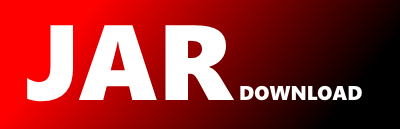
com.google.api.services.chat.v1.model.GoogleAppsCardV1Action Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* An action that describes the behavior when the form is submitted. For example, you can invoke an
* Apps Script script to handle the form. If the action is triggered, the form values are sent to
* the server. [Google Workspace Add-ons and Chat
* apps](https://developers.google.com/workspace/extend):
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1Action extends com.google.api.client.json.GenericJson {
/**
* A custom function to invoke when the containing element is clicked or otherwise activated. For
* example usage, see [Read form data](https://developers.google.com/workspace/chat/read-form-
* data).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String function;
/**
* Optional. Required when opening a
* [dialog](https://developers.google.com/workspace/chat/dialogs). What to do in response to an
* interaction with a user, such as a user clicking a button in a card message. If unspecified,
* the app responds by executing an `action`—like opening a link or running a function—as normal.
* By specifying an `interaction`, the app can respond in special interactive ways. For example,
* by setting `interaction` to `OPEN_DIALOG`, the app can open a
* [dialog](https://developers.google.com/workspace/chat/dialogs). When specified, a loading
* indicator isn't shown. If specified for an add-on, the entire card is stripped and nothing is
* shown in the client. [Google Chat apps](https://developers.google.com/workspace/chat):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String interaction;
/**
* Specifies the loading indicator that the action displays while making the call to the action.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String loadIndicator;
/**
* List of action parameters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List parameters;
/**
* Indicates whether form values persist after the action. The default value is `false`. If
* `true`, form values remain after the action is triggered. To let the user make changes while
* the action is being processed, set
* [`LoadIndicator`](https://developers.google.com/workspace/add-
* ons/reference/rpc/google.apps.card.v1#loadindicator) to `NONE`. For [card
* messages](https://developers.google.com/workspace/chat/api/guides/v1/messages/create#create) in
* Chat apps, you must also set the action's [`ResponseType`](https://developers.google.com/worksp
* ace/chat/api/reference/rest/v1/spaces.messages#responsetype) to `UPDATE_MESSAGE` and use the
* same [`card_id`](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces.mess
* ages#CardWithId) from the card that contained the action. If `false`, the form values are
* cleared when the action is triggered. To prevent the user from making changes while the action
* is being processed, set [`LoadIndicator`](https://developers.google.com/workspace/add-
* ons/reference/rpc/google.apps.card.v1#loadindicator) to `SPINNER`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean persistValues;
/**
* A custom function to invoke when the containing element is clicked or otherwise activated. For
* example usage, see [Read form data](https://developers.google.com/workspace/chat/read-form-
* data).
* @return value or {@code null} for none
*/
public java.lang.String getFunction() {
return function;
}
/**
* A custom function to invoke when the containing element is clicked or otherwise activated. For
* example usage, see [Read form data](https://developers.google.com/workspace/chat/read-form-
* data).
* @param function function or {@code null} for none
*/
public GoogleAppsCardV1Action setFunction(java.lang.String function) {
this.function = function;
return this;
}
/**
* Optional. Required when opening a
* [dialog](https://developers.google.com/workspace/chat/dialogs). What to do in response to an
* interaction with a user, such as a user clicking a button in a card message. If unspecified,
* the app responds by executing an `action`—like opening a link or running a function—as normal.
* By specifying an `interaction`, the app can respond in special interactive ways. For example,
* by setting `interaction` to `OPEN_DIALOG`, the app can open a
* [dialog](https://developers.google.com/workspace/chat/dialogs). When specified, a loading
* indicator isn't shown. If specified for an add-on, the entire card is stripped and nothing is
* shown in the client. [Google Chat apps](https://developers.google.com/workspace/chat):
* @return value or {@code null} for none
*/
public java.lang.String getInteraction() {
return interaction;
}
/**
* Optional. Required when opening a
* [dialog](https://developers.google.com/workspace/chat/dialogs). What to do in response to an
* interaction with a user, such as a user clicking a button in a card message. If unspecified,
* the app responds by executing an `action`—like opening a link or running a function—as normal.
* By specifying an `interaction`, the app can respond in special interactive ways. For example,
* by setting `interaction` to `OPEN_DIALOG`, the app can open a
* [dialog](https://developers.google.com/workspace/chat/dialogs). When specified, a loading
* indicator isn't shown. If specified for an add-on, the entire card is stripped and nothing is
* shown in the client. [Google Chat apps](https://developers.google.com/workspace/chat):
* @param interaction interaction or {@code null} for none
*/
public GoogleAppsCardV1Action setInteraction(java.lang.String interaction) {
this.interaction = interaction;
return this;
}
/**
* Specifies the loading indicator that the action displays while making the call to the action.
* @return value or {@code null} for none
*/
public java.lang.String getLoadIndicator() {
return loadIndicator;
}
/**
* Specifies the loading indicator that the action displays while making the call to the action.
* @param loadIndicator loadIndicator or {@code null} for none
*/
public GoogleAppsCardV1Action setLoadIndicator(java.lang.String loadIndicator) {
this.loadIndicator = loadIndicator;
return this;
}
/**
* List of action parameters.
* @return value or {@code null} for none
*/
public java.util.List getParameters() {
return parameters;
}
/**
* List of action parameters.
* @param parameters parameters or {@code null} for none
*/
public GoogleAppsCardV1Action setParameters(java.util.List parameters) {
this.parameters = parameters;
return this;
}
/**
* Indicates whether form values persist after the action. The default value is `false`. If
* `true`, form values remain after the action is triggered. To let the user make changes while
* the action is being processed, set
* [`LoadIndicator`](https://developers.google.com/workspace/add-
* ons/reference/rpc/google.apps.card.v1#loadindicator) to `NONE`. For [card
* messages](https://developers.google.com/workspace/chat/api/guides/v1/messages/create#create) in
* Chat apps, you must also set the action's [`ResponseType`](https://developers.google.com/worksp
* ace/chat/api/reference/rest/v1/spaces.messages#responsetype) to `UPDATE_MESSAGE` and use the
* same [`card_id`](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces.mess
* ages#CardWithId) from the card that contained the action. If `false`, the form values are
* cleared when the action is triggered. To prevent the user from making changes while the action
* is being processed, set [`LoadIndicator`](https://developers.google.com/workspace/add-
* ons/reference/rpc/google.apps.card.v1#loadindicator) to `SPINNER`.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPersistValues() {
return persistValues;
}
/**
* Indicates whether form values persist after the action. The default value is `false`. If
* `true`, form values remain after the action is triggered. To let the user make changes while
* the action is being processed, set
* [`LoadIndicator`](https://developers.google.com/workspace/add-
* ons/reference/rpc/google.apps.card.v1#loadindicator) to `NONE`. For [card
* messages](https://developers.google.com/workspace/chat/api/guides/v1/messages/create#create) in
* Chat apps, you must also set the action's [`ResponseType`](https://developers.google.com/worksp
* ace/chat/api/reference/rest/v1/spaces.messages#responsetype) to `UPDATE_MESSAGE` and use the
* same [`card_id`](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces.mess
* ages#CardWithId) from the card that contained the action. If `false`, the form values are
* cleared when the action is triggered. To prevent the user from making changes while the action
* is being processed, set [`LoadIndicator`](https://developers.google.com/workspace/add-
* ons/reference/rpc/google.apps.card.v1#loadindicator) to `SPINNER`.
* @param persistValues persistValues or {@code null} for none
*/
public GoogleAppsCardV1Action setPersistValues(java.lang.Boolean persistValues) {
this.persistValues = persistValues;
return this;
}
@Override
public GoogleAppsCardV1Action set(String fieldName, Object value) {
return (GoogleAppsCardV1Action) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1Action clone() {
return (GoogleAppsCardV1Action) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy