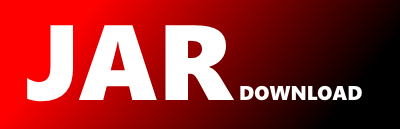
com.google.api.services.chat.v1.model.DeprecatedEvent Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* A Google Chat app interaction event that represents and contains data about a user's interaction
* with a Chat app. To configure your Chat app to receive interaction events, see [Receive and
* respond to user interactions](https://developers.google.com/workspace/chat/receive-respond-
* interactions). In addition to receiving events from user interactions, Chat apps can receive
* events about changes to spaces, such as when a new member is added to a space. To learn about
* space events, see [Work with events from Google
* Chat](https://developers.google.com/workspace/chat/events-overview).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DeprecatedEvent extends com.google.api.client.json.GenericJson {
/**
* For `CARD_CLICKED` interaction events, the form action data associated when a user clicks a
* card or dialog. To learn more, see [Read form data input by users on
* cards](https://developers.google.com/workspace/chat/read-form-data).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FormAction action;
/**
* Represents information about the user's client, such as locale, host app, and platform. For
* Chat apps, `CommonEventObject` includes information submitted by users interacting with
* [dialogs](https://developers.google.com/workspace/chat/dialogs), like data entered on a card.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CommonEventObject common;
/**
* For `MESSAGE` interaction events, the URL that users must be redirected to after they complete
* an authorization or configuration flow outside of Google Chat. For more information, see
* [Connect a Chat app with other services and
* tools](https://developers.google.com/workspace/chat/connect-web-services-tools).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String configCompleteRedirectUrl;
/**
* The type of [dialog](https://developers.google.com/workspace/chat/dialogs) interaction event
* received.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dialogEventType;
/**
* The timestamp indicating when the interaction event occurred.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String eventTime;
/**
* For `CARD_CLICKED` and `MESSAGE` interaction events, whether the user is interacting with or
* about to interact with a [dialog](https://developers.google.com/workspace/chat/dialogs).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isDialogEvent;
/**
* For `ADDED_TO_SPACE`, `CARD_CLICKED`, and `MESSAGE` interaction events, the message that
* triggered the interaction event, if applicable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Message message;
/**
* The space in which the user interacted with the Chat app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Space space;
/**
* The Chat app-defined key for the thread related to the interaction event. See [`spaces.messages
* .thread.threadKey`](/chat/api/reference/rest/v1/spaces.messages#Thread.FIELDS.thread_key) for
* more information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String threadKey;
/**
* A secret value that legacy Chat apps can use to verify if a request is from Google. Google
* randomly generates the token, and its value remains static. You can obtain, revoke, or
* regenerate the token from the [Chat API configuration
* page](https://console.cloud.google.com/apis/api/chat.googleapis.com/hangouts-chat) in the
* Google Cloud Console. Modern Chat apps don't use this field. It is absent from API responses
* and the [Chat API configuration
* page](https://console.cloud.google.com/apis/api/chat.googleapis.com/hangouts-chat).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String token;
/**
* The [type](/workspace/chat/api/reference/rest/v1/EventType) of user interaction with the Chat
* app, such as `MESSAGE` or `ADDED_TO_SPACE`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The user that interacted with the Chat app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private User user;
/**
* For `CARD_CLICKED` interaction events, the form action data associated when a user clicks a
* card or dialog. To learn more, see [Read form data input by users on
* cards](https://developers.google.com/workspace/chat/read-form-data).
* @return value or {@code null} for none
*/
public FormAction getAction() {
return action;
}
/**
* For `CARD_CLICKED` interaction events, the form action data associated when a user clicks a
* card or dialog. To learn more, see [Read form data input by users on
* cards](https://developers.google.com/workspace/chat/read-form-data).
* @param action action or {@code null} for none
*/
public DeprecatedEvent setAction(FormAction action) {
this.action = action;
return this;
}
/**
* Represents information about the user's client, such as locale, host app, and platform. For
* Chat apps, `CommonEventObject` includes information submitted by users interacting with
* [dialogs](https://developers.google.com/workspace/chat/dialogs), like data entered on a card.
* @return value or {@code null} for none
*/
public CommonEventObject getCommon() {
return common;
}
/**
* Represents information about the user's client, such as locale, host app, and platform. For
* Chat apps, `CommonEventObject` includes information submitted by users interacting with
* [dialogs](https://developers.google.com/workspace/chat/dialogs), like data entered on a card.
* @param common common or {@code null} for none
*/
public DeprecatedEvent setCommon(CommonEventObject common) {
this.common = common;
return this;
}
/**
* For `MESSAGE` interaction events, the URL that users must be redirected to after they complete
* an authorization or configuration flow outside of Google Chat. For more information, see
* [Connect a Chat app with other services and
* tools](https://developers.google.com/workspace/chat/connect-web-services-tools).
* @return value or {@code null} for none
*/
public java.lang.String getConfigCompleteRedirectUrl() {
return configCompleteRedirectUrl;
}
/**
* For `MESSAGE` interaction events, the URL that users must be redirected to after they complete
* an authorization or configuration flow outside of Google Chat. For more information, see
* [Connect a Chat app with other services and
* tools](https://developers.google.com/workspace/chat/connect-web-services-tools).
* @param configCompleteRedirectUrl configCompleteRedirectUrl or {@code null} for none
*/
public DeprecatedEvent setConfigCompleteRedirectUrl(java.lang.String configCompleteRedirectUrl) {
this.configCompleteRedirectUrl = configCompleteRedirectUrl;
return this;
}
/**
* The type of [dialog](https://developers.google.com/workspace/chat/dialogs) interaction event
* received.
* @return value or {@code null} for none
*/
public java.lang.String getDialogEventType() {
return dialogEventType;
}
/**
* The type of [dialog](https://developers.google.com/workspace/chat/dialogs) interaction event
* received.
* @param dialogEventType dialogEventType or {@code null} for none
*/
public DeprecatedEvent setDialogEventType(java.lang.String dialogEventType) {
this.dialogEventType = dialogEventType;
return this;
}
/**
* The timestamp indicating when the interaction event occurred.
* @return value or {@code null} for none
*/
public String getEventTime() {
return eventTime;
}
/**
* The timestamp indicating when the interaction event occurred.
* @param eventTime eventTime or {@code null} for none
*/
public DeprecatedEvent setEventTime(String eventTime) {
this.eventTime = eventTime;
return this;
}
/**
* For `CARD_CLICKED` and `MESSAGE` interaction events, whether the user is interacting with or
* about to interact with a [dialog](https://developers.google.com/workspace/chat/dialogs).
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsDialogEvent() {
return isDialogEvent;
}
/**
* For `CARD_CLICKED` and `MESSAGE` interaction events, whether the user is interacting with or
* about to interact with a [dialog](https://developers.google.com/workspace/chat/dialogs).
* @param isDialogEvent isDialogEvent or {@code null} for none
*/
public DeprecatedEvent setIsDialogEvent(java.lang.Boolean isDialogEvent) {
this.isDialogEvent = isDialogEvent;
return this;
}
/**
* For `ADDED_TO_SPACE`, `CARD_CLICKED`, and `MESSAGE` interaction events, the message that
* triggered the interaction event, if applicable.
* @return value or {@code null} for none
*/
public Message getMessage() {
return message;
}
/**
* For `ADDED_TO_SPACE`, `CARD_CLICKED`, and `MESSAGE` interaction events, the message that
* triggered the interaction event, if applicable.
* @param message message or {@code null} for none
*/
public DeprecatedEvent setMessage(Message message) {
this.message = message;
return this;
}
/**
* The space in which the user interacted with the Chat app.
* @return value or {@code null} for none
*/
public Space getSpace() {
return space;
}
/**
* The space in which the user interacted with the Chat app.
* @param space space or {@code null} for none
*/
public DeprecatedEvent setSpace(Space space) {
this.space = space;
return this;
}
/**
* The Chat app-defined key for the thread related to the interaction event. See [`spaces.messages
* .thread.threadKey`](/chat/api/reference/rest/v1/spaces.messages#Thread.FIELDS.thread_key) for
* more information.
* @return value or {@code null} for none
*/
public java.lang.String getThreadKey() {
return threadKey;
}
/**
* The Chat app-defined key for the thread related to the interaction event. See [`spaces.messages
* .thread.threadKey`](/chat/api/reference/rest/v1/spaces.messages#Thread.FIELDS.thread_key) for
* more information.
* @param threadKey threadKey or {@code null} for none
*/
public DeprecatedEvent setThreadKey(java.lang.String threadKey) {
this.threadKey = threadKey;
return this;
}
/**
* A secret value that legacy Chat apps can use to verify if a request is from Google. Google
* randomly generates the token, and its value remains static. You can obtain, revoke, or
* regenerate the token from the [Chat API configuration
* page](https://console.cloud.google.com/apis/api/chat.googleapis.com/hangouts-chat) in the
* Google Cloud Console. Modern Chat apps don't use this field. It is absent from API responses
* and the [Chat API configuration
* page](https://console.cloud.google.com/apis/api/chat.googleapis.com/hangouts-chat).
* @return value or {@code null} for none
*/
public java.lang.String getToken() {
return token;
}
/**
* A secret value that legacy Chat apps can use to verify if a request is from Google. Google
* randomly generates the token, and its value remains static. You can obtain, revoke, or
* regenerate the token from the [Chat API configuration
* page](https://console.cloud.google.com/apis/api/chat.googleapis.com/hangouts-chat) in the
* Google Cloud Console. Modern Chat apps don't use this field. It is absent from API responses
* and the [Chat API configuration
* page](https://console.cloud.google.com/apis/api/chat.googleapis.com/hangouts-chat).
* @param token token or {@code null} for none
*/
public DeprecatedEvent setToken(java.lang.String token) {
this.token = token;
return this;
}
/**
* The [type](/workspace/chat/api/reference/rest/v1/EventType) of user interaction with the Chat
* app, such as `MESSAGE` or `ADDED_TO_SPACE`.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The [type](/workspace/chat/api/reference/rest/v1/EventType) of user interaction with the Chat
* app, such as `MESSAGE` or `ADDED_TO_SPACE`.
* @param type type or {@code null} for none
*/
public DeprecatedEvent setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* The user that interacted with the Chat app.
* @return value or {@code null} for none
*/
public User getUser() {
return user;
}
/**
* The user that interacted with the Chat app.
* @param user user or {@code null} for none
*/
public DeprecatedEvent setUser(User user) {
this.user = user;
return this;
}
@Override
public DeprecatedEvent set(String fieldName, Object value) {
return (DeprecatedEvent) super.set(fieldName, value);
}
@Override
public DeprecatedEvent clone() {
return (DeprecatedEvent) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy