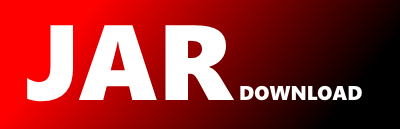
target.apidocs.com.google.api.services.calendar.model.Event.html Maven / Gradle / Ivy
Event (Calendar API v3-rev20241101-2.0.0)
com.google.api.services.calendar.model
Class Event
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.calendar.model.Event
-
public final class Event
extends com.google.api.client.json.GenericJson
Model definition for Event.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Calendar API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
Event.Creator
The creator of the event.
static class
Event.ExtendedProperties
Extended properties of the event.
static class
Event.Gadget
A gadget that extends this event.
static class
Event.Organizer
The organizer of the event.
static class
Event.Reminders
Information about the event's reminders for the authenticated user.
static class
Event.Source
Source from which the event was created.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Event()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Event
clone()
Boolean
getAnyoneCanAddSelf()
Whether anyone can invite themselves to the event (deprecated).
List<EventAttachment>
getAttachments()
File attachments for the event.
List<EventAttendee>
getAttendees()
The attendees of the event.
Boolean
getAttendeesOmitted()
Whether attendees may have been omitted from the event's representation.
EventBirthdayProperties
getBirthdayProperties()
Birthday or special event data.
String
getColorId()
The color of the event.
ConferenceData
getConferenceData()
The conference-related information, such as details of a Google Meet conference.
com.google.api.client.util.DateTime
getCreated()
Creation time of the event (as a RFC3339 timestamp).
Event.Creator
getCreator()
The creator of the event.
String
getDescription()
Description of the event.
EventDateTime
getEnd()
The (exclusive) end time of the event.
Boolean
getEndTimeUnspecified()
Whether the end time is actually unspecified.
String
getEtag()
ETag of the resource.
String
getEventType()
Specific type of the event.
Event.ExtendedProperties
getExtendedProperties()
Extended properties of the event.
EventFocusTimeProperties
getFocusTimeProperties()
Focus Time event data.
Event.Gadget
getGadget()
A gadget that extends this event.
Boolean
getGuestsCanInviteOthers()
Whether attendees other than the organizer can invite others to the event.
Boolean
getGuestsCanModify()
Whether attendees other than the organizer can modify the event.
Boolean
getGuestsCanSeeOtherGuests()
Whether attendees other than the organizer can see who the event's attendees are.
String
getHangoutLink()
An absolute link to the Google Hangout associated with this event.
String
getHtmlLink()
An absolute link to this event in the Google Calendar Web UI.
String
getICalUID()
Event unique identifier as defined in RFC5545.
String
getId()
Opaque identifier of the event.
String
getKind()
Type of the resource ("calendar#event").
String
getLocation()
Geographic location of the event as free-form text.
Boolean
getLocked()
Whether this is a locked event copy where no changes can be made to the main event fields
"summary", "description", "location", "start", "end" or "recurrence".
Event.Organizer
getOrganizer()
The organizer of the event.
EventDateTime
getOriginalStartTime()
For an instance of a recurring event, this is the time at which this event would start
according to the recurrence data in the recurring event identified by recurringEventId.
EventOutOfOfficeProperties
getOutOfOfficeProperties()
Out of office event data.
Boolean
getPrivateCopy()
If set to True, Event propagation is disabled.
List<String>
getRecurrence()
List of RRULE, EXRULE, RDATE and EXDATE lines for a recurring event, as specified in RFC5545.
String
getRecurringEventId()
For an instance of a recurring event, this is the id of the recurring event to which this
instance belongs.
Event.Reminders
getReminders()
Information about the event's reminders for the authenticated user.
Integer
getSequence()
Sequence number as per iCalendar.
Event.Source
getSource()
Source from which the event was created.
EventDateTime
getStart()
The (inclusive) start time of the event.
String
getStatus()
Status of the event.
String
getSummary()
Title of the event.
String
getTransparency()
Whether the event blocks time on the calendar.
com.google.api.client.util.DateTime
getUpdated()
Last modification time of the main event data (as a RFC3339 timestamp).
String
getVisibility()
Visibility of the event.
EventWorkingLocationProperties
getWorkingLocationProperties()
Working location event data.
boolean
isAnyoneCanAddSelf()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isAttendeesOmitted()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isEndTimeUnspecified()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isGuestsCanInviteOthers()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isGuestsCanModify()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isGuestsCanSeeOtherGuests()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isLocked()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isPrivateCopy()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Event
set(String fieldName,
Object value)
Event
setAnyoneCanAddSelf(Boolean anyoneCanAddSelf)
Whether anyone can invite themselves to the event (deprecated).
Event
setAttachments(List<EventAttachment> attachments)
File attachments for the event.
Event
setAttendees(List<EventAttendee> attendees)
The attendees of the event.
Event
setAttendeesOmitted(Boolean attendeesOmitted)
Whether attendees may have been omitted from the event's representation.
Event
setBirthdayProperties(EventBirthdayProperties birthdayProperties)
Birthday or special event data.
Event
setColorId(String colorId)
The color of the event.
Event
setConferenceData(ConferenceData conferenceData)
The conference-related information, such as details of a Google Meet conference.
Event
setCreated(com.google.api.client.util.DateTime created)
Creation time of the event (as a RFC3339 timestamp).
Event
setCreator(Event.Creator creator)
The creator of the event.
Event
setDescription(String description)
Description of the event.
Event
setEnd(EventDateTime end)
The (exclusive) end time of the event.
Event
setEndTimeUnspecified(Boolean endTimeUnspecified)
Whether the end time is actually unspecified.
Event
setEtag(String etag)
ETag of the resource.
Event
setEventType(String eventType)
Specific type of the event.
Event
setExtendedProperties(Event.ExtendedProperties extendedProperties)
Extended properties of the event.
Event
setFocusTimeProperties(EventFocusTimeProperties focusTimeProperties)
Focus Time event data.
Event
setGadget(Event.Gadget gadget)
A gadget that extends this event.
Event
setGuestsCanInviteOthers(Boolean guestsCanInviteOthers)
Whether attendees other than the organizer can invite others to the event.
Event
setGuestsCanModify(Boolean guestsCanModify)
Whether attendees other than the organizer can modify the event.
Event
setGuestsCanSeeOtherGuests(Boolean guestsCanSeeOtherGuests)
Whether attendees other than the organizer can see who the event's attendees are.
Event
setHangoutLink(String hangoutLink)
An absolute link to the Google Hangout associated with this event.
Event
setHtmlLink(String htmlLink)
An absolute link to this event in the Google Calendar Web UI.
Event
setICalUID(String iCalUID)
Event unique identifier as defined in RFC5545.
Event
setId(String id)
Opaque identifier of the event.
Event
setKind(String kind)
Type of the resource ("calendar#event").
Event
setLocation(String location)
Geographic location of the event as free-form text.
Event
setLocked(Boolean locked)
Whether this is a locked event copy where no changes can be made to the main event fields
"summary", "description", "location", "start", "end" or "recurrence".
Event
setOrganizer(Event.Organizer organizer)
The organizer of the event.
Event
setOriginalStartTime(EventDateTime originalStartTime)
For an instance of a recurring event, this is the time at which this event would start
according to the recurrence data in the recurring event identified by recurringEventId.
Event
setOutOfOfficeProperties(EventOutOfOfficeProperties outOfOfficeProperties)
Out of office event data.
Event
setPrivateCopy(Boolean privateCopy)
If set to True, Event propagation is disabled.
Event
setRecurrence(List<String> recurrence)
List of RRULE, EXRULE, RDATE and EXDATE lines for a recurring event, as specified in RFC5545.
Event
setRecurringEventId(String recurringEventId)
For an instance of a recurring event, this is the id of the recurring event to which this
instance belongs.
Event
setReminders(Event.Reminders reminders)
Information about the event's reminders for the authenticated user.
Event
setSequence(Integer sequence)
Sequence number as per iCalendar.
Event
setSource(Event.Source source)
Source from which the event was created.
Event
setStart(EventDateTime start)
The (inclusive) start time of the event.
Event
setStatus(String status)
Status of the event.
Event
setSummary(String summary)
Title of the event.
Event
setTransparency(String transparency)
Whether the event blocks time on the calendar.
Event
setUpdated(com.google.api.client.util.DateTime updated)
Last modification time of the main event data (as a RFC3339 timestamp).
Event
setVisibility(String visibility)
Visibility of the event.
Event
setWorkingLocationProperties(EventWorkingLocationProperties workingLocationProperties)
Working location event data.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAnyoneCanAddSelf
public Boolean getAnyoneCanAddSelf()
Whether anyone can invite themselves to the event (deprecated). Optional. The default is False.
- Returns:
- value or
null
for none
-
setAnyoneCanAddSelf
public Event setAnyoneCanAddSelf(Boolean anyoneCanAddSelf)
Whether anyone can invite themselves to the event (deprecated). Optional. The default is False.
- Parameters:
anyoneCanAddSelf
- anyoneCanAddSelf or null
for none
-
isAnyoneCanAddSelf
public boolean isAnyoneCanAddSelf()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Whether anyone can invite themselves to the event (deprecated). Optional. The default is False.
-
getAttachments
public List<EventAttachment> getAttachments()
File attachments for the event. In order to modify attachments the supportsAttachments request
parameter should be set to true. There can be at most 25 attachments per event,
- Returns:
- value or
null
for none
-
setAttachments
public Event setAttachments(List<EventAttachment> attachments)
File attachments for the event. In order to modify attachments the supportsAttachments request
parameter should be set to true. There can be at most 25 attachments per event,
- Parameters:
attachments
- attachments or null
for none
-
getAttendees
public List<EventAttendee> getAttendees()
The attendees of the event. See the Events with attendees guide for more information on
scheduling events with other calendar users. Service accounts need to use domain-wide
delegation of authority to populate the attendee list.
- Returns:
- value or
null
for none
-
setAttendees
public Event setAttendees(List<EventAttendee> attendees)
The attendees of the event. See the Events with attendees guide for more information on
scheduling events with other calendar users. Service accounts need to use domain-wide
delegation of authority to populate the attendee list.
- Parameters:
attendees
- attendees or null
for none
-
getAttendeesOmitted
public Boolean getAttendeesOmitted()
Whether attendees may have been omitted from the event's representation. When retrieving an
event, this may be due to a restriction specified by the maxAttendee query parameter. When
updating an event, this can be used to only update the participant's response. Optional. The
default is False.
- Returns:
- value or
null
for none
-
setAttendeesOmitted
public Event setAttendeesOmitted(Boolean attendeesOmitted)
Whether attendees may have been omitted from the event's representation. When retrieving an
event, this may be due to a restriction specified by the maxAttendee query parameter. When
updating an event, this can be used to only update the participant's response. Optional. The
default is False.
- Parameters:
attendeesOmitted
- attendeesOmitted or null
for none
-
isAttendeesOmitted
public boolean isAttendeesOmitted()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Whether attendees may have been omitted from the event's representation. When retrieving an event,
this may be due to a restriction specified by the maxAttendee query parameter. When updating an
event, this can be used to only update the participant's response. Optional. The default is False.
-
getBirthdayProperties
public EventBirthdayProperties getBirthdayProperties()
Birthday or special event data. Used if eventType is "birthday". Immutable.
- Returns:
- value or
null
for none
-
setBirthdayProperties
public Event setBirthdayProperties(EventBirthdayProperties birthdayProperties)
Birthday or special event data. Used if eventType is "birthday". Immutable.
- Parameters:
birthdayProperties
- birthdayProperties or null
for none
-
getColorId
public String getColorId()
The color of the event. This is an ID referring to an entry in the event section of the colors
definition (see the colors endpoint). Optional.
- Returns:
- value or
null
for none
-
setColorId
public Event setColorId(String colorId)
The color of the event. This is an ID referring to an entry in the event section of the colors
definition (see the colors endpoint). Optional.
- Parameters:
colorId
- colorId or null
for none
-
getConferenceData
public ConferenceData getConferenceData()
The conference-related information, such as details of a Google Meet conference. To create new
conference details use the createRequest field. To persist your changes, remember to set the
conferenceDataVersion request parameter to 1 for all event modification requests.
- Returns:
- value or
null
for none
-
setConferenceData
public Event setConferenceData(ConferenceData conferenceData)
The conference-related information, such as details of a Google Meet conference. To create new
conference details use the createRequest field. To persist your changes, remember to set the
conferenceDataVersion request parameter to 1 for all event modification requests.
- Parameters:
conferenceData
- conferenceData or null
for none
-
getCreated
public com.google.api.client.util.DateTime getCreated()
Creation time of the event (as a RFC3339 timestamp). Read-only.
- Returns:
- value or
null
for none
-
setCreated
public Event setCreated(com.google.api.client.util.DateTime created)
Creation time of the event (as a RFC3339 timestamp). Read-only.
- Parameters:
created
- created or null
for none
-
getCreator
public Event.Creator getCreator()
The creator of the event. Read-only.
- Returns:
- value or
null
for none
-
setCreator
public Event setCreator(Event.Creator creator)
The creator of the event. Read-only.
- Parameters:
creator
- creator or null
for none
-
getDescription
public String getDescription()
Description of the event. Can contain HTML. Optional.
- Returns:
- value or
null
for none
-
setDescription
public Event setDescription(String description)
Description of the event. Can contain HTML. Optional.
- Parameters:
description
- description or null
for none
-
getEnd
public EventDateTime getEnd()
The (exclusive) end time of the event. For a recurring event, this is the end time of the first
instance.
- Returns:
- value or
null
for none
-
setEnd
public Event setEnd(EventDateTime end)
The (exclusive) end time of the event. For a recurring event, this is the end time of the first
instance.
- Parameters:
end
- end or null
for none
-
getEndTimeUnspecified
public Boolean getEndTimeUnspecified()
Whether the end time is actually unspecified. An end time is still provided for compatibility
reasons, even if this attribute is set to True. The default is False.
- Returns:
- value or
null
for none
-
setEndTimeUnspecified
public Event setEndTimeUnspecified(Boolean endTimeUnspecified)
Whether the end time is actually unspecified. An end time is still provided for compatibility
reasons, even if this attribute is set to True. The default is False.
- Parameters:
endTimeUnspecified
- endTimeUnspecified or null
for none
-
isEndTimeUnspecified
public boolean isEndTimeUnspecified()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Whether the end time is actually unspecified. An end time is still provided for compatibility
reasons, even if this attribute is set to True. The default is False.
-
getEtag
public String getEtag()
ETag of the resource.
- Returns:
- value or
null
for none
-
setEtag
public Event setEtag(String etag)
ETag of the resource.
- Parameters:
etag
- etag or null
for none
-
getEventType
public String getEventType()
Specific type of the event. This cannot be modified after the event is created. Possible values
are: - "birthday" - A special all-day event with an annual recurrence. - "default" - A regular
event or not further specified. - "focusTime" - A focus-time event. - "fromGmail" - An event
from Gmail. This type of event cannot be created. - "outOfOffice" - An out-of-office event. -
"workingLocation" - A working location event.
- Returns:
- value or
null
for none
-
setEventType
public Event setEventType(String eventType)
Specific type of the event. This cannot be modified after the event is created. Possible values
are: - "birthday" - A special all-day event with an annual recurrence. - "default" - A regular
event or not further specified. - "focusTime" - A focus-time event. - "fromGmail" - An event
from Gmail. This type of event cannot be created. - "outOfOffice" - An out-of-office event. -
"workingLocation" - A working location event.
- Parameters:
eventType
- eventType or null
for none
-
getExtendedProperties
public Event.ExtendedProperties getExtendedProperties()
Extended properties of the event.
- Returns:
- value or
null
for none
-
setExtendedProperties
public Event setExtendedProperties(Event.ExtendedProperties extendedProperties)
Extended properties of the event.
- Parameters:
extendedProperties
- extendedProperties or null
for none
-
getFocusTimeProperties
public EventFocusTimeProperties getFocusTimeProperties()
Focus Time event data. Used if eventType is focusTime.
- Returns:
- value or
null
for none
-
setFocusTimeProperties
public Event setFocusTimeProperties(EventFocusTimeProperties focusTimeProperties)
Focus Time event data. Used if eventType is focusTime.
- Parameters:
focusTimeProperties
- focusTimeProperties or null
for none
-
getGadget
public Event.Gadget getGadget()
A gadget that extends this event. Gadgets are deprecated; this structure is instead only used
for returning birthday calendar metadata.
- Returns:
- value or
null
for none
-
setGadget
public Event setGadget(Event.Gadget gadget)
A gadget that extends this event. Gadgets are deprecated; this structure is instead only used
for returning birthday calendar metadata.
- Parameters:
gadget
- gadget or null
for none
-
getGuestsCanInviteOthers
public Boolean getGuestsCanInviteOthers()
Whether attendees other than the organizer can invite others to the event. Optional. The
default is True.
- Returns:
- value or
null
for none
-
setGuestsCanInviteOthers
public Event setGuestsCanInviteOthers(Boolean guestsCanInviteOthers)
Whether attendees other than the organizer can invite others to the event. Optional. The
default is True.
- Parameters:
guestsCanInviteOthers
- guestsCanInviteOthers or null
for none
-
isGuestsCanInviteOthers
public boolean isGuestsCanInviteOthers()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Whether attendees other than the organizer can invite others to the event. Optional. The default is
True.
-
getGuestsCanModify
public Boolean getGuestsCanModify()
Whether attendees other than the organizer can modify the event. Optional. The default is
False.
- Returns:
- value or
null
for none
-
setGuestsCanModify
public Event setGuestsCanModify(Boolean guestsCanModify)
Whether attendees other than the organizer can modify the event. Optional. The default is
False.
- Parameters:
guestsCanModify
- guestsCanModify or null
for none
-
isGuestsCanModify
public boolean isGuestsCanModify()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Whether attendees other than the organizer can modify the event. Optional. The default is False.
-
getGuestsCanSeeOtherGuests
public Boolean getGuestsCanSeeOtherGuests()
Whether attendees other than the organizer can see who the event's attendees are. Optional. The
default is True.
- Returns:
- value or
null
for none
-
setGuestsCanSeeOtherGuests
public Event setGuestsCanSeeOtherGuests(Boolean guestsCanSeeOtherGuests)
Whether attendees other than the organizer can see who the event's attendees are. Optional. The
default is True.
- Parameters:
guestsCanSeeOtherGuests
- guestsCanSeeOtherGuests or null
for none
-
isGuestsCanSeeOtherGuests
public boolean isGuestsCanSeeOtherGuests()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Whether attendees other than the organizer can see who the event's attendees are. Optional. The
default is True.
-
getHangoutLink
public String getHangoutLink()
An absolute link to the Google Hangout associated with this event. Read-only.
- Returns:
- value or
null
for none
-
setHangoutLink
public Event setHangoutLink(String hangoutLink)
An absolute link to the Google Hangout associated with this event. Read-only.
- Parameters:
hangoutLink
- hangoutLink or null
for none
-
getHtmlLink
public String getHtmlLink()
An absolute link to this event in the Google Calendar Web UI. Read-only.
- Returns:
- value or
null
for none
-
setHtmlLink
public Event setHtmlLink(String htmlLink)
An absolute link to this event in the Google Calendar Web UI. Read-only.
- Parameters:
htmlLink
- htmlLink or null
for none
-
getICalUID
public String getICalUID()
Event unique identifier as defined in RFC5545. It is used to uniquely identify events accross
calendaring systems and must be supplied when importing events via the import method. Note that
the iCalUID and the id are not identical and only one of them should be supplied at event
creation time. One difference in their semantics is that in recurring events, all occurrences
of one event have different ids while they all share the same iCalUIDs. To retrieve an event
using its iCalUID, call the events.list method using the iCalUID parameter. To retrieve an
event using its id, call the events.get method.
- Returns:
- value or
null
for none
-
setICalUID
public Event setICalUID(String iCalUID)
Event unique identifier as defined in RFC5545. It is used to uniquely identify events accross
calendaring systems and must be supplied when importing events via the import method. Note that
the iCalUID and the id are not identical and only one of them should be supplied at event
creation time. One difference in their semantics is that in recurring events, all occurrences
of one event have different ids while they all share the same iCalUIDs. To retrieve an event
using its iCalUID, call the events.list method using the iCalUID parameter. To retrieve an
event using its id, call the events.get method.
- Parameters:
iCalUID
- iCalUID or null
for none
-
getId
public String getId()
Opaque identifier of the event. When creating new single or recurring events, you can specify
their IDs. Provided IDs must follow these rules: - characters allowed in the ID are those used
in base32hex encoding, i.e. lowercase letters a-v and digits 0-9, see section 3.1.2 in RFC2938
- the length of the ID must be between 5 and 1024 characters - the ID must be unique per
calendar Due to the globally distributed nature of the system, we cannot guarantee that ID
collisions will be detected at event creation time. To minimize the risk of collisions we
recommend using an established UUID algorithm such as one described in RFC4122. If you do not
specify an ID, it will be automatically generated by the server. Note that the icalUID and the
id are not identical and only one of them should be supplied at event creation time. One
difference in their semantics is that in recurring events, all occurrences of one event have
different ids while they all share the same icalUIDs.
- Returns:
- value or
null
for none
-
setId
public Event setId(String id)
Opaque identifier of the event. When creating new single or recurring events, you can specify
their IDs. Provided IDs must follow these rules: - characters allowed in the ID are those used
in base32hex encoding, i.e. lowercase letters a-v and digits 0-9, see section 3.1.2 in RFC2938
- the length of the ID must be between 5 and 1024 characters - the ID must be unique per
calendar Due to the globally distributed nature of the system, we cannot guarantee that ID
collisions will be detected at event creation time. To minimize the risk of collisions we
recommend using an established UUID algorithm such as one described in RFC4122. If you do not
specify an ID, it will be automatically generated by the server. Note that the icalUID and the
id are not identical and only one of them should be supplied at event creation time. One
difference in their semantics is that in recurring events, all occurrences of one event have
different ids while they all share the same icalUIDs.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
Type of the resource ("calendar#event").
- Returns:
- value or
null
for none
-
setKind
public Event setKind(String kind)
Type of the resource ("calendar#event").
- Parameters:
kind
- kind or null
for none
-
getLocation
public String getLocation()
Geographic location of the event as free-form text. Optional.
- Returns:
- value or
null
for none
-
setLocation
public Event setLocation(String location)
Geographic location of the event as free-form text. Optional.
- Parameters:
location
- location or null
for none
-
getLocked
public Boolean getLocked()
Whether this is a locked event copy where no changes can be made to the main event fields
"summary", "description", "location", "start", "end" or "recurrence". The default is False.
Read-Only.
- Returns:
- value or
null
for none
-
setLocked
public Event setLocked(Boolean locked)
Whether this is a locked event copy where no changes can be made to the main event fields
"summary", "description", "location", "start", "end" or "recurrence". The default is False.
Read-Only.
- Parameters:
locked
- locked or null
for none
-
isLocked
public boolean isLocked()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Whether this is a locked event copy where no changes can be made to the main event fields
"summary", "description", "location", "start", "end" or "recurrence". The default is False. Read-
Only.
-
getOrganizer
public Event.Organizer getOrganizer()
The organizer of the event. If the organizer is also an attendee, this is indicated with a
separate entry in attendees with the organizer field set to True. To change the organizer, use
the move operation. Read-only, except when importing an event.
- Returns:
- value or
null
for none
-
setOrganizer
public Event setOrganizer(Event.Organizer organizer)
The organizer of the event. If the organizer is also an attendee, this is indicated with a
separate entry in attendees with the organizer field set to True. To change the organizer, use
the move operation. Read-only, except when importing an event.
- Parameters:
organizer
- organizer or null
for none
-
getOriginalStartTime
public EventDateTime getOriginalStartTime()
For an instance of a recurring event, this is the time at which this event would start
according to the recurrence data in the recurring event identified by recurringEventId. It
uniquely identifies the instance within the recurring event series even if the instance was
moved to a different time. Immutable.
- Returns:
- value or
null
for none
-
setOriginalStartTime
public Event setOriginalStartTime(EventDateTime originalStartTime)
For an instance of a recurring event, this is the time at which this event would start
according to the recurrence data in the recurring event identified by recurringEventId. It
uniquely identifies the instance within the recurring event series even if the instance was
moved to a different time. Immutable.
- Parameters:
originalStartTime
- originalStartTime or null
for none
-
getOutOfOfficeProperties
public EventOutOfOfficeProperties getOutOfOfficeProperties()
Out of office event data. Used if eventType is outOfOffice.
- Returns:
- value or
null
for none
-
setOutOfOfficeProperties
public Event setOutOfOfficeProperties(EventOutOfOfficeProperties outOfOfficeProperties)
Out of office event data. Used if eventType is outOfOffice.
- Parameters:
outOfOfficeProperties
- outOfOfficeProperties or null
for none
-
getPrivateCopy
public Boolean getPrivateCopy()
If set to True, Event propagation is disabled. Note that it is not the same thing as Private
event properties. Optional. Immutable. The default is False.
- Returns:
- value or
null
for none
-
setPrivateCopy
public Event setPrivateCopy(Boolean privateCopy)
If set to True, Event propagation is disabled. Note that it is not the same thing as Private
event properties. Optional. Immutable. The default is False.
- Parameters:
privateCopy
- privateCopy or null
for none
-
isPrivateCopy
public boolean isPrivateCopy()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
If set to True, Event propagation is disabled. Note that it is not the same thing as Private event
properties. Optional. Immutable. The default is False.
-
getRecurrence
public List<String> getRecurrence()
List of RRULE, EXRULE, RDATE and EXDATE lines for a recurring event, as specified in RFC5545.
Note that DTSTART and DTEND lines are not allowed in this field; event start and end times are
specified in the start and end fields. This field is omitted for single events or instances of
recurring events.
- Returns:
- value or
null
for none
-
setRecurrence
public Event setRecurrence(List<String> recurrence)
List of RRULE, EXRULE, RDATE and EXDATE lines for a recurring event, as specified in RFC5545.
Note that DTSTART and DTEND lines are not allowed in this field; event start and end times are
specified in the start and end fields. This field is omitted for single events or instances of
recurring events.
- Parameters:
recurrence
- recurrence or null
for none
-
getRecurringEventId
public String getRecurringEventId()
For an instance of a recurring event, this is the id of the recurring event to which this
instance belongs. Immutable.
- Returns:
- value or
null
for none
-
setRecurringEventId
public Event setRecurringEventId(String recurringEventId)
For an instance of a recurring event, this is the id of the recurring event to which this
instance belongs. Immutable.
- Parameters:
recurringEventId
- recurringEventId or null
for none
-
getReminders
public Event.Reminders getReminders()
Information about the event's reminders for the authenticated user. Note that changing
reminders does not also change the updated property of the enclosing event.
- Returns:
- value or
null
for none
-
setReminders
public Event setReminders(Event.Reminders reminders)
Information about the event's reminders for the authenticated user. Note that changing
reminders does not also change the updated property of the enclosing event.
- Parameters:
reminders
- reminders or null
for none
-
getSequence
public Integer getSequence()
Sequence number as per iCalendar.
- Returns:
- value or
null
for none
-
setSequence
public Event setSequence(Integer sequence)
Sequence number as per iCalendar.
- Parameters:
sequence
- sequence or null
for none
-
getSource
public Event.Source getSource()
Source from which the event was created. For example, a web page, an email message or any
document identifiable by an URL with HTTP or HTTPS scheme. Can only be seen or modified by the
creator of the event.
- Returns:
- value or
null
for none
-
setSource
public Event setSource(Event.Source source)
Source from which the event was created. For example, a web page, an email message or any
document identifiable by an URL with HTTP or HTTPS scheme. Can only be seen or modified by the
creator of the event.
- Parameters:
source
- source or null
for none
-
getStart
public EventDateTime getStart()
The (inclusive) start time of the event. For a recurring event, this is the start time of the
first instance.
- Returns:
- value or
null
for none
-
setStart
public Event setStart(EventDateTime start)
The (inclusive) start time of the event. For a recurring event, this is the start time of the
first instance.
- Parameters:
start
- start or null
for none
-
getStatus
public String getStatus()
Status of the event. Optional. Possible values are: - "confirmed" - The event is confirmed.
This is the default status. - "tentative" - The event is tentatively confirmed. - "cancelled"
- The event is cancelled (deleted). The list method returns cancelled events only on
incremental sync (when syncToken or updatedMin are specified) or if the showDeleted flag is set
to true. The get method always returns them. A cancelled status represents two different states
depending on the event type: - Cancelled exceptions of an uncancelled recurring event
indicate that this instance should no longer be presented to the user. Clients should store
these events for the lifetime of the parent recurring event. Cancelled exceptions are only
guaranteed to have values for the id, recurringEventId and originalStartTime fields populated.
The other fields might be empty. - All other cancelled events represent deleted events.
Clients should remove their locally synced copies. Such cancelled events will eventually
disappear, so do not rely on them being available indefinitely. Deleted events are only
guaranteed to have the id field populated. On the organizer's calendar, cancelled events
continue to expose event details (summary, location, etc.) so that they can be restored
(undeleted). Similarly, the events to which the user was invited and that they manually removed
continue to provide details. However, incremental sync requests with showDeleted set to false
will not return these details. If an event changes its organizer (for example via the move
operation) and the original organizer is not on the attendee list, it will leave behind a
cancelled event where only the id field is guaranteed to be populated.
- Returns:
- value or
null
for none
-
setStatus
public Event setStatus(String status)
Status of the event. Optional. Possible values are: - "confirmed" - The event is confirmed.
This is the default status. - "tentative" - The event is tentatively confirmed. - "cancelled"
- The event is cancelled (deleted). The list method returns cancelled events only on
incremental sync (when syncToken or updatedMin are specified) or if the showDeleted flag is set
to true. The get method always returns them. A cancelled status represents two different states
depending on the event type: - Cancelled exceptions of an uncancelled recurring event
indicate that this instance should no longer be presented to the user. Clients should store
these events for the lifetime of the parent recurring event. Cancelled exceptions are only
guaranteed to have values for the id, recurringEventId and originalStartTime fields populated.
The other fields might be empty. - All other cancelled events represent deleted events.
Clients should remove their locally synced copies. Such cancelled events will eventually
disappear, so do not rely on them being available indefinitely. Deleted events are only
guaranteed to have the id field populated. On the organizer's calendar, cancelled events
continue to expose event details (summary, location, etc.) so that they can be restored
(undeleted). Similarly, the events to which the user was invited and that they manually removed
continue to provide details. However, incremental sync requests with showDeleted set to false
will not return these details. If an event changes its organizer (for example via the move
operation) and the original organizer is not on the attendee list, it will leave behind a
cancelled event where only the id field is guaranteed to be populated.
- Parameters:
status
- status or null
for none
-
getSummary
public String getSummary()
Title of the event.
- Returns:
- value or
null
for none
-
setSummary
public Event setSummary(String summary)
Title of the event.
- Parameters:
summary
- summary or null
for none
-
getTransparency
public String getTransparency()
Whether the event blocks time on the calendar. Optional. Possible values are: - "opaque" -
Default value. The event does block time on the calendar. This is equivalent to setting Show me
as to Busy in the Calendar UI. - "transparent" - The event does not block time on the
calendar. This is equivalent to setting Show me as to Available in the Calendar UI.
- Returns:
- value or
null
for none
-
setTransparency
public Event setTransparency(String transparency)
Whether the event blocks time on the calendar. Optional. Possible values are: - "opaque" -
Default value. The event does block time on the calendar. This is equivalent to setting Show me
as to Busy in the Calendar UI. - "transparent" - The event does not block time on the
calendar. This is equivalent to setting Show me as to Available in the Calendar UI.
- Parameters:
transparency
- transparency or null
for none
-
getUpdated
public com.google.api.client.util.DateTime getUpdated()
Last modification time of the main event data (as a RFC3339 timestamp). Updating event
reminders will not cause this to change. Read-only.
- Returns:
- value or
null
for none
-
setUpdated
public Event setUpdated(com.google.api.client.util.DateTime updated)
Last modification time of the main event data (as a RFC3339 timestamp). Updating event
reminders will not cause this to change. Read-only.
- Parameters:
updated
- updated or null
for none
-
getVisibility
public String getVisibility()
Visibility of the event. Optional. Possible values are: - "default" - Uses the default
visibility for events on the calendar. This is the default value. - "public" - The event is
public and event details are visible to all readers of the calendar. - "private" - The event
is private and only event attendees may view event details. - "confidential" - The event is
private. This value is provided for compatibility reasons.
- Returns:
- value or
null
for none
-
setVisibility
public Event setVisibility(String visibility)
Visibility of the event. Optional. Possible values are: - "default" - Uses the default
visibility for events on the calendar. This is the default value. - "public" - The event is
public and event details are visible to all readers of the calendar. - "private" - The event
is private and only event attendees may view event details. - "confidential" - The event is
private. This value is provided for compatibility reasons.
- Parameters:
visibility
- visibility or null
for none
-
getWorkingLocationProperties
public EventWorkingLocationProperties getWorkingLocationProperties()
Working location event data.
- Returns:
- value or
null
for none
-
setWorkingLocationProperties
public Event setWorkingLocationProperties(EventWorkingLocationProperties workingLocationProperties)
Working location event data.
- Parameters:
workingLocationProperties
- workingLocationProperties or null
for none
-
set
public Event set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Event clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.