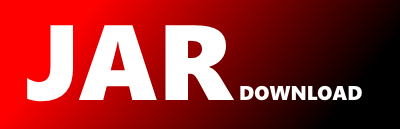
com.google.api.services.calendar.model.EventWorkingLocationProperties Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.calendar.model;
/**
* Model definition for EventWorkingLocationProperties.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Calendar API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class EventWorkingLocationProperties extends com.google.api.client.json.GenericJson {
/**
* If present, specifies that the user is working from a custom location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CustomLocation customLocation;
/**
* If present, specifies that the user is working at home.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Object homeOffice;
/**
* If present, specifies that the user is working from an office.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private OfficeLocation officeLocation;
/**
* Type of the working location. Possible values are: - "homeOffice" - The user is working at
* home. - "officeLocation" - The user is working from an office. - "customLocation" - The user
* is working from a custom location. Any details are specified in a sub-field of the specified
* name, but this field may be missing if empty. Any other fields are ignored. Required when
* adding working location properties.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* If present, specifies that the user is working from a custom location.
* @return value or {@code null} for none
*/
public CustomLocation getCustomLocation() {
return customLocation;
}
/**
* If present, specifies that the user is working from a custom location.
* @param customLocation customLocation or {@code null} for none
*/
public EventWorkingLocationProperties setCustomLocation(CustomLocation customLocation) {
this.customLocation = customLocation;
return this;
}
/**
* If present, specifies that the user is working at home.
* @return value or {@code null} for none
*/
public java.lang.Object getHomeOffice() {
return homeOffice;
}
/**
* If present, specifies that the user is working at home.
* @param homeOffice homeOffice or {@code null} for none
*/
public EventWorkingLocationProperties setHomeOffice(java.lang.Object homeOffice) {
this.homeOffice = homeOffice;
return this;
}
/**
* If present, specifies that the user is working from an office.
* @return value or {@code null} for none
*/
public OfficeLocation getOfficeLocation() {
return officeLocation;
}
/**
* If present, specifies that the user is working from an office.
* @param officeLocation officeLocation or {@code null} for none
*/
public EventWorkingLocationProperties setOfficeLocation(OfficeLocation officeLocation) {
this.officeLocation = officeLocation;
return this;
}
/**
* Type of the working location. Possible values are: - "homeOffice" - The user is working at
* home. - "officeLocation" - The user is working from an office. - "customLocation" - The user
* is working from a custom location. Any details are specified in a sub-field of the specified
* name, but this field may be missing if empty. Any other fields are ignored. Required when
* adding working location properties.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Type of the working location. Possible values are: - "homeOffice" - The user is working at
* home. - "officeLocation" - The user is working from an office. - "customLocation" - The user
* is working from a custom location. Any details are specified in a sub-field of the specified
* name, but this field may be missing if empty. Any other fields are ignored. Required when
* adding working location properties.
* @param type type or {@code null} for none
*/
public EventWorkingLocationProperties setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public EventWorkingLocationProperties set(String fieldName, Object value) {
return (EventWorkingLocationProperties) super.set(fieldName, value);
}
@Override
public EventWorkingLocationProperties clone() {
return (EventWorkingLocationProperties) super.clone();
}
/**
* If present, specifies that the user is working from a custom location.
*/
public static final class CustomLocation extends com.google.api.client.json.GenericJson {
/**
* An optional extra label for additional information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String label;
/**
* An optional extra label for additional information.
* @return value or {@code null} for none
*/
public java.lang.String getLabel() {
return label;
}
/**
* An optional extra label for additional information.
* @param label label or {@code null} for none
*/
public CustomLocation setLabel(java.lang.String label) {
this.label = label;
return this;
}
@Override
public CustomLocation set(String fieldName, Object value) {
return (CustomLocation) super.set(fieldName, value);
}
@Override
public CustomLocation clone() {
return (CustomLocation) super.clone();
}
}
/**
* If present, specifies that the user is working from an office.
*/
public static final class OfficeLocation extends com.google.api.client.json.GenericJson {
/**
* An optional building identifier. This should reference a building ID in the organization's
* Resources database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String buildingId;
/**
* An optional desk identifier.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deskId;
/**
* An optional floor identifier.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String floorId;
/**
* An optional floor section identifier.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String floorSectionId;
/**
* The office name that's displayed in Calendar Web and Mobile clients. We recommend you reference
* a building name in the organization's Resources database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String label;
/**
* An optional building identifier. This should reference a building ID in the organization's
* Resources database.
* @return value or {@code null} for none
*/
public java.lang.String getBuildingId() {
return buildingId;
}
/**
* An optional building identifier. This should reference a building ID in the organization's
* Resources database.
* @param buildingId buildingId or {@code null} for none
*/
public OfficeLocation setBuildingId(java.lang.String buildingId) {
this.buildingId = buildingId;
return this;
}
/**
* An optional desk identifier.
* @return value or {@code null} for none
*/
public java.lang.String getDeskId() {
return deskId;
}
/**
* An optional desk identifier.
* @param deskId deskId or {@code null} for none
*/
public OfficeLocation setDeskId(java.lang.String deskId) {
this.deskId = deskId;
return this;
}
/**
* An optional floor identifier.
* @return value or {@code null} for none
*/
public java.lang.String getFloorId() {
return floorId;
}
/**
* An optional floor identifier.
* @param floorId floorId or {@code null} for none
*/
public OfficeLocation setFloorId(java.lang.String floorId) {
this.floorId = floorId;
return this;
}
/**
* An optional floor section identifier.
* @return value or {@code null} for none
*/
public java.lang.String getFloorSectionId() {
return floorSectionId;
}
/**
* An optional floor section identifier.
* @param floorSectionId floorSectionId or {@code null} for none
*/
public OfficeLocation setFloorSectionId(java.lang.String floorSectionId) {
this.floorSectionId = floorSectionId;
return this;
}
/**
* The office name that's displayed in Calendar Web and Mobile clients. We recommend you reference
* a building name in the organization's Resources database.
* @return value or {@code null} for none
*/
public java.lang.String getLabel() {
return label;
}
/**
* The office name that's displayed in Calendar Web and Mobile clients. We recommend you reference
* a building name in the organization's Resources database.
* @param label label or {@code null} for none
*/
public OfficeLocation setLabel(java.lang.String label) {
this.label = label;
return this;
}
@Override
public OfficeLocation set(String fieldName, Object value) {
return (OfficeLocation) super.set(fieldName, value);
}
@Override
public OfficeLocation clone() {
return (OfficeLocation) super.clone();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy