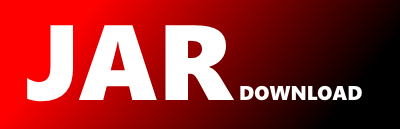
com.google.api.services.chromemanagement.v1.model.GoogleChromeManagementV1TelemetryEvent Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chromemanagement.v1.model;
/**
* Telemetry data reported by a managed device.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Chrome Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleChromeManagementV1TelemetryEvent extends com.google.api.client.json.GenericJson {
/**
* Output only. Payload for app install event. Present only when `event_type` is `APP_INSTALLED`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryAppInstallEvent appInstallEvent;
/**
* Output only. Payload for app launch event.Present only when `event_type` is `APP_LAUNCHED`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryAppLaunchEvent appLaunchEvent;
/**
* Output only. Payload for app uninstall event. Present only when `event_type` is
* `APP_UNINSTALLED`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryAppUninstallEvent appUninstallEvent;
/**
* Output only. Payload for audio severe underrun event. Present only when the `event_type` field
* is `AUDIO_SEVERE_UNDERRUN`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryAudioSevereUnderrunEvent audioSevereUnderrunEvent;
/**
* Output only. Information about the device associated with the event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryDeviceInfo device;
/**
* The event type of the current event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String eventType;
/**
* Output only. Payload for HTTPS latency change event. Present only when `event_type` is
* `NETWORK_HTTPS_LATENCY_CHANGE`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryHttpsLatencyChangeEvent httpsLatencyChangeEvent;
/**
* Output only. Resource name of the event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. Payload for network connection state change event. Present only when `event_type`
* is `NETWORK_STATE_CHANGE`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryNetworkConnectionStateChangeEvent networkStateChangeEvent;
/**
* Timestamp that represents when the event was reported.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String reportTime;
/**
* Output only. Payload for usb peripherals event. Present only when the `event_type` field is
* either `USB_ADDED` or `USB_REMOVED`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryUsbPeripheralsEvent usbPeripheralsEvent;
/**
* Output only. Information about the user associated with the event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryUserInfo user;
/**
* Output only. Payload for VPN connection state change event. Present only when `event_type` is
* `VPN_CONNECTION_STATE_CHANGE`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryNetworkConnectionStateChangeEvent vpnConnectionStateChangeEvent;
/**
* Output only. Payload for WiFi signal strength events. Present only when `event_type` is
* `WIFI_SIGNAL_STRENGTH_LOW` or `WIFI_SIGNAL_STRENGTH_RECOVERED`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementV1TelemetryNetworkSignalStrengthEvent wifiSignalStrengthEvent;
/**
* Output only. Payload for app install event. Present only when `event_type` is `APP_INSTALLED`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryAppInstallEvent getAppInstallEvent() {
return appInstallEvent;
}
/**
* Output only. Payload for app install event. Present only when `event_type` is `APP_INSTALLED`.
* @param appInstallEvent appInstallEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setAppInstallEvent(GoogleChromeManagementV1TelemetryAppInstallEvent appInstallEvent) {
this.appInstallEvent = appInstallEvent;
return this;
}
/**
* Output only. Payload for app launch event.Present only when `event_type` is `APP_LAUNCHED`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryAppLaunchEvent getAppLaunchEvent() {
return appLaunchEvent;
}
/**
* Output only. Payload for app launch event.Present only when `event_type` is `APP_LAUNCHED`.
* @param appLaunchEvent appLaunchEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setAppLaunchEvent(GoogleChromeManagementV1TelemetryAppLaunchEvent appLaunchEvent) {
this.appLaunchEvent = appLaunchEvent;
return this;
}
/**
* Output only. Payload for app uninstall event. Present only when `event_type` is
* `APP_UNINSTALLED`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryAppUninstallEvent getAppUninstallEvent() {
return appUninstallEvent;
}
/**
* Output only. Payload for app uninstall event. Present only when `event_type` is
* `APP_UNINSTALLED`.
* @param appUninstallEvent appUninstallEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setAppUninstallEvent(GoogleChromeManagementV1TelemetryAppUninstallEvent appUninstallEvent) {
this.appUninstallEvent = appUninstallEvent;
return this;
}
/**
* Output only. Payload for audio severe underrun event. Present only when the `event_type` field
* is `AUDIO_SEVERE_UNDERRUN`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryAudioSevereUnderrunEvent getAudioSevereUnderrunEvent() {
return audioSevereUnderrunEvent;
}
/**
* Output only. Payload for audio severe underrun event. Present only when the `event_type` field
* is `AUDIO_SEVERE_UNDERRUN`.
* @param audioSevereUnderrunEvent audioSevereUnderrunEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setAudioSevereUnderrunEvent(GoogleChromeManagementV1TelemetryAudioSevereUnderrunEvent audioSevereUnderrunEvent) {
this.audioSevereUnderrunEvent = audioSevereUnderrunEvent;
return this;
}
/**
* Output only. Information about the device associated with the event.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryDeviceInfo getDevice() {
return device;
}
/**
* Output only. Information about the device associated with the event.
* @param device device or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setDevice(GoogleChromeManagementV1TelemetryDeviceInfo device) {
this.device = device;
return this;
}
/**
* The event type of the current event.
* @return value or {@code null} for none
*/
public java.lang.String getEventType() {
return eventType;
}
/**
* The event type of the current event.
* @param eventType eventType or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setEventType(java.lang.String eventType) {
this.eventType = eventType;
return this;
}
/**
* Output only. Payload for HTTPS latency change event. Present only when `event_type` is
* `NETWORK_HTTPS_LATENCY_CHANGE`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryHttpsLatencyChangeEvent getHttpsLatencyChangeEvent() {
return httpsLatencyChangeEvent;
}
/**
* Output only. Payload for HTTPS latency change event. Present only when `event_type` is
* `NETWORK_HTTPS_LATENCY_CHANGE`.
* @param httpsLatencyChangeEvent httpsLatencyChangeEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setHttpsLatencyChangeEvent(GoogleChromeManagementV1TelemetryHttpsLatencyChangeEvent httpsLatencyChangeEvent) {
this.httpsLatencyChangeEvent = httpsLatencyChangeEvent;
return this;
}
/**
* Output only. Resource name of the event.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the event.
* @param name name or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. Payload for network connection state change event. Present only when `event_type`
* is `NETWORK_STATE_CHANGE`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryNetworkConnectionStateChangeEvent getNetworkStateChangeEvent() {
return networkStateChangeEvent;
}
/**
* Output only. Payload for network connection state change event. Present only when `event_type`
* is `NETWORK_STATE_CHANGE`.
* @param networkStateChangeEvent networkStateChangeEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setNetworkStateChangeEvent(GoogleChromeManagementV1TelemetryNetworkConnectionStateChangeEvent networkStateChangeEvent) {
this.networkStateChangeEvent = networkStateChangeEvent;
return this;
}
/**
* Timestamp that represents when the event was reported.
* @return value or {@code null} for none
*/
public String getReportTime() {
return reportTime;
}
/**
* Timestamp that represents when the event was reported.
* @param reportTime reportTime or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setReportTime(String reportTime) {
this.reportTime = reportTime;
return this;
}
/**
* Output only. Payload for usb peripherals event. Present only when the `event_type` field is
* either `USB_ADDED` or `USB_REMOVED`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryUsbPeripheralsEvent getUsbPeripheralsEvent() {
return usbPeripheralsEvent;
}
/**
* Output only. Payload for usb peripherals event. Present only when the `event_type` field is
* either `USB_ADDED` or `USB_REMOVED`.
* @param usbPeripheralsEvent usbPeripheralsEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setUsbPeripheralsEvent(GoogleChromeManagementV1TelemetryUsbPeripheralsEvent usbPeripheralsEvent) {
this.usbPeripheralsEvent = usbPeripheralsEvent;
return this;
}
/**
* Output only. Information about the user associated with the event.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryUserInfo getUser() {
return user;
}
/**
* Output only. Information about the user associated with the event.
* @param user user or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setUser(GoogleChromeManagementV1TelemetryUserInfo user) {
this.user = user;
return this;
}
/**
* Output only. Payload for VPN connection state change event. Present only when `event_type` is
* `VPN_CONNECTION_STATE_CHANGE`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryNetworkConnectionStateChangeEvent getVpnConnectionStateChangeEvent() {
return vpnConnectionStateChangeEvent;
}
/**
* Output only. Payload for VPN connection state change event. Present only when `event_type` is
* `VPN_CONNECTION_STATE_CHANGE`.
* @param vpnConnectionStateChangeEvent vpnConnectionStateChangeEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setVpnConnectionStateChangeEvent(GoogleChromeManagementV1TelemetryNetworkConnectionStateChangeEvent vpnConnectionStateChangeEvent) {
this.vpnConnectionStateChangeEvent = vpnConnectionStateChangeEvent;
return this;
}
/**
* Output only. Payload for WiFi signal strength events. Present only when `event_type` is
* `WIFI_SIGNAL_STRENGTH_LOW` or `WIFI_SIGNAL_STRENGTH_RECOVERED`.
* @return value or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryNetworkSignalStrengthEvent getWifiSignalStrengthEvent() {
return wifiSignalStrengthEvent;
}
/**
* Output only. Payload for WiFi signal strength events. Present only when `event_type` is
* `WIFI_SIGNAL_STRENGTH_LOW` or `WIFI_SIGNAL_STRENGTH_RECOVERED`.
* @param wifiSignalStrengthEvent wifiSignalStrengthEvent or {@code null} for none
*/
public GoogleChromeManagementV1TelemetryEvent setWifiSignalStrengthEvent(GoogleChromeManagementV1TelemetryNetworkSignalStrengthEvent wifiSignalStrengthEvent) {
this.wifiSignalStrengthEvent = wifiSignalStrengthEvent;
return this;
}
@Override
public GoogleChromeManagementV1TelemetryEvent set(String fieldName, Object value) {
return (GoogleChromeManagementV1TelemetryEvent) super.set(fieldName, value);
}
@Override
public GoogleChromeManagementV1TelemetryEvent clone() {
return (GoogleChromeManagementV1TelemetryEvent) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy