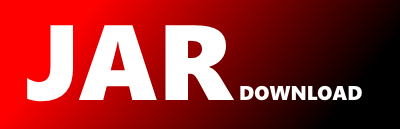
com.google.api.services.bigtableadmin.v2.model.CreateTableRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.bigtableadmin.v2.model;
/**
* Request message for google.bigtable.admin.v2.BigtableTableAdmin.CreateTable
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Bigtable Admin API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CreateTableRequest extends com.google.api.client.json.GenericJson {
/**
* The optional list of row keys that will be used to initially split the table into several
* tablets (tablets are similar to HBase regions). Given two split keys, `s1` and `s2`, three
* tablets will be created, spanning the key ranges: `[, s1), [s1, s2), [s2, )`. Example: * Row
* keys := `["a", "apple", "custom", "customer_1", "customer_2",` `"other", "zz"]` *
* initial_split_keys := `["apple", "customer_1", "customer_2", "other"]` * Key assignment: -
* Tablet 1 `[, apple) => {"a"}.` - Tablet 2 `[apple, customer_1) => {"apple", "custom"}.` -
* Tablet 3 `[customer_1, customer_2) => {"customer_1"}.` - Tablet 4 `[customer_2, other) =>
* {"customer_2"}.` - Tablet 5 `[other, ) => {"other", "zz"}.`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List initialSplits;
/**
* Required. The Table to create.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Table table;
/**
* Required. The name by which the new table should be referred to within the parent instance,
* e.g., `foobar` rather than `{parent}/tables/foobar`. Maximum 50 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String tableId;
/**
* The optional list of row keys that will be used to initially split the table into several
* tablets (tablets are similar to HBase regions). Given two split keys, `s1` and `s2`, three
* tablets will be created, spanning the key ranges: `[, s1), [s1, s2), [s2, )`. Example: * Row
* keys := `["a", "apple", "custom", "customer_1", "customer_2",` `"other", "zz"]` *
* initial_split_keys := `["apple", "customer_1", "customer_2", "other"]` * Key assignment: -
* Tablet 1 `[, apple) => {"a"}.` - Tablet 2 `[apple, customer_1) => {"apple", "custom"}.` -
* Tablet 3 `[customer_1, customer_2) => {"customer_1"}.` - Tablet 4 `[customer_2, other) =>
* {"customer_2"}.` - Tablet 5 `[other, ) => {"other", "zz"}.`
* @return value or {@code null} for none
*/
public java.util.List getInitialSplits() {
return initialSplits;
}
/**
* The optional list of row keys that will be used to initially split the table into several
* tablets (tablets are similar to HBase regions). Given two split keys, `s1` and `s2`, three
* tablets will be created, spanning the key ranges: `[, s1), [s1, s2), [s2, )`. Example: * Row
* keys := `["a", "apple", "custom", "customer_1", "customer_2",` `"other", "zz"]` *
* initial_split_keys := `["apple", "customer_1", "customer_2", "other"]` * Key assignment: -
* Tablet 1 `[, apple) => {"a"}.` - Tablet 2 `[apple, customer_1) => {"apple", "custom"}.` -
* Tablet 3 `[customer_1, customer_2) => {"customer_1"}.` - Tablet 4 `[customer_2, other) =>
* {"customer_2"}.` - Tablet 5 `[other, ) => {"other", "zz"}.`
* @param initialSplits initialSplits or {@code null} for none
*/
public CreateTableRequest setInitialSplits(java.util.List initialSplits) {
this.initialSplits = initialSplits;
return this;
}
/**
* Required. The Table to create.
* @return value or {@code null} for none
*/
public Table getTable() {
return table;
}
/**
* Required. The Table to create.
* @param table table or {@code null} for none
*/
public CreateTableRequest setTable(Table table) {
this.table = table;
return this;
}
/**
* Required. The name by which the new table should be referred to within the parent instance,
* e.g., `foobar` rather than `{parent}/tables/foobar`. Maximum 50 characters.
* @return value or {@code null} for none
*/
public java.lang.String getTableId() {
return tableId;
}
/**
* Required. The name by which the new table should be referred to within the parent instance,
* e.g., `foobar` rather than `{parent}/tables/foobar`. Maximum 50 characters.
* @param tableId tableId or {@code null} for none
*/
public CreateTableRequest setTableId(java.lang.String tableId) {
this.tableId = tableId;
return this;
}
@Override
public CreateTableRequest set(String fieldName, Object value) {
return (CreateTableRequest) super.set(fieldName, value);
}
@Override
public CreateTableRequest clone() {
return (CreateTableRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy