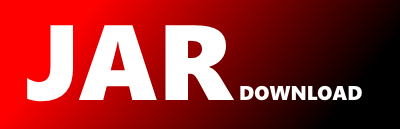
com.google.api.services.chromemanagement.v1.model.GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.chromemanagement.v1.model;
/**
* A certificate provisioning process.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Chrome Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess extends com.google.api.client.json.GenericJson {
/**
* Output only. A JSON string that contains the administrator-provided configuration for the
* certification authority service. This field can be missing if no configuration was given.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String caConnectionAdapterConfigReference;
/**
* Output only. The client certificate is being provisioned for a ChromeOS device. This contains
* information about the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementVersionsV1alpha1ChromeOsDevice chromeOsDevice;
/**
* Output only. The client certificate is being provisioned for a ChromeOS user session. This
* contains information about the user session.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleChromeManagementVersionsV1alpha1ChromeOsUserSession chromeOsUserSession;
/**
* Output only. A message describing why this `CertificateProvisioningProcess` failed. Presence of
* this field indicates that the `CertificateProvisioningProcess` has failed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String failureMessage;
/**
* Output only. The issued certificate for this `CertificateProvisioningProcess` in PEM format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String issuedCertificate;
/**
* Identifier. Resource name of the `CertificateProvisioningProcess`. The name pattern is given as
* `customers/{customer}/certificateProvisioningProcesses/{certificate_provisioning_process}` with
* `{customer}` being the obfuscated customer id and `{certificate_provisioning_process}` being
* the certificate provisioning process id.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. A JSON string that contains the administrator-provided configuration for the
* certificate provisioning profile. This field can be missing if no configuration was given.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String profileAdapterConfigReference;
/**
* Output only. The ID of the certificate provisioning profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String provisioningProfileId;
/**
* Output only. The data that the client was asked to sign. This field is only present after the
* `SignData` operation has been initiated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String signData;
/**
* Output only. The signature of `signature_algorithm`, generated using the client's private key
* using `signature_algorithm`. This field is only present after the`SignData` operation has
* finished.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String signature;
/**
* Output only. The signature algorithm that the adapter expects the client and backend components
* to use when processing `sign_data`. This field is only present after the `SignData` operation
* has been initiated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String signatureAlgorithm;
/**
* Output only. Server-generated timestamp of when the certificate provisioning process has been
* created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* Output only. The public key for which a certificate should be provisioned. Represented as a
* DER-encoded X.509 SubjectPublicKeyInfo.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String subjectPublicKeyInfo;
/**
* Output only. A JSON string that contains the administrator-provided configuration for the
* certification authority service. This field can be missing if no configuration was given.
* @return value or {@code null} for none
*/
public java.lang.String getCaConnectionAdapterConfigReference() {
return caConnectionAdapterConfigReference;
}
/**
* Output only. A JSON string that contains the administrator-provided configuration for the
* certification authority service. This field can be missing if no configuration was given.
* @param caConnectionAdapterConfigReference caConnectionAdapterConfigReference or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setCaConnectionAdapterConfigReference(java.lang.String caConnectionAdapterConfigReference) {
this.caConnectionAdapterConfigReference = caConnectionAdapterConfigReference;
return this;
}
/**
* Output only. The client certificate is being provisioned for a ChromeOS device. This contains
* information about the device.
* @return value or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1ChromeOsDevice getChromeOsDevice() {
return chromeOsDevice;
}
/**
* Output only. The client certificate is being provisioned for a ChromeOS device. This contains
* information about the device.
* @param chromeOsDevice chromeOsDevice or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setChromeOsDevice(GoogleChromeManagementVersionsV1alpha1ChromeOsDevice chromeOsDevice) {
this.chromeOsDevice = chromeOsDevice;
return this;
}
/**
* Output only. The client certificate is being provisioned for a ChromeOS user session. This
* contains information about the user session.
* @return value or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1ChromeOsUserSession getChromeOsUserSession() {
return chromeOsUserSession;
}
/**
* Output only. The client certificate is being provisioned for a ChromeOS user session. This
* contains information about the user session.
* @param chromeOsUserSession chromeOsUserSession or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setChromeOsUserSession(GoogleChromeManagementVersionsV1alpha1ChromeOsUserSession chromeOsUserSession) {
this.chromeOsUserSession = chromeOsUserSession;
return this;
}
/**
* Output only. A message describing why this `CertificateProvisioningProcess` failed. Presence of
* this field indicates that the `CertificateProvisioningProcess` has failed.
* @return value or {@code null} for none
*/
public java.lang.String getFailureMessage() {
return failureMessage;
}
/**
* Output only. A message describing why this `CertificateProvisioningProcess` failed. Presence of
* this field indicates that the `CertificateProvisioningProcess` has failed.
* @param failureMessage failureMessage or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setFailureMessage(java.lang.String failureMessage) {
this.failureMessage = failureMessage;
return this;
}
/**
* Output only. The issued certificate for this `CertificateProvisioningProcess` in PEM format.
* @return value or {@code null} for none
*/
public java.lang.String getIssuedCertificate() {
return issuedCertificate;
}
/**
* Output only. The issued certificate for this `CertificateProvisioningProcess` in PEM format.
* @param issuedCertificate issuedCertificate or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setIssuedCertificate(java.lang.String issuedCertificate) {
this.issuedCertificate = issuedCertificate;
return this;
}
/**
* Identifier. Resource name of the `CertificateProvisioningProcess`. The name pattern is given as
* `customers/{customer}/certificateProvisioningProcesses/{certificate_provisioning_process}` with
* `{customer}` being the obfuscated customer id and `{certificate_provisioning_process}` being
* the certificate provisioning process id.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Resource name of the `CertificateProvisioningProcess`. The name pattern is given as
* `customers/{customer}/certificateProvisioningProcesses/{certificate_provisioning_process}` with
* `{customer}` being the obfuscated customer id and `{certificate_provisioning_process}` being
* the certificate provisioning process id.
* @param name name or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. A JSON string that contains the administrator-provided configuration for the
* certificate provisioning profile. This field can be missing if no configuration was given.
* @return value or {@code null} for none
*/
public java.lang.String getProfileAdapterConfigReference() {
return profileAdapterConfigReference;
}
/**
* Output only. A JSON string that contains the administrator-provided configuration for the
* certificate provisioning profile. This field can be missing if no configuration was given.
* @param profileAdapterConfigReference profileAdapterConfigReference or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setProfileAdapterConfigReference(java.lang.String profileAdapterConfigReference) {
this.profileAdapterConfigReference = profileAdapterConfigReference;
return this;
}
/**
* Output only. The ID of the certificate provisioning profile.
* @return value or {@code null} for none
*/
public java.lang.String getProvisioningProfileId() {
return provisioningProfileId;
}
/**
* Output only. The ID of the certificate provisioning profile.
* @param provisioningProfileId provisioningProfileId or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setProvisioningProfileId(java.lang.String provisioningProfileId) {
this.provisioningProfileId = provisioningProfileId;
return this;
}
/**
* Output only. The data that the client was asked to sign. This field is only present after the
* `SignData` operation has been initiated.
* @see #decodeSignData()
* @return value or {@code null} for none
*/
public java.lang.String getSignData() {
return signData;
}
/**
* Output only. The data that the client was asked to sign. This field is only present after the
* `SignData` operation has been initiated.
* @see #getSignData()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeSignData() {
return com.google.api.client.util.Base64.decodeBase64(signData);
}
/**
* Output only. The data that the client was asked to sign. This field is only present after the
* `SignData` operation has been initiated.
* @see #encodeSignData()
* @param signData signData or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setSignData(java.lang.String signData) {
this.signData = signData;
return this;
}
/**
* Output only. The data that the client was asked to sign. This field is only present after the
* `SignData` operation has been initiated.
* @see #setSignData()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess encodeSignData(byte[] signData) {
this.signData = com.google.api.client.util.Base64.encodeBase64URLSafeString(signData);
return this;
}
/**
* Output only. The signature of `signature_algorithm`, generated using the client's private key
* using `signature_algorithm`. This field is only present after the`SignData` operation has
* finished.
* @see #decodeSignature()
* @return value or {@code null} for none
*/
public java.lang.String getSignature() {
return signature;
}
/**
* Output only. The signature of `signature_algorithm`, generated using the client's private key
* using `signature_algorithm`. This field is only present after the`SignData` operation has
* finished.
* @see #getSignature()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeSignature() {
return com.google.api.client.util.Base64.decodeBase64(signature);
}
/**
* Output only. The signature of `signature_algorithm`, generated using the client's private key
* using `signature_algorithm`. This field is only present after the`SignData` operation has
* finished.
* @see #encodeSignature()
* @param signature signature or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setSignature(java.lang.String signature) {
this.signature = signature;
return this;
}
/**
* Output only. The signature of `signature_algorithm`, generated using the client's private key
* using `signature_algorithm`. This field is only present after the`SignData` operation has
* finished.
* @see #setSignature()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess encodeSignature(byte[] signature) {
this.signature = com.google.api.client.util.Base64.encodeBase64URLSafeString(signature);
return this;
}
/**
* Output only. The signature algorithm that the adapter expects the client and backend components
* to use when processing `sign_data`. This field is only present after the `SignData` operation
* has been initiated.
* @return value or {@code null} for none
*/
public java.lang.String getSignatureAlgorithm() {
return signatureAlgorithm;
}
/**
* Output only. The signature algorithm that the adapter expects the client and backend components
* to use when processing `sign_data`. This field is only present after the `SignData` operation
* has been initiated.
* @param signatureAlgorithm signatureAlgorithm or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setSignatureAlgorithm(java.lang.String signatureAlgorithm) {
this.signatureAlgorithm = signatureAlgorithm;
return this;
}
/**
* Output only. Server-generated timestamp of when the certificate provisioning process has been
* created.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* Output only. Server-generated timestamp of when the certificate provisioning process has been
* created.
* @param startTime startTime or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* Output only. The public key for which a certificate should be provisioned. Represented as a
* DER-encoded X.509 SubjectPublicKeyInfo.
* @see #decodeSubjectPublicKeyInfo()
* @return value or {@code null} for none
*/
public java.lang.String getSubjectPublicKeyInfo() {
return subjectPublicKeyInfo;
}
/**
* Output only. The public key for which a certificate should be provisioned. Represented as a
* DER-encoded X.509 SubjectPublicKeyInfo.
* @see #getSubjectPublicKeyInfo()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeSubjectPublicKeyInfo() {
return com.google.api.client.util.Base64.decodeBase64(subjectPublicKeyInfo);
}
/**
* Output only. The public key for which a certificate should be provisioned. Represented as a
* DER-encoded X.509 SubjectPublicKeyInfo.
* @see #encodeSubjectPublicKeyInfo()
* @param subjectPublicKeyInfo subjectPublicKeyInfo or {@code null} for none
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess setSubjectPublicKeyInfo(java.lang.String subjectPublicKeyInfo) {
this.subjectPublicKeyInfo = subjectPublicKeyInfo;
return this;
}
/**
* Output only. The public key for which a certificate should be provisioned. Represented as a
* DER-encoded X.509 SubjectPublicKeyInfo.
* @see #setSubjectPublicKeyInfo()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess encodeSubjectPublicKeyInfo(byte[] subjectPublicKeyInfo) {
this.subjectPublicKeyInfo = com.google.api.client.util.Base64.encodeBase64URLSafeString(subjectPublicKeyInfo);
return this;
}
@Override
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess set(String fieldName, Object value) {
return (GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess) super.set(fieldName, value);
}
@Override
public GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess clone() {
return (GoogleChromeManagementVersionsV1alpha1CertificateProvisioningProcess) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy