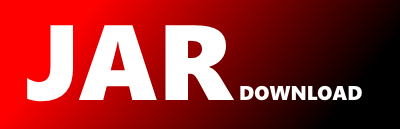
target.apidocs.com.google.api.services.chat.v1.HangoutsChat.Spaces.Messages.html Maven / Gradle / Ivy
HangoutsChat.Spaces.Messages (Google Chat API v1-rev20240829-2.0.0)
com.google.api.services.chat.v1
Class HangoutsChat.Spaces.Messages
- java.lang.Object
-
- com.google.api.services.chat.v1.HangoutsChat.Spaces.Messages
-
- Enclosing class:
- HangoutsChat.Spaces
public class HangoutsChat.Spaces.Messages
extends Object
The "messages" collection of methods.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
class
HangoutsChat.Spaces.Messages.Attachments
The "attachments" collection of methods.
class
HangoutsChat.Spaces.Messages.Create
class
HangoutsChat.Spaces.Messages.Delete
class
HangoutsChat.Spaces.Messages.Get
class
HangoutsChat.Spaces.Messages.List
class
HangoutsChat.Spaces.Messages.Patch
class
HangoutsChat.Spaces.Messages.Reactions
The "reactions" collection of methods.
class
HangoutsChat.Spaces.Messages.Update
-
Constructor Summary
Constructors
Constructor and Description
Messages()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
HangoutsChat.Spaces.Messages.Attachments
attachments()
An accessor for creating requests from the Attachments collection.
HangoutsChat.Spaces.Messages.Create
create(String parent,
Message content)
Creates a message in a Google Chat space.
HangoutsChat.Spaces.Messages.Delete
delete(String name)
Deletes a message.
HangoutsChat.Spaces.Messages.Get
get(String name)
Returns details about a message.
HangoutsChat.Spaces.Messages.List
list(String parent)
Lists messages in a space that the caller is a member of, including messages from blocked members
and spaces.
HangoutsChat.Spaces.Messages.Patch
patch(String name,
Message content)
Updates a message.
HangoutsChat.Spaces.Messages.Reactions
reactions()
An accessor for creating requests from the Reactions collection.
HangoutsChat.Spaces.Messages.Update
update(String name,
Message content)
Updates a message.
-
-
Method Detail
-
create
public HangoutsChat.Spaces.Messages.Create create(String parent,
Message content)
throws IOException
Creates a message in a Google Chat space. For an example, see [Send a
message](https://developers.google.com/workspace/chat/create-messages). The `create()` method
requires either user or app authentication. Chat attributes the message sender differently
depending on the type of authentication that you use in your request. The following image shows
how Chat attributes a message when you use app authentication. Chat displays the Chat app as the
message sender. The content of the message can contain text (`text`), cards (`cardsV2`), and
accessory widgets (`accessoryWidgets`).  The
following image shows how Chat attributes a message when you use user authentication. Chat
displays the user as the message sender and attributes the Chat app to the message by displaying
its name. The content of message can only contain text (`text`).  The
maximum message size, including the message contents, is 32,000 bytes.
Create a request for the method "messages.create".
This request holds the parameters needed by the chat server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
parent
- Required. The resource name of the space in which to create a message. Format: `spaces/{space}`
content
- the Message
- Returns:
- the request
- Throws:
IOException
-
delete
public HangoutsChat.Spaces.Messages.Delete delete(String name)
throws IOException
Deletes a message. For an example, see [Delete a
message](https://developers.google.com/workspace/chat/delete-messages). Requires
[authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
[app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
authorize-chat-user). When using app authentication, requests can only delete messages created by
the calling Chat app.
Create a request for the method "messages.delete".
This request holds the parameters needed by the chat server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
custom ID for your message, you can use the value from the `clientAssignedMessageId` field
for `{message}`. For details, see [Name a message]
(https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
- Returns:
- the request
- Throws:
IOException
-
get
public HangoutsChat.Spaces.Messages.Get get(String name)
throws IOException
Returns details about a message. For an example, see [Get details about a
message](https://developers.google.com/workspace/chat/get-messages). Requires
[authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
[app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
authorize-chat-user). Note: Might return a message from a blocked member or space.
Create a request for the method "messages.get".
This request holds the parameters needed by the chat server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Required. Resource name of the message. Format: `spaces/{space}/messages/{message}` If you've set a
custom ID for your message, you can use the value from the `clientAssignedMessageId` field
for `{message}`. For details, see [Name a message]
(https://developers.google.com/workspace/chat/create-messages#name_a_created_message).
- Returns:
- the request
- Throws:
IOException
-
list
public HangoutsChat.Spaces.Messages.List list(String parent)
throws IOException
Lists messages in a space that the caller is a member of, including messages from blocked members
and spaces. If you list messages from a space with no messages, the response is an empty object.
When using a REST/HTTP interface, the response contains an empty JSON object, `{}`. For an
example, see [List
messages](https://developers.google.com/workspace/chat/api/guides/v1/messages/list). Requires
[user authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
user).
Create a request for the method "messages.list".
This request holds the parameters needed by the chat server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
parent
- Required. The resource name of the space to list messages from. Format: `spaces/{space}`
- Returns:
- the request
- Throws:
IOException
-
patch
public HangoutsChat.Spaces.Messages.Patch patch(String name,
Message content)
throws IOException
Updates a message. There's a difference between the `patch` and `update` methods. The `patch`
method uses a `patch` request while the `update` method uses a `put` request. We recommend using
the `patch` method. For an example, see [Update a
message](https://developers.google.com/workspace/chat/update-messages). Requires
[authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
[app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
authorize-chat-user). When using app authentication, requests can only update messages created by
the calling Chat app.
Create a request for the method "messages.patch".
This request holds the parameters needed by the chat server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
of the space where the message is posted and `{message}` is a system-assigned ID for the
message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
custom ID when you create a message, you can use this ID to specify the message in a
request by replacing `{message}` with the value from the `clientAssignedMessageId` field.
For example, `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
message](https://developers.google.com/workspace/chat/create-
messages#name_a_created_message).
content
- the Message
- Returns:
- the request
- Throws:
IOException
-
update
public HangoutsChat.Spaces.Messages.Update update(String name,
Message content)
throws IOException
Updates a message. There's a difference between the `patch` and `update` methods. The `patch`
method uses a `patch` request while the `update` method uses a `put` request. We recommend using
the `patch` method. For an example, see [Update a
message](https://developers.google.com/workspace/chat/update-messages). Requires
[authentication](https://developers.google.com/workspace/chat/authenticate-authorize). Supports
[app authentication](https://developers.google.com/workspace/chat/authenticate-authorize-chat-
app) and [user authentication](https://developers.google.com/workspace/chat/authenticate-
authorize-chat-user). When using app authentication, requests can only update messages created by
the calling Chat app.
Create a request for the method "messages.update".
This request holds the parameters needed by the chat server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Resource name of the message. Format: `spaces/{space}/messages/{message}` Where `{space}` is the ID
of the space where the message is posted and `{message}` is a system-assigned ID for the
message. For example, `spaces/AAAAAAAAAAA/messages/BBBBBBBBBBB.BBBBBBBBBBB`. If you set a
custom ID when you create a message, you can use this ID to specify the message in a
request by replacing `{message}` with the value from the `clientAssignedMessageId` field.
For example, `spaces/AAAAAAAAAAA/messages/client-custom-name`. For details, see [Name a
message](https://developers.google.com/workspace/chat/create-
messages#name_a_created_message).
content
- the Message
- Returns:
- the request
- Throws:
IOException
-
attachments
public HangoutsChat.Spaces.Messages.Attachments attachments()
An accessor for creating requests from the Attachments collection.
The typical use is:
HangoutsChat chat = new HangoutsChat(...);
HangoutsChat.Attachments.List request = chat.attachments().list(parameters ...)
- Returns:
- the resource collection
-
reactions
public HangoutsChat.Spaces.Messages.Reactions reactions()
An accessor for creating requests from the Reactions collection.
The typical use is:
HangoutsChat chat = new HangoutsChat(...);
HangoutsChat.Reactions.List request = chat.reactions().list(parameters ...)
- Returns:
- the resource collection
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy