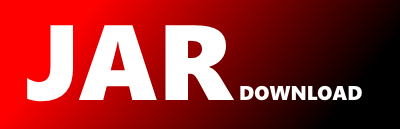
com.google.api.services.chromemanagement.v1.model.GoogleChromeManagementV1MemoryInfo Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chromemanagement.v1.model;
/**
* Memory information of a device. * This field has both telemetry and device information: -
* `totalRamBytes` - Device information - `availableRamBytes` - Telemetry information -
* `totalMemoryEncryption` - Device information * Data for this field is controlled via policy:
* [ReportDeviceMemoryInfo](https://chromeenterprise.google/policies/#ReportDeviceMemoryInfo) * Data
* Collection Frequency: - `totalRamBytes` - Only at upload - `availableRamBytes` - Every 10 minutes
* - `totalMemoryEncryption` - at device startup * Default Data Reporting Frequency: -
* `totalRamBytes` - 3 hours - `availableRamBytes` - 3 hours - `totalMemoryEncryption` - at device
* startup - Policy Controlled: Yes * Cache: If the device is offline, the collected data is stored
* locally, and will be reported when the device is next online: only for `totalMemoryEncryption` *
* Reported for affiliated users only: N/A * Granular permission needed: TELEMETRY_API_MEMORY_INFO
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Chrome Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleChromeManagementV1MemoryInfo extends com.google.api.client.json.GenericJson {
/**
* Output only. Amount of available RAM in bytes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long availableRamBytes;
/**
* Output only. Total memory encryption info for the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TotalMemoryEncryptionInfo totalMemoryEncryption;
/**
* Output only. Total RAM in bytes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalRamBytes;
/**
* Output only. Amount of available RAM in bytes.
* @return value or {@code null} for none
*/
public java.lang.Long getAvailableRamBytes() {
return availableRamBytes;
}
/**
* Output only. Amount of available RAM in bytes.
* @param availableRamBytes availableRamBytes or {@code null} for none
*/
public GoogleChromeManagementV1MemoryInfo setAvailableRamBytes(java.lang.Long availableRamBytes) {
this.availableRamBytes = availableRamBytes;
return this;
}
/**
* Output only. Total memory encryption info for the device.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TotalMemoryEncryptionInfo getTotalMemoryEncryption() {
return totalMemoryEncryption;
}
/**
* Output only. Total memory encryption info for the device.
* @param totalMemoryEncryption totalMemoryEncryption or {@code null} for none
*/
public GoogleChromeManagementV1MemoryInfo setTotalMemoryEncryption(GoogleChromeManagementV1TotalMemoryEncryptionInfo totalMemoryEncryption) {
this.totalMemoryEncryption = totalMemoryEncryption;
return this;
}
/**
* Output only. Total RAM in bytes.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalRamBytes() {
return totalRamBytes;
}
/**
* Output only. Total RAM in bytes.
* @param totalRamBytes totalRamBytes or {@code null} for none
*/
public GoogleChromeManagementV1MemoryInfo setTotalRamBytes(java.lang.Long totalRamBytes) {
this.totalRamBytes = totalRamBytes;
return this;
}
@Override
public GoogleChromeManagementV1MemoryInfo set(String fieldName, Object value) {
return (GoogleChromeManagementV1MemoryInfo) super.set(fieldName, value);
}
@Override
public GoogleChromeManagementV1MemoryInfo clone() {
return (GoogleChromeManagementV1MemoryInfo) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy