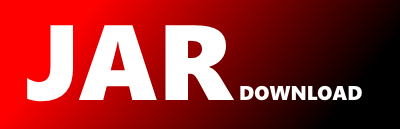
com.google.api.services.chromemanagement.v1.model.GoogleChromeManagementVersionsV1DeviceInfo Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chromemanagement.v1.model;
/**
* Information of a device that runs a Chrome browser profile.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Chrome Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleChromeManagementVersionsV1DeviceInfo extends com.google.api.client.json.GenericJson {
/**
* Output only. Device ID that identifies the affiliated device on which the profile exists. If
* the device type is CHROME_BROWSER, then this represents a unique Directory API ID of the device
* that can be used in Admin SDK Browsers API.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String affiliatedDeviceId;
/**
* Output only. Type of the device on which the profile exists.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deviceType;
/**
* Output only. Hostname of the device on which the profile exists.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hostname;
/**
* Output only. Machine name of the device on which the profile exists. On platforms which do not
* report the machine name (currently iOS and Android) this is instead set to the browser's
* device_id - but note that this is a different device_id than the |affiliated_device_id|.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String machine;
/**
* Output only. Device ID that identifies the affiliated device on which the profile exists. If
* the device type is CHROME_BROWSER, then this represents a unique Directory API ID of the device
* that can be used in Admin SDK Browsers API.
* @return value or {@code null} for none
*/
public java.lang.String getAffiliatedDeviceId() {
return affiliatedDeviceId;
}
/**
* Output only. Device ID that identifies the affiliated device on which the profile exists. If
* the device type is CHROME_BROWSER, then this represents a unique Directory API ID of the device
* that can be used in Admin SDK Browsers API.
* @param affiliatedDeviceId affiliatedDeviceId or {@code null} for none
*/
public GoogleChromeManagementVersionsV1DeviceInfo setAffiliatedDeviceId(java.lang.String affiliatedDeviceId) {
this.affiliatedDeviceId = affiliatedDeviceId;
return this;
}
/**
* Output only. Type of the device on which the profile exists.
* @return value or {@code null} for none
*/
public java.lang.String getDeviceType() {
return deviceType;
}
/**
* Output only. Type of the device on which the profile exists.
* @param deviceType deviceType or {@code null} for none
*/
public GoogleChromeManagementVersionsV1DeviceInfo setDeviceType(java.lang.String deviceType) {
this.deviceType = deviceType;
return this;
}
/**
* Output only. Hostname of the device on which the profile exists.
* @return value or {@code null} for none
*/
public java.lang.String getHostname() {
return hostname;
}
/**
* Output only. Hostname of the device on which the profile exists.
* @param hostname hostname or {@code null} for none
*/
public GoogleChromeManagementVersionsV1DeviceInfo setHostname(java.lang.String hostname) {
this.hostname = hostname;
return this;
}
/**
* Output only. Machine name of the device on which the profile exists. On platforms which do not
* report the machine name (currently iOS and Android) this is instead set to the browser's
* device_id - but note that this is a different device_id than the |affiliated_device_id|.
* @return value or {@code null} for none
*/
public java.lang.String getMachine() {
return machine;
}
/**
* Output only. Machine name of the device on which the profile exists. On platforms which do not
* report the machine name (currently iOS and Android) this is instead set to the browser's
* device_id - but note that this is a different device_id than the |affiliated_device_id|.
* @param machine machine or {@code null} for none
*/
public GoogleChromeManagementVersionsV1DeviceInfo setMachine(java.lang.String machine) {
this.machine = machine;
return this;
}
@Override
public GoogleChromeManagementVersionsV1DeviceInfo set(String fieldName, Object value) {
return (GoogleChromeManagementVersionsV1DeviceInfo) super.set(fieldName, value);
}
@Override
public GoogleChromeManagementVersionsV1DeviceInfo clone() {
return (GoogleChromeManagementVersionsV1DeviceInfo) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy