(fields);
}
@Override
public SearchAssignments setKey(java.lang.String key) {
return (SearchAssignments) super.setKey(key);
}
@Override
public SearchAssignments setOauthToken(java.lang.String oauthToken) {
return (SearchAssignments) super.setOauthToken(oauthToken);
}
@Override
public SearchAssignments setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchAssignments) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchAssignments setQuotaUser(java.lang.String quotaUser) {
return (SearchAssignments) super.setQuotaUser(quotaUser);
}
@Override
public SearchAssignments setUploadType(java.lang.String uploadType) {
return (SearchAssignments) super.setUploadType(uploadType);
}
@Override
public SearchAssignments setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchAssignments) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the admin project(containing project and location), e.g.:
* `projects/myproject/locations/US`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the admin project(containing project and location), e.g.:
`projects/myproject/locations/US`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the admin project(containing project and location), e.g.:
* `projects/myproject/locations/US`.
*/
public SearchAssignments setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of items to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of items to return per page. */
public SearchAssignments setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous List request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous List request, if any. */
public SearchAssignments setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Please specify resource name as assignee in the query. Examples: *
* `assignee=projects/myproject` * `assignee=folders/123` * `assignee=organizations/456`
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Please specify resource name as assignee in the query. Examples: * `assignee=projects/myproject` *
`assignee=folders/123` * `assignee=organizations/456`
*/
public java.lang.String getQuery() {
return query;
}
/**
* Please specify resource name as assignee in the query. Examples: *
* `assignee=projects/myproject` * `assignee=folders/123` * `assignee=organizations/456`
*/
public SearchAssignments setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public SearchAssignments set(String parameterName, Object value) {
return (SearchAssignments) super.set(parameterName, value);
}
}
/**
* Updates a BI reservation. Only fields specified in the `field_mask` are updated. A singleton BI
* reservation always exists with default size 0. In order to reserve BI capacity it needs to be
* updated to an amount greater than 0. In order to release BI capacity reservation size must be set
* to 0.
*
* Create a request for the method "locations.updateBiReservation".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link UpdateBiReservation#execute()} method to invoke the remote
* operation.
*
* @param name The resource name of the singleton BI reservation. Reservation names have the form
* `projects/{project_id}/locations/{location_id}/biReservation`.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.BiReservation}
* @return the request
*/
public UpdateBiReservation updateBiReservation(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.BiReservation content) throws java.io.IOException {
UpdateBiReservation result = new UpdateBiReservation(name, content);
initialize(result);
return result;
}
public class UpdateBiReservation extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/biReservation$");
/**
* Updates a BI reservation. Only fields specified in the `field_mask` are updated. A singleton BI
* reservation always exists with default size 0. In order to reserve BI capacity it needs to be
* updated to an amount greater than 0. In order to release BI capacity reservation size must be
* set to 0.
*
* Create a request for the method "locations.updateBiReservation".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link UpdateBiReservation#execute()} method to invoke the
* remote operation. {@link UpdateBiReservation#initialize(com.google.api.client.googleapis.se
* rvices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name The resource name of the singleton BI reservation. Reservation names have the form
* `projects/{project_id}/locations/{location_id}/biReservation`.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.BiReservation}
* @since 1.13
*/
protected UpdateBiReservation(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.BiReservation content) {
super(BigQueryReservation.this, "PATCH", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.BiReservation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/biReservation$");
}
}
@Override
public UpdateBiReservation set$Xgafv(java.lang.String $Xgafv) {
return (UpdateBiReservation) super.set$Xgafv($Xgafv);
}
@Override
public UpdateBiReservation setAccessToken(java.lang.String accessToken) {
return (UpdateBiReservation) super.setAccessToken(accessToken);
}
@Override
public UpdateBiReservation setAlt(java.lang.String alt) {
return (UpdateBiReservation) super.setAlt(alt);
}
@Override
public UpdateBiReservation setCallback(java.lang.String callback) {
return (UpdateBiReservation) super.setCallback(callback);
}
@Override
public UpdateBiReservation setFields(java.lang.String fields) {
return (UpdateBiReservation) super.setFields(fields);
}
@Override
public UpdateBiReservation setKey(java.lang.String key) {
return (UpdateBiReservation) super.setKey(key);
}
@Override
public UpdateBiReservation setOauthToken(java.lang.String oauthToken) {
return (UpdateBiReservation) super.setOauthToken(oauthToken);
}
@Override
public UpdateBiReservation setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateBiReservation) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateBiReservation setQuotaUser(java.lang.String quotaUser) {
return (UpdateBiReservation) super.setQuotaUser(quotaUser);
}
@Override
public UpdateBiReservation setUploadType(java.lang.String uploadType) {
return (UpdateBiReservation) super.setUploadType(uploadType);
}
@Override
public UpdateBiReservation setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateBiReservation) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the singleton BI reservation. Reservation names have the form
* `projects/{project_id}/locations/{location_id}/biReservation`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the singleton BI reservation. Reservation names have the form
`projects/{project_id}/locations/{location_id}/biReservation`.
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the singleton BI reservation. Reservation names have the form
* `projects/{project_id}/locations/{location_id}/biReservation`.
*/
public UpdateBiReservation setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/biReservation$");
}
this.name = name;
return this;
}
/** A list of fields to be updated in this request. */
@com.google.api.client.util.Key
private String updateMask;
/** A list of fields to be updated in this request.
*/
public String getUpdateMask() {
return updateMask;
}
/** A list of fields to be updated in this request. */
public UpdateBiReservation setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateBiReservation set(String parameterName, Object value) {
return (UpdateBiReservation) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the CapacityCommitments collection.
*
* The typical use is:
*
* {@code BigQueryReservation bigqueryreservation = new BigQueryReservation(...);}
* {@code BigQueryReservation.CapacityCommitments.List request = bigqueryreservation.capacityCommitments().list(parameters ...)}
*
*
* @return the resource collection
*/
public CapacityCommitments capacityCommitments() {
return new CapacityCommitments();
}
/**
* The "capacityCommitments" collection of methods.
*/
public class CapacityCommitments {
/**
* Creates a new capacity commitment resource.
*
* Create a request for the method "capacityCommitments.create".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the parent reservation. E.g., `projects/myproject/locations/US`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}/capacityCommitments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new capacity commitment resource.
*
* Create a request for the method "capacityCommitments.create".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the parent reservation. E.g., `projects/myproject/locations/US`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment content) {
super(BigQueryReservation.this, "POST", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the parent reservation. E.g.,
* `projects/myproject/locations/US`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the parent reservation. E.g., `projects/myproject/locations/US`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the parent reservation. E.g.,
* `projects/myproject/locations/US`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The optional capacity commitment ID. Capacity commitment name will be generated
* automatically if this field is empty. This field must only contain lower case
* alphanumeric characters or dashes. The first and last character cannot be a dash. Max
* length is 64 characters. NOTE: this ID won't be kept if the capacity commitment is
* split or merged.
*/
@com.google.api.client.util.Key
private java.lang.String capacityCommitmentId;
/** The optional capacity commitment ID. Capacity commitment name will be generated automatically if
this field is empty. This field must only contain lower case alphanumeric characters or dashes. The
first and last character cannot be a dash. Max length is 64 characters. NOTE: this ID won't be kept
if the capacity commitment is split or merged.
*/
public java.lang.String getCapacityCommitmentId() {
return capacityCommitmentId;
}
/**
* The optional capacity commitment ID. Capacity commitment name will be generated
* automatically if this field is empty. This field must only contain lower case
* alphanumeric characters or dashes. The first and last character cannot be a dash. Max
* length is 64 characters. NOTE: this ID won't be kept if the capacity commitment is
* split or merged.
*/
public Create setCapacityCommitmentId(java.lang.String capacityCommitmentId) {
this.capacityCommitmentId = capacityCommitmentId;
return this;
}
/**
* If true, fail the request if another project in the organization has a capacity
* commitment.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enforceSingleAdminProjectPerOrg;
/** If true, fail the request if another project in the organization has a capacity commitment.
*/
public java.lang.Boolean getEnforceSingleAdminProjectPerOrg() {
return enforceSingleAdminProjectPerOrg;
}
/**
* If true, fail the request if another project in the organization has a capacity
* commitment.
*/
public Create setEnforceSingleAdminProjectPerOrg(java.lang.Boolean enforceSingleAdminProjectPerOrg) {
this.enforceSingleAdminProjectPerOrg = enforceSingleAdminProjectPerOrg;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a capacity commitment. Attempting to delete capacity commitment before its
* commitment_end_time will fail with the error code `google.rpc.Code.FAILED_PRECONDITION`.
*
* Create a request for the method "capacityCommitments.delete".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the capacity commitment to delete. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
/**
* Deletes a capacity commitment. Attempting to delete capacity commitment before its
* commitment_end_time will fail with the error code `google.rpc.Code.FAILED_PRECONDITION`.
*
* Create a request for the method "capacityCommitments.delete".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the capacity commitment to delete. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(BigQueryReservation.this, "DELETE", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the capacity commitment to delete. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the capacity commitment to delete. E.g.,
`projects/myproject/locations/US/capacityCommitments/123`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the capacity commitment to delete. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
this.name = name;
return this;
}
/**
* Can be used to force delete commitments even if assignments exist. Deleting commitments
* with assignments may cause queries to fail if they no longer have access to slots.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Can be used to force delete commitments even if assignments exist. Deleting commitments with
assignments may cause queries to fail if they no longer have access to slots.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Can be used to force delete commitments even if assignments exist. Deleting commitments
* with assignments may cause queries to fail if they no longer have access to slots.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns information about the capacity commitment.
*
* Create a request for the method "capacityCommitments.get".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the capacity commitment to retrieve. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
/**
* Returns information about the capacity commitment.
*
* Create a request for the method "capacityCommitments.get".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the capacity commitment to retrieve. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the capacity commitment to retrieve. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the capacity commitment to retrieve. E.g.,
`projects/myproject/locations/US/capacityCommitments/123`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the capacity commitment to retrieve. E.g.,
* `projects/myproject/locations/US/capacityCommitments/123`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the capacity commitments for the admin project.
*
* Create a request for the method "capacityCommitments.list".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the parent reservation. E.g., `projects/myproject/locations/US`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}/capacityCommitments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists all the capacity commitments for the admin project.
*
* Create a request for the method "capacityCommitments.list".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the parent reservation. E.g., `projects/myproject/locations/US`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.ListCapacityCommitmentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the parent reservation. E.g.,
* `projects/myproject/locations/US`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the parent reservation. E.g., `projects/myproject/locations/US`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the parent reservation. E.g.,
* `projects/myproject/locations/US`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of items to return. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of items to return. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous List request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous List request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Merges capacity commitments of the same plan into a single commitment. The resulting capacity
* commitment has the greater commitment_end_time out of the to-be-merged capacity commitments.
* Attempting to merge capacity commitments of different plan will fail with the error code
* `google.rpc.Code.FAILED_PRECONDITION`.
*
* Create a request for the method "capacityCommitments.merge".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Merge#execute()} method to invoke the remote operation.
*
* @param parent Parent resource that identifies admin project and location e.g., `projects/myproject/locations/us`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.MergeCapacityCommitmentsRequest}
* @return the request
*/
public Merge merge(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.MergeCapacityCommitmentsRequest content) throws java.io.IOException {
Merge result = new Merge(parent, content);
initialize(result);
return result;
}
public class Merge extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}/capacityCommitments:merge";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Merges capacity commitments of the same plan into a single commitment. The resulting capacity
* commitment has the greater commitment_end_time out of the to-be-merged capacity commitments.
* Attempting to merge capacity commitments of different plan will fail with the error code
* `google.rpc.Code.FAILED_PRECONDITION`.
*
* Create a request for the method "capacityCommitments.merge".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Merge#execute()} method to invoke the remote
* operation. {@link
* Merge#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Parent resource that identifies admin project and location e.g., `projects/myproject/locations/us`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.MergeCapacityCommitmentsRequest}
* @since 1.13
*/
protected Merge(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.MergeCapacityCommitmentsRequest content) {
super(BigQueryReservation.this, "POST", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Merge set$Xgafv(java.lang.String $Xgafv) {
return (Merge) super.set$Xgafv($Xgafv);
}
@Override
public Merge setAccessToken(java.lang.String accessToken) {
return (Merge) super.setAccessToken(accessToken);
}
@Override
public Merge setAlt(java.lang.String alt) {
return (Merge) super.setAlt(alt);
}
@Override
public Merge setCallback(java.lang.String callback) {
return (Merge) super.setCallback(callback);
}
@Override
public Merge setFields(java.lang.String fields) {
return (Merge) super.setFields(fields);
}
@Override
public Merge setKey(java.lang.String key) {
return (Merge) super.setKey(key);
}
@Override
public Merge setOauthToken(java.lang.String oauthToken) {
return (Merge) super.setOauthToken(oauthToken);
}
@Override
public Merge setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Merge) super.setPrettyPrint(prettyPrint);
}
@Override
public Merge setQuotaUser(java.lang.String quotaUser) {
return (Merge) super.setQuotaUser(quotaUser);
}
@Override
public Merge setUploadType(java.lang.String uploadType) {
return (Merge) super.setUploadType(uploadType);
}
@Override
public Merge setUploadProtocol(java.lang.String uploadProtocol) {
return (Merge) super.setUploadProtocol(uploadProtocol);
}
/**
* Parent resource that identifies admin project and location e.g.,
* `projects/myproject/locations/us`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Parent resource that identifies admin project and location e.g., `projects/myproject/locations/us`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Parent resource that identifies admin project and location e.g.,
* `projects/myproject/locations/us`
*/
public Merge setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Merge set(String parameterName, Object value) {
return (Merge) super.set(parameterName, value);
}
}
/**
* Updates an existing capacity commitment. Only `plan` and `renewal_plan` fields can be updated.
* Plan can only be changed to a plan of a longer commitment period. Attempting to change to a plan
* with shorter commitment period will fail with the error code
* `google.rpc.Code.FAILED_PRECONDITION`.
*
* Create a request for the method "capacityCommitments.patch".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of the capacity commitment, e.g.,
* `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only
* contain lower case alphanumeric characters or dashes. It must start with a letter and must
* not end with a dash. Its maximum length is 64 characters.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
/**
* Updates an existing capacity commitment. Only `plan` and `renewal_plan` fields can be updated.
* Plan can only be changed to a plan of a longer commitment period. Attempting to change to a
* plan with shorter commitment period will fail with the error code
* `google.rpc.Code.FAILED_PRECONDITION`.
*
* Create a request for the method "capacityCommitments.patch".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of the capacity commitment, e.g.,
* `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only
* contain lower case alphanumeric characters or dashes. It must start with a letter and must
* not end with a dash. Its maximum length is 64 characters.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment content) {
super(BigQueryReservation.this, "PATCH", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of the capacity commitment, e.g.,
* `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only
* contain lower case alphanumeric characters or dashes. It must start with a letter and
* must not end with a dash. Its maximum length is 64 characters.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of the capacity commitment, e.g.,
`projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only contain lower
case alphanumeric characters or dashes. It must start with a letter and must not end with a dash.
Its maximum length is 64 characters.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the capacity commitment, e.g.,
* `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only
* contain lower case alphanumeric characters or dashes. It must start with a letter and
* must not end with a dash. Its maximum length is 64 characters.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
this.name = name;
return this;
}
/** Standard field mask for the set of fields to be updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Standard field mask for the set of fields to be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Standard field mask for the set of fields to be updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Splits capacity commitment to two commitments of the same plan and `commitment_end_time`. A
* common use case is to enable downgrading commitments. For example, in order to downgrade from
* 10000 slots to 8000, you might split a 10000 capacity commitment into commitments of 2000 and
* 8000. Then, you delete the first one after the commitment end time passes.
*
* Create a request for the method "capacityCommitments.split".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Split#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name e.g.,: `projects/myproject/locations/US/capacityCommitments/123`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.SplitCapacityCommitmentRequest}
* @return the request
*/
public Split split(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.SplitCapacityCommitmentRequest content) throws java.io.IOException {
Split result = new Split(name, content);
initialize(result);
return result;
}
public class Split extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}:split";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
/**
* Splits capacity commitment to two commitments of the same plan and `commitment_end_time`. A
* common use case is to enable downgrading commitments. For example, in order to downgrade from
* 10000 slots to 8000, you might split a 10000 capacity commitment into commitments of 2000 and
* 8000. Then, you delete the first one after the commitment end time passes.
*
* Create a request for the method "capacityCommitments.split".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Split#execute()} method to invoke the remote
* operation. {@link
* Split#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name e.g.,: `projects/myproject/locations/US/capacityCommitments/123`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.SplitCapacityCommitmentRequest}
* @since 1.13
*/
protected Split(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.SplitCapacityCommitmentRequest content) {
super(BigQueryReservation.this, "POST", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.SplitCapacityCommitmentResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
}
@Override
public Split set$Xgafv(java.lang.String $Xgafv) {
return (Split) super.set$Xgafv($Xgafv);
}
@Override
public Split setAccessToken(java.lang.String accessToken) {
return (Split) super.setAccessToken(accessToken);
}
@Override
public Split setAlt(java.lang.String alt) {
return (Split) super.setAlt(alt);
}
@Override
public Split setCallback(java.lang.String callback) {
return (Split) super.setCallback(callback);
}
@Override
public Split setFields(java.lang.String fields) {
return (Split) super.setFields(fields);
}
@Override
public Split setKey(java.lang.String key) {
return (Split) super.setKey(key);
}
@Override
public Split setOauthToken(java.lang.String oauthToken) {
return (Split) super.setOauthToken(oauthToken);
}
@Override
public Split setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Split) super.setPrettyPrint(prettyPrint);
}
@Override
public Split setQuotaUser(java.lang.String quotaUser) {
return (Split) super.setQuotaUser(quotaUser);
}
@Override
public Split setUploadType(java.lang.String uploadType) {
return (Split) super.setUploadType(uploadType);
}
@Override
public Split setUploadProtocol(java.lang.String uploadProtocol) {
return (Split) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name e.g.,:
* `projects/myproject/locations/US/capacityCommitments/123`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name e.g.,: `projects/myproject/locations/US/capacityCommitments/123`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name e.g.,:
* `projects/myproject/locations/US/capacityCommitments/123`
*/
public Split setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/capacityCommitments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Split set(String parameterName, Object value) {
return (Split) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Reservations collection.
*
* The typical use is:
*
* {@code BigQueryReservation bigqueryreservation = new BigQueryReservation(...);}
* {@code BigQueryReservation.Reservations.List request = bigqueryreservation.reservations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Reservations reservations() {
return new Reservations();
}
/**
* The "reservations" collection of methods.
*/
public class Reservations {
/**
* Creates a new reservation resource.
*
* Create a request for the method "reservations.create".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Project, location. E.g., `projects/myproject/locations/US`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Reservation}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.Reservation content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}/reservations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new reservation resource.
*
* Create a request for the method "reservations.create".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Project, location. E.g., `projects/myproject/locations/US`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Reservation}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.Reservation content) {
super(BigQueryReservation.this, "POST", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.Reservation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. Project, location. E.g., `projects/myproject/locations/US` */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Project, location. E.g., `projects/myproject/locations/US`
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Project, location. E.g., `projects/myproject/locations/US` */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The reservation ID. It must only contain lower case alphanumeric characters or dashes.
* It must start with a letter and must not end with a dash. Its maximum length is 64
* characters.
*/
@com.google.api.client.util.Key
private java.lang.String reservationId;
/** The reservation ID. It must only contain lower case alphanumeric characters or dashes. It must
start with a letter and must not end with a dash. Its maximum length is 64 characters.
*/
public java.lang.String getReservationId() {
return reservationId;
}
/**
* The reservation ID. It must only contain lower case alphanumeric characters or dashes.
* It must start with a letter and must not end with a dash. Its maximum length is 64
* characters.
*/
public Create setReservationId(java.lang.String reservationId) {
this.reservationId = reservationId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a reservation. Returns `google.rpc.Code.FAILED_PRECONDITION` when reservation has
* assignments.
*
* Create a request for the method "reservations.delete".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
/**
* Deletes a reservation. Returns `google.rpc.Code.FAILED_PRECONDITION` when reservation has
* assignments.
*
* Create a request for the method "reservations.delete".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(BigQueryReservation.this, "DELETE", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the reservation to retrieve. E.g.,
`projects/myproject/locations/US/reservations/team1-prod`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns information about the reservation.
*
* Create a request for the method "reservations.get".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
/**
* Returns information about the reservation.
*
* Create a request for the method "reservations.get".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.Reservation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the reservation to retrieve. E.g.,
`projects/myproject/locations/US/reservations/team1-prod`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the reservation to retrieve. E.g.,
* `projects/myproject/locations/US/reservations/team1-prod`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the reservations for the project in the specified location.
*
* Create a request for the method "reservations.list".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource name containing project and location, e.g.:
* `projects/myproject/locations/US`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}/reservations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists all the reservations for the project in the specified location.
*
* Create a request for the method "reservations.list".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource name containing project and location, e.g.:
* `projects/myproject/locations/US`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.ListReservationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource name containing project and location, e.g.:
* `projects/myproject/locations/US`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource name containing project and location, e.g.:
`projects/myproject/locations/US`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource name containing project and location, e.g.:
* `projects/myproject/locations/US`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of items to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of items to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous List request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous List request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing reservation resource.
*
* Create a request for the method "reservations.patch".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The resource name of the reservation, e.g., `projects/locations/reservations/team1-prod`. The
* reservation_id must only contain lower case alphanumeric characters or dashes. It must
* start with a letter and must not end with a dash. Its maximum length is 64 characters.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Reservation}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.Reservation content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
/**
* Updates an existing reservation resource.
*
* Create a request for the method "reservations.patch".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource name of the reservation, e.g., `projects/locations/reservations/team1-prod`. The
* reservation_id must only contain lower case alphanumeric characters or dashes. It must
* start with a letter and must not end with a dash. Its maximum length is 64 characters.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Reservation}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.Reservation content) {
super(BigQueryReservation.this, "PATCH", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.Reservation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the reservation, e.g.,
* `projects/locations/reservations/team1-prod`. The reservation_id must only contain
* lower case alphanumeric characters or dashes. It must start with a letter and must not
* end with a dash. Its maximum length is 64 characters.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the reservation, e.g., `projects/locations/reservations/team1-prod`. The
reservation_id must only contain lower case alphanumeric characters or dashes. It must start with a
letter and must not end with a dash. Its maximum length is 64 characters.
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the reservation, e.g.,
* `projects/locations/reservations/team1-prod`. The reservation_id must only contain
* lower case alphanumeric characters or dashes. It must start with a letter and must not
* end with a dash. Its maximum length is 64 characters.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
this.name = name;
return this;
}
/** Standard field mask for the set of fields to be updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Standard field mask for the set of fields to be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Standard field mask for the set of fields to be updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Assignments collection.
*
* The typical use is:
*
* {@code BigQueryReservation bigqueryreservation = new BigQueryReservation(...);}
* {@code BigQueryReservation.Assignments.List request = bigqueryreservation.assignments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Assignments assignments() {
return new Assignments();
}
/**
* The "assignments" collection of methods.
*/
public class Assignments {
/**
* Creates an assignment object which allows the given project to submit jobs of a certain type
* using slots from the specified reservation. Currently a resource (project, folder, organization)
* can only have one assignment per each (job_type, location) combination, and that reservation will
* be used for all jobs of the matching type. Different assignments can be created on different
* levels of the projects, folders or organization hierarchy. During query execution, the assignment
* is looked up at the project, folder and organization levels in that order. The first assignment
* found is applied to the query. When creating assignments, it does not matter if other assignments
* exist at higher levels. Example: * The organization `organizationA` contains two projects,
* `project1` and `project2`. * Assignments for all three entities (`organizationA`, `project1`, and
* `project2`) could all be created and mapped to the same or different reservations. "None"
* assignments represent an absence of the assignment. Projects assigned to None use on-demand
* pricing. To create a "None" assignment, use "none" as a reservation_id in the parent. Example
* parent: `projects/myproject/locations/US/reservations/none`. Returns
* `google.rpc.Code.PERMISSION_DENIED` if user does not have 'bigquery.admin' permissions on the
* project using the reservation and the project that owns this reservation. Returns
* `google.rpc.Code.INVALID_ARGUMENT` when location of the assignment does not match location of the
* reservation.
*
* Create a request for the method "assignments.create".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource name of the assignment E.g.
* `projects/myproject/locations/US/reservations/team1-prod`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Assignment}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.Assignment content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}/assignments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
/**
* Creates an assignment object which allows the given project to submit jobs of a certain type
* using slots from the specified reservation. Currently a resource (project, folder,
* organization) can only have one assignment per each (job_type, location) combination, and that
* reservation will be used for all jobs of the matching type. Different assignments can be
* created on different levels of the projects, folders or organization hierarchy. During query
* execution, the assignment is looked up at the project, folder and organization levels in that
* order. The first assignment found is applied to the query. When creating assignments, it does
* not matter if other assignments exist at higher levels. Example: * The organization
* `organizationA` contains two projects, `project1` and `project2`. * Assignments for all three
* entities (`organizationA`, `project1`, and `project2`) could all be created and mapped to the
* same or different reservations. "None" assignments represent an absence of the assignment.
* Projects assigned to None use on-demand pricing. To create a "None" assignment, use "none" as a
* reservation_id in the parent. Example parent:
* `projects/myproject/locations/US/reservations/none`. Returns
* `google.rpc.Code.PERMISSION_DENIED` if user does not have 'bigquery.admin' permissions on the
* project using the reservation and the project that owns this reservation. Returns
* `google.rpc.Code.INVALID_ARGUMENT` when location of the assignment does not match location of
* the reservation.
*
* Create a request for the method "assignments.create".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource name of the assignment E.g.
* `projects/myproject/locations/US/reservations/team1-prod`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Assignment}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.bigqueryreservation.v1.model.Assignment content) {
super(BigQueryReservation.this, "POST", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.Assignment.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource name of the assignment E.g.
* `projects/myproject/locations/US/reservations/team1-prod`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource name of the assignment E.g.
`projects/myproject/locations/US/reservations/team1-prod`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource name of the assignment E.g.
* `projects/myproject/locations/US/reservations/team1-prod`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The optional assignment ID. Assignment name will be generated automatically if this
* field is empty. This field must only contain lower case alphanumeric characters or
* dashes. Max length is 64 characters.
*/
@com.google.api.client.util.Key
private java.lang.String assignmentId;
/** The optional assignment ID. Assignment name will be generated automatically if this field is empty.
This field must only contain lower case alphanumeric characters or dashes. Max length is 64
characters.
*/
public java.lang.String getAssignmentId() {
return assignmentId;
}
/**
* The optional assignment ID. Assignment name will be generated automatically if this
* field is empty. This field must only contain lower case alphanumeric characters or
* dashes. Max length is 64 characters.
*/
public Create setAssignmentId(java.lang.String assignmentId) {
this.assignmentId = assignmentId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a assignment. No expansion will happen. Example: * Organization `organizationA` contains
* two projects, `project1` and `project2`. * Reservation `res1` exists and was created previously.
* * CreateAssignment was used previously to define the following associations between entities and
* reservations: `` and `` In this example, deletion of the `` assignment won't affect the other
* assignment ``. After said deletion, queries from `project1` will still use `res1` while queries
* from `project2` will switch to use on-demand mode.
*
* Create a request for the method "assignments.delete".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the resource, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
/**
* Deletes a assignment. No expansion will happen. Example: * Organization `organizationA`
* contains two projects, `project1` and `project2`. * Reservation `res1` exists and was created
* previously. * CreateAssignment was used previously to define the following associations between
* entities and reservations: `` and `` In this example, deletion of the `` assignment won't
* affect the other assignment ``. After said deletion, queries from `project1` will still use
* `res1` while queries from `project2` will switch to use on-demand mode.
*
* Create a request for the method "assignments.delete".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the resource, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(BigQueryReservation.this, "DELETE", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the resource, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the resource, e.g.
`projects/myproject/locations/US/reservations/team1-prod/assignments/123`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the resource, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists assignments. Only explicitly created assignments will be returned. Example: * Organization
* `organizationA` contains two projects, `project1` and `project2`. * Reservation `res1` exists and
* was created previously. * CreateAssignment was used previously to define the following
* associations between entities and reservations: `` and `` In this example, ListAssignments will
* just return the above two assignments for reservation `res1`, and no expansion/merge will happen.
* The wildcard "-" can be used for reservations in the request. In that case all assignments
* belongs to the specified project and location will be listed. **Note** "-" cannot be used for
* projects nor locations.
*
* Create a request for the method "assignments.list".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource name e.g.: `projects/myproject/locations/US/reservations/team1-prod`
* Or: `projects/myproject/locations/US/reservations/-`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+parent}/assignments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
/**
* Lists assignments. Only explicitly created assignments will be returned. Example: *
* Organization `organizationA` contains two projects, `project1` and `project2`. * Reservation
* `res1` exists and was created previously. * CreateAssignment was used previously to define the
* following associations between entities and reservations: `` and `` In this example,
* ListAssignments will just return the above two assignments for reservation `res1`, and no
* expansion/merge will happen. The wildcard "-" can be used for reservations in the request. In
* that case all assignments belongs to the specified project and location will be listed.
* **Note** "-" cannot be used for projects nor locations.
*
* Create a request for the method "assignments.list".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource name e.g.: `projects/myproject/locations/US/reservations/team1-prod`
* Or: `projects/myproject/locations/US/reservations/-`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(BigQueryReservation.this, "GET", REST_PATH, null, com.google.api.services.bigqueryreservation.v1.model.ListAssignmentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource name e.g.:
* `projects/myproject/locations/US/reservations/team1-prod` Or:
* `projects/myproject/locations/US/reservations/-`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource name e.g.: `projects/myproject/locations/US/reservations/team1-prod`
Or: `projects/myproject/locations/US/reservations/-`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource name e.g.:
* `projects/myproject/locations/US/reservations/team1-prod` Or:
* `projects/myproject/locations/US/reservations/-`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of items to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of items to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous List request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous List request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Moves an assignment under a new reservation. This differs from removing an existing assignment
* and recreating a new one by providing a transactional change that ensures an assignee always has
* an associated reservation.
*
* Create a request for the method "assignments.move".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Move#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the assignment, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.MoveAssignmentRequest}
* @return the request
*/
public Move move(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.MoveAssignmentRequest content) throws java.io.IOException {
Move result = new Move(name, content);
initialize(result);
return result;
}
public class Move extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}:move";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
/**
* Moves an assignment under a new reservation. This differs from removing an existing assignment
* and recreating a new one by providing a transactional change that ensures an assignee always
* has an associated reservation.
*
* Create a request for the method "assignments.move".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Move#execute()} method to invoke the remote operation.
* {@link
* Move#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the assignment, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.MoveAssignmentRequest}
* @since 1.13
*/
protected Move(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.MoveAssignmentRequest content) {
super(BigQueryReservation.this, "POST", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.Assignment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
}
}
@Override
public Move set$Xgafv(java.lang.String $Xgafv) {
return (Move) super.set$Xgafv($Xgafv);
}
@Override
public Move setAccessToken(java.lang.String accessToken) {
return (Move) super.setAccessToken(accessToken);
}
@Override
public Move setAlt(java.lang.String alt) {
return (Move) super.setAlt(alt);
}
@Override
public Move setCallback(java.lang.String callback) {
return (Move) super.setCallback(callback);
}
@Override
public Move setFields(java.lang.String fields) {
return (Move) super.setFields(fields);
}
@Override
public Move setKey(java.lang.String key) {
return (Move) super.setKey(key);
}
@Override
public Move setOauthToken(java.lang.String oauthToken) {
return (Move) super.setOauthToken(oauthToken);
}
@Override
public Move setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Move) super.setPrettyPrint(prettyPrint);
}
@Override
public Move setQuotaUser(java.lang.String quotaUser) {
return (Move) super.setQuotaUser(quotaUser);
}
@Override
public Move setUploadType(java.lang.String uploadType) {
return (Move) super.setUploadType(uploadType);
}
@Override
public Move setUploadProtocol(java.lang.String uploadProtocol) {
return (Move) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the assignment, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the assignment, e.g.
`projects/myproject/locations/US/reservations/team1-prod/assignments/123`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the assignment, e.g.
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`
*/
public Move setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Move set(String parameterName, Object value) {
return (Move) super.set(parameterName, value);
}
}
/**
* Updates an existing assignment. Only the `priority` field can be updated.
*
* Create a request for the method "assignments.patch".
*
* This request holds the parameters needed by the bigqueryreservation server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Name of the resource. E.g.:
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`. The
* assignment_id must only contain lower case alphanumeric characters or dashes and the max
* length is 64 characters.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Assignment}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.Assignment content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends BigQueryReservationRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
/**
* Updates an existing assignment. Only the `priority` field can be updated.
*
* Create a request for the method "assignments.patch".
*
* This request holds the parameters needed by the the bigqueryreservation server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Name of the resource. E.g.:
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`. The
* assignment_id must only contain lower case alphanumeric characters or dashes and the max
* length is 64 characters.
* @param content the {@link com.google.api.services.bigqueryreservation.v1.model.Assignment}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.bigqueryreservation.v1.model.Assignment content) {
super(BigQueryReservation.this, "PATCH", REST_PATH, content, com.google.api.services.bigqueryreservation.v1.model.Assignment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Name of the resource. E.g.:
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`. The
* assignment_id must only contain lower case alphanumeric characters or dashes and the
* max length is 64 characters.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Name of the resource. E.g.:
`projects/myproject/locations/US/reservations/team1-prod/assignments/123`. The assignment_id must
only contain lower case alphanumeric characters or dashes and the max length is 64 characters.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Name of the resource. E.g.:
* `projects/myproject/locations/US/reservations/team1-prod/assignments/123`. The
* assignment_id must only contain lower case alphanumeric characters or dashes and the
* max length is 64 characters.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/reservations/[^/]+/assignments/[^/]+$");
}
this.name = name;
return this;
}
/** Standard field mask for the set of fields to be updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Standard field mask for the set of fields to be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Standard field mask for the set of fields to be updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
}
}
/**
* Builder for {@link BigQueryReservation}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link BigQueryReservation}. */
@Override
public BigQueryReservation build() {
return new BigQueryReservation(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link BigQueryReservationRequestInitializer}.
*
* @since 1.12
*/
public Builder setBigQueryReservationRequestInitializer(
BigQueryReservationRequestInitializer bigqueryreservationRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(bigqueryreservationRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}