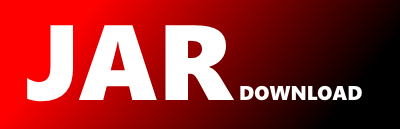
com.google.api.services.civicinfo.CivicInfo Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-10-18 at 16:55:10 UTC
* Modify at your own risk.
*/
package com.google.api.services.civicinfo;
/**
* Service definition for CivicInfo (v2).
*
*
* Provides polling places, early vote locations, contest data, election officials, and government representatives for U.S. residential addresses.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CivicInfoRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CivicInfo extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Google Civic Information API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "civicinfo/v2/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CivicInfo(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CivicInfo(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Divisions collection.
*
* The typical use is:
*
* {@code CivicInfo civicinfo = new CivicInfo(...);}
* {@code CivicInfo.Divisions.List request = civicinfo.divisions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Divisions divisions() {
return new Divisions();
}
/**
* The "divisions" collection of methods.
*/
public class Divisions {
/**
* Searches for political divisions by their natural name or OCD ID.
*
* Create a request for the method "divisions.search".
*
* This request holds the parameters needed by the civicinfo server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Search search() throws java.io.IOException {
Search result = new Search();
initialize(result);
return result;
}
public class Search extends CivicInfoRequest {
private static final String REST_PATH = "divisions";
/**
* Searches for political divisions by their natural name or OCD ID.
*
* Create a request for the method "divisions.search".
*
* This request holds the parameters needed by the the civicinfo server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Search() {
super(CivicInfo.this, "GET", REST_PATH, null, com.google.api.services.civicinfo.model.DivisionSearchResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUserIp(java.lang.String userIp) {
return (Search) super.setUserIp(userIp);
}
/**
* The search query. Queries can cover any parts of a OCD ID or a human readable division
* name. All words given in the query are treated as required patterns. In addition to that,
* most query operators of the Apache Lucene library are supported. See
* http://lucene.apache.org/core/2_9_4/queryparsersyntax.html
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** The search query. Queries can cover any parts of a OCD ID or a human readable division name. All
words given in the query are treated as required patterns. In addition to that, most query
operators of the Apache Lucene library are supported. See
http://lucene.apache.org/core/2_9_4/queryparsersyntax.html
*/
public java.lang.String getQuery() {
return query;
}
/**
* The search query. Queries can cover any parts of a OCD ID or a human readable division
* name. All words given in the query are treated as required patterns. In addition to that,
* most query operators of the Apache Lucene library are supported. See
* http://lucene.apache.org/core/2_9_4/queryparsersyntax.html
*/
public Search setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Elections collection.
*
* The typical use is:
*
* {@code CivicInfo civicinfo = new CivicInfo(...);}
* {@code CivicInfo.Elections.List request = civicinfo.elections().list(parameters ...)}
*
*
* @return the resource collection
*/
public Elections elections() {
return new Elections();
}
/**
* The "elections" collection of methods.
*/
public class Elections {
/**
* List of available elections to query.
*
* Create a request for the method "elections.electionQuery".
*
* This request holds the parameters needed by the civicinfo server. After setting any optional
* parameters, call the {@link ElectionQuery#execute()} method to invoke the remote operation.
*
* @return the request
*/
public ElectionQuery electionQuery() throws java.io.IOException {
ElectionQuery result = new ElectionQuery();
initialize(result);
return result;
}
public class ElectionQuery extends CivicInfoRequest {
private static final String REST_PATH = "elections";
/**
* List of available elections to query.
*
* Create a request for the method "elections.electionQuery".
*
* This request holds the parameters needed by the the civicinfo server. After setting any
* optional parameters, call the {@link ElectionQuery#execute()} method to invoke the remote
* operation. {@link ElectionQuery#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @since 1.13
*/
protected ElectionQuery() {
super(CivicInfo.this, "GET", REST_PATH, null, com.google.api.services.civicinfo.model.ElectionsQueryResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ElectionQuery setAlt(java.lang.String alt) {
return (ElectionQuery) super.setAlt(alt);
}
@Override
public ElectionQuery setFields(java.lang.String fields) {
return (ElectionQuery) super.setFields(fields);
}
@Override
public ElectionQuery setKey(java.lang.String key) {
return (ElectionQuery) super.setKey(key);
}
@Override
public ElectionQuery setOauthToken(java.lang.String oauthToken) {
return (ElectionQuery) super.setOauthToken(oauthToken);
}
@Override
public ElectionQuery setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ElectionQuery) super.setPrettyPrint(prettyPrint);
}
@Override
public ElectionQuery setQuotaUser(java.lang.String quotaUser) {
return (ElectionQuery) super.setQuotaUser(quotaUser);
}
@Override
public ElectionQuery setUserIp(java.lang.String userIp) {
return (ElectionQuery) super.setUserIp(userIp);
}
@Override
public ElectionQuery set(String parameterName, Object value) {
return (ElectionQuery) super.set(parameterName, value);
}
}
/**
* Looks up information relevant to a voter based on the voter's registered address.
*
* Create a request for the method "elections.voterInfoQuery".
*
* This request holds the parameters needed by the civicinfo server. After setting any optional
* parameters, call the {@link VoterInfoQuery#execute()} method to invoke the remote operation.
*
* @param address The registered address of the voter to look up.
* @return the request
*/
public VoterInfoQuery voterInfoQuery(java.lang.String address) throws java.io.IOException {
VoterInfoQuery result = new VoterInfoQuery(address);
initialize(result);
return result;
}
public class VoterInfoQuery extends CivicInfoRequest {
private static final String REST_PATH = "voterinfo";
/**
* Looks up information relevant to a voter based on the voter's registered address.
*
* Create a request for the method "elections.voterInfoQuery".
*
* This request holds the parameters needed by the the civicinfo server. After setting any
* optional parameters, call the {@link VoterInfoQuery#execute()} method to invoke the remote
* operation. {@link VoterInfoQuery#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param address The registered address of the voter to look up.
* @since 1.13
*/
protected VoterInfoQuery(java.lang.String address) {
super(CivicInfo.this, "GET", REST_PATH, null, com.google.api.services.civicinfo.model.VoterInfoResponse.class);
this.address = com.google.api.client.util.Preconditions.checkNotNull(address, "Required parameter address must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public VoterInfoQuery setAlt(java.lang.String alt) {
return (VoterInfoQuery) super.setAlt(alt);
}
@Override
public VoterInfoQuery setFields(java.lang.String fields) {
return (VoterInfoQuery) super.setFields(fields);
}
@Override
public VoterInfoQuery setKey(java.lang.String key) {
return (VoterInfoQuery) super.setKey(key);
}
@Override
public VoterInfoQuery setOauthToken(java.lang.String oauthToken) {
return (VoterInfoQuery) super.setOauthToken(oauthToken);
}
@Override
public VoterInfoQuery setPrettyPrint(java.lang.Boolean prettyPrint) {
return (VoterInfoQuery) super.setPrettyPrint(prettyPrint);
}
@Override
public VoterInfoQuery setQuotaUser(java.lang.String quotaUser) {
return (VoterInfoQuery) super.setQuotaUser(quotaUser);
}
@Override
public VoterInfoQuery setUserIp(java.lang.String userIp) {
return (VoterInfoQuery) super.setUserIp(userIp);
}
/** The registered address of the voter to look up. */
@com.google.api.client.util.Key
private java.lang.String address;
/** The registered address of the voter to look up.
*/
public java.lang.String getAddress() {
return address;
}
/** The registered address of the voter to look up. */
public VoterInfoQuery setAddress(java.lang.String address) {
this.address = address;
return this;
}
/**
* The unique ID of the election to look up. A list of election IDs can be obtained at
* https://www.googleapis.com/civicinfo/{version}/elections
*/
@com.google.api.client.util.Key
private java.lang.Long electionId;
/** The unique ID of the election to look up. A list of election IDs can be obtained at
https://www.googleapis.com/civicinfo/{version}/elections [default: 0]
*/
public java.lang.Long getElectionId() {
return electionId;
}
/**
* The unique ID of the election to look up. A list of election IDs can be obtained at
* https://www.googleapis.com/civicinfo/{version}/elections
*/
public VoterInfoQuery setElectionId(java.lang.Long electionId) {
this.electionId = electionId;
return this;
}
/** If set to true, only data from official state sources will be returned. */
@com.google.api.client.util.Key
private java.lang.Boolean officialOnly;
/** If set to true, only data from official state sources will be returned. [default: false]
*/
public java.lang.Boolean getOfficialOnly() {
return officialOnly;
}
/** If set to true, only data from official state sources will be returned. */
public VoterInfoQuery setOfficialOnly(java.lang.Boolean officialOnly) {
this.officialOnly = officialOnly;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* If set to true, only data from official state sources will be returned.
*
*/
public boolean isOfficialOnly() {
if (officialOnly == null || officialOnly == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return officialOnly;
}
/**
* If set to true, the query will return the success codeand include any partial information
* when it is unable to determine a matching address or unable to determine the election for
* electionId=0 queries.
*/
@com.google.api.client.util.Key
private java.lang.Boolean returnAllAvailableData;
/** If set to true, the query will return the success codeand include any partial information when it
is unable to determine a matching address or unable to determine the election for electionId=0
queries. [default: false]
*/
public java.lang.Boolean getReturnAllAvailableData() {
return returnAllAvailableData;
}
/**
* If set to true, the query will return the success codeand include any partial information
* when it is unable to determine a matching address or unable to determine the election for
* electionId=0 queries.
*/
public VoterInfoQuery setReturnAllAvailableData(java.lang.Boolean returnAllAvailableData) {
this.returnAllAvailableData = returnAllAvailableData;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* If set to true, the query will return the success codeand include any partial information when it
is unable to determine a matching address or unable to determine the election for electionId=0
queries.
*
*/
public boolean isReturnAllAvailableData() {
if (returnAllAvailableData == null || returnAllAvailableData == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return returnAllAvailableData;
}
@Override
public VoterInfoQuery set(String parameterName, Object value) {
return (VoterInfoQuery) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Representatives collection.
*
* The typical use is:
*
* {@code CivicInfo civicinfo = new CivicInfo(...);}
* {@code CivicInfo.Representatives.List request = civicinfo.representatives().list(parameters ...)}
*
*
* @return the resource collection
*/
public Representatives representatives() {
return new Representatives();
}
/**
* The "representatives" collection of methods.
*/
public class Representatives {
/**
* Looks up political geography and representative information for a single address.
*
* Create a request for the method "representatives.representativeInfoByAddress".
*
* This request holds the parameters needed by the civicinfo server. After setting any optional
* parameters, call the {@link RepresentativeInfoByAddress#execute()} method to invoke the remote
* operation.
*
* @return the request
*/
public RepresentativeInfoByAddress representativeInfoByAddress() throws java.io.IOException {
RepresentativeInfoByAddress result = new RepresentativeInfoByAddress();
initialize(result);
return result;
}
public class RepresentativeInfoByAddress extends CivicInfoRequest {
private static final String REST_PATH = "representatives";
/**
* Looks up political geography and representative information for a single address.
*
* Create a request for the method "representatives.representativeInfoByAddress".
*
* This request holds the parameters needed by the the civicinfo server. After setting any
* optional parameters, call the {@link RepresentativeInfoByAddress#execute()} method to invoke
* the remote operation. {@link RepresentativeInfoByAddress#initialize(com.google.api.client.g
* oogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @since 1.13
*/
protected RepresentativeInfoByAddress() {
super(CivicInfo.this, "GET", REST_PATH, null, com.google.api.services.civicinfo.model.RepresentativeInfoResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public RepresentativeInfoByAddress setAlt(java.lang.String alt) {
return (RepresentativeInfoByAddress) super.setAlt(alt);
}
@Override
public RepresentativeInfoByAddress setFields(java.lang.String fields) {
return (RepresentativeInfoByAddress) super.setFields(fields);
}
@Override
public RepresentativeInfoByAddress setKey(java.lang.String key) {
return (RepresentativeInfoByAddress) super.setKey(key);
}
@Override
public RepresentativeInfoByAddress setOauthToken(java.lang.String oauthToken) {
return (RepresentativeInfoByAddress) super.setOauthToken(oauthToken);
}
@Override
public RepresentativeInfoByAddress setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RepresentativeInfoByAddress) super.setPrettyPrint(prettyPrint);
}
@Override
public RepresentativeInfoByAddress setQuotaUser(java.lang.String quotaUser) {
return (RepresentativeInfoByAddress) super.setQuotaUser(quotaUser);
}
@Override
public RepresentativeInfoByAddress setUserIp(java.lang.String userIp) {
return (RepresentativeInfoByAddress) super.setUserIp(userIp);
}
/**
* The address to look up. May only be specified if the field ocdId is not given in the URL.
*/
@com.google.api.client.util.Key
private java.lang.String address;
/** The address to look up. May only be specified if the field ocdId is not given in the URL.
*/
public java.lang.String getAddress() {
return address;
}
/**
* The address to look up. May only be specified if the field ocdId is not given in the URL.
*/
public RepresentativeInfoByAddress setAddress(java.lang.String address) {
this.address = address;
return this;
}
/**
* Whether to return information about offices and officials. If false, only the top-level
* district information will be returned.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeOffices;
/** Whether to return information about offices and officials. If false, only the top-level district
information will be returned. [default: true]
*/
public java.lang.Boolean getIncludeOffices() {
return includeOffices;
}
/**
* Whether to return information about offices and officials. If false, only the top-level
* district information will be returned.
*/
public RepresentativeInfoByAddress setIncludeOffices(java.lang.Boolean includeOffices) {
this.includeOffices = includeOffices;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Whether to return information about offices and officials. If false, only the top-level district
information will be returned.
*
*/
public boolean isIncludeOffices() {
if (includeOffices == null || includeOffices == com.google.api.client.util.Data.NULL_BOOLEAN) {
return true;
}
return includeOffices;
}
/**
* A list of office levels to filter by. Only offices that serve at least one of these levels
* will be returned. Divisions that don't contain a matching office will not be returned.
*/
@com.google.api.client.util.Key
private java.util.List levels;
/** A list of office levels to filter by. Only offices that serve at least one of these levels will be
returned. Divisions that don't contain a matching office will not be returned.
*/
public java.util.List getLevels() {
return levels;
}
/**
* A list of office levels to filter by. Only offices that serve at least one of these levels
* will be returned. Divisions that don't contain a matching office will not be returned.
*/
public RepresentativeInfoByAddress setLevels(java.util.List levels) {
this.levels = levels;
return this;
}
/**
* A list of office roles to filter by. Only offices fulfilling one of these roles will be
* returned. Divisions that don't contain a matching office will not be returned.
*/
@com.google.api.client.util.Key
private java.util.List roles;
/** A list of office roles to filter by. Only offices fulfilling one of these roles will be returned.
Divisions that don't contain a matching office will not be returned.
*/
public java.util.List getRoles() {
return roles;
}
/**
* A list of office roles to filter by. Only offices fulfilling one of these roles will be
* returned. Divisions that don't contain a matching office will not be returned.
*/
public RepresentativeInfoByAddress setRoles(java.util.List roles) {
this.roles = roles;
return this;
}
@Override
public RepresentativeInfoByAddress set(String parameterName, Object value) {
return (RepresentativeInfoByAddress) super.set(parameterName, value);
}
}
/**
* Looks up representative information for a single geographic division.
*
* Create a request for the method "representatives.representativeInfoByDivision".
*
* This request holds the parameters needed by the civicinfo server. After setting any optional
* parameters, call the {@link RepresentativeInfoByDivision#execute()} method to invoke the remote
* operation.
*
* @param ocdId The Open Civic Data division identifier of the division to look up.
* @return the request
*/
public RepresentativeInfoByDivision representativeInfoByDivision(java.lang.String ocdId) throws java.io.IOException {
RepresentativeInfoByDivision result = new RepresentativeInfoByDivision(ocdId);
initialize(result);
return result;
}
public class RepresentativeInfoByDivision extends CivicInfoRequest {
private static final String REST_PATH = "representatives/{ocdId}";
/**
* Looks up representative information for a single geographic division.
*
* Create a request for the method "representatives.representativeInfoByDivision".
*
* This request holds the parameters needed by the the civicinfo server. After setting any
* optional parameters, call the {@link RepresentativeInfoByDivision#execute()} method to invoke
* the remote operation. {@link RepresentativeInfoByDivision#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param ocdId The Open Civic Data division identifier of the division to look up.
* @since 1.13
*/
protected RepresentativeInfoByDivision(java.lang.String ocdId) {
super(CivicInfo.this, "GET", REST_PATH, null, com.google.api.services.civicinfo.model.RepresentativeInfoData.class);
this.ocdId = com.google.api.client.util.Preconditions.checkNotNull(ocdId, "Required parameter ocdId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public RepresentativeInfoByDivision setAlt(java.lang.String alt) {
return (RepresentativeInfoByDivision) super.setAlt(alt);
}
@Override
public RepresentativeInfoByDivision setFields(java.lang.String fields) {
return (RepresentativeInfoByDivision) super.setFields(fields);
}
@Override
public RepresentativeInfoByDivision setKey(java.lang.String key) {
return (RepresentativeInfoByDivision) super.setKey(key);
}
@Override
public RepresentativeInfoByDivision setOauthToken(java.lang.String oauthToken) {
return (RepresentativeInfoByDivision) super.setOauthToken(oauthToken);
}
@Override
public RepresentativeInfoByDivision setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RepresentativeInfoByDivision) super.setPrettyPrint(prettyPrint);
}
@Override
public RepresentativeInfoByDivision setQuotaUser(java.lang.String quotaUser) {
return (RepresentativeInfoByDivision) super.setQuotaUser(quotaUser);
}
@Override
public RepresentativeInfoByDivision setUserIp(java.lang.String userIp) {
return (RepresentativeInfoByDivision) super.setUserIp(userIp);
}
/** The Open Civic Data division identifier of the division to look up. */
@com.google.api.client.util.Key
private java.lang.String ocdId;
/** The Open Civic Data division identifier of the division to look up.
*/
public java.lang.String getOcdId() {
return ocdId;
}
/** The Open Civic Data division identifier of the division to look up. */
public RepresentativeInfoByDivision setOcdId(java.lang.String ocdId) {
this.ocdId = ocdId;
return this;
}
/**
* A list of office levels to filter by. Only offices that serve at least one of these levels
* will be returned. Divisions that don't contain a matching office will not be returned.
*/
@com.google.api.client.util.Key
private java.util.List levels;
/** A list of office levels to filter by. Only offices that serve at least one of these levels will be
returned. Divisions that don't contain a matching office will not be returned.
*/
public java.util.List getLevels() {
return levels;
}
/**
* A list of office levels to filter by. Only offices that serve at least one of these levels
* will be returned. Divisions that don't contain a matching office will not be returned.
*/
public RepresentativeInfoByDivision setLevels(java.util.List levels) {
this.levels = levels;
return this;
}
/**
* If true, information about all divisions contained in the division requested will be
* included as well. For example, if querying ocd-division/country:us/district:dc, this would
* also return all DC's wards and ANCs.
*/
@com.google.api.client.util.Key
private java.lang.Boolean recursive;
/** If true, information about all divisions contained in the division requested will be included as
well. For example, if querying ocd-division/country:us/district:dc, this would also return all DC's
wards and ANCs.
*/
public java.lang.Boolean getRecursive() {
return recursive;
}
/**
* If true, information about all divisions contained in the division requested will be
* included as well. For example, if querying ocd-division/country:us/district:dc, this would
* also return all DC's wards and ANCs.
*/
public RepresentativeInfoByDivision setRecursive(java.lang.Boolean recursive) {
this.recursive = recursive;
return this;
}
/**
* A list of office roles to filter by. Only offices fulfilling one of these roles will be
* returned. Divisions that don't contain a matching office will not be returned.
*/
@com.google.api.client.util.Key
private java.util.List roles;
/** A list of office roles to filter by. Only offices fulfilling one of these roles will be returned.
Divisions that don't contain a matching office will not be returned.
*/
public java.util.List getRoles() {
return roles;
}
/**
* A list of office roles to filter by. Only offices fulfilling one of these roles will be
* returned. Divisions that don't contain a matching office will not be returned.
*/
public RepresentativeInfoByDivision setRoles(java.util.List roles) {
this.roles = roles;
return this;
}
@Override
public RepresentativeInfoByDivision set(String parameterName, Object value) {
return (RepresentativeInfoByDivision) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link CivicInfo}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link CivicInfo}. */
@Override
public CivicInfo build() {
return new CivicInfo(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CivicInfoRequestInitializer}.
*
* @since 1.12
*/
public Builder setCivicInfoRequestInitializer(
CivicInfoRequestInitializer civicinfoRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(civicinfoRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}