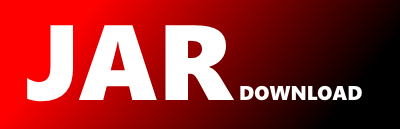
com.google.api.services.books.model.Volumeannotation Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-06-30 at 20:01:26 UTC
* Modify at your own risk.
*/
package com.google.api.services.books.model;
/**
* Model definition for Volumeannotation.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Books API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Volumeannotation extends com.google.api.client.json.GenericJson {
/**
* The annotation data id for this volume annotation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String annotationDataId;
/**
* Link to get data for this annotation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String annotationDataLink;
/**
* The type of annotation this is.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String annotationType;
/**
* The content ranges to identify the selected text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ContentRanges contentRanges;
/**
* Data for this annotation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String data;
/**
* Indicates that this annotation is deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean deleted;
/**
* Unique id of this volume annotation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* Resource Type
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The Layer this annotation is for.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String layerId;
/**
* Pages the annotation spans.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List pageIds;
/**
* Excerpt from the volume.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selectedText;
/**
* URL to this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Timestamp for the last time this anntoation was updated. (RFC 3339 UTC date-time format).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime updated;
/**
* The Volume this annotation is for.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String volumeId;
/**
* The annotation data id for this volume annotation.
* @return value or {@code null} for none
*/
public java.lang.String getAnnotationDataId() {
return annotationDataId;
}
/**
* The annotation data id for this volume annotation.
* @param annotationDataId annotationDataId or {@code null} for none
*/
public Volumeannotation setAnnotationDataId(java.lang.String annotationDataId) {
this.annotationDataId = annotationDataId;
return this;
}
/**
* Link to get data for this annotation.
* @return value or {@code null} for none
*/
public java.lang.String getAnnotationDataLink() {
return annotationDataLink;
}
/**
* Link to get data for this annotation.
* @param annotationDataLink annotationDataLink or {@code null} for none
*/
public Volumeannotation setAnnotationDataLink(java.lang.String annotationDataLink) {
this.annotationDataLink = annotationDataLink;
return this;
}
/**
* The type of annotation this is.
* @return value or {@code null} for none
*/
public java.lang.String getAnnotationType() {
return annotationType;
}
/**
* The type of annotation this is.
* @param annotationType annotationType or {@code null} for none
*/
public Volumeannotation setAnnotationType(java.lang.String annotationType) {
this.annotationType = annotationType;
return this;
}
/**
* The content ranges to identify the selected text.
* @return value or {@code null} for none
*/
public ContentRanges getContentRanges() {
return contentRanges;
}
/**
* The content ranges to identify the selected text.
* @param contentRanges contentRanges or {@code null} for none
*/
public Volumeannotation setContentRanges(ContentRanges contentRanges) {
this.contentRanges = contentRanges;
return this;
}
/**
* Data for this annotation.
* @return value or {@code null} for none
*/
public java.lang.String getData() {
return data;
}
/**
* Data for this annotation.
* @param data data or {@code null} for none
*/
public Volumeannotation setData(java.lang.String data) {
this.data = data;
return this;
}
/**
* Indicates that this annotation is deleted.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDeleted() {
return deleted;
}
/**
* Indicates that this annotation is deleted.
* @param deleted deleted or {@code null} for none
*/
public Volumeannotation setDeleted(java.lang.Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Unique id of this volume annotation.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* Unique id of this volume annotation.
* @param id id or {@code null} for none
*/
public Volumeannotation setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Resource Type
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Resource Type
* @param kind kind or {@code null} for none
*/
public Volumeannotation setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The Layer this annotation is for.
* @return value or {@code null} for none
*/
public java.lang.String getLayerId() {
return layerId;
}
/**
* The Layer this annotation is for.
* @param layerId layerId or {@code null} for none
*/
public Volumeannotation setLayerId(java.lang.String layerId) {
this.layerId = layerId;
return this;
}
/**
* Pages the annotation spans.
* @return value or {@code null} for none
*/
public java.util.List getPageIds() {
return pageIds;
}
/**
* Pages the annotation spans.
* @param pageIds pageIds or {@code null} for none
*/
public Volumeannotation setPageIds(java.util.List pageIds) {
this.pageIds = pageIds;
return this;
}
/**
* Excerpt from the volume.
* @return value or {@code null} for none
*/
public java.lang.String getSelectedText() {
return selectedText;
}
/**
* Excerpt from the volume.
* @param selectedText selectedText or {@code null} for none
*/
public Volumeannotation setSelectedText(java.lang.String selectedText) {
this.selectedText = selectedText;
return this;
}
/**
* URL to this resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* URL to this resource.
* @param selfLink selfLink or {@code null} for none
*/
public Volumeannotation setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* Timestamp for the last time this anntoation was updated. (RFC 3339 UTC date-time format).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getUpdated() {
return updated;
}
/**
* Timestamp for the last time this anntoation was updated. (RFC 3339 UTC date-time format).
* @param updated updated or {@code null} for none
*/
public Volumeannotation setUpdated(com.google.api.client.util.DateTime updated) {
this.updated = updated;
return this;
}
/**
* The Volume this annotation is for.
* @return value or {@code null} for none
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/**
* The Volume this annotation is for.
* @param volumeId volumeId or {@code null} for none
*/
public Volumeannotation setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public Volumeannotation set(String fieldName, Object value) {
return (Volumeannotation) super.set(fieldName, value);
}
@Override
public Volumeannotation clone() {
return (Volumeannotation) super.clone();
}
/**
* The content ranges to identify the selected text.
*/
public static final class ContentRanges extends com.google.api.client.json.GenericJson {
/**
* Range in CFI format for this annotation for version above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BooksAnnotationsRange cfiRange;
/**
* Content version applicable to ranges below.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/**
* Range in GB image format for this annotation for version above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BooksAnnotationsRange gbImageRange;
/**
* Range in GB text format for this annotation for version above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BooksAnnotationsRange gbTextRange;
/**
* Range in CFI format for this annotation for version above.
* @return value or {@code null} for none
*/
public BooksAnnotationsRange getCfiRange() {
return cfiRange;
}
/**
* Range in CFI format for this annotation for version above.
* @param cfiRange cfiRange or {@code null} for none
*/
public ContentRanges setCfiRange(BooksAnnotationsRange cfiRange) {
this.cfiRange = cfiRange;
return this;
}
/**
* Content version applicable to ranges below.
* @return value or {@code null} for none
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/**
* Content version applicable to ranges below.
* @param contentVersion contentVersion or {@code null} for none
*/
public ContentRanges setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/**
* Range in GB image format for this annotation for version above.
* @return value or {@code null} for none
*/
public BooksAnnotationsRange getGbImageRange() {
return gbImageRange;
}
/**
* Range in GB image format for this annotation for version above.
* @param gbImageRange gbImageRange or {@code null} for none
*/
public ContentRanges setGbImageRange(BooksAnnotationsRange gbImageRange) {
this.gbImageRange = gbImageRange;
return this;
}
/**
* Range in GB text format for this annotation for version above.
* @return value or {@code null} for none
*/
public BooksAnnotationsRange getGbTextRange() {
return gbTextRange;
}
/**
* Range in GB text format for this annotation for version above.
* @param gbTextRange gbTextRange or {@code null} for none
*/
public ContentRanges setGbTextRange(BooksAnnotationsRange gbTextRange) {
this.gbTextRange = gbTextRange;
return this;
}
@Override
public ContentRanges set(String fieldName, Object value) {
return (ContentRanges) super.set(fieldName, value);
}
@Override
public ContentRanges clone() {
return (ContentRanges) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy