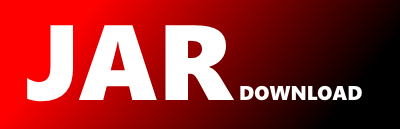
com.google.api.services.bigtableadmin.v2.model.Table Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.bigtableadmin.v2.model;
/**
* A collection of user data indexed by row, column, and timestamp. Each table is served using the
* resources of its parent cluster.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Bigtable Admin API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Table extends com.google.api.client.json.GenericJson {
/**
* If specified, automated backups are enabled for this table. Otherwise, automated backups are
* disabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AutomatedBackupPolicy automatedBackupPolicy;
/**
* If specified, enable the change stream on this table. Otherwise, the change stream is disabled
* and the change stream is not retained.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ChangeStreamConfig changeStreamConfig;
/**
* Output only. Map from cluster ID to per-cluster table state. If it could not be determined
* whether or not the table has data in a particular cluster (for example, if its zone is
* unavailable), then there will be an entry for the cluster with UNKNOWN `replication_status`.
* Views: `REPLICATION_VIEW`, `ENCRYPTION_VIEW`, `FULL`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map clusterStates;
static {
// hack to force ProGuard to consider ClusterState used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ClusterState.class);
}
/**
* The column families configured for this table, mapped by column family ID. Views:
* `SCHEMA_VIEW`, `STATS_VIEW`, `FULL`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map columnFamilies;
static {
// hack to force ProGuard to consider ColumnFamily used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ColumnFamily.class);
}
/**
* Set to true to make the table protected against data loss. i.e. deleting the following
* resources through Admin APIs are prohibited: * The table. * The column families in the table. *
* The instance containing the table. Note one can still delete the data stored in the table
* through Data APIs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean deletionProtection;
/**
* Immutable. The granularity (i.e. `MILLIS`) at which timestamps are stored in this table.
* Timestamps not matching the granularity will be rejected. If unspecified at creation time, the
* value will be set to `MILLIS`. Views: `SCHEMA_VIEW`, `FULL`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String granularity;
/**
* The unique name of the table. Values are of the form
* `projects/{project}/instances/{instance}/tables/_a-zA-Z0-9*`. Views: `NAME_ONLY`,
* `SCHEMA_VIEW`, `REPLICATION_VIEW`, `STATS_VIEW`, `FULL`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. If this table was restored from another data source (e.g. a backup), this field
* will be populated with information about the restore.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RestoreInfo restoreInfo;
/**
* Output only. Only available with STATS_VIEW, this includes summary statistics about the entire
* table contents. For statistics about a specific column family, see ColumnFamilyStats in the
* mapped ColumnFamily collection above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TableStats stats;
/**
* If specified, automated backups are enabled for this table. Otherwise, automated backups are
* disabled.
* @return value or {@code null} for none
*/
public AutomatedBackupPolicy getAutomatedBackupPolicy() {
return automatedBackupPolicy;
}
/**
* If specified, automated backups are enabled for this table. Otherwise, automated backups are
* disabled.
* @param automatedBackupPolicy automatedBackupPolicy or {@code null} for none
*/
public Table setAutomatedBackupPolicy(AutomatedBackupPolicy automatedBackupPolicy) {
this.automatedBackupPolicy = automatedBackupPolicy;
return this;
}
/**
* If specified, enable the change stream on this table. Otherwise, the change stream is disabled
* and the change stream is not retained.
* @return value or {@code null} for none
*/
public ChangeStreamConfig getChangeStreamConfig() {
return changeStreamConfig;
}
/**
* If specified, enable the change stream on this table. Otherwise, the change stream is disabled
* and the change stream is not retained.
* @param changeStreamConfig changeStreamConfig or {@code null} for none
*/
public Table setChangeStreamConfig(ChangeStreamConfig changeStreamConfig) {
this.changeStreamConfig = changeStreamConfig;
return this;
}
/**
* Output only. Map from cluster ID to per-cluster table state. If it could not be determined
* whether or not the table has data in a particular cluster (for example, if its zone is
* unavailable), then there will be an entry for the cluster with UNKNOWN `replication_status`.
* Views: `REPLICATION_VIEW`, `ENCRYPTION_VIEW`, `FULL`
* @return value or {@code null} for none
*/
public java.util.Map getClusterStates() {
return clusterStates;
}
/**
* Output only. Map from cluster ID to per-cluster table state. If it could not be determined
* whether or not the table has data in a particular cluster (for example, if its zone is
* unavailable), then there will be an entry for the cluster with UNKNOWN `replication_status`.
* Views: `REPLICATION_VIEW`, `ENCRYPTION_VIEW`, `FULL`
* @param clusterStates clusterStates or {@code null} for none
*/
public Table setClusterStates(java.util.Map clusterStates) {
this.clusterStates = clusterStates;
return this;
}
/**
* The column families configured for this table, mapped by column family ID. Views:
* `SCHEMA_VIEW`, `STATS_VIEW`, `FULL`
* @return value or {@code null} for none
*/
public java.util.Map getColumnFamilies() {
return columnFamilies;
}
/**
* The column families configured for this table, mapped by column family ID. Views:
* `SCHEMA_VIEW`, `STATS_VIEW`, `FULL`
* @param columnFamilies columnFamilies or {@code null} for none
*/
public Table setColumnFamilies(java.util.Map columnFamilies) {
this.columnFamilies = columnFamilies;
return this;
}
/**
* Set to true to make the table protected against data loss. i.e. deleting the following
* resources through Admin APIs are prohibited: * The table. * The column families in the table. *
* The instance containing the table. Note one can still delete the data stored in the table
* through Data APIs.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDeletionProtection() {
return deletionProtection;
}
/**
* Set to true to make the table protected against data loss. i.e. deleting the following
* resources through Admin APIs are prohibited: * The table. * The column families in the table. *
* The instance containing the table. Note one can still delete the data stored in the table
* through Data APIs.
* @param deletionProtection deletionProtection or {@code null} for none
*/
public Table setDeletionProtection(java.lang.Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
return this;
}
/**
* Immutable. The granularity (i.e. `MILLIS`) at which timestamps are stored in this table.
* Timestamps not matching the granularity will be rejected. If unspecified at creation time, the
* value will be set to `MILLIS`. Views: `SCHEMA_VIEW`, `FULL`.
* @return value or {@code null} for none
*/
public java.lang.String getGranularity() {
return granularity;
}
/**
* Immutable. The granularity (i.e. `MILLIS`) at which timestamps are stored in this table.
* Timestamps not matching the granularity will be rejected. If unspecified at creation time, the
* value will be set to `MILLIS`. Views: `SCHEMA_VIEW`, `FULL`.
* @param granularity granularity or {@code null} for none
*/
public Table setGranularity(java.lang.String granularity) {
this.granularity = granularity;
return this;
}
/**
* The unique name of the table. Values are of the form
* `projects/{project}/instances/{instance}/tables/_a-zA-Z0-9*`. Views: `NAME_ONLY`,
* `SCHEMA_VIEW`, `REPLICATION_VIEW`, `STATS_VIEW`, `FULL`
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The unique name of the table. Values are of the form
* `projects/{project}/instances/{instance}/tables/_a-zA-Z0-9*`. Views: `NAME_ONLY`,
* `SCHEMA_VIEW`, `REPLICATION_VIEW`, `STATS_VIEW`, `FULL`
* @param name name or {@code null} for none
*/
public Table setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. If this table was restored from another data source (e.g. a backup), this field
* will be populated with information about the restore.
* @return value or {@code null} for none
*/
public RestoreInfo getRestoreInfo() {
return restoreInfo;
}
/**
* Output only. If this table was restored from another data source (e.g. a backup), this field
* will be populated with information about the restore.
* @param restoreInfo restoreInfo or {@code null} for none
*/
public Table setRestoreInfo(RestoreInfo restoreInfo) {
this.restoreInfo = restoreInfo;
return this;
}
/**
* Output only. Only available with STATS_VIEW, this includes summary statistics about the entire
* table contents. For statistics about a specific column family, see ColumnFamilyStats in the
* mapped ColumnFamily collection above.
* @return value or {@code null} for none
*/
public TableStats getStats() {
return stats;
}
/**
* Output only. Only available with STATS_VIEW, this includes summary statistics about the entire
* table contents. For statistics about a specific column family, see ColumnFamilyStats in the
* mapped ColumnFamily collection above.
* @param stats stats or {@code null} for none
*/
public Table setStats(TableStats stats) {
this.stats = stats;
return this;
}
@Override
public Table set(String fieldName, Object value) {
return (Table) super.set(fieldName, value);
}
@Override
public Table clone() {
return (Table) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy