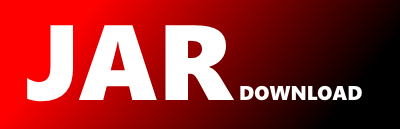
com.google.api.services.books.Books Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-11-07 19:12:12 UTC)
* on 2018-01-03 at 05:29:05 UTC
* Modify at your own risk.
*/
package com.google.api.services.books;
/**
* Service definition for Books (v1).
*
*
* Searches for books and manages your Google Books library.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link BooksRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Books extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.18.0-rc of the Books API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "books/v1/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Books(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Books(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Bookshelves collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Bookshelves.List request = books.bookshelves().list(parameters ...)}
*
*
* @return the resource collection
*/
public Bookshelves bookshelves() {
return new Bookshelves();
}
/**
* The "bookshelves" collection of methods.
*/
public class Bookshelves {
/**
* Retrieves metadata for a specific bookshelf for the specified user.
*
* Create a request for the method "bookshelves.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId ID of user for whom to retrieve bookshelves.
* @param shelf ID of bookshelf to retrieve.
* @return the request
*/
public Get get(java.lang.String userId, java.lang.String shelf) throws java.io.IOException {
Get result = new Get(userId, shelf);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "users/{userId}/bookshelves/{shelf}";
/**
* Retrieves metadata for a specific bookshelf for the specified user.
*
* Create a request for the method "bookshelves.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId ID of user for whom to retrieve bookshelves.
* @param shelf ID of bookshelf to retrieve.
* @since 1.13
*/
protected Get(java.lang.String userId, java.lang.String shelf) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Bookshelf.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** ID of user for whom to retrieve bookshelves. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of user for whom to retrieve bookshelves.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of user for whom to retrieve bookshelves. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** ID of bookshelf to retrieve. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** ID of bookshelf to retrieve.
*/
public java.lang.String getShelf() {
return shelf;
}
/** ID of bookshelf to retrieve. */
public Get setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a list of public bookshelves for the specified user.
*
* Create a request for the method "bookshelves.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId ID of user for whom to retrieve bookshelves.
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "users/{userId}/bookshelves";
/**
* Retrieves a list of public bookshelves for the specified user.
*
* Create a request for the method "bookshelves.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId ID of user for whom to retrieve bookshelves.
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Bookshelves.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** ID of user for whom to retrieve bookshelves. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of user for whom to retrieve bookshelves.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of user for whom to retrieve bookshelves. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Volumes collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Volumes.List request = books.volumes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Volumes volumes() {
return new Volumes();
}
/**
* The "volumes" collection of methods.
*/
public class Volumes {
/**
* Retrieves volumes in a specific bookshelf for the specified user.
*
* Create a request for the method "volumes.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId ID of user for whom to retrieve bookshelf volumes.
* @param shelf ID of bookshelf to retrieve volumes.
* @return the request
*/
public List list(java.lang.String userId, java.lang.String shelf) throws java.io.IOException {
List result = new List(userId, shelf);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "users/{userId}/bookshelves/{shelf}/volumes";
/**
* Retrieves volumes in a specific bookshelf for the specified user.
*
* Create a request for the method "volumes.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId ID of user for whom to retrieve bookshelf volumes.
* @param shelf ID of bookshelf to retrieve volumes.
* @since 1.13
*/
protected List(java.lang.String userId, java.lang.String shelf) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** ID of user for whom to retrieve bookshelf volumes. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of user for whom to retrieve bookshelf volumes.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of user for whom to retrieve bookshelf volumes. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** ID of bookshelf to retrieve volumes. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** ID of bookshelf to retrieve volumes.
*/
public java.lang.String getShelf() {
return shelf;
}
/** ID of bookshelf to retrieve volumes. */
public List setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** Maximum number of results to return */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return
[minimum: 0]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Set to true to show pre-ordered books. Defaults to false. */
@com.google.api.client.util.Key
private java.lang.Boolean showPreorders;
/** Set to true to show pre-ordered books. Defaults to false.
*/
public java.lang.Boolean getShowPreorders() {
return showPreorders;
}
/** Set to true to show pre-ordered books. Defaults to false. */
public List setShowPreorders(java.lang.Boolean showPreorders) {
this.showPreorders = showPreorders;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** Index of the first element to return (starts at 0) */
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/** Index of the first element to return (starts at 0)
[minimum: 0]
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
/** Index of the first element to return (starts at 0) */
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Cloudloading collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Cloudloading.List request = books.cloudloading().list(parameters ...)}
*
*
* @return the resource collection
*/
public Cloudloading cloudloading() {
return new Cloudloading();
}
/**
* The "cloudloading" collection of methods.
*/
public class Cloudloading {
/**
* Create a request for the method "cloudloading.addBook".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link AddBook#execute()} method to invoke the remote operation.
*
* @return the request
*/
public AddBook addBook() throws java.io.IOException {
AddBook result = new AddBook();
initialize(result);
return result;
}
public class AddBook extends BooksRequest {
private static final String REST_PATH = "cloudloading/addBook";
/**
* Create a request for the method "cloudloading.addBook".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link AddBook#execute()} method to invoke the remote operation.
* {@link
* AddBook#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected AddBook() {
super(Books.this, "POST", REST_PATH, null, com.google.api.services.books.model.BooksCloudloadingResource.class);
}
@Override
public AddBook setAlt(java.lang.String alt) {
return (AddBook) super.setAlt(alt);
}
@Override
public AddBook setFields(java.lang.String fields) {
return (AddBook) super.setFields(fields);
}
@Override
public AddBook setKey(java.lang.String key) {
return (AddBook) super.setKey(key);
}
@Override
public AddBook setOauthToken(java.lang.String oauthToken) {
return (AddBook) super.setOauthToken(oauthToken);
}
@Override
public AddBook setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddBook) super.setPrettyPrint(prettyPrint);
}
@Override
public AddBook setQuotaUser(java.lang.String quotaUser) {
return (AddBook) super.setQuotaUser(quotaUser);
}
@Override
public AddBook setUserIp(java.lang.String userIp) {
return (AddBook) super.setUserIp(userIp);
}
/** A drive document id. The upload_client_token must not be set. */
@com.google.api.client.util.Key("drive_document_id")
private java.lang.String driveDocumentId;
/** A drive document id. The upload_client_token must not be set.
*/
public java.lang.String getDriveDocumentId() {
return driveDocumentId;
}
/** A drive document id. The upload_client_token must not be set. */
public AddBook setDriveDocumentId(java.lang.String driveDocumentId) {
this.driveDocumentId = driveDocumentId;
return this;
}
/** The document MIME type. It can be set only if the drive_document_id is set. */
@com.google.api.client.util.Key("mime_type")
private java.lang.String mimeType;
/** The document MIME type. It can be set only if the drive_document_id is set.
*/
public java.lang.String getMimeType() {
return mimeType;
}
/** The document MIME type. It can be set only if the drive_document_id is set. */
public AddBook setMimeType(java.lang.String mimeType) {
this.mimeType = mimeType;
return this;
}
/** The document name. It can be set only if the drive_document_id is set. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The document name. It can be set only if the drive_document_id is set.
*/
public java.lang.String getName() {
return name;
}
/** The document name. It can be set only if the drive_document_id is set. */
public AddBook setName(java.lang.String name) {
this.name = name;
return this;
}
@com.google.api.client.util.Key("upload_client_token")
private java.lang.String uploadClientToken;
/**
*/
public java.lang.String getUploadClientToken() {
return uploadClientToken;
}
public AddBook setUploadClientToken(java.lang.String uploadClientToken) {
this.uploadClientToken = uploadClientToken;
return this;
}
@Override
public AddBook set(String parameterName, Object value) {
return (AddBook) super.set(parameterName, value);
}
}
/**
* Remove the book and its contents
*
* Create a request for the method "cloudloading.deleteBook".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link DeleteBook#execute()} method to invoke the remote operation.
*
* @param volumeId The id of the book to be removed.
* @return the request
*/
public DeleteBook deleteBook(java.lang.String volumeId) throws java.io.IOException {
DeleteBook result = new DeleteBook(volumeId);
initialize(result);
return result;
}
public class DeleteBook extends BooksRequest {
private static final String REST_PATH = "cloudloading/deleteBook";
/**
* Remove the book and its contents
*
* Create a request for the method "cloudloading.deleteBook".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link DeleteBook#execute()} method to invoke the remote operation.
* {@link
* DeleteBook#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId The id of the book to be removed.
* @since 1.13
*/
protected DeleteBook(java.lang.String volumeId) {
super(Books.this, "POST", REST_PATH, null, Void.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public DeleteBook setAlt(java.lang.String alt) {
return (DeleteBook) super.setAlt(alt);
}
@Override
public DeleteBook setFields(java.lang.String fields) {
return (DeleteBook) super.setFields(fields);
}
@Override
public DeleteBook setKey(java.lang.String key) {
return (DeleteBook) super.setKey(key);
}
@Override
public DeleteBook setOauthToken(java.lang.String oauthToken) {
return (DeleteBook) super.setOauthToken(oauthToken);
}
@Override
public DeleteBook setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DeleteBook) super.setPrettyPrint(prettyPrint);
}
@Override
public DeleteBook setQuotaUser(java.lang.String quotaUser) {
return (DeleteBook) super.setQuotaUser(quotaUser);
}
@Override
public DeleteBook setUserIp(java.lang.String userIp) {
return (DeleteBook) super.setUserIp(userIp);
}
/** The id of the book to be removed. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The id of the book to be removed.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The id of the book to be removed. */
public DeleteBook setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public DeleteBook set(String parameterName, Object value) {
return (DeleteBook) super.set(parameterName, value);
}
}
/**
* Create a request for the method "cloudloading.updateBook".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link UpdateBook#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.books.model.BooksCloudloadingResource}
* @return the request
*/
public UpdateBook updateBook(com.google.api.services.books.model.BooksCloudloadingResource content) throws java.io.IOException {
UpdateBook result = new UpdateBook(content);
initialize(result);
return result;
}
public class UpdateBook extends BooksRequest {
private static final String REST_PATH = "cloudloading/updateBook";
/**
* Create a request for the method "cloudloading.updateBook".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link UpdateBook#execute()} method to invoke the remote operation.
* {@link
* UpdateBook#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.books.model.BooksCloudloadingResource}
* @since 1.13
*/
protected UpdateBook(com.google.api.services.books.model.BooksCloudloadingResource content) {
super(Books.this, "POST", REST_PATH, content, com.google.api.services.books.model.BooksCloudloadingResource.class);
}
@Override
public UpdateBook setAlt(java.lang.String alt) {
return (UpdateBook) super.setAlt(alt);
}
@Override
public UpdateBook setFields(java.lang.String fields) {
return (UpdateBook) super.setFields(fields);
}
@Override
public UpdateBook setKey(java.lang.String key) {
return (UpdateBook) super.setKey(key);
}
@Override
public UpdateBook setOauthToken(java.lang.String oauthToken) {
return (UpdateBook) super.setOauthToken(oauthToken);
}
@Override
public UpdateBook setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateBook) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateBook setQuotaUser(java.lang.String quotaUser) {
return (UpdateBook) super.setQuotaUser(quotaUser);
}
@Override
public UpdateBook setUserIp(java.lang.String userIp) {
return (UpdateBook) super.setUserIp(userIp);
}
@Override
public UpdateBook set(String parameterName, Object value) {
return (UpdateBook) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Dictionary collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Dictionary.List request = books.dictionary().list(parameters ...)}
*
*
* @return the resource collection
*/
public Dictionary dictionary() {
return new Dictionary();
}
/**
* The "dictionary" collection of methods.
*/
public class Dictionary {
/**
* Returns a list of offline dictionary metadata available
*
* Create a request for the method "dictionary.listOfflineMetadata".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link ListOfflineMetadata#execute()} method to invoke the remote operation.
*
* @param cpksver The device/version ID from which to request the data.
* @return the request
*/
public ListOfflineMetadata listOfflineMetadata(java.lang.String cpksver) throws java.io.IOException {
ListOfflineMetadata result = new ListOfflineMetadata(cpksver);
initialize(result);
return result;
}
public class ListOfflineMetadata extends BooksRequest {
private static final String REST_PATH = "dictionary/listOfflineMetadata";
/**
* Returns a list of offline dictionary metadata available
*
* Create a request for the method "dictionary.listOfflineMetadata".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link ListOfflineMetadata#execute()} method to invoke the remote
* operation. {@link ListOfflineMetadata#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param cpksver The device/version ID from which to request the data.
* @since 1.13
*/
protected ListOfflineMetadata(java.lang.String cpksver) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Metadata.class);
this.cpksver = com.google.api.client.util.Preconditions.checkNotNull(cpksver, "Required parameter cpksver must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListOfflineMetadata setAlt(java.lang.String alt) {
return (ListOfflineMetadata) super.setAlt(alt);
}
@Override
public ListOfflineMetadata setFields(java.lang.String fields) {
return (ListOfflineMetadata) super.setFields(fields);
}
@Override
public ListOfflineMetadata setKey(java.lang.String key) {
return (ListOfflineMetadata) super.setKey(key);
}
@Override
public ListOfflineMetadata setOauthToken(java.lang.String oauthToken) {
return (ListOfflineMetadata) super.setOauthToken(oauthToken);
}
@Override
public ListOfflineMetadata setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListOfflineMetadata) super.setPrettyPrint(prettyPrint);
}
@Override
public ListOfflineMetadata setQuotaUser(java.lang.String quotaUser) {
return (ListOfflineMetadata) super.setQuotaUser(quotaUser);
}
@Override
public ListOfflineMetadata setUserIp(java.lang.String userIp) {
return (ListOfflineMetadata) super.setUserIp(userIp);
}
/** The device/version ID from which to request the data. */
@com.google.api.client.util.Key
private java.lang.String cpksver;
/** The device/version ID from which to request the data.
*/
public java.lang.String getCpksver() {
return cpksver;
}
/** The device/version ID from which to request the data. */
public ListOfflineMetadata setCpksver(java.lang.String cpksver) {
this.cpksver = cpksver;
return this;
}
@Override
public ListOfflineMetadata set(String parameterName, Object value) {
return (ListOfflineMetadata) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Familysharing collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Familysharing.List request = books.familysharing().list(parameters ...)}
*
*
* @return the resource collection
*/
public Familysharing familysharing() {
return new Familysharing();
}
/**
* The "familysharing" collection of methods.
*/
public class Familysharing {
/**
* Gets information regarding the family that the user is part of.
*
* Create a request for the method "familysharing.getFamilyInfo".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link GetFamilyInfo#execute()} method to invoke the remote operation.
*
* @return the request
*/
public GetFamilyInfo getFamilyInfo() throws java.io.IOException {
GetFamilyInfo result = new GetFamilyInfo();
initialize(result);
return result;
}
public class GetFamilyInfo extends BooksRequest {
private static final String REST_PATH = "familysharing/getFamilyInfo";
/**
* Gets information regarding the family that the user is part of.
*
* Create a request for the method "familysharing.getFamilyInfo".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link GetFamilyInfo#execute()} method to invoke the remote operation.
* {@link GetFamilyInfo#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @since 1.13
*/
protected GetFamilyInfo() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.FamilyInfo.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetFamilyInfo setAlt(java.lang.String alt) {
return (GetFamilyInfo) super.setAlt(alt);
}
@Override
public GetFamilyInfo setFields(java.lang.String fields) {
return (GetFamilyInfo) super.setFields(fields);
}
@Override
public GetFamilyInfo setKey(java.lang.String key) {
return (GetFamilyInfo) super.setKey(key);
}
@Override
public GetFamilyInfo setOauthToken(java.lang.String oauthToken) {
return (GetFamilyInfo) super.setOauthToken(oauthToken);
}
@Override
public GetFamilyInfo setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetFamilyInfo) super.setPrettyPrint(prettyPrint);
}
@Override
public GetFamilyInfo setQuotaUser(java.lang.String quotaUser) {
return (GetFamilyInfo) super.setQuotaUser(quotaUser);
}
@Override
public GetFamilyInfo setUserIp(java.lang.String userIp) {
return (GetFamilyInfo) super.setUserIp(userIp);
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public GetFamilyInfo setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public GetFamilyInfo set(String parameterName, Object value) {
return (GetFamilyInfo) super.set(parameterName, value);
}
}
/**
* Initiates sharing of the content with the user's family. Empty response indicates success.
*
* Create a request for the method "familysharing.share".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Share#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Share share() throws java.io.IOException {
Share result = new Share();
initialize(result);
return result;
}
public class Share extends BooksRequest {
private static final String REST_PATH = "familysharing/share";
/**
* Initiates sharing of the content with the user's family. Empty response indicates success.
*
* Create a request for the method "familysharing.share".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Share#execute()} method to invoke the remote operation. {@link
* Share#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Share() {
super(Books.this, "POST", REST_PATH, null, Void.class);
}
@Override
public Share setAlt(java.lang.String alt) {
return (Share) super.setAlt(alt);
}
@Override
public Share setFields(java.lang.String fields) {
return (Share) super.setFields(fields);
}
@Override
public Share setKey(java.lang.String key) {
return (Share) super.setKey(key);
}
@Override
public Share setOauthToken(java.lang.String oauthToken) {
return (Share) super.setOauthToken(oauthToken);
}
@Override
public Share setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Share) super.setPrettyPrint(prettyPrint);
}
@Override
public Share setQuotaUser(java.lang.String quotaUser) {
return (Share) super.setQuotaUser(quotaUser);
}
@Override
public Share setUserIp(java.lang.String userIp) {
return (Share) super.setUserIp(userIp);
}
/** The docid to share. */
@com.google.api.client.util.Key
private java.lang.String docId;
/** The docid to share.
*/
public java.lang.String getDocId() {
return docId;
}
/** The docid to share. */
public Share setDocId(java.lang.String docId) {
this.docId = docId;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Share setSource(java.lang.String source) {
this.source = source;
return this;
}
/** The volume to share. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to share.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to share. */
public Share setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public Share set(String parameterName, Object value) {
return (Share) super.set(parameterName, value);
}
}
/**
* Initiates revoking content that has already been shared with the user's family. Empty response
* indicates success.
*
* Create a request for the method "familysharing.unshare".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Unshare#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Unshare unshare() throws java.io.IOException {
Unshare result = new Unshare();
initialize(result);
return result;
}
public class Unshare extends BooksRequest {
private static final String REST_PATH = "familysharing/unshare";
/**
* Initiates revoking content that has already been shared with the user's family. Empty response
* indicates success.
*
* Create a request for the method "familysharing.unshare".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Unshare#execute()} method to invoke the remote operation.
* {@link
* Unshare#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Unshare() {
super(Books.this, "POST", REST_PATH, null, Void.class);
}
@Override
public Unshare setAlt(java.lang.String alt) {
return (Unshare) super.setAlt(alt);
}
@Override
public Unshare setFields(java.lang.String fields) {
return (Unshare) super.setFields(fields);
}
@Override
public Unshare setKey(java.lang.String key) {
return (Unshare) super.setKey(key);
}
@Override
public Unshare setOauthToken(java.lang.String oauthToken) {
return (Unshare) super.setOauthToken(oauthToken);
}
@Override
public Unshare setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unshare) super.setPrettyPrint(prettyPrint);
}
@Override
public Unshare setQuotaUser(java.lang.String quotaUser) {
return (Unshare) super.setQuotaUser(quotaUser);
}
@Override
public Unshare setUserIp(java.lang.String userIp) {
return (Unshare) super.setUserIp(userIp);
}
/** The docid to unshare. */
@com.google.api.client.util.Key
private java.lang.String docId;
/** The docid to unshare.
*/
public java.lang.String getDocId() {
return docId;
}
/** The docid to unshare. */
public Unshare setDocId(java.lang.String docId) {
this.docId = docId;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Unshare setSource(java.lang.String source) {
this.source = source;
return this;
}
/** The volume to unshare. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to unshare.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to unshare. */
public Unshare setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public Unshare set(String parameterName, Object value) {
return (Unshare) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Layers collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Layers.List request = books.layers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Layers layers() {
return new Layers();
}
/**
* The "layers" collection of methods.
*/
public class Layers {
/**
* Gets the layer summary for a volume.
*
* Create a request for the method "layers.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param volumeId The volume to retrieve layers for.
* @param summaryId The ID for the layer to get the summary for.
* @return the request
*/
public Get get(java.lang.String volumeId, java.lang.String summaryId) throws java.io.IOException {
Get result = new Get(volumeId, summaryId);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}/layersummary/{summaryId}";
/**
* Gets the layer summary for a volume.
*
* Create a request for the method "layers.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId The volume to retrieve layers for.
* @param summaryId The ID for the layer to get the summary for.
* @since 1.13
*/
protected Get(java.lang.String volumeId, java.lang.String summaryId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Layersummary.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.summaryId = com.google.api.client.util.Preconditions.checkNotNull(summaryId, "Required parameter summaryId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The volume to retrieve layers for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to retrieve layers for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to retrieve layers for. */
public Get setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The ID for the layer to get the summary for. */
@com.google.api.client.util.Key
private java.lang.String summaryId;
/** The ID for the layer to get the summary for.
*/
public java.lang.String getSummaryId() {
return summaryId;
}
/** The ID for the layer to get the summary for. */
public Get setSummaryId(java.lang.String summaryId) {
this.summaryId = summaryId;
return this;
}
/** The content version for the requested volume. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** The content version for the requested volume.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** The content version for the requested volume. */
public Get setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List the layer summaries for a volume.
*
* Create a request for the method "layers.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param volumeId The volume to retrieve layers for.
* @return the request
*/
public List list(java.lang.String volumeId) throws java.io.IOException {
List result = new List(volumeId);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}/layersummary";
/**
* List the layer summaries for a volume.
*
* Create a request for the method "layers.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId The volume to retrieve layers for.
* @since 1.13
*/
protected List(java.lang.String volumeId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Layersummaries.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The volume to retrieve layers for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to retrieve layers for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to retrieve layers for. */
public List setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The content version for the requested volume. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** The content version for the requested volume.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** The content version for the requested volume. */
public List setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** Maximum number of results to return */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return
[minimum: 0] [maximum: 200]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The value of the nextToken from the previous page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The value of the nextToken from the previous page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the AnnotationData collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.AnnotationData.List request = books.annotationData().list(parameters ...)}
*
*
* @return the resource collection
*/
public AnnotationData annotationData() {
return new AnnotationData();
}
/**
* The "annotationData" collection of methods.
*/
public class AnnotationData {
/**
* Gets the annotation data.
*
* Create a request for the method "annotationData.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param volumeId The volume to retrieve annotations for.
* @param layerId The ID for the layer to get the annotations.
* @param annotationDataId The ID of the annotation data to retrieve.
* @param contentVersion The content version for the volume you are trying to retrieve.
* @return the request
*/
public Get get(java.lang.String volumeId, java.lang.String layerId, java.lang.String annotationDataId, java.lang.String contentVersion) throws java.io.IOException {
Get result = new Get(volumeId, layerId, annotationDataId, contentVersion);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}/layers/{layerId}/data/{annotationDataId}";
/**
* Gets the annotation data.
*
* Create a request for the method "annotationData.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId The volume to retrieve annotations for.
* @param layerId The ID for the layer to get the annotations.
* @param annotationDataId The ID of the annotation data to retrieve.
* @param contentVersion The content version for the volume you are trying to retrieve.
* @since 1.13
*/
protected Get(java.lang.String volumeId, java.lang.String layerId, java.lang.String annotationDataId, java.lang.String contentVersion) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Annotationdata.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.layerId = com.google.api.client.util.Preconditions.checkNotNull(layerId, "Required parameter layerId must be specified.");
this.annotationDataId = com.google.api.client.util.Preconditions.checkNotNull(annotationDataId, "Required parameter annotationDataId must be specified.");
this.contentVersion = com.google.api.client.util.Preconditions.checkNotNull(contentVersion, "Required parameter contentVersion must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The volume to retrieve annotations for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to retrieve annotations for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to retrieve annotations for. */
public Get setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The ID for the layer to get the annotations. */
@com.google.api.client.util.Key
private java.lang.String layerId;
/** The ID for the layer to get the annotations.
*/
public java.lang.String getLayerId() {
return layerId;
}
/** The ID for the layer to get the annotations. */
public Get setLayerId(java.lang.String layerId) {
this.layerId = layerId;
return this;
}
/** The ID of the annotation data to retrieve. */
@com.google.api.client.util.Key
private java.lang.String annotationDataId;
/** The ID of the annotation data to retrieve.
*/
public java.lang.String getAnnotationDataId() {
return annotationDataId;
}
/** The ID of the annotation data to retrieve. */
public Get setAnnotationDataId(java.lang.String annotationDataId) {
this.annotationDataId = annotationDataId;
return this;
}
/** The content version for the volume you are trying to retrieve. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** The content version for the volume you are trying to retrieve.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** The content version for the volume you are trying to retrieve. */
public Get setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** For the dictionary layer. Whether or not to allow web definitions. */
@com.google.api.client.util.Key
private java.lang.Boolean allowWebDefinitions;
/** For the dictionary layer. Whether or not to allow web definitions.
*/
public java.lang.Boolean getAllowWebDefinitions() {
return allowWebDefinitions;
}
/** For the dictionary layer. Whether or not to allow web definitions. */
public Get setAllowWebDefinitions(java.lang.Boolean allowWebDefinitions) {
this.allowWebDefinitions = allowWebDefinitions;
return this;
}
/**
* The requested pixel height for any images. If height is provided width must also be
* provided.
*/
@com.google.api.client.util.Key
private java.lang.Integer h;
/** The requested pixel height for any images. If height is provided width must also be provided.
*/
public java.lang.Integer getH() {
return h;
}
/**
* The requested pixel height for any images. If height is provided width must also be
* provided.
*/
public Get setH(java.lang.Integer h) {
this.h = h;
return this;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
public Get setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** The requested scale for the image. */
@com.google.api.client.util.Key
private java.lang.Integer scale;
/** The requested scale for the image.
[minimum: 0]
*/
public java.lang.Integer getScale() {
return scale;
}
/** The requested scale for the image. */
public Get setScale(java.lang.Integer scale) {
this.scale = scale;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
/**
* The requested pixel width for any images. If width is provided height must also be
* provided.
*/
@com.google.api.client.util.Key
private java.lang.Integer w;
/** The requested pixel width for any images. If width is provided height must also be provided.
*/
public java.lang.Integer getW() {
return w;
}
/**
* The requested pixel width for any images. If width is provided height must also be
* provided.
*/
public Get setW(java.lang.Integer w) {
this.w = w;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the annotation data for a volume and layer.
*
* Create a request for the method "annotationData.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param volumeId The volume to retrieve annotation data for.
* @param layerId The ID for the layer to get the annotation data.
* @param contentVersion The content version for the requested volume.
* @return the request
*/
public List list(java.lang.String volumeId, java.lang.String layerId, java.lang.String contentVersion) throws java.io.IOException {
List result = new List(volumeId, layerId, contentVersion);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}/layers/{layerId}/data";
/**
* Gets the annotation data for a volume and layer.
*
* Create a request for the method "annotationData.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId The volume to retrieve annotation data for.
* @param layerId The ID for the layer to get the annotation data.
* @param contentVersion The content version for the requested volume.
* @since 1.13
*/
protected List(java.lang.String volumeId, java.lang.String layerId, java.lang.String contentVersion) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Annotationsdata.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.layerId = com.google.api.client.util.Preconditions.checkNotNull(layerId, "Required parameter layerId must be specified.");
this.contentVersion = com.google.api.client.util.Preconditions.checkNotNull(contentVersion, "Required parameter contentVersion must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The volume to retrieve annotation data for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to retrieve annotation data for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to retrieve annotation data for. */
public List setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The ID for the layer to get the annotation data. */
@com.google.api.client.util.Key
private java.lang.String layerId;
/** The ID for the layer to get the annotation data.
*/
public java.lang.String getLayerId() {
return layerId;
}
/** The ID for the layer to get the annotation data. */
public List setLayerId(java.lang.String layerId) {
this.layerId = layerId;
return this;
}
/** The content version for the requested volume. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** The content version for the requested volume.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** The content version for the requested volume. */
public List setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** The list of Annotation Data Ids to retrieve. Pagination is ignored if this is set. */
@com.google.api.client.util.Key
private java.util.List annotationDataId;
/** The list of Annotation Data Ids to retrieve. Pagination is ignored if this is set.
*/
public java.util.List getAnnotationDataId() {
return annotationDataId;
}
/** The list of Annotation Data Ids to retrieve. Pagination is ignored if this is set. */
public List setAnnotationDataId(java.util.List annotationDataId) {
this.annotationDataId = annotationDataId;
return this;
}
/**
* The requested pixel height for any images. If height is provided width must also be
* provided.
*/
@com.google.api.client.util.Key
private java.lang.Integer h;
/** The requested pixel height for any images. If height is provided width must also be provided.
*/
public java.lang.Integer getH() {
return h;
}
/**
* The requested pixel height for any images. If height is provided width must also be
* provided.
*/
public List setH(java.lang.Integer h) {
this.h = h;
return this;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
public List setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** Maximum number of results to return */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return
[minimum: 0] [maximum: 200]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The value of the nextToken from the previous page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The value of the nextToken from the previous page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The requested scale for the image. */
@com.google.api.client.util.Key
private java.lang.Integer scale;
/** The requested scale for the image.
[minimum: 0]
*/
public java.lang.Integer getScale() {
return scale;
}
/** The requested scale for the image. */
public List setScale(java.lang.Integer scale) {
this.scale = scale;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive). */
@com.google.api.client.util.Key
private java.lang.String updatedMax;
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive).
*/
public java.lang.String getUpdatedMax() {
return updatedMax;
}
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive). */
public List setUpdatedMax(java.lang.String updatedMax) {
this.updatedMax = updatedMax;
return this;
}
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive). */
@com.google.api.client.util.Key
private java.lang.String updatedMin;
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive).
*/
public java.lang.String getUpdatedMin() {
return updatedMin;
}
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive). */
public List setUpdatedMin(java.lang.String updatedMin) {
this.updatedMin = updatedMin;
return this;
}
/**
* The requested pixel width for any images. If width is provided height must also be
* provided.
*/
@com.google.api.client.util.Key
private java.lang.Integer w;
/** The requested pixel width for any images. If width is provided height must also be provided.
*/
public java.lang.Integer getW() {
return w;
}
/**
* The requested pixel width for any images. If width is provided height must also be
* provided.
*/
public List setW(java.lang.Integer w) {
this.w = w;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the VolumeAnnotations collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.VolumeAnnotations.List request = books.volumeAnnotations().list(parameters ...)}
*
*
* @return the resource collection
*/
public VolumeAnnotations volumeAnnotations() {
return new VolumeAnnotations();
}
/**
* The "volumeAnnotations" collection of methods.
*/
public class VolumeAnnotations {
/**
* Gets the volume annotation.
*
* Create a request for the method "volumeAnnotations.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param volumeId The volume to retrieve annotations for.
* @param layerId The ID for the layer to get the annotations.
* @param annotationId The ID of the volume annotation to retrieve.
* @return the request
*/
public Get get(java.lang.String volumeId, java.lang.String layerId, java.lang.String annotationId) throws java.io.IOException {
Get result = new Get(volumeId, layerId, annotationId);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}/layers/{layerId}/annotations/{annotationId}";
/**
* Gets the volume annotation.
*
* Create a request for the method "volumeAnnotations.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId The volume to retrieve annotations for.
* @param layerId The ID for the layer to get the annotations.
* @param annotationId The ID of the volume annotation to retrieve.
* @since 1.13
*/
protected Get(java.lang.String volumeId, java.lang.String layerId, java.lang.String annotationId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumeannotation.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.layerId = com.google.api.client.util.Preconditions.checkNotNull(layerId, "Required parameter layerId must be specified.");
this.annotationId = com.google.api.client.util.Preconditions.checkNotNull(annotationId, "Required parameter annotationId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The volume to retrieve annotations for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to retrieve annotations for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to retrieve annotations for. */
public Get setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The ID for the layer to get the annotations. */
@com.google.api.client.util.Key
private java.lang.String layerId;
/** The ID for the layer to get the annotations.
*/
public java.lang.String getLayerId() {
return layerId;
}
/** The ID for the layer to get the annotations. */
public Get setLayerId(java.lang.String layerId) {
this.layerId = layerId;
return this;
}
/** The ID of the volume annotation to retrieve. */
@com.google.api.client.util.Key
private java.lang.String annotationId;
/** The ID of the volume annotation to retrieve.
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/** The ID of the volume annotation to retrieve. */
public Get setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
public Get setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the volume annotations for a volume and layer.
*
* Create a request for the method "volumeAnnotations.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param volumeId The volume to retrieve annotations for.
* @param layerId The ID for the layer to get the annotations.
* @param contentVersion The content version for the requested volume.
* @return the request
*/
public List list(java.lang.String volumeId, java.lang.String layerId, java.lang.String contentVersion) throws java.io.IOException {
List result = new List(volumeId, layerId, contentVersion);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}/layers/{layerId}";
/**
* Gets the volume annotations for a volume and layer.
*
* Create a request for the method "volumeAnnotations.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId The volume to retrieve annotations for.
* @param layerId The ID for the layer to get the annotations.
* @param contentVersion The content version for the requested volume.
* @since 1.13
*/
protected List(java.lang.String volumeId, java.lang.String layerId, java.lang.String contentVersion) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumeannotations.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.layerId = com.google.api.client.util.Preconditions.checkNotNull(layerId, "Required parameter layerId must be specified.");
this.contentVersion = com.google.api.client.util.Preconditions.checkNotNull(contentVersion, "Required parameter contentVersion must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The volume to retrieve annotations for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to retrieve annotations for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to retrieve annotations for. */
public List setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The ID for the layer to get the annotations. */
@com.google.api.client.util.Key
private java.lang.String layerId;
/** The ID for the layer to get the annotations.
*/
public java.lang.String getLayerId() {
return layerId;
}
/** The ID for the layer to get the annotations. */
public List setLayerId(java.lang.String layerId) {
this.layerId = layerId;
return this;
}
/** The content version for the requested volume. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** The content version for the requested volume.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** The content version for the requested volume. */
public List setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** The end offset to end retrieving data from. */
@com.google.api.client.util.Key
private java.lang.String endOffset;
/** The end offset to end retrieving data from.
*/
public java.lang.String getEndOffset() {
return endOffset;
}
/** The end offset to end retrieving data from. */
public List setEndOffset(java.lang.String endOffset) {
this.endOffset = endOffset;
return this;
}
/** The end position to end retrieving data from. */
@com.google.api.client.util.Key
private java.lang.String endPosition;
/** The end position to end retrieving data from.
*/
public java.lang.String getEndPosition() {
return endPosition;
}
/** The end position to end retrieving data from. */
public List setEndPosition(java.lang.String endPosition) {
this.endPosition = endPosition;
return this;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* The locale information for the data. ISO-639-1 language and ISO-3166-1 country code. Ex:
* 'en_US'.
*/
public List setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** Maximum number of results to return */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return
[minimum: 0] [maximum: 200]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The value of the nextToken from the previous page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The value of the nextToken from the previous page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Set to true to return deleted annotations. updatedMin must be in the request to use this.
* Defaults to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** Set to true to return deleted annotations. updatedMin must be in the request to use this. Defaults
to false.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/**
* Set to true to return deleted annotations. updatedMin must be in the request to use this.
* Defaults to false.
*/
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** The start offset to start retrieving data from. */
@com.google.api.client.util.Key
private java.lang.String startOffset;
/** The start offset to start retrieving data from.
*/
public java.lang.String getStartOffset() {
return startOffset;
}
/** The start offset to start retrieving data from. */
public List setStartOffset(java.lang.String startOffset) {
this.startOffset = startOffset;
return this;
}
/** The start position to start retrieving data from. */
@com.google.api.client.util.Key
private java.lang.String startPosition;
/** The start position to start retrieving data from.
*/
public java.lang.String getStartPosition() {
return startPosition;
}
/** The start position to start retrieving data from. */
public List setStartPosition(java.lang.String startPosition) {
this.startPosition = startPosition;
return this;
}
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive). */
@com.google.api.client.util.Key
private java.lang.String updatedMax;
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive).
*/
public java.lang.String getUpdatedMax() {
return updatedMax;
}
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive). */
public List setUpdatedMax(java.lang.String updatedMax) {
this.updatedMax = updatedMax;
return this;
}
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive). */
@com.google.api.client.util.Key
private java.lang.String updatedMin;
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive).
*/
public java.lang.String getUpdatedMin() {
return updatedMin;
}
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive). */
public List setUpdatedMin(java.lang.String updatedMin) {
this.updatedMin = updatedMin;
return this;
}
/** The version of the volume annotations that you are requesting. */
@com.google.api.client.util.Key
private java.lang.String volumeAnnotationsVersion;
/** The version of the volume annotations that you are requesting.
*/
public java.lang.String getVolumeAnnotationsVersion() {
return volumeAnnotationsVersion;
}
/** The version of the volume annotations that you are requesting. */
public List setVolumeAnnotationsVersion(java.lang.String volumeAnnotationsVersion) {
this.volumeAnnotationsVersion = volumeAnnotationsVersion;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Myconfig collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Myconfig.List request = books.myconfig().list(parameters ...)}
*
*
* @return the resource collection
*/
public Myconfig myconfig() {
return new Myconfig();
}
/**
* The "myconfig" collection of methods.
*/
public class Myconfig {
/**
* Gets the current settings for the user.
*
* Create a request for the method "myconfig.getUserSettings".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link GetUserSettings#execute()} method to invoke the remote operation.
*
* @return the request
*/
public GetUserSettings getUserSettings() throws java.io.IOException {
GetUserSettings result = new GetUserSettings();
initialize(result);
return result;
}
public class GetUserSettings extends BooksRequest {
private static final String REST_PATH = "myconfig/getUserSettings";
/**
* Gets the current settings for the user.
*
* Create a request for the method "myconfig.getUserSettings".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link GetUserSettings#execute()} method to invoke the remote operation.
* {@link GetUserSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @since 1.13
*/
protected GetUserSettings() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Usersettings.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetUserSettings setAlt(java.lang.String alt) {
return (GetUserSettings) super.setAlt(alt);
}
@Override
public GetUserSettings setFields(java.lang.String fields) {
return (GetUserSettings) super.setFields(fields);
}
@Override
public GetUserSettings setKey(java.lang.String key) {
return (GetUserSettings) super.setKey(key);
}
@Override
public GetUserSettings setOauthToken(java.lang.String oauthToken) {
return (GetUserSettings) super.setOauthToken(oauthToken);
}
@Override
public GetUserSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetUserSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetUserSettings setQuotaUser(java.lang.String quotaUser) {
return (GetUserSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetUserSettings setUserIp(java.lang.String userIp) {
return (GetUserSettings) super.setUserIp(userIp);
}
@Override
public GetUserSettings set(String parameterName, Object value) {
return (GetUserSettings) super.set(parameterName, value);
}
}
/**
* Release downloaded content access restriction.
*
* Create a request for the method "myconfig.releaseDownloadAccess".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link ReleaseDownloadAccess#execute()} method to invoke the remote
* operation.
*
* @param volumeIds The volume(s) to release restrictions for.
* @param cpksver The device/version ID from which to release the restriction.
* @return the request
*/
public ReleaseDownloadAccess releaseDownloadAccess(java.util.List volumeIds, java.lang.String cpksver) throws java.io.IOException {
ReleaseDownloadAccess result = new ReleaseDownloadAccess(volumeIds, cpksver);
initialize(result);
return result;
}
public class ReleaseDownloadAccess extends BooksRequest {
private static final String REST_PATH = "myconfig/releaseDownloadAccess";
/**
* Release downloaded content access restriction.
*
* Create a request for the method "myconfig.releaseDownloadAccess".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link ReleaseDownloadAccess#execute()} method to invoke the remote
* operation. {@link ReleaseDownloadAccess#initialize(com.google.api.client.googleapis.service
* s.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param volumeIds The volume(s) to release restrictions for.
* @param cpksver The device/version ID from which to release the restriction.
* @since 1.13
*/
protected ReleaseDownloadAccess(java.util.List volumeIds, java.lang.String cpksver) {
super(Books.this, "POST", REST_PATH, null, com.google.api.services.books.model.DownloadAccesses.class);
this.volumeIds = com.google.api.client.util.Preconditions.checkNotNull(volumeIds, "Required parameter volumeIds must be specified.");
this.cpksver = com.google.api.client.util.Preconditions.checkNotNull(cpksver, "Required parameter cpksver must be specified.");
}
@Override
public ReleaseDownloadAccess setAlt(java.lang.String alt) {
return (ReleaseDownloadAccess) super.setAlt(alt);
}
@Override
public ReleaseDownloadAccess setFields(java.lang.String fields) {
return (ReleaseDownloadAccess) super.setFields(fields);
}
@Override
public ReleaseDownloadAccess setKey(java.lang.String key) {
return (ReleaseDownloadAccess) super.setKey(key);
}
@Override
public ReleaseDownloadAccess setOauthToken(java.lang.String oauthToken) {
return (ReleaseDownloadAccess) super.setOauthToken(oauthToken);
}
@Override
public ReleaseDownloadAccess setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReleaseDownloadAccess) super.setPrettyPrint(prettyPrint);
}
@Override
public ReleaseDownloadAccess setQuotaUser(java.lang.String quotaUser) {
return (ReleaseDownloadAccess) super.setQuotaUser(quotaUser);
}
@Override
public ReleaseDownloadAccess setUserIp(java.lang.String userIp) {
return (ReleaseDownloadAccess) super.setUserIp(userIp);
}
/** The volume(s) to release restrictions for. */
@com.google.api.client.util.Key
private java.util.List volumeIds;
/** The volume(s) to release restrictions for.
*/
public java.util.List getVolumeIds() {
return volumeIds;
}
/** The volume(s) to release restrictions for. */
public ReleaseDownloadAccess setVolumeIds(java.util.List volumeIds) {
this.volumeIds = volumeIds;
return this;
}
/** The device/version ID from which to release the restriction. */
@com.google.api.client.util.Key
private java.lang.String cpksver;
/** The device/version ID from which to release the restriction.
*/
public java.lang.String getCpksver() {
return cpksver;
}
/** The device/version ID from which to release the restriction. */
public ReleaseDownloadAccess setCpksver(java.lang.String cpksver) {
this.cpksver = cpksver;
return this;
}
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US. */
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US.
*/
public java.lang.String getLocale() {
return locale;
}
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US. */
public ReleaseDownloadAccess setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public ReleaseDownloadAccess setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public ReleaseDownloadAccess set(String parameterName, Object value) {
return (ReleaseDownloadAccess) super.set(parameterName, value);
}
}
/**
* Request concurrent and download access restrictions.
*
* Create a request for the method "myconfig.requestAccess".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link RequestAccess#execute()} method to invoke the remote operation.
*
* @param source String to identify the originator of this request.
* @param volumeId The volume to request concurrent/download restrictions for.
* @param nonce The client nonce value.
* @param cpksver The device/version ID from which to request the restrictions.
* @return the request
*/
public RequestAccess requestAccess(java.lang.String source, java.lang.String volumeId, java.lang.String nonce, java.lang.String cpksver) throws java.io.IOException {
RequestAccess result = new RequestAccess(source, volumeId, nonce, cpksver);
initialize(result);
return result;
}
public class RequestAccess extends BooksRequest {
private static final String REST_PATH = "myconfig/requestAccess";
/**
* Request concurrent and download access restrictions.
*
* Create a request for the method "myconfig.requestAccess".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link RequestAccess#execute()} method to invoke the remote operation.
* {@link RequestAccess#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param source String to identify the originator of this request.
* @param volumeId The volume to request concurrent/download restrictions for.
* @param nonce The client nonce value.
* @param cpksver The device/version ID from which to request the restrictions.
* @since 1.13
*/
protected RequestAccess(java.lang.String source, java.lang.String volumeId, java.lang.String nonce, java.lang.String cpksver) {
super(Books.this, "POST", REST_PATH, null, com.google.api.services.books.model.RequestAccess.class);
this.source = com.google.api.client.util.Preconditions.checkNotNull(source, "Required parameter source must be specified.");
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.nonce = com.google.api.client.util.Preconditions.checkNotNull(nonce, "Required parameter nonce must be specified.");
this.cpksver = com.google.api.client.util.Preconditions.checkNotNull(cpksver, "Required parameter cpksver must be specified.");
}
@Override
public RequestAccess setAlt(java.lang.String alt) {
return (RequestAccess) super.setAlt(alt);
}
@Override
public RequestAccess setFields(java.lang.String fields) {
return (RequestAccess) super.setFields(fields);
}
@Override
public RequestAccess setKey(java.lang.String key) {
return (RequestAccess) super.setKey(key);
}
@Override
public RequestAccess setOauthToken(java.lang.String oauthToken) {
return (RequestAccess) super.setOauthToken(oauthToken);
}
@Override
public RequestAccess setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RequestAccess) super.setPrettyPrint(prettyPrint);
}
@Override
public RequestAccess setQuotaUser(java.lang.String quotaUser) {
return (RequestAccess) super.setQuotaUser(quotaUser);
}
@Override
public RequestAccess setUserIp(java.lang.String userIp) {
return (RequestAccess) super.setUserIp(userIp);
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public RequestAccess setSource(java.lang.String source) {
this.source = source;
return this;
}
/** The volume to request concurrent/download restrictions for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to request concurrent/download restrictions for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to request concurrent/download restrictions for. */
public RequestAccess setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The client nonce value. */
@com.google.api.client.util.Key
private java.lang.String nonce;
/** The client nonce value.
*/
public java.lang.String getNonce() {
return nonce;
}
/** The client nonce value. */
public RequestAccess setNonce(java.lang.String nonce) {
this.nonce = nonce;
return this;
}
/** The device/version ID from which to request the restrictions. */
@com.google.api.client.util.Key
private java.lang.String cpksver;
/** The device/version ID from which to request the restrictions.
*/
public java.lang.String getCpksver() {
return cpksver;
}
/** The device/version ID from which to request the restrictions. */
public RequestAccess setCpksver(java.lang.String cpksver) {
this.cpksver = cpksver;
return this;
}
/** The type of access license to request. If not specified, the default is BOTH. */
@com.google.api.client.util.Key
private java.lang.String licenseTypes;
/** The type of access license to request. If not specified, the default is BOTH.
*/
public java.lang.String getLicenseTypes() {
return licenseTypes;
}
/** The type of access license to request. If not specified, the default is BOTH. */
public RequestAccess setLicenseTypes(java.lang.String licenseTypes) {
this.licenseTypes = licenseTypes;
return this;
}
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US. */
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US.
*/
public java.lang.String getLocale() {
return locale;
}
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US. */
public RequestAccess setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
@Override
public RequestAccess set(String parameterName, Object value) {
return (RequestAccess) super.set(parameterName, value);
}
}
/**
* Request downloaded content access for specified volumes on the My eBooks shelf.
*
* Create a request for the method "myconfig.syncVolumeLicenses".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link SyncVolumeLicenses#execute()} method to invoke the remote operation.
*
* @param source String to identify the originator of this request.
* @param nonce The client nonce value.
* @param cpksver The device/version ID from which to release the restriction.
* @return the request
*/
public SyncVolumeLicenses syncVolumeLicenses(java.lang.String source, java.lang.String nonce, java.lang.String cpksver) throws java.io.IOException {
SyncVolumeLicenses result = new SyncVolumeLicenses(source, nonce, cpksver);
initialize(result);
return result;
}
public class SyncVolumeLicenses extends BooksRequest {
private static final String REST_PATH = "myconfig/syncVolumeLicenses";
/**
* Request downloaded content access for specified volumes on the My eBooks shelf.
*
* Create a request for the method "myconfig.syncVolumeLicenses".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link SyncVolumeLicenses#execute()} method to invoke the remote
* operation. {@link SyncVolumeLicenses#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param source String to identify the originator of this request.
* @param nonce The client nonce value.
* @param cpksver The device/version ID from which to release the restriction.
* @since 1.13
*/
protected SyncVolumeLicenses(java.lang.String source, java.lang.String nonce, java.lang.String cpksver) {
super(Books.this, "POST", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
this.source = com.google.api.client.util.Preconditions.checkNotNull(source, "Required parameter source must be specified.");
this.nonce = com.google.api.client.util.Preconditions.checkNotNull(nonce, "Required parameter nonce must be specified.");
this.cpksver = com.google.api.client.util.Preconditions.checkNotNull(cpksver, "Required parameter cpksver must be specified.");
}
@Override
public SyncVolumeLicenses setAlt(java.lang.String alt) {
return (SyncVolumeLicenses) super.setAlt(alt);
}
@Override
public SyncVolumeLicenses setFields(java.lang.String fields) {
return (SyncVolumeLicenses) super.setFields(fields);
}
@Override
public SyncVolumeLicenses setKey(java.lang.String key) {
return (SyncVolumeLicenses) super.setKey(key);
}
@Override
public SyncVolumeLicenses setOauthToken(java.lang.String oauthToken) {
return (SyncVolumeLicenses) super.setOauthToken(oauthToken);
}
@Override
public SyncVolumeLicenses setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SyncVolumeLicenses) super.setPrettyPrint(prettyPrint);
}
@Override
public SyncVolumeLicenses setQuotaUser(java.lang.String quotaUser) {
return (SyncVolumeLicenses) super.setQuotaUser(quotaUser);
}
@Override
public SyncVolumeLicenses setUserIp(java.lang.String userIp) {
return (SyncVolumeLicenses) super.setUserIp(userIp);
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public SyncVolumeLicenses setSource(java.lang.String source) {
this.source = source;
return this;
}
/** The client nonce value. */
@com.google.api.client.util.Key
private java.lang.String nonce;
/** The client nonce value.
*/
public java.lang.String getNonce() {
return nonce;
}
/** The client nonce value. */
public SyncVolumeLicenses setNonce(java.lang.String nonce) {
this.nonce = nonce;
return this;
}
/** The device/version ID from which to release the restriction. */
@com.google.api.client.util.Key
private java.lang.String cpksver;
/** The device/version ID from which to release the restriction.
*/
public java.lang.String getCpksver() {
return cpksver;
}
/** The device/version ID from which to release the restriction. */
public SyncVolumeLicenses setCpksver(java.lang.String cpksver) {
this.cpksver = cpksver;
return this;
}
/** List of features supported by the client, i.e., 'RENTALS' */
@com.google.api.client.util.Key
private java.util.List features;
/** List of features supported by the client, i.e., 'RENTALS'
*/
public java.util.List getFeatures() {
return features;
}
/** List of features supported by the client, i.e., 'RENTALS' */
public SyncVolumeLicenses setFeatures(java.util.List features) {
this.features = features;
return this;
}
/** Set to true to include non-comics series. Defaults to false. */
@com.google.api.client.util.Key
private java.lang.Boolean includeNonComicsSeries;
/** Set to true to include non-comics series. Defaults to false.
*/
public java.lang.Boolean getIncludeNonComicsSeries() {
return includeNonComicsSeries;
}
/** Set to true to include non-comics series. Defaults to false. */
public SyncVolumeLicenses setIncludeNonComicsSeries(java.lang.Boolean includeNonComicsSeries) {
this.includeNonComicsSeries = includeNonComicsSeries;
return this;
}
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US. */
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US.
*/
public java.lang.String getLocale() {
return locale;
}
/** ISO-639-1, ISO-3166-1 codes for message localization, i.e. en_US. */
public SyncVolumeLicenses setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** Set to true to show pre-ordered books. Defaults to false. */
@com.google.api.client.util.Key
private java.lang.Boolean showPreorders;
/** Set to true to show pre-ordered books. Defaults to false.
*/
public java.lang.Boolean getShowPreorders() {
return showPreorders;
}
/** Set to true to show pre-ordered books. Defaults to false. */
public SyncVolumeLicenses setShowPreorders(java.lang.Boolean showPreorders) {
this.showPreorders = showPreorders;
return this;
}
/** The volume(s) to request download restrictions for. */
@com.google.api.client.util.Key
private java.util.List volumeIds;
/** The volume(s) to request download restrictions for.
*/
public java.util.List getVolumeIds() {
return volumeIds;
}
/** The volume(s) to request download restrictions for. */
public SyncVolumeLicenses setVolumeIds(java.util.List volumeIds) {
this.volumeIds = volumeIds;
return this;
}
@Override
public SyncVolumeLicenses set(String parameterName, Object value) {
return (SyncVolumeLicenses) super.set(parameterName, value);
}
}
/**
* Sets the settings for the user. If a sub-object is specified, it will overwrite the existing sub-
* object stored in the server. Unspecified sub-objects will retain the existing value.
*
* Create a request for the method "myconfig.updateUserSettings".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link UpdateUserSettings#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.books.model.Usersettings}
* @return the request
*/
public UpdateUserSettings updateUserSettings(com.google.api.services.books.model.Usersettings content) throws java.io.IOException {
UpdateUserSettings result = new UpdateUserSettings(content);
initialize(result);
return result;
}
public class UpdateUserSettings extends BooksRequest {
private static final String REST_PATH = "myconfig/updateUserSettings";
/**
* Sets the settings for the user. If a sub-object is specified, it will overwrite the existing
* sub-object stored in the server. Unspecified sub-objects will retain the existing value.
*
* Create a request for the method "myconfig.updateUserSettings".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link UpdateUserSettings#execute()} method to invoke the remote
* operation. {@link UpdateUserSettings#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param content the {@link com.google.api.services.books.model.Usersettings}
* @since 1.13
*/
protected UpdateUserSettings(com.google.api.services.books.model.Usersettings content) {
super(Books.this, "POST", REST_PATH, content, com.google.api.services.books.model.Usersettings.class);
}
@Override
public UpdateUserSettings setAlt(java.lang.String alt) {
return (UpdateUserSettings) super.setAlt(alt);
}
@Override
public UpdateUserSettings setFields(java.lang.String fields) {
return (UpdateUserSettings) super.setFields(fields);
}
@Override
public UpdateUserSettings setKey(java.lang.String key) {
return (UpdateUserSettings) super.setKey(key);
}
@Override
public UpdateUserSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateUserSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateUserSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateUserSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateUserSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateUserSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateUserSettings setUserIp(java.lang.String userIp) {
return (UpdateUserSettings) super.setUserIp(userIp);
}
@Override
public UpdateUserSettings set(String parameterName, Object value) {
return (UpdateUserSettings) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Mylibrary collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Mylibrary.List request = books.mylibrary().list(parameters ...)}
*
*
* @return the resource collection
*/
public Mylibrary mylibrary() {
return new Mylibrary();
}
/**
* The "mylibrary" collection of methods.
*/
public class Mylibrary {
/**
* An accessor for creating requests from the Annotations collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Annotations.List request = books.annotations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Annotations annotations() {
return new Annotations();
}
/**
* The "annotations" collection of methods.
*/
public class Annotations {
/**
* Deletes an annotation.
*
* Create a request for the method "annotations.delete".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param annotationId The ID for the annotation to delete.
* @return the request
*/
public Delete delete(java.lang.String annotationId) throws java.io.IOException {
Delete result = new Delete(annotationId);
initialize(result);
return result;
}
public class Delete extends BooksRequest {
private static final String REST_PATH = "mylibrary/annotations/{annotationId}";
/**
* Deletes an annotation.
*
* Create a request for the method "annotations.delete".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationId The ID for the annotation to delete.
* @since 1.13
*/
protected Delete(java.lang.String annotationId) {
super(Books.this, "DELETE", REST_PATH, null, Void.class);
this.annotationId = com.google.api.client.util.Preconditions.checkNotNull(annotationId, "Required parameter annotationId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** The ID for the annotation to delete. */
@com.google.api.client.util.Key
private java.lang.String annotationId;
/** The ID for the annotation to delete.
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/** The ID for the annotation to delete. */
public Delete setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Delete setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Inserts a new annotation.
*
* Create a request for the method "annotations.insert".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.books.model.Annotation}
* @return the request
*/
public Insert insert(com.google.api.services.books.model.Annotation content) throws java.io.IOException {
Insert result = new Insert(content);
initialize(result);
return result;
}
public class Insert extends BooksRequest {
private static final String REST_PATH = "mylibrary/annotations";
/**
* Inserts a new annotation.
*
* Create a request for the method "annotations.insert".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.books.model.Annotation}
* @since 1.13
*/
protected Insert(com.google.api.services.books.model.Annotation content) {
super(Books.this, "POST", REST_PATH, content, com.google.api.services.books.model.Annotation.class);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID for the annotation to insert. */
@com.google.api.client.util.Key
private java.lang.String annotationId;
/** The ID for the annotation to insert.
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/** The ID for the annotation to insert. */
public Insert setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
/** ISO-3166-1 code to override the IP-based location. */
@com.google.api.client.util.Key
private java.lang.String country;
/** ISO-3166-1 code to override the IP-based location.
*/
public java.lang.String getCountry() {
return country;
}
/** ISO-3166-1 code to override the IP-based location. */
public Insert setCountry(java.lang.String country) {
this.country = country;
return this;
}
/** Requests that only the summary of the specified layer be provided in the response. */
@com.google.api.client.util.Key
private java.lang.Boolean showOnlySummaryInResponse;
/** Requests that only the summary of the specified layer be provided in the response.
*/
public java.lang.Boolean getShowOnlySummaryInResponse() {
return showOnlySummaryInResponse;
}
/** Requests that only the summary of the specified layer be provided in the response. */
public Insert setShowOnlySummaryInResponse(java.lang.Boolean showOnlySummaryInResponse) {
this.showOnlySummaryInResponse = showOnlySummaryInResponse;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Insert setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of annotations, possibly filtered.
*
* Create a request for the method "annotations.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "mylibrary/annotations";
/**
* Retrieves a list of annotations, possibly filtered.
*
* Create a request for the method "annotations.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Annotations.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The content version for the requested volume. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** The content version for the requested volume.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** The content version for the requested volume. */
public List setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** The layer ID to limit annotation by. */
@com.google.api.client.util.Key
private java.lang.String layerId;
/** The layer ID to limit annotation by.
*/
public java.lang.String getLayerId() {
return layerId;
}
/** The layer ID to limit annotation by. */
public List setLayerId(java.lang.String layerId) {
this.layerId = layerId;
return this;
}
/** The layer ID(s) to limit annotation by. */
@com.google.api.client.util.Key
private java.util.List layerIds;
/** The layer ID(s) to limit annotation by.
*/
public java.util.List getLayerIds() {
return layerIds;
}
/** The layer ID(s) to limit annotation by. */
public List setLayerIds(java.util.List layerIds) {
this.layerIds = layerIds;
return this;
}
/** Maximum number of results to return */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return
[minimum: 0] [maximum: 40]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The value of the nextToken from the previous page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The value of the nextToken from the previous page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Set to true to return deleted annotations. updatedMin must be in the request to use this.
* Defaults to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** Set to true to return deleted annotations. updatedMin must be in the request to use this. Defaults
to false.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/**
* Set to true to return deleted annotations. updatedMin must be in the request to use this.
* Defaults to false.
*/
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive). */
@com.google.api.client.util.Key
private java.lang.String updatedMax;
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive).
*/
public java.lang.String getUpdatedMax() {
return updatedMax;
}
/** RFC 3339 timestamp to restrict to items updated prior to this timestamp (exclusive). */
public List setUpdatedMax(java.lang.String updatedMax) {
this.updatedMax = updatedMax;
return this;
}
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive). */
@com.google.api.client.util.Key
private java.lang.String updatedMin;
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive).
*/
public java.lang.String getUpdatedMin() {
return updatedMin;
}
/** RFC 3339 timestamp to restrict to items updated since this timestamp (inclusive). */
public List setUpdatedMin(java.lang.String updatedMin) {
this.updatedMin = updatedMin;
return this;
}
/** The volume to restrict annotations to. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** The volume to restrict annotations to.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** The volume to restrict annotations to. */
public List setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Gets the summary of specified layers.
*
* Create a request for the method "annotations.summary".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Summary#execute()} method to invoke the remote operation.
*
* @param layerIds Array of layer IDs to get the summary for.
* @param volumeId Volume id to get the summary for.
* @return the request
*/
public Summary summary(java.util.List layerIds, java.lang.String volumeId) throws java.io.IOException {
Summary result = new Summary(layerIds, volumeId);
initialize(result);
return result;
}
public class Summary extends BooksRequest {
private static final String REST_PATH = "mylibrary/annotations/summary";
/**
* Gets the summary of specified layers.
*
* Create a request for the method "annotations.summary".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Summary#execute()} method to invoke the remote operation.
* {@link
* Summary#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param layerIds Array of layer IDs to get the summary for.
* @param volumeId Volume id to get the summary for.
* @since 1.13
*/
protected Summary(java.util.List layerIds, java.lang.String volumeId) {
super(Books.this, "POST", REST_PATH, null, com.google.api.services.books.model.AnnotationsSummary.class);
this.layerIds = com.google.api.client.util.Preconditions.checkNotNull(layerIds, "Required parameter layerIds must be specified.");
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public Summary setAlt(java.lang.String alt) {
return (Summary) super.setAlt(alt);
}
@Override
public Summary setFields(java.lang.String fields) {
return (Summary) super.setFields(fields);
}
@Override
public Summary setKey(java.lang.String key) {
return (Summary) super.setKey(key);
}
@Override
public Summary setOauthToken(java.lang.String oauthToken) {
return (Summary) super.setOauthToken(oauthToken);
}
@Override
public Summary setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Summary) super.setPrettyPrint(prettyPrint);
}
@Override
public Summary setQuotaUser(java.lang.String quotaUser) {
return (Summary) super.setQuotaUser(quotaUser);
}
@Override
public Summary setUserIp(java.lang.String userIp) {
return (Summary) super.setUserIp(userIp);
}
/** Array of layer IDs to get the summary for. */
@com.google.api.client.util.Key
private java.util.List layerIds;
/** Array of layer IDs to get the summary for.
*/
public java.util.List getLayerIds() {
return layerIds;
}
/** Array of layer IDs to get the summary for. */
public Summary setLayerIds(java.util.List layerIds) {
this.layerIds = layerIds;
return this;
}
/** Volume id to get the summary for. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** Volume id to get the summary for.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** Volume id to get the summary for. */
public Summary setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public Summary set(String parameterName, Object value) {
return (Summary) super.set(parameterName, value);
}
}
/**
* Updates an existing annotation.
*
* Create a request for the method "annotations.update".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param annotationId The ID for the annotation to update.
* @param content the {@link com.google.api.services.books.model.Annotation}
* @return the request
*/
public Update update(java.lang.String annotationId, com.google.api.services.books.model.Annotation content) throws java.io.IOException {
Update result = new Update(annotationId, content);
initialize(result);
return result;
}
public class Update extends BooksRequest {
private static final String REST_PATH = "mylibrary/annotations/{annotationId}";
/**
* Updates an existing annotation.
*
* Create a request for the method "annotations.update".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationId The ID for the annotation to update.
* @param content the {@link com.google.api.services.books.model.Annotation}
* @since 1.13
*/
protected Update(java.lang.String annotationId, com.google.api.services.books.model.Annotation content) {
super(Books.this, "PUT", REST_PATH, content, com.google.api.services.books.model.Annotation.class);
this.annotationId = com.google.api.client.util.Preconditions.checkNotNull(annotationId, "Required parameter annotationId must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/** The ID for the annotation to update. */
@com.google.api.client.util.Key
private java.lang.String annotationId;
/** The ID for the annotation to update.
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/** The ID for the annotation to update. */
public Update setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Update setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Bookshelves collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Bookshelves.List request = books.bookshelves().list(parameters ...)}
*
*
* @return the resource collection
*/
public Bookshelves bookshelves() {
return new Bookshelves();
}
/**
* The "bookshelves" collection of methods.
*/
public class Bookshelves {
/**
* Adds a volume to a bookshelf.
*
* Create a request for the method "bookshelves.addVolume".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link AddVolume#execute()} method to invoke the remote operation.
*
* @param shelf ID of bookshelf to which to add a volume.
* @param volumeId ID of volume to add.
* @return the request
*/
public AddVolume addVolume(java.lang.String shelf, java.lang.String volumeId) throws java.io.IOException {
AddVolume result = new AddVolume(shelf, volumeId);
initialize(result);
return result;
}
public class AddVolume extends BooksRequest {
private static final String REST_PATH = "mylibrary/bookshelves/{shelf}/addVolume";
/**
* Adds a volume to a bookshelf.
*
* Create a request for the method "bookshelves.addVolume".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link AddVolume#execute()} method to invoke the remote operation.
* {@link
* AddVolume#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param shelf ID of bookshelf to which to add a volume.
* @param volumeId ID of volume to add.
* @since 1.13
*/
protected AddVolume(java.lang.String shelf, java.lang.String volumeId) {
super(Books.this, "POST", REST_PATH, null, Void.class);
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public AddVolume setAlt(java.lang.String alt) {
return (AddVolume) super.setAlt(alt);
}
@Override
public AddVolume setFields(java.lang.String fields) {
return (AddVolume) super.setFields(fields);
}
@Override
public AddVolume setKey(java.lang.String key) {
return (AddVolume) super.setKey(key);
}
@Override
public AddVolume setOauthToken(java.lang.String oauthToken) {
return (AddVolume) super.setOauthToken(oauthToken);
}
@Override
public AddVolume setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddVolume) super.setPrettyPrint(prettyPrint);
}
@Override
public AddVolume setQuotaUser(java.lang.String quotaUser) {
return (AddVolume) super.setQuotaUser(quotaUser);
}
@Override
public AddVolume setUserIp(java.lang.String userIp) {
return (AddVolume) super.setUserIp(userIp);
}
/** ID of bookshelf to which to add a volume. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** ID of bookshelf to which to add a volume.
*/
public java.lang.String getShelf() {
return shelf;
}
/** ID of bookshelf to which to add a volume. */
public AddVolume setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** ID of volume to add. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of volume to add.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of volume to add. */
public AddVolume setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The reason for which the book is added to the library. */
@com.google.api.client.util.Key
private java.lang.String reason;
/** The reason for which the book is added to the library.
*/
public java.lang.String getReason() {
return reason;
}
/** The reason for which the book is added to the library. */
public AddVolume setReason(java.lang.String reason) {
this.reason = reason;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public AddVolume setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public AddVolume set(String parameterName, Object value) {
return (AddVolume) super.set(parameterName, value);
}
}
/**
* Clears all volumes from a bookshelf.
*
* Create a request for the method "bookshelves.clearVolumes".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link ClearVolumes#execute()} method to invoke the remote operation.
*
* @param shelf ID of bookshelf from which to remove a volume.
* @return the request
*/
public ClearVolumes clearVolumes(java.lang.String shelf) throws java.io.IOException {
ClearVolumes result = new ClearVolumes(shelf);
initialize(result);
return result;
}
public class ClearVolumes extends BooksRequest {
private static final String REST_PATH = "mylibrary/bookshelves/{shelf}/clearVolumes";
/**
* Clears all volumes from a bookshelf.
*
* Create a request for the method "bookshelves.clearVolumes".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link ClearVolumes#execute()} method to invoke the remote operation.
* {@link
* ClearVolumes#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param shelf ID of bookshelf from which to remove a volume.
* @since 1.13
*/
protected ClearVolumes(java.lang.String shelf) {
super(Books.this, "POST", REST_PATH, null, Void.class);
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
}
@Override
public ClearVolumes setAlt(java.lang.String alt) {
return (ClearVolumes) super.setAlt(alt);
}
@Override
public ClearVolumes setFields(java.lang.String fields) {
return (ClearVolumes) super.setFields(fields);
}
@Override
public ClearVolumes setKey(java.lang.String key) {
return (ClearVolumes) super.setKey(key);
}
@Override
public ClearVolumes setOauthToken(java.lang.String oauthToken) {
return (ClearVolumes) super.setOauthToken(oauthToken);
}
@Override
public ClearVolumes setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ClearVolumes) super.setPrettyPrint(prettyPrint);
}
@Override
public ClearVolumes setQuotaUser(java.lang.String quotaUser) {
return (ClearVolumes) super.setQuotaUser(quotaUser);
}
@Override
public ClearVolumes setUserIp(java.lang.String userIp) {
return (ClearVolumes) super.setUserIp(userIp);
}
/** ID of bookshelf from which to remove a volume. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** ID of bookshelf from which to remove a volume.
*/
public java.lang.String getShelf() {
return shelf;
}
/** ID of bookshelf from which to remove a volume. */
public ClearVolumes setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public ClearVolumes setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public ClearVolumes set(String parameterName, Object value) {
return (ClearVolumes) super.set(parameterName, value);
}
}
/**
* Retrieves metadata for a specific bookshelf belonging to the authenticated user.
*
* Create a request for the method "bookshelves.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param shelf ID of bookshelf to retrieve.
* @return the request
*/
public Get get(java.lang.String shelf) throws java.io.IOException {
Get result = new Get(shelf);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "mylibrary/bookshelves/{shelf}";
/**
* Retrieves metadata for a specific bookshelf belonging to the authenticated user.
*
* Create a request for the method "bookshelves.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param shelf ID of bookshelf to retrieve.
* @since 1.13
*/
protected Get(java.lang.String shelf) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Bookshelf.class);
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** ID of bookshelf to retrieve. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** ID of bookshelf to retrieve.
*/
public java.lang.String getShelf() {
return shelf;
}
/** ID of bookshelf to retrieve. */
public Get setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a list of bookshelves belonging to the authenticated user.
*
* Create a request for the method "bookshelves.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "mylibrary/bookshelves";
/**
* Retrieves a list of bookshelves belonging to the authenticated user.
*
* Create a request for the method "bookshelves.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Bookshelves.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Moves a volume within a bookshelf.
*
* Create a request for the method "bookshelves.moveVolume".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link MoveVolume#execute()} method to invoke the remote operation.
*
* @param shelf ID of bookshelf with the volume.
* @param volumeId ID of volume to move.
* @param volumePosition Position on shelf to move the item (0 puts the item before the current first item, 1 puts it between
* the first and the second and so on.)
* @return the request
*/
public MoveVolume moveVolume(java.lang.String shelf, java.lang.String volumeId, java.lang.Integer volumePosition) throws java.io.IOException {
MoveVolume result = new MoveVolume(shelf, volumeId, volumePosition);
initialize(result);
return result;
}
public class MoveVolume extends BooksRequest {
private static final String REST_PATH = "mylibrary/bookshelves/{shelf}/moveVolume";
/**
* Moves a volume within a bookshelf.
*
* Create a request for the method "bookshelves.moveVolume".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link MoveVolume#execute()} method to invoke the remote operation.
* {@link
* MoveVolume#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param shelf ID of bookshelf with the volume.
* @param volumeId ID of volume to move.
* @param volumePosition Position on shelf to move the item (0 puts the item before the current first item, 1 puts it between
* the first and the second and so on.)
* @since 1.13
*/
protected MoveVolume(java.lang.String shelf, java.lang.String volumeId, java.lang.Integer volumePosition) {
super(Books.this, "POST", REST_PATH, null, Void.class);
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.volumePosition = com.google.api.client.util.Preconditions.checkNotNull(volumePosition, "Required parameter volumePosition must be specified.");
}
@Override
public MoveVolume setAlt(java.lang.String alt) {
return (MoveVolume) super.setAlt(alt);
}
@Override
public MoveVolume setFields(java.lang.String fields) {
return (MoveVolume) super.setFields(fields);
}
@Override
public MoveVolume setKey(java.lang.String key) {
return (MoveVolume) super.setKey(key);
}
@Override
public MoveVolume setOauthToken(java.lang.String oauthToken) {
return (MoveVolume) super.setOauthToken(oauthToken);
}
@Override
public MoveVolume setPrettyPrint(java.lang.Boolean prettyPrint) {
return (MoveVolume) super.setPrettyPrint(prettyPrint);
}
@Override
public MoveVolume setQuotaUser(java.lang.String quotaUser) {
return (MoveVolume) super.setQuotaUser(quotaUser);
}
@Override
public MoveVolume setUserIp(java.lang.String userIp) {
return (MoveVolume) super.setUserIp(userIp);
}
/** ID of bookshelf with the volume. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** ID of bookshelf with the volume.
*/
public java.lang.String getShelf() {
return shelf;
}
/** ID of bookshelf with the volume. */
public MoveVolume setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** ID of volume to move. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of volume to move.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of volume to move. */
public MoveVolume setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/**
* Position on shelf to move the item (0 puts the item before the current first item, 1 puts
* it between the first and the second and so on.)
*/
@com.google.api.client.util.Key
private java.lang.Integer volumePosition;
/** Position on shelf to move the item (0 puts the item before the current first item, 1 puts it
between the first and the second and so on.)
*/
public java.lang.Integer getVolumePosition() {
return volumePosition;
}
/**
* Position on shelf to move the item (0 puts the item before the current first item, 1 puts
* it between the first and the second and so on.)
*/
public MoveVolume setVolumePosition(java.lang.Integer volumePosition) {
this.volumePosition = volumePosition;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public MoveVolume setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public MoveVolume set(String parameterName, Object value) {
return (MoveVolume) super.set(parameterName, value);
}
}
/**
* Removes a volume from a bookshelf.
*
* Create a request for the method "bookshelves.removeVolume".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link RemoveVolume#execute()} method to invoke the remote operation.
*
* @param shelf ID of bookshelf from which to remove a volume.
* @param volumeId ID of volume to remove.
* @return the request
*/
public RemoveVolume removeVolume(java.lang.String shelf, java.lang.String volumeId) throws java.io.IOException {
RemoveVolume result = new RemoveVolume(shelf, volumeId);
initialize(result);
return result;
}
public class RemoveVolume extends BooksRequest {
private static final String REST_PATH = "mylibrary/bookshelves/{shelf}/removeVolume";
/**
* Removes a volume from a bookshelf.
*
* Create a request for the method "bookshelves.removeVolume".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link RemoveVolume#execute()} method to invoke the remote operation.
* {@link
* RemoveVolume#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param shelf ID of bookshelf from which to remove a volume.
* @param volumeId ID of volume to remove.
* @since 1.13
*/
protected RemoveVolume(java.lang.String shelf, java.lang.String volumeId) {
super(Books.this, "POST", REST_PATH, null, Void.class);
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public RemoveVolume setAlt(java.lang.String alt) {
return (RemoveVolume) super.setAlt(alt);
}
@Override
public RemoveVolume setFields(java.lang.String fields) {
return (RemoveVolume) super.setFields(fields);
}
@Override
public RemoveVolume setKey(java.lang.String key) {
return (RemoveVolume) super.setKey(key);
}
@Override
public RemoveVolume setOauthToken(java.lang.String oauthToken) {
return (RemoveVolume) super.setOauthToken(oauthToken);
}
@Override
public RemoveVolume setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveVolume) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveVolume setQuotaUser(java.lang.String quotaUser) {
return (RemoveVolume) super.setQuotaUser(quotaUser);
}
@Override
public RemoveVolume setUserIp(java.lang.String userIp) {
return (RemoveVolume) super.setUserIp(userIp);
}
/** ID of bookshelf from which to remove a volume. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** ID of bookshelf from which to remove a volume.
*/
public java.lang.String getShelf() {
return shelf;
}
/** ID of bookshelf from which to remove a volume. */
public RemoveVolume setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** ID of volume to remove. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of volume to remove.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of volume to remove. */
public RemoveVolume setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** The reason for which the book is removed from the library. */
@com.google.api.client.util.Key
private java.lang.String reason;
/** The reason for which the book is removed from the library.
*/
public java.lang.String getReason() {
return reason;
}
/** The reason for which the book is removed from the library. */
public RemoveVolume setReason(java.lang.String reason) {
this.reason = reason;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public RemoveVolume setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public RemoveVolume set(String parameterName, Object value) {
return (RemoveVolume) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Volumes collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Volumes.List request = books.volumes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Volumes volumes() {
return new Volumes();
}
/**
* The "volumes" collection of methods.
*/
public class Volumes {
/**
* Gets volume information for volumes on a bookshelf.
*
* Create a request for the method "volumes.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param shelf The bookshelf ID or name retrieve volumes for.
* @return the request
*/
public List list(java.lang.String shelf) throws java.io.IOException {
List result = new List(shelf);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "mylibrary/bookshelves/{shelf}/volumes";
/**
* Gets volume information for volumes on a bookshelf.
*
* Create a request for the method "volumes.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param shelf The bookshelf ID or name retrieve volumes for.
* @since 1.13
*/
protected List(java.lang.String shelf) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
this.shelf = com.google.api.client.util.Preconditions.checkNotNull(shelf, "Required parameter shelf must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The bookshelf ID or name retrieve volumes for. */
@com.google.api.client.util.Key
private java.lang.String shelf;
/** The bookshelf ID or name retrieve volumes for.
*/
public java.lang.String getShelf() {
return shelf;
}
/** The bookshelf ID or name retrieve volumes for. */
public List setShelf(java.lang.String shelf) {
this.shelf = shelf;
return this;
}
/** ISO-3166-1 code to override the IP-based location. */
@com.google.api.client.util.Key
private java.lang.String country;
/** ISO-3166-1 code to override the IP-based location.
*/
public java.lang.String getCountry() {
return country;
}
/** ISO-3166-1 code to override the IP-based location. */
public List setCountry(java.lang.String country) {
this.country = country;
return this;
}
/** Maximum number of results to return */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return
[minimum: 0]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Restrict information returned to a set of selected fields. */
@com.google.api.client.util.Key
private java.lang.String projection;
/** Restrict information returned to a set of selected fields.
*/
public java.lang.String getProjection() {
return projection;
}
/** Restrict information returned to a set of selected fields. */
public List setProjection(java.lang.String projection) {
this.projection = projection;
return this;
}
/** Full-text search query string in this bookshelf. */
@com.google.api.client.util.Key
private java.lang.String q;
/** Full-text search query string in this bookshelf.
*/
public java.lang.String getQ() {
return q;
}
/** Full-text search query string in this bookshelf. */
public List setQ(java.lang.String q) {
this.q = q;
return this;
}
/** Set to true to show pre-ordered books. Defaults to false. */
@com.google.api.client.util.Key
private java.lang.Boolean showPreorders;
/** Set to true to show pre-ordered books. Defaults to false.
*/
public java.lang.Boolean getShowPreorders() {
return showPreorders;
}
/** Set to true to show pre-ordered books. Defaults to false. */
public List setShowPreorders(java.lang.Boolean showPreorders) {
this.showPreorders = showPreorders;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** Index of the first element to return (starts at 0) */
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/** Index of the first element to return (starts at 0)
[minimum: 0]
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
/** Index of the first element to return (starts at 0) */
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Readingpositions collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Readingpositions.List request = books.readingpositions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Readingpositions readingpositions() {
return new Readingpositions();
}
/**
* The "readingpositions" collection of methods.
*/
public class Readingpositions {
/**
* Retrieves my reading position information for a volume.
*
* Create a request for the method "readingpositions.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param volumeId ID of volume for which to retrieve a reading position.
* @return the request
*/
public Get get(java.lang.String volumeId) throws java.io.IOException {
Get result = new Get(volumeId);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "mylibrary/readingpositions/{volumeId}";
/**
* Retrieves my reading position information for a volume.
*
* Create a request for the method "readingpositions.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId ID of volume for which to retrieve a reading position.
* @since 1.13
*/
protected Get(java.lang.String volumeId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.ReadingPosition.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** ID of volume for which to retrieve a reading position. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of volume for which to retrieve a reading position.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of volume for which to retrieve a reading position. */
public Get setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** Volume content version for which this reading position is requested. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** Volume content version for which this reading position is requested.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** Volume content version for which this reading position is requested. */
public Get setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Sets my reading position information for a volume.
*
* Create a request for the method "readingpositions.setPosition".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link SetPosition#execute()} method to invoke the remote operation.
*
* @param volumeId ID of volume for which to update the reading position.
* @param timestamp RFC 3339 UTC format timestamp associated with this reading position.
* @param position Position string for the new volume reading position.
* @return the request
*/
public SetPosition setPosition(java.lang.String volumeId, java.lang.String timestamp, java.lang.String position) throws java.io.IOException {
SetPosition result = new SetPosition(volumeId, timestamp, position);
initialize(result);
return result;
}
public class SetPosition extends BooksRequest {
private static final String REST_PATH = "mylibrary/readingpositions/{volumeId}/setPosition";
/**
* Sets my reading position information for a volume.
*
* Create a request for the method "readingpositions.setPosition".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link SetPosition#execute()} method to invoke the remote operation.
* {@link
* SetPosition#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId ID of volume for which to update the reading position.
* @param timestamp RFC 3339 UTC format timestamp associated with this reading position.
* @param position Position string for the new volume reading position.
* @since 1.13
*/
protected SetPosition(java.lang.String volumeId, java.lang.String timestamp, java.lang.String position) {
super(Books.this, "POST", REST_PATH, null, Void.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
this.timestamp = com.google.api.client.util.Preconditions.checkNotNull(timestamp, "Required parameter timestamp must be specified.");
this.position = com.google.api.client.util.Preconditions.checkNotNull(position, "Required parameter position must be specified.");
}
@Override
public SetPosition setAlt(java.lang.String alt) {
return (SetPosition) super.setAlt(alt);
}
@Override
public SetPosition setFields(java.lang.String fields) {
return (SetPosition) super.setFields(fields);
}
@Override
public SetPosition setKey(java.lang.String key) {
return (SetPosition) super.setKey(key);
}
@Override
public SetPosition setOauthToken(java.lang.String oauthToken) {
return (SetPosition) super.setOauthToken(oauthToken);
}
@Override
public SetPosition setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetPosition) super.setPrettyPrint(prettyPrint);
}
@Override
public SetPosition setQuotaUser(java.lang.String quotaUser) {
return (SetPosition) super.setQuotaUser(quotaUser);
}
@Override
public SetPosition setUserIp(java.lang.String userIp) {
return (SetPosition) super.setUserIp(userIp);
}
/** ID of volume for which to update the reading position. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of volume for which to update the reading position.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of volume for which to update the reading position. */
public SetPosition setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** RFC 3339 UTC format timestamp associated with this reading position. */
@com.google.api.client.util.Key
private java.lang.String timestamp;
/** RFC 3339 UTC format timestamp associated with this reading position.
*/
public java.lang.String getTimestamp() {
return timestamp;
}
/** RFC 3339 UTC format timestamp associated with this reading position. */
public SetPosition setTimestamp(java.lang.String timestamp) {
this.timestamp = timestamp;
return this;
}
/** Position string for the new volume reading position. */
@com.google.api.client.util.Key
private java.lang.String position;
/** Position string for the new volume reading position.
*/
public java.lang.String getPosition() {
return position;
}
/** Position string for the new volume reading position. */
public SetPosition setPosition(java.lang.String position) {
this.position = position;
return this;
}
/** Action that caused this reading position to be set. */
@com.google.api.client.util.Key
private java.lang.String action;
/** Action that caused this reading position to be set.
*/
public java.lang.String getAction() {
return action;
}
/** Action that caused this reading position to be set. */
public SetPosition setAction(java.lang.String action) {
this.action = action;
return this;
}
/** Volume content version for which this reading position applies. */
@com.google.api.client.util.Key
private java.lang.String contentVersion;
/** Volume content version for which this reading position applies.
*/
public java.lang.String getContentVersion() {
return contentVersion;
}
/** Volume content version for which this reading position applies. */
public SetPosition setContentVersion(java.lang.String contentVersion) {
this.contentVersion = contentVersion;
return this;
}
/** Random persistent device cookie optional on set position. */
@com.google.api.client.util.Key
private java.lang.String deviceCookie;
/** Random persistent device cookie optional on set position.
*/
public java.lang.String getDeviceCookie() {
return deviceCookie;
}
/** Random persistent device cookie optional on set position. */
public SetPosition setDeviceCookie(java.lang.String deviceCookie) {
this.deviceCookie = deviceCookie;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public SetPosition setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public SetPosition set(String parameterName, Object value) {
return (SetPosition) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Notification collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Notification.List request = books.notification().list(parameters ...)}
*
*
* @return the resource collection
*/
public Notification notification() {
return new Notification();
}
/**
* The "notification" collection of methods.
*/
public class Notification {
/**
* Returns notification details for a given notification id.
*
* Create a request for the method "notification.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param notificationId String to identify the notification.
* @return the request
*/
public Get get(java.lang.String notificationId) throws java.io.IOException {
Get result = new Get(notificationId);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "notification/get";
/**
* Returns notification details for a given notification id.
*
* Create a request for the method "notification.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param notificationId String to identify the notification.
* @since 1.13
*/
protected Get(java.lang.String notificationId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Notification.class);
this.notificationId = com.google.api.client.util.Preconditions.checkNotNull(notificationId, "Required parameter notificationId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** String to identify the notification. */
@com.google.api.client.util.Key("notification_id")
private java.lang.String notificationId;
/** String to identify the notification.
*/
public java.lang.String getNotificationId() {
return notificationId;
}
/** String to identify the notification. */
public Get setNotificationId(java.lang.String notificationId) {
this.notificationId = notificationId;
return this;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* notification title and body.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating notification title
and body.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* notification title and body.
*/
public Get setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Onboarding collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Onboarding.List request = books.onboarding().list(parameters ...)}
*
*
* @return the resource collection
*/
public Onboarding onboarding() {
return new Onboarding();
}
/**
* The "onboarding" collection of methods.
*/
public class Onboarding {
/**
* List categories for onboarding experience.
*
* Create a request for the method "onboarding.listCategories".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link ListCategories#execute()} method to invoke the remote operation.
*
* @return the request
*/
public ListCategories listCategories() throws java.io.IOException {
ListCategories result = new ListCategories();
initialize(result);
return result;
}
public class ListCategories extends BooksRequest {
private static final String REST_PATH = "onboarding/listCategories";
/**
* List categories for onboarding experience.
*
* Create a request for the method "onboarding.listCategories".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link ListCategories#execute()} method to invoke the remote operation.
* {@link ListCategories#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @since 1.13
*/
protected ListCategories() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Category.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListCategories setAlt(java.lang.String alt) {
return (ListCategories) super.setAlt(alt);
}
@Override
public ListCategories setFields(java.lang.String fields) {
return (ListCategories) super.setFields(fields);
}
@Override
public ListCategories setKey(java.lang.String key) {
return (ListCategories) super.setKey(key);
}
@Override
public ListCategories setOauthToken(java.lang.String oauthToken) {
return (ListCategories) super.setOauthToken(oauthToken);
}
@Override
public ListCategories setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListCategories) super.setPrettyPrint(prettyPrint);
}
@Override
public ListCategories setQuotaUser(java.lang.String quotaUser) {
return (ListCategories) super.setQuotaUser(quotaUser);
}
@Override
public ListCategories setUserIp(java.lang.String userIp) {
return (ListCategories) super.setUserIp(userIp);
}
/** ISO-639-1 language and ISO-3166-1 country code. Default is en-US if unset. */
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Default is en-US if unset.
*/
public java.lang.String getLocale() {
return locale;
}
/** ISO-639-1 language and ISO-3166-1 country code. Default is en-US if unset. */
public ListCategories setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
@Override
public ListCategories set(String parameterName, Object value) {
return (ListCategories) super.set(parameterName, value);
}
}
/**
* List available volumes under categories for onboarding experience.
*
* Create a request for the method "onboarding.listCategoryVolumes".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link ListCategoryVolumes#execute()} method to invoke the remote operation.
*
* @return the request
*/
public ListCategoryVolumes listCategoryVolumes() throws java.io.IOException {
ListCategoryVolumes result = new ListCategoryVolumes();
initialize(result);
return result;
}
public class ListCategoryVolumes extends BooksRequest {
private static final String REST_PATH = "onboarding/listCategoryVolumes";
/**
* List available volumes under categories for onboarding experience.
*
* Create a request for the method "onboarding.listCategoryVolumes".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link ListCategoryVolumes#execute()} method to invoke the remote
* operation. {@link ListCategoryVolumes#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @since 1.13
*/
protected ListCategoryVolumes() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volume2.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListCategoryVolumes setAlt(java.lang.String alt) {
return (ListCategoryVolumes) super.setAlt(alt);
}
@Override
public ListCategoryVolumes setFields(java.lang.String fields) {
return (ListCategoryVolumes) super.setFields(fields);
}
@Override
public ListCategoryVolumes setKey(java.lang.String key) {
return (ListCategoryVolumes) super.setKey(key);
}
@Override
public ListCategoryVolumes setOauthToken(java.lang.String oauthToken) {
return (ListCategoryVolumes) super.setOauthToken(oauthToken);
}
@Override
public ListCategoryVolumes setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListCategoryVolumes) super.setPrettyPrint(prettyPrint);
}
@Override
public ListCategoryVolumes setQuotaUser(java.lang.String quotaUser) {
return (ListCategoryVolumes) super.setQuotaUser(quotaUser);
}
@Override
public ListCategoryVolumes setUserIp(java.lang.String userIp) {
return (ListCategoryVolumes) super.setUserIp(userIp);
}
/** List of category ids requested. */
@com.google.api.client.util.Key
private java.util.List categoryId;
/** List of category ids requested.
*/
public java.util.List getCategoryId() {
return categoryId;
}
/** List of category ids requested. */
public ListCategoryVolumes setCategoryId(java.util.List categoryId) {
this.categoryId = categoryId;
return this;
}
/** ISO-639-1 language and ISO-3166-1 country code. Default is en-US if unset. */
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Default is en-US if unset.
*/
public java.lang.String getLocale() {
return locale;
}
/** ISO-639-1 language and ISO-3166-1 country code. Default is en-US if unset. */
public ListCategoryVolumes setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/**
* The maximum allowed maturity rating of returned volumes. Books with a higher maturity
* rating are filtered out.
*/
@com.google.api.client.util.Key
private java.lang.String maxAllowedMaturityRating;
/** The maximum allowed maturity rating of returned volumes. Books with a higher maturity rating are
filtered out.
*/
public java.lang.String getMaxAllowedMaturityRating() {
return maxAllowedMaturityRating;
}
/**
* The maximum allowed maturity rating of returned volumes. Books with a higher maturity
* rating are filtered out.
*/
public ListCategoryVolumes setMaxAllowedMaturityRating(java.lang.String maxAllowedMaturityRating) {
this.maxAllowedMaturityRating = maxAllowedMaturityRating;
return this;
}
/** Number of maximum results per page to be included in the response. */
@com.google.api.client.util.Key
private java.lang.Long pageSize;
/** Number of maximum results per page to be included in the response.
*/
public java.lang.Long getPageSize() {
return pageSize;
}
/** Number of maximum results per page to be included in the response. */
public ListCategoryVolumes setPageSize(java.lang.Long pageSize) {
this.pageSize = pageSize;
return this;
}
/** The value of the nextToken from the previous page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The value of the nextToken from the previous page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous page. */
public ListCategoryVolumes setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListCategoryVolumes set(String parameterName, Object value) {
return (ListCategoryVolumes) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Personalizedstream collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Personalizedstream.List request = books.personalizedstream().list(parameters ...)}
*
*
* @return the resource collection
*/
public Personalizedstream personalizedstream() {
return new Personalizedstream();
}
/**
* The "personalizedstream" collection of methods.
*/
public class Personalizedstream {
/**
* Returns a stream of personalized book clusters
*
* Create a request for the method "personalizedstream.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Get get() throws java.io.IOException {
Get result = new Get();
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "personalizedstream/get";
/**
* Returns a stream of personalized book clusters
*
* Create a request for the method "personalizedstream.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Get() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Discoveryclusters.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating recommendations.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
public Get setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
@com.google.api.client.util.Key
private java.lang.String maxAllowedMaturityRating;
/** The maximum allowed maturity rating of returned recommendations. Books with a higher maturity
rating are filtered out.
*/
public java.lang.String getMaxAllowedMaturityRating() {
return maxAllowedMaturityRating;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
public Get setMaxAllowedMaturityRating(java.lang.String maxAllowedMaturityRating) {
this.maxAllowedMaturityRating = maxAllowedMaturityRating;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Promooffer collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Promooffer.List request = books.promooffer().list(parameters ...)}
*
*
* @return the resource collection
*/
public Promooffer promooffer() {
return new Promooffer();
}
/**
* The "promooffer" collection of methods.
*/
public class Promooffer {
/**
* Create a request for the method "promooffer.accept".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Accept#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Accept accept() throws java.io.IOException {
Accept result = new Accept();
initialize(result);
return result;
}
public class Accept extends BooksRequest {
private static final String REST_PATH = "promooffer/accept";
/**
* Create a request for the method "promooffer.accept".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Accept#execute()} method to invoke the remote operation. {@link
* Accept#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Accept() {
super(Books.this, "POST", REST_PATH, null, Void.class);
}
@Override
public Accept setAlt(java.lang.String alt) {
return (Accept) super.setAlt(alt);
}
@Override
public Accept setFields(java.lang.String fields) {
return (Accept) super.setFields(fields);
}
@Override
public Accept setKey(java.lang.String key) {
return (Accept) super.setKey(key);
}
@Override
public Accept setOauthToken(java.lang.String oauthToken) {
return (Accept) super.setOauthToken(oauthToken);
}
@Override
public Accept setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Accept) super.setPrettyPrint(prettyPrint);
}
@Override
public Accept setQuotaUser(java.lang.String quotaUser) {
return (Accept) super.setQuotaUser(quotaUser);
}
@Override
public Accept setUserIp(java.lang.String userIp) {
return (Accept) super.setUserIp(userIp);
}
/** device android_id */
@com.google.api.client.util.Key
private java.lang.String androidId;
/** device android_id
*/
public java.lang.String getAndroidId() {
return androidId;
}
/** device android_id */
public Accept setAndroidId(java.lang.String androidId) {
this.androidId = androidId;
return this;
}
/** device device */
@com.google.api.client.util.Key
private java.lang.String device;
/** device device
*/
public java.lang.String getDevice() {
return device;
}
/** device device */
public Accept setDevice(java.lang.String device) {
this.device = device;
return this;
}
/** device manufacturer */
@com.google.api.client.util.Key
private java.lang.String manufacturer;
/** device manufacturer
*/
public java.lang.String getManufacturer() {
return manufacturer;
}
/** device manufacturer */
public Accept setManufacturer(java.lang.String manufacturer) {
this.manufacturer = manufacturer;
return this;
}
/** device model */
@com.google.api.client.util.Key
private java.lang.String model;
/** device model
*/
public java.lang.String getModel() {
return model;
}
/** device model */
public Accept setModel(java.lang.String model) {
this.model = model;
return this;
}
@com.google.api.client.util.Key
private java.lang.String offerId;
/**
*/
public java.lang.String getOfferId() {
return offerId;
}
public Accept setOfferId(java.lang.String offerId) {
this.offerId = offerId;
return this;
}
/** device product */
@com.google.api.client.util.Key
private java.lang.String product;
/** device product
*/
public java.lang.String getProduct() {
return product;
}
/** device product */
public Accept setProduct(java.lang.String product) {
this.product = product;
return this;
}
/** device serial */
@com.google.api.client.util.Key
private java.lang.String serial;
/** device serial
*/
public java.lang.String getSerial() {
return serial;
}
/** device serial */
public Accept setSerial(java.lang.String serial) {
this.serial = serial;
return this;
}
/** Volume id to exercise the offer */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** Volume id to exercise the offer
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** Volume id to exercise the offer */
public Accept setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public Accept set(String parameterName, Object value) {
return (Accept) super.set(parameterName, value);
}
}
/**
* Create a request for the method "promooffer.dismiss".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Dismiss dismiss() throws java.io.IOException {
Dismiss result = new Dismiss();
initialize(result);
return result;
}
public class Dismiss extends BooksRequest {
private static final String REST_PATH = "promooffer/dismiss";
/**
* Create a request for the method "promooffer.dismiss".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
* {@link
* Dismiss#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Dismiss() {
super(Books.this, "POST", REST_PATH, null, Void.class);
}
@Override
public Dismiss setAlt(java.lang.String alt) {
return (Dismiss) super.setAlt(alt);
}
@Override
public Dismiss setFields(java.lang.String fields) {
return (Dismiss) super.setFields(fields);
}
@Override
public Dismiss setKey(java.lang.String key) {
return (Dismiss) super.setKey(key);
}
@Override
public Dismiss setOauthToken(java.lang.String oauthToken) {
return (Dismiss) super.setOauthToken(oauthToken);
}
@Override
public Dismiss setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Dismiss) super.setPrettyPrint(prettyPrint);
}
@Override
public Dismiss setQuotaUser(java.lang.String quotaUser) {
return (Dismiss) super.setQuotaUser(quotaUser);
}
@Override
public Dismiss setUserIp(java.lang.String userIp) {
return (Dismiss) super.setUserIp(userIp);
}
/** device android_id */
@com.google.api.client.util.Key
private java.lang.String androidId;
/** device android_id
*/
public java.lang.String getAndroidId() {
return androidId;
}
/** device android_id */
public Dismiss setAndroidId(java.lang.String androidId) {
this.androidId = androidId;
return this;
}
/** device device */
@com.google.api.client.util.Key
private java.lang.String device;
/** device device
*/
public java.lang.String getDevice() {
return device;
}
/** device device */
public Dismiss setDevice(java.lang.String device) {
this.device = device;
return this;
}
/** device manufacturer */
@com.google.api.client.util.Key
private java.lang.String manufacturer;
/** device manufacturer
*/
public java.lang.String getManufacturer() {
return manufacturer;
}
/** device manufacturer */
public Dismiss setManufacturer(java.lang.String manufacturer) {
this.manufacturer = manufacturer;
return this;
}
/** device model */
@com.google.api.client.util.Key
private java.lang.String model;
/** device model
*/
public java.lang.String getModel() {
return model;
}
/** device model */
public Dismiss setModel(java.lang.String model) {
this.model = model;
return this;
}
/** Offer to dimiss */
@com.google.api.client.util.Key
private java.lang.String offerId;
/** Offer to dimiss
*/
public java.lang.String getOfferId() {
return offerId;
}
/** Offer to dimiss */
public Dismiss setOfferId(java.lang.String offerId) {
this.offerId = offerId;
return this;
}
/** device product */
@com.google.api.client.util.Key
private java.lang.String product;
/** device product
*/
public java.lang.String getProduct() {
return product;
}
/** device product */
public Dismiss setProduct(java.lang.String product) {
this.product = product;
return this;
}
/** device serial */
@com.google.api.client.util.Key
private java.lang.String serial;
/** device serial
*/
public java.lang.String getSerial() {
return serial;
}
/** device serial */
public Dismiss setSerial(java.lang.String serial) {
this.serial = serial;
return this;
}
@Override
public Dismiss set(String parameterName, Object value) {
return (Dismiss) super.set(parameterName, value);
}
}
/**
* Returns a list of promo offers available to the user
*
* Create a request for the method "promooffer.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Get get() throws java.io.IOException {
Get result = new Get();
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "promooffer/get";
/**
* Returns a list of promo offers available to the user
*
* Create a request for the method "promooffer.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Get() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Offers.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** device android_id */
@com.google.api.client.util.Key
private java.lang.String androidId;
/** device android_id
*/
public java.lang.String getAndroidId() {
return androidId;
}
/** device android_id */
public Get setAndroidId(java.lang.String androidId) {
this.androidId = androidId;
return this;
}
/** device device */
@com.google.api.client.util.Key
private java.lang.String device;
/** device device
*/
public java.lang.String getDevice() {
return device;
}
/** device device */
public Get setDevice(java.lang.String device) {
this.device = device;
return this;
}
/** device manufacturer */
@com.google.api.client.util.Key
private java.lang.String manufacturer;
/** device manufacturer
*/
public java.lang.String getManufacturer() {
return manufacturer;
}
/** device manufacturer */
public Get setManufacturer(java.lang.String manufacturer) {
this.manufacturer = manufacturer;
return this;
}
/** device model */
@com.google.api.client.util.Key
private java.lang.String model;
/** device model
*/
public java.lang.String getModel() {
return model;
}
/** device model */
public Get setModel(java.lang.String model) {
this.model = model;
return this;
}
/** device product */
@com.google.api.client.util.Key
private java.lang.String product;
/** device product
*/
public java.lang.String getProduct() {
return product;
}
/** device product */
public Get setProduct(java.lang.String product) {
this.product = product;
return this;
}
/** device serial */
@com.google.api.client.util.Key
private java.lang.String serial;
/** device serial
*/
public java.lang.String getSerial() {
return serial;
}
/** device serial */
public Get setSerial(java.lang.String serial) {
this.serial = serial;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Series collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Series.List request = books.series().list(parameters ...)}
*
*
* @return the resource collection
*/
public Series series() {
return new Series();
}
/**
* The "series" collection of methods.
*/
public class Series {
/**
* Returns Series metadata for the given series ids.
*
* Create a request for the method "series.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param seriesId String that identifies the series
* @return the request
*/
public Get get(java.util.List seriesId) throws java.io.IOException {
Get result = new Get(seriesId);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "series/get";
/**
* Returns Series metadata for the given series ids.
*
* Create a request for the method "series.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param seriesId String that identifies the series
* @since 1.13
*/
protected Get(java.util.List seriesId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Series.class);
this.seriesId = com.google.api.client.util.Preconditions.checkNotNull(seriesId, "Required parameter seriesId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** String that identifies the series */
@com.google.api.client.util.Key("series_id")
private java.util.List seriesId;
/** String that identifies the series
*/
public java.util.List getSeriesId() {
return seriesId;
}
/** String that identifies the series */
public Get setSeriesId(java.util.List seriesId) {
this.seriesId = seriesId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Membership collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Membership.List request = books.membership().list(parameters ...)}
*
*
* @return the resource collection
*/
public Membership membership() {
return new Membership();
}
/**
* The "membership" collection of methods.
*/
public class Membership {
/**
* Returns Series membership data given the series id.
*
* Create a request for the method "membership.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param seriesId String that identifies the series
* @return the request
*/
public Get get(java.lang.String seriesId) throws java.io.IOException {
Get result = new Get(seriesId);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "series/membership/get";
/**
* Returns Series membership data given the series id.
*
* Create a request for the method "membership.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param seriesId String that identifies the series
* @since 1.13
*/
protected Get(java.lang.String seriesId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Seriesmembership.class);
this.seriesId = com.google.api.client.util.Preconditions.checkNotNull(seriesId, "Required parameter seriesId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** String that identifies the series */
@com.google.api.client.util.Key("series_id")
private java.lang.String seriesId;
/** String that identifies the series
*/
public java.lang.String getSeriesId() {
return seriesId;
}
/** String that identifies the series */
public Get setSeriesId(java.lang.String seriesId) {
this.seriesId = seriesId;
return this;
}
/** Number of maximum results per page to be included in the response. */
@com.google.api.client.util.Key("page_size")
private java.lang.Long pageSize;
/** Number of maximum results per page to be included in the response.
*/
public java.lang.Long getPageSize() {
return pageSize;
}
/** Number of maximum results per page to be included in the response. */
public Get setPageSize(java.lang.Long pageSize) {
this.pageSize = pageSize;
return this;
}
/** The value of the nextToken from the previous page. */
@com.google.api.client.util.Key("page_token")
private java.lang.String pageToken;
/** The value of the nextToken from the previous page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous page. */
public Get setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Volumes collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Volumes.List request = books.volumes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Volumes volumes() {
return new Volumes();
}
/**
* The "volumes" collection of methods.
*/
public class Volumes {
/**
* Gets volume information for a single volume.
*
* Create a request for the method "volumes.get".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param volumeId ID of volume to retrieve.
* @return the request
*/
public Get get(java.lang.String volumeId) throws java.io.IOException {
Get result = new Get(volumeId);
initialize(result);
return result;
}
public class Get extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}";
/**
* Gets volume information for a single volume.
*
* Create a request for the method "volumes.get".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId ID of volume to retrieve.
* @since 1.13
*/
protected Get(java.lang.String volumeId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volume.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** ID of volume to retrieve. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of volume to retrieve.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of volume to retrieve. */
public Get setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** ISO-3166-1 code to override the IP-based location. */
@com.google.api.client.util.Key
private java.lang.String country;
/** ISO-3166-1 code to override the IP-based location.
*/
public java.lang.String getCountry() {
return country;
}
/** ISO-3166-1 code to override the IP-based location. */
public Get setCountry(java.lang.String country) {
this.country = country;
return this;
}
/** Set to true to include non-comics series. Defaults to false. */
@com.google.api.client.util.Key
private java.lang.Boolean includeNonComicsSeries;
/** Set to true to include non-comics series. Defaults to false.
*/
public java.lang.Boolean getIncludeNonComicsSeries() {
return includeNonComicsSeries;
}
/** Set to true to include non-comics series. Defaults to false. */
public Get setIncludeNonComicsSeries(java.lang.Boolean includeNonComicsSeries) {
this.includeNonComicsSeries = includeNonComicsSeries;
return this;
}
/** Brand results for partner ID. */
@com.google.api.client.util.Key
private java.lang.String partner;
/** Brand results for partner ID.
*/
public java.lang.String getPartner() {
return partner;
}
/** Brand results for partner ID. */
public Get setPartner(java.lang.String partner) {
this.partner = partner;
return this;
}
/** Restrict information returned to a set of selected fields. */
@com.google.api.client.util.Key
private java.lang.String projection;
/** Restrict information returned to a set of selected fields.
*/
public java.lang.String getProjection() {
return projection;
}
/** Restrict information returned to a set of selected fields. */
public Get setProjection(java.lang.String projection) {
this.projection = projection;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Get setSource(java.lang.String source) {
this.source = source;
return this;
}
@com.google.api.client.util.Key("user_library_consistent_read")
private java.lang.Boolean userLibraryConsistentRead;
/**
*/
public java.lang.Boolean getUserLibraryConsistentRead() {
return userLibraryConsistentRead;
}
public Get setUserLibraryConsistentRead(java.lang.Boolean userLibraryConsistentRead) {
this.userLibraryConsistentRead = userLibraryConsistentRead;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Performs a book search.
*
* Create a request for the method "volumes.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param q Full-text search query string.
* @return the request
*/
public List list(java.lang.String q) throws java.io.IOException {
List result = new List(q);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes";
/**
* Performs a book search.
*
* Create a request for the method "volumes.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param q Full-text search query string.
* @since 1.13
*/
protected List(java.lang.String q) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
this.q = com.google.api.client.util.Preconditions.checkNotNull(q, "Required parameter q must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Full-text search query string. */
@com.google.api.client.util.Key
private java.lang.String q;
/** Full-text search query string.
*/
public java.lang.String getQ() {
return q;
}
/** Full-text search query string. */
public List setQ(java.lang.String q) {
this.q = q;
return this;
}
/** Restrict to volumes by download availability. */
@com.google.api.client.util.Key
private java.lang.String download;
/** Restrict to volumes by download availability.
*/
public java.lang.String getDownload() {
return download;
}
/** Restrict to volumes by download availability. */
public List setDownload(java.lang.String download) {
this.download = download;
return this;
}
/** Filter search results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Filter search results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Filter search results. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Restrict results to books with this language code. */
@com.google.api.client.util.Key
private java.lang.String langRestrict;
/** Restrict results to books with this language code.
*/
public java.lang.String getLangRestrict() {
return langRestrict;
}
/** Restrict results to books with this language code. */
public List setLangRestrict(java.lang.String langRestrict) {
this.langRestrict = langRestrict;
return this;
}
/** Restrict search to this user's library. */
@com.google.api.client.util.Key
private java.lang.String libraryRestrict;
/** Restrict search to this user's library.
*/
public java.lang.String getLibraryRestrict() {
return libraryRestrict;
}
/** Restrict search to this user's library. */
public List setLibraryRestrict(java.lang.String libraryRestrict) {
this.libraryRestrict = libraryRestrict;
return this;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
@com.google.api.client.util.Key
private java.lang.String maxAllowedMaturityRating;
/** The maximum allowed maturity rating of returned recommendations. Books with a higher maturity
rating are filtered out.
*/
public java.lang.String getMaxAllowedMaturityRating() {
return maxAllowedMaturityRating;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
public List setMaxAllowedMaturityRating(java.lang.String maxAllowedMaturityRating) {
this.maxAllowedMaturityRating = maxAllowedMaturityRating;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return.
[minimum: 0] [maximum: 40]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Sort search results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sort search results.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Sort search results. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** Restrict and brand results for partner ID. */
@com.google.api.client.util.Key
private java.lang.String partner;
/** Restrict and brand results for partner ID.
*/
public java.lang.String getPartner() {
return partner;
}
/** Restrict and brand results for partner ID. */
public List setPartner(java.lang.String partner) {
this.partner = partner;
return this;
}
/** Restrict to books or magazines. */
@com.google.api.client.util.Key
private java.lang.String printType;
/** Restrict to books or magazines.
*/
public java.lang.String getPrintType() {
return printType;
}
/** Restrict to books or magazines. */
public List setPrintType(java.lang.String printType) {
this.printType = printType;
return this;
}
/** Restrict information returned to a set of selected fields. */
@com.google.api.client.util.Key
private java.lang.String projection;
/** Restrict information returned to a set of selected fields.
*/
public java.lang.String getProjection() {
return projection;
}
/** Restrict information returned to a set of selected fields. */
public List setProjection(java.lang.String projection) {
this.projection = projection;
return this;
}
/** Set to true to show books available for preorder. Defaults to false. */
@com.google.api.client.util.Key
private java.lang.Boolean showPreorders;
/** Set to true to show books available for preorder. Defaults to false.
*/
public java.lang.Boolean getShowPreorders() {
return showPreorders;
}
/** Set to true to show books available for preorder. Defaults to false. */
public List setShowPreorders(java.lang.Boolean showPreorders) {
this.showPreorders = showPreorders;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** Index of the first result to return (starts at 0) */
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/** Index of the first result to return (starts at 0)
[minimum: 0]
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
/** Index of the first result to return (starts at 0) */
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Associated collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Associated.List request = books.associated().list(parameters ...)}
*
*
* @return the resource collection
*/
public Associated associated() {
return new Associated();
}
/**
* The "associated" collection of methods.
*/
public class Associated {
/**
* Return a list of associated books.
*
* Create a request for the method "associated.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param volumeId ID of the source volume.
* @return the request
*/
public List list(java.lang.String volumeId) throws java.io.IOException {
List result = new List(volumeId);
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes/{volumeId}/associated";
/**
* Return a list of associated books.
*
* Create a request for the method "associated.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param volumeId ID of the source volume.
* @since 1.13
*/
protected List(java.lang.String volumeId) {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** ID of the source volume. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of the source volume.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of the source volume. */
public List setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/** Association type. */
@com.google.api.client.util.Key
private java.lang.String association;
/** Association type.
*/
public java.lang.String getAssociation() {
return association;
}
/** Association type. */
public List setAssociation(java.lang.String association) {
this.association = association;
return this;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating recommendations.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
public List setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
@com.google.api.client.util.Key
private java.lang.String maxAllowedMaturityRating;
/** The maximum allowed maturity rating of returned recommendations. Books with a higher maturity
rating are filtered out.
*/
public java.lang.String getMaxAllowedMaturityRating() {
return maxAllowedMaturityRating;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
public List setMaxAllowedMaturityRating(java.lang.String maxAllowedMaturityRating) {
this.maxAllowedMaturityRating = maxAllowedMaturityRating;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Mybooks collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Mybooks.List request = books.mybooks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Mybooks mybooks() {
return new Mybooks();
}
/**
* The "mybooks" collection of methods.
*/
public class Mybooks {
/**
* Return a list of books in My Library.
*
* Create a request for the method "mybooks.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes/mybooks";
/**
* Return a list of books in My Library.
*
* Create a request for the method "mybooks.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** How the book was acquired */
@com.google.api.client.util.Key
private java.util.List acquireMethod;
/** How the book was acquired
*/
public java.util.List getAcquireMethod() {
return acquireMethod;
}
/** How the book was acquired */
public List setAcquireMethod(java.util.List acquireMethod) {
this.acquireMethod = acquireMethod;
return this;
}
/** ISO-3166-1 code to override the IP-based location. */
@com.google.api.client.util.Key
private java.lang.String country;
/** ISO-3166-1 code to override the IP-based location.
*/
public java.lang.String getCountry() {
return country;
}
/** ISO-3166-1 code to override the IP-based location. */
public List setCountry(java.lang.String country) {
this.country = country;
return this;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex:'en_US'. Used for generating
* recommendations.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Ex:'en_US'. Used for generating recommendations.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex:'en_US'. Used for generating
* recommendations.
*/
public List setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return.
[minimum: 0] [maximum: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The processing state of the user uploaded volumes to be returned. Applicable only if the
* UPLOADED is specified in the acquireMethod.
*/
@com.google.api.client.util.Key
private java.util.List processingState;
/** The processing state of the user uploaded volumes to be returned. Applicable only if the UPLOADED
is specified in the acquireMethod.
*/
public java.util.List getProcessingState() {
return processingState;
}
/**
* The processing state of the user uploaded volumes to be returned. Applicable only if the
* UPLOADED is specified in the acquireMethod.
*/
public List setProcessingState(java.util.List processingState) {
this.processingState = processingState;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** Index of the first result to return (starts at 0) */
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/** Index of the first result to return (starts at 0)
[minimum: 0]
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
/** Index of the first result to return (starts at 0) */
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Recommended collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Recommended.List request = books.recommended().list(parameters ...)}
*
*
* @return the resource collection
*/
public Recommended recommended() {
return new Recommended();
}
/**
* The "recommended" collection of methods.
*/
public class Recommended {
/**
* Return a list of recommended books for the current user.
*
* Create a request for the method "recommended.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes/recommended";
/**
* Return a list of recommended books for the current user.
*
* Create a request for the method "recommended.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating recommendations.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
public List setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
@com.google.api.client.util.Key
private java.lang.String maxAllowedMaturityRating;
/** The maximum allowed maturity rating of returned recommendations. Books with a higher maturity
rating are filtered out.
*/
public java.lang.String getMaxAllowedMaturityRating() {
return maxAllowedMaturityRating;
}
/**
* The maximum allowed maturity rating of returned recommendations. Books with a higher
* maturity rating are filtered out.
*/
public List setMaxAllowedMaturityRating(java.lang.String maxAllowedMaturityRating) {
this.maxAllowedMaturityRating = maxAllowedMaturityRating;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Rate a recommended book for the current user.
*
* Create a request for the method "recommended.rate".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link Rate#execute()} method to invoke the remote operation.
*
* @param rating Rating to be given to the volume.
* @param volumeId ID of the source volume.
* @return the request
*/
public Rate rate(java.lang.String rating, java.lang.String volumeId) throws java.io.IOException {
Rate result = new Rate(rating, volumeId);
initialize(result);
return result;
}
public class Rate extends BooksRequest {
private static final String REST_PATH = "volumes/recommended/rate";
/**
* Rate a recommended book for the current user.
*
* Create a request for the method "recommended.rate".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link Rate#execute()} method to invoke the remote operation. {@link
* Rate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param rating Rating to be given to the volume.
* @param volumeId ID of the source volume.
* @since 1.13
*/
protected Rate(java.lang.String rating, java.lang.String volumeId) {
super(Books.this, "POST", REST_PATH, null, com.google.api.services.books.model.BooksVolumesRecommendedRateResponse.class);
this.rating = com.google.api.client.util.Preconditions.checkNotNull(rating, "Required parameter rating must be specified.");
this.volumeId = com.google.api.client.util.Preconditions.checkNotNull(volumeId, "Required parameter volumeId must be specified.");
}
@Override
public Rate setAlt(java.lang.String alt) {
return (Rate) super.setAlt(alt);
}
@Override
public Rate setFields(java.lang.String fields) {
return (Rate) super.setFields(fields);
}
@Override
public Rate setKey(java.lang.String key) {
return (Rate) super.setKey(key);
}
@Override
public Rate setOauthToken(java.lang.String oauthToken) {
return (Rate) super.setOauthToken(oauthToken);
}
@Override
public Rate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rate) super.setPrettyPrint(prettyPrint);
}
@Override
public Rate setQuotaUser(java.lang.String quotaUser) {
return (Rate) super.setQuotaUser(quotaUser);
}
@Override
public Rate setUserIp(java.lang.String userIp) {
return (Rate) super.setUserIp(userIp);
}
/** Rating to be given to the volume. */
@com.google.api.client.util.Key
private java.lang.String rating;
/** Rating to be given to the volume.
*/
public java.lang.String getRating() {
return rating;
}
/** Rating to be given to the volume. */
public Rate setRating(java.lang.String rating) {
this.rating = rating;
return this;
}
/** ID of the source volume. */
@com.google.api.client.util.Key
private java.lang.String volumeId;
/** ID of the source volume.
*/
public java.lang.String getVolumeId() {
return volumeId;
}
/** ID of the source volume. */
public Rate setVolumeId(java.lang.String volumeId) {
this.volumeId = volumeId;
return this;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating recommendations.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
public Rate setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public Rate setSource(java.lang.String source) {
this.source = source;
return this;
}
@Override
public Rate set(String parameterName, Object value) {
return (Rate) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Useruploaded collection.
*
* The typical use is:
*
* {@code Books books = new Books(...);}
* {@code Books.Useruploaded.List request = books.useruploaded().list(parameters ...)}
*
*
* @return the resource collection
*/
public Useruploaded useruploaded() {
return new Useruploaded();
}
/**
* The "useruploaded" collection of methods.
*/
public class Useruploaded {
/**
* Return a list of books uploaded by the current user.
*
* Create a request for the method "useruploaded.list".
*
* This request holds the parameters needed by the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends BooksRequest {
private static final String REST_PATH = "volumes/useruploaded";
/**
* Return a list of books uploaded by the current user.
*
* Create a request for the method "useruploaded.list".
*
* This request holds the parameters needed by the the books server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Books.this, "GET", REST_PATH, null, com.google.api.services.books.model.Volumes.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/** ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating recommendations.
*/
public java.lang.String getLocale() {
return locale;
}
/**
* ISO-639-1 language and ISO-3166-1 country code. Ex: 'en_US'. Used for generating
* recommendations.
*/
public List setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return.
[minimum: 0] [maximum: 40]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The processing state of the user uploaded volumes to be returned. */
@com.google.api.client.util.Key
private java.util.List processingState;
/** The processing state of the user uploaded volumes to be returned.
*/
public java.util.List getProcessingState() {
return processingState;
}
/** The processing state of the user uploaded volumes to be returned. */
public List setProcessingState(java.util.List processingState) {
this.processingState = processingState;
return this;
}
/** String to identify the originator of this request. */
@com.google.api.client.util.Key
private java.lang.String source;
/** String to identify the originator of this request.
*/
public java.lang.String getSource() {
return source;
}
/** String to identify the originator of this request. */
public List setSource(java.lang.String source) {
this.source = source;
return this;
}
/** Index of the first result to return (starts at 0) */
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/** Index of the first result to return (starts at 0)
[minimum: 0]
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
/** Index of the first result to return (starts at 0) */
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
/**
* The ids of the volumes to be returned. If not specified all that match the
* processingState are returned.
*/
@com.google.api.client.util.Key
private java.util.List volumeId;
/** The ids of the volumes to be returned. If not specified all that match the processingState are
returned.
*/
public java.util.List getVolumeId() {
return volumeId;
}
/**
* The ids of the volumes to be returned. If not specified all that match the
* processingState are returned.
*/
public List setVolumeId(java.util.List volumeId) {
this.volumeId = volumeId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link Books}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Books}. */
@Override
public Books build() {
return new Books(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link BooksRequestInitializer}.
*
* @since 1.12
*/
public Builder setBooksRequestInitializer(
BooksRequestInitializer booksRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(booksRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}