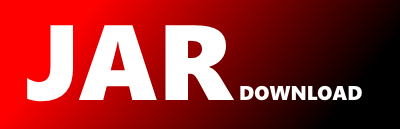
com.google.api.services.chat.v1.model.Message Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* A message in a Google Chat space.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Message extends com.google.api.client.json.GenericJson {
/**
* One or more interactive widgets that appear at the bottom of a message. You can add accessory
* widgets to messages that contain text, cards, or both text and cards. Not supported for
* messages that contain dialogs. For details, see [Add interactive widgets at the bottom of a
* message](https://developers.google.com/workspace/chat/create-messages#add-accessory-widgets).
* Creating a message with accessory widgets requires [app authentication]
* (https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List accessoryWidgets;
static {
// hack to force ProGuard to consider AccessoryWidget used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AccessoryWidget.class);
}
/**
* Input only. Parameters that a Chat app can use to configure how its response is posted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ActionResponse actionResponse;
/**
* Output only. Annotations associated with the `text` in this message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List annotations;
static {
// hack to force ProGuard to consider Annotation used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Annotation.class);
}
/**
* Output only. Plain-text body of the message with all Chat app mentions stripped out.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String argumentText;
/**
* Output only. GIF images that are attached to the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List attachedGifs;
static {
// hack to force ProGuard to consider AttachedGif used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AttachedGif.class);
}
/**
* User-uploaded attachment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List attachment;
static {
// hack to force ProGuard to consider Attachment used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Attachment.class);
}
/**
* Deprecated: Use `cards_v2` instead. Rich, formatted, and interactive cards that you can use to
* display UI elements such as: formatted texts, buttons, and clickable images. Cards are normally
* displayed below the plain-text body of the message. `cards` and `cards_v2` can have a maximum
* size of 32 KB.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cards;
static {
// hack to force ProGuard to consider Card used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Card.class);
}
/**
* An array of [cards](https://developers.google.com/workspace/chat/api/reference/rest/v1/cards).
* Only Chat apps can create cards. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* messages can't contain cards. To learn how to create a message that contains cards, see [Send a
* message](https://developers.google.com/workspace/chat/create-messages). [Card
* builder](https://addons.gsuite.google.com/uikit/builder)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cardsV2;
static {
// hack to force ProGuard to consider CardWithId used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(CardWithId.class);
}
/**
* Optional. A custom ID for the message. You can use field to identify a message, or to get,
* delete, or update a message. To set a custom ID, specify the [`messageId`](https://developers.g
* oogle.com/workspace/chat/api/reference/rest/v1/spaces.messages/create#body.QUERY_PARAMETERS.mes
* sage_id) field when you create the message. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clientAssignedMessageId;
/**
* Optional. Immutable. For spaces created in Chat, the time at which the message was created.
* This field is output only, except when used in import mode spaces. For import mode spaces, set
* this field to the historical timestamp at which the message was created in the source in order
* to preserve the original creation time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. The time at which the message was deleted in Google Chat. If the message is never
* deleted, this field is empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String deleteTime;
/**
* Output only. Information about a deleted message. A message is deleted when `delete_time` is
* set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeletionMetadata deletionMetadata;
/**
* Output only. The list of emoji reaction summaries on the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List emojiReactionSummaries;
static {
// hack to force ProGuard to consider EmojiReactionSummary used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(EmojiReactionSummary.class);
}
/**
* A plain-text description of the message's cards, used when the actual cards can't be
* displayed—for example, mobile notifications.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fallbackText;
/**
* Output only. Contains the message `text` with markups added to communicate formatting. This
* field might not capture all formatting visible in the UI, but includes the following: * [Markup
* syntax](https://developers.google.com/workspace/chat/format-messages) for bold, italic,
* strikethrough, monospace, monospace block, and bulleted list. * [User
* mentions](https://developers.google.com/workspace/chat/format-messages#messages-@mention) using
* the format ``. * Custom hyperlinks using the format `<{url}|{rendered_text}>` where the first
* string is the URL and the second is the rendered text—for example, ``. * Custom emoji using the
* format `:{emoji_name}:`—for example, `:smile:`. This doesn't apply to Unicode emoji, such as
* `U+1F600` for a grinning face emoji. For more information, see [View text formatting sent in a
* message](https://developers.google.com/workspace/chat/format-
* messages#view_text_formatting_sent_in_a_message)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String formattedText;
/**
* Output only. The time at which the message was last edited by a user. If the message has never
* been edited, this field is empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String lastUpdateTime;
/**
* Output only. A URL in `spaces.messages.text` that matches a link preview pattern. For more
* information, see [Preview links](https://developers.google.com/workspace/chat/preview-links).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MatchedUrl matchedUrl;
/**
* Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is
* the ID of the space where the message is posted and `{message}` is a system-assigned ID for the
* message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
* custom ID when you create a message, you can use this ID to specify the message in a request by
* replacing `{message}` with the value from the `clientAssignedMessageId` field. For example,
* `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Immutable. Input for creating a message, otherwise output only. The user that can view the
* message. When set, the message is private and only visible to the specified user and the Chat
* app. Link previews and attachments aren't supported for private messages. Only Chat apps can
* send private messages. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user) to send a
* message, the message can't be private and must omit this field. For details, see [Send a
* message privately](https://developers.google.com/workspace/chat/create-messages#private).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private User privateMessageViewer;
/**
* Output only. Information about a message that's quoted by a Google Chat user in a space. Google
* Chat users can quote a message to reply to it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private QuotedMessageMetadata quotedMessageMetadata;
/**
* Output only. The user who created the message. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* output populates the
* [user](https://developers.google.com/workspace/chat/api/reference/rest/v1/User) `name` and
* `type`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private User sender;
/**
* Output only. Slash command information, if applicable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SlashCommand slashCommand;
/**
* If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* output populates the
* [space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) `name`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Space space;
/**
* Plain-text body of the message. The first link to an image, video, or web page generates a
* [preview chip](https://developers.google.com/workspace/chat/preview-links). You can also
* [@mention a Google Chat user](https://developers.google.com/workspace/chat/format-
* messages#messages-@mention), or everyone in the space. To learn about creating text messages,
* see [Send a message](https://developers.google.com/workspace/chat/create-messages).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String text;
/**
* The thread the message belongs to. For example usage, see [Start or reply to a message
* thread](https://developers.google.com/workspace/chat/create-messages#create-message-thread).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Thread thread;
/**
* Output only. When `true`, the message is a response in a reply thread. When `false`, the
* message is visible in the space's top-level conversation as either the first message of a
* thread or a message with no threaded replies. If the space doesn't support reply in threads,
* this field is always `false`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean threadReply;
/**
* One or more interactive widgets that appear at the bottom of a message. You can add accessory
* widgets to messages that contain text, cards, or both text and cards. Not supported for
* messages that contain dialogs. For details, see [Add interactive widgets at the bottom of a
* message](https://developers.google.com/workspace/chat/create-messages#add-accessory-widgets).
* Creating a message with accessory widgets requires [app authentication]
* (https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
* @return value or {@code null} for none
*/
public java.util.List getAccessoryWidgets() {
return accessoryWidgets;
}
/**
* One or more interactive widgets that appear at the bottom of a message. You can add accessory
* widgets to messages that contain text, cards, or both text and cards. Not supported for
* messages that contain dialogs. For details, see [Add interactive widgets at the bottom of a
* message](https://developers.google.com/workspace/chat/create-messages#add-accessory-widgets).
* Creating a message with accessory widgets requires [app authentication]
* (https://developers.google.com/workspace/chat/authenticate-authorize-chat-app).
* @param accessoryWidgets accessoryWidgets or {@code null} for none
*/
public Message setAccessoryWidgets(java.util.List accessoryWidgets) {
this.accessoryWidgets = accessoryWidgets;
return this;
}
/**
* Input only. Parameters that a Chat app can use to configure how its response is posted.
* @return value or {@code null} for none
*/
public ActionResponse getActionResponse() {
return actionResponse;
}
/**
* Input only. Parameters that a Chat app can use to configure how its response is posted.
* @param actionResponse actionResponse or {@code null} for none
*/
public Message setActionResponse(ActionResponse actionResponse) {
this.actionResponse = actionResponse;
return this;
}
/**
* Output only. Annotations associated with the `text` in this message.
* @return value or {@code null} for none
*/
public java.util.List getAnnotations() {
return annotations;
}
/**
* Output only. Annotations associated with the `text` in this message.
* @param annotations annotations or {@code null} for none
*/
public Message setAnnotations(java.util.List annotations) {
this.annotations = annotations;
return this;
}
/**
* Output only. Plain-text body of the message with all Chat app mentions stripped out.
* @return value or {@code null} for none
*/
public java.lang.String getArgumentText() {
return argumentText;
}
/**
* Output only. Plain-text body of the message with all Chat app mentions stripped out.
* @param argumentText argumentText or {@code null} for none
*/
public Message setArgumentText(java.lang.String argumentText) {
this.argumentText = argumentText;
return this;
}
/**
* Output only. GIF images that are attached to the message.
* @return value or {@code null} for none
*/
public java.util.List getAttachedGifs() {
return attachedGifs;
}
/**
* Output only. GIF images that are attached to the message.
* @param attachedGifs attachedGifs or {@code null} for none
*/
public Message setAttachedGifs(java.util.List attachedGifs) {
this.attachedGifs = attachedGifs;
return this;
}
/**
* User-uploaded attachment.
* @return value or {@code null} for none
*/
public java.util.List getAttachment() {
return attachment;
}
/**
* User-uploaded attachment.
* @param attachment attachment or {@code null} for none
*/
public Message setAttachment(java.util.List attachment) {
this.attachment = attachment;
return this;
}
/**
* Deprecated: Use `cards_v2` instead. Rich, formatted, and interactive cards that you can use to
* display UI elements such as: formatted texts, buttons, and clickable images. Cards are normally
* displayed below the plain-text body of the message. `cards` and `cards_v2` can have a maximum
* size of 32 KB.
* @return value or {@code null} for none
*/
public java.util.List getCards() {
return cards;
}
/**
* Deprecated: Use `cards_v2` instead. Rich, formatted, and interactive cards that you can use to
* display UI elements such as: formatted texts, buttons, and clickable images. Cards are normally
* displayed below the plain-text body of the message. `cards` and `cards_v2` can have a maximum
* size of 32 KB.
* @param cards cards or {@code null} for none
*/
public Message setCards(java.util.List cards) {
this.cards = cards;
return this;
}
/**
* An array of [cards](https://developers.google.com/workspace/chat/api/reference/rest/v1/cards).
* Only Chat apps can create cards. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* messages can't contain cards. To learn how to create a message that contains cards, see [Send a
* message](https://developers.google.com/workspace/chat/create-messages). [Card
* builder](https://addons.gsuite.google.com/uikit/builder)
* @return value or {@code null} for none
*/
public java.util.List getCardsV2() {
return cardsV2;
}
/**
* An array of [cards](https://developers.google.com/workspace/chat/api/reference/rest/v1/cards).
* Only Chat apps can create cards. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* messages can't contain cards. To learn how to create a message that contains cards, see [Send a
* message](https://developers.google.com/workspace/chat/create-messages). [Card
* builder](https://addons.gsuite.google.com/uikit/builder)
* @param cardsV2 cardsV2 or {@code null} for none
*/
public Message setCardsV2(java.util.List cardsV2) {
this.cardsV2 = cardsV2;
return this;
}
/**
* Optional. A custom ID for the message. You can use field to identify a message, or to get,
* delete, or update a message. To set a custom ID, specify the [`messageId`](https://developers.g
* oogle.com/workspace/chat/api/reference/rest/v1/spaces.messages/create#body.QUERY_PARAMETERS.mes
* sage_id) field when you create the message. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @return value or {@code null} for none
*/
public java.lang.String getClientAssignedMessageId() {
return clientAssignedMessageId;
}
/**
* Optional. A custom ID for the message. You can use field to identify a message, or to get,
* delete, or update a message. To set a custom ID, specify the [`messageId`](https://developers.g
* oogle.com/workspace/chat/api/reference/rest/v1/spaces.messages/create#body.QUERY_PARAMETERS.mes
* sage_id) field when you create the message. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @param clientAssignedMessageId clientAssignedMessageId or {@code null} for none
*/
public Message setClientAssignedMessageId(java.lang.String clientAssignedMessageId) {
this.clientAssignedMessageId = clientAssignedMessageId;
return this;
}
/**
* Optional. Immutable. For spaces created in Chat, the time at which the message was created.
* This field is output only, except when used in import mode spaces. For import mode spaces, set
* this field to the historical timestamp at which the message was created in the source in order
* to preserve the original creation time.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Optional. Immutable. For spaces created in Chat, the time at which the message was created.
* This field is output only, except when used in import mode spaces. For import mode spaces, set
* this field to the historical timestamp at which the message was created in the source in order
* to preserve the original creation time.
* @param createTime createTime or {@code null} for none
*/
public Message setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. The time at which the message was deleted in Google Chat. If the message is never
* deleted, this field is empty.
* @return value or {@code null} for none
*/
public String getDeleteTime() {
return deleteTime;
}
/**
* Output only. The time at which the message was deleted in Google Chat. If the message is never
* deleted, this field is empty.
* @param deleteTime deleteTime or {@code null} for none
*/
public Message setDeleteTime(String deleteTime) {
this.deleteTime = deleteTime;
return this;
}
/**
* Output only. Information about a deleted message. A message is deleted when `delete_time` is
* set.
* @return value or {@code null} for none
*/
public DeletionMetadata getDeletionMetadata() {
return deletionMetadata;
}
/**
* Output only. Information about a deleted message. A message is deleted when `delete_time` is
* set.
* @param deletionMetadata deletionMetadata or {@code null} for none
*/
public Message setDeletionMetadata(DeletionMetadata deletionMetadata) {
this.deletionMetadata = deletionMetadata;
return this;
}
/**
* Output only. The list of emoji reaction summaries on the message.
* @return value or {@code null} for none
*/
public java.util.List getEmojiReactionSummaries() {
return emojiReactionSummaries;
}
/**
* Output only. The list of emoji reaction summaries on the message.
* @param emojiReactionSummaries emojiReactionSummaries or {@code null} for none
*/
public Message setEmojiReactionSummaries(java.util.List emojiReactionSummaries) {
this.emojiReactionSummaries = emojiReactionSummaries;
return this;
}
/**
* A plain-text description of the message's cards, used when the actual cards can't be
* displayed—for example, mobile notifications.
* @return value or {@code null} for none
*/
public java.lang.String getFallbackText() {
return fallbackText;
}
/**
* A plain-text description of the message's cards, used when the actual cards can't be
* displayed—for example, mobile notifications.
* @param fallbackText fallbackText or {@code null} for none
*/
public Message setFallbackText(java.lang.String fallbackText) {
this.fallbackText = fallbackText;
return this;
}
/**
* Output only. Contains the message `text` with markups added to communicate formatting. This
* field might not capture all formatting visible in the UI, but includes the following: * [Markup
* syntax](https://developers.google.com/workspace/chat/format-messages) for bold, italic,
* strikethrough, monospace, monospace block, and bulleted list. * [User
* mentions](https://developers.google.com/workspace/chat/format-messages#messages-@mention) using
* the format ``. * Custom hyperlinks using the format `<{url}|{rendered_text}>` where the first
* string is the URL and the second is the rendered text—for example, ``. * Custom emoji using the
* format `:{emoji_name}:`—for example, `:smile:`. This doesn't apply to Unicode emoji, such as
* `U+1F600` for a grinning face emoji. For more information, see [View text formatting sent in a
* message](https://developers.google.com/workspace/chat/format-
* messages#view_text_formatting_sent_in_a_message)
* @return value or {@code null} for none
*/
public java.lang.String getFormattedText() {
return formattedText;
}
/**
* Output only. Contains the message `text` with markups added to communicate formatting. This
* field might not capture all formatting visible in the UI, but includes the following: * [Markup
* syntax](https://developers.google.com/workspace/chat/format-messages) for bold, italic,
* strikethrough, monospace, monospace block, and bulleted list. * [User
* mentions](https://developers.google.com/workspace/chat/format-messages#messages-@mention) using
* the format ``. * Custom hyperlinks using the format `<{url}|{rendered_text}>` where the first
* string is the URL and the second is the rendered text—for example, ``. * Custom emoji using the
* format `:{emoji_name}:`—for example, `:smile:`. This doesn't apply to Unicode emoji, such as
* `U+1F600` for a grinning face emoji. For more information, see [View text formatting sent in a
* message](https://developers.google.com/workspace/chat/format-
* messages#view_text_formatting_sent_in_a_message)
* @param formattedText formattedText or {@code null} for none
*/
public Message setFormattedText(java.lang.String formattedText) {
this.formattedText = formattedText;
return this;
}
/**
* Output only. The time at which the message was last edited by a user. If the message has never
* been edited, this field is empty.
* @return value or {@code null} for none
*/
public String getLastUpdateTime() {
return lastUpdateTime;
}
/**
* Output only. The time at which the message was last edited by a user. If the message has never
* been edited, this field is empty.
* @param lastUpdateTime lastUpdateTime or {@code null} for none
*/
public Message setLastUpdateTime(String lastUpdateTime) {
this.lastUpdateTime = lastUpdateTime;
return this;
}
/**
* Output only. A URL in `spaces.messages.text` that matches a link preview pattern. For more
* information, see [Preview links](https://developers.google.com/workspace/chat/preview-links).
* @return value or {@code null} for none
*/
public MatchedUrl getMatchedUrl() {
return matchedUrl;
}
/**
* Output only. A URL in `spaces.messages.text` that matches a link preview pattern. For more
* information, see [Preview links](https://developers.google.com/workspace/chat/preview-links).
* @param matchedUrl matchedUrl or {@code null} for none
*/
public Message setMatchedUrl(MatchedUrl matchedUrl) {
this.matchedUrl = matchedUrl;
return this;
}
/**
* Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is
* the ID of the space where the message is posted and `{message}` is a system-assigned ID for the
* message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
* custom ID when you create a message, you can use this ID to specify the message in a request by
* replacing `{message}` with the value from the `clientAssignedMessageId` field. For example,
* `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is
* the ID of the space where the message is posted and `{message}` is a system-assigned ID for the
* message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
* custom ID when you create a message, you can use this ID to specify the message in a request by
* replacing `{message}` with the value from the `clientAssignedMessageId` field. For example,
* `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
* message](https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
* @param name name or {@code null} for none
*/
public Message setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Immutable. Input for creating a message, otherwise output only. The user that can view the
* message. When set, the message is private and only visible to the specified user and the Chat
* app. Link previews and attachments aren't supported for private messages. Only Chat apps can
* send private messages. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user) to send a
* message, the message can't be private and must omit this field. For details, see [Send a
* message privately](https://developers.google.com/workspace/chat/create-messages#private).
* @return value or {@code null} for none
*/
public User getPrivateMessageViewer() {
return privateMessageViewer;
}
/**
* Immutable. Input for creating a message, otherwise output only. The user that can view the
* message. When set, the message is private and only visible to the specified user and the Chat
* app. Link previews and attachments aren't supported for private messages. Only Chat apps can
* send private messages. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user) to send a
* message, the message can't be private and must omit this field. For details, see [Send a
* message privately](https://developers.google.com/workspace/chat/create-messages#private).
* @param privateMessageViewer privateMessageViewer or {@code null} for none
*/
public Message setPrivateMessageViewer(User privateMessageViewer) {
this.privateMessageViewer = privateMessageViewer;
return this;
}
/**
* Output only. Information about a message that's quoted by a Google Chat user in a space. Google
* Chat users can quote a message to reply to it.
* @return value or {@code null} for none
*/
public QuotedMessageMetadata getQuotedMessageMetadata() {
return quotedMessageMetadata;
}
/**
* Output only. Information about a message that's quoted by a Google Chat user in a space. Google
* Chat users can quote a message to reply to it.
* @param quotedMessageMetadata quotedMessageMetadata or {@code null} for none
*/
public Message setQuotedMessageMetadata(QuotedMessageMetadata quotedMessageMetadata) {
this.quotedMessageMetadata = quotedMessageMetadata;
return this;
}
/**
* Output only. The user who created the message. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* output populates the
* [user](https://developers.google.com/workspace/chat/api/reference/rest/v1/User) `name` and
* `type`.
* @return value or {@code null} for none
*/
public User getSender() {
return sender;
}
/**
* Output only. The user who created the message. If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* output populates the
* [user](https://developers.google.com/workspace/chat/api/reference/rest/v1/User) `name` and
* `type`.
* @param sender sender or {@code null} for none
*/
public Message setSender(User sender) {
this.sender = sender;
return this;
}
/**
* Output only. Slash command information, if applicable.
* @return value or {@code null} for none
*/
public SlashCommand getSlashCommand() {
return slashCommand;
}
/**
* Output only. Slash command information, if applicable.
* @param slashCommand slashCommand or {@code null} for none
*/
public Message setSlashCommand(SlashCommand slashCommand) {
this.slashCommand = slashCommand;
return this;
}
/**
* If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* output populates the
* [space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) `name`.
* @return value or {@code null} for none
*/
public Space getSpace() {
return space;
}
/**
* If your Chat app [authenticates as a
* user](https://developers.google.com/workspace/chat/authenticate-authorize-chat-user), the
* output populates the
* [space](https://developers.google.com/workspace/chat/api/reference/rest/v1/spaces) `name`.
* @param space space or {@code null} for none
*/
public Message setSpace(Space space) {
this.space = space;
return this;
}
/**
* Plain-text body of the message. The first link to an image, video, or web page generates a
* [preview chip](https://developers.google.com/workspace/chat/preview-links). You can also
* [@mention a Google Chat user](https://developers.google.com/workspace/chat/format-
* messages#messages-@mention), or everyone in the space. To learn about creating text messages,
* see [Send a message](https://developers.google.com/workspace/chat/create-messages).
* @return value or {@code null} for none
*/
public java.lang.String getText() {
return text;
}
/**
* Plain-text body of the message. The first link to an image, video, or web page generates a
* [preview chip](https://developers.google.com/workspace/chat/preview-links). You can also
* [@mention a Google Chat user](https://developers.google.com/workspace/chat/format-
* messages#messages-@mention), or everyone in the space. To learn about creating text messages,
* see [Send a message](https://developers.google.com/workspace/chat/create-messages).
* @param text text or {@code null} for none
*/
public Message setText(java.lang.String text) {
this.text = text;
return this;
}
/**
* The thread the message belongs to. For example usage, see [Start or reply to a message
* thread](https://developers.google.com/workspace/chat/create-messages#create-message-thread).
* @return value or {@code null} for none
*/
public Thread getThread() {
return thread;
}
/**
* The thread the message belongs to. For example usage, see [Start or reply to a message
* thread](https://developers.google.com/workspace/chat/create-messages#create-message-thread).
* @param thread thread or {@code null} for none
*/
public Message setThread(Thread thread) {
this.thread = thread;
return this;
}
/**
* Output only. When `true`, the message is a response in a reply thread. When `false`, the
* message is visible in the space's top-level conversation as either the first message of a
* thread or a message with no threaded replies. If the space doesn't support reply in threads,
* this field is always `false`.
* @return value or {@code null} for none
*/
public java.lang.Boolean getThreadReply() {
return threadReply;
}
/**
* Output only. When `true`, the message is a response in a reply thread. When `false`, the
* message is visible in the space's top-level conversation as either the first message of a
* thread or a message with no threaded replies. If the space doesn't support reply in threads,
* this field is always `false`.
* @param threadReply threadReply or {@code null} for none
*/
public Message setThreadReply(java.lang.Boolean threadReply) {
this.threadReply = threadReply;
return this;
}
@Override
public Message set(String fieldName, Object value) {
return (Message) super.set(fieldName, value);
}
@Override
public Message clone() {
return (Message) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy