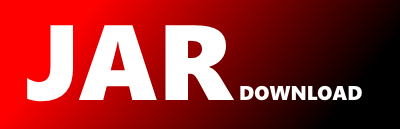
com.google.api.services.bigqueryreservation.v1.model.Reservation Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.bigqueryreservation.v1.model;
/**
* A reservation is a mechanism used to guarantee slots to users.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the BigQuery Reservation API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Reservation extends com.google.api.client.json.GenericJson {
/**
* The configuration parameters for the auto scaling feature. Note this is an alpha feature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Autoscale autoscale;
/**
* Job concurrency target which sets a soft upper bound on the number of jobs that can run
* concurrently in this reservation. This is a soft target due to asynchronous nature of the
* system and various optimizations for small queries. Default value is 0 which means that
* concurrency target will be automatically computed by the system. NOTE: this field is exposed as
* `target_job_concurrency` in the Information Schema, DDL and BQ CLI.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long concurrency;
/**
* Output only. Creation time of the reservation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String creationTime;
/**
* Edition of the reservation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String edition;
/**
* If false, any query or pipeline job using this reservation will use idle slots from other
* reservations within the same admin project. If true, a query or pipeline job using this
* reservation will execute with the slot capacity specified in the slot_capacity field at most.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean ignoreIdleSlots;
/**
* Applicable only for reservations located within one of the BigQuery multi-regions (US or EU).
* If set to true, this reservation is placed in the organization's secondary region which is
* designated for disaster recovery purposes. If false, this reservation is placed in the
* organization's default region. NOTE: this is a preview feature. Project must be allow-listed in
* order to set this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean multiRegionAuxiliary;
/**
* The resource name of the reservation, e.g., `projects/locations/reservations/team1-prod`. The
* reservation_id must only contain lower case alphanumeric characters or dashes. It must start
* with a letter and must not end with a dash. Its maximum length is 64 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Minimum slots available to this reservation. A slot is a unit of computational power in
* BigQuery, and serves as the unit of parallelism. Queries using this reservation might use more
* slots during runtime if ignore_idle_slots is set to false. If total slot_capacity of the
* reservation and its siblings exceeds the total slot_count of all capacity commitments, the
* request will fail with `google.rpc.Code.RESOURCE_EXHAUSTED`. NOTE: for reservations in US or EU
* multi-regions, slot capacity constraints are checked separately for default and auxiliary
* regions. See multi_region_auxiliary flag for more details.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long slotCapacity;
/**
* Output only. Last update time of the reservation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* The configuration parameters for the auto scaling feature. Note this is an alpha feature.
* @return value or {@code null} for none
*/
public Autoscale getAutoscale() {
return autoscale;
}
/**
* The configuration parameters for the auto scaling feature. Note this is an alpha feature.
* @param autoscale autoscale or {@code null} for none
*/
public Reservation setAutoscale(Autoscale autoscale) {
this.autoscale = autoscale;
return this;
}
/**
* Job concurrency target which sets a soft upper bound on the number of jobs that can run
* concurrently in this reservation. This is a soft target due to asynchronous nature of the
* system and various optimizations for small queries. Default value is 0 which means that
* concurrency target will be automatically computed by the system. NOTE: this field is exposed as
* `target_job_concurrency` in the Information Schema, DDL and BQ CLI.
* @return value or {@code null} for none
*/
public java.lang.Long getConcurrency() {
return concurrency;
}
/**
* Job concurrency target which sets a soft upper bound on the number of jobs that can run
* concurrently in this reservation. This is a soft target due to asynchronous nature of the
* system and various optimizations for small queries. Default value is 0 which means that
* concurrency target will be automatically computed by the system. NOTE: this field is exposed as
* `target_job_concurrency` in the Information Schema, DDL and BQ CLI.
* @param concurrency concurrency or {@code null} for none
*/
public Reservation setConcurrency(java.lang.Long concurrency) {
this.concurrency = concurrency;
return this;
}
/**
* Output only. Creation time of the reservation.
* @return value or {@code null} for none
*/
public String getCreationTime() {
return creationTime;
}
/**
* Output only. Creation time of the reservation.
* @param creationTime creationTime or {@code null} for none
*/
public Reservation setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* Edition of the reservation.
* @return value or {@code null} for none
*/
public java.lang.String getEdition() {
return edition;
}
/**
* Edition of the reservation.
* @param edition edition or {@code null} for none
*/
public Reservation setEdition(java.lang.String edition) {
this.edition = edition;
return this;
}
/**
* If false, any query or pipeline job using this reservation will use idle slots from other
* reservations within the same admin project. If true, a query or pipeline job using this
* reservation will execute with the slot capacity specified in the slot_capacity field at most.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIgnoreIdleSlots() {
return ignoreIdleSlots;
}
/**
* If false, any query or pipeline job using this reservation will use idle slots from other
* reservations within the same admin project. If true, a query or pipeline job using this
* reservation will execute with the slot capacity specified in the slot_capacity field at most.
* @param ignoreIdleSlots ignoreIdleSlots or {@code null} for none
*/
public Reservation setIgnoreIdleSlots(java.lang.Boolean ignoreIdleSlots) {
this.ignoreIdleSlots = ignoreIdleSlots;
return this;
}
/**
* Applicable only for reservations located within one of the BigQuery multi-regions (US or EU).
* If set to true, this reservation is placed in the organization's secondary region which is
* designated for disaster recovery purposes. If false, this reservation is placed in the
* organization's default region. NOTE: this is a preview feature. Project must be allow-listed in
* order to set this field.
* @return value or {@code null} for none
*/
public java.lang.Boolean getMultiRegionAuxiliary() {
return multiRegionAuxiliary;
}
/**
* Applicable only for reservations located within one of the BigQuery multi-regions (US or EU).
* If set to true, this reservation is placed in the organization's secondary region which is
* designated for disaster recovery purposes. If false, this reservation is placed in the
* organization's default region. NOTE: this is a preview feature. Project must be allow-listed in
* order to set this field.
* @param multiRegionAuxiliary multiRegionAuxiliary or {@code null} for none
*/
public Reservation setMultiRegionAuxiliary(java.lang.Boolean multiRegionAuxiliary) {
this.multiRegionAuxiliary = multiRegionAuxiliary;
return this;
}
/**
* The resource name of the reservation, e.g., `projects/locations/reservations/team1-prod`. The
* reservation_id must only contain lower case alphanumeric characters or dashes. It must start
* with a letter and must not end with a dash. Its maximum length is 64 characters.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the reservation, e.g., `projects/locations/reservations/team1-prod`. The
* reservation_id must only contain lower case alphanumeric characters or dashes. It must start
* with a letter and must not end with a dash. Its maximum length is 64 characters.
* @param name name or {@code null} for none
*/
public Reservation setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Minimum slots available to this reservation. A slot is a unit of computational power in
* BigQuery, and serves as the unit of parallelism. Queries using this reservation might use more
* slots during runtime if ignore_idle_slots is set to false. If total slot_capacity of the
* reservation and its siblings exceeds the total slot_count of all capacity commitments, the
* request will fail with `google.rpc.Code.RESOURCE_EXHAUSTED`. NOTE: for reservations in US or EU
* multi-regions, slot capacity constraints are checked separately for default and auxiliary
* regions. See multi_region_auxiliary flag for more details.
* @return value or {@code null} for none
*/
public java.lang.Long getSlotCapacity() {
return slotCapacity;
}
/**
* Minimum slots available to this reservation. A slot is a unit of computational power in
* BigQuery, and serves as the unit of parallelism. Queries using this reservation might use more
* slots during runtime if ignore_idle_slots is set to false. If total slot_capacity of the
* reservation and its siblings exceeds the total slot_count of all capacity commitments, the
* request will fail with `google.rpc.Code.RESOURCE_EXHAUSTED`. NOTE: for reservations in US or EU
* multi-regions, slot capacity constraints are checked separately for default and auxiliary
* regions. See multi_region_auxiliary flag for more details.
* @param slotCapacity slotCapacity or {@code null} for none
*/
public Reservation setSlotCapacity(java.lang.Long slotCapacity) {
this.slotCapacity = slotCapacity;
return this;
}
/**
* Output only. Last update time of the reservation.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. Last update time of the reservation.
* @param updateTime updateTime or {@code null} for none
*/
public Reservation setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public Reservation set(String fieldName, Object value) {
return (Reservation) super.set(fieldName, value);
}
@Override
public Reservation clone() {
return (Reservation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy