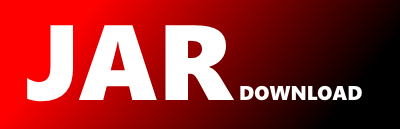
com.google.api.services.chat.v1.model.CommonEventObject Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* Represents information about the user's client, such as locale, host app, and platform. For Chat
* apps, `CommonEventObject` includes data submitted by users interacting with cards, like data
* entered in [dialogs](https://developers.google.com/chat/how-tos/dialogs).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CommonEventObject extends com.google.api.client.json.GenericJson {
/**
* A map containing the values that a user inputs in a widget from a card or dialog. The map keys
* are the string IDs assigned to each widget, and the values represent inputs to the widget. For
* details, see [Process information inputted by
* users](https://developers.google.com/chat/ui/read-form-data).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map formInputs;
/**
* The hostApp enum which indicates the app the add-on is invoked from. Always `CHAT` for Chat
* apps.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hostApp;
/**
* Name of the invoked function associated with the widget. Only set for Chat apps.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String invokedFunction;
/**
* Custom [parameters](/chat/api/reference/rest/v1/cards#ActionParameter) passed to the invoked
* function. Both keys and values must be strings.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map parameters;
/**
* The platform enum which indicates the platform where the event originates (`WEB`, `IOS`, or
* `ANDROID`). Not supported by Chat apps.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String platform;
/**
* The timezone ID and offset from Coordinated Universal Time (UTC). Only supported for the event
* types [`CARD_CLICKED`](https://developers.google.com/chat/api/reference/rest/v1/EventType#ENUM_
* VALUES.CARD_CLICKED) and [`SUBMIT_DIALOG`](https://developers.google.com/chat/api/reference/res
* t/v1/DialogEventType#ENUM_VALUES.SUBMIT_DIALOG).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TimeZone timeZone;
/**
* The full `locale.displayName` in the format of [ISO 639 language code]-[ISO 3166 country/region
* code] such as "en-US".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String userLocale;
/**
* A map containing the values that a user inputs in a widget from a card or dialog. The map keys
* are the string IDs assigned to each widget, and the values represent inputs to the widget. For
* details, see [Process information inputted by
* users](https://developers.google.com/chat/ui/read-form-data).
* @return value or {@code null} for none
*/
public java.util.Map getFormInputs() {
return formInputs;
}
/**
* A map containing the values that a user inputs in a widget from a card or dialog. The map keys
* are the string IDs assigned to each widget, and the values represent inputs to the widget. For
* details, see [Process information inputted by
* users](https://developers.google.com/chat/ui/read-form-data).
* @param formInputs formInputs or {@code null} for none
*/
public CommonEventObject setFormInputs(java.util.Map formInputs) {
this.formInputs = formInputs;
return this;
}
/**
* The hostApp enum which indicates the app the add-on is invoked from. Always `CHAT` for Chat
* apps.
* @return value or {@code null} for none
*/
public java.lang.String getHostApp() {
return hostApp;
}
/**
* The hostApp enum which indicates the app the add-on is invoked from. Always `CHAT` for Chat
* apps.
* @param hostApp hostApp or {@code null} for none
*/
public CommonEventObject setHostApp(java.lang.String hostApp) {
this.hostApp = hostApp;
return this;
}
/**
* Name of the invoked function associated with the widget. Only set for Chat apps.
* @return value or {@code null} for none
*/
public java.lang.String getInvokedFunction() {
return invokedFunction;
}
/**
* Name of the invoked function associated with the widget. Only set for Chat apps.
* @param invokedFunction invokedFunction or {@code null} for none
*/
public CommonEventObject setInvokedFunction(java.lang.String invokedFunction) {
this.invokedFunction = invokedFunction;
return this;
}
/**
* Custom [parameters](/chat/api/reference/rest/v1/cards#ActionParameter) passed to the invoked
* function. Both keys and values must be strings.
* @return value or {@code null} for none
*/
public java.util.Map getParameters() {
return parameters;
}
/**
* Custom [parameters](/chat/api/reference/rest/v1/cards#ActionParameter) passed to the invoked
* function. Both keys and values must be strings.
* @param parameters parameters or {@code null} for none
*/
public CommonEventObject setParameters(java.util.Map parameters) {
this.parameters = parameters;
return this;
}
/**
* The platform enum which indicates the platform where the event originates (`WEB`, `IOS`, or
* `ANDROID`). Not supported by Chat apps.
* @return value or {@code null} for none
*/
public java.lang.String getPlatform() {
return platform;
}
/**
* The platform enum which indicates the platform where the event originates (`WEB`, `IOS`, or
* `ANDROID`). Not supported by Chat apps.
* @param platform platform or {@code null} for none
*/
public CommonEventObject setPlatform(java.lang.String platform) {
this.platform = platform;
return this;
}
/**
* The timezone ID and offset from Coordinated Universal Time (UTC). Only supported for the event
* types [`CARD_CLICKED`](https://developers.google.com/chat/api/reference/rest/v1/EventType#ENUM_
* VALUES.CARD_CLICKED) and [`SUBMIT_DIALOG`](https://developers.google.com/chat/api/reference/res
* t/v1/DialogEventType#ENUM_VALUES.SUBMIT_DIALOG).
* @return value or {@code null} for none
*/
public TimeZone getTimeZone() {
return timeZone;
}
/**
* The timezone ID and offset from Coordinated Universal Time (UTC). Only supported for the event
* types [`CARD_CLICKED`](https://developers.google.com/chat/api/reference/rest/v1/EventType#ENUM_
* VALUES.CARD_CLICKED) and [`SUBMIT_DIALOG`](https://developers.google.com/chat/api/reference/res
* t/v1/DialogEventType#ENUM_VALUES.SUBMIT_DIALOG).
* @param timeZone timeZone or {@code null} for none
*/
public CommonEventObject setTimeZone(TimeZone timeZone) {
this.timeZone = timeZone;
return this;
}
/**
* The full `locale.displayName` in the format of [ISO 639 language code]-[ISO 3166 country/region
* code] such as "en-US".
* @return value or {@code null} for none
*/
public java.lang.String getUserLocale() {
return userLocale;
}
/**
* The full `locale.displayName` in the format of [ISO 639 language code]-[ISO 3166 country/region
* code] such as "en-US".
* @param userLocale userLocale or {@code null} for none
*/
public CommonEventObject setUserLocale(java.lang.String userLocale) {
this.userLocale = userLocale;
return this;
}
@Override
public CommonEventObject set(String fieldName, Object value) {
return (CommonEventObject) super.set(fieldName, value);
}
@Override
public CommonEventObject clone() {
return (CommonEventObject) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy