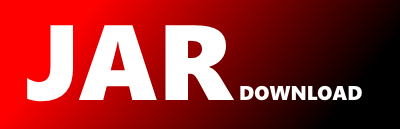
com.google.api.services.chat.v1.model.GoogleAppsCardV1Icon Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* An icon displayed in a widget on a card. For an example in Google Chat apps, see [Add an
* icon](https://developers.google.com/workspace/chat/add-text-image-card-dialog#add_an_icon).
* Supports [built-in](https://developers.google.com/workspace/chat/format-messages#builtinicons)
* and [custom](https://developers.google.com/workspace/chat/format-messages#customicons) icons.
* [Google Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1Icon extends com.google.api.client.json.GenericJson {
/**
* Optional. A description of the icon used for accessibility. If unspecified, the default value
* `Button` is provided. As a best practice, you should set a helpful description for what the
* icon displays, and if applicable, what it does. For example, `A user's account portrait`, or
* `Opens a new browser tab and navigates to the Google Chat developer documentation at
* https://developers.google.com/workspace/chat`. If the icon is set in a `Button`, the `altText`
* appears as helper text when the user hovers over the button. However, if the button also sets
* `text`, the icon's `altText` is ignored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String altText;
/**
* Display a custom icon hosted at an HTTPS URL. For example: ``` "iconUrl":
* "https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png" ``` Supported
* file types include `.png` and `.jpg`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String iconUrl;
/**
* The crop style applied to the image. In some cases, applying a `CIRCLE` crop causes the image
* to be drawn larger than a built-in icon.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imageType;
/**
* Display one of the built-in icons provided by Google Workspace. For example, to display an
* airplane icon, specify `AIRPLANE`. For a bus, specify `BUS`. For a full list of supported
* icons, see [built-in icons](https://developers.google.com/workspace/chat/format-
* messages#builtinicons).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String knownIcon;
/**
* Display one of the [Google Material Icons](https://fonts.google.com/icons). For example, to
* display a [checkbox icon](https://fonts.google.com/icons?selected=Material%20Symbols%20Outlined
* %3Acheck_box%3AFILL%400%3Bwght%40400%3BGRAD%400%3Bopsz%4048), use ``` "material_icon": {
* "name": "check_box" } ``` [Google Chat apps](https://developers.google.com/workspace/chat):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1MaterialIcon materialIcon;
/**
* Optional. A description of the icon used for accessibility. If unspecified, the default value
* `Button` is provided. As a best practice, you should set a helpful description for what the
* icon displays, and if applicable, what it does. For example, `A user's account portrait`, or
* `Opens a new browser tab and navigates to the Google Chat developer documentation at
* https://developers.google.com/workspace/chat`. If the icon is set in a `Button`, the `altText`
* appears as helper text when the user hovers over the button. However, if the button also sets
* `text`, the icon's `altText` is ignored.
* @return value or {@code null} for none
*/
public java.lang.String getAltText() {
return altText;
}
/**
* Optional. A description of the icon used for accessibility. If unspecified, the default value
* `Button` is provided. As a best practice, you should set a helpful description for what the
* icon displays, and if applicable, what it does. For example, `A user's account portrait`, or
* `Opens a new browser tab and navigates to the Google Chat developer documentation at
* https://developers.google.com/workspace/chat`. If the icon is set in a `Button`, the `altText`
* appears as helper text when the user hovers over the button. However, if the button also sets
* `text`, the icon's `altText` is ignored.
* @param altText altText or {@code null} for none
*/
public GoogleAppsCardV1Icon setAltText(java.lang.String altText) {
this.altText = altText;
return this;
}
/**
* Display a custom icon hosted at an HTTPS URL. For example: ``` "iconUrl":
* "https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png" ``` Supported
* file types include `.png` and `.jpg`.
* @return value or {@code null} for none
*/
public java.lang.String getIconUrl() {
return iconUrl;
}
/**
* Display a custom icon hosted at an HTTPS URL. For example: ``` "iconUrl":
* "https://developers.google.com/workspace/chat/images/quickstart-app-avatar.png" ``` Supported
* file types include `.png` and `.jpg`.
* @param iconUrl iconUrl or {@code null} for none
*/
public GoogleAppsCardV1Icon setIconUrl(java.lang.String iconUrl) {
this.iconUrl = iconUrl;
return this;
}
/**
* The crop style applied to the image. In some cases, applying a `CIRCLE` crop causes the image
* to be drawn larger than a built-in icon.
* @return value or {@code null} for none
*/
public java.lang.String getImageType() {
return imageType;
}
/**
* The crop style applied to the image. In some cases, applying a `CIRCLE` crop causes the image
* to be drawn larger than a built-in icon.
* @param imageType imageType or {@code null} for none
*/
public GoogleAppsCardV1Icon setImageType(java.lang.String imageType) {
this.imageType = imageType;
return this;
}
/**
* Display one of the built-in icons provided by Google Workspace. For example, to display an
* airplane icon, specify `AIRPLANE`. For a bus, specify `BUS`. For a full list of supported
* icons, see [built-in icons](https://developers.google.com/workspace/chat/format-
* messages#builtinicons).
* @return value or {@code null} for none
*/
public java.lang.String getKnownIcon() {
return knownIcon;
}
/**
* Display one of the built-in icons provided by Google Workspace. For example, to display an
* airplane icon, specify `AIRPLANE`. For a bus, specify `BUS`. For a full list of supported
* icons, see [built-in icons](https://developers.google.com/workspace/chat/format-
* messages#builtinicons).
* @param knownIcon knownIcon or {@code null} for none
*/
public GoogleAppsCardV1Icon setKnownIcon(java.lang.String knownIcon) {
this.knownIcon = knownIcon;
return this;
}
/**
* Display one of the [Google Material Icons](https://fonts.google.com/icons). For example, to
* display a [checkbox icon](https://fonts.google.com/icons?selected=Material%20Symbols%20Outlined
* %3Acheck_box%3AFILL%400%3Bwght%40400%3BGRAD%400%3Bopsz%4048), use ``` "material_icon": {
* "name": "check_box" } ``` [Google Chat apps](https://developers.google.com/workspace/chat):
* @return value or {@code null} for none
*/
public GoogleAppsCardV1MaterialIcon getMaterialIcon() {
return materialIcon;
}
/**
* Display one of the [Google Material Icons](https://fonts.google.com/icons). For example, to
* display a [checkbox icon](https://fonts.google.com/icons?selected=Material%20Symbols%20Outlined
* %3Acheck_box%3AFILL%400%3Bwght%40400%3BGRAD%400%3Bopsz%4048), use ``` "material_icon": {
* "name": "check_box" } ``` [Google Chat apps](https://developers.google.com/workspace/chat):
* @param materialIcon materialIcon or {@code null} for none
*/
public GoogleAppsCardV1Icon setMaterialIcon(GoogleAppsCardV1MaterialIcon materialIcon) {
this.materialIcon = materialIcon;
return this;
}
@Override
public GoogleAppsCardV1Icon set(String fieldName, Object value) {
return (GoogleAppsCardV1Icon) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1Icon clone() {
return (GoogleAppsCardV1Icon) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy