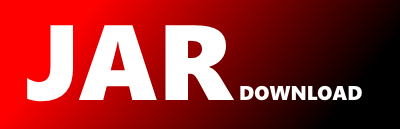
com.google.api.services.chat.v1.model.GoogleAppsCardV1Button Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* A text, icon, or text and icon button that users can click. For an example in Google Chat apps,
* see [Add a button](https://developers.google.com/workspace/chat/design-interactive-card-
* dialog#add_a_button). To make an image a clickable button, specify an `Image` (not an
* `ImageComponent`) and set an `onClick` action. [Google Workspace Add-ons and Chat
* apps](https://developers.google.com/workspace/extend):
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1Button extends com.google.api.client.json.GenericJson {
/**
* The alternative text that's used for accessibility. Set descriptive text that lets users know
* what the button does. For example, if a button opens a hyperlink, you might write: "Opens a new
* browser tab and navigates to the Google Chat developer documentation at
* https://developers.google.com/workspace/chat".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String altText;
/**
* Optional. The color of the button. If set, the button `type` is set to `FILLED` and the color
* of `text` and `icon` fields are set to a contrasting color for readability. For example, if the
* button color is set to blue, any text or icons in the button are set to white. To set the
* button color, specify a value for the `red`, `green`, and `blue` fields. The value must be a
* float number between 0 and 1 based on the RGB color value, where `0` (0/255) represents the
* absence of color and `1` (255/255) represents the maximum intensity of the color. For example,
* the following sets the color to red at its maximum intensity: ``` "color": { "red": 1, "green":
* 0, "blue": 0, } ``` The `alpha` field is unavailable for button color. If specified, this field
* is ignored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Color color;
/**
* If `true`, the button is displayed in an inactive state and doesn't respond to user actions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean disabled;
/**
* An icon displayed inside the button. If both `icon` and `text` are set, then the icon appears
* before the text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Icon icon;
/**
* Required. The action to perform when a user clicks the button, such as opening a hyperlink or
* running a custom function.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1OnClick onClick;
/**
* The text displayed inside the button.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String text;
/**
* The alternative text that's used for accessibility. Set descriptive text that lets users know
* what the button does. For example, if a button opens a hyperlink, you might write: "Opens a new
* browser tab and navigates to the Google Chat developer documentation at
* https://developers.google.com/workspace/chat".
* @return value or {@code null} for none
*/
public java.lang.String getAltText() {
return altText;
}
/**
* The alternative text that's used for accessibility. Set descriptive text that lets users know
* what the button does. For example, if a button opens a hyperlink, you might write: "Opens a new
* browser tab and navigates to the Google Chat developer documentation at
* https://developers.google.com/workspace/chat".
* @param altText altText or {@code null} for none
*/
public GoogleAppsCardV1Button setAltText(java.lang.String altText) {
this.altText = altText;
return this;
}
/**
* Optional. The color of the button. If set, the button `type` is set to `FILLED` and the color
* of `text` and `icon` fields are set to a contrasting color for readability. For example, if the
* button color is set to blue, any text or icons in the button are set to white. To set the
* button color, specify a value for the `red`, `green`, and `blue` fields. The value must be a
* float number between 0 and 1 based on the RGB color value, where `0` (0/255) represents the
* absence of color and `1` (255/255) represents the maximum intensity of the color. For example,
* the following sets the color to red at its maximum intensity: ``` "color": { "red": 1, "green":
* 0, "blue": 0, } ``` The `alpha` field is unavailable for button color. If specified, this field
* is ignored.
* @return value or {@code null} for none
*/
public Color getColor() {
return color;
}
/**
* Optional. The color of the button. If set, the button `type` is set to `FILLED` and the color
* of `text` and `icon` fields are set to a contrasting color for readability. For example, if the
* button color is set to blue, any text or icons in the button are set to white. To set the
* button color, specify a value for the `red`, `green`, and `blue` fields. The value must be a
* float number between 0 and 1 based on the RGB color value, where `0` (0/255) represents the
* absence of color and `1` (255/255) represents the maximum intensity of the color. For example,
* the following sets the color to red at its maximum intensity: ``` "color": { "red": 1, "green":
* 0, "blue": 0, } ``` The `alpha` field is unavailable for button color. If specified, this field
* is ignored.
* @param color color or {@code null} for none
*/
public GoogleAppsCardV1Button setColor(Color color) {
this.color = color;
return this;
}
/**
* If `true`, the button is displayed in an inactive state and doesn't respond to user actions.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDisabled() {
return disabled;
}
/**
* If `true`, the button is displayed in an inactive state and doesn't respond to user actions.
* @param disabled disabled or {@code null} for none
*/
public GoogleAppsCardV1Button setDisabled(java.lang.Boolean disabled) {
this.disabled = disabled;
return this;
}
/**
* An icon displayed inside the button. If both `icon` and `text` are set, then the icon appears
* before the text.
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Icon getIcon() {
return icon;
}
/**
* An icon displayed inside the button. If both `icon` and `text` are set, then the icon appears
* before the text.
* @param icon icon or {@code null} for none
*/
public GoogleAppsCardV1Button setIcon(GoogleAppsCardV1Icon icon) {
this.icon = icon;
return this;
}
/**
* Required. The action to perform when a user clicks the button, such as opening a hyperlink or
* running a custom function.
* @return value or {@code null} for none
*/
public GoogleAppsCardV1OnClick getOnClick() {
return onClick;
}
/**
* Required. The action to perform when a user clicks the button, such as opening a hyperlink or
* running a custom function.
* @param onClick onClick or {@code null} for none
*/
public GoogleAppsCardV1Button setOnClick(GoogleAppsCardV1OnClick onClick) {
this.onClick = onClick;
return this;
}
/**
* The text displayed inside the button.
* @return value or {@code null} for none
*/
public java.lang.String getText() {
return text;
}
/**
* The text displayed inside the button.
* @param text text or {@code null} for none
*/
public GoogleAppsCardV1Button setText(java.lang.String text) {
this.text = text;
return this;
}
@Override
public GoogleAppsCardV1Button set(String fieldName, Object value) {
return (GoogleAppsCardV1Button) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1Button clone() {
return (GoogleAppsCardV1Button) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy