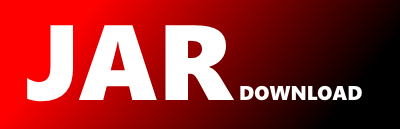
com.google.api.services.books.model.Geolayerdata Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-11-07 19:12:12 UTC)
* on 2018-01-03 at 05:29:05 UTC
* Modify at your own risk.
*/
package com.google.api.services.books.model;
/**
* Model definition for Geolayerdata.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Books API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Geolayerdata extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Common common;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Geo geo;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* @return value or {@code null} for none
*/
public Common getCommon() {
return common;
}
/**
* @param common common or {@code null} for none
*/
public Geolayerdata setCommon(Common common) {
this.common = common;
return this;
}
/**
* @return value or {@code null} for none
*/
public Geo getGeo() {
return geo;
}
/**
* @param geo geo or {@code null} for none
*/
public Geolayerdata setGeo(Geo geo) {
this.geo = geo;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* @param kind kind or {@code null} for none
*/
public Geolayerdata setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Geolayerdata set(String fieldName, Object value) {
return (Geolayerdata) super.set(fieldName, value);
}
@Override
public Geolayerdata clone() {
return (Geolayerdata) super.clone();
}
/**
* Model definition for GeolayerdataCommon.
*/
public static final class Common extends com.google.api.client.json.GenericJson {
/**
* The language of the information url and description.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lang;
/**
* The URL for the preview image information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String previewImageUrl;
/**
* The description for this location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String snippet;
/**
* The URL for information for this location. Ex: wikipedia link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String snippetUrl;
/**
* The display title and localized canonical name to use when searching for this entity on Google
* search.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The language of the information url and description.
* @return value or {@code null} for none
*/
public java.lang.String getLang() {
return lang;
}
/**
* The language of the information url and description.
* @param lang lang or {@code null} for none
*/
public Common setLang(java.lang.String lang) {
this.lang = lang;
return this;
}
/**
* The URL for the preview image information.
* @return value or {@code null} for none
*/
public java.lang.String getPreviewImageUrl() {
return previewImageUrl;
}
/**
* The URL for the preview image information.
* @param previewImageUrl previewImageUrl or {@code null} for none
*/
public Common setPreviewImageUrl(java.lang.String previewImageUrl) {
this.previewImageUrl = previewImageUrl;
return this;
}
/**
* The description for this location.
* @return value or {@code null} for none
*/
public java.lang.String getSnippet() {
return snippet;
}
/**
* The description for this location.
* @param snippet snippet or {@code null} for none
*/
public Common setSnippet(java.lang.String snippet) {
this.snippet = snippet;
return this;
}
/**
* The URL for information for this location. Ex: wikipedia link.
* @return value or {@code null} for none
*/
public java.lang.String getSnippetUrl() {
return snippetUrl;
}
/**
* The URL for information for this location. Ex: wikipedia link.
* @param snippetUrl snippetUrl or {@code null} for none
*/
public Common setSnippetUrl(java.lang.String snippetUrl) {
this.snippetUrl = snippetUrl;
return this;
}
/**
* The display title and localized canonical name to use when searching for this entity on Google
* search.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* The display title and localized canonical name to use when searching for this entity on Google
* search.
* @param title title or {@code null} for none
*/
public Common setTitle(java.lang.String title) {
this.title = title;
return this;
}
@Override
public Common set(String fieldName, Object value) {
return (Common) super.set(fieldName, value);
}
@Override
public Common clone() {
return (Common) super.clone();
}
}
/**
* Model definition for GeolayerdataGeo.
*/
public static final class Geo extends com.google.api.client.json.GenericJson {
/**
* The boundary of the location as a set of loops containing pairs of latitude, longitude
* coordinates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List> boundary;
/**
* The cache policy active for this data. EX: UNRESTRICTED, RESTRICTED, NEVER
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cachePolicy;
/**
* The country code of the location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String countryCode;
/**
* The latitude of the location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double latitude;
/**
* The longitude of the location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double longitude;
/**
* The type of map that should be used for this location. EX: HYBRID, ROADMAP, SATELLITE, TERRAIN
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mapType;
/**
* The viewport for showing this location. This is a latitude, longitude rectangle.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Viewport viewport;
/**
* The Zoom level to use for the map. Zoom levels between 0 (the lowest zoom level, in which the
* entire world can be seen on one map) to 21+ (down to individual buildings). See:
* https://developers.google.com/maps/documentation/staticmaps/#Zoomlevels
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer zoom;
/**
* The boundary of the location as a set of loops containing pairs of latitude, longitude
* coordinates.
* @return value or {@code null} for none
*/
public java.util.List> getBoundary() {
return boundary;
}
/**
* The boundary of the location as a set of loops containing pairs of latitude, longitude
* coordinates.
* @param boundary boundary or {@code null} for none
*/
public Geo setBoundary(java.util.List> boundary) {
this.boundary = boundary;
return this;
}
/**
* The cache policy active for this data. EX: UNRESTRICTED, RESTRICTED, NEVER
* @return value or {@code null} for none
*/
public java.lang.String getCachePolicy() {
return cachePolicy;
}
/**
* The cache policy active for this data. EX: UNRESTRICTED, RESTRICTED, NEVER
* @param cachePolicy cachePolicy or {@code null} for none
*/
public Geo setCachePolicy(java.lang.String cachePolicy) {
this.cachePolicy = cachePolicy;
return this;
}
/**
* The country code of the location.
* @return value or {@code null} for none
*/
public java.lang.String getCountryCode() {
return countryCode;
}
/**
* The country code of the location.
* @param countryCode countryCode or {@code null} for none
*/
public Geo setCountryCode(java.lang.String countryCode) {
this.countryCode = countryCode;
return this;
}
/**
* The latitude of the location.
* @return value or {@code null} for none
*/
public java.lang.Double getLatitude() {
return latitude;
}
/**
* The latitude of the location.
* @param latitude latitude or {@code null} for none
*/
public Geo setLatitude(java.lang.Double latitude) {
this.latitude = latitude;
return this;
}
/**
* The longitude of the location.
* @return value or {@code null} for none
*/
public java.lang.Double getLongitude() {
return longitude;
}
/**
* The longitude of the location.
* @param longitude longitude or {@code null} for none
*/
public Geo setLongitude(java.lang.Double longitude) {
this.longitude = longitude;
return this;
}
/**
* The type of map that should be used for this location. EX: HYBRID, ROADMAP, SATELLITE, TERRAIN
* @return value or {@code null} for none
*/
public java.lang.String getMapType() {
return mapType;
}
/**
* The type of map that should be used for this location. EX: HYBRID, ROADMAP, SATELLITE, TERRAIN
* @param mapType mapType or {@code null} for none
*/
public Geo setMapType(java.lang.String mapType) {
this.mapType = mapType;
return this;
}
/**
* The viewport for showing this location. This is a latitude, longitude rectangle.
* @return value or {@code null} for none
*/
public Viewport getViewport() {
return viewport;
}
/**
* The viewport for showing this location. This is a latitude, longitude rectangle.
* @param viewport viewport or {@code null} for none
*/
public Geo setViewport(Viewport viewport) {
this.viewport = viewport;
return this;
}
/**
* The Zoom level to use for the map. Zoom levels between 0 (the lowest zoom level, in which the
* entire world can be seen on one map) to 21+ (down to individual buildings). See:
* https://developers.google.com/maps/documentation/staticmaps/#Zoomlevels
* @return value or {@code null} for none
*/
public java.lang.Integer getZoom() {
return zoom;
}
/**
* The Zoom level to use for the map. Zoom levels between 0 (the lowest zoom level, in which the
* entire world can be seen on one map) to 21+ (down to individual buildings). See:
* https://developers.google.com/maps/documentation/staticmaps/#Zoomlevels
* @param zoom zoom or {@code null} for none
*/
public Geo setZoom(java.lang.Integer zoom) {
this.zoom = zoom;
return this;
}
@Override
public Geo set(String fieldName, Object value) {
return (Geo) super.set(fieldName, value);
}
@Override
public Geo clone() {
return (Geo) super.clone();
}
/**
* Model definition for GeolayerdataGeoBoundary.
*/
public static final class Boundary extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long latitude;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long longitude;
/**
* @return value or {@code null} for none
*/
public java.lang.Long getLatitude() {
return latitude;
}
/**
* @param latitude latitude or {@code null} for none
*/
public Boundary setLatitude(java.lang.Long latitude) {
this.latitude = latitude;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getLongitude() {
return longitude;
}
/**
* @param longitude longitude or {@code null} for none
*/
public Boundary setLongitude(java.lang.Long longitude) {
this.longitude = longitude;
return this;
}
@Override
public Boundary set(String fieldName, Object value) {
return (Boundary) super.set(fieldName, value);
}
@Override
public Boundary clone() {
return (Boundary) super.clone();
}
}
/**
* The viewport for showing this location. This is a latitude, longitude rectangle.
*/
public static final class Viewport extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Hi hi;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Lo lo;
/**
* @return value or {@code null} for none
*/
public Hi getHi() {
return hi;
}
/**
* @param hi hi or {@code null} for none
*/
public Viewport setHi(Hi hi) {
this.hi = hi;
return this;
}
/**
* @return value or {@code null} for none
*/
public Lo getLo() {
return lo;
}
/**
* @param lo lo or {@code null} for none
*/
public Viewport setLo(Lo lo) {
this.lo = lo;
return this;
}
@Override
public Viewport set(String fieldName, Object value) {
return (Viewport) super.set(fieldName, value);
}
@Override
public Viewport clone() {
return (Viewport) super.clone();
}
/**
* Model definition for GeolayerdataGeoViewportHi.
*/
public static final class Hi extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double latitude;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double longitude;
/**
* @return value or {@code null} for none
*/
public java.lang.Double getLatitude() {
return latitude;
}
/**
* @param latitude latitude or {@code null} for none
*/
public Hi setLatitude(java.lang.Double latitude) {
this.latitude = latitude;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Double getLongitude() {
return longitude;
}
/**
* @param longitude longitude or {@code null} for none
*/
public Hi setLongitude(java.lang.Double longitude) {
this.longitude = longitude;
return this;
}
@Override
public Hi set(String fieldName, Object value) {
return (Hi) super.set(fieldName, value);
}
@Override
public Hi clone() {
return (Hi) super.clone();
}
}
/**
* Model definition for GeolayerdataGeoViewportLo.
*/
public static final class Lo extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double latitude;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double longitude;
/**
* @return value or {@code null} for none
*/
public java.lang.Double getLatitude() {
return latitude;
}
/**
* @param latitude latitude or {@code null} for none
*/
public Lo setLatitude(java.lang.Double latitude) {
this.latitude = latitude;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Double getLongitude() {
return longitude;
}
/**
* @param longitude longitude or {@code null} for none
*/
public Lo setLongitude(java.lang.Double longitude) {
this.longitude = longitude;
return this;
}
@Override
public Lo set(String fieldName, Object value) {
return (Lo) super.set(fieldName, value);
}
@Override
public Lo clone() {
return (Lo) super.clone();
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy