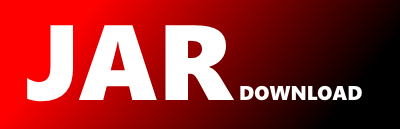
com.google.api.services.chat.v1.model.SetUpSpaceRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chat.v1.model;
/**
* Request to create a space and add specified users to it.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Chat API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SetUpSpaceRequest extends com.google.api.client.json.GenericJson {
/**
* Optional. The Google Chat users or groups to invite to join the space. Omit the calling user,
* as they are added automatically. The set currently allows up to 20 memberships (in addition to
* the caller). For human membership, the `Membership.member` field must contain a `user` with
* `name` populated (format: `users/{user}`) and `type` set to `User.Type.HUMAN`. You can only add
* human users when setting up a space (adding Chat apps is only supported for direct message
* setup with the calling app). You can also add members using the user's email as an alias for
* {user}. For example, the `user.name` can be `users/[email protected]`. To invite Gmail users or
* users from external Google Workspace domains, user's email must be used for `{user}`. For
* Google group membership, the `Membership.group_member` field must contain a `group` with `name`
* populated (format `groups/{group}`). You can only add Google groups when setting
* `Space.spaceType` to `SPACE`. Optional when setting `Space.spaceType` to `SPACE`. Required when
* setting `Space.spaceType` to `GROUP_CHAT`, along with at least two memberships. Required when
* setting `Space.spaceType` to `DIRECT_MESSAGE` with a human user, along with exactly one
* membership. Must be empty when creating a 1:1 conversation between a human and the calling Chat
* app (when setting `Space.spaceType` to `DIRECT_MESSAGE` and `Space.singleUserBotDm` to `true`).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List memberships;
static {
// hack to force ProGuard to consider Membership used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Membership.class);
}
/**
* Optional. A unique identifier for this request. A random UUID is recommended. Specifying an
* existing request ID returns the space created with that ID instead of creating a new space.
* Specifying an existing request ID from the same Chat app with a different authenticated user
* returns an error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/**
* Required. The `Space.spaceType` field is required. To create a space, set `Space.spaceType` to
* `SPACE` and set `Space.displayName`. If you receive the error message `ALREADY_EXISTS` when
* setting up a space, try a different `displayName`. An existing space within the Google
* Workspace organization might already use this display name. To create a group chat, set
* `Space.spaceType` to `GROUP_CHAT`. Don't set `Space.displayName`. To create a 1:1 conversation
* between humans, set `Space.spaceType` to `DIRECT_MESSAGE` and set `Space.singleUserBotDm` to
* `false`. Don't set `Space.displayName` or `Space.spaceDetails`. To create an 1:1 conversation
* between a human and the calling Chat app, set `Space.spaceType` to `DIRECT_MESSAGE` and
* `Space.singleUserBotDm` to `true`. Don't set `Space.displayName` or `Space.spaceDetails`. If a
* `DIRECT_MESSAGE` space already exists, that space is returned instead of creating a new space.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Space space;
/**
* Optional. The Google Chat users or groups to invite to join the space. Omit the calling user,
* as they are added automatically. The set currently allows up to 20 memberships (in addition to
* the caller). For human membership, the `Membership.member` field must contain a `user` with
* `name` populated (format: `users/{user}`) and `type` set to `User.Type.HUMAN`. You can only add
* human users when setting up a space (adding Chat apps is only supported for direct message
* setup with the calling app). You can also add members using the user's email as an alias for
* {user}. For example, the `user.name` can be `users/[email protected]`. To invite Gmail users or
* users from external Google Workspace domains, user's email must be used for `{user}`. For
* Google group membership, the `Membership.group_member` field must contain a `group` with `name`
* populated (format `groups/{group}`). You can only add Google groups when setting
* `Space.spaceType` to `SPACE`. Optional when setting `Space.spaceType` to `SPACE`. Required when
* setting `Space.spaceType` to `GROUP_CHAT`, along with at least two memberships. Required when
* setting `Space.spaceType` to `DIRECT_MESSAGE` with a human user, along with exactly one
* membership. Must be empty when creating a 1:1 conversation between a human and the calling Chat
* app (when setting `Space.spaceType` to `DIRECT_MESSAGE` and `Space.singleUserBotDm` to `true`).
* @return value or {@code null} for none
*/
public java.util.List getMemberships() {
return memberships;
}
/**
* Optional. The Google Chat users or groups to invite to join the space. Omit the calling user,
* as they are added automatically. The set currently allows up to 20 memberships (in addition to
* the caller). For human membership, the `Membership.member` field must contain a `user` with
* `name` populated (format: `users/{user}`) and `type` set to `User.Type.HUMAN`. You can only add
* human users when setting up a space (adding Chat apps is only supported for direct message
* setup with the calling app). You can also add members using the user's email as an alias for
* {user}. For example, the `user.name` can be `users/[email protected]`. To invite Gmail users or
* users from external Google Workspace domains, user's email must be used for `{user}`. For
* Google group membership, the `Membership.group_member` field must contain a `group` with `name`
* populated (format `groups/{group}`). You can only add Google groups when setting
* `Space.spaceType` to `SPACE`. Optional when setting `Space.spaceType` to `SPACE`. Required when
* setting `Space.spaceType` to `GROUP_CHAT`, along with at least two memberships. Required when
* setting `Space.spaceType` to `DIRECT_MESSAGE` with a human user, along with exactly one
* membership. Must be empty when creating a 1:1 conversation between a human and the calling Chat
* app (when setting `Space.spaceType` to `DIRECT_MESSAGE` and `Space.singleUserBotDm` to `true`).
* @param memberships memberships or {@code null} for none
*/
public SetUpSpaceRequest setMemberships(java.util.List memberships) {
this.memberships = memberships;
return this;
}
/**
* Optional. A unique identifier for this request. A random UUID is recommended. Specifying an
* existing request ID returns the space created with that ID instead of creating a new space.
* Specifying an existing request ID from the same Chat app with a different authenticated user
* returns an error.
* @return value or {@code null} for none
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique identifier for this request. A random UUID is recommended. Specifying an
* existing request ID returns the space created with that ID instead of creating a new space.
* Specifying an existing request ID from the same Chat app with a different authenticated user
* returns an error.
* @param requestId requestId or {@code null} for none
*/
public SetUpSpaceRequest setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. The `Space.spaceType` field is required. To create a space, set `Space.spaceType` to
* `SPACE` and set `Space.displayName`. If you receive the error message `ALREADY_EXISTS` when
* setting up a space, try a different `displayName`. An existing space within the Google
* Workspace organization might already use this display name. To create a group chat, set
* `Space.spaceType` to `GROUP_CHAT`. Don't set `Space.displayName`. To create a 1:1 conversation
* between humans, set `Space.spaceType` to `DIRECT_MESSAGE` and set `Space.singleUserBotDm` to
* `false`. Don't set `Space.displayName` or `Space.spaceDetails`. To create an 1:1 conversation
* between a human and the calling Chat app, set `Space.spaceType` to `DIRECT_MESSAGE` and
* `Space.singleUserBotDm` to `true`. Don't set `Space.displayName` or `Space.spaceDetails`. If a
* `DIRECT_MESSAGE` space already exists, that space is returned instead of creating a new space.
* @return value or {@code null} for none
*/
public Space getSpace() {
return space;
}
/**
* Required. The `Space.spaceType` field is required. To create a space, set `Space.spaceType` to
* `SPACE` and set `Space.displayName`. If you receive the error message `ALREADY_EXISTS` when
* setting up a space, try a different `displayName`. An existing space within the Google
* Workspace organization might already use this display name. To create a group chat, set
* `Space.spaceType` to `GROUP_CHAT`. Don't set `Space.displayName`. To create a 1:1 conversation
* between humans, set `Space.spaceType` to `DIRECT_MESSAGE` and set `Space.singleUserBotDm` to
* `false`. Don't set `Space.displayName` or `Space.spaceDetails`. To create an 1:1 conversation
* between a human and the calling Chat app, set `Space.spaceType` to `DIRECT_MESSAGE` and
* `Space.singleUserBotDm` to `true`. Don't set `Space.displayName` or `Space.spaceDetails`. If a
* `DIRECT_MESSAGE` space already exists, that space is returned instead of creating a new space.
* @param space space or {@code null} for none
*/
public SetUpSpaceRequest setSpace(Space space) {
this.space = space;
return this;
}
@Override
public SetUpSpaceRequest set(String fieldName, Object value) {
return (SetUpSpaceRequest) super.set(fieldName, value);
}
@Override
public SetUpSpaceRequest clone() {
return (SetUpSpaceRequest) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy