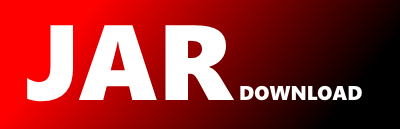
com.google.api.services.chat.v1.model.GoogleAppsCardV1Card Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* A card interface displayed in a Google Chat message or Google Workspace Add-on. Cards support a
* defined layout, interactive UI elements like buttons, and rich media like images. Use cards to
* present detailed information, gather information from users, and guide users to take a next step.
* [Card builder](https://addons.gsuite.google.com/uikit/builder) To learn how to build cards, see
* the following documentation: * For Google Chat apps, see [Design the components of a card or
* dialog](https://developers.google.com/workspace/chat/design-components-card-dialog). * For Google
* Workspace Add-ons, see [Card-based interfaces](https://developers.google.com/apps-script/add-
* ons/concepts/cards). **Example: Card message for a Google Chat app** 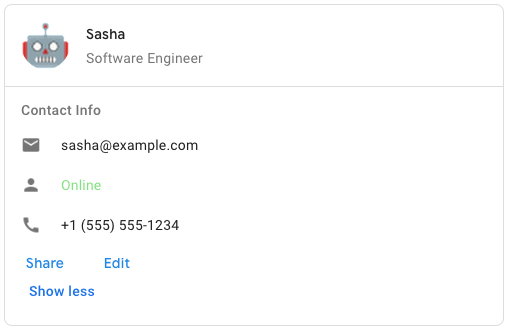 To create the
* sample card message in Google Chat, use the following JSON: ``` { "cardsV2": [ { "cardId":
* "unique-card-id", "card": { "header": { "title": "Sasha", "subtitle": "Software Engineer",
* "imageUrl": "https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png",
* "imageType": "CIRCLE", "imageAltText": "Avatar for Sasha" }, "sections": [ { "header": "Contact
* Info", "collapsible": true, "uncollapsibleWidgetsCount": 1, "widgets": [ { "decoratedText": {
* "startIcon": { "knownIcon": "EMAIL" }, "text": "[email protected]" } }, { "decoratedText": {
* "startIcon": { "knownIcon": "PERSON" }, "text": "Online" } }, { "decoratedText": { "startIcon": {
* "knownIcon": "PHONE" }, "text": "+1 (555) 555-1234" } }, { "buttonList": { "buttons": [ { "text":
* "Share", "onClick": { "openLink": { "url": "https://example.com/share" } } }, { "text": "Edit",
* "onClick": { "action": { "function": "goToView", "parameters": [ { "key": "viewType", "value":
* "EDIT" } ] } } } ] } } ] } ] } } ] } ```
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1Card extends com.google.api.client.json.GenericJson {
/**
* The card's actions. Actions are added to the card's toolbar menu. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons): For example, the following JSON
* constructs a card action menu with `Settings` and `Send Feedback` options: ``` "card_actions":
* [ { "actionLabel": "Settings", "onClick": { "action": { "functionName": "goToView",
* "parameters": [ { "key": "viewType", "value": "SETTING" } ], "loadIndicator":
* "LoadIndicator.SPINNER" } } }, { "actionLabel": "Send Feedback", "onClick": { "openLink": {
* "url": "https://example.com/feedback" } } } ] ```
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cardActions;
/**
* In Google Workspace Add-ons, sets the display properties of the `peekCardHeader`. [Google
* Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayStyle;
/**
* The fixed footer shown at the bottom of this card. Setting `fixedFooter` without specifying a
* `primaryButton` or a `secondaryButton` causes an error. For Chat apps, you can use fixed
* footers in [dialogs](https://developers.google.com/workspace/chat/dialogs), but not [card
* messages](https://developers.google.com/workspace/chat/create-messages#create). [Google
* Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1CardFixedFooter fixedFooter;
/**
* The header of the card. A header usually contains a leading image and a title. Headers always
* appear at the top of a card.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1CardHeader header;
/**
* Name of the card. Used as a card identifier in card navigation. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* When displaying contextual content, the peek card header acts as a placeholder so that the user
* can navigate forward between the homepage cards and the contextual cards. [Google Workspace
* Add-ons](https://developers.google.com/workspace/add-ons):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1CardHeader peekCardHeader;
/**
* The divider style between sections.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sectionDividerStyle;
/**
* Contains a collection of widgets. Each section has its own, optional header. Sections are
* visually separated by a line divider. For an example in Google Chat apps, see [Define a section
* of a card](https://developers.google.com/workspace/chat/design-components-card-
* dialog#define_a_section_of_a_card).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sections;
/**
* The card's actions. Actions are added to the card's toolbar menu. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons): For example, the following JSON
* constructs a card action menu with `Settings` and `Send Feedback` options: ``` "card_actions":
* [ { "actionLabel": "Settings", "onClick": { "action": { "functionName": "goToView",
* "parameters": [ { "key": "viewType", "value": "SETTING" } ], "loadIndicator":
* "LoadIndicator.SPINNER" } } }, { "actionLabel": "Send Feedback", "onClick": { "openLink": {
* "url": "https://example.com/feedback" } } } ] ```
* @return value or {@code null} for none
*/
public java.util.List getCardActions() {
return cardActions;
}
/**
* The card's actions. Actions are added to the card's toolbar menu. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons): For example, the following JSON
* constructs a card action menu with `Settings` and `Send Feedback` options: ``` "card_actions":
* [ { "actionLabel": "Settings", "onClick": { "action": { "functionName": "goToView",
* "parameters": [ { "key": "viewType", "value": "SETTING" } ], "loadIndicator":
* "LoadIndicator.SPINNER" } } }, { "actionLabel": "Send Feedback", "onClick": { "openLink": {
* "url": "https://example.com/feedback" } } } ] ```
* @param cardActions cardActions or {@code null} for none
*/
public GoogleAppsCardV1Card setCardActions(java.util.List cardActions) {
this.cardActions = cardActions;
return this;
}
/**
* In Google Workspace Add-ons, sets the display properties of the `peekCardHeader`. [Google
* Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* @return value or {@code null} for none
*/
public java.lang.String getDisplayStyle() {
return displayStyle;
}
/**
* In Google Workspace Add-ons, sets the display properties of the `peekCardHeader`. [Google
* Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* @param displayStyle displayStyle or {@code null} for none
*/
public GoogleAppsCardV1Card setDisplayStyle(java.lang.String displayStyle) {
this.displayStyle = displayStyle;
return this;
}
/**
* The fixed footer shown at the bottom of this card. Setting `fixedFooter` without specifying a
* `primaryButton` or a `secondaryButton` causes an error. For Chat apps, you can use fixed
* footers in [dialogs](https://developers.google.com/workspace/chat/dialogs), but not [card
* messages](https://developers.google.com/workspace/chat/create-messages#create). [Google
* Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
* @return value or {@code null} for none
*/
public GoogleAppsCardV1CardFixedFooter getFixedFooter() {
return fixedFooter;
}
/**
* The fixed footer shown at the bottom of this card. Setting `fixedFooter` without specifying a
* `primaryButton` or a `secondaryButton` causes an error. For Chat apps, you can use fixed
* footers in [dialogs](https://developers.google.com/workspace/chat/dialogs), but not [card
* messages](https://developers.google.com/workspace/chat/create-messages#create). [Google
* Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
* @param fixedFooter fixedFooter or {@code null} for none
*/
public GoogleAppsCardV1Card setFixedFooter(GoogleAppsCardV1CardFixedFooter fixedFooter) {
this.fixedFooter = fixedFooter;
return this;
}
/**
* The header of the card. A header usually contains a leading image and a title. Headers always
* appear at the top of a card.
* @return value or {@code null} for none
*/
public GoogleAppsCardV1CardHeader getHeader() {
return header;
}
/**
* The header of the card. A header usually contains a leading image and a title. Headers always
* appear at the top of a card.
* @param header header or {@code null} for none
*/
public GoogleAppsCardV1Card setHeader(GoogleAppsCardV1CardHeader header) {
this.header = header;
return this;
}
/**
* Name of the card. Used as a card identifier in card navigation. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons):
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the card. Used as a card identifier in card navigation. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons):
* @param name name or {@code null} for none
*/
public GoogleAppsCardV1Card setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* When displaying contextual content, the peek card header acts as a placeholder so that the user
* can navigate forward between the homepage cards and the contextual cards. [Google Workspace
* Add-ons](https://developers.google.com/workspace/add-ons):
* @return value or {@code null} for none
*/
public GoogleAppsCardV1CardHeader getPeekCardHeader() {
return peekCardHeader;
}
/**
* When displaying contextual content, the peek card header acts as a placeholder so that the user
* can navigate forward between the homepage cards and the contextual cards. [Google Workspace
* Add-ons](https://developers.google.com/workspace/add-ons):
* @param peekCardHeader peekCardHeader or {@code null} for none
*/
public GoogleAppsCardV1Card setPeekCardHeader(GoogleAppsCardV1CardHeader peekCardHeader) {
this.peekCardHeader = peekCardHeader;
return this;
}
/**
* The divider style between sections.
* @return value or {@code null} for none
*/
public java.lang.String getSectionDividerStyle() {
return sectionDividerStyle;
}
/**
* The divider style between sections.
* @param sectionDividerStyle sectionDividerStyle or {@code null} for none
*/
public GoogleAppsCardV1Card setSectionDividerStyle(java.lang.String sectionDividerStyle) {
this.sectionDividerStyle = sectionDividerStyle;
return this;
}
/**
* Contains a collection of widgets. Each section has its own, optional header. Sections are
* visually separated by a line divider. For an example in Google Chat apps, see [Define a section
* of a card](https://developers.google.com/workspace/chat/design-components-card-
* dialog#define_a_section_of_a_card).
* @return value or {@code null} for none
*/
public java.util.List getSections() {
return sections;
}
/**
* Contains a collection of widgets. Each section has its own, optional header. Sections are
* visually separated by a line divider. For an example in Google Chat apps, see [Define a section
* of a card](https://developers.google.com/workspace/chat/design-components-card-
* dialog#define_a_section_of_a_card).
* @param sections sections or {@code null} for none
*/
public GoogleAppsCardV1Card setSections(java.util.List sections) {
this.sections = sections;
return this;
}
@Override
public GoogleAppsCardV1Card set(String fieldName, Object value) {
return (GoogleAppsCardV1Card) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1Card clone() {
return (GoogleAppsCardV1Card) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy