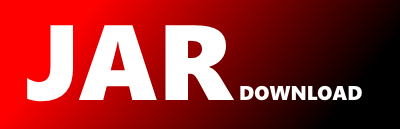
com.google.api.services.chat.v1.model.GoogleAppsCardV1OnClick Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* Represents how to respond when users click an interactive element on a card, such as a button.
* [Google Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1OnClick extends com.google.api.client.json.GenericJson {
/**
* If specified, an action is triggered by this `onClick`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Action action;
/**
* A new card is pushed to the card stack after clicking if specified. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Card card;
/**
* An add-on triggers this action when the action needs to open a link. This differs from the
* `open_link` above in that this needs to talk to server to get the link. Thus some preparation
* work is required for web client to do before the open link action response comes back. [Google
* Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Action openDynamicLinkAction;
/**
* If specified, this `onClick` triggers an open link action.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1OpenLink openLink;
/**
* If specified, an action is triggered by this `onClick`.
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Action getAction() {
return action;
}
/**
* If specified, an action is triggered by this `onClick`.
* @param action action or {@code null} for none
*/
public GoogleAppsCardV1OnClick setAction(GoogleAppsCardV1Action action) {
this.action = action;
return this;
}
/**
* A new card is pushed to the card stack after clicking if specified. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons):
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Card getCard() {
return card;
}
/**
* A new card is pushed to the card stack after clicking if specified. [Google Workspace Add-
* ons](https://developers.google.com/workspace/add-ons):
* @param card card or {@code null} for none
*/
public GoogleAppsCardV1OnClick setCard(GoogleAppsCardV1Card card) {
this.card = card;
return this;
}
/**
* An add-on triggers this action when the action needs to open a link. This differs from the
* `open_link` above in that this needs to talk to server to get the link. Thus some preparation
* work is required for web client to do before the open link action response comes back. [Google
* Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Action getOpenDynamicLinkAction() {
return openDynamicLinkAction;
}
/**
* An add-on triggers this action when the action needs to open a link. This differs from the
* `open_link` above in that this needs to talk to server to get the link. Thus some preparation
* work is required for web client to do before the open link action response comes back. [Google
* Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* @param openDynamicLinkAction openDynamicLinkAction or {@code null} for none
*/
public GoogleAppsCardV1OnClick setOpenDynamicLinkAction(GoogleAppsCardV1Action openDynamicLinkAction) {
this.openDynamicLinkAction = openDynamicLinkAction;
return this;
}
/**
* If specified, this `onClick` triggers an open link action.
* @return value or {@code null} for none
*/
public GoogleAppsCardV1OpenLink getOpenLink() {
return openLink;
}
/**
* If specified, this `onClick` triggers an open link action.
* @param openLink openLink or {@code null} for none
*/
public GoogleAppsCardV1OnClick setOpenLink(GoogleAppsCardV1OpenLink openLink) {
this.openLink = openLink;
return this;
}
@Override
public GoogleAppsCardV1OnClick set(String fieldName, Object value) {
return (GoogleAppsCardV1OnClick) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1OnClick clone() {
return (GoogleAppsCardV1OnClick) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy