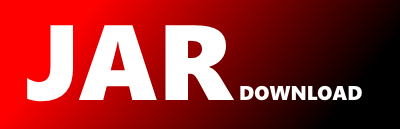
com.google.api.services.chromemanagement.v1.model.GoogleChromeManagementV1TelemetryDevice Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chromemanagement.v1.model;
/**
* Telemetry data collected from a managed device. * Granular permission needed:
* TELEMETRY_API_DEVICE
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Chrome Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleChromeManagementV1TelemetryDevice extends com.google.api.client.json.GenericJson {
/**
* Output only. App reports collected periodically sorted in a decreasing order of report_time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List appReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1AppReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1AppReport.class);
}
/**
* Output only. Audio reports collected periodically sorted in a decreasing order of report_time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List audioStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1AudioStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1AudioStatusReport.class);
}
/**
* Output only. Information on battery specs for the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List batteryInfo;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1BatteryInfo used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1BatteryInfo.class);
}
/**
* Output only. Battery reports collected periodically.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List batteryStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1BatteryStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1BatteryStatusReport.class);
}
/**
* Output only. Boot performance reports of the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List bootPerformanceReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1BootPerformanceReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1BootPerformanceReport.class);
}
/**
* Output only. Information regarding CPU specs for the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cpuInfo;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1CpuInfo used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1CpuInfo.class);
}
/**
* Output only. CPU status reports collected periodically sorted in a decreasing order of
* report_time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cpuStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1CpuStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1CpuStatusReport.class);
}
/**
* Output only. Google Workspace Customer whose enterprise enrolled the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/**
* Output only. The unique Directory API ID of the device. This value is the same as the Admin
* Console's Directory API ID in the ChromeOS Devices tab
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deviceId;
/**
* Output only. Contains information regarding Graphic peripherals for the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1GraphicsInfo graphicsInfo;
/**
* Output only. Graphics reports collected periodically.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List graphicsStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1GraphicsStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1GraphicsStatusReport.class);
}
/**
* Output only. Heartbeat status report containing timestamps periodically sorted in decreasing
* order of report_time
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List heartbeatStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1HeartbeatStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1HeartbeatStatusReport.class);
}
/**
* Output only. Kiosk app status report for the kiosk device
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List kioskAppStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1KioskAppStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1KioskAppStatusReport.class);
}
/**
* Output only. Information regarding memory specs for the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1MemoryInfo memoryInfo;
/**
* Output only. Memory status reports collected periodically sorted decreasing by report_time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List memoryStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1MemoryStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1MemoryStatusReport.class);
}
/**
* Output only. Resource name of the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. Network bandwidth reports collected periodically sorted in a decreasing order of
* report_time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List networkBandwidthReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1NetworkBandwidthReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1NetworkBandwidthReport.class);
}
/**
* Output only. Network diagnostics collected periodically.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List networkDiagnosticsReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1NetworkDiagnosticsReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1NetworkDiagnosticsReport.class);
}
/**
* Output only. Network devices information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1NetworkInfo networkInfo;
/**
* Output only. Network specs collected periodically.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List networkStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1NetworkStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1NetworkStatusReport.class);
}
/**
* Output only. Organization unit ID of the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orgUnitId;
/**
* Output only. Contains relevant information regarding ChromeOS update status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List osUpdateStatus;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1OsUpdateStatus used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1OsUpdateStatus.class);
}
/**
* Output only. Peripherals reports collected periodically sorted in a decreasing order of
* report_time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List peripheralsReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1PeripheralsReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1PeripheralsReport.class);
}
/**
* Output only. Runtime counters reports collected device lifetime runtime, as well as the counts
* of S0->S3, S0->S4, and S0->S5 transitions, meaning entering into sleep, hibernation, and power-
* off states
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List runtimeCountersReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1RuntimeCountersReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1RuntimeCountersReport.class);
}
/**
* Output only. Device serial number. This value is the same as the Admin Console's Serial Number
* in the ChromeOS Devices tab.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serialNumber;
/**
* Output only. Information of storage specs for the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1StorageInfo storageInfo;
/**
* Output only. Storage reports collected periodically.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List storageStatusReport;
static {
// hack to force ProGuard to consider GoogleChromeManagementV1StorageStatusReport used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleChromeManagementV1StorageStatusReport.class);
}
/**
* Output only. Information on Thunderbolt bus.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List thunderboltInfo;
/**
* Output only. App reports collected periodically sorted in a decreasing order of report_time.
* @return value or {@code null} for none
*/
public java.util.List getAppReport() {
return appReport;
}
/**
* Output only. App reports collected periodically sorted in a decreasing order of report_time.
* @param appReport appReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setAppReport(java.util.List appReport) {
this.appReport = appReport;
return this;
}
/**
* Output only. Audio reports collected periodically sorted in a decreasing order of report_time.
* @return value or {@code null} for none
*/
public java.util.List getAudioStatusReport() {
return audioStatusReport;
}
/**
* Output only. Audio reports collected periodically sorted in a decreasing order of report_time.
* @param audioStatusReport audioStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setAudioStatusReport(java.util.List audioStatusReport) {
this.audioStatusReport = audioStatusReport;
return this;
}
/**
* Output only. Information on battery specs for the device.
* @return value or {@code null} for none
*/
public java.util.List getBatteryInfo() {
return batteryInfo;
}
/**
* Output only. Information on battery specs for the device.
* @param batteryInfo batteryInfo or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setBatteryInfo(java.util.List batteryInfo) {
this.batteryInfo = batteryInfo;
return this;
}
/**
* Output only. Battery reports collected periodically.
* @return value or {@code null} for none
*/
public java.util.List getBatteryStatusReport() {
return batteryStatusReport;
}
/**
* Output only. Battery reports collected periodically.
* @param batteryStatusReport batteryStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setBatteryStatusReport(java.util.List batteryStatusReport) {
this.batteryStatusReport = batteryStatusReport;
return this;
}
/**
* Output only. Boot performance reports of the device.
* @return value or {@code null} for none
*/
public java.util.List getBootPerformanceReport() {
return bootPerformanceReport;
}
/**
* Output only. Boot performance reports of the device.
* @param bootPerformanceReport bootPerformanceReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setBootPerformanceReport(java.util.List bootPerformanceReport) {
this.bootPerformanceReport = bootPerformanceReport;
return this;
}
/**
* Output only. Information regarding CPU specs for the device.
* @return value or {@code null} for none
*/
public java.util.List getCpuInfo() {
return cpuInfo;
}
/**
* Output only. Information regarding CPU specs for the device.
* @param cpuInfo cpuInfo or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setCpuInfo(java.util.List cpuInfo) {
this.cpuInfo = cpuInfo;
return this;
}
/**
* Output only. CPU status reports collected periodically sorted in a decreasing order of
* report_time.
* @return value or {@code null} for none
*/
public java.util.List getCpuStatusReport() {
return cpuStatusReport;
}
/**
* Output only. CPU status reports collected periodically sorted in a decreasing order of
* report_time.
* @param cpuStatusReport cpuStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setCpuStatusReport(java.util.List cpuStatusReport) {
this.cpuStatusReport = cpuStatusReport;
return this;
}
/**
* Output only. Google Workspace Customer whose enterprise enrolled the device.
* @return value or {@code null} for none
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Output only. Google Workspace Customer whose enterprise enrolled the device.
* @param customer customer or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
/**
* Output only. The unique Directory API ID of the device. This value is the same as the Admin
* Console's Directory API ID in the ChromeOS Devices tab
* @return value or {@code null} for none
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/**
* Output only. The unique Directory API ID of the device. This value is the same as the Admin
* Console's Directory API ID in the ChromeOS Devices tab
* @param deviceId deviceId or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/**
* Output only. Contains information regarding Graphic peripherals for the device.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1GraphicsInfo getGraphicsInfo() {
return graphicsInfo;
}
/**
* Output only. Contains information regarding Graphic peripherals for the device.
* @param graphicsInfo graphicsInfo or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setGraphicsInfo(GoogleChromeManagementV1GraphicsInfo graphicsInfo) {
this.graphicsInfo = graphicsInfo;
return this;
}
/**
* Output only. Graphics reports collected periodically.
* @return value or {@code null} for none
*/
public java.util.List getGraphicsStatusReport() {
return graphicsStatusReport;
}
/**
* Output only. Graphics reports collected periodically.
* @param graphicsStatusReport graphicsStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setGraphicsStatusReport(java.util.List graphicsStatusReport) {
this.graphicsStatusReport = graphicsStatusReport;
return this;
}
/**
* Output only. Heartbeat status report containing timestamps periodically sorted in decreasing
* order of report_time
* @return value or {@code null} for none
*/
public java.util.List getHeartbeatStatusReport() {
return heartbeatStatusReport;
}
/**
* Output only. Heartbeat status report containing timestamps periodically sorted in decreasing
* order of report_time
* @param heartbeatStatusReport heartbeatStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setHeartbeatStatusReport(java.util.List heartbeatStatusReport) {
this.heartbeatStatusReport = heartbeatStatusReport;
return this;
}
/**
* Output only. Kiosk app status report for the kiosk device
* @return value or {@code null} for none
*/
public java.util.List getKioskAppStatusReport() {
return kioskAppStatusReport;
}
/**
* Output only. Kiosk app status report for the kiosk device
* @param kioskAppStatusReport kioskAppStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setKioskAppStatusReport(java.util.List kioskAppStatusReport) {
this.kioskAppStatusReport = kioskAppStatusReport;
return this;
}
/**
* Output only. Information regarding memory specs for the device.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1MemoryInfo getMemoryInfo() {
return memoryInfo;
}
/**
* Output only. Information regarding memory specs for the device.
* @param memoryInfo memoryInfo or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setMemoryInfo(GoogleChromeManagementV1MemoryInfo memoryInfo) {
this.memoryInfo = memoryInfo;
return this;
}
/**
* Output only. Memory status reports collected periodically sorted decreasing by report_time.
* @return value or {@code null} for none
*/
public java.util.List getMemoryStatusReport() {
return memoryStatusReport;
}
/**
* Output only. Memory status reports collected periodically sorted decreasing by report_time.
* @param memoryStatusReport memoryStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setMemoryStatusReport(java.util.List memoryStatusReport) {
this.memoryStatusReport = memoryStatusReport;
return this;
}
/**
* Output only. Resource name of the device.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the device.
* @param name name or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. Network bandwidth reports collected periodically sorted in a decreasing order of
* report_time.
* @return value or {@code null} for none
*/
public java.util.List getNetworkBandwidthReport() {
return networkBandwidthReport;
}
/**
* Output only. Network bandwidth reports collected periodically sorted in a decreasing order of
* report_time.
* @param networkBandwidthReport networkBandwidthReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setNetworkBandwidthReport(java.util.List networkBandwidthReport) {
this.networkBandwidthReport = networkBandwidthReport;
return this;
}
/**
* Output only. Network diagnostics collected periodically.
* @return value or {@code null} for none
*/
public java.util.List getNetworkDiagnosticsReport() {
return networkDiagnosticsReport;
}
/**
* Output only. Network diagnostics collected periodically.
* @param networkDiagnosticsReport networkDiagnosticsReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setNetworkDiagnosticsReport(java.util.List networkDiagnosticsReport) {
this.networkDiagnosticsReport = networkDiagnosticsReport;
return this;
}
/**
* Output only. Network devices information.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1NetworkInfo getNetworkInfo() {
return networkInfo;
}
/**
* Output only. Network devices information.
* @param networkInfo networkInfo or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setNetworkInfo(GoogleChromeManagementV1NetworkInfo networkInfo) {
this.networkInfo = networkInfo;
return this;
}
/**
* Output only. Network specs collected periodically.
* @return value or {@code null} for none
*/
public java.util.List getNetworkStatusReport() {
return networkStatusReport;
}
/**
* Output only. Network specs collected periodically.
* @param networkStatusReport networkStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setNetworkStatusReport(java.util.List networkStatusReport) {
this.networkStatusReport = networkStatusReport;
return this;
}
/**
* Output only. Organization unit ID of the device.
* @return value or {@code null} for none
*/
public java.lang.String getOrgUnitId() {
return orgUnitId;
}
/**
* Output only. Organization unit ID of the device.
* @param orgUnitId orgUnitId or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setOrgUnitId(java.lang.String orgUnitId) {
this.orgUnitId = orgUnitId;
return this;
}
/**
* Output only. Contains relevant information regarding ChromeOS update status.
* @return value or {@code null} for none
*/
public java.util.List getOsUpdateStatus() {
return osUpdateStatus;
}
/**
* Output only. Contains relevant information regarding ChromeOS update status.
* @param osUpdateStatus osUpdateStatus or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setOsUpdateStatus(java.util.List osUpdateStatus) {
this.osUpdateStatus = osUpdateStatus;
return this;
}
/**
* Output only. Peripherals reports collected periodically sorted in a decreasing order of
* report_time.
* @return value or {@code null} for none
*/
public java.util.List getPeripheralsReport() {
return peripheralsReport;
}
/**
* Output only. Peripherals reports collected periodically sorted in a decreasing order of
* report_time.
* @param peripheralsReport peripheralsReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setPeripheralsReport(java.util.List peripheralsReport) {
this.peripheralsReport = peripheralsReport;
return this;
}
/**
* Output only. Runtime counters reports collected device lifetime runtime, as well as the counts
* of S0->S3, S0->S4, and S0->S5 transitions, meaning entering into sleep, hibernation, and power-
* off states
* @return value or {@code null} for none
*/
public java.util.List getRuntimeCountersReport() {
return runtimeCountersReport;
}
/**
* Output only. Runtime counters reports collected device lifetime runtime, as well as the counts
* of S0->S3, S0->S4, and S0->S5 transitions, meaning entering into sleep, hibernation, and power-
* off states
* @param runtimeCountersReport runtimeCountersReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setRuntimeCountersReport(java.util.List runtimeCountersReport) {
this.runtimeCountersReport = runtimeCountersReport;
return this;
}
/**
* Output only. Device serial number. This value is the same as the Admin Console's Serial Number
* in the ChromeOS Devices tab.
* @return value or {@code null} for none
*/
public java.lang.String getSerialNumber() {
return serialNumber;
}
/**
* Output only. Device serial number. This value is the same as the Admin Console's Serial Number
* in the ChromeOS Devices tab.
* @param serialNumber serialNumber or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setSerialNumber(java.lang.String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
/**
* Output only. Information of storage specs for the device.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1StorageInfo getStorageInfo() {
return storageInfo;
}
/**
* Output only. Information of storage specs for the device.
* @param storageInfo storageInfo or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setStorageInfo(GoogleChromeManagementV1StorageInfo storageInfo) {
this.storageInfo = storageInfo;
return this;
}
/**
* Output only. Storage reports collected periodically.
* @return value or {@code null} for none
*/
public java.util.List getStorageStatusReport() {
return storageStatusReport;
}
/**
* Output only. Storage reports collected periodically.
* @param storageStatusReport storageStatusReport or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setStorageStatusReport(java.util.List storageStatusReport) {
this.storageStatusReport = storageStatusReport;
return this;
}
/**
* Output only. Information on Thunderbolt bus.
* @return value or {@code null} for none
*/
public java.util.List getThunderboltInfo() {
return thunderboltInfo;
}
/**
* Output only. Information on Thunderbolt bus.
* @param thunderboltInfo thunderboltInfo or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDevice setThunderboltInfo(java.util.List thunderboltInfo) {
this.thunderboltInfo = thunderboltInfo;
return this;
}
@Override
public GoogleChromeManagementV1TelemetryDevice set(String fieldName, Object value) {
return (GoogleChromeManagementV1TelemetryDevice) super.set(fieldName, value);
}
@Override
public GoogleChromeManagementV1TelemetryDevice clone() {
return (GoogleChromeManagementV1TelemetryDevice) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy