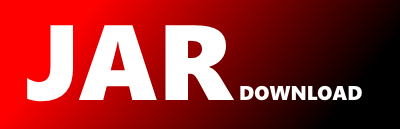
com.google.api.services.chat.v1.model.GoogleAppsCardV1DateTimePicker Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* Lets users input a date, a time, or both a date and a time. For an example in Google Chat apps,
* see [Let a user pick a date and time](https://developers.google.com/workspace/chat/design-
* interactive-card-dialog#let_a_user_pick_a_date_and_time). Users can input text or use the picker
* to select dates and times. If users input an invalid date or time, the picker shows an error that
* prompts users to input the information correctly. [Google Workspace Add-ons and Chat
* apps](https://developers.google.com/workspace/extend):
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1DateTimePicker extends com.google.api.client.json.GenericJson {
/**
* The text that prompts users to input a date, a time, or a date and time. For example, if users
* are scheduling an appointment, use a label such as `Appointment date` or `Appointment date and
* time`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String label;
/**
* The name by which the `DateTimePicker` is identified in a form input event. For details about
* working with form inputs, see [Receive form
* data](https://developers.google.com/workspace/chat/read-form-data).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Triggered when the user clicks **Save** or **Clear** from the `DateTimePicker` interface.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Action onChangeAction;
/**
* The number representing the time zone offset from UTC, in minutes. If set, the `value_ms_epoch`
* is displayed in the specified time zone. If unset, the value defaults to the user's time zone
* setting.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer timezoneOffsetDate;
/**
* Whether the widget supports inputting a date, a time, or the date and time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The default value displayed in the widget, in milliseconds since [Unix epoch
* time](https://en.wikipedia.org/wiki/Unix_time). Specify the value based on the type of picker
* (`DateTimePickerType`): * `DATE_AND_TIME`: a calendar date and time in UTC. For example, to
* represent January 1, 2023 at 12:00 PM UTC, use `1672574400000`. * `DATE_ONLY`: a calendar date
* at 00:00:00 UTC. For example, to represent January 1, 2023, use `1672531200000`. * `TIME_ONLY`:
* a time in UTC. For example, to represent 12:00 PM, use `43200000` (or `12 * 60 * 60 * 1000`).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long valueMsEpoch;
/**
* The text that prompts users to input a date, a time, or a date and time. For example, if users
* are scheduling an appointment, use a label such as `Appointment date` or `Appointment date and
* time`.
* @return value or {@code null} for none
*/
public java.lang.String getLabel() {
return label;
}
/**
* The text that prompts users to input a date, a time, or a date and time. For example, if users
* are scheduling an appointment, use a label such as `Appointment date` or `Appointment date and
* time`.
* @param label label or {@code null} for none
*/
public GoogleAppsCardV1DateTimePicker setLabel(java.lang.String label) {
this.label = label;
return this;
}
/**
* The name by which the `DateTimePicker` is identified in a form input event. For details about
* working with form inputs, see [Receive form
* data](https://developers.google.com/workspace/chat/read-form-data).
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name by which the `DateTimePicker` is identified in a form input event. For details about
* working with form inputs, see [Receive form
* data](https://developers.google.com/workspace/chat/read-form-data).
* @param name name or {@code null} for none
*/
public GoogleAppsCardV1DateTimePicker setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Triggered when the user clicks **Save** or **Clear** from the `DateTimePicker` interface.
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Action getOnChangeAction() {
return onChangeAction;
}
/**
* Triggered when the user clicks **Save** or **Clear** from the `DateTimePicker` interface.
* @param onChangeAction onChangeAction or {@code null} for none
*/
public GoogleAppsCardV1DateTimePicker setOnChangeAction(GoogleAppsCardV1Action onChangeAction) {
this.onChangeAction = onChangeAction;
return this;
}
/**
* The number representing the time zone offset from UTC, in minutes. If set, the `value_ms_epoch`
* is displayed in the specified time zone. If unset, the value defaults to the user's time zone
* setting.
* @return value or {@code null} for none
*/
public java.lang.Integer getTimezoneOffsetDate() {
return timezoneOffsetDate;
}
/**
* The number representing the time zone offset from UTC, in minutes. If set, the `value_ms_epoch`
* is displayed in the specified time zone. If unset, the value defaults to the user's time zone
* setting.
* @param timezoneOffsetDate timezoneOffsetDate or {@code null} for none
*/
public GoogleAppsCardV1DateTimePicker setTimezoneOffsetDate(java.lang.Integer timezoneOffsetDate) {
this.timezoneOffsetDate = timezoneOffsetDate;
return this;
}
/**
* Whether the widget supports inputting a date, a time, or the date and time.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Whether the widget supports inputting a date, a time, or the date and time.
* @param type type or {@code null} for none
*/
public GoogleAppsCardV1DateTimePicker setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* The default value displayed in the widget, in milliseconds since [Unix epoch
* time](https://en.wikipedia.org/wiki/Unix_time). Specify the value based on the type of picker
* (`DateTimePickerType`): * `DATE_AND_TIME`: a calendar date and time in UTC. For example, to
* represent January 1, 2023 at 12:00 PM UTC, use `1672574400000`. * `DATE_ONLY`: a calendar date
* at 00:00:00 UTC. For example, to represent January 1, 2023, use `1672531200000`. * `TIME_ONLY`:
* a time in UTC. For example, to represent 12:00 PM, use `43200000` (or `12 * 60 * 60 * 1000`).
* @return value or {@code null} for none
*/
public java.lang.Long getValueMsEpoch() {
return valueMsEpoch;
}
/**
* The default value displayed in the widget, in milliseconds since [Unix epoch
* time](https://en.wikipedia.org/wiki/Unix_time). Specify the value based on the type of picker
* (`DateTimePickerType`): * `DATE_AND_TIME`: a calendar date and time in UTC. For example, to
* represent January 1, 2023 at 12:00 PM UTC, use `1672574400000`. * `DATE_ONLY`: a calendar date
* at 00:00:00 UTC. For example, to represent January 1, 2023, use `1672531200000`. * `TIME_ONLY`:
* a time in UTC. For example, to represent 12:00 PM, use `43200000` (or `12 * 60 * 60 * 1000`).
* @param valueMsEpoch valueMsEpoch or {@code null} for none
*/
public GoogleAppsCardV1DateTimePicker setValueMsEpoch(java.lang.Long valueMsEpoch) {
this.valueMsEpoch = valueMsEpoch;
return this;
}
@Override
public GoogleAppsCardV1DateTimePicker set(String fieldName, Object value) {
return (GoogleAppsCardV1DateTimePicker) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1DateTimePicker clone() {
return (GoogleAppsCardV1DateTimePicker) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy