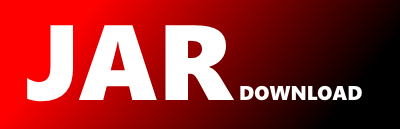
com.google.api.services.bigqueryreservation.v1.model.CapacityCommitment Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.bigqueryreservation.v1.model;
/**
* Capacity commitment is a way to purchase compute capacity for BigQuery jobs (in the form of
* slots) with some committed period of usage. Annual commitments renew by default. Commitments can
* be removed after their commitment end time passes. In order to remove annual commitment, its plan
* needs to be changed to monthly or flex first. A capacity commitment resource exists as a child
* resource of the admin project.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the BigQuery Reservation API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CapacityCommitment extends com.google.api.client.json.GenericJson {
/**
* Output only. The end of the current commitment period. It is applicable only for ACTIVE
* capacity commitments. Note after renewal, commitment_end_time is the time the renewed
* commitment expires. So itwould be at a time after commitment_start_time + committed period,
* because we don't change commitment_start_time ,
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String commitmentEndTime;
/**
* Output only. The start of the current commitment period. It is applicable only for ACTIVE
* capacity commitments. Note after the commitment is renewed, commitment_start_time won't be
* changed. It refers to the start time of the original commitment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String commitmentStartTime;
/**
* Edition of the capacity commitment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String edition;
/**
* Output only. For FAILED commitment plan, provides the reason of failure.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Status failureStatus;
/**
* Output only. If true, the commitment is a flat-rate commitment, otherwise, it's an edition
* commitment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isFlatRate;
/**
* Applicable only for commitments located within one of the BigQuery multi-regions (US or EU). If
* set to true, this commitment is placed in the organization's secondary region which is
* designated for disaster recovery purposes. If false, this commitment is placed in the
* organization's default region. NOTE: this is a preview feature. Project must be allow-listed in
* order to set this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean multiRegionAuxiliary;
/**
* Output only. The resource name of the capacity commitment, e.g.,
* `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only contain
* lower case alphanumeric characters or dashes. It must start with a letter and must not end with
* a dash. Its maximum length is 64 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Capacity commitment commitment plan.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String plan;
/**
* The plan this capacity commitment is converted to after commitment_end_time passes. Once the
* plan is changed, committed period is extended according to commitment plan. Only applicable for
* ANNUAL and TRIAL commitments.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String renewalPlan;
/**
* Number of slots in this commitment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long slotCount;
/**
* Output only. State of the commitment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Output only. The end of the current commitment period. It is applicable only for ACTIVE
* capacity commitments. Note after renewal, commitment_end_time is the time the renewed
* commitment expires. So itwould be at a time after commitment_start_time + committed period,
* because we don't change commitment_start_time ,
* @return value or {@code null} for none
*/
public String getCommitmentEndTime() {
return commitmentEndTime;
}
/**
* Output only. The end of the current commitment period. It is applicable only for ACTIVE
* capacity commitments. Note after renewal, commitment_end_time is the time the renewed
* commitment expires. So itwould be at a time after commitment_start_time + committed period,
* because we don't change commitment_start_time ,
* @param commitmentEndTime commitmentEndTime or {@code null} for none
*/
public CapacityCommitment setCommitmentEndTime(String commitmentEndTime) {
this.commitmentEndTime = commitmentEndTime;
return this;
}
/**
* Output only. The start of the current commitment period. It is applicable only for ACTIVE
* capacity commitments. Note after the commitment is renewed, commitment_start_time won't be
* changed. It refers to the start time of the original commitment.
* @return value or {@code null} for none
*/
public String getCommitmentStartTime() {
return commitmentStartTime;
}
/**
* Output only. The start of the current commitment period. It is applicable only for ACTIVE
* capacity commitments. Note after the commitment is renewed, commitment_start_time won't be
* changed. It refers to the start time of the original commitment.
* @param commitmentStartTime commitmentStartTime or {@code null} for none
*/
public CapacityCommitment setCommitmentStartTime(String commitmentStartTime) {
this.commitmentStartTime = commitmentStartTime;
return this;
}
/**
* Edition of the capacity commitment.
* @return value or {@code null} for none
*/
public java.lang.String getEdition() {
return edition;
}
/**
* Edition of the capacity commitment.
* @param edition edition or {@code null} for none
*/
public CapacityCommitment setEdition(java.lang.String edition) {
this.edition = edition;
return this;
}
/**
* Output only. For FAILED commitment plan, provides the reason of failure.
* @return value or {@code null} for none
*/
public Status getFailureStatus() {
return failureStatus;
}
/**
* Output only. For FAILED commitment plan, provides the reason of failure.
* @param failureStatus failureStatus or {@code null} for none
*/
public CapacityCommitment setFailureStatus(Status failureStatus) {
this.failureStatus = failureStatus;
return this;
}
/**
* Output only. If true, the commitment is a flat-rate commitment, otherwise, it's an edition
* commitment.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsFlatRate() {
return isFlatRate;
}
/**
* Output only. If true, the commitment is a flat-rate commitment, otherwise, it's an edition
* commitment.
* @param isFlatRate isFlatRate or {@code null} for none
*/
public CapacityCommitment setIsFlatRate(java.lang.Boolean isFlatRate) {
this.isFlatRate = isFlatRate;
return this;
}
/**
* Applicable only for commitments located within one of the BigQuery multi-regions (US or EU). If
* set to true, this commitment is placed in the organization's secondary region which is
* designated for disaster recovery purposes. If false, this commitment is placed in the
* organization's default region. NOTE: this is a preview feature. Project must be allow-listed in
* order to set this field.
* @return value or {@code null} for none
*/
public java.lang.Boolean getMultiRegionAuxiliary() {
return multiRegionAuxiliary;
}
/**
* Applicable only for commitments located within one of the BigQuery multi-regions (US or EU). If
* set to true, this commitment is placed in the organization's secondary region which is
* designated for disaster recovery purposes. If false, this commitment is placed in the
* organization's default region. NOTE: this is a preview feature. Project must be allow-listed in
* order to set this field.
* @param multiRegionAuxiliary multiRegionAuxiliary or {@code null} for none
*/
public CapacityCommitment setMultiRegionAuxiliary(java.lang.Boolean multiRegionAuxiliary) {
this.multiRegionAuxiliary = multiRegionAuxiliary;
return this;
}
/**
* Output only. The resource name of the capacity commitment, e.g.,
* `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only contain
* lower case alphanumeric characters or dashes. It must start with a letter and must not end with
* a dash. Its maximum length is 64 characters.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the capacity commitment, e.g.,
* `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only contain
* lower case alphanumeric characters or dashes. It must start with a letter and must not end with
* a dash. Its maximum length is 64 characters.
* @param name name or {@code null} for none
*/
public CapacityCommitment setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Capacity commitment commitment plan.
* @return value or {@code null} for none
*/
public java.lang.String getPlan() {
return plan;
}
/**
* Capacity commitment commitment plan.
* @param plan plan or {@code null} for none
*/
public CapacityCommitment setPlan(java.lang.String plan) {
this.plan = plan;
return this;
}
/**
* The plan this capacity commitment is converted to after commitment_end_time passes. Once the
* plan is changed, committed period is extended according to commitment plan. Only applicable for
* ANNUAL and TRIAL commitments.
* @return value or {@code null} for none
*/
public java.lang.String getRenewalPlan() {
return renewalPlan;
}
/**
* The plan this capacity commitment is converted to after commitment_end_time passes. Once the
* plan is changed, committed period is extended according to commitment plan. Only applicable for
* ANNUAL and TRIAL commitments.
* @param renewalPlan renewalPlan or {@code null} for none
*/
public CapacityCommitment setRenewalPlan(java.lang.String renewalPlan) {
this.renewalPlan = renewalPlan;
return this;
}
/**
* Number of slots in this commitment.
* @return value or {@code null} for none
*/
public java.lang.Long getSlotCount() {
return slotCount;
}
/**
* Number of slots in this commitment.
* @param slotCount slotCount or {@code null} for none
*/
public CapacityCommitment setSlotCount(java.lang.Long slotCount) {
this.slotCount = slotCount;
return this;
}
/**
* Output only. State of the commitment.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. State of the commitment.
* @param state state or {@code null} for none
*/
public CapacityCommitment setState(java.lang.String state) {
this.state = state;
return this;
}
@Override
public CapacityCommitment set(String fieldName, Object value) {
return (CapacityCommitment) super.set(fieldName, value);
}
@Override
public CapacityCommitment clone() {
return (CapacityCommitment) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy