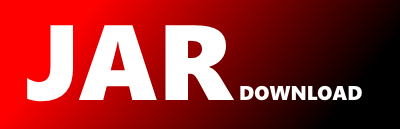
com.google.api.services.books.model.ConcurrentAccessRestriction Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-06-30 at 20:01:26 UTC
* Modify at your own risk.
*/
package com.google.api.services.books.model;
/**
* Model definition for ConcurrentAccessRestriction.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Books API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ConcurrentAccessRestriction extends com.google.api.client.json.GenericJson {
/**
* Whether access is granted for this (user, device, volume).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean deviceAllowed;
/**
* Resource type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The maximum number of concurrent access licenses for this volume.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxConcurrentDevices;
/**
* Error/warning message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String message;
/**
* Client nonce for verification. Download access and client-validation only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String nonce;
/**
* Error/warning reason code.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String reasonCode;
/**
* Whether this volume has any concurrent access restrictions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean restricted;
/**
* Response signature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String signature;
/**
* Client app identifier for verification. Download access and client-validation only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String source;
/**
* Time in seconds for license auto-expiration.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer timeWindowSeconds;
/**
* Identifies the volume for which this entry applies.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String volumeId;
/**
* Whether access is granted for this (user, device, volume).
* @return value or {@code null} for none
*/
public java.lang.Boolean getDeviceAllowed() {
return deviceAllowed;
}
/**
* Whether access is granted for this (user, device, volume).
* @param deviceAllowed deviceAllowed or {@code null} for none
*/
public ConcurrentAccessRestriction setDeviceAllowed(java.lang.Boolean deviceAllowed) {
this.deviceAllowed = deviceAllowed;
return this;
}
/**
* Resource type.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Resource type.
* @param kind kind or {@code null} for none
*/
public ConcurrentAccessRestriction setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The maximum number of concurrent access licenses for this volume.
* @return value or {@code null} for none
*/
public java.lang.Integer getMaxConcurrentDevices() {
return maxConcurrentDevices;
}
/**
* The maximum number of concurrent access licenses for this volume.
* @param maxConcurrentDevices maxConcurrentDevices or {@code null} for none
*/
public ConcurrentAccessRestriction setMaxConcurrentDevices(java.lang.Integer maxConcurrentDevices) {
this.maxConcurrentDevices = maxConcurrentDevices;
return this;
}
/**
* Error/warning message.
* @return value or {@code null} for none
*/
public java.lang.String getMessage() {
return message;
}
/**
* Error/warning message.
* @param message message or {@code null} for none
*/
public ConcurrentAccessRestriction setMessage(java.lang.String message) {
this.message = message;
return this;
}
/**
* Client nonce for verification. Download access and client-validation only.
* @return value or {@code null} for none
*/
public java.lang.String getNonce() {
return nonce;
}
/**
* Client nonce for verification. Download access and client-validation only.
* @param nonce nonce or {@code null} for none
*/
public ConcurrentAccessRestriction setNonce(java.lang.String nonce) {
this.nonce = nonce;
return this;
}
/**
* Error/warning reason code.
* @return value or {@code null} for none
*/
public java.lang.String getReasonCode() {
return reasonCode;
}
/**
* Error/warning reason code.
* @param reasonCode reasonCode or {@code null} for none
*/
public ConcurrentAccessRestriction setReasonCode(java.lang.String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* Whether this volume has any concurrent access restrictions.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRestricted() {
return restricted;
}
/**
* Whether this volume has any concurrent access restrictions.
* @param restricted restricted or {@code null} for none
*/
public ConcurrentAccessRestriction setRestricted(java.lang.Boolean restricted) {
this.restricted = restricted;
return this;
}
/**
* Response signature.
* @return value or {@code null} for none
*/
public java.lang.String getSignature() {
return signature;
}
/**
* Response signature.
* @param signature signature or {@code null} for none
*/
public ConcurrentAccessRestriction setSignature(java.lang.String signature) {
this.signature = signature;
return this;
}
/**
* Client app identifier for verification. Download access and client-validation only.
* @return value or {@code null} for none
*/
public java.lang.String getSource() {
return source;
}
/**
* Client app identifier for verification. Download access and client-validation only.
* @param source source or {@code null} for none
*/
public ConcurrentAccessRestriction setSource(java.lang.String source) {
this.source = source;
return this;
}
/**
* Time in seconds for license auto-expiration.
* @return value or {@code null} for none
*/
public java.lang.Integer getTimeWindowSeconds() {
return timeWindowSeconds;
}
/**
* Time in seconds for license auto-expiration.
* @param timeWindowSeconds timeWindowSeconds or {@code null} for none
*/
public ConcurrentAccessRestriction setTimeWindowSeconds(java.lang.Integer timeWindowSeconds) {
this.timeWindowSeconds = timeWindowSeconds;
return this;
}
/**
* Identifies the volume for which this entry applies.
* @return value or {@code null} for none
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/**
* Identifies the volume for which this entry applies.
* @param volumeId volumeId or {@code null} for none
*/
public ConcurrentAccessRestriction setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public ConcurrentAccessRestriction set(String fieldName, Object value) {
return (ConcurrentAccessRestriction) super.set(fieldName, value);
}
@Override
public ConcurrentAccessRestriction clone() {
return (ConcurrentAccessRestriction) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy