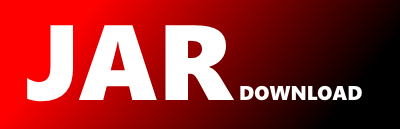
com.google.api.services.chat.v1.model.AccessSettings Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* Represents the [access setting](https://support.google.com/chat/answer/11971020) of the space.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AccessSettings extends com.google.api.client.json.GenericJson {
/**
* Output only. Indicates the access state of the space.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String accessState;
/**
* Optional. The resource name of the [target
* audience](https://support.google.com/a/answer/9934697) who can discover the space, join the
* space, and preview the messages in the space. If unset, only users or Google Groups who have
* been individually invited or added to the space can access it. For details, see [Make a space
* discoverable to a target audience](https://developers.google.com/workspace/chat/space-target-
* audience). Format: `audiences/{audience}` To use the default target audience for the Google
* Workspace organization, set to `audiences/default`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String audience;
/**
* Output only. Indicates the access state of the space.
* @return value or {@code null} for none
*/
public java.lang.String getAccessState() {
return accessState;
}
/**
* Output only. Indicates the access state of the space.
* @param accessState accessState or {@code null} for none
*/
public AccessSettings setAccessState(java.lang.String accessState) {
this.accessState = accessState;
return this;
}
/**
* Optional. The resource name of the [target
* audience](https://support.google.com/a/answer/9934697) who can discover the space, join the
* space, and preview the messages in the space. If unset, only users or Google Groups who have
* been individually invited or added to the space can access it. For details, see [Make a space
* discoverable to a target audience](https://developers.google.com/workspace/chat/space-target-
* audience). Format: `audiences/{audience}` To use the default target audience for the Google
* Workspace organization, set to `audiences/default`.
* @return value or {@code null} for none
*/
public java.lang.String getAudience() {
return audience;
}
/**
* Optional. The resource name of the [target
* audience](https://support.google.com/a/answer/9934697) who can discover the space, join the
* space, and preview the messages in the space. If unset, only users or Google Groups who have
* been individually invited or added to the space can access it. For details, see [Make a space
* discoverable to a target audience](https://developers.google.com/workspace/chat/space-target-
* audience). Format: `audiences/{audience}` To use the default target audience for the Google
* Workspace organization, set to `audiences/default`.
* @param audience audience or {@code null} for none
*/
public AccessSettings setAudience(java.lang.String audience) {
this.audience = audience;
return this;
}
@Override
public AccessSettings set(String fieldName, Object value) {
return (AccessSettings) super.set(fieldName, value);
}
@Override
public AccessSettings clone() {
return (AccessSettings) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy