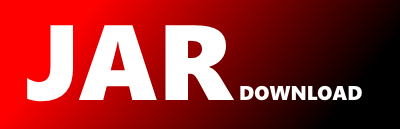
com.google.api.services.chat.v1.model.GoogleAppsCardV1TextInput Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* A field in which users can enter text. Supports suggestions and on-change actions. For an example
* in Google Chat apps, see [Add a field in which a user can enter
* text](https://developers.google.com/workspace/chat/design-interactive-card-
* dialog#add_a_field_in_which_a_user_can_enter_text). Chat apps receive and can process the value
* of entered text during form input events. For details about working with form inputs, see
* [Receive form data](https://developers.google.com/workspace/chat/read-form-data). When you need
* to collect undefined or abstract data from users, use a text input. To collect defined or
* enumerated data from users, use the SelectionInput widget. [Google Workspace Add-ons and Chat
* apps](https://developers.google.com/workspace/extend):
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCardV1TextInput extends com.google.api.client.json.GenericJson {
/**
* Optional. Specify what action to take when the text input field provides suggestions to users
* who interact with it. If unspecified, the suggestions are set by `initialSuggestions` and are
* processed by the client. If specified, the app takes the action specified here, such as running
* a custom function. [Google Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Action autoCompleteAction;
/**
* Text that appears below the text input field meant to assist users by prompting them to enter a
* certain value. This text is always visible. Required if `label` is unspecified. Otherwise,
* optional.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hintText;
/**
* Suggested values that users can enter. These values appear when users click inside the text
* input field. As users type, the suggested values dynamically filter to match what the users
* have typed. For example, a text input field for programming language might suggest Java,
* JavaScript, Python, and C++. When users start typing `Jav`, the list of suggestions filters to
* show just `Java` and `JavaScript`. Suggested values help guide users to enter values that your
* app can make sense of. When referring to JavaScript, some users might enter `javascript` and
* others `java script`. Suggesting `JavaScript` can standardize how users interact with your app.
* When specified, `TextInput.type` is always `SINGLE_LINE`, even if it's set to `MULTIPLE_LINE`.
* [Google Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Suggestions initialSuggestions;
/**
* The text that appears above the text input field in the user interface. Specify text that helps
* the user enter the information your app needs. For example, if you are asking someone's name,
* but specifically need their surname, write `surname` instead of `name`. Required if `hintText`
* is unspecified. Otherwise, optional.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String label;
/**
* The name by which the text input is identified in a form input event. For details about working
* with form inputs, see [Receive form data](https://developers.google.com/workspace/chat/read-
* form-data).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* What to do when a change occurs in the text input field. For example, a user adding to the
* field or deleting text. Examples of actions to take include running a custom function or
* opening a [dialog](https://developers.google.com/workspace/chat/dialogs) in Google Chat.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleAppsCardV1Action onChangeAction;
/**
* Text that appears in the text input field when the field is empty. Use this text to prompt
* users to enter a value. For example, `Enter a number from 0 to 100`. [Google Chat
* apps](https://developers.google.com/workspace/chat):
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String placeholderText;
/**
* How a text input field appears in the user interface. For example, whether the field is single
* or multi-line.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The value entered by a user, returned as part of a form input event. For details about working
* with form inputs, see [Receive form data](https://developers.google.com/workspace/chat/read-
* form-data).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* Optional. Specify what action to take when the text input field provides suggestions to users
* who interact with it. If unspecified, the suggestions are set by `initialSuggestions` and are
* processed by the client. If specified, the app takes the action specified here, such as running
* a custom function. [Google Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Action getAutoCompleteAction() {
return autoCompleteAction;
}
/**
* Optional. Specify what action to take when the text input field provides suggestions to users
* who interact with it. If unspecified, the suggestions are set by `initialSuggestions` and are
* processed by the client. If specified, the app takes the action specified here, such as running
* a custom function. [Google Workspace Add-ons](https://developers.google.com/workspace/add-ons):
* @param autoCompleteAction autoCompleteAction or {@code null} for none
*/
public GoogleAppsCardV1TextInput setAutoCompleteAction(GoogleAppsCardV1Action autoCompleteAction) {
this.autoCompleteAction = autoCompleteAction;
return this;
}
/**
* Text that appears below the text input field meant to assist users by prompting them to enter a
* certain value. This text is always visible. Required if `label` is unspecified. Otherwise,
* optional.
* @return value or {@code null} for none
*/
public java.lang.String getHintText() {
return hintText;
}
/**
* Text that appears below the text input field meant to assist users by prompting them to enter a
* certain value. This text is always visible. Required if `label` is unspecified. Otherwise,
* optional.
* @param hintText hintText or {@code null} for none
*/
public GoogleAppsCardV1TextInput setHintText(java.lang.String hintText) {
this.hintText = hintText;
return this;
}
/**
* Suggested values that users can enter. These values appear when users click inside the text
* input field. As users type, the suggested values dynamically filter to match what the users
* have typed. For example, a text input field for programming language might suggest Java,
* JavaScript, Python, and C++. When users start typing `Jav`, the list of suggestions filters to
* show just `Java` and `JavaScript`. Suggested values help guide users to enter values that your
* app can make sense of. When referring to JavaScript, some users might enter `javascript` and
* others `java script`. Suggesting `JavaScript` can standardize how users interact with your app.
* When specified, `TextInput.type` is always `SINGLE_LINE`, even if it's set to `MULTIPLE_LINE`.
* [Google Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Suggestions getInitialSuggestions() {
return initialSuggestions;
}
/**
* Suggested values that users can enter. These values appear when users click inside the text
* input field. As users type, the suggested values dynamically filter to match what the users
* have typed. For example, a text input field for programming language might suggest Java,
* JavaScript, Python, and C++. When users start typing `Jav`, the list of suggestions filters to
* show just `Java` and `JavaScript`. Suggested values help guide users to enter values that your
* app can make sense of. When referring to JavaScript, some users might enter `javascript` and
* others `java script`. Suggesting `JavaScript` can standardize how users interact with your app.
* When specified, `TextInput.type` is always `SINGLE_LINE`, even if it's set to `MULTIPLE_LINE`.
* [Google Workspace Add-ons and Chat apps](https://developers.google.com/workspace/extend):
* @param initialSuggestions initialSuggestions or {@code null} for none
*/
public GoogleAppsCardV1TextInput setInitialSuggestions(GoogleAppsCardV1Suggestions initialSuggestions) {
this.initialSuggestions = initialSuggestions;
return this;
}
/**
* The text that appears above the text input field in the user interface. Specify text that helps
* the user enter the information your app needs. For example, if you are asking someone's name,
* but specifically need their surname, write `surname` instead of `name`. Required if `hintText`
* is unspecified. Otherwise, optional.
* @return value or {@code null} for none
*/
public java.lang.String getLabel() {
return label;
}
/**
* The text that appears above the text input field in the user interface. Specify text that helps
* the user enter the information your app needs. For example, if you are asking someone's name,
* but specifically need their surname, write `surname` instead of `name`. Required if `hintText`
* is unspecified. Otherwise, optional.
* @param label label or {@code null} for none
*/
public GoogleAppsCardV1TextInput setLabel(java.lang.String label) {
this.label = label;
return this;
}
/**
* The name by which the text input is identified in a form input event. For details about working
* with form inputs, see [Receive form data](https://developers.google.com/workspace/chat/read-
* form-data).
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name by which the text input is identified in a form input event. For details about working
* with form inputs, see [Receive form data](https://developers.google.com/workspace/chat/read-
* form-data).
* @param name name or {@code null} for none
*/
public GoogleAppsCardV1TextInput setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* What to do when a change occurs in the text input field. For example, a user adding to the
* field or deleting text. Examples of actions to take include running a custom function or
* opening a [dialog](https://developers.google.com/workspace/chat/dialogs) in Google Chat.
* @return value or {@code null} for none
*/
public GoogleAppsCardV1Action getOnChangeAction() {
return onChangeAction;
}
/**
* What to do when a change occurs in the text input field. For example, a user adding to the
* field or deleting text. Examples of actions to take include running a custom function or
* opening a [dialog](https://developers.google.com/workspace/chat/dialogs) in Google Chat.
* @param onChangeAction onChangeAction or {@code null} for none
*/
public GoogleAppsCardV1TextInput setOnChangeAction(GoogleAppsCardV1Action onChangeAction) {
this.onChangeAction = onChangeAction;
return this;
}
/**
* Text that appears in the text input field when the field is empty. Use this text to prompt
* users to enter a value. For example, `Enter a number from 0 to 100`. [Google Chat
* apps](https://developers.google.com/workspace/chat):
* @return value or {@code null} for none
*/
public java.lang.String getPlaceholderText() {
return placeholderText;
}
/**
* Text that appears in the text input field when the field is empty. Use this text to prompt
* users to enter a value. For example, `Enter a number from 0 to 100`. [Google Chat
* apps](https://developers.google.com/workspace/chat):
* @param placeholderText placeholderText or {@code null} for none
*/
public GoogleAppsCardV1TextInput setPlaceholderText(java.lang.String placeholderText) {
this.placeholderText = placeholderText;
return this;
}
/**
* How a text input field appears in the user interface. For example, whether the field is single
* or multi-line.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* How a text input field appears in the user interface. For example, whether the field is single
* or multi-line.
* @param type type or {@code null} for none
*/
public GoogleAppsCardV1TextInput setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* The value entered by a user, returned as part of a form input event. For details about working
* with form inputs, see [Receive form data](https://developers.google.com/workspace/chat/read-
* form-data).
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* The value entered by a user, returned as part of a form input event. For details about working
* with form inputs, see [Receive form data](https://developers.google.com/workspace/chat/read-
* form-data).
* @param value value or {@code null} for none
*/
public GoogleAppsCardV1TextInput setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public GoogleAppsCardV1TextInput set(String fieldName, Object value) {
return (GoogleAppsCardV1TextInput) super.set(fieldName, value);
}
@Override
public GoogleAppsCardV1TextInput clone() {
return (GoogleAppsCardV1TextInput) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy