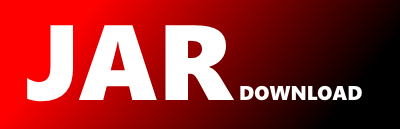
com.google.api.services.chat.v1.model.GoogleAppsCardV1MaterialIcon Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* A [Google Material Icon](https://fonts.google.com/icons), which includes over 2500+ options. For
* example, to display a [checkbox icon](https://fonts.google.com/icons?selected=Material%20Symbols%
* 20Outlined%3Acheck_box%3AFILL%400%3Bwght%40400%3BGRAD%400%3Bopsz%4048) with customized weight and
* grade, write the following: ``` { "name": "check_box", "fill": true, "weight": 300, "grade": -25
* } ``` [Google Chat apps](https://developers.google.com/workspace/chat):
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1MaterialIcon extends com.google.api.client.json.GenericJson {
/**
* Whether the icon renders as filled. Default value is false. To preview different icon settings,
* go to [Google Font Icons](https://fonts.google.com/icons) and adjust the settings under
* **Customize**.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean fill;
/**
* Weight and grade affect a symbol’s thickness. Adjustments to grade are more granular than
* adjustments to weight and have a small impact on the size of the symbol. Choose from {-25, 0,
* 200}. If absent, default value is 0. If any other value is specified, the default value is
* used. To preview different icon settings, go to [Google Font
* Icons](https://fonts.google.com/icons) and adjust the settings under **Customize**.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer grade;
/**
* The icon name defined in the [Google Material Icon](https://fonts.google.com/icons), for
* example, `check_box`. Any invalid names are abandoned and replaced with empty string and
* results in the icon failing to render.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The stroke weight of the icon. Choose from {100, 200, 300, 400, 500, 600, 700}. If absent,
* default value is 400. If any other value is specified, the default value is used. To preview
* different icon settings, go to [Google Font Icons](https://fonts.google.com/icons) and adjust
* the settings under **Customize**.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer weight;
/**
* Whether the icon renders as filled. Default value is false. To preview different icon settings,
* go to [Google Font Icons](https://fonts.google.com/icons) and adjust the settings under
* **Customize**.
* @return value or {@code null} for none
*/
public java.lang.Boolean getFill() {
return fill;
}
/**
* Whether the icon renders as filled. Default value is false. To preview different icon settings,
* go to [Google Font Icons](https://fonts.google.com/icons) and adjust the settings under
* **Customize**.
* @param fill fill or {@code null} for none
*/
public GoogleAppsCardV1MaterialIcon setFill(java.lang.Boolean fill) {
this.fill = fill;
return this;
}
/**
* Weight and grade affect a symbol’s thickness. Adjustments to grade are more granular than
* adjustments to weight and have a small impact on the size of the symbol. Choose from {-25, 0,
* 200}. If absent, default value is 0. If any other value is specified, the default value is
* used. To preview different icon settings, go to [Google Font
* Icons](https://fonts.google.com/icons) and adjust the settings under **Customize**.
* @return value or {@code null} for none
*/
public java.lang.Integer getGrade() {
return grade;
}
/**
* Weight and grade affect a symbol’s thickness. Adjustments to grade are more granular than
* adjustments to weight and have a small impact on the size of the symbol. Choose from {-25, 0,
* 200}. If absent, default value is 0. If any other value is specified, the default value is
* used. To preview different icon settings, go to [Google Font
* Icons](https://fonts.google.com/icons) and adjust the settings under **Customize**.
* @param grade grade or {@code null} for none
*/
public GoogleAppsCardV1MaterialIcon setGrade(java.lang.Integer grade) {
this.grade = grade;
return this;
}
/**
* The icon name defined in the [Google Material Icon](https://fonts.google.com/icons), for
* example, `check_box`. Any invalid names are abandoned and replaced with empty string and
* results in the icon failing to render.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The icon name defined in the [Google Material Icon](https://fonts.google.com/icons), for
* example, `check_box`. Any invalid names are abandoned and replaced with empty string and
* results in the icon failing to render.
* @param name name or {@code null} for none
*/
public GoogleAppsCardV1MaterialIcon setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The stroke weight of the icon. Choose from {100, 200, 300, 400, 500, 600, 700}. If absent,
* default value is 400. If any other value is specified, the default value is used. To preview
* different icon settings, go to [Google Font Icons](https://fonts.google.com/icons) and adjust
* the settings under **Customize**.
* @return value or {@code null} for none
*/
public java.lang.Integer getWeight() {
return weight;
}
/**
* The stroke weight of the icon. Choose from {100, 200, 300, 400, 500, 600, 700}. If absent,
* default value is 400. If any other value is specified, the default value is used. To preview
* different icon settings, go to [Google Font Icons](https://fonts.google.com/icons) and adjust
* the settings under **Customize**.
* @param weight weight or {@code null} for none
*/
public GoogleAppsCardV1MaterialIcon setWeight(java.lang.Integer weight) {
this.weight = weight;
return this;
}
@Override
public GoogleAppsCardV1MaterialIcon set(String fieldName, Object value) {
return (GoogleAppsCardV1MaterialIcon) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1MaterialIcon clone() {
return (GoogleAppsCardV1MaterialIcon) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy