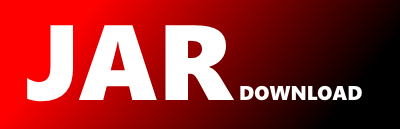
com.google.api.services.chat.v1.HangoutsChat Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1;
/**
* Service definition for HangoutsChat (v1).
*
*
* The Google Chat API lets you build Chat apps to integrate your services with Google Chat and manage Chat resources such as spaces, members, and messages.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link HangoutsChatRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class HangoutsChat extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Google Chat API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://chat.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://chat.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public HangoutsChat(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
HangoutsChat(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Media collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Media.List request = chat.media().list(parameters ...)}
*
*
* @return the resource collection
*/
public Media media() {
return new Media();
}
/**
* The "media" collection of methods.
*/
public class Media {
/**
* Downloads media. Download is supported on the URI `/v1/media/{+name}?alt=media`.
*
* Create a request for the method "media.download".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Download#execute()} method to invoke the remote operation.
*
* @param resourceName Name of the media that is being downloaded. See ReadRequest.resource_name.
* @return the request
*/
public Download download(java.lang.String resourceName) throws java.io.IOException {
Download result = new Download(resourceName);
initialize(result);
return result;
}
public class Download extends HangoutsChatRequest {
private static final String REST_PATH = "v1/media/{+resourceName}";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^.*$");
/**
* Downloads media. Download is supported on the URI `/v1/media/{+name}?alt=media`.
*
* Create a request for the method "media.download".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Download#execute()} method to invoke the remote operation.
* {@link
* Download#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resourceName Name of the media that is being downloaded. See ReadRequest.resource_name.
* @since 1.13
*/
protected Download(java.lang.String resourceName) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.Media.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^.*$");
}
initializeMediaDownload();
}
@Override
public void executeMediaAndDownloadTo(java.io.OutputStream outputStream) throws java.io.IOException {
super.executeMediaAndDownloadTo(outputStream);
}
@Override
public java.io.InputStream executeMediaAsInputStream() throws java.io.IOException {
return super.executeMediaAsInputStream();
}
@Override
public com.google.api.client.http.HttpResponse executeMedia() throws java.io.IOException {
return super.executeMedia();
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Download set$Xgafv(java.lang.String $Xgafv) {
return (Download) super.set$Xgafv($Xgafv);
}
@Override
public Download setAccessToken(java.lang.String accessToken) {
return (Download) super.setAccessToken(accessToken);
}
@Override
public Download setAlt(java.lang.String alt) {
return (Download) super.setAlt(alt);
}
@Override
public Download setCallback(java.lang.String callback) {
return (Download) super.setCallback(callback);
}
@Override
public Download setFields(java.lang.String fields) {
return (Download) super.setFields(fields);
}
@Override
public Download setKey(java.lang.String key) {
return (Download) super.setKey(key);
}
@Override
public Download setOauthToken(java.lang.String oauthToken) {
return (Download) super.setOauthToken(oauthToken);
}
@Override
public Download setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Download) super.setPrettyPrint(prettyPrint);
}
@Override
public Download setQuotaUser(java.lang.String quotaUser) {
return (Download) super.setQuotaUser(quotaUser);
}
@Override
public Download setUploadType(java.lang.String uploadType) {
return (Download) super.setUploadType(uploadType);
}
@Override
public Download setUploadProtocol(java.lang.String uploadProtocol) {
return (Download) super.setUploadProtocol(uploadProtocol);
}
/** Name of the media that is being downloaded. See ReadRequest.resource_name. */
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** Name of the media that is being downloaded. See ReadRequest.resource_name.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/** Name of the media that is being downloaded. See ReadRequest.resource_name. */
public Download setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^.*$");
}
this.resourceName = resourceName;
return this;
}
@Override
public Download set(String parameterName, Object value) {
return (Download) super.set(parameterName, value);
}
}
/**
* Uploads an attachment. For an example, see [Upload media as a file
* attachment](https://developers.google.com/workspace/chat/upload-media-attachments). Requires user
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* You can upload attachments up to 200 MB. Certain file types aren't supported. For details, see
* [File types blocked by Google Chat](https://support.google.com/chat/answer/7651457?&co=GENIE.Plat
* form%3DDesktop#File%20types%20blocked%20in%20Google%20Chat).
*
* Create a request for the method "media.upload".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the Chat space in which the attachment is uploaded. Format
* "spaces/{space}".
* @param content the {@link com.google.api.services.chat.v1.model.UploadAttachmentRequest}
* @return the request
*/
public Upload upload(java.lang.String parent, com.google.api.services.chat.v1.model.UploadAttachmentRequest content) throws java.io.IOException {
Upload result = new Upload(parent, content);
initialize(result);
return result;
}
/**
* Uploads an attachment. For an example, see [Upload media as a file
* attachment](https://developers.google.com/workspace/chat/upload-media-attachments). Requires user
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* You can upload attachments up to 200 MB. Certain file types aren't supported. For details, see
* [File types blocked by Google Chat](https://support.google.com/chat/answer/7651457?&co=GENIE.Plat
* form%3DDesktop#File%20types%20blocked%20in%20Google%20Chat).
*
* Create a request for the method "media.upload".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param parent Required. Resource name of the Chat space in which the attachment is uploaded. Format
* "spaces/{space}".
* @param content the {@link com.google.api.services.chat.v1.model.UploadAttachmentRequest} media metadata or {@code null} if none
* @param mediaContent The media HTTP content.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Upload upload(java.lang.String parent, com.google.api.services.chat.v1.model.UploadAttachmentRequest content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Upload result = new Upload(parent, content, mediaContent);
initialize(result);
return result;
}
public class Upload extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/attachments:upload";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Uploads an attachment. For an example, see [Upload media as a file
* attachment](https://developers.google.com/workspace/chat/upload-media-attachments). Requires
* user [authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user). You can upload attachments up to 200 MB. Certain file types aren't supported. For
* details, see [File types blocked by Google Chat](https://support.google.com/chat/answer/7651457
* ?&co=GENIE.Platform%3DDesktop#File%20types%20blocked%20in%20Google%20Chat).
*
* Create a request for the method "media.upload".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Upload#execute()} method to invoke the remote operation. {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the Chat space in which the attachment is uploaded. Format
* "spaces/{space}".
* @param content the {@link com.google.api.services.chat.v1.model.UploadAttachmentRequest}
* @since 1.13
*/
protected Upload(java.lang.String parent, com.google.api.services.chat.v1.model.UploadAttachmentRequest content) {
super(HangoutsChat.this, "POST", REST_PATH, content, com.google.api.services.chat.v1.model.UploadAttachmentResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
}
/**
* Uploads an attachment. For an example, see [Upload media as a file
* attachment](https://developers.google.com/workspace/chat/upload-media-attachments). Requires
* user [authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user). You can upload attachments up to 200 MB. Certain file types aren't supported. For
* details, see [File types blocked by Google Chat](https://support.google.com/chat/answer/7651457
* ?&co=GENIE.Platform%3DDesktop#File%20types%20blocked%20in%20Google%20Chat).
*
* Create a request for the method "media.upload".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Upload#execute()} method to invoke the remote operation. {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param parent Required. Resource name of the Chat space in which the attachment is uploaded. Format
* "spaces/{space}".
* @param content the {@link com.google.api.services.chat.v1.model.UploadAttachmentRequest} media metadata or {@code null} if none
* @param mediaContent The media HTTP content.
* @since 1.13
*/
protected Upload(java.lang.String parent, com.google.api.services.chat.v1.model.UploadAttachmentRequest content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(HangoutsChat.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.chat.v1.model.UploadAttachmentResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
com.google.api.client.util.Preconditions.checkNotNull(mediaContent, "Required parameter mediaContent must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Upload set$Xgafv(java.lang.String $Xgafv) {
return (Upload) super.set$Xgafv($Xgafv);
}
@Override
public Upload setAccessToken(java.lang.String accessToken) {
return (Upload) super.setAccessToken(accessToken);
}
@Override
public Upload setAlt(java.lang.String alt) {
return (Upload) super.setAlt(alt);
}
@Override
public Upload setCallback(java.lang.String callback) {
return (Upload) super.setCallback(callback);
}
@Override
public Upload setFields(java.lang.String fields) {
return (Upload) super.setFields(fields);
}
@Override
public Upload setKey(java.lang.String key) {
return (Upload) super.setKey(key);
}
@Override
public Upload setOauthToken(java.lang.String oauthToken) {
return (Upload) super.setOauthToken(oauthToken);
}
@Override
public Upload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Upload) super.setPrettyPrint(prettyPrint);
}
@Override
public Upload setQuotaUser(java.lang.String quotaUser) {
return (Upload) super.setQuotaUser(quotaUser);
}
@Override
public Upload setUploadType(java.lang.String uploadType) {
return (Upload) super.setUploadType(uploadType);
}
@Override
public Upload setUploadProtocol(java.lang.String uploadProtocol) {
return (Upload) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the Chat space in which the attachment is uploaded. Format
* "spaces/{space}".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the Chat space in which the attachment is uploaded. Format
"spaces/{space}".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the Chat space in which the attachment is uploaded. Format
* "spaces/{space}".
*/
public Upload setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Upload set(String parameterName, Object value) {
return (Upload) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Spaces collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Spaces.List request = chat.spaces().list(parameters ...)}
*
*
* @return the resource collection
*/
public Spaces spaces() {
return new Spaces();
}
/**
* The "spaces" collection of methods.
*/
public class Spaces {
/**
* Completes the [import process](https://developers.google.com/workspace/chat/import-data) for the
* specified space and makes it visible to users. Requires app authentication and domain-wide
* delegation. For more information, see [Authorize Google Chat apps to import
* data](https://developers.google.com/workspace/chat/authorize-import).
*
* Create a request for the method "spaces.completeImport".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link CompleteImport#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the import mode space. Format: `spaces/{space}`
* @param content the {@link com.google.api.services.chat.v1.model.CompleteImportSpaceRequest}
* @return the request
*/
public CompleteImport completeImport(java.lang.String name, com.google.api.services.chat.v1.model.CompleteImportSpaceRequest content) throws java.io.IOException {
CompleteImport result = new CompleteImport(name, content);
initialize(result);
return result;
}
public class CompleteImport extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}:completeImport";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Completes the [import process](https://developers.google.com/workspace/chat/import-data) for
* the specified space and makes it visible to users. Requires app authentication and domain-wide
* delegation. For more information, see [Authorize Google Chat apps to import
* data](https://developers.google.com/workspace/chat/authorize-import).
*
* Create a request for the method "spaces.completeImport".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link CompleteImport#execute()} method to invoke the remote operation.
* {@link CompleteImport#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. Resource name of the import mode space. Format: `spaces/{space}`
* @param content the {@link com.google.api.services.chat.v1.model.CompleteImportSpaceRequest}
* @since 1.13
*/
protected CompleteImport(java.lang.String name, com.google.api.services.chat.v1.model.CompleteImportSpaceRequest content) {
super(HangoutsChat.this, "POST", REST_PATH, content, com.google.api.services.chat.v1.model.CompleteImportSpaceResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public CompleteImport set$Xgafv(java.lang.String $Xgafv) {
return (CompleteImport) super.set$Xgafv($Xgafv);
}
@Override
public CompleteImport setAccessToken(java.lang.String accessToken) {
return (CompleteImport) super.setAccessToken(accessToken);
}
@Override
public CompleteImport setAlt(java.lang.String alt) {
return (CompleteImport) super.setAlt(alt);
}
@Override
public CompleteImport setCallback(java.lang.String callback) {
return (CompleteImport) super.setCallback(callback);
}
@Override
public CompleteImport setFields(java.lang.String fields) {
return (CompleteImport) super.setFields(fields);
}
@Override
public CompleteImport setKey(java.lang.String key) {
return (CompleteImport) super.setKey(key);
}
@Override
public CompleteImport setOauthToken(java.lang.String oauthToken) {
return (CompleteImport) super.setOauthToken(oauthToken);
}
@Override
public CompleteImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CompleteImport) super.setPrettyPrint(prettyPrint);
}
@Override
public CompleteImport setQuotaUser(java.lang.String quotaUser) {
return (CompleteImport) super.setQuotaUser(quotaUser);
}
@Override
public CompleteImport setUploadType(java.lang.String uploadType) {
return (CompleteImport) super.setUploadType(uploadType);
}
@Override
public CompleteImport setUploadProtocol(java.lang.String uploadProtocol) {
return (CompleteImport) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the import mode space. Format: `spaces/{space}` */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the import mode space. Format: `spaces/{space}`
*/
public java.lang.String getName() {
return name;
}
/** Required. Resource name of the import mode space. Format: `spaces/{space}` */
public CompleteImport setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
this.name = name;
return this;
}
@Override
public CompleteImport set(String parameterName, Object value) {
return (CompleteImport) super.set(parameterName, value);
}
}
/**
* Creates a named space. Spaces grouped by topics aren't supported. For an example, see [Create a
* space](https://developers.google.com/workspace/chat/create-spaces). If you receive the error
* message `ALREADY_EXISTS` when creating a space, try a different `displayName`. An existing space
* within the Google Workspace organization might already use this display name. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "spaces.create".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.chat.v1.model.Space}
* @return the request
*/
public Create create(com.google.api.services.chat.v1.model.Space content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends HangoutsChatRequest {
private static final String REST_PATH = "v1/spaces";
/**
* Creates a named space. Spaces grouped by topics aren't supported. For an example, see [Create a
* space](https://developers.google.com/workspace/chat/create-spaces). If you receive the error
* message `ALREADY_EXISTS` when creating a space, try a different `displayName`. An existing
* space within the Google Workspace organization might already use this display name. Requires
* [user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user).
*
* Create a request for the method "spaces.create".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.chat.v1.model.Space}
* @since 1.13
*/
protected Create(com.google.api.services.chat.v1.model.Space content) {
super(HangoutsChat.this, "POST", REST_PATH, content, com.google.api.services.chat.v1.model.Space.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. A unique identifier for this request. A random UUID is recommended. Specifying an
* existing request ID returns the space created with that ID instead of creating a new space.
* Specifying an existing request ID from the same Chat app with a different authenticated
* user returns an error.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique identifier for this request. A random UUID is recommended. Specifying an
existing request ID returns the space created with that ID instead of creating a new space.
Specifying an existing request ID from the same Chat app with a different authenticated user
returns an error.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique identifier for this request. A random UUID is recommended. Specifying an
* existing request ID returns the space created with that ID instead of creating a new space.
* Specifying an existing request ID from the same Chat app with a different authenticated
* user returns an error.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a named space. Always performs a cascading delete, which means that the space's child
* resources—like messages posted in the space and memberships in the space—are also deleted. For an
* example, see [Delete a space](https://developers.google.com/workspace/chat/delete-spaces).
* Requires [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user) from a user who has permission to delete the space.
*
* Create a request for the method "spaces.delete".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the space to delete. Format: `spaces/{space}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Deletes a named space. Always performs a cascading delete, which means that the space's child
* resources—like messages posted in the space and memberships in the space—are also deleted. For
* an example, see [Delete a space](https://developers.google.com/workspace/chat/delete-spaces).
* Requires [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user) from a user who has permission to delete the space.
*
* Create a request for the method "spaces.delete".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the space to delete. Format: `spaces/{space}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(HangoutsChat.this, "DELETE", REST_PATH, null, com.google.api.services.chat.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the space to delete. Format: `spaces/{space}` */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the space to delete. Format: `spaces/{space}`
*/
public java.lang.String getName() {
return name;
}
/** Required. Resource name of the space to delete. Format: `spaces/{space}` */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
this.name = name;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.delete`
* [OAuth 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-
* api-scopes).
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.delete` [OAuth
[ 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-scopes).
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.delete`
* [OAuth 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-
* api-scopes).
*/
public Delete setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns the existing direct message with the specified user. If no direct message space is found,
* returns a `404 NOT_FOUND` error. For an example, see [Find a direct
* message](/chat/api/guides/v1/spaces/find-direct-message). With [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user),
* returns the direct message space between the specified user and the authenticated user. With [app
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-app),
* returns the direct message space between the specified user and the calling Chat app. Requires
* [user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user) or [app authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-app).
*
* Create a request for the method "spaces.findDirectMessage".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link FindDirectMessage#execute()} method to invoke the remote operation.
*
* @return the request
*/
public FindDirectMessage findDirectMessage() throws java.io.IOException {
FindDirectMessage result = new FindDirectMessage();
initialize(result);
return result;
}
public class FindDirectMessage extends HangoutsChatRequest {
private static final String REST_PATH = "v1/spaces:findDirectMessage";
/**
* Returns the existing direct message with the specified user. If no direct message space is
* found, returns a `404 NOT_FOUND` error. For an example, see [Find a direct
* message](/chat/api/guides/v1/spaces/find-direct-message). With [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user),
* returns the direct message space between the specified user and the authenticated user. With
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app), returns the direct message space between the specified user and the calling Chat app.
* Requires [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user) or [app
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
*
* Create a request for the method "spaces.findDirectMessage".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link FindDirectMessage#execute()} method to invoke the remote operation.
* {@link FindDirectMessage#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @since 1.13
*/
protected FindDirectMessage() {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.Space.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public FindDirectMessage set$Xgafv(java.lang.String $Xgafv) {
return (FindDirectMessage) super.set$Xgafv($Xgafv);
}
@Override
public FindDirectMessage setAccessToken(java.lang.String accessToken) {
return (FindDirectMessage) super.setAccessToken(accessToken);
}
@Override
public FindDirectMessage setAlt(java.lang.String alt) {
return (FindDirectMessage) super.setAlt(alt);
}
@Override
public FindDirectMessage setCallback(java.lang.String callback) {
return (FindDirectMessage) super.setCallback(callback);
}
@Override
public FindDirectMessage setFields(java.lang.String fields) {
return (FindDirectMessage) super.setFields(fields);
}
@Override
public FindDirectMessage setKey(java.lang.String key) {
return (FindDirectMessage) super.setKey(key);
}
@Override
public FindDirectMessage setOauthToken(java.lang.String oauthToken) {
return (FindDirectMessage) super.setOauthToken(oauthToken);
}
@Override
public FindDirectMessage setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FindDirectMessage) super.setPrettyPrint(prettyPrint);
}
@Override
public FindDirectMessage setQuotaUser(java.lang.String quotaUser) {
return (FindDirectMessage) super.setQuotaUser(quotaUser);
}
@Override
public FindDirectMessage setUploadType(java.lang.String uploadType) {
return (FindDirectMessage) super.setUploadType(uploadType);
}
@Override
public FindDirectMessage setUploadProtocol(java.lang.String uploadProtocol) {
return (FindDirectMessage) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the user to find direct message with. Format: `users/{user}`,
* where `{user}` is either the `id` for the
* [person](https://developers.google.com/people/api/rest/v1/people) from the People API, or
* the `id` for the [user](https://developers.google.com/admin-
* sdk/directory/reference/rest/v1/users) in the Directory API. For example, if the People API
* profile ID is `123456789`, you can find a direct message with that person by using
* `users/123456789` as the `name`. When [authenticated as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), you
* can use the email as an alias for `{user}`. For example, `users/[email protected]` where
* `[email protected]` is the email of the Google Chat user.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the user to find direct message with. Format: `users/{user}`, where
`{user}` is either the `id` for the
[person](https://developers.google.com/people/api/rest/v1/people) from the People API, or the `id`
for the [user](https://developers.google.com/admin-sdk/directory/reference/rest/v1/users) in the
Directory API. For example, if the People API profile ID is `123456789`, you can find a direct
message with that person by using `users/123456789` as the `name`. When [authenticated as a
user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), you can use
the email as an alias for `{user}`. For example, `users/[email protected]` where
`[email protected]` is the email of the Google Chat user.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the user to find direct message with. Format: `users/{user}`,
* where `{user}` is either the `id` for the
* [person](https://developers.google.com/people/api/rest/v1/people) from the People API, or
* the `id` for the [user](https://developers.google.com/admin-
* sdk/directory/reference/rest/v1/users) in the Directory API. For example, if the People API
* profile ID is `123456789`, you can find a direct message with that person by using
* `users/123456789` as the `name`. When [authenticated as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), you
* can use the email as an alias for `{user}`. For example, `users/[email protected]` where
* `[email protected]` is the email of the Google Chat user.
*/
public FindDirectMessage setName(java.lang.String name) {
this.name = name;
return this;
}
@Override
public FindDirectMessage set(String parameterName, Object value) {
return (FindDirectMessage) super.set(parameterName, value);
}
}
/**
* Returns details about a space. For an example, see [Get details about a
* space](https://developers.google.com/workspace/chat/get-spaces). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "spaces.get".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the space, in the form `spaces/{space}`. Format: `spaces/{space}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Returns details about a space. For an example, see [Get details about a
* space](https://developers.google.com/workspace/chat/get-spaces). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "spaces.get".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the space, in the form `spaces/{space}`. Format: `spaces/{space}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.Space.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the space, in the form `spaces/{space}`. Format:
* `spaces/{space}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the space, in the form `spaces/{space}`. Format: `spaces/{space}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the space, in the form `spaces/{space}`. Format:
* `spaces/{space}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
this.name = name;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.spaces`
* or `chat.admin.spaces.readonly` [OAuth 2.0
* scopes](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes).
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.spaces` or
[ `chat.admin.spaces.readonly` [OAuth 2.0
[ scopes](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-scopes).
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.spaces`
* or `chat.admin.spaces.readonly` [OAuth 2.0
* scopes](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes).
*/
public Get setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists spaces the caller is a member of. Group chats and DMs aren't listed until the first message
* is sent. For an example, see [List spaces](https://developers.google.com/workspace/chat/list-
* spaces). Requires [authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize). Supports [app
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-app) and
* [user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user). Lists spaces visible to the caller or authenticated user. Group chats and DMs aren't
* listed until the first message is sent. To list all named spaces by Google Workspace
* organization, use the [`spaces.search()`](https://developers.google.com/workspace/chat/api/refere
* nce/rest/v1/spaces/search) method using Workspace administrator privileges instead.
*
* Create a request for the method "spaces.list".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends HangoutsChatRequest {
private static final String REST_PATH = "v1/spaces";
/**
* Lists spaces the caller is a member of. Group chats and DMs aren't listed until the first
* message is sent. For an example, see [List
* spaces](https://developers.google.com/workspace/chat/list-spaces). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). Lists spaces visible to the caller or authenticated user. Group chats and
* DMs aren't listed until the first message is sent. To list all named spaces by Google Workspace
* organization, use the [`spaces.search()`](https://developers.google.com/workspace/chat/api/refe
* rence/rest/v1/spaces/search) method using Workspace administrator privileges instead.
*
* Create a request for the method "spaces.list".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.ListSpacesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. A query filter. You can filter spaces by the space type ([`space_type`](https://d
* evelopers.google.com/workspace/chat/api/reference/rest/v1/spaces#spacetype)). To filter by
* space type, you must specify valid enum value, such as `SPACE` or `GROUP_CHAT` (the
* `space_type` can't be `SPACE_TYPE_UNSPECIFIED`). To query for multiple space types, use the
* `OR` operator. For example, the following queries are valid: ``` space_type = "SPACE"
* spaceType = "GROUP_CHAT" OR spaceType = "DIRECT_MESSAGE" ``` Invalid queries are rejected
* by the server with an `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A query filter. You can filter spaces by the space type ([`space_type`](https://developer
s.google.com/workspace/chat/api/reference/rest/v1/spaces#spacetype)). To filter by space type, you
must specify valid enum value, such as `SPACE` or `GROUP_CHAT` (the `space_type` can't be
`SPACE_TYPE_UNSPECIFIED`). To query for multiple space types, use the `OR` operator. For example,
the following queries are valid: ``` space_type = "SPACE" spaceType = "GROUP_CHAT" OR spaceType =
"DIRECT_MESSAGE" ``` Invalid queries are rejected by the server with an `INVALID_ARGUMENT` error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A query filter. You can filter spaces by the space type ([`space_type`](https://d
* evelopers.google.com/workspace/chat/api/reference/rest/v1/spaces#spacetype)). To filter by
* space type, you must specify valid enum value, such as `SPACE` or `GROUP_CHAT` (the
* `space_type` can't be `SPACE_TYPE_UNSPECIFIED`). To query for multiple space types, use the
* `OR` operator. For example, the following queries are valid: ``` space_type = "SPACE"
* spaceType = "GROUP_CHAT" OR spaceType = "DIRECT_MESSAGE" ``` Invalid queries are rejected
* by the server with an `INVALID_ARGUMENT` error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of spaces to return. The service might return fewer than this
* value. If unspecified, at most 100 spaces are returned. The maximum value is 1000. If you
* use a value more than 1000, it's automatically changed to 1000. Negative values return an
* `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of spaces to return. The service might return fewer than this value.
If unspecified, at most 100 spaces are returned. The maximum value is 1000. If you use a value more
than 1000, it's automatically changed to 1000. Negative values return an `INVALID_ARGUMENT` error.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of spaces to return. The service might return fewer than this
* value. If unspecified, at most 100 spaces are returned. The maximum value is 1000. If you
* use a value more than 1000, it's automatically changed to 1000. Negative values return an
* `INVALID_ARGUMENT` error.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous list spaces call. Provide this parameter
* to retrieve the subsequent page. When paginating, the filter value should match the call
* that provided the page token. Passing a different value may lead to unexpected results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous list spaces call. Provide this parameter to
retrieve the subsequent page. When paginating, the filter value should match the call that provided
the page token. Passing a different value may lead to unexpected results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous list spaces call. Provide this parameter
* to retrieve the subsequent page. When paginating, the filter value should match the call
* that provided the page token. Passing a different value may lead to unexpected results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a space. For an example, see [Update a
* space](https://developers.google.com/workspace/chat/update-spaces). If you're updating the
* `displayName` field and receive the error message `ALREADY_EXISTS`, try a different display
* name.. An existing space within the Google Workspace organization might already use this display
* name. Requires [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "spaces.patch".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Resource name of the space. Format: `spaces/{space}` Where `{space}` represents the system-assigned
* ID for the space. You can obtain the space ID by calling the [`spaces.list()`](https://dev
* elopers.google.com/workspace/chat/api/reference/rest/v1/spaces/list) method or from the
* space URL. For example, if the space URL is
* `https://mail.google.com/mail/u/0/#chat/space/AAAAAAAAA`, the space ID is `AAAAAAAAA`.
* @param content the {@link com.google.api.services.chat.v1.model.Space}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.chat.v1.model.Space content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Updates a space. For an example, see [Update a
* space](https://developers.google.com/workspace/chat/update-spaces). If you're updating the
* `displayName` field and receive the error message `ALREADY_EXISTS`, try a different display
* name.. An existing space within the Google Workspace organization might already use this
* display name. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "spaces.patch".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name of the space. Format: `spaces/{space}` Where `{space}` represents the system-assigned
* ID for the space. You can obtain the space ID by calling the [`spaces.list()`](https://dev
* elopers.google.com/workspace/chat/api/reference/rest/v1/spaces/list) method or from the
* space URL. For example, if the space URL is
* `https://mail.google.com/mail/u/0/#chat/space/AAAAAAAAA`, the space ID is `AAAAAAAAA`.
* @param content the {@link com.google.api.services.chat.v1.model.Space}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.chat.v1.model.Space content) {
super(HangoutsChat.this, "PATCH", REST_PATH, content, com.google.api.services.chat.v1.model.Space.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name of the space. Format: `spaces/{space}` Where `{space}` represents the system-
* assigned ID for the space. You can obtain the space ID by calling the [`spaces.list()`](htt
* ps://developers.google.com/workspace/chat/api/reference/rest/v1/spaces/list) method or from
* the space URL. For example, if the space URL is
* `https://mail.google.com/mail/u/0/#chat/space/AAAAAAAAA`, the space ID is `AAAAAAAAA`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name of the space. Format: `spaces/{space}` Where `{space}` represents the system-assigned
ID for the space. You can obtain the space ID by calling the
[`spaces.list()`](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces/list)
method or from the space URL. For example, if the space URL is
`https://mail.google.com/mail/u/0/#chat/space/AAAAAAAAA`, the space ID is `AAAAAAAAA`.
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the space. Format: `spaces/{space}` Where `{space}` represents the system-
* assigned ID for the space. You can obtain the space ID by calling the [`spaces.list()`](htt
* ps://developers.google.com/workspace/chat/api/reference/rest/v1/spaces/list) method or from
* the space URL. For example, if the space URL is
* `https://mail.google.com/mail/u/0/#chat/space/AAAAAAAAA`, the space ID is `AAAAAAAAA`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The updated field paths, comma separated if there are multiple. You can update
* the following fields for a space: - `space_details` - `display_name`: Only supports
* updating the display name for spaces where `spaceType` field is `SPACE`. If you receive the
* error message `ALREADY_EXISTS`, try a different value. An existing space within the Google
* Workspace organization might already use this display name. - `space_type`: Only supports
* changing a `GROUP_CHAT` space type to `SPACE`. Include `display_name` together with
* `space_type` in the update mask and ensure that the specified space has a non-empty display
* name and the `SPACE` space type. Including the `space_type` mask and the `SPACE` type in
* the specified space when updating the display name is optional if the existing space
* already has the `SPACE` type. Trying to update the space type in other ways results in an
* invalid argument error. `space_type` is not supported with admin access. -
* `space_history_state`: Updates [space history
* settings](https://support.google.com/chat/answer/7664687) by turning history on or off for
* the space. Only supported if history settings are enabled for the Google Workspace
* organization. To update the space history state, you must omit all other field masks in
* your request. `space_history_state` is not supported with admin access. -
* `access_settings.audience`: Updates the [access
* setting](https://support.google.com/chat/answer/11971020) of who can discover the space,
* join the space, and preview the messages in named space where `spaceType` field is `SPACE`.
* If the existing space has a target audience, you can remove the audience and restrict space
* access by omitting a value for this field mask. To update access settings for a space, the
* authenticating user must be a space manager and omit all other field masks in your request.
* You can't update this field if the space is in [import
* mode](https://developers.google.com/workspace/chat/import-data-overview). To learn more,
* see [Make a space discoverable to specific
* users](https://developers.google.com/workspace/chat/space-target-audience).
* `access_settings.audience` is not supported with admin access. - Developer Preview:
* Supports changing the [permission
* settings](https://support.google.com/chat/answer/13340792) of a space, supported field
* paths include: `permission_settings.manage_members_and_groups`,
* `permission_settings.modify_space_details`, `permission_settings.toggle_history`,
* `permission_settings.use_at_mention_all`, `permission_settings.manage_apps`,
* `permission_settings.manage_webhooks`, `permission_settings.reply_messages` (Warning:
* mutually exclusive with all other non-permission settings field paths).
* `permission_settings` is not supported with admin access.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The updated field paths, comma separated if there are multiple. You can update the
following fields for a space: - `space_details` - `display_name`: Only supports updating the
display name for spaces where `spaceType` field is `SPACE`. If you receive the error message
`ALREADY_EXISTS`, try a different value. An existing space within the Google Workspace organization
might already use this display name. - `space_type`: Only supports changing a `GROUP_CHAT` space
type to `SPACE`. Include `display_name` together with `space_type` in the update mask and ensure
that the specified space has a non-empty display name and the `SPACE` space type. Including the
`space_type` mask and the `SPACE` type in the specified space when updating the display name is
optional if the existing space already has the `SPACE` type. Trying to update the space type in
other ways results in an invalid argument error. `space_type` is not supported with admin access. -
`space_history_state`: Updates [space history
settings](https://support.google.com/chat/answer/7664687) by turning history on or off for the
space. Only supported if history settings are enabled for the Google Workspace organization. To
update the space history state, you must omit all other field masks in your request.
`space_history_state` is not supported with admin access. - `access_settings.audience`: Updates the
[access setting](https://support.google.com/chat/answer/11971020) of who can discover the space,
join the space, and preview the messages in named space where `spaceType` field is `SPACE`. If the
existing space has a target audience, you can remove the audience and restrict space access by
omitting a value for this field mask. To update access settings for a space, the authenticating
user must be a space manager and omit all other field masks in your request. You can't update this
field if the space is in [import mode](https://developers.google.com/workspace/chat/import-data-
overview). To learn more, see [Make a space discoverable to specific
users](https://developers.google.com/workspace/chat/space-target-audience).
`access_settings.audience` is not supported with admin access. - Developer Preview: Supports
changing the [permission settings](https://support.google.com/chat/answer/13340792) of a space,
supported field paths include: `permission_settings.manage_members_and_groups`,
`permission_settings.modify_space_details`, `permission_settings.toggle_history`,
`permission_settings.use_at_mention_all`, `permission_settings.manage_apps`,
`permission_settings.manage_webhooks`, `permission_settings.reply_messages` (Warning: mutually
exclusive with all other non-permission settings field paths). `permission_settings` is not
supported with admin access.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The updated field paths, comma separated if there are multiple. You can update
* the following fields for a space: - `space_details` - `display_name`: Only supports
* updating the display name for spaces where `spaceType` field is `SPACE`. If you receive the
* error message `ALREADY_EXISTS`, try a different value. An existing space within the Google
* Workspace organization might already use this display name. - `space_type`: Only supports
* changing a `GROUP_CHAT` space type to `SPACE`. Include `display_name` together with
* `space_type` in the update mask and ensure that the specified space has a non-empty display
* name and the `SPACE` space type. Including the `space_type` mask and the `SPACE` type in
* the specified space when updating the display name is optional if the existing space
* already has the `SPACE` type. Trying to update the space type in other ways results in an
* invalid argument error. `space_type` is not supported with admin access. -
* `space_history_state`: Updates [space history
* settings](https://support.google.com/chat/answer/7664687) by turning history on or off for
* the space. Only supported if history settings are enabled for the Google Workspace
* organization. To update the space history state, you must omit all other field masks in
* your request. `space_history_state` is not supported with admin access. -
* `access_settings.audience`: Updates the [access
* setting](https://support.google.com/chat/answer/11971020) of who can discover the space,
* join the space, and preview the messages in named space where `spaceType` field is `SPACE`.
* If the existing space has a target audience, you can remove the audience and restrict space
* access by omitting a value for this field mask. To update access settings for a space, the
* authenticating user must be a space manager and omit all other field masks in your request.
* You can't update this field if the space is in [import
* mode](https://developers.google.com/workspace/chat/import-data-overview). To learn more,
* see [Make a space discoverable to specific
* users](https://developers.google.com/workspace/chat/space-target-audience).
* `access_settings.audience` is not supported with admin access. - Developer Preview:
* Supports changing the [permission
* settings](https://support.google.com/chat/answer/13340792) of a space, supported field
* paths include: `permission_settings.manage_members_and_groups`,
* `permission_settings.modify_space_details`, `permission_settings.toggle_history`,
* `permission_settings.use_at_mention_all`, `permission_settings.manage_apps`,
* `permission_settings.manage_webhooks`, `permission_settings.reply_messages` (Warning:
* mutually exclusive with all other non-permission settings field paths).
* `permission_settings` is not supported with admin access.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.spaces`
* [OAuth 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-
* api-scopes). Some `FieldMask` values are not supported using admin access. For details, see
* the description of `update_mask`.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.spaces` [OAuth
[ 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-scopes).
[ Some `FieldMask` values are not supported using admin access. For details, see the description of
[ `update_mask`.
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.spaces`
* [OAuth 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-
* api-scopes). Some `FieldMask` values are not supported using admin access. For details, see
* the description of `update_mask`.
*/
public Patch setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). Returns a list of spaces
* based on a user's search. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* The user must be an administrator for the Google Workspace organization. In the request, set
* `use_admin_access` to `true`.
*
* Create a request for the method "spaces.search".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Search search() throws java.io.IOException {
Search result = new Search();
initialize(result);
return result;
}
public class Search extends HangoutsChatRequest {
private static final String REST_PATH = "v1/spaces:search";
/**
* [Developer Preview](https://developers.google.com/workspace/preview). Returns a list of spaces
* based on a user's search. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* The user must be an administrator for the Google Workspace organization. In the request, set
* `use_admin_access` to `true`.
*
* Create a request for the method "spaces.search".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation. {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Search() {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.SearchSpacesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. How the list of spaces is ordered. Supported attributes to order by are: -
* `membership_count.joined_direct_human_user_count` — Denotes the count of human users that
* have directly joined a space. - `last_active_time` — Denotes the time when last eligible
* item is added to any topic of this space. - `create_time` — Denotes the time of the space
* creation. Valid ordering operation values are: - `ASC` for ascending. Default value. -
* `DESC` for descending. The supported syntax are: -
* `membership_count.joined_direct_human_user_count DESC` -
* `membership_count.joined_direct_human_user_count ASC` - `last_active_time DESC` -
* `last_active_time ASC` - `create_time DESC` - `create_time ASC`
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. How the list of spaces is ordered. Supported attributes to order by are: -
`membership_count.joined_direct_human_user_count` — Denotes the count of human users that have
directly joined a space. - `last_active_time` — Denotes the time when last eligible item is added
to any topic of this space. - `create_time` — Denotes the time of the space creation. Valid
ordering operation values are: - `ASC` for ascending. Default value. - `DESC` for descending. The
supported syntax are: - `membership_count.joined_direct_human_user_count DESC` -
`membership_count.joined_direct_human_user_count ASC` - `last_active_time DESC` - `last_active_time
ASC` - `create_time DESC` - `create_time ASC`
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. How the list of spaces is ordered. Supported attributes to order by are: -
* `membership_count.joined_direct_human_user_count` — Denotes the count of human users that
* have directly joined a space. - `last_active_time` — Denotes the time when last eligible
* item is added to any topic of this space. - `create_time` — Denotes the time of the space
* creation. Valid ordering operation values are: - `ASC` for ascending. Default value. -
* `DESC` for descending. The supported syntax are: -
* `membership_count.joined_direct_human_user_count DESC` -
* `membership_count.joined_direct_human_user_count ASC` - `last_active_time DESC` -
* `last_active_time ASC` - `create_time DESC` - `create_time ASC`
*/
public Search setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of spaces to return. The service may return fewer than this value. If
* unspecified, at most 100 spaces are returned. The maximum value is 1000. If you use a value
* more than 1000, it's automatically changed to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of spaces to return. The service may return fewer than this value. If
unspecified, at most 100 spaces are returned. The maximum value is 1000. If you use a value more
than 1000, it's automatically changed to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of spaces to return. The service may return fewer than this value. If
* unspecified, at most 100 spaces are returned. The maximum value is 1000. If you use a value
* more than 1000, it's automatically changed to 1000.
*/
public Search setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token, received from the previous search spaces call. Provide this parameter to retrieve
* the subsequent page. When paginating, all other parameters provided should match the call
* that provided the page token. Passing different values to the other parameters might lead
* to unexpected results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token, received from the previous search spaces call. Provide this parameter to retrieve the
subsequent page. When paginating, all other parameters provided should match the call that provided
the page token. Passing different values to the other parameters might lead to unexpected results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token, received from the previous search spaces call. Provide this parameter to retrieve
* the subsequent page. When paginating, all other parameters provided should match the call
* that provided the page token. Passing different values to the other parameters might lead
* to unexpected results.
*/
public Search setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. A search query. You can search by using the following parameters: - `create_time`
* - `customer` - `display_name` - `external_user_allowed` - `last_active_time` -
* `space_history_state` - `space_type` `create_time` and `last_active_time` accept a
* timestamp in [RFC-3339](https://www.rfc-editor.org/rfc/rfc3339) format and the supported
* comparison operators are: `=`, `<`, `>`, `<=`, `>=`. `customer` is required and is used to
* indicate which customer to fetch spaces from. `customers/my_customer` is the only supported
* value. `display_name` only accepts the `HAS` (`:`) operator. The text to match is first
* tokenized into tokens and each token is prefix-matched case-insensitively and independently
* as a substring anywhere in the space's `display_name`. For example, `Fun Eve` matches `Fun
* event` or `The evening was fun`, but not `notFun event` or `even`. `external_user_allowed`
* accepts either `true` or `false`. `space_history_state` only accepts values from the
* [`historyState`] (https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces
* #Space.HistoryState) field of a `space` resource. `space_type` is required and the only
* valid value is `SPACE`. Across different fields, only `AND` operators are supported. A
* valid example is `space_type = "SPACE" AND display_name:"Hello"` and an invalid example is
* `space_type = "SPACE" OR display_name:"Hello"`. Among the same field, `space_type` doesn't
* support `AND` or `OR` operators. `display_name`, 'space_history_state', and
* 'external_user_allowed' only support `OR` operators. `last_active_time` and `create_time`
* support both `AND` and `OR` operators. `AND` can only be used to represent an interval,
* such as `last_active_time < "2022-01-01T00:00:00+00:00" AND last_active_time >
* "2023-01-01T00:00:00+00:00"`. The following example queries are valid: ``` customer =
* "customers/my_customer" AND space_type = "SPACE" customer = "customers/my_customer" AND
* space_type = "SPACE" AND display_name:"Hello World" customer = "customers/my_customer" AND
* space_type = "SPACE" AND (last_active_time < "2020-01-01T00:00:00+00:00" OR
* last_active_time > "2022-01-01T00:00:00+00:00") customer = "customers/my_customer" AND
* space_type = "SPACE" AND (display_name:"Hello World" OR display_name:"Fun event") AND
* (last_active_time > "2020-01-01T00:00:00+00:00" AND last_active_time <
* "2022-01-01T00:00:00+00:00") customer = "customers/my_customer" AND space_type = "SPACE"
* AND (create_time > "2019-01-01T00:00:00+00:00" AND create_time <
* "2020-01-01T00:00:00+00:00") AND (external_user_allowed = "true") AND (space_history_state
* = "HISTORY_ON" OR space_history_state = "HISTORY_OFF") ```
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. A search query. You can search by using the following parameters: - `create_time` -
`customer` - `display_name` - `external_user_allowed` - `last_active_time` - `space_history_state`
- `space_type` `create_time` and `last_active_time` accept a timestamp in
[RFC-3339](https://www.rfc-editor.org/rfc/rfc3339) format and the supported comparison operators
are: `=`, `<`, `>`, `<=`, `>=`. `customer` is required and is used to indicate which customer to
fetch spaces from. `customers/my_customer` is the only supported value. `display_name` only accepts
the `HAS` (`:`) operator. The text to match is first tokenized into tokens and each token is
prefix-matched case-insensitively and independently as a substring anywhere in the space's
`display_name`. For example, `Fun Eve` matches `Fun event` or `The evening was fun`, but not
`notFun event` or `even`. `external_user_allowed` accepts either `true` or `false`.
`space_history_state` only accepts values from the [`historyState`]
(https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces#Space.HistoryState)
field of a `space` resource. `space_type` is required and the only valid value is `SPACE`. Across
different fields, only `AND` operators are supported. A valid example is `space_type = "SPACE" AND
display_name:"Hello"` and an invalid example is `space_type = "SPACE" OR display_name:"Hello"`.
Among the same field, `space_type` doesn't support `AND` or `OR` operators. `display_name`,
'space_history_state', and 'external_user_allowed' only support `OR` operators. `last_active_time`
and `create_time` support both `AND` and `OR` operators. `AND` can only be used to represent an
interval, such as `last_active_time < "2022-01-01T00:00:00+00:00" AND last_active_time >
"2023-01-01T00:00:00+00:00"`. The following example queries are valid: ``` customer =
"customers/my_customer" AND space_type = "SPACE" customer = "customers/my_customer" AND space_type
= "SPACE" AND display_name:"Hello World" customer = "customers/my_customer" AND space_type =
"SPACE" AND (last_active_time < "2020-01-01T00:00:00+00:00" OR last_active_time >
"2022-01-01T00:00:00+00:00") customer = "customers/my_customer" AND space_type = "SPACE" AND
(display_name:"Hello World" OR display_name:"Fun event") AND (last_active_time >
"2020-01-01T00:00:00+00:00" AND last_active_time < "2022-01-01T00:00:00+00:00") customer =
"customers/my_customer" AND space_type = "SPACE" AND (create_time > "2019-01-01T00:00:00+00:00" AND
create_time < "2020-01-01T00:00:00+00:00") AND (external_user_allowed = "true") AND
(space_history_state = "HISTORY_ON" OR space_history_state = "HISTORY_OFF") ```
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. A search query. You can search by using the following parameters: - `create_time`
* - `customer` - `display_name` - `external_user_allowed` - `last_active_time` -
* `space_history_state` - `space_type` `create_time` and `last_active_time` accept a
* timestamp in [RFC-3339](https://www.rfc-editor.org/rfc/rfc3339) format and the supported
* comparison operators are: `=`, `<`, `>`, `<=`, `>=`. `customer` is required and is used to
* indicate which customer to fetch spaces from. `customers/my_customer` is the only supported
* value. `display_name` only accepts the `HAS` (`:`) operator. The text to match is first
* tokenized into tokens and each token is prefix-matched case-insensitively and independently
* as a substring anywhere in the space's `display_name`. For example, `Fun Eve` matches `Fun
* event` or `The evening was fun`, but not `notFun event` or `even`. `external_user_allowed`
* accepts either `true` or `false`. `space_history_state` only accepts values from the
* [`historyState`] (https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces
* #Space.HistoryState) field of a `space` resource. `space_type` is required and the only
* valid value is `SPACE`. Across different fields, only `AND` operators are supported. A
* valid example is `space_type = "SPACE" AND display_name:"Hello"` and an invalid example is
* `space_type = "SPACE" OR display_name:"Hello"`. Among the same field, `space_type` doesn't
* support `AND` or `OR` operators. `display_name`, 'space_history_state', and
* 'external_user_allowed' only support `OR` operators. `last_active_time` and `create_time`
* support both `AND` and `OR` operators. `AND` can only be used to represent an interval,
* such as `last_active_time < "2022-01-01T00:00:00+00:00" AND last_active_time >
* "2023-01-01T00:00:00+00:00"`. The following example queries are valid: ``` customer =
* "customers/my_customer" AND space_type = "SPACE" customer = "customers/my_customer" AND
* space_type = "SPACE" AND display_name:"Hello World" customer = "customers/my_customer" AND
* space_type = "SPACE" AND (last_active_time < "2020-01-01T00:00:00+00:00" OR
* last_active_time > "2022-01-01T00:00:00+00:00") customer = "customers/my_customer" AND
* space_type = "SPACE" AND (display_name:"Hello World" OR display_name:"Fun event") AND
* (last_active_time > "2020-01-01T00:00:00+00:00" AND last_active_time <
* "2022-01-01T00:00:00+00:00") customer = "customers/my_customer" AND space_type = "SPACE"
* AND (create_time > "2019-01-01T00:00:00+00:00" AND create_time <
* "2020-01-01T00:00:00+00:00") AND (external_user_allowed = "true") AND (space_history_state
* = "HISTORY_ON" OR space_history_state = "HISTORY_OFF") ```
*/
public Search setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* When `true`, the method runs using the user's Google Workspace administrator privileges.
* The calling user must be a Google Workspace administrator with the [manage chat and spaces
* conversations privilege](https://support.google.com/a/answer/13369245). Requires either the
* `chat.admin.spaces.readonly` or `chat.admin.spaces` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). This method currently only supports admin access, thus only `true` is accepted for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/** When `true`, the method runs using the user's Google Workspace administrator privileges. The
calling user must be a Google Workspace administrator with the [manage chat and spaces
conversations privilege](https://support.google.com/a/answer/13369245). Requires either the
`chat.admin.spaces.readonly` or `chat.admin.spaces` [OAuth 2.0
scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-scopes). This
method currently only supports admin access, thus only `true` is accepted for this field.
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* When `true`, the method runs using the user's Google Workspace administrator privileges.
* The calling user must be a Google Workspace administrator with the [manage chat and spaces
* conversations privilege](https://support.google.com/a/answer/13369245). Requires either the
* `chat.admin.spaces.readonly` or `chat.admin.spaces` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). This method currently only supports admin access, thus only `true` is accepted for
* this field.
*/
public Search setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Creates a space and adds specified users to it. The calling user is automatically added to the
* space, and shouldn't be specified as a membership in the request. For an example, see [Set up a
* space with initial members](https://developers.google.com/workspace/chat/set-up-spaces). To
* specify the human members to add, add memberships with the appropriate `membership.member.name`.
* To add a human user, use `users/{user}`, where `{user}` can be the email address for the user.
* For users in the same Workspace organization `{user}` can also be the `id` for the person from
* the People API, or the `id` for the user in the Directory API. For example, if the People API
* Person profile ID for `[email protected]` is `123456789`, you can add the user to the space by
* setting the `membership.member.name` to `users/[email protected]` or `users/123456789`. To specify
* the Google groups to add, add memberships with the appropriate `membership.group_member.name`. To
* add or invite a Google group, use `groups/{group}`, where `{group}` is the `id` for the group
* from the Cloud Identity Groups API. For example, you can use [Cloud Identity Groups lookup
* API](https://cloud.google.com/identity/docs/reference/rest/v1/groups/lookup) to retrieve the ID
* `123456789` for group email `[email protected]`, then you can add the group to the space by
* setting the `membership.group_member.name` to `groups/123456789`. Group email is not supported,
* and Google groups can only be added as members in named spaces. For a named space or group chat,
* if the caller blocks, or is blocked by some members, or doesn't have permission to add some
* members, then those members aren't added to the created space. To create a direct message (DM)
* between the calling user and another human user, specify exactly one membership to represent the
* human user. If one user blocks the other, the request fails and the DM isn't created. To create a
* DM between the calling user and the calling app, set `Space.singleUserBotDm` to `true` and don't
* specify any memberships. You can only use this method to set up a DM with the calling app. To add
* the calling app as a member of a space or an existing DM between two human users, see [Invite or
* add a user or app to a space](https://developers.google.com/workspace/chat/create-members). If a
* DM already exists between two users, even when one user blocks the other at the time a request is
* made, then the existing DM is returned. Spaces with threaded replies aren't supported. If you
* receive the error message `ALREADY_EXISTS` when setting up a space, try a different
* `displayName`. An existing space within the Google Workspace organization might already use this
* display name. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "spaces.setup".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Setup#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.chat.v1.model.SetUpSpaceRequest}
* @return the request
*/
public Setup setup(com.google.api.services.chat.v1.model.SetUpSpaceRequest content) throws java.io.IOException {
Setup result = new Setup(content);
initialize(result);
return result;
}
public class Setup extends HangoutsChatRequest {
private static final String REST_PATH = "v1/spaces:setup";
/**
* Creates a space and adds specified users to it. The calling user is automatically added to the
* space, and shouldn't be specified as a membership in the request. For an example, see [Set up a
* space with initial members](https://developers.google.com/workspace/chat/set-up-spaces). To
* specify the human members to add, add memberships with the appropriate
* `membership.member.name`. To add a human user, use `users/{user}`, where `{user}` can be the
* email address for the user. For users in the same Workspace organization `{user}` can also be
* the `id` for the person from the People API, or the `id` for the user in the Directory API. For
* example, if the People API Person profile ID for `[email protected]` is `123456789`, you can add
* the user to the space by setting the `membership.member.name` to `users/[email protected]` or
* `users/123456789`. To specify the Google groups to add, add memberships with the appropriate
* `membership.group_member.name`. To add or invite a Google group, use `groups/{group}`, where
* `{group}` is the `id` for the group from the Cloud Identity Groups API. For example, you can
* use [Cloud Identity Groups lookup
* API](https://cloud.google.com/identity/docs/reference/rest/v1/groups/lookup) to retrieve the ID
* `123456789` for group email `[email protected]`, then you can add the group to the space by
* setting the `membership.group_member.name` to `groups/123456789`. Group email is not supported,
* and Google groups can only be added as members in named spaces. For a named space or group
* chat, if the caller blocks, or is blocked by some members, or doesn't have permission to add
* some members, then those members aren't added to the created space. To create a direct message
* (DM) between the calling user and another human user, specify exactly one membership to
* represent the human user. If one user blocks the other, the request fails and the DM isn't
* created. To create a DM between the calling user and the calling app, set
* `Space.singleUserBotDm` to `true` and don't specify any memberships. You can only use this
* method to set up a DM with the calling app. To add the calling app as a member of a space or an
* existing DM between two human users, see [Invite or add a user or app to a
* space](https://developers.google.com/workspace/chat/create-members). If a DM already exists
* between two users, even when one user blocks the other at the time a request is made, then the
* existing DM is returned. Spaces with threaded replies aren't supported. If you receive the
* error message `ALREADY_EXISTS` when setting up a space, try a different `displayName`. An
* existing space within the Google Workspace organization might already use this display name.
* Requires [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "spaces.setup".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Setup#execute()} method to invoke the remote operation. {@link
* Setup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.chat.v1.model.SetUpSpaceRequest}
* @since 1.13
*/
protected Setup(com.google.api.services.chat.v1.model.SetUpSpaceRequest content) {
super(HangoutsChat.this, "POST", REST_PATH, content, com.google.api.services.chat.v1.model.Space.class);
}
@Override
public Setup set$Xgafv(java.lang.String $Xgafv) {
return (Setup) super.set$Xgafv($Xgafv);
}
@Override
public Setup setAccessToken(java.lang.String accessToken) {
return (Setup) super.setAccessToken(accessToken);
}
@Override
public Setup setAlt(java.lang.String alt) {
return (Setup) super.setAlt(alt);
}
@Override
public Setup setCallback(java.lang.String callback) {
return (Setup) super.setCallback(callback);
}
@Override
public Setup setFields(java.lang.String fields) {
return (Setup) super.setFields(fields);
}
@Override
public Setup setKey(java.lang.String key) {
return (Setup) super.setKey(key);
}
@Override
public Setup setOauthToken(java.lang.String oauthToken) {
return (Setup) super.setOauthToken(oauthToken);
}
@Override
public Setup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Setup) super.setPrettyPrint(prettyPrint);
}
@Override
public Setup setQuotaUser(java.lang.String quotaUser) {
return (Setup) super.setQuotaUser(quotaUser);
}
@Override
public Setup setUploadType(java.lang.String uploadType) {
return (Setup) super.setUploadType(uploadType);
}
@Override
public Setup setUploadProtocol(java.lang.String uploadProtocol) {
return (Setup) super.setUploadProtocol(uploadProtocol);
}
@Override
public Setup set(String parameterName, Object value) {
return (Setup) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Members collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Members.List request = chat.members().list(parameters ...)}
*
*
* @return the resource collection
*/
public Members members() {
return new Members();
}
/**
* The "members" collection of methods.
*/
public class Members {
/**
* Creates a membership for the calling Chat app, a user, or a Google Group. Creating memberships
* for other Chat apps isn't supported. When creating a membership, if the specified member has
* their auto-accept policy turned off, then they're invited, and must accept the space invitation
* before joining. Otherwise, creating a membership adds the member directly to the specified space.
* Requires [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). For example usage, see: - [Invite or add a user to a
* space](https://developers.google.com/workspace/chat/create-members#create-user-membership). -
* [Invite or add a Google Group to a space](https://developers.google.com/workspace/chat/create-
* members#create-group-membership). - [Add the Chat app to a
* space](https://developers.google.com/workspace/chat/create-members#create-membership-calling-
* api).
*
* Create a request for the method "members.create".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the space for which to create the membership. Format: spaces/{space}
* @param content the {@link com.google.api.services.chat.v1.model.Membership}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.chat.v1.model.Membership content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/members";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Creates a membership for the calling Chat app, a user, or a Google Group. Creating memberships
* for other Chat apps isn't supported. When creating a membership, if the specified member has
* their auto-accept policy turned off, then they're invited, and must accept the space invitation
* before joining. Otherwise, creating a membership adds the member directly to the specified
* space. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* For example usage, see: - [Invite or add a user to a
* space](https://developers.google.com/workspace/chat/create-members#create-user-membership). -
* [Invite or add a Google Group to a space](https://developers.google.com/workspace/chat/create-
* members#create-group-membership). - [Add the Chat app to a
* space](https://developers.google.com/workspace/chat/create-members#create-membership-calling-
* api).
*
* Create a request for the method "members.create".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the space for which to create the membership. Format: spaces/{space}
* @param content the {@link com.google.api.services.chat.v1.model.Membership}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.chat.v1.model.Membership content) {
super(HangoutsChat.this, "POST", REST_PATH, content, com.google.api.services.chat.v1.model.Membership.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the space for which to create the membership. Format:
* spaces/{space}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the space for which to create the membership. Format: spaces/{space}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the space for which to create the membership. Format:
* spaces/{space}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Creating app memberships or creating memberships for users outside the
* administrator's Google Workspace organization isn't supported using admin access.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.memberships`
[ [OAuth 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
[ scopes). Creating app memberships or creating memberships for users outside the administrator's
[ Google Workspace organization isn't supported using admin access.
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Creating app memberships or creating memberships for users outside the
* administrator's Google Workspace organization isn't supported using admin access.
*/
public Create setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a membership. For an example, see [Remove a user or a Google Chat app from a
* space](https://developers.google.com/workspace/chat/delete-members). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "members.delete".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the membership to delete. Chat apps can delete human users' or their own
* memberships. Chat apps can't delete other apps' memberships. When deleting a human
* membership, requires the `chat.memberships` scope and `spaces/{space}/members/{member}`
* format. You can use the email as an alias for `{member}`. For example,
* `spaces/{space}/members/[email protected]` where `[email protected]` is the email of the
* Google Chat user. When deleting an app membership, requires the `chat.memberships.app`
* scope and `spaces/{space}/members/app` format. Format: `spaces/{space}/members/{member}`
* or `spaces/{space}/members/app`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/members/[^/]+$");
/**
* Deletes a membership. For an example, see [Remove a user or a Google Chat app from a
* space](https://developers.google.com/workspace/chat/delete-members). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "members.delete".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the membership to delete. Chat apps can delete human users' or their own
* memberships. Chat apps can't delete other apps' memberships. When deleting a human
* membership, requires the `chat.memberships` scope and `spaces/{space}/members/{member}`
* format. You can use the email as an alias for `{member}`. For example,
* `spaces/{space}/members/[email protected]` where `[email protected]` is the email of the
* Google Chat user. When deleting an app membership, requires the `chat.memberships.app`
* scope and `spaces/{space}/members/app` format. Format: `spaces/{space}/members/{member}`
* or `spaces/{space}/members/app`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(HangoutsChat.this, "DELETE", REST_PATH, null, com.google.api.services.chat.v1.model.Membership.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/members/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the membership to delete. Chat apps can delete human users' or
* their own memberships. Chat apps can't delete other apps' memberships. When deleting a
* human membership, requires the `chat.memberships` scope and
* `spaces/{space}/members/{member}` format. You can use the email as an alias for
* `{member}`. For example, `spaces/{space}/members/[email protected]` where
* `[email protected]` is the email of the Google Chat user. When deleting an app
* membership, requires the `chat.memberships.app` scope and `spaces/{space}/members/app`
* format. Format: `spaces/{space}/members/{member}` or `spaces/{space}/members/app`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the membership to delete. Chat apps can delete human users' or their own
memberships. Chat apps can't delete other apps' memberships. When deleting a human membership,
requires the `chat.memberships` scope and `spaces/{space}/members/{member}` format. You can use the
email as an alias for `{member}`. For example, `spaces/{space}/members/[email protected]` where
`[email protected]` is the email of the Google Chat user. When deleting an app membership, requires
the `chat.memberships.app` scope and `spaces/{space}/members/app` format. Format:
`spaces/{space}/members/{member}` or `spaces/{space}/members/app`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the membership to delete. Chat apps can delete human users' or
* their own memberships. Chat apps can't delete other apps' memberships. When deleting a
* human membership, requires the `chat.memberships` scope and
* `spaces/{space}/members/{member}` format. You can use the email as an alias for
* `{member}`. For example, `spaces/{space}/members/[email protected]` where
* `[email protected]` is the email of the Google Chat user. When deleting an app
* membership, requires the `chat.memberships.app` scope and `spaces/{space}/members/app`
* format. Format: `spaces/{space}/members/{member}` or `spaces/{space}/members/app`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/members/[^/]+$");
}
this.name = name;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Deleting app memberships in a space isn't supported using admin access.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.memberships`
[ [OAuth 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
[ scopes). Deleting app memberships in a space isn't supported using admin access.
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Deleting app memberships in a space isn't supported using admin access.
*/
public Delete setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns details about a membership. For an example, see [Get details about a user's or Google
* Chat app's membership](https://developers.google.com/workspace/chat/get-members). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "members.get".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the membership to retrieve. To get the app's own membership [by using
* user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-
* chat-user), you can optionally use `spaces/{space}/members/app`. Format:
* `spaces/{space}/members/{member}` or `spaces/{space}/members/app` When [authenticated as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), you
* can use the user's email as an alias for `{member}`. For example,
* `spaces/{space}/members/[email protected]` where `[email protected]` is the email of the
* Google Chat user.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/members/[^/]+$");
/**
* Returns details about a membership. For an example, see [Get details about a user's or Google
* Chat app's membership](https://developers.google.com/workspace/chat/get-members). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "members.get".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the membership to retrieve. To get the app's own membership [by using
* user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-
* chat-user), you can optionally use `spaces/{space}/members/app`. Format:
* `spaces/{space}/members/{member}` or `spaces/{space}/members/app` When [authenticated as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), you
* can use the user's email as an alias for `{member}`. For example,
* `spaces/{space}/members/[email protected]` where `[email protected]` is the email of the
* Google Chat user.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.Membership.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/members/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the membership to retrieve. To get the app's own membership
* [by using user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user), you can optionally use `spaces/{space}/members/app`. Format:
* `spaces/{space}/members/{member}` or `spaces/{space}/members/app` When [authenticated as
* a user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user),
* you can use the user's email as an alias for `{member}`. For example,
* `spaces/{space}/members/[email protected]` where `[email protected]` is the email of the
* Google Chat user.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the membership to retrieve. To get the app's own membership [by using
user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
user), you can optionally use `spaces/{space}/members/app`. Format:
`spaces/{space}/members/{member}` or `spaces/{space}/members/app` When [authenticated as a
user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), you can use
the user's email as an alias for `{member}`. For example,
`spaces/{space}/members/[email protected]` where `[email protected]` is the email of the Google
Chat user.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the membership to retrieve. To get the app's own membership
* [by using user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user), you can optionally use `spaces/{space}/members/app`. Format:
* `spaces/{space}/members/{member}` or `spaces/{space}/members/app` When [authenticated as
* a user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user),
* you can use the user's email as an alias for `{member}`. For example,
* `spaces/{space}/members/[email protected]` where `[email protected]` is the email of the
* Google Chat user.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/members/[^/]+$");
}
this.name = name;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` or `chat.admin.memberships.readonly` [OAuth 2.0
* scopes](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Getting app memberships in a space isn't supported when using admin access.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.memberships` or
[ `chat.admin.memberships.readonly` [OAuth 2.0
[ scopes](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-scopes).
[ Getting app memberships in a space isn't supported when using admin access.
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` or `chat.admin.memberships.readonly` [OAuth 2.0
* scopes](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Getting app memberships in a space isn't supported when using admin access.
*/
public Get setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists memberships in a space. For an example, see [List users and Google Chat apps in a
* space](https://developers.google.com/workspace/chat/list-members). Listing memberships with [app
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-app)
* lists memberships in spaces that the Chat app has access to, but excludes Chat app memberships,
* including its own. Listing memberships with [User
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user)
* lists memberships in spaces that the authenticated user has access to. Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "members.list".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the space for which to fetch a membership list. Format:
* spaces/{space}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/members";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Lists memberships in a space. For an example, see [List users and Google Chat apps in a
* space](https://developers.google.com/workspace/chat/list-members). Listing memberships with
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) lists memberships in spaces that the Chat app has access to, but excludes Chat app
* memberships, including its own. Listing memberships with [User
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user)
* lists memberships in spaces that the authenticated user has access to. Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "members.list".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the space for which to fetch a membership list. Format:
* spaces/{space}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.ListMembershipsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the space for which to fetch a membership list. Format:
* spaces/{space}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the space for which to fetch a membership list. Format:
spaces/{space}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the space for which to fetch a membership list. Format:
* spaces/{space}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A query filter. You can filter memberships by a member's role ([`role`](https:/
* /developers.google.com/workspace/chat/api/reference/rest/v1/spaces.members#membershiprole
* )) and type ([`member.type`](https://developers.google.com/workspace/chat/api/reference/r
* est/v1/User#type)). To filter by role, set `role` to `ROLE_MEMBER` or `ROLE_MANAGER`. To
* filter by type, set `member.type` to `HUMAN` or `BOT`. Developer Preview: You can also
* filter for `member.type` using the `!=` operator. To filter by both role and type, use
* the `AND` operator. To filter by either role or type, use the `OR` operator. Either
* `member.type = "HUMAN"` or `member.type != "BOT"` is required when `use_admin_access` is
* set to true. Other member type filters will be rejected. For example, the following
* queries are valid: ``` role = "ROLE_MANAGER" OR role = "ROLE_MEMBER" member.type =
* "HUMAN" AND role = "ROLE_MANAGER" member.type != "BOT" ``` The following queries are
* invalid: ``` member.type = "HUMAN" AND member.type = "BOT" role = "ROLE_MANAGER" AND role
* = "ROLE_MEMBER" ``` Invalid queries are rejected by the server with an `INVALID_ARGUMENT`
* error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A query filter. You can filter memberships by a member's role ([`role`](https://developer
s.google.com/workspace/chat/api/reference/rest/v1/spaces.members#membershiprole)) and type
([`member.type`](https://developers.google.com/workspace/chat/api/reference/rest/v1/User#type)). To
filter by role, set `role` to `ROLE_MEMBER` or `ROLE_MANAGER`. To filter by type, set `member.type`
to `HUMAN` or `BOT`. Developer Preview: You can also filter for `member.type` using the `!=`
operator. To filter by both role and type, use the `AND` operator. To filter by either role or
type, use the `OR` operator. Either `member.type = "HUMAN"` or `member.type != "BOT"` is required
when `use_admin_access` is set to true. Other member type filters will be rejected. For example,
the following queries are valid: ``` role = "ROLE_MANAGER" OR role = "ROLE_MEMBER" member.type =
"HUMAN" AND role = "ROLE_MANAGER" member.type != "BOT" ``` The following queries are invalid: ```
member.type = "HUMAN" AND member.type = "BOT" role = "ROLE_MANAGER" AND role = "ROLE_MEMBER" ```
Invalid queries are rejected by the server with an `INVALID_ARGUMENT` error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A query filter. You can filter memberships by a member's role ([`role`](https:/
* /developers.google.com/workspace/chat/api/reference/rest/v1/spaces.members#membershiprole
* )) and type ([`member.type`](https://developers.google.com/workspace/chat/api/reference/r
* est/v1/User#type)). To filter by role, set `role` to `ROLE_MEMBER` or `ROLE_MANAGER`. To
* filter by type, set `member.type` to `HUMAN` or `BOT`. Developer Preview: You can also
* filter for `member.type` using the `!=` operator. To filter by both role and type, use
* the `AND` operator. To filter by either role or type, use the `OR` operator. Either
* `member.type = "HUMAN"` or `member.type != "BOT"` is required when `use_admin_access` is
* set to true. Other member type filters will be rejected. For example, the following
* queries are valid: ``` role = "ROLE_MANAGER" OR role = "ROLE_MEMBER" member.type =
* "HUMAN" AND role = "ROLE_MANAGER" member.type != "BOT" ``` The following queries are
* invalid: ``` member.type = "HUMAN" AND member.type = "BOT" role = "ROLE_MANAGER" AND role
* = "ROLE_MEMBER" ``` Invalid queries are rejected by the server with an `INVALID_ARGUMENT`
* error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of memberships to return. The service might return fewer
* than this value. If unspecified, at most 100 memberships are returned. The maximum value
* is 1000. If you use a value more than 1000, it's automatically changed to 1000. Negative
* values return an `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of memberships to return. The service might return fewer than this
value. If unspecified, at most 100 memberships are returned. The maximum value is 1000. If you use
a value more than 1000, it's automatically changed to 1000. Negative values return an
`INVALID_ARGUMENT` error.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of memberships to return. The service might return fewer
* than this value. If unspecified, at most 100 memberships are returned. The maximum value
* is 1000. If you use a value more than 1000, it's automatically changed to 1000. Negative
* values return an `INVALID_ARGUMENT` error.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous call to list memberships. Provide this
* parameter to retrieve the subsequent page. When paginating, all other parameters provided
* should match the call that provided the page token. Passing different values to the other
* parameters might lead to unexpected results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous call to list memberships. Provide this parameter
to retrieve the subsequent page. When paginating, all other parameters provided should match the
call that provided the page token. Passing different values to the other parameters might lead to
unexpected results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous call to list memberships. Provide this
* parameter to retrieve the subsequent page. When paginating, all other parameters provided
* should match the call that provided the page token. Passing different values to the other
* parameters might lead to unexpected results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. When `true`, also returns memberships associated with a Google Group, in
* addition to other types of memberships. If a filter is set, Google Group memberships that
* don't match the filter criteria aren't returned.
*/
@com.google.api.client.util.Key
private java.lang.Boolean showGroups;
/** Optional. When `true`, also returns memberships associated with a Google Group, in addition to
other types of memberships. If a filter is set, Google Group memberships that don't match the
filter criteria aren't returned.
*/
public java.lang.Boolean getShowGroups() {
return showGroups;
}
/**
* Optional. When `true`, also returns memberships associated with a Google Group, in
* addition to other types of memberships. If a filter is set, Google Group memberships that
* don't match the filter criteria aren't returned.
*/
public List setShowGroups(java.lang.Boolean showGroups) {
this.showGroups = showGroups;
return this;
}
/**
* Optional. When `true`, also returns memberships associated with invited members, in
* addition to other types of memberships. If a filter is set, invited memberships that
* don't match the filter criteria aren't returned. Currently requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user).
*/
@com.google.api.client.util.Key
private java.lang.Boolean showInvited;
/** Optional. When `true`, also returns memberships associated with invited members, in addition to
other types of memberships. If a filter is set, invited memberships that don't match the filter
criteria aren't returned. Currently requires [user
authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*/
public java.lang.Boolean getShowInvited() {
return showInvited;
}
/**
* Optional. When `true`, also returns memberships associated with invited members, in
* addition to other types of memberships. If a filter is set, invited memberships that
* don't match the filter criteria aren't returned. Currently requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user).
*/
public List setShowInvited(java.lang.Boolean showInvited) {
this.showInvited = showInvited;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires either the
* `chat.admin.memberships.readonly` or `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Listing app memberships in a space isn't supported when using admin access.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires either the
[ `chat.admin.memberships.readonly` or `chat.admin.memberships` [OAuth 2.0
[ scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-scopes).
[ Listing app memberships in a space isn't supported when using admin access.
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires either the
* `chat.admin.memberships.readonly` or `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes). Listing app memberships in a space isn't supported when using admin access.
*/
public List setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a membership. For an example, see [Update a user's membership in a
* space](https://developers.google.com/workspace/chat/update-members). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "members.patch".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Resource name of the membership, assigned by the server. Format: `spaces/{space}/members/{member}`
* @param content the {@link com.google.api.services.chat.v1.model.Membership}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.chat.v1.model.Membership content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/members/[^/]+$");
/**
* Updates a membership. For an example, see [Update a user's membership in a
* space](https://developers.google.com/workspace/chat/update-members). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "members.patch".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name of the membership, assigned by the server. Format: `spaces/{space}/members/{member}`
* @param content the {@link com.google.api.services.chat.v1.model.Membership}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.chat.v1.model.Membership content) {
super(HangoutsChat.this, "PATCH", REST_PATH, content, com.google.api.services.chat.v1.model.Membership.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/members/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name of the membership, assigned by the server. Format:
* `spaces/{space}/members/{member}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name of the membership, assigned by the server. Format: `spaces/{space}/members/{member}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the membership, assigned by the server. Format:
* `spaces/{space}/members/{member}`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/members/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The field paths to update. Separate multiple values with commas or use `*` to
* update all field paths. Currently supported field paths: - `role`
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The field paths to update. Separate multiple values with commas or use `*` to update all
field paths. Currently supported field paths: - `role`
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The field paths to update. Separate multiple values with commas or use `*` to
* update all field paths. Currently supported field paths: - `role`
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes).
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAdminAccess;
/**[ Developer Preview](https://developers.google.com/workspace/preview). When `true`, the method runs
[ using the user's Google Workspace administrator privileges. The calling user must be a Google
[ Workspace administrator with the [manage chat and spaces conversations
[ privilege](https://support.google.com/a/answer/13369245). Requires the `chat.admin.memberships`
[ [OAuth 2.0 scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
[ scopes).
[
*/
public java.lang.Boolean getUseAdminAccess() {
return useAdminAccess;
}
/**
* [Developer Preview](https://developers.google.com/workspace/preview). When `true`, the
* method runs using the user's Google Workspace administrator privileges. The calling user
* must be a Google Workspace administrator with the [manage chat and spaces conversations
* privilege](https://support.google.com/a/answer/13369245). Requires the
* `chat.admin.memberships` [OAuth 2.0
* scope](https://developers.google.com/workspace/chat/authenticate-authorize#chat-api-
* scopes).
*/
public Patch setUseAdminAccess(java.lang.Boolean useAdminAccess) {
this.useAdminAccess = useAdminAccess;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Messages collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Messages.List request = chat.messages().list(parameters ...)}
*
*
* @return the resource collection
*/
public Messages messages() {
return new Messages();
}
/**
* The "messages" collection of methods.
*/
public class Messages {
/**
* Creates a message in a Google Chat space. For an example, see [Send a
* message](https://developers.google.com/workspace/chat/create-messages). The `create()` method
* requires either user or app authentication. Chat attributes the message sender differently
* depending on the type of authentication that you use in your request. The following image shows
* how Chat attributes a message when you use app authentication. Chat displays the Chat app as the
* message sender. The content of the message can contain text (`text`), cards (`cardsV2`), and
* accessory widgets (`accessoryWidgets`).  The
* following image shows how Chat attributes a message when you use user authentication. Chat
* displays the user as the message sender and attributes the Chat app to the message by displaying
* its name. The content of message can only contain text (`text`).  The
* maximum message size, including the message contents, is 32,000 bytes.
*
* Create a request for the method "messages.create".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the space in which to create a message. Format: `spaces/{space}`
* @param content the {@link com.google.api.services.chat.v1.model.Message}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.chat.v1.model.Message content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/messages";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Creates a message in a Google Chat space. For an example, see [Send a
* message](https://developers.google.com/workspace/chat/create-messages). The `create()` method
* requires either user or app authentication. Chat attributes the message sender differently
* depending on the type of authentication that you use in your request. The following image shows
* how Chat attributes a message when you use app authentication. Chat displays the Chat app as
* the message sender. The content of the message can contain text (`text`), cards (`cardsV2`),
* and accessory widgets (`accessoryWidgets`).  The
* following image shows how Chat attributes a message when you use user authentication. Chat
* displays the user as the message sender and attributes the Chat app to the message by
* displaying its name. The content of message can only contain text (`text`). 
* The maximum message size, including the message contents, is 32,000 bytes.
*
* Create a request for the method "messages.create".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the space in which to create a message. Format: `spaces/{space}`
* @param content the {@link com.google.api.services.chat.v1.model.Message}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.chat.v1.model.Message content) {
super(HangoutsChat.this, "POST", REST_PATH, content, com.google.api.services.chat.v1.model.Message.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the space in which to create a message. Format:
* `spaces/{space}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the space in which to create a message. Format: `spaces/{space}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the space in which to create a message. Format:
* `spaces/{space}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A custom ID for a message. Lets Chat apps get, update, or delete a message
* without needing to store the system-assigned ID in the message's resource name
* (represented in the message `name` field). The value for this field must meet the
* following requirements: * Begins with `client-`. For example, `client-custom-name` is a
* valid custom ID, but `custom-name` is not. * Contains up to 63 characters and only
* lowercase letters, numbers, and hyphens. * Is unique within a space. A Chat app can't use
* the same custom ID for different messages. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
*/
@com.google.api.client.util.Key
private java.lang.String messageId;
/** Optional. A custom ID for a message. Lets Chat apps get, update, or delete a message without
needing to store the system-assigned ID in the message's resource name (represented in the message
`name` field). The value for this field must meet the following requirements: * Begins with
`client-`. For example, `client-custom-name` is a valid custom ID, but `custom-name` is not. *
Contains up to 63 characters and only lowercase letters, numbers, and hyphens. * Is unique within a
space. A Chat app can't use the same custom ID for different messages. For details, see [Name a
message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
public java.lang.String getMessageId() {
return messageId;
}
/**
* Optional. A custom ID for a message. Lets Chat apps get, update, or delete a message
* without needing to store the system-assigned ID in the message's resource name
* (represented in the message `name` field). The value for this field must meet the
* following requirements: * Begins with `client-`. For example, `client-custom-name` is a
* valid custom ID, but `custom-name` is not. * Contains up to 63 characters and only
* lowercase letters, numbers, and hyphens. * Is unique within a space. A Chat app can't use
* the same custom ID for different messages. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
*/
public Create setMessageId(java.lang.String messageId) {
this.messageId = messageId;
return this;
}
/**
* Optional. Specifies whether a message starts a thread or replies to one. Only supported
* in named spaces.
*/
@com.google.api.client.util.Key
private java.lang.String messageReplyOption;
/** Optional. Specifies whether a message starts a thread or replies to one. Only supported in named
spaces.
*/
public java.lang.String getMessageReplyOption() {
return messageReplyOption;
}
/**
* Optional. Specifies whether a message starts a thread or replies to one. Only supported
* in named spaces.
*/
public Create setMessageReplyOption(java.lang.String messageReplyOption) {
this.messageReplyOption = messageReplyOption;
return this;
}
/**
* Optional. A unique request ID for this message. Specifying an existing request ID returns
* the message created with that ID instead of creating a new message.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique request ID for this message. Specifying an existing request ID returns the
message created with that ID instead of creating a new message.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique request ID for this message. Specifying an existing request ID returns
* the message created with that ID instead of creating a new message.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Optional. Deprecated: Use thread.thread_key instead. ID for the thread. Supports up to
* 4000 characters. To start or add to a thread, create a message and specify a `threadKey`
* or the thread.name. For example usage, see [Start or reply to a message
* thread](https://developers.google.com/workspace/chat/create-messages#create-message-
* thread).
*/
@com.google.api.client.util.Key
private java.lang.String threadKey;
/** Optional. Deprecated: Use thread.thread_key instead. ID for the thread. Supports up to 4000
characters. To start or add to a thread, create a message and specify a `threadKey` or the
thread.name. For example usage, see [Start or reply to a message
thread](https://developers.google.com/workspace/chat/create-messages#create-message-thread).
*/
public java.lang.String getThreadKey() {
return threadKey;
}
/**
* Optional. Deprecated: Use thread.thread_key instead. ID for the thread. Supports up to
* 4000 characters. To start or add to a thread, create a message and specify a `threadKey`
* or the thread.name. For example usage, see [Start or reply to a message
* thread](https://developers.google.com/workspace/chat/create-messages#create-message-
* thread).
*/
public Create setThreadKey(java.lang.String threadKey) {
this.threadKey = threadKey;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a message. For an example, see [Delete a
* message](https://developers.google.com/workspace/chat/delete-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). When using app authentication, requests can only delete messages created by
* the calling Chat app.
*
* Create a request for the method "messages.delete".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
* custom ID for your message, you can use the value from the `clientAssignedMessageId` field
* for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+$");
/**
* Deletes a message. For an example, see [Delete a
* message](https://developers.google.com/workspace/chat/delete-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). When using app authentication, requests can only delete messages created
* by the calling Chat app.
*
* Create a request for the method "messages.delete".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
* custom ID for your message, you can use the value from the `clientAssignedMessageId` field
* for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(HangoutsChat.this, "DELETE", REST_PATH, null, com.google.api.services.chat.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If
* you've set a custom ID for your message, you can use the value from the
* `clientAssignedMessageId` field for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
custom ID for your message, you can use the value from the `clientAssignedMessageId` field for
`{message}`. For details, see [Name a message]
(https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If
* you've set a custom ID for your message, you can use the value from the
* `clientAssignedMessageId` field for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
this.name = name;
return this;
}
/**
* When `true`, deleting a message also deletes its threaded replies. When `false`, if a
* message has threaded replies, deletion fails. Only applies when [authenticating as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user). Has
* no effect when [authenticating as a Chat app]
* (https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** When `true`, deleting a message also deletes its threaded replies. When `false`, if a message has
threaded replies, deletion fails. Only applies when [authenticating as a
user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user). Has no effect
when [authenticating as a Chat app] (https://developers.google.com/workspace/chat/authenticate-
authorize-chat-app).
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* When `true`, deleting a message also deletes its threaded replies. When `false`, if a
* message has threaded replies, deletion fails. Only applies when [authenticating as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user). Has
* no effect when [authenticating as a Chat app]
* (https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns details about a message. For an example, see [Get details about a
* message](https://developers.google.com/workspace/chat/get-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). Note: Might return a message from a blocked member or space.
*
* Create a request for the method "messages.get".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
* custom ID for your message, you can use the value from the `clientAssignedMessageId` field
* for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+$");
/**
* Returns details about a message. For an example, see [Get details about a
* message](https://developers.google.com/workspace/chat/get-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). Note: Might return a message from a blocked member or space.
*
* Create a request for the method "messages.get".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
* custom ID for your message, you can use the value from the `clientAssignedMessageId` field
* for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @since 1.13
*/
protected Get(java.lang.String name) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.Message.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If
* you've set a custom ID for your message, you can use the value from the
* `clientAssignedMessageId` field for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
custom ID for your message, you can use the value from the `clientAssignedMessageId` field for
`{message}`. For details, see [Name a message]
(https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If
* you've set a custom ID for your message, you can use the value from the
* `clientAssignedMessageId` field for `{message}`. For details, see [Name a message]
* (https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists messages in a space that the caller is a member of, including messages from blocked members
* and spaces. If you list messages from a space with no messages, the response is an empty object.
* When using a REST/HTTP interface, the response contains an empty JSON object, `{}`. For an
* example, see [List
* messages](https://developers.google.com/workspace/chat/api/guides/v1/messages/list). Requires
* [user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user).
*
* Create a request for the method "messages.list".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the space to list messages from. Format: `spaces/{space}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/messages";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Lists messages in a space that the caller is a member of, including messages from blocked
* members and spaces. If you list messages from a space with no messages, the response is an
* empty object. When using a REST/HTTP interface, the response contains an empty JSON object,
* `{}`. For an example, see [List
* messages](https://developers.google.com/workspace/chat/api/guides/v1/messages/list). Requires
* [user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* user).
*
* Create a request for the method "messages.list".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the space to list messages from. Format: `spaces/{space}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.ListMessagesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the space to list messages from. Format: `spaces/{space}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the space to list messages from. Format: `spaces/{space}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the space to list messages from. Format: `spaces/{space}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A query filter. You can filter messages by date (`create_time`) and thread
* (`thread.name`). To filter messages by the date they were created, specify the
* `create_time` with a timestamp in [RFC-3339](https://www.rfc-editor.org/rfc/rfc3339)
* format and double quotation marks. For example, `"2023-04-21T11:30:00-04:00"`. You can
* use the greater than operator `>` to list messages that were created after a timestamp,
* or the less than operator `<` to list messages that were created before a timestamp. To
* filter messages within a time interval, use the `AND` operator between two timestamps. To
* filter by thread, specify the `thread.name`, formatted as
* `spaces/{space}/threads/{thread}`. You can only specify one `thread.name` per query. To
* filter by both thread and date, use the `AND` operator in your query. For example, the
* following queries are valid: ``` create_time > "2012-04-21T11:30:00-04:00" create_time >
* "2012-04-21T11:30:00-04:00" AND thread.name = spaces/AAAAAAAAAAA/threads/123 create_time
* > "2012-04-21T11:30:00+00:00" AND create_time < "2013-01-01T00:00:00+00:00" AND
* thread.name = spaces/AAAAAAAAAAA/threads/123 thread.name = spaces/AAAAAAAAAAA/threads/123
* ``` Invalid queries are rejected by the server with an `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A query filter. You can filter messages by date (`create_time`) and thread (`thread.name`). To
filter messages by the date they were created, specify the `create_time` with a timestamp in
[RFC-3339](https://www.rfc-editor.org/rfc/rfc3339) format and double quotation marks. For example,
`"2023-04-21T11:30:00-04:00"`. You can use the greater than operator `>` to list messages that were
created after a timestamp, or the less than operator `<` to list messages that were created before
a timestamp. To filter messages within a time interval, use the `AND` operator between two
timestamps. To filter by thread, specify the `thread.name`, formatted as
`spaces/{space}/threads/{thread}`. You can only specify one `thread.name` per query. To filter by
both thread and date, use the `AND` operator in your query. For example, the following queries are
valid: ``` create_time > "2012-04-21T11:30:00-04:00" create_time > "2012-04-21T11:30:00-04:00" AND
thread.name = spaces/AAAAAAAAAAA/threads/123 create_time > "2012-04-21T11:30:00+00:00" AND
create_time < "2013-01-01T00:00:00+00:00" AND thread.name = spaces/AAAAAAAAAAA/threads/123
thread.name = spaces/AAAAAAAAAAA/threads/123 ``` Invalid queries are rejected by the server with an
`INVALID_ARGUMENT` error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A query filter. You can filter messages by date (`create_time`) and thread
* (`thread.name`). To filter messages by the date they were created, specify the
* `create_time` with a timestamp in [RFC-3339](https://www.rfc-editor.org/rfc/rfc3339)
* format and double quotation marks. For example, `"2023-04-21T11:30:00-04:00"`. You can
* use the greater than operator `>` to list messages that were created after a timestamp,
* or the less than operator `<` to list messages that were created before a timestamp. To
* filter messages within a time interval, use the `AND` operator between two timestamps. To
* filter by thread, specify the `thread.name`, formatted as
* `spaces/{space}/threads/{thread}`. You can only specify one `thread.name` per query. To
* filter by both thread and date, use the `AND` operator in your query. For example, the
* following queries are valid: ``` create_time > "2012-04-21T11:30:00-04:00" create_time >
* "2012-04-21T11:30:00-04:00" AND thread.name = spaces/AAAAAAAAAAA/threads/123 create_time
* > "2012-04-21T11:30:00+00:00" AND create_time < "2013-01-01T00:00:00+00:00" AND
* thread.name = spaces/AAAAAAAAAAA/threads/123 thread.name = spaces/AAAAAAAAAAA/threads/123
* ``` Invalid queries are rejected by the server with an `INVALID_ARGUMENT` error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional, if resuming from a previous query. How the list of messages is ordered. Specify
* a value to order by an ordering operation. Valid ordering operation values are as
* follows: - `ASC` for ascending. - `DESC` for descending. The default ordering is
* `create_time ASC`.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional, if resuming from a previous query. How the list of messages is ordered. Specify a value
to order by an ordering operation. Valid ordering operation values are as follows: - `ASC` for
ascending. - `DESC` for descending. The default ordering is `create_time ASC`.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional, if resuming from a previous query. How the list of messages is ordered. Specify
* a value to order by an ordering operation. Valid ordering operation values are as
* follows: - `ASC` for ascending. - `DESC` for descending. The default ordering is
* `create_time ASC`.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of messages returned. The service might return fewer messages than
* this value. If unspecified, at most 25 are returned. The maximum value is 1000. If you
* use a value more than 1000, it's automatically changed to 1000. Negative values return an
* `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of messages returned. The service might return fewer messages than this value.
If unspecified, at most 25 are returned. The maximum value is 1000. If you use a value more than
1000, it's automatically changed to 1000. Negative values return an `INVALID_ARGUMENT` error.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of messages returned. The service might return fewer messages than
* this value. If unspecified, at most 25 are returned. The maximum value is 1000. If you
* use a value more than 1000, it's automatically changed to 1000. Negative values return an
* `INVALID_ARGUMENT` error.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional, if resuming from a previous query. A page token received from a previous list
* messages call. Provide this parameter to retrieve the subsequent page. When paginating,
* all other parameters provided should match the call that provided the page token. Passing
* different values to the other parameters might lead to unexpected results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional, if resuming from a previous query. A page token received from a previous list messages
call. Provide this parameter to retrieve the subsequent page. When paginating, all other parameters
provided should match the call that provided the page token. Passing different values to the other
parameters might lead to unexpected results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional, if resuming from a previous query. A page token received from a previous list
* messages call. Provide this parameter to retrieve the subsequent page. When paginating,
* all other parameters provided should match the call that provided the page token. Passing
* different values to the other parameters might lead to unexpected results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Whether to include deleted messages. Deleted messages include deleted time and metadata
* about their deletion, but message content is unavailable.
*/
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** Whether to include deleted messages. Deleted messages include deleted time and metadata about their
deletion, but message content is unavailable.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/**
* Whether to include deleted messages. Deleted messages include deleted time and metadata
* about their deletion, but message content is unavailable.
*/
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a message. There's a difference between the `patch` and `update` methods. The `patch`
* method uses a `patch` request while the `update` method uses a `put` request. We recommend using
* the `patch` method. For an example, see [Update a
* message](https://developers.google.com/workspace/chat/update-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). When using app authentication, requests can only update messages created by
* the calling Chat app.
*
* Create a request for the method "messages.patch".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
* of the space where the message is posted and `{message}` is a system-assigned ID for the
* message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
* custom ID when you create a message, you can use this ID to specify the message in a
* request by replacing `{message}` with the value from the `clientAssignedMessageId` field.
* For example, `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
* @param content the {@link com.google.api.services.chat.v1.model.Message}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.chat.v1.model.Message content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+$");
/**
* Updates a message. There's a difference between the `patch` and `update` methods. The `patch`
* method uses a `patch` request while the `update` method uses a `put` request. We recommend
* using the `patch` method. For an example, see [Update a
* message](https://developers.google.com/workspace/chat/update-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). When using app authentication, requests can only update messages created
* by the calling Chat app.
*
* Create a request for the method "messages.patch".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
* of the space where the message is posted and `{message}` is a system-assigned ID for the
* message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
* custom ID when you create a message, you can use this ID to specify the message in a
* request by replacing `{message}` with the value from the `clientAssignedMessageId` field.
* For example, `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
* @param content the {@link com.google.api.services.chat.v1.model.Message}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.chat.v1.model.Message content) {
super(HangoutsChat.this, "PATCH", REST_PATH, content, com.google.api.services.chat.v1.model.Message.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}`
* is the ID of the space where the message is posted and `{message}` is a system-assigned
* ID for the message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`.
* If you set a custom ID when you create a message, you can use this ID to specify the
* message in a request by replacing `{message}` with the value from the
* `clientAssignedMessageId` field. For example, `spaces/AAAAAAAAAAA/messages/client-custom-
* name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
of the space where the message is posted and `{message}` is a system-assigned ID for the message.
For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a custom ID when you
create a message, you can use this ID to specify the message in a request by replacing `{message}`
with the value from the `clientAssignedMessageId` field. For example,
`spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}`
* is the ID of the space where the message is posted and `{message}` is a system-assigned
* ID for the message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`.
* If you set a custom ID when you create a message, you can use this ID to specify the
* message in a request by replacing `{message}` with the value from the
* `clientAssignedMessageId` field. For example, `spaces/AAAAAAAAAAA/messages/client-custom-
* name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. If `true` and the message isn't found, a new message is created and
* `updateMask` is ignored. The specified message ID must be [client-
* assigned](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message) or the request fails.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowMissing;
/** Optional. If `true` and the message isn't found, a new message is created and `updateMask` is
ignored. The specified message ID must be [client-
assigned](https://developers.google.com/workspace/chat/create-messages#name_a_created_message) or
the request fails.
*/
public java.lang.Boolean getAllowMissing() {
return allowMissing;
}
/**
* Optional. If `true` and the message isn't found, a new message is created and
* `updateMask` is ignored. The specified message ID must be [client-
* assigned](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message) or the request fails.
*/
public Patch setAllowMissing(java.lang.Boolean allowMissing) {
this.allowMissing = allowMissing;
return this;
}
/**
* Required. The field paths to update. Separate multiple values with commas or use `*` to
* update all field paths. Currently supported field paths: - `text` - `attachment` -
* `cards` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `cards_v2` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `accessory_widgets` (Requires [app authentication](/chat/api/guides/auth/service-
* accounts).)
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The field paths to update. Separate multiple values with commas or use `*` to update all
field paths. Currently supported field paths: - `text` - `attachment` - `cards` (Requires [app
authentication](/chat/api/guides/auth/service-accounts).) - `cards_v2` (Requires [app
authentication](/chat/api/guides/auth/service-accounts).) - `accessory_widgets` (Requires [app
authentication](/chat/api/guides/auth/service-accounts).)
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The field paths to update. Separate multiple values with commas or use `*` to
* update all field paths. Currently supported field paths: - `text` - `attachment` -
* `cards` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `cards_v2` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `accessory_widgets` (Requires [app authentication](/chat/api/guides/auth/service-
* accounts).)
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a message. There's a difference between the `patch` and `update` methods. The `patch`
* method uses a `patch` request while the `update` method uses a `put` request. We recommend using
* the `patch` method. For an example, see [Update a
* message](https://developers.google.com/workspace/chat/update-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). When using app authentication, requests can only update messages created by
* the calling Chat app.
*
* Create a request for the method "messages.update".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param name Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
* of the space where the message is posted and `{message}` is a system-assigned ID for the
* message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
* custom ID when you create a message, you can use this ID to specify the message in a
* request by replacing `{message}` with the value from the `clientAssignedMessageId` field.
* For example, `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
* @param content the {@link com.google.api.services.chat.v1.model.Message}
* @return the request
*/
public Update update(java.lang.String name, com.google.api.services.chat.v1.model.Message content) throws java.io.IOException {
Update result = new Update(name, content);
initialize(result);
return result;
}
public class Update extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+$");
/**
* Updates a message. There's a difference between the `patch` and `update` methods. The `patch`
* method uses a `patch` request while the `update` method uses a `put` request. We recommend
* using the `patch` method. For an example, see [Update a
* message](https://developers.google.com/workspace/chat/update-messages). Requires
* [authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
* [app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
* app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user). When using app authentication, requests can only update messages created
* by the calling Chat app.
*
* Create a request for the method "messages.update".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
* of the space where the message is posted and `{message}` is a system-assigned ID for the
* message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
* custom ID when you create a message, you can use this ID to specify the message in a
* request by replacing `{message}` with the value from the `clientAssignedMessageId` field.
* For example, `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
* @param content the {@link com.google.api.services.chat.v1.model.Message}
* @since 1.13
*/
protected Update(java.lang.String name, com.google.api.services.chat.v1.model.Message content) {
super(HangoutsChat.this, "PUT", REST_PATH, content, com.google.api.services.chat.v1.model.Message.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}`
* is the ID of the space where the message is posted and `{message}` is a system-assigned
* ID for the message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`.
* If you set a custom ID when you create a message, you can use this ID to specify the
* message in a request by replacing `{message}` with the value from the
* `clientAssignedMessageId` field. For example, `spaces/AAAAAAAAAAA/messages/client-custom-
* name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
of the space where the message is posted and `{message}` is a system-assigned ID for the message.
For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a custom ID when you
create a message, you can use this ID to specify the message in a request by replacing `{message}`
with the value from the `clientAssignedMessageId` field. For example,
`spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}`
* is the ID of the space where the message is posted and `{message}` is a system-assigned
* ID for the message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`.
* If you set a custom ID when you create a message, you can use this ID to specify the
* message in a request by replacing `{message}` with the value from the
* `clientAssignedMessageId` field. For example, `spaces/AAAAAAAAAAA/messages/client-custom-
* name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message).
*/
public Update setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. If `true` and the message isn't found, a new message is created and
* `updateMask` is ignored. The specified message ID must be [client-
* assigned](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message) or the request fails.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowMissing;
/** Optional. If `true` and the message isn't found, a new message is created and `updateMask` is
ignored. The specified message ID must be [client-
assigned](https://developers.google.com/workspace/chat/create-messages#name_a_created_message) or
the request fails.
*/
public java.lang.Boolean getAllowMissing() {
return allowMissing;
}
/**
* Optional. If `true` and the message isn't found, a new message is created and
* `updateMask` is ignored. The specified message ID must be [client-
* assigned](https://developers.google.com/workspace/chat/create-
* messages#name_a_created_message) or the request fails.
*/
public Update setAllowMissing(java.lang.Boolean allowMissing) {
this.allowMissing = allowMissing;
return this;
}
/**
* Required. The field paths to update. Separate multiple values with commas or use `*` to
* update all field paths. Currently supported field paths: - `text` - `attachment` -
* `cards` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `cards_v2` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `accessory_widgets` (Requires [app authentication](/chat/api/guides/auth/service-
* accounts).)
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The field paths to update. Separate multiple values with commas or use `*` to update all
field paths. Currently supported field paths: - `text` - `attachment` - `cards` (Requires [app
authentication](/chat/api/guides/auth/service-accounts).) - `cards_v2` (Requires [app
authentication](/chat/api/guides/auth/service-accounts).) - `accessory_widgets` (Requires [app
authentication](/chat/api/guides/auth/service-accounts).)
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The field paths to update. Separate multiple values with commas or use `*` to
* update all field paths. Currently supported field paths: - `text` - `attachment` -
* `cards` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `cards_v2` (Requires [app authentication](/chat/api/guides/auth/service-accounts).) -
* `accessory_widgets` (Requires [app authentication](/chat/api/guides/auth/service-
* accounts).)
*/
public Update setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Attachments collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Attachments.List request = chat.attachments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Attachments attachments() {
return new Attachments();
}
/**
* The "attachments" collection of methods.
*/
public class Attachments {
/**
* Gets the metadata of a message attachment. The attachment data is fetched using the [media
* API](https://developers.google.com/workspace/chat/api/reference/rest/v1/media/download). For an
* example, see [Get metadata about a message
* attachment](https://developers.google.com/workspace/chat/get-media-attachments). Requires [app
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
*
* Create a request for the method "attachments.get".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the attachment, in the form
* `spaces/{space}/messages/{message}/attachments/{attachment}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+/attachments/[^/]+$");
/**
* Gets the metadata of a message attachment. The attachment data is fetched using the [media
* API](https://developers.google.com/workspace/chat/api/reference/rest/v1/media/download). For an
* example, see [Get metadata about a message
* attachment](https://developers.google.com/workspace/chat/get-media-attachments). Requires [app
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
*
* Create a request for the method "attachments.get".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the attachment, in the form
* `spaces/{space}/messages/{message}/attachments/{attachment}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.Attachment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+/attachments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the attachment, in the form
* `spaces/{space}/messages/{message}/attachments/{attachment}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the attachment, in the form
`spaces/{space}/messages/{message}/attachments/{attachment}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the attachment, in the form
* `spaces/{space}/messages/{message}/attachments/{attachment}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+/attachments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Reactions collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Reactions.List request = chat.reactions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Reactions reactions() {
return new Reactions();
}
/**
* The "reactions" collection of methods.
*/
public class Reactions {
/**
* Creates a reaction and adds it to a message. Only unicode emojis are supported. For an example,
* see [Add a reaction to a message](https://developers.google.com/workspace/chat/create-reactions).
* Requires [user authentication](https://developers.google.com/workspace/chat/authenticate-
* authorize-chat-user).
*
* Create a request for the method "reactions.create".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The message where the reaction is created. Format: `spaces/{space}/messages/{message}`
* @param content the {@link com.google.api.services.chat.v1.model.Reaction}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.chat.v1.model.Reaction content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/reactions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+$");
/**
* Creates a reaction and adds it to a message. Only unicode emojis are supported. For an example,
* see [Add a reaction to a message](https://developers.google.com/workspace/chat/create-
* reactions). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "reactions.create".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The message where the reaction is created. Format: `spaces/{space}/messages/{message}`
* @param content the {@link com.google.api.services.chat.v1.model.Reaction}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.chat.v1.model.Reaction content) {
super(HangoutsChat.this, "POST", REST_PATH, content, com.google.api.services.chat.v1.model.Reaction.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The message where the reaction is created. Format:
* `spaces/{space}/messages/{message}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The message where the reaction is created. Format: `spaces/{space}/messages/{message}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The message where the reaction is created. Format:
* `spaces/{space}/messages/{message}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a reaction to a message. Only unicode emojis are supported. For an example, see [Delete a
* reaction](https://developers.google.com/workspace/chat/delete-reactions). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "reactions.delete".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the reaction to delete. Format:
* `spaces/{space}/messages/{message}/reactions/{reaction}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+/reactions/[^/]+$");
/**
* Deletes a reaction to a message. Only unicode emojis are supported. For an example, see [Delete
* a reaction](https://developers.google.com/workspace/chat/delete-reactions). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "reactions.delete".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the reaction to delete. Format:
* `spaces/{space}/messages/{message}/reactions/{reaction}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(HangoutsChat.this, "DELETE", REST_PATH, null, com.google.api.services.chat.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+/reactions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the reaction to delete. Format:
* `spaces/{space}/messages/{message}/reactions/{reaction}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the reaction to delete. Format:
`spaces/{space}/messages/{message}/reactions/{reaction}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the reaction to delete. Format:
* `spaces/{space}/messages/{message}/reactions/{reaction}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+/reactions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists reactions to a message. For an example, see [List reactions for a
* message](https://developers.google.com/workspace/chat/list-reactions). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "reactions.list".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The message users reacted to. Format: `spaces/{space}/messages/{message}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/reactions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/messages/[^/]+$");
/**
* Lists reactions to a message. For an example, see [List reactions for a
* message](https://developers.google.com/workspace/chat/list-reactions). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "reactions.list".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The message users reacted to. Format: `spaces/{space}/messages/{message}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.ListReactionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The message users reacted to. Format: `spaces/{space}/messages/{message}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The message users reacted to. Format: `spaces/{space}/messages/{message}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The message users reacted to. Format: `spaces/{space}/messages/{message}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+/messages/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A query filter. You can filter reactions by
* [emoji](https://developers.google.com/workspace/chat/api/reference/rest/v1/Emoji)
* (either `emoji.unicode` or `emoji.custom_emoji.uid`) and
* [user](https://developers.google.com/workspace/chat/api/reference/rest/v1/User)
* (`user.name`). To filter reactions for multiple emojis or users, join similar fields
* with the `OR` operator, such as `emoji.unicode = "🙂" OR emoji.unicode = "👍"` and
* `user.name = "users/AAAAAA" OR user.name = "users/BBBBBB"`. To filter reactions by
* emoji and user, use the `AND` operator, such as `emoji.unicode = "🙂" AND user.name =
* "users/AAAAAA"`. If your query uses both `AND` and `OR`, group them with parentheses.
* For example, the following queries are valid: ``` user.name = "users/{user}"
* emoji.unicode = "🙂" emoji.custom_emoji.uid = "{uid}" emoji.unicode = "🙂" OR
* emoji.unicode = "👍" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}"
* emoji.unicode = "🙂" AND user.name = "users/{user}" (emoji.unicode = "🙂" OR
* emoji.custom_emoji.uid = "{uid}") AND user.name = "users/{user}" ``` The following
* queries are invalid: ``` emoji.unicode = "🙂" AND emoji.unicode = "👍" emoji.unicode =
* "🙂" AND emoji.custom_emoji.uid = "{uid}" emoji.unicode = "🙂" OR user.name =
* "users/{user}" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}" OR user.name =
* "users/{user}" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}" AND user.name =
* "users/{user}" ``` Invalid queries are rejected by the server with an
* `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A query filter. You can filter reactions by
[emoji](https://developers.google.com/workspace/chat/api/reference/rest/v1/Emoji) (either
`emoji.unicode` or `emoji.custom_emoji.uid`) and
[user](https://developers.google.com/workspace/chat/api/reference/rest/v1/User) (`user.name`). To
filter reactions for multiple emojis or users, join similar fields with the `OR` operator, such as
`emoji.unicode = "🙂" OR emoji.unicode = "👍"` and `user.name = "users/AAAAAA" OR user.name =
"users/BBBBBB"`. To filter reactions by emoji and user, use the `AND` operator, such as
`emoji.unicode = "🙂" AND user.name = "users/AAAAAA"`. If your query uses both `AND` and `OR`, group
them with parentheses. For example, the following queries are valid: ``` user.name = "users/{user}"
emoji.unicode = "🙂" emoji.custom_emoji.uid = "{uid}" emoji.unicode = "🙂" OR emoji.unicode = "👍"
emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}" emoji.unicode = "🙂" AND user.name =
"users/{user}" (emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}") AND user.name =
"users/{user}" ``` The following queries are invalid: ``` emoji.unicode = "🙂" AND emoji.unicode =
"👍" emoji.unicode = "🙂" AND emoji.custom_emoji.uid = "{uid}" emoji.unicode = "🙂" OR user.name =
"users/{user}" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}" OR user.name =
"users/{user}" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}" AND user.name =
"users/{user}" ``` Invalid queries are rejected by the server with an `INVALID_ARGUMENT` error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A query filter. You can filter reactions by
* [emoji](https://developers.google.com/workspace/chat/api/reference/rest/v1/Emoji)
* (either `emoji.unicode` or `emoji.custom_emoji.uid`) and
* [user](https://developers.google.com/workspace/chat/api/reference/rest/v1/User)
* (`user.name`). To filter reactions for multiple emojis or users, join similar fields
* with the `OR` operator, such as `emoji.unicode = "🙂" OR emoji.unicode = "👍"` and
* `user.name = "users/AAAAAA" OR user.name = "users/BBBBBB"`. To filter reactions by
* emoji and user, use the `AND` operator, such as `emoji.unicode = "🙂" AND user.name =
* "users/AAAAAA"`. If your query uses both `AND` and `OR`, group them with parentheses.
* For example, the following queries are valid: ``` user.name = "users/{user}"
* emoji.unicode = "🙂" emoji.custom_emoji.uid = "{uid}" emoji.unicode = "🙂" OR
* emoji.unicode = "👍" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}"
* emoji.unicode = "🙂" AND user.name = "users/{user}" (emoji.unicode = "🙂" OR
* emoji.custom_emoji.uid = "{uid}") AND user.name = "users/{user}" ``` The following
* queries are invalid: ``` emoji.unicode = "🙂" AND emoji.unicode = "👍" emoji.unicode =
* "🙂" AND emoji.custom_emoji.uid = "{uid}" emoji.unicode = "🙂" OR user.name =
* "users/{user}" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}" OR user.name =
* "users/{user}" emoji.unicode = "🙂" OR emoji.custom_emoji.uid = "{uid}" AND user.name =
* "users/{user}" ``` Invalid queries are rejected by the server with an
* `INVALID_ARGUMENT` error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of reactions returned. The service can return fewer
* reactions than this value. If unspecified, the default value is 25. The maximum value
* is 200; values above 200 are changed to 200.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of reactions returned. The service can return fewer reactions than
this value. If unspecified, the default value is 25. The maximum value is 200; values above 200 are
changed to 200.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of reactions returned. The service can return fewer
* reactions than this value. If unspecified, the default value is 25. The maximum value
* is 200; values above 200 are changed to 200.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. (If resuming from a previous query.) A page token received from a previous
* list reactions call. Provide this to retrieve the subsequent page. When paginating, the
* filter value should match the call that provided the page token. Passing a different
* value might lead to unexpected results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. (If resuming from a previous query.) A page token received from a previous list reactions
call. Provide this to retrieve the subsequent page. When paginating, the filter value should match
the call that provided the page token. Passing a different value might lead to unexpected results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. (If resuming from a previous query.) A page token received from a previous
* list reactions call. Provide this to retrieve the subsequent page. When paginating, the
* filter value should match the call that provided the page token. Passing a different
* value might lead to unexpected results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the SpaceEvents collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.SpaceEvents.List request = chat.spaceEvents().list(parameters ...)}
*
*
* @return the resource collection
*/
public SpaceEvents spaceEvents() {
return new SpaceEvents();
}
/**
* The "spaceEvents" collection of methods.
*/
public class SpaceEvents {
/**
* Returns an event from a Google Chat space. The [event payload](https://developers.google.com/work
* space/chat/api/reference/rest/v1/spaces.spaceEvents#SpaceEvent.FIELDS.oneof_payload) contains the
* most recent version of the resource that changed. For example, if you request an event about a
* new message but the message was later updated, the server returns the updated `Message` resource
* in the event payload. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* To get an event, the authenticated user must be a member of the space. For an example, see [Get
* details about an event from a Google Chat
* space](https://developers.google.com/workspace/chat/get-space-event).
*
* Create a request for the method "spaceEvents.get".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the space event. Format: `spaces/{space}/spaceEvents/{spaceEvent}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+/spaceEvents/[^/]+$");
/**
* Returns an event from a Google Chat space. The [event payload](https://developers.google.com/wo
* rkspace/chat/api/reference/rest/v1/spaces.spaceEvents#SpaceEvent.FIELDS.oneof_payload) contains
* the most recent version of the resource that changed. For example, if you request an event
* about a new message but the message was later updated, the server returns the updated `Message`
* resource in the event payload. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* To get an event, the authenticated user must be a member of the space. For an example, see [Get
* details about an event from a Google Chat
* space](https://developers.google.com/workspace/chat/get-space-event).
*
* Create a request for the method "spaceEvents.get".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the space event. Format: `spaces/{space}/spaceEvents/{spaceEvent}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.SpaceEvent.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/spaceEvents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the space event. Format:
* `spaces/{space}/spaceEvents/{spaceEvent}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the space event. Format: `spaces/{space}/spaceEvents/{spaceEvent}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the space event. Format:
* `spaces/{space}/spaceEvents/{spaceEvent}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^spaces/[^/]+/spaceEvents/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists events from a Google Chat space. For each event, the [payload](https://developers.google.co
* m/workspace/chat/api/reference/rest/v1/spaces.spaceEvents#SpaceEvent.FIELDS.oneof_payload)
* contains the most recent version of the Chat resource. For example, if you list events about new
* space members, the server returns `Membership` resources that contain the latest membership
* details. If new members were removed during the requested period, the event payload contains an
* empty `Membership` resource. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* To list events, the authenticated user must be a member of the space. For an example, see [List
* events from a Google Chat space](https://developers.google.com/workspace/chat/list-space-events).
*
* Create a request for the method "spaceEvents.list".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the [Google Chat
* space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) where
* the events occurred. Format: `spaces/{space}`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+parent}/spaceEvents";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^spaces/[^/]+$");
/**
* Lists events from a Google Chat space. For each event, the [payload](https://developers.google.
* com/workspace/chat/api/reference/rest/v1/spaces.spaceEvents#SpaceEvent.FIELDS.oneof_payload)
* contains the most recent version of the Chat resource. For example, if you list events about
* new space members, the server returns `Membership` resources that contain the latest membership
* details. If new members were removed during the requested period, the event payload contains an
* empty `Membership` resource. Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
* To list events, the authenticated user must be a member of the space. For an example, see [List
* events from a Google Chat space](https://developers.google.com/workspace/chat/list-space-
* events).
*
* Create a request for the method "spaceEvents.list".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the [Google Chat
* space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) where
* the events occurred. Format: `spaces/{space}`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.ListSpaceEventsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the [Google Chat
* space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) where
* the events occurred. Format: `spaces/{space}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the [Google Chat
space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) where the events
occurred. Format: `spaces/{space}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the [Google Chat
* space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) where
* the events occurred. Format: `spaces/{space}`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^spaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A query filter. You must specify at least one event type (`event_type`) using
* the has `:` operator. To filter by multiple event types, use the `OR` operator. Omit
* batch event types in your filter. The request automatically returns any related batch
* events. For example, if you filter by new reactions
* (`google.workspace.chat.reaction.v1.created`), the server also returns batch new
* reactions events (`google.workspace.chat.reaction.v1.batchCreated`). For a list of
* supported event types, see the [`SpaceEvents` reference documentation](https://developers
* .google.com/workspace/chat/api/reference/rest/v1/spaces.spaceEvents#SpaceEvent.FIELDS.eve
* nt_type). Optionally, you can also filter by start time (`start_time`) and end time
* (`end_time`): * `start_time`: Exclusive timestamp from which to start listing space
* events. You can list events that occurred up to 28 days ago. If unspecified, lists space
* events from the past 28 days. * `end_time`: Inclusive timestamp until which space events
* are listed. If unspecified, lists events up to the time of the request. To specify a
* start or end time, use the equals `=` operator and format in [RFC-3339](https://www.rfc-
* editor.org/rfc/rfc3339). To filter by both `start_time` and `end_time`, use the `AND`
* operator. For example, the following queries are valid: ```
* start_time="2023-08-23T19:20:33+00:00" AND end_time="2023-08-23T19:21:54+00:00" ``` ```
* start_time="2023-08-23T19:20:33+00:00" AND
* (event_types:"google.workspace.chat.space.v1.updated" OR
* event_types:"google.workspace.chat.message.v1.created") ``` The following queries are
* invalid: ``` start_time="2023-08-23T19:20:33+00:00" OR
* end_time="2023-08-23T19:21:54+00:00" ``` ```
* event_types:"google.workspace.chat.space.v1.updated" AND
* event_types:"google.workspace.chat.message.v1.created" ``` Invalid queries are rejected
* by the server with an `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Required. A query filter. You must specify at least one event type (`event_type`) using the has `:`
operator. To filter by multiple event types, use the `OR` operator. Omit batch event types in your
filter. The request automatically returns any related batch events. For example, if you filter by
new reactions (`google.workspace.chat.reaction.v1.created`), the server also returns batch new
reactions events (`google.workspace.chat.reaction.v1.batchCreated`). For a list of supported event
types, see the [`SpaceEvents` reference documentation](https://developers.google.com/workspace/chat
/api/reference/rest/v1/spaces.spaceEvents#SpaceEvent.FIELDS.event_type). Optionally, you can also
filter by start time (`start_time`) and end time (`end_time`): * `start_time`: Exclusive timestamp
from which to start listing space events. You can list events that occurred up to 28 days ago. If
unspecified, lists space events from the past 28 days. * `end_time`: Inclusive timestamp until
which space events are listed. If unspecified, lists events up to the time of the request. To
specify a start or end time, use the equals `=` operator and format in [RFC-3339](https://www.rfc-
editor.org/rfc/rfc3339). To filter by both `start_time` and `end_time`, use the `AND` operator. For
example, the following queries are valid: ``` start_time="2023-08-23T19:20:33+00:00" AND
end_time="2023-08-23T19:21:54+00:00" ``` ``` start_time="2023-08-23T19:20:33+00:00" AND
(event_types:"google.workspace.chat.space.v1.updated" OR
event_types:"google.workspace.chat.message.v1.created") ``` The following queries are invalid: ```
start_time="2023-08-23T19:20:33+00:00" OR end_time="2023-08-23T19:21:54+00:00" ``` ```
event_types:"google.workspace.chat.space.v1.updated" AND
event_types:"google.workspace.chat.message.v1.created" ``` Invalid queries are rejected by the
server with an `INVALID_ARGUMENT` error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Required. A query filter. You must specify at least one event type (`event_type`) using
* the has `:` operator. To filter by multiple event types, use the `OR` operator. Omit
* batch event types in your filter. The request automatically returns any related batch
* events. For example, if you filter by new reactions
* (`google.workspace.chat.reaction.v1.created`), the server also returns batch new
* reactions events (`google.workspace.chat.reaction.v1.batchCreated`). For a list of
* supported event types, see the [`SpaceEvents` reference documentation](https://developers
* .google.com/workspace/chat/api/reference/rest/v1/spaces.spaceEvents#SpaceEvent.FIELDS.eve
* nt_type). Optionally, you can also filter by start time (`start_time`) and end time
* (`end_time`): * `start_time`: Exclusive timestamp from which to start listing space
* events. You can list events that occurred up to 28 days ago. If unspecified, lists space
* events from the past 28 days. * `end_time`: Inclusive timestamp until which space events
* are listed. If unspecified, lists events up to the time of the request. To specify a
* start or end time, use the equals `=` operator and format in [RFC-3339](https://www.rfc-
* editor.org/rfc/rfc3339). To filter by both `start_time` and `end_time`, use the `AND`
* operator. For example, the following queries are valid: ```
* start_time="2023-08-23T19:20:33+00:00" AND end_time="2023-08-23T19:21:54+00:00" ``` ```
* start_time="2023-08-23T19:20:33+00:00" AND
* (event_types:"google.workspace.chat.space.v1.updated" OR
* event_types:"google.workspace.chat.message.v1.created") ``` The following queries are
* invalid: ``` start_time="2023-08-23T19:20:33+00:00" OR
* end_time="2023-08-23T19:21:54+00:00" ``` ```
* event_types:"google.workspace.chat.space.v1.updated" AND
* event_types:"google.workspace.chat.message.v1.created" ``` Invalid queries are rejected
* by the server with an `INVALID_ARGUMENT` error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of space events returned. The service might return fewer
* than this value. Negative values return an `INVALID_ARGUMENT` error.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of space events returned. The service might return fewer than this
value. Negative values return an `INVALID_ARGUMENT` error.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of space events returned. The service might return fewer
* than this value. Negative values return an `INVALID_ARGUMENT` error.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous list space events call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to list space events
* must match the call that provided the page token. Passing different values to the other
* parameters might lead to unexpected results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous list space events call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to list space events must match the
call that provided the page token. Passing different values to the other parameters might lead to
unexpected results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous list space events call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to list space events
* must match the call that provided the page token. Passing different values to the other
* parameters might lead to unexpected results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Users.List request = chat.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* An accessor for creating requests from the Spaces collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Spaces.List request = chat.spaces().list(parameters ...)}
*
*
* @return the resource collection
*/
public Spaces spaces() {
return new Spaces();
}
/**
* The "spaces" collection of methods.
*/
public class Spaces {
/**
* Returns details about a user's read state within a space, used to identify read and unread
* messages. For an example, see [Get details about a user's space read
* state](https://developers.google.com/workspace/chat/get-space-read-state). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "spaces.getSpaceReadState".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link GetSpaceReadState#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the space read state to retrieve. Only supports getting read state for
* the calling user. To refer to the calling user, set one of the following: - The `me`
* alias. For example, `users/me/spaces/{space}/spaceReadState`. - Their Workspace email
* address. For example, `users/[email protected]/spaces/{space}/spaceReadState`. - Their user
* id. For example, `users/123456789/spaces/{space}/spaceReadState`. Format:
* users/{user}/spaces/{space}/spaceReadState
* @return the request
*/
public GetSpaceReadState getSpaceReadState(java.lang.String name) throws java.io.IOException {
GetSpaceReadState result = new GetSpaceReadState(name);
initialize(result);
return result;
}
public class GetSpaceReadState extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^users/[^/]+/spaces/[^/]+/spaceReadState$");
/**
* Returns details about a user's read state within a space, used to identify read and unread
* messages. For an example, see [Get details about a user's space read
* state](https://developers.google.com/workspace/chat/get-space-read-state). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "spaces.getSpaceReadState".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link GetSpaceReadState#execute()} method to invoke the remote operation.
* {@link GetSpaceReadState#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. Resource name of the space read state to retrieve. Only supports getting read state for
* the calling user. To refer to the calling user, set one of the following: - The `me`
* alias. For example, `users/me/spaces/{space}/spaceReadState`. - Their Workspace email
* address. For example, `users/[email protected]/spaces/{space}/spaceReadState`. - Their user
* id. For example, `users/123456789/spaces/{space}/spaceReadState`. Format:
* users/{user}/spaces/{space}/spaceReadState
* @since 1.13
*/
protected GetSpaceReadState(java.lang.String name) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.SpaceReadState.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^users/[^/]+/spaces/[^/]+/spaceReadState$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSpaceReadState set$Xgafv(java.lang.String $Xgafv) {
return (GetSpaceReadState) super.set$Xgafv($Xgafv);
}
@Override
public GetSpaceReadState setAccessToken(java.lang.String accessToken) {
return (GetSpaceReadState) super.setAccessToken(accessToken);
}
@Override
public GetSpaceReadState setAlt(java.lang.String alt) {
return (GetSpaceReadState) super.setAlt(alt);
}
@Override
public GetSpaceReadState setCallback(java.lang.String callback) {
return (GetSpaceReadState) super.setCallback(callback);
}
@Override
public GetSpaceReadState setFields(java.lang.String fields) {
return (GetSpaceReadState) super.setFields(fields);
}
@Override
public GetSpaceReadState setKey(java.lang.String key) {
return (GetSpaceReadState) super.setKey(key);
}
@Override
public GetSpaceReadState setOauthToken(java.lang.String oauthToken) {
return (GetSpaceReadState) super.setOauthToken(oauthToken);
}
@Override
public GetSpaceReadState setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSpaceReadState) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSpaceReadState setQuotaUser(java.lang.String quotaUser) {
return (GetSpaceReadState) super.setQuotaUser(quotaUser);
}
@Override
public GetSpaceReadState setUploadType(java.lang.String uploadType) {
return (GetSpaceReadState) super.setUploadType(uploadType);
}
@Override
public GetSpaceReadState setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSpaceReadState) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the space read state to retrieve. Only supports getting read
* state for the calling user. To refer to the calling user, set one of the following: - The
* `me` alias. For example, `users/me/spaces/{space}/spaceReadState`. - Their Workspace
* email address. For example, `users/[email protected]/spaces/{space}/spaceReadState`. -
* Their user id. For example, `users/123456789/spaces/{space}/spaceReadState`. Format:
* users/{user}/spaces/{space}/spaceReadState
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the space read state to retrieve. Only supports getting read state for
the calling user. To refer to the calling user, set one of the following: - The `me` alias. For
example, `users/me/spaces/{space}/spaceReadState`. - Their Workspace email address. For example,
`users/[email protected]/spaces/{space}/spaceReadState`. - Their user id. For example,
`users/123456789/spaces/{space}/spaceReadState`. Format: users/{user}/spaces/{space}/spaceReadState
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the space read state to retrieve. Only supports getting read
* state for the calling user. To refer to the calling user, set one of the following: - The
* `me` alias. For example, `users/me/spaces/{space}/spaceReadState`. - Their Workspace
* email address. For example, `users/[email protected]/spaces/{space}/spaceReadState`. -
* Their user id. For example, `users/123456789/spaces/{space}/spaceReadState`. Format:
* users/{user}/spaces/{space}/spaceReadState
*/
public GetSpaceReadState setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^users/[^/]+/spaces/[^/]+/spaceReadState$");
}
this.name = name;
return this;
}
@Override
public GetSpaceReadState set(String parameterName, Object value) {
return (GetSpaceReadState) super.set(parameterName, value);
}
}
/**
* Updates a user's read state within a space, used to identify read and unread messages. For an
* example, see [Update a user's space read
* state](https://developers.google.com/workspace/chat/update-space-read-state). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "spaces.updateSpaceReadState".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link UpdateSpaceReadState#execute()} method to invoke the remote
* operation.
*
* @param name Resource name of the space read state. Format: `users/{user}/spaces/{space}/spaceReadState`
* @param content the {@link com.google.api.services.chat.v1.model.SpaceReadState}
* @return the request
*/
public UpdateSpaceReadState updateSpaceReadState(java.lang.String name, com.google.api.services.chat.v1.model.SpaceReadState content) throws java.io.IOException {
UpdateSpaceReadState result = new UpdateSpaceReadState(name, content);
initialize(result);
return result;
}
public class UpdateSpaceReadState extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^users/[^/]+/spaces/[^/]+/spaceReadState$");
/**
* Updates a user's read state within a space, used to identify read and unread messages. For an
* example, see [Update a user's space read
* state](https://developers.google.com/workspace/chat/update-space-read-state). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "spaces.updateSpaceReadState".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link UpdateSpaceReadState#execute()} method to invoke the remote
* operation. {@link UpdateSpaceReadState#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Resource name of the space read state. Format: `users/{user}/spaces/{space}/spaceReadState`
* @param content the {@link com.google.api.services.chat.v1.model.SpaceReadState}
* @since 1.13
*/
protected UpdateSpaceReadState(java.lang.String name, com.google.api.services.chat.v1.model.SpaceReadState content) {
super(HangoutsChat.this, "PATCH", REST_PATH, content, com.google.api.services.chat.v1.model.SpaceReadState.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^users/[^/]+/spaces/[^/]+/spaceReadState$");
}
}
@Override
public UpdateSpaceReadState set$Xgafv(java.lang.String $Xgafv) {
return (UpdateSpaceReadState) super.set$Xgafv($Xgafv);
}
@Override
public UpdateSpaceReadState setAccessToken(java.lang.String accessToken) {
return (UpdateSpaceReadState) super.setAccessToken(accessToken);
}
@Override
public UpdateSpaceReadState setAlt(java.lang.String alt) {
return (UpdateSpaceReadState) super.setAlt(alt);
}
@Override
public UpdateSpaceReadState setCallback(java.lang.String callback) {
return (UpdateSpaceReadState) super.setCallback(callback);
}
@Override
public UpdateSpaceReadState setFields(java.lang.String fields) {
return (UpdateSpaceReadState) super.setFields(fields);
}
@Override
public UpdateSpaceReadState setKey(java.lang.String key) {
return (UpdateSpaceReadState) super.setKey(key);
}
@Override
public UpdateSpaceReadState setOauthToken(java.lang.String oauthToken) {
return (UpdateSpaceReadState) super.setOauthToken(oauthToken);
}
@Override
public UpdateSpaceReadState setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateSpaceReadState) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateSpaceReadState setQuotaUser(java.lang.String quotaUser) {
return (UpdateSpaceReadState) super.setQuotaUser(quotaUser);
}
@Override
public UpdateSpaceReadState setUploadType(java.lang.String uploadType) {
return (UpdateSpaceReadState) super.setUploadType(uploadType);
}
@Override
public UpdateSpaceReadState setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateSpaceReadState) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name of the space read state. Format:
* `users/{user}/spaces/{space}/spaceReadState`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name of the space read state. Format: `users/{user}/spaces/{space}/spaceReadState`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the space read state. Format:
* `users/{user}/spaces/{space}/spaceReadState`
*/
public UpdateSpaceReadState setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^users/[^/]+/spaces/[^/]+/spaceReadState$");
}
this.name = name;
return this;
}
/**
* Required. The field paths to update. Currently supported field paths: - `last_read_time`
* When the `last_read_time` is before the latest message create time, the space appears as
* unread in the UI. To mark the space as read, set `last_read_time` to any value later
* (larger) than the latest message create time. The `last_read_time` is coerced to match
* the latest message create time. Note that the space read state only affects the read
* state of messages that are visible in the space's top-level conversation. Replies in
* threads are unaffected by this timestamp, and instead rely on the thread read state.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The field paths to update. Currently supported field paths: - `last_read_time` When the
`last_read_time` is before the latest message create time, the space appears as unread in the UI.
To mark the space as read, set `last_read_time` to any value later (larger) than the latest message
create time. The `last_read_time` is coerced to match the latest message create time. Note that the
space read state only affects the read state of messages that are visible in the space's top-level
conversation. Replies in threads are unaffected by this timestamp, and instead rely on the thread
read state.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The field paths to update. Currently supported field paths: - `last_read_time`
* When the `last_read_time` is before the latest message create time, the space appears as
* unread in the UI. To mark the space as read, set `last_read_time` to any value later
* (larger) than the latest message create time. The `last_read_time` is coerced to match
* the latest message create time. Note that the space read state only affects the read
* state of messages that are visible in the space's top-level conversation. Replies in
* threads are unaffected by this timestamp, and instead rely on the thread read state.
*/
public UpdateSpaceReadState setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateSpaceReadState set(String parameterName, Object value) {
return (UpdateSpaceReadState) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Threads collection.
*
* The typical use is:
*
* {@code HangoutsChat chat = new HangoutsChat(...);}
* {@code HangoutsChat.Threads.List request = chat.threads().list(parameters ...)}
*
*
* @return the resource collection
*/
public Threads threads() {
return new Threads();
}
/**
* The "threads" collection of methods.
*/
public class Threads {
/**
* Returns details about a user's read state within a thread, used to identify read and unread
* messages. For an example, see [Get details about a user's thread read
* state](https://developers.google.com/workspace/chat/get-thread-read-state). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "threads.getThreadReadState".
*
* This request holds the parameters needed by the chat server. After setting any optional
* parameters, call the {@link GetThreadReadState#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the thread read state to retrieve. Only supports getting read state for
* the calling user. To refer to the calling user, set one of the following: - The `me`
* alias. For example, `users/me/spaces/{space}/threads/{thread}/threadReadState`. - Their
* Workspace email address. For example,
* `users/[email protected]/spaces/{space}/threads/{thread}/threadReadState`. - Their user id.
* For example, `users/123456789/spaces/{space}/threads/{thread}/threadReadState`. Format:
* users/{user}/spaces/{space}/threads/{thread}/threadReadState
* @return the request
*/
public GetThreadReadState getThreadReadState(java.lang.String name) throws java.io.IOException {
GetThreadReadState result = new GetThreadReadState(name);
initialize(result);
return result;
}
public class GetThreadReadState extends HangoutsChatRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^users/[^/]+/spaces/[^/]+/threads/[^/]+/threadReadState$");
/**
* Returns details about a user's read state within a thread, used to identify read and unread
* messages. For an example, see [Get details about a user's thread read
* state](https://developers.google.com/workspace/chat/get-thread-read-state). Requires [user
* authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user).
*
* Create a request for the method "threads.getThreadReadState".
*
* This request holds the parameters needed by the the chat server. After setting any optional
* parameters, call the {@link GetThreadReadState#execute()} method to invoke the remote
* operation. {@link GetThreadReadState#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. Resource name of the thread read state to retrieve. Only supports getting read state for
* the calling user. To refer to the calling user, set one of the following: - The `me`
* alias. For example, `users/me/spaces/{space}/threads/{thread}/threadReadState`. - Their
* Workspace email address. For example,
* `users/[email protected]/spaces/{space}/threads/{thread}/threadReadState`. - Their user id.
* For example, `users/123456789/spaces/{space}/threads/{thread}/threadReadState`. Format:
* users/{user}/spaces/{space}/threads/{thread}/threadReadState
* @since 1.13
*/
protected GetThreadReadState(java.lang.String name) {
super(HangoutsChat.this, "GET", REST_PATH, null, com.google.api.services.chat.v1.model.ThreadReadState.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^users/[^/]+/spaces/[^/]+/threads/[^/]+/threadReadState$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetThreadReadState set$Xgafv(java.lang.String $Xgafv) {
return (GetThreadReadState) super.set$Xgafv($Xgafv);
}
@Override
public GetThreadReadState setAccessToken(java.lang.String accessToken) {
return (GetThreadReadState) super.setAccessToken(accessToken);
}
@Override
public GetThreadReadState setAlt(java.lang.String alt) {
return (GetThreadReadState) super.setAlt(alt);
}
@Override
public GetThreadReadState setCallback(java.lang.String callback) {
return (GetThreadReadState) super.setCallback(callback);
}
@Override
public GetThreadReadState setFields(java.lang.String fields) {
return (GetThreadReadState) super.setFields(fields);
}
@Override
public GetThreadReadState setKey(java.lang.String key) {
return (GetThreadReadState) super.setKey(key);
}
@Override
public GetThreadReadState setOauthToken(java.lang.String oauthToken) {
return (GetThreadReadState) super.setOauthToken(oauthToken);
}
@Override
public GetThreadReadState setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetThreadReadState) super.setPrettyPrint(prettyPrint);
}
@Override
public GetThreadReadState setQuotaUser(java.lang.String quotaUser) {
return (GetThreadReadState) super.setQuotaUser(quotaUser);
}
@Override
public GetThreadReadState setUploadType(java.lang.String uploadType) {
return (GetThreadReadState) super.setUploadType(uploadType);
}
@Override
public GetThreadReadState setUploadProtocol(java.lang.String uploadProtocol) {
return (GetThreadReadState) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the thread read state to retrieve. Only supports getting
* read state for the calling user. To refer to the calling user, set one of the
* following: - The `me` alias. For example,
* `users/me/spaces/{space}/threads/{thread}/threadReadState`. - Their Workspace email
* address. For example,
* `users/[email protected]/spaces/{space}/threads/{thread}/threadReadState`. - Their user
* id. For example, `users/123456789/spaces/{space}/threads/{thread}/threadReadState`.
* Format: users/{user}/spaces/{space}/threads/{thread}/threadReadState
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the thread read state to retrieve. Only supports getting read state for
the calling user. To refer to the calling user, set one of the following: - The `me` alias. For
example, `users/me/spaces/{space}/threads/{thread}/threadReadState`. - Their Workspace email
address. For example, `users/[email protected]/spaces/{space}/threads/{thread}/threadReadState`. -
Their user id. For example, `users/123456789/spaces/{space}/threads/{thread}/threadReadState`.
Format: users/{user}/spaces/{space}/threads/{thread}/threadReadState
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the thread read state to retrieve. Only supports getting
* read state for the calling user. To refer to the calling user, set one of the
* following: - The `me` alias. For example,
* `users/me/spaces/{space}/threads/{thread}/threadReadState`. - Their Workspace email
* address. For example,
* `users/[email protected]/spaces/{space}/threads/{thread}/threadReadState`. - Their user
* id. For example, `users/123456789/spaces/{space}/threads/{thread}/threadReadState`.
* Format: users/{user}/spaces/{space}/threads/{thread}/threadReadState
*/
public GetThreadReadState setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^users/[^/]+/spaces/[^/]+/threads/[^/]+/threadReadState$");
}
this.name = name;
return this;
}
@Override
public GetThreadReadState set(String parameterName, Object value) {
return (GetThreadReadState) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link HangoutsChat}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link HangoutsChat}. */
@Override
public HangoutsChat build() {
return new HangoutsChat(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link HangoutsChatRequestInitializer}.
*
* @since 1.12
*/
public Builder setHangoutsChatRequestInitializer(
HangoutsChatRequestInitializer hangoutschatRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(hangoutschatRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}