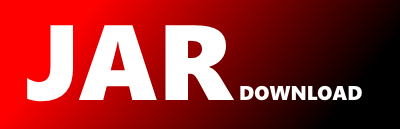
com.google.api.services.cloudasset.v1.CloudAsset Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudasset.v1;
/**
* Service definition for CloudAsset (v1).
*
*
* The Cloud Asset API manages the history and inventory of Google Cloud resources.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudAssetRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudAsset extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Asset API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://cloudasset.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://cloudasset.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudAsset(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudAsset(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Assets collection.
*
* The typical use is:
*
* {@code CloudAsset cloudasset = new CloudAsset(...);}
* {@code CloudAsset.Assets.List request = cloudasset.assets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Assets assets() {
return new Assets();
}
/**
* The "assets" collection of methods.
*/
public class Assets {
/**
* Lists assets with time and resource types and returns paged results in response.
*
* Create a request for the method "assets.list".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the organization, folder, or project the assets belong to. Format:
* "organizations/[organization-number]" (such as "organizations/123"), "projects/[project-
* id]" (such as "projects/my-project-id"), "projects/[project-number]" (such as
* "projects/12345"), or "folders/[folder-number]" (such as "folders/12345").
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}/assets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Lists assets with time and resource types and returns paged results in response.
*
* Create a request for the method "assets.list".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the organization, folder, or project the assets belong to. Format:
* "organizations/[organization-number]" (such as "organizations/123"), "projects/[project-
* id]" (such as "projects/my-project-id"), "projects/[project-number]" (such as
* "projects/12345"), or "folders/[folder-number]" (such as "folders/12345").
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.ListAssetsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the organization, folder, or project the assets belong to. Format:
* "organizations/[organization-number]" (such as "organizations/123"), "projects/[project-
* id]" (such as "projects/my-project-id"), "projects/[project-number]" (such as
* "projects/12345"), or "folders/[folder-number]" (such as "folders/12345").
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the organization, folder, or project the assets belong to. Format:
"organizations/[organization-number]" (such as "organizations/123"), "projects/[project-id]" (such
as "projects/my-project-id"), "projects/[project-number]" (such as "projects/12345"), or
"folders/[folder-number]" (such as "folders/12345").
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the organization, folder, or project the assets belong to. Format:
* "organizations/[organization-number]" (such as "organizations/123"), "projects/[project-
* id]" (such as "projects/my-project-id"), "projects/[project-number]" (such as
* "projects/12345"), or "folders/[folder-number]" (such as "folders/12345").
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A list of asset types to take a snapshot for. For example: "compute.googleapis.com/Disk".
* Regular expression is also supported. For example: * "compute.googleapis.com.*" snapshots
* resources whose asset type starts with "compute.googleapis.com". * ".*Instance" snapshots
* resources whose asset type ends with "Instance". * ".*Instance.*" snapshots resources whose
* asset type contains "Instance". See [RE2](https://github.com/google/re2/wiki/Syntax) for
* all supported regular expression syntax. If the regular expression does not match any
* supported asset type, an INVALID_ARGUMENT error will be returned. If specified, only
* matching assets will be returned, otherwise, it will snapshot all asset types. See
* [Introduction to Cloud Asset Inventory](https://cloud.google.com/asset-
* inventory/docs/overview) for all supported asset types.
*/
@com.google.api.client.util.Key
private java.util.List assetTypes;
/** A list of asset types to take a snapshot for. For example: "compute.googleapis.com/Disk". Regular
expression is also supported. For example: * "compute.googleapis.com.*" snapshots resources whose
asset type starts with "compute.googleapis.com". * ".*Instance" snapshots resources whose asset
type ends with "Instance". * ".*Instance.*" snapshots resources whose asset type contains
"Instance". See [RE2](https://github.com/google/re2/wiki/Syntax) for all supported regular
expression syntax. If the regular expression does not match any supported asset type, an
INVALID_ARGUMENT error will be returned. If specified, only matching assets will be returned,
otherwise, it will snapshot all asset types. See [Introduction to Cloud Asset
Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset types.
*/
public java.util.List getAssetTypes() {
return assetTypes;
}
/**
* A list of asset types to take a snapshot for. For example: "compute.googleapis.com/Disk".
* Regular expression is also supported. For example: * "compute.googleapis.com.*" snapshots
* resources whose asset type starts with "compute.googleapis.com". * ".*Instance" snapshots
* resources whose asset type ends with "Instance". * ".*Instance.*" snapshots resources whose
* asset type contains "Instance". See [RE2](https://github.com/google/re2/wiki/Syntax) for
* all supported regular expression syntax. If the regular expression does not match any
* supported asset type, an INVALID_ARGUMENT error will be returned. If specified, only
* matching assets will be returned, otherwise, it will snapshot all asset types. See
* [Introduction to Cloud Asset Inventory](https://cloud.google.com/asset-
* inventory/docs/overview) for all supported asset types.
*/
public List setAssetTypes(java.util.List assetTypes) {
this.assetTypes = assetTypes;
return this;
}
/** Asset content type. If not specified, no content but the asset name will be returned. */
@com.google.api.client.util.Key
private java.lang.String contentType;
/** Asset content type. If not specified, no content but the asset name will be returned.
*/
public java.lang.String getContentType() {
return contentType;
}
/** Asset content type. If not specified, no content but the asset name will be returned. */
public List setContentType(java.lang.String contentType) {
this.contentType = contentType;
return this;
}
/**
* The maximum number of assets to be returned in a single response. Default is 100, minimum
* is 1, and maximum is 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of assets to be returned in a single response. Default is 100, minimum is 1, and
maximum is 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of assets to be returned in a single response. Default is 100, minimum
* is 1, and maximum is 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The `next_page_token` returned from the previous `ListAssetsResponse`, or unspecified for
* the first `ListAssetsRequest`. It is a continuation of a prior `ListAssets` call, and the
* API should return the next page of assets.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` returned from the previous `ListAssetsResponse`, or unspecified for the first
`ListAssetsRequest`. It is a continuation of a prior `ListAssets` call, and the API should return
the next page of assets.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The `next_page_token` returned from the previous `ListAssetsResponse`, or unspecified for
* the first `ListAssetsRequest`. It is a continuation of a prior `ListAssets` call, and the
* API should return the next page of assets.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Timestamp to take an asset snapshot. This can only be set to a timestamp between the
* current time and the current time minus 35 days (inclusive). If not specified, the current
* time will be used. Due to delays in resource data collection and indexing, there is a
* volatile window during which running the same query may get different results.
*/
@com.google.api.client.util.Key
private String readTime;
/** Timestamp to take an asset snapshot. This can only be set to a timestamp between the current time
and the current time minus 35 days (inclusive). If not specified, the current time will be used.
Due to delays in resource data collection and indexing, there is a volatile window during which
running the same query may get different results.
*/
public String getReadTime() {
return readTime;
}
/**
* Timestamp to take an asset snapshot. This can only be set to a timestamp between the
* current time and the current time minus 35 days (inclusive). If not specified, the current
* time will be used. Due to delays in resource data collection and indexing, there is a
* volatile window during which running the same query may get different results.
*/
public List setReadTime(String readTime) {
this.readTime = readTime;
return this;
}
/**
* A list of relationship types to output, for example: `INSTANCE_TO_INSTANCEGROUP`. This
* field should only be specified if content_type=RELATIONSHIP. * If specified: it snapshots
* specified relationships. It returns an error if any of the [relationship_types] doesn't
* belong to the supported relationship types of the [asset_types] or if any of the
* [asset_types] doesn't belong to the source types of the [relationship_types]. * Otherwise:
* it snapshots the supported relationships for all [asset_types] or returns an error if any
* of the [asset_types] has no relationship support. An unspecified asset types field means
* all supported asset_types. See [Introduction to Cloud Asset
* Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset
* types and relationship types.
*/
@com.google.api.client.util.Key
private java.util.List relationshipTypes;
/** A list of relationship types to output, for example: `INSTANCE_TO_INSTANCEGROUP`. This field should
only be specified if content_type=RELATIONSHIP. * If specified: it snapshots specified
relationships. It returns an error if any of the [relationship_types] doesn't belong to the
supported relationship types of the [asset_types] or if any of the [asset_types] doesn't belong to
the source types of the [relationship_types]. * Otherwise: it snapshots the supported relationships
for all [asset_types] or returns an error if any of the [asset_types] has no relationship support.
An unspecified asset types field means all supported asset_types. See [Introduction to Cloud Asset
Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset types
and relationship types.
*/
public java.util.List getRelationshipTypes() {
return relationshipTypes;
}
/**
* A list of relationship types to output, for example: `INSTANCE_TO_INSTANCEGROUP`. This
* field should only be specified if content_type=RELATIONSHIP. * If specified: it snapshots
* specified relationships. It returns an error if any of the [relationship_types] doesn't
* belong to the supported relationship types of the [asset_types] or if any of the
* [asset_types] doesn't belong to the source types of the [relationship_types]. * Otherwise:
* it snapshots the supported relationships for all [asset_types] or returns an error if any
* of the [asset_types] has no relationship support. An unspecified asset types field means
* all supported asset_types. See [Introduction to Cloud Asset
* Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset
* types and relationship types.
*/
public List setRelationshipTypes(java.util.List relationshipTypes) {
this.relationshipTypes = relationshipTypes;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the EffectiveIamPolicies collection.
*
* The typical use is:
*
* {@code CloudAsset cloudasset = new CloudAsset(...);}
* {@code CloudAsset.EffectiveIamPolicies.List request = cloudasset.effectiveIamPolicies().list(parameters ...)}
*
*
* @return the resource collection
*/
public EffectiveIamPolicies effectiveIamPolicies() {
return new EffectiveIamPolicies();
}
/**
* The "effectiveIamPolicies" collection of methods.
*/
public class EffectiveIamPolicies {
/**
* Gets effective IAM policies for a batch of resources.
*
* Create a request for the method "effectiveIamPolicies.batchGet".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link BatchGet#execute()} method to invoke the remote operation.
*
* @param scope Required. Only IAM policies on or below the scope will be returned. This can only be an organization
* number (such as "organizations/123"), a folder number (such as "folders/123"), a project
* ID (such as "projects/my-project-id"), or a project number (such as "projects/12345"). To
* know how to get organization ID, visit [here ](https://cloud.google.com/resource-
* manager/docs/creating-managing-organization#retrieving_your_organization_id). To know how
* to get folder or project ID, visit [here ](https://cloud.google.com/resource-
* manager/docs/creating-managing-folders#viewing_or_listing_folders_and_projects).
* @return the request
*/
public BatchGet batchGet(java.lang.String scope) throws java.io.IOException {
BatchGet result = new BatchGet(scope);
initialize(result);
return result;
}
public class BatchGet extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}/effectiveIamPolicies:batchGet";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Gets effective IAM policies for a batch of resources.
*
* Create a request for the method "effectiveIamPolicies.batchGet".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link BatchGet#execute()} method to invoke the remote operation.
* {@link
* BatchGet#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param scope Required. Only IAM policies on or below the scope will be returned. This can only be an organization
* number (such as "organizations/123"), a folder number (such as "folders/123"), a project
* ID (such as "projects/my-project-id"), or a project number (such as "projects/12345"). To
* know how to get organization ID, visit [here ](https://cloud.google.com/resource-
* manager/docs/creating-managing-organization#retrieving_your_organization_id). To know how
* to get folder or project ID, visit [here ](https://cloud.google.com/resource-
* manager/docs/creating-managing-folders#viewing_or_listing_folders_and_projects).
* @since 1.13
*/
protected BatchGet(java.lang.String scope) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.BatchGetEffectiveIamPoliciesResponse.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public BatchGet set$Xgafv(java.lang.String $Xgafv) {
return (BatchGet) super.set$Xgafv($Xgafv);
}
@Override
public BatchGet setAccessToken(java.lang.String accessToken) {
return (BatchGet) super.setAccessToken(accessToken);
}
@Override
public BatchGet setAlt(java.lang.String alt) {
return (BatchGet) super.setAlt(alt);
}
@Override
public BatchGet setCallback(java.lang.String callback) {
return (BatchGet) super.setCallback(callback);
}
@Override
public BatchGet setFields(java.lang.String fields) {
return (BatchGet) super.setFields(fields);
}
@Override
public BatchGet setKey(java.lang.String key) {
return (BatchGet) super.setKey(key);
}
@Override
public BatchGet setOauthToken(java.lang.String oauthToken) {
return (BatchGet) super.setOauthToken(oauthToken);
}
@Override
public BatchGet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchGet) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchGet setQuotaUser(java.lang.String quotaUser) {
return (BatchGet) super.setQuotaUser(quotaUser);
}
@Override
public BatchGet setUploadType(java.lang.String uploadType) {
return (BatchGet) super.setUploadType(uploadType);
}
@Override
public BatchGet setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchGet) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Only IAM policies on or below the scope will be returned. This can only be an
* organization number (such as "organizations/123"), a folder number (such as "folders/123"),
* a project ID (such as "projects/my-project-id"), or a project number (such as
* "projects/12345"). To know how to get organization ID, visit [here
* ](https://cloud.google.com/resource-manager/docs/creating-managing-
* organization#retrieving_your_organization_id). To know how to get folder or project ID,
* visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. Only IAM policies on or below the scope will be returned. This can only be an
organization number (such as "organizations/123"), a folder number (such as "folders/123"), a
project ID (such as "projects/my-project-id"), or a project number (such as "projects/12345"). To
know how to get organization ID, visit [here ](https://cloud.google.com/resource-
manager/docs/creating-managing-organization#retrieving_your_organization_id). To know how to get
folder or project ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-
managing-folders#viewing_or_listing_folders_and_projects).
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. Only IAM policies on or below the scope will be returned. This can only be an
* organization number (such as "organizations/123"), a folder number (such as "folders/123"),
* a project ID (such as "projects/my-project-id"), or a project number (such as
* "projects/12345"). To know how to get organization ID, visit [here
* ](https://cloud.google.com/resource-manager/docs/creating-managing-
* organization#retrieving_your_organization_id). To know how to get folder or project ID,
* visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
*/
public BatchGet setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
/**
* Required. The names refer to the [full_resource_names] (https://cloud.google.com/asset-
* inventory/docs/resource-name-format) of the asset types [supported by search
* APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types). A maximum of 20
* resources' effective policies can be retrieved in a batch.
*/
@com.google.api.client.util.Key
private java.util.List names;
/** Required. The names refer to the [full_resource_names] (https://cloud.google.com/asset-
inventory/docs/resource-name-format) of the asset types [supported by search
APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types). A maximum of 20
resources' effective policies can be retrieved in a batch.
*/
public java.util.List getNames() {
return names;
}
/**
* Required. The names refer to the [full_resource_names] (https://cloud.google.com/asset-
* inventory/docs/resource-name-format) of the asset types [supported by search
* APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types). A maximum of 20
* resources' effective policies can be retrieved in a batch.
*/
public BatchGet setNames(java.util.List names) {
this.names = names;
return this;
}
@Override
public BatchGet set(String parameterName, Object value) {
return (BatchGet) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Feeds collection.
*
* The typical use is:
*
* {@code CloudAsset cloudasset = new CloudAsset(...);}
* {@code CloudAsset.Feeds.List request = cloudasset.feeds().list(parameters ...)}
*
*
* @return the resource collection
*/
public Feeds feeds() {
return new Feeds();
}
/**
* The "feeds" collection of methods.
*/
public class Feeds {
/**
* Creates a feed in a parent project/folder/organization to listen to its asset updates.
*
* Create a request for the method "feeds.create".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project/folder/organization where this feed should be created in. It can
* only be an organization number (such as "organizations/123"), a folder number (such as
* "folders/123"), a project ID (such as "projects/my-project-id"), or a project number (such
* as "projects/12345").
* @param content the {@link com.google.api.services.cloudasset.v1.model.CreateFeedRequest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudasset.v1.model.CreateFeedRequest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}/feeds";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Creates a feed in a parent project/folder/organization to listen to its asset updates.
*
* Create a request for the method "feeds.create".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project/folder/organization where this feed should be created in. It can
* only be an organization number (such as "organizations/123"), a folder number (such as
* "folders/123"), a project ID (such as "projects/my-project-id"), or a project number (such
* as "projects/12345").
* @param content the {@link com.google.api.services.cloudasset.v1.model.CreateFeedRequest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudasset.v1.model.CreateFeedRequest content) {
super(CloudAsset.this, "POST", REST_PATH, content, com.google.api.services.cloudasset.v1.model.Feed.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project/folder/organization where this feed should be created in.
* It can only be an organization number (such as "organizations/123"), a folder number (such
* as "folders/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345").
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project/folder/organization where this feed should be created in. It can
only be an organization number (such as "organizations/123"), a folder number (such as
"folders/123"), a project ID (such as "projects/my-project-id"), or a project number (such as
"projects/12345").
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project/folder/organization where this feed should be created in.
* It can only be an organization number (such as "organizations/123"), a folder number (such
* as "folders/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345").
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an asset feed.
*
* Create a request for the method "feeds.delete".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/feeds/[^/]+$");
/**
* Deletes an asset feed.
*
* Create a request for the method "feeds.delete".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudAsset.this, "DELETE", REST_PATH, null, com.google.api.services.cloudasset.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/feeds/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the feed and it must be in the format of:
projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
organizations/organization_number/feeds/feed_id
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/feeds/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details about an asset feed.
*
* Create a request for the method "feeds.get".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/feeds/[^/]+$");
/**
* Gets details about an asset feed.
*
* Create a request for the method "feeds.get".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.Feed.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/feeds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Feed and it must be in the format of:
projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
organizations/organization_number/feeds/feed_id
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Feed and it must be in the format of:
* projects/project_number/feeds/feed_id folders/folder_number/feeds/feed_id
* organizations/organization_number/feeds/feed_id
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/feeds/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all asset feeds in a parent project/folder/organization.
*
* Create a request for the method "feeds.list".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent project/folder/organization whose feeds are to be listed. It can only be using
* project/folder/organization number (such as "folders/12345")", or a project ID (such as
* "projects/my-project-id").
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}/feeds";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Lists all asset feeds in a parent project/folder/organization.
*
* Create a request for the method "feeds.list".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent project/folder/organization whose feeds are to be listed. It can only be using
* project/folder/organization number (such as "folders/12345")", or a project ID (such as
* "projects/my-project-id").
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.ListFeedsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent project/folder/organization whose feeds are to be listed. It can only
* be using project/folder/organization number (such as "folders/12345")", or a project ID
* (such as "projects/my-project-id").
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent project/folder/organization whose feeds are to be listed. It can only be using
project/folder/organization number (such as "folders/12345")", or a project ID (such as
"projects/my-project-id").
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent project/folder/organization whose feeds are to be listed. It can only
* be using project/folder/organization number (such as "folders/12345")", or a project ID
* (such as "projects/my-project-id").
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an asset feed configuration.
*
* Create a request for the method "feeds.patch".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The format will be projects/{project_number}/feeds/{client-assigned_feed_identifier} or
* folders/{folder_number}/feeds/{client-assigned_feed_identifier} or
* organizations/{organization_number}/feeds/{client-assigned_feed_identifier} The client-
* assigned feed identifier must be unique within the parent project/folder/organization.
* @param content the {@link com.google.api.services.cloudasset.v1.model.UpdateFeedRequest}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudasset.v1.model.UpdateFeedRequest content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/feeds/[^/]+$");
/**
* Updates an asset feed configuration.
*
* Create a request for the method "feeds.patch".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The format will be projects/{project_number}/feeds/{client-assigned_feed_identifier} or
* folders/{folder_number}/feeds/{client-assigned_feed_identifier} or
* organizations/{organization_number}/feeds/{client-assigned_feed_identifier} The client-
* assigned feed identifier must be unique within the parent project/folder/organization.
* @param content the {@link com.google.api.services.cloudasset.v1.model.UpdateFeedRequest}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudasset.v1.model.UpdateFeedRequest content) {
super(CloudAsset.this, "PATCH", REST_PATH, content, com.google.api.services.cloudasset.v1.model.Feed.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/feeds/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The format will be projects/{project_number}/feeds/{client-
* assigned_feed_identifier} or folders/{folder_number}/feeds/{client-
* assigned_feed_identifier} or organizations/{organization_number}/feeds/{client-
* assigned_feed_identifier} The client-assigned feed identifier must be unique within the
* parent project/folder/organization.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The format will be projects/{project_number}/feeds/{client-assigned_feed_identifier} or
folders/{folder_number}/feeds/{client-assigned_feed_identifier} or
organizations/{organization_number}/feeds/{client-assigned_feed_identifier} The client-assigned
feed identifier must be unique within the parent project/folder/organization.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The format will be projects/{project_number}/feeds/{client-
* assigned_feed_identifier} or folders/{folder_number}/feeds/{client-
* assigned_feed_identifier} or organizations/{organization_number}/feeds/{client-
* assigned_feed_identifier} The client-assigned feed identifier must be unique within the
* parent project/folder/organization.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/feeds/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudAsset cloudasset = new CloudAsset(...);}
* {@code CloudAsset.Operations.List request = cloudasset.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/operations/[^/]+/.*$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/operations/[^/]+/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/operations/[^/]+/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SavedQueries collection.
*
* The typical use is:
*
* {@code CloudAsset cloudasset = new CloudAsset(...);}
* {@code CloudAsset.SavedQueries.List request = cloudasset.savedQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public SavedQueries savedQueries() {
return new SavedQueries();
}
/**
* The "savedQueries" collection of methods.
*/
public class SavedQueries {
/**
* Creates a saved query in a parent project/folder/organization.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project/folder/organization where this saved_query should be created in.
* It can only be an organization number (such as "organizations/123"), a folder number (such
* as "folders/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345").
* @param content the {@link com.google.api.services.cloudasset.v1.model.SavedQuery}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudasset.v1.model.SavedQuery content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Creates a saved query in a parent project/folder/organization.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project/folder/organization where this saved_query should be created in.
* It can only be an organization number (such as "organizations/123"), a folder number (such
* as "folders/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345").
* @param content the {@link com.google.api.services.cloudasset.v1.model.SavedQuery}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudasset.v1.model.SavedQuery content) {
super(CloudAsset.this, "POST", REST_PATH, content, com.google.api.services.cloudasset.v1.model.SavedQuery.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project/folder/organization where this saved_query should be
* created in. It can only be an organization number (such as "organizations/123"), a folder
* number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or a
* project number (such as "projects/12345").
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project/folder/organization where this saved_query should be created in.
It can only be an organization number (such as "organizations/123"), a folder number (such as
"folders/123"), a project ID (such as "projects/my-project-id"), or a project number (such as
"projects/12345").
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project/folder/organization where this saved_query should be
* created in. It can only be an organization number (such as "organizations/123"), a folder
* number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or a
* project number (such as "projects/12345").
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the saved query, which must be unique in the specified parent.
* It will become the final component of the saved query's resource name. This value should be
* 4-63 characters, and valid characters are `a-z-`. Notice that this field is required in the
* saved query creation, and the `name` field of the `saved_query` will be ignored.
*/
@com.google.api.client.util.Key
private java.lang.String savedQueryId;
/** Required. The ID to use for the saved query, which must be unique in the specified parent. It will
become the final component of the saved query's resource name. This value should be 4-63
characters, and valid characters are `a-z-`. Notice that this field is required in the saved query
creation, and the `name` field of the `saved_query` will be ignored.
*/
public java.lang.String getSavedQueryId() {
return savedQueryId;
}
/**
* Required. The ID to use for the saved query, which must be unique in the specified parent.
* It will become the final component of the saved query's resource name. This value should be
* 4-63 characters, and valid characters are `a-z-`. Notice that this field is required in the
* saved query creation, and the `name` field of the `saved_query` will be ignored.
*/
public Create setSavedQueryId(java.lang.String savedQueryId) {
this.savedQueryId = savedQueryId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a saved query.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the saved query to delete. It must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/savedQueries/[^/]+$");
/**
* Deletes a saved query.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the saved query to delete. It must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudAsset.this, "DELETE", REST_PATH, null, com.google.api.services.cloudasset.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/savedQueries/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the saved query to delete. It must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the saved query to delete. It must be in the format of: *
projects/project_number/savedQueries/saved_query_id *
folders/folder_number/savedQueries/saved_query_id *
organizations/organization_number/savedQueries/saved_query_id
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the saved query to delete. It must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/savedQueries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details about a saved query.
*
* Create a request for the method "savedQueries.get".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the saved query and it must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/savedQueries/[^/]+$");
/**
* Gets details about a saved query.
*
* Create a request for the method "savedQueries.get".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the saved query and it must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.SavedQuery.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/savedQueries/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the saved query and it must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the saved query and it must be in the format of: *
projects/project_number/savedQueries/saved_query_id *
folders/folder_number/savedQueries/saved_query_id *
organizations/organization_number/savedQueries/saved_query_id
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the saved query and it must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/savedQueries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all saved queries in a parent project/folder/organization.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent project/folder/organization whose savedQueries are to be listed. It can only be
* using project/folder/organization number (such as "folders/12345")", or a project ID (such
* as "projects/my-project-id").
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Lists all saved queries in a parent project/folder/organization.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent project/folder/organization whose savedQueries are to be listed. It can only be
* using project/folder/organization number (such as "folders/12345")", or a project ID (such
* as "projects/my-project-id").
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.ListSavedQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent project/folder/organization whose savedQueries are to be listed. It
* can only be using project/folder/organization number (such as "folders/12345")", or a
* project ID (such as "projects/my-project-id").
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent project/folder/organization whose savedQueries are to be listed. It can only
be using project/folder/organization number (such as "folders/12345")", or a project ID (such as
"projects/my-project-id").
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent project/folder/organization whose savedQueries are to be listed. It
* can only be using project/folder/organization number (such as "folders/12345")", or a
* project ID (such as "projects/my-project-id").
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The expression to filter resources. The expression is a list of zero or more
* restrictions combined via logical operators `AND` and `OR`. When `AND` and `OR` are both
* used in the expression, parentheses must be appropriately used to group the combinations.
* The expression may also contain regular expressions. See https://google.aip.dev/160 for
* more information on the grammar.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. The expression to filter resources. The expression is a list of zero or more restrictions
combined via logical operators `AND` and `OR`. When `AND` and `OR` are both used in the expression,
parentheses must be appropriately used to group the combinations. The expression may also contain
regular expressions. See https://google.aip.dev/160 for more information on the grammar.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. The expression to filter resources. The expression is a list of zero or more
* restrictions combined via logical operators `AND` and `OR`. When `AND` and `OR` are both
* used in the expression, parentheses must be appropriately used to group the combinations.
* The expression may also contain regular expressions. See https://google.aip.dev/160 for
* more information on the grammar.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of saved queries to return per page. The service may return
* fewer than this value. If unspecified, at most 50 will be returned. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of saved queries to return per page. The service may return fewer than
this value. If unspecified, at most 50 will be returned. The maximum value is 1000; values above
1000 will be coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of saved queries to return per page. The service may return
* fewer than this value. If unspecified, at most 50 will be returned. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListSavedQueries` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSavedQueries` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListSavedQueries` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListSavedQueries` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListSavedQueries` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSavedQueries` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a saved query.
*
* Create a request for the method "savedQueries.patch".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The resource name of the saved query. The format must be: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
* @param content the {@link com.google.api.services.cloudasset.v1.model.SavedQuery}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudasset.v1.model.SavedQuery content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/savedQueries/[^/]+$");
/**
* Updates a saved query.
*
* Create a request for the method "savedQueries.patch".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource name of the saved query. The format must be: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
* @param content the {@link com.google.api.services.cloudasset.v1.model.SavedQuery}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudasset.v1.model.SavedQuery content) {
super(CloudAsset.this, "PATCH", REST_PATH, content, com.google.api.services.cloudasset.v1.model.SavedQuery.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/savedQueries/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the saved query. The format must be: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the saved query. The format must be: *
projects/project_number/savedQueries/saved_query_id *
folders/folder_number/savedQueries/saved_query_id *
organizations/organization_number/savedQueries/saved_query_id
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the saved query. The format must be: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/savedQueries/[^/]+$");
}
this.name = name;
return this;
}
/** Required. The list of fields to update. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. The list of fields to update.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. The list of fields to update. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the V1 collection.
*
* The typical use is:
*
* {@code CloudAsset cloudasset = new CloudAsset(...);}
* {@code CloudAsset.V1.List request = cloudasset.v1().list(parameters ...)}
*
*
* @return the resource collection
*/
public V1 v1() {
return new V1();
}
/**
* The "v1" collection of methods.
*/
public class V1 {
/**
* Analyzes IAM policies to answer which identities have what accesses on which resources.
*
* Create a request for the method "v1.analyzeIamPolicy".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link AnalyzeIamPolicy#execute()} method to invoke the remote operation.
*
* @param scope Required. The relative name of the root asset. Only resources and IAM policies within the scope will
* be analyzed. This can only be an organization number (such as "organizations/123"), a
* folder number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or
* a project number (such as "projects/12345"). To know how to get organization ID, visit
* [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* organization#retrieving_your_organization_id). To know how to get folder or project ID,
* visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
* @return the request
*/
public AnalyzeIamPolicy analyzeIamPolicy(java.lang.String scope) throws java.io.IOException {
AnalyzeIamPolicy result = new AnalyzeIamPolicy(scope);
initialize(result);
return result;
}
public class AnalyzeIamPolicy extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}:analyzeIamPolicy";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Analyzes IAM policies to answer which identities have what accesses on which resources.
*
* Create a request for the method "v1.analyzeIamPolicy".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link AnalyzeIamPolicy#execute()} method to invoke the remote
* operation. {@link AnalyzeIamPolicy#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param scope Required. The relative name of the root asset. Only resources and IAM policies within the scope will
* be analyzed. This can only be an organization number (such as "organizations/123"), a
* folder number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or
* a project number (such as "projects/12345"). To know how to get organization ID, visit
* [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* organization#retrieving_your_organization_id). To know how to get folder or project ID,
* visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
* @since 1.13
*/
protected AnalyzeIamPolicy(java.lang.String scope) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.AnalyzeIamPolicyResponse.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public AnalyzeIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (AnalyzeIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public AnalyzeIamPolicy setAccessToken(java.lang.String accessToken) {
return (AnalyzeIamPolicy) super.setAccessToken(accessToken);
}
@Override
public AnalyzeIamPolicy setAlt(java.lang.String alt) {
return (AnalyzeIamPolicy) super.setAlt(alt);
}
@Override
public AnalyzeIamPolicy setCallback(java.lang.String callback) {
return (AnalyzeIamPolicy) super.setCallback(callback);
}
@Override
public AnalyzeIamPolicy setFields(java.lang.String fields) {
return (AnalyzeIamPolicy) super.setFields(fields);
}
@Override
public AnalyzeIamPolicy setKey(java.lang.String key) {
return (AnalyzeIamPolicy) super.setKey(key);
}
@Override
public AnalyzeIamPolicy setOauthToken(java.lang.String oauthToken) {
return (AnalyzeIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public AnalyzeIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AnalyzeIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public AnalyzeIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (AnalyzeIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public AnalyzeIamPolicy setUploadType(java.lang.String uploadType) {
return (AnalyzeIamPolicy) super.setUploadType(uploadType);
}
@Override
public AnalyzeIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (AnalyzeIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The relative name of the root asset. Only resources and IAM policies within the
* scope will be analyzed. This can only be an organization number (such as
* "organizations/123"), a folder number (such as "folders/123"), a project ID (such as
* "projects/my-project-id"), or a project number (such as "projects/12345"). To know how to
* get organization ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-
* managing-organization#retrieving_your_organization_id). To know how to get folder or
* project ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. The relative name of the root asset. Only resources and IAM policies within the scope
will be analyzed. This can only be an organization number (such as "organizations/123"), a folder
number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or a project
number (such as "projects/12345"). To know how to get organization ID, visit [here
](https://cloud.google.com/resource-manager/docs/creating-managing-
organization#retrieving_your_organization_id). To know how to get folder or project ID, visit [here
](https://cloud.google.com/resource-manager/docs/creating-managing-
folders#viewing_or_listing_folders_and_projects).
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. The relative name of the root asset. Only resources and IAM policies within the
* scope will be analyzed. This can only be an organization number (such as
* "organizations/123"), a folder number (such as "folders/123"), a project ID (such as
* "projects/my-project-id"), or a project number (such as "projects/12345"). To know how to
* get organization ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-
* managing-organization#retrieving_your_organization_id). To know how to get folder or
* project ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
*/
public AnalyzeIamPolicy setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
/** Optional. The permissions to appear in result. */
@com.google.api.client.util.Key("analysisQuery.accessSelector.permissions")
private java.util.List analysisQueryAccessSelectorPermissions;
/** Optional. The permissions to appear in result.
*/
public java.util.List getAnalysisQueryAccessSelectorPermissions() {
return analysisQueryAccessSelectorPermissions;
}
/** Optional. The permissions to appear in result. */
public AnalyzeIamPolicy setAnalysisQueryAccessSelectorPermissions(java.util.List analysisQueryAccessSelectorPermissions) {
this.analysisQueryAccessSelectorPermissions = analysisQueryAccessSelectorPermissions;
return this;
}
/** Optional. The roles to appear in result. */
@com.google.api.client.util.Key("analysisQuery.accessSelector.roles")
private java.util.List analysisQueryAccessSelectorRoles;
/** Optional. The roles to appear in result.
*/
public java.util.List getAnalysisQueryAccessSelectorRoles() {
return analysisQueryAccessSelectorRoles;
}
/** Optional. The roles to appear in result. */
public AnalyzeIamPolicy setAnalysisQueryAccessSelectorRoles(java.util.List analysisQueryAccessSelectorRoles) {
this.analysisQueryAccessSelectorRoles = analysisQueryAccessSelectorRoles;
return this;
}
/**
* The hypothetical access timestamp to evaluate IAM conditions. Note that this value must not
* be earlier than the current time; otherwise, an INVALID_ARGUMENT error will be returned.
*/
@com.google.api.client.util.Key("analysisQuery.conditionContext.accessTime")
private String analysisQueryConditionContextAccessTime;
/** The hypothetical access timestamp to evaluate IAM conditions. Note that this value must not be
earlier than the current time; otherwise, an INVALID_ARGUMENT error will be returned.
*/
public String getAnalysisQueryConditionContextAccessTime() {
return analysisQueryConditionContextAccessTime;
}
/**
* The hypothetical access timestamp to evaluate IAM conditions. Note that this value must not
* be earlier than the current time; otherwise, an INVALID_ARGUMENT error will be returned.
*/
public AnalyzeIamPolicy setAnalysisQueryConditionContextAccessTime(String analysisQueryConditionContextAccessTime) {
this.analysisQueryConditionContextAccessTime = analysisQueryConditionContextAccessTime;
return this;
}
/**
* Required. The identity appear in the form of principals in [IAM policy
* binding](https://cloud.google.com/iam/reference/rest/v1/Binding). The examples of supported
* forms are: "user:[email protected]", "group:[email protected]", "domain:google.com",
* "serviceAccount:[email protected]". Notice that wildcard characters
* (such as * and ?) are not supported. You must give a specific identity.
*/
@com.google.api.client.util.Key("analysisQuery.identitySelector.identity")
private java.lang.String analysisQueryIdentitySelectorIdentity;
/** Required. The identity appear in the form of principals in [IAM policy
binding](https://cloud.google.com/iam/reference/rest/v1/Binding). The examples of supported forms
are: "user:[email protected]", "group:[email protected]", "domain:google.com", "serviceAccount:my-
[email protected]". Notice that wildcard characters (such as * and ?) are not
supported. You must give a specific identity.
*/
public java.lang.String getAnalysisQueryIdentitySelectorIdentity() {
return analysisQueryIdentitySelectorIdentity;
}
/**
* Required. The identity appear in the form of principals in [IAM policy
* binding](https://cloud.google.com/iam/reference/rest/v1/Binding). The examples of supported
* forms are: "user:[email protected]", "group:[email protected]", "domain:google.com",
* "serviceAccount:[email protected]". Notice that wildcard characters
* (such as * and ?) are not supported. You must give a specific identity.
*/
public AnalyzeIamPolicy setAnalysisQueryIdentitySelectorIdentity(java.lang.String analysisQueryIdentitySelectorIdentity) {
this.analysisQueryIdentitySelectorIdentity = analysisQueryIdentitySelectorIdentity;
return this;
}
/**
* Optional. If true, the response will include access analysis from identities to resources
* via service account impersonation. This is a very expensive operation, because many derived
* queries will be executed. We highly recommend you use
* AssetService.AnalyzeIamPolicyLongrunning RPC instead. For example, if the request analyzes
* for which resources user A has permission P, and there's an IAM policy states user A has
* iam.serviceAccounts.getAccessToken permission to a service account SA, and there's another
* IAM policy states service account SA has permission P to a Google Cloud folder F, then user
* A potentially has access to the Google Cloud folder F. And those advanced analysis results
* will be included in AnalyzeIamPolicyResponse.service_account_impersonation_analysis.
* Another example, if the request analyzes for who has permission P to a Google Cloud folder
* F, and there's an IAM policy states user A has iam.serviceAccounts.actAs permission to a
* service account SA, and there's another IAM policy states service account SA has permission
* P to the Google Cloud folder F, then user A potentially has access to the Google Cloud
* folder F. And those advanced analysis results will be included in
* AnalyzeIamPolicyResponse.service_account_impersonation_analysis. Only the following
* permissions are considered in this analysis: * `iam.serviceAccounts.actAs` *
* `iam.serviceAccounts.signBlob` * `iam.serviceAccounts.signJwt` *
* `iam.serviceAccounts.getAccessToken` * `iam.serviceAccounts.getOpenIdToken` *
* `iam.serviceAccounts.implicitDelegation` Default is false.
*/
@com.google.api.client.util.Key("analysisQuery.options.analyzeServiceAccountImpersonation")
private java.lang.Boolean analysisQueryOptionsAnalyzeServiceAccountImpersonation;
/** Optional. If true, the response will include access analysis from identities to resources via
service account impersonation. This is a very expensive operation, because many derived queries
will be executed. We highly recommend you use AssetService.AnalyzeIamPolicyLongrunning RPC instead.
For example, if the request analyzes for which resources user A has permission P, and there's an
IAM policy states user A has iam.serviceAccounts.getAccessToken permission to a service account SA,
and there's another IAM policy states service account SA has permission P to a Google Cloud folder
F, then user A potentially has access to the Google Cloud folder F. And those advanced analysis
results will be included in AnalyzeIamPolicyResponse.service_account_impersonation_analysis.
Another example, if the request analyzes for who has permission P to a Google Cloud folder F, and
there's an IAM policy states user A has iam.serviceAccounts.actAs permission to a service account
SA, and there's another IAM policy states service account SA has permission P to the Google Cloud
folder F, then user A potentially has access to the Google Cloud folder F. And those advanced
analysis results will be included in
AnalyzeIamPolicyResponse.service_account_impersonation_analysis. Only the following permissions are
considered in this analysis: * `iam.serviceAccounts.actAs` * `iam.serviceAccounts.signBlob` *
`iam.serviceAccounts.signJwt` * `iam.serviceAccounts.getAccessToken` *
`iam.serviceAccounts.getOpenIdToken` * `iam.serviceAccounts.implicitDelegation` Default is false.
*/
public java.lang.Boolean getAnalysisQueryOptionsAnalyzeServiceAccountImpersonation() {
return analysisQueryOptionsAnalyzeServiceAccountImpersonation;
}
/**
* Optional. If true, the response will include access analysis from identities to resources
* via service account impersonation. This is a very expensive operation, because many derived
* queries will be executed. We highly recommend you use
* AssetService.AnalyzeIamPolicyLongrunning RPC instead. For example, if the request analyzes
* for which resources user A has permission P, and there's an IAM policy states user A has
* iam.serviceAccounts.getAccessToken permission to a service account SA, and there's another
* IAM policy states service account SA has permission P to a Google Cloud folder F, then user
* A potentially has access to the Google Cloud folder F. And those advanced analysis results
* will be included in AnalyzeIamPolicyResponse.service_account_impersonation_analysis.
* Another example, if the request analyzes for who has permission P to a Google Cloud folder
* F, and there's an IAM policy states user A has iam.serviceAccounts.actAs permission to a
* service account SA, and there's another IAM policy states service account SA has permission
* P to the Google Cloud folder F, then user A potentially has access to the Google Cloud
* folder F. And those advanced analysis results will be included in
* AnalyzeIamPolicyResponse.service_account_impersonation_analysis. Only the following
* permissions are considered in this analysis: * `iam.serviceAccounts.actAs` *
* `iam.serviceAccounts.signBlob` * `iam.serviceAccounts.signJwt` *
* `iam.serviceAccounts.getAccessToken` * `iam.serviceAccounts.getOpenIdToken` *
* `iam.serviceAccounts.implicitDelegation` Default is false.
*/
public AnalyzeIamPolicy setAnalysisQueryOptionsAnalyzeServiceAccountImpersonation(java.lang.Boolean analysisQueryOptionsAnalyzeServiceAccountImpersonation) {
this.analysisQueryOptionsAnalyzeServiceAccountImpersonation = analysisQueryOptionsAnalyzeServiceAccountImpersonation;
return this;
}
/**
* Optional. If true, the identities section of the result will expand any Google groups
* appearing in an IAM policy binding. If IamPolicyAnalysisQuery.identity_selector is
* specified, the identity in the result will be determined by the selector, and this flag is
* not allowed to set. If true, the default max expansion per group is 1000 for
* AssetService.AnalyzeIamPolicy][]. Default is false.
*/
@com.google.api.client.util.Key("analysisQuery.options.expandGroups")
private java.lang.Boolean analysisQueryOptionsExpandGroups;
/** Optional. If true, the identities section of the result will expand any Google groups appearing in
an IAM policy binding. If IamPolicyAnalysisQuery.identity_selector is specified, the identity in
the result will be determined by the selector, and this flag is not allowed to set. If true, the
default max expansion per group is 1000 for AssetService.AnalyzeIamPolicy][]. Default is false.
*/
public java.lang.Boolean getAnalysisQueryOptionsExpandGroups() {
return analysisQueryOptionsExpandGroups;
}
/**
* Optional. If true, the identities section of the result will expand any Google groups
* appearing in an IAM policy binding. If IamPolicyAnalysisQuery.identity_selector is
* specified, the identity in the result will be determined by the selector, and this flag is
* not allowed to set. If true, the default max expansion per group is 1000 for
* AssetService.AnalyzeIamPolicy][]. Default is false.
*/
public AnalyzeIamPolicy setAnalysisQueryOptionsExpandGroups(java.lang.Boolean analysisQueryOptionsExpandGroups) {
this.analysisQueryOptionsExpandGroups = analysisQueryOptionsExpandGroups;
return this;
}
/**
* Optional. If true and IamPolicyAnalysisQuery.resource_selector is not specified, the
* resource section of the result will expand any resource attached to an IAM policy to
* include resources lower in the resource hierarchy. For example, if the request analyzes for
* which resources user A has permission P, and the results include an IAM policy with P on a
* Google Cloud folder, the results will also include resources in that folder with permission
* P. If true and IamPolicyAnalysisQuery.resource_selector is specified, the resource section
* of the result will expand the specified resource to include resources lower in the resource
* hierarchy. Only project or lower resources are supported. Folder and organization resources
* cannot be used together with this option. For example, if the request analyzes for which
* users have permission P on a Google Cloud project with this option enabled, the results
* will include all users who have permission P on that project or any lower resource. If
* true, the default max expansion per resource is 1000 for AssetService.AnalyzeIamPolicy][]
* and 100000 for AssetService.AnalyzeIamPolicyLongrunning][]. Default is false.
*/
@com.google.api.client.util.Key("analysisQuery.options.expandResources")
private java.lang.Boolean analysisQueryOptionsExpandResources;
/** Optional. If true and IamPolicyAnalysisQuery.resource_selector is not specified, the resource
section of the result will expand any resource attached to an IAM policy to include resources lower
in the resource hierarchy. For example, if the request analyzes for which resources user A has
permission P, and the results include an IAM policy with P on a Google Cloud folder, the results
will also include resources in that folder with permission P. If true and
IamPolicyAnalysisQuery.resource_selector is specified, the resource section of the result will
expand the specified resource to include resources lower in the resource hierarchy. Only project or
lower resources are supported. Folder and organization resources cannot be used together with this
option. For example, if the request analyzes for which users have permission P on a Google Cloud
project with this option enabled, the results will include all users who have permission P on that
project or any lower resource. If true, the default max expansion per resource is 1000 for
AssetService.AnalyzeIamPolicy][] and 100000 for AssetService.AnalyzeIamPolicyLongrunning][].
Default is false.
*/
public java.lang.Boolean getAnalysisQueryOptionsExpandResources() {
return analysisQueryOptionsExpandResources;
}
/**
* Optional. If true and IamPolicyAnalysisQuery.resource_selector is not specified, the
* resource section of the result will expand any resource attached to an IAM policy to
* include resources lower in the resource hierarchy. For example, if the request analyzes for
* which resources user A has permission P, and the results include an IAM policy with P on a
* Google Cloud folder, the results will also include resources in that folder with permission
* P. If true and IamPolicyAnalysisQuery.resource_selector is specified, the resource section
* of the result will expand the specified resource to include resources lower in the resource
* hierarchy. Only project or lower resources are supported. Folder and organization resources
* cannot be used together with this option. For example, if the request analyzes for which
* users have permission P on a Google Cloud project with this option enabled, the results
* will include all users who have permission P on that project or any lower resource. If
* true, the default max expansion per resource is 1000 for AssetService.AnalyzeIamPolicy][]
* and 100000 for AssetService.AnalyzeIamPolicyLongrunning][]. Default is false.
*/
public AnalyzeIamPolicy setAnalysisQueryOptionsExpandResources(java.lang.Boolean analysisQueryOptionsExpandResources) {
this.analysisQueryOptionsExpandResources = analysisQueryOptionsExpandResources;
return this;
}
/**
* Optional. If true, the access section of result will expand any roles appearing in IAM
* policy bindings to include their permissions. If IamPolicyAnalysisQuery.access_selector is
* specified, the access section of the result will be determined by the selector, and this
* flag is not allowed to set. Default is false.
*/
@com.google.api.client.util.Key("analysisQuery.options.expandRoles")
private java.lang.Boolean analysisQueryOptionsExpandRoles;
/** Optional. If true, the access section of result will expand any roles appearing in IAM policy
bindings to include their permissions. If IamPolicyAnalysisQuery.access_selector is specified, the
access section of the result will be determined by the selector, and this flag is not allowed to
set. Default is false.
*/
public java.lang.Boolean getAnalysisQueryOptionsExpandRoles() {
return analysisQueryOptionsExpandRoles;
}
/**
* Optional. If true, the access section of result will expand any roles appearing in IAM
* policy bindings to include their permissions. If IamPolicyAnalysisQuery.access_selector is
* specified, the access section of the result will be determined by the selector, and this
* flag is not allowed to set. Default is false.
*/
public AnalyzeIamPolicy setAnalysisQueryOptionsExpandRoles(java.lang.Boolean analysisQueryOptionsExpandRoles) {
this.analysisQueryOptionsExpandRoles = analysisQueryOptionsExpandRoles;
return this;
}
/**
* Optional. If true, the result will output the relevant membership relationships between
* groups and other groups, and between groups and principals. Default is false.
*/
@com.google.api.client.util.Key("analysisQuery.options.outputGroupEdges")
private java.lang.Boolean analysisQueryOptionsOutputGroupEdges;
/** Optional. If true, the result will output the relevant membership relationships between groups and
other groups, and between groups and principals. Default is false.
*/
public java.lang.Boolean getAnalysisQueryOptionsOutputGroupEdges() {
return analysisQueryOptionsOutputGroupEdges;
}
/**
* Optional. If true, the result will output the relevant membership relationships between
* groups and other groups, and between groups and principals. Default is false.
*/
public AnalyzeIamPolicy setAnalysisQueryOptionsOutputGroupEdges(java.lang.Boolean analysisQueryOptionsOutputGroupEdges) {
this.analysisQueryOptionsOutputGroupEdges = analysisQueryOptionsOutputGroupEdges;
return this;
}
/**
* Optional. If true, the result will output the relevant parent/child relationships between
* resources. Default is false.
*/
@com.google.api.client.util.Key("analysisQuery.options.outputResourceEdges")
private java.lang.Boolean analysisQueryOptionsOutputResourceEdges;
/** Optional. If true, the result will output the relevant parent/child relationships between
resources. Default is false.
*/
public java.lang.Boolean getAnalysisQueryOptionsOutputResourceEdges() {
return analysisQueryOptionsOutputResourceEdges;
}
/**
* Optional. If true, the result will output the relevant parent/child relationships between
* resources. Default is false.
*/
public AnalyzeIamPolicy setAnalysisQueryOptionsOutputResourceEdges(java.lang.Boolean analysisQueryOptionsOutputResourceEdges) {
this.analysisQueryOptionsOutputResourceEdges = analysisQueryOptionsOutputResourceEdges;
return this;
}
/**
* Required. The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-
* name-format) of a resource of [supported resource types](https://cloud.google.com/asset-
* inventory/docs/supported-asset-types#analyzable_asset_types).
*/
@com.google.api.client.util.Key("analysisQuery.resourceSelector.fullResourceName")
private java.lang.String analysisQueryResourceSelectorFullResourceName;
/** Required. The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-name-
format) of a resource of [supported resource types](https://cloud.google.com/asset-
inventory/docs/supported-asset-types#analyzable_asset_types).
*/
public java.lang.String getAnalysisQueryResourceSelectorFullResourceName() {
return analysisQueryResourceSelectorFullResourceName;
}
/**
* Required. The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-
* name-format) of a resource of [supported resource types](https://cloud.google.com/asset-
* inventory/docs/supported-asset-types#analyzable_asset_types).
*/
public AnalyzeIamPolicy setAnalysisQueryResourceSelectorFullResourceName(java.lang.String analysisQueryResourceSelectorFullResourceName) {
this.analysisQueryResourceSelectorFullResourceName = analysisQueryResourceSelectorFullResourceName;
return this;
}
/**
* Optional. Amount of time executable has to complete. See JSON representation of
* [Duration](https://developers.google.com/protocol-buffers/docs/proto3#json). If this field
* is set with a value less than the RPC deadline, and the execution of your query hasn't
* finished in the specified execution timeout, you will get a response with partial result.
* Otherwise, your query's execution will continue until the RPC deadline. If it's not
* finished until then, you will get a DEADLINE_EXCEEDED error. Default is empty.
*/
@com.google.api.client.util.Key
private String executionTimeout;
/** Optional. Amount of time executable has to complete. See JSON representation of
[Duration](https://developers.google.com/protocol-buffers/docs/proto3#json). If this field is set
with a value less than the RPC deadline, and the execution of your query hasn't finished in the
specified execution timeout, you will get a response with partial result. Otherwise, your query's
execution will continue until the RPC deadline. If it's not finished until then, you will get a
DEADLINE_EXCEEDED error. Default is empty.
*/
public String getExecutionTimeout() {
return executionTimeout;
}
/**
* Optional. Amount of time executable has to complete. See JSON representation of
* [Duration](https://developers.google.com/protocol-buffers/docs/proto3#json). If this field
* is set with a value less than the RPC deadline, and the execution of your query hasn't
* finished in the specified execution timeout, you will get a response with partial result.
* Otherwise, your query's execution will continue until the RPC deadline. If it's not
* finished until then, you will get a DEADLINE_EXCEEDED error. Default is empty.
*/
public AnalyzeIamPolicy setExecutionTimeout(String executionTimeout) {
this.executionTimeout = executionTimeout;
return this;
}
/**
* Optional. The name of a saved query, which must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id If both `analysis_query` and
* `saved_analysis_query` are provided, they will be merged together with the
* `saved_analysis_query` as base and the `analysis_query` as overrides. For more details of
* the merge behavior, refer to the [MergeFrom](https://developers.google.com/protocol-
* buffers/docs/reference/cpp/google.protobuf.message#Message.MergeFrom.details) page. Note
* that you cannot override primitive fields with default value, such as 0 or empty string,
* etc., because we use proto3, which doesn't support field presence yet.
*/
@com.google.api.client.util.Key
private java.lang.String savedAnalysisQuery;
/** Optional. The name of a saved query, which must be in the format of: *
projects/project_number/savedQueries/saved_query_id *
folders/folder_number/savedQueries/saved_query_id *
organizations/organization_number/savedQueries/saved_query_id If both `analysis_query` and
`saved_analysis_query` are provided, they will be merged together with the `saved_analysis_query`
as base and the `analysis_query` as overrides. For more details of the merge behavior, refer to the
[MergeFrom](https://developers.google.com/protocol-
buffers/docs/reference/cpp/google.protobuf.message#Message.MergeFrom.details) page. Note that you
cannot override primitive fields with default value, such as 0 or empty string, etc., because we
use proto3, which doesn't support field presence yet.
*/
public java.lang.String getSavedAnalysisQuery() {
return savedAnalysisQuery;
}
/**
* Optional. The name of a saved query, which must be in the format of: *
* projects/project_number/savedQueries/saved_query_id *
* folders/folder_number/savedQueries/saved_query_id *
* organizations/organization_number/savedQueries/saved_query_id If both `analysis_query` and
* `saved_analysis_query` are provided, they will be merged together with the
* `saved_analysis_query` as base and the `analysis_query` as overrides. For more details of
* the merge behavior, refer to the [MergeFrom](https://developers.google.com/protocol-
* buffers/docs/reference/cpp/google.protobuf.message#Message.MergeFrom.details) page. Note
* that you cannot override primitive fields with default value, such as 0 or empty string,
* etc., because we use proto3, which doesn't support field presence yet.
*/
public AnalyzeIamPolicy setSavedAnalysisQuery(java.lang.String savedAnalysisQuery) {
this.savedAnalysisQuery = savedAnalysisQuery;
return this;
}
@Override
public AnalyzeIamPolicy set(String parameterName, Object value) {
return (AnalyzeIamPolicy) super.set(parameterName, value);
}
}
/**
* Analyzes IAM policies asynchronously to answer which identities have what accesses on which
* resources, and writes the analysis results to a Google Cloud Storage or a BigQuery destination.
* For Cloud Storage destination, the output format is the JSON format that represents a
* AnalyzeIamPolicyResponse. This method implements the google.longrunning.Operation, which allows
* you to track the operation status. We recommend intervals of at least 2 seconds with exponential
* backoff retry to poll the operation result. The metadata contains the metadata for the long-
* running operation.
*
* Create a request for the method "v1.analyzeIamPolicyLongrunning".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link AnalyzeIamPolicyLongrunning#execute()} method to invoke the remote
* operation.
*
* @param scope Required. The relative name of the root asset. Only resources and IAM policies within the scope will
* be analyzed. This can only be an organization number (such as "organizations/123"), a
* folder number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or
* a project number (such as "projects/12345"). To know how to get organization ID, visit
* [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* organization#retrieving_your_organization_id). To know how to get folder or project ID,
* visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
* @param content the {@link com.google.api.services.cloudasset.v1.model.AnalyzeIamPolicyLongrunningRequest}
* @return the request
*/
public AnalyzeIamPolicyLongrunning analyzeIamPolicyLongrunning(java.lang.String scope, com.google.api.services.cloudasset.v1.model.AnalyzeIamPolicyLongrunningRequest content) throws java.io.IOException {
AnalyzeIamPolicyLongrunning result = new AnalyzeIamPolicyLongrunning(scope, content);
initialize(result);
return result;
}
public class AnalyzeIamPolicyLongrunning extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}:analyzeIamPolicyLongrunning";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Analyzes IAM policies asynchronously to answer which identities have what accesses on which
* resources, and writes the analysis results to a Google Cloud Storage or a BigQuery destination.
* For Cloud Storage destination, the output format is the JSON format that represents a
* AnalyzeIamPolicyResponse. This method implements the google.longrunning.Operation, which allows
* you to track the operation status. We recommend intervals of at least 2 seconds with
* exponential backoff retry to poll the operation result. The metadata contains the metadata for
* the long-running operation.
*
* Create a request for the method "v1.analyzeIamPolicyLongrunning".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link AnalyzeIamPolicyLongrunning#execute()} method to invoke
* the remote operation. {@link AnalyzeIamPolicyLongrunning#initialize(com.google.api.client.g
* oogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param scope Required. The relative name of the root asset. Only resources and IAM policies within the scope will
* be analyzed. This can only be an organization number (such as "organizations/123"), a
* folder number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or
* a project number (such as "projects/12345"). To know how to get organization ID, visit
* [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* organization#retrieving_your_organization_id). To know how to get folder or project ID,
* visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
* @param content the {@link com.google.api.services.cloudasset.v1.model.AnalyzeIamPolicyLongrunningRequest}
* @since 1.13
*/
protected AnalyzeIamPolicyLongrunning(java.lang.String scope, com.google.api.services.cloudasset.v1.model.AnalyzeIamPolicyLongrunningRequest content) {
super(CloudAsset.this, "POST", REST_PATH, content, com.google.api.services.cloudasset.v1.model.Operation.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public AnalyzeIamPolicyLongrunning set$Xgafv(java.lang.String $Xgafv) {
return (AnalyzeIamPolicyLongrunning) super.set$Xgafv($Xgafv);
}
@Override
public AnalyzeIamPolicyLongrunning setAccessToken(java.lang.String accessToken) {
return (AnalyzeIamPolicyLongrunning) super.setAccessToken(accessToken);
}
@Override
public AnalyzeIamPolicyLongrunning setAlt(java.lang.String alt) {
return (AnalyzeIamPolicyLongrunning) super.setAlt(alt);
}
@Override
public AnalyzeIamPolicyLongrunning setCallback(java.lang.String callback) {
return (AnalyzeIamPolicyLongrunning) super.setCallback(callback);
}
@Override
public AnalyzeIamPolicyLongrunning setFields(java.lang.String fields) {
return (AnalyzeIamPolicyLongrunning) super.setFields(fields);
}
@Override
public AnalyzeIamPolicyLongrunning setKey(java.lang.String key) {
return (AnalyzeIamPolicyLongrunning) super.setKey(key);
}
@Override
public AnalyzeIamPolicyLongrunning setOauthToken(java.lang.String oauthToken) {
return (AnalyzeIamPolicyLongrunning) super.setOauthToken(oauthToken);
}
@Override
public AnalyzeIamPolicyLongrunning setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AnalyzeIamPolicyLongrunning) super.setPrettyPrint(prettyPrint);
}
@Override
public AnalyzeIamPolicyLongrunning setQuotaUser(java.lang.String quotaUser) {
return (AnalyzeIamPolicyLongrunning) super.setQuotaUser(quotaUser);
}
@Override
public AnalyzeIamPolicyLongrunning setUploadType(java.lang.String uploadType) {
return (AnalyzeIamPolicyLongrunning) super.setUploadType(uploadType);
}
@Override
public AnalyzeIamPolicyLongrunning setUploadProtocol(java.lang.String uploadProtocol) {
return (AnalyzeIamPolicyLongrunning) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The relative name of the root asset. Only resources and IAM policies within the
* scope will be analyzed. This can only be an organization number (such as
* "organizations/123"), a folder number (such as "folders/123"), a project ID (such as
* "projects/my-project-id"), or a project number (such as "projects/12345"). To know how to
* get organization ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-
* managing-organization#retrieving_your_organization_id). To know how to get folder or
* project ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. The relative name of the root asset. Only resources and IAM policies within the scope
will be analyzed. This can only be an organization number (such as "organizations/123"), a folder
number (such as "folders/123"), a project ID (such as "projects/my-project-id"), or a project
number (such as "projects/12345"). To know how to get organization ID, visit [here
](https://cloud.google.com/resource-manager/docs/creating-managing-
organization#retrieving_your_organization_id). To know how to get folder or project ID, visit [here
](https://cloud.google.com/resource-manager/docs/creating-managing-
folders#viewing_or_listing_folders_and_projects).
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. The relative name of the root asset. Only resources and IAM policies within the
* scope will be analyzed. This can only be an organization number (such as
* "organizations/123"), a folder number (such as "folders/123"), a project ID (such as
* "projects/my-project-id"), or a project number (such as "projects/12345"). To know how to
* get organization ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-
* managing-organization#retrieving_your_organization_id). To know how to get folder or
* project ID, visit [here ](https://cloud.google.com/resource-manager/docs/creating-managing-
* folders#viewing_or_listing_folders_and_projects).
*/
public AnalyzeIamPolicyLongrunning setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
@Override
public AnalyzeIamPolicyLongrunning set(String parameterName, Object value) {
return (AnalyzeIamPolicyLongrunning) super.set(parameterName, value);
}
}
/**
* Analyze moving a resource to a specified destination without kicking off the actual move. The
* analysis is best effort depending on the user's permissions of viewing different hierarchical
* policies and configurations. The policies and configuration are subject to change before the
* actual resource migration takes place.
*
* Create a request for the method "v1.analyzeMove".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link AnalyzeMove#execute()} method to invoke the remote operation.
*
* @param resource Required. Name of the resource to perform the analysis against. Only Google Cloud projects are
* supported as of today. Hence, this can only be a project ID (such as "projects/my-project-
* id") or a project number (such as "projects/12345").
* @return the request
*/
public AnalyzeMove analyzeMove(java.lang.String resource) throws java.io.IOException {
AnalyzeMove result = new AnalyzeMove(resource);
initialize(result);
return result;
}
public class AnalyzeMove extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+resource}:analyzeMove";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Analyze moving a resource to a specified destination without kicking off the actual move. The
* analysis is best effort depending on the user's permissions of viewing different hierarchical
* policies and configurations. The policies and configuration are subject to change before the
* actual resource migration takes place.
*
* Create a request for the method "v1.analyzeMove".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link AnalyzeMove#execute()} method to invoke the remote
* operation. {@link
* AnalyzeMove#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource Required. Name of the resource to perform the analysis against. Only Google Cloud projects are
* supported as of today. Hence, this can only be a project ID (such as "projects/my-project-
* id") or a project number (such as "projects/12345").
* @since 1.13
*/
protected AnalyzeMove(java.lang.String resource) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.AnalyzeMoveResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public AnalyzeMove set$Xgafv(java.lang.String $Xgafv) {
return (AnalyzeMove) super.set$Xgafv($Xgafv);
}
@Override
public AnalyzeMove setAccessToken(java.lang.String accessToken) {
return (AnalyzeMove) super.setAccessToken(accessToken);
}
@Override
public AnalyzeMove setAlt(java.lang.String alt) {
return (AnalyzeMove) super.setAlt(alt);
}
@Override
public AnalyzeMove setCallback(java.lang.String callback) {
return (AnalyzeMove) super.setCallback(callback);
}
@Override
public AnalyzeMove setFields(java.lang.String fields) {
return (AnalyzeMove) super.setFields(fields);
}
@Override
public AnalyzeMove setKey(java.lang.String key) {
return (AnalyzeMove) super.setKey(key);
}
@Override
public AnalyzeMove setOauthToken(java.lang.String oauthToken) {
return (AnalyzeMove) super.setOauthToken(oauthToken);
}
@Override
public AnalyzeMove setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AnalyzeMove) super.setPrettyPrint(prettyPrint);
}
@Override
public AnalyzeMove setQuotaUser(java.lang.String quotaUser) {
return (AnalyzeMove) super.setQuotaUser(quotaUser);
}
@Override
public AnalyzeMove setUploadType(java.lang.String uploadType) {
return (AnalyzeMove) super.setUploadType(uploadType);
}
@Override
public AnalyzeMove setUploadProtocol(java.lang.String uploadProtocol) {
return (AnalyzeMove) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the resource to perform the analysis against. Only Google Cloud projects
* are supported as of today. Hence, this can only be a project ID (such as "projects/my-
* project-id") or a project number (such as "projects/12345").
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** Required. Name of the resource to perform the analysis against. Only Google Cloud projects are
supported as of today. Hence, this can only be a project ID (such as "projects/my-project-id") or a
project number (such as "projects/12345").
*/
public java.lang.String getResource() {
return resource;
}
/**
* Required. Name of the resource to perform the analysis against. Only Google Cloud projects
* are supported as of today. Hence, this can only be a project ID (such as "projects/my-
* project-id") or a project number (such as "projects/12345").
*/
public AnalyzeMove setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Required. Name of the Google Cloud folder or organization to reparent the target resource.
* The analysis will be performed against hypothetically moving the resource to this specified
* desitination parent. This can only be a folder number (such as "folders/123") or an
* organization number (such as "organizations/123").
*/
@com.google.api.client.util.Key
private java.lang.String destinationParent;
/** Required. Name of the Google Cloud folder or organization to reparent the target resource. The
analysis will be performed against hypothetically moving the resource to this specified
desitination parent. This can only be a folder number (such as "folders/123") or an organization
number (such as "organizations/123").
*/
public java.lang.String getDestinationParent() {
return destinationParent;
}
/**
* Required. Name of the Google Cloud folder or organization to reparent the target resource.
* The analysis will be performed against hypothetically moving the resource to this specified
* desitination parent. This can only be a folder number (such as "folders/123") or an
* organization number (such as "organizations/123").
*/
public AnalyzeMove setDestinationParent(java.lang.String destinationParent) {
this.destinationParent = destinationParent;
return this;
}
/**
* Analysis view indicating what information should be included in the analysis response. If
* unspecified, the default view is FULL.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Analysis view indicating what information should be included in the analysis response. If
unspecified, the default view is FULL.
*/
public java.lang.String getView() {
return view;
}
/**
* Analysis view indicating what information should be included in the analysis response. If
* unspecified, the default view is FULL.
*/
public AnalyzeMove setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public AnalyzeMove set(String parameterName, Object value) {
return (AnalyzeMove) super.set(parameterName, value);
}
}
/**
* Analyzes organization policies under a scope.
*
* Create a request for the method "v1.analyzeOrgPolicies".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link AnalyzeOrgPolicies#execute()} method to invoke the remote operation.
*
* @param scope Required. The organization to scope the request. Only organization policies within the scope will be
* analyzed. * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
* @return the request
*/
public AnalyzeOrgPolicies analyzeOrgPolicies(java.lang.String scope) throws java.io.IOException {
AnalyzeOrgPolicies result = new AnalyzeOrgPolicies(scope);
initialize(result);
return result;
}
public class AnalyzeOrgPolicies extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}:analyzeOrgPolicies";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Analyzes organization policies under a scope.
*
* Create a request for the method "v1.analyzeOrgPolicies".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link AnalyzeOrgPolicies#execute()} method to invoke the remote
* operation. {@link AnalyzeOrgPolicies#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param scope Required. The organization to scope the request. Only organization policies within the scope will be
* analyzed. * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
* @since 1.13
*/
protected AnalyzeOrgPolicies(java.lang.String scope) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.AnalyzeOrgPoliciesResponse.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public AnalyzeOrgPolicies set$Xgafv(java.lang.String $Xgafv) {
return (AnalyzeOrgPolicies) super.set$Xgafv($Xgafv);
}
@Override
public AnalyzeOrgPolicies setAccessToken(java.lang.String accessToken) {
return (AnalyzeOrgPolicies) super.setAccessToken(accessToken);
}
@Override
public AnalyzeOrgPolicies setAlt(java.lang.String alt) {
return (AnalyzeOrgPolicies) super.setAlt(alt);
}
@Override
public AnalyzeOrgPolicies setCallback(java.lang.String callback) {
return (AnalyzeOrgPolicies) super.setCallback(callback);
}
@Override
public AnalyzeOrgPolicies setFields(java.lang.String fields) {
return (AnalyzeOrgPolicies) super.setFields(fields);
}
@Override
public AnalyzeOrgPolicies setKey(java.lang.String key) {
return (AnalyzeOrgPolicies) super.setKey(key);
}
@Override
public AnalyzeOrgPolicies setOauthToken(java.lang.String oauthToken) {
return (AnalyzeOrgPolicies) super.setOauthToken(oauthToken);
}
@Override
public AnalyzeOrgPolicies setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AnalyzeOrgPolicies) super.setPrettyPrint(prettyPrint);
}
@Override
public AnalyzeOrgPolicies setQuotaUser(java.lang.String quotaUser) {
return (AnalyzeOrgPolicies) super.setQuotaUser(quotaUser);
}
@Override
public AnalyzeOrgPolicies setUploadType(java.lang.String uploadType) {
return (AnalyzeOrgPolicies) super.setUploadType(uploadType);
}
@Override
public AnalyzeOrgPolicies setUploadProtocol(java.lang.String uploadProtocol) {
return (AnalyzeOrgPolicies) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The organization to scope the request. Only organization policies within the
* scope will be analyzed. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. The organization to scope the request. Only organization policies within the scope will
be analyzed. * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. The organization to scope the request. Only organization policies within the
* scope will be analyzed. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
*/
public AnalyzeOrgPolicies setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
/**
* Required. The name of the constraint to analyze organization policies for. The response
* only contains analyzed organization policies for the provided constraint.
*/
@com.google.api.client.util.Key
private java.lang.String constraint;
/** Required. The name of the constraint to analyze organization policies for. The response only
contains analyzed organization policies for the provided constraint.
*/
public java.lang.String getConstraint() {
return constraint;
}
/**
* Required. The name of the constraint to analyze organization policies for. The response
* only contains analyzed organization policies for the provided constraint.
*/
public AnalyzeOrgPolicies setConstraint(java.lang.String constraint) {
this.constraint = constraint;
return this;
}
/**
* The expression to filter AnalyzeOrgPoliciesResponse.org_policy_results. Filtering is
* currently available for bare literal values and the following fields: *
* consolidated_policy.attached_resource * consolidated_policy.rules.enforce When filtering by
* a specific field, the only supported operator is `=`. For example, filtering by
* consolidated_policy.attached_resource="//cloudresourcemanager.googleapis.com/folders/001"
* will return all the Organization Policy results attached to "folders/001".
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** The expression to filter AnalyzeOrgPoliciesResponse.org_policy_results. Filtering is currently
available for bare literal values and the following fields: * consolidated_policy.attached_resource
* consolidated_policy.rules.enforce When filtering by a specific field, the only supported operator
is `=`. For example, filtering by
consolidated_policy.attached_resource="//cloudresourcemanager.googleapis.com/folders/001" will
return all the Organization Policy results attached to "folders/001".
*/
public java.lang.String getFilter() {
return filter;
}
/**
* The expression to filter AnalyzeOrgPoliciesResponse.org_policy_results. Filtering is
* currently available for bare literal values and the following fields: *
* consolidated_policy.attached_resource * consolidated_policy.rules.enforce When filtering by
* a specific field, the only supported operator is `=`. For example, filtering by
* consolidated_policy.attached_resource="//cloudresourcemanager.googleapis.com/folders/001"
* will return all the Organization Policy results attached to "folders/001".
*/
public AnalyzeOrgPolicies setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of items to return per page. If unspecified,
* AnalyzeOrgPoliciesResponse.org_policy_results will contain 20 items with a maximum of 200.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return per page. If unspecified,
AnalyzeOrgPoliciesResponse.org_policy_results will contain 20 items with a maximum of 200.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return per page. If unspecified,
* AnalyzeOrgPoliciesResponse.org_policy_results will contain 20 items with a maximum of 200.
*/
public AnalyzeOrgPolicies setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The pagination token to retrieve the next page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The pagination token to retrieve the next page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The pagination token to retrieve the next page. */
public AnalyzeOrgPolicies setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public AnalyzeOrgPolicies set(String parameterName, Object value) {
return (AnalyzeOrgPolicies) super.set(parameterName, value);
}
}
/**
* Analyzes organization policies governed assets (Google Cloud resources or policies) under a
* scope. This RPC supports custom constraints and the following canned constraints: *
* constraints/ainotebooks.accessMode * constraints/ainotebooks.disableFileDownloads *
* constraints/ainotebooks.disableRootAccess * constraints/ainotebooks.disableTerminal *
* constraints/ainotebooks.environmentOptions * constraints/ainotebooks.requireAutoUpgradeSchedule *
* constraints/ainotebooks.restrictVpcNetworks * constraints/compute.disableGuestAttributesAccess *
* constraints/compute.disableInstanceDataAccessApis *
* constraints/compute.disableNestedVirtualization * constraints/compute.disableSerialPortAccess *
* constraints/compute.disableSerialPortLogging * constraints/compute.disableVpcExternalIpv6 *
* constraints/compute.requireOsLogin * constraints/compute.requireShieldedVm *
* constraints/compute.restrictLoadBalancerCreationForTypes *
* constraints/compute.restrictProtocolForwardingCreationForTypes *
* constraints/compute.restrictXpnProjectLienRemoval *
* constraints/compute.setNewProjectDefaultToZonalDNSOnly *
* constraints/compute.skipDefaultNetworkCreation * constraints/compute.trustedImageProjects *
* constraints/compute.vmCanIpForward * constraints/compute.vmExternalIpAccess *
* constraints/gcp.detailedAuditLoggingMode * constraints/gcp.resourceLocations *
* constraints/iam.allowedPolicyMemberDomains *
* constraints/iam.automaticIamGrantsForDefaultServiceAccounts *
* constraints/iam.disableServiceAccountCreation * constraints/iam.disableServiceAccountKeyCreation
* * constraints/iam.disableServiceAccountKeyUpload *
* constraints/iam.restrictCrossProjectServiceAccountLienRemoval *
* constraints/iam.serviceAccountKeyExpiryHours * constraints/resourcemanager.accessBoundaries *
* constraints/resourcemanager.allowedExportDestinations *
* constraints/sql.restrictAuthorizedNetworks *
* constraints/sql.restrictNoncompliantDiagnosticDataAccess *
* constraints/sql.restrictNoncompliantResourceCreation * constraints/sql.restrictPublicIp *
* constraints/storage.publicAccessPrevention * constraints/storage.restrictAuthTypes *
* constraints/storage.uniformBucketLevelAccess This RPC only returns either resources of types
* [supported by search APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types)
* or IAM policies.
*
* Create a request for the method "v1.analyzeOrgPolicyGovernedAssets".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link AnalyzeOrgPolicyGovernedAssets#execute()} method to invoke the remote
* operation.
*
* @param scope Required. The organization to scope the request. Only organization policies within the scope will be
* analyzed. The output assets will also be limited to the ones governed by those in-scope
* organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
* @return the request
*/
public AnalyzeOrgPolicyGovernedAssets analyzeOrgPolicyGovernedAssets(java.lang.String scope) throws java.io.IOException {
AnalyzeOrgPolicyGovernedAssets result = new AnalyzeOrgPolicyGovernedAssets(scope);
initialize(result);
return result;
}
public class AnalyzeOrgPolicyGovernedAssets extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}:analyzeOrgPolicyGovernedAssets";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Analyzes organization policies governed assets (Google Cloud resources or policies) under a
* scope. This RPC supports custom constraints and the following canned constraints: *
* constraints/ainotebooks.accessMode * constraints/ainotebooks.disableFileDownloads *
* constraints/ainotebooks.disableRootAccess * constraints/ainotebooks.disableTerminal *
* constraints/ainotebooks.environmentOptions * constraints/ainotebooks.requireAutoUpgradeSchedule
* * constraints/ainotebooks.restrictVpcNetworks *
* constraints/compute.disableGuestAttributesAccess *
* constraints/compute.disableInstanceDataAccessApis *
* constraints/compute.disableNestedVirtualization * constraints/compute.disableSerialPortAccess *
* constraints/compute.disableSerialPortLogging * constraints/compute.disableVpcExternalIpv6 *
* constraints/compute.requireOsLogin * constraints/compute.requireShieldedVm *
* constraints/compute.restrictLoadBalancerCreationForTypes *
* constraints/compute.restrictProtocolForwardingCreationForTypes *
* constraints/compute.restrictXpnProjectLienRemoval *
* constraints/compute.setNewProjectDefaultToZonalDNSOnly *
* constraints/compute.skipDefaultNetworkCreation * constraints/compute.trustedImageProjects *
* constraints/compute.vmCanIpForward * constraints/compute.vmExternalIpAccess *
* constraints/gcp.detailedAuditLoggingMode * constraints/gcp.resourceLocations *
* constraints/iam.allowedPolicyMemberDomains *
* constraints/iam.automaticIamGrantsForDefaultServiceAccounts *
* constraints/iam.disableServiceAccountCreation *
* constraints/iam.disableServiceAccountKeyCreation *
* constraints/iam.disableServiceAccountKeyUpload *
* constraints/iam.restrictCrossProjectServiceAccountLienRemoval *
* constraints/iam.serviceAccountKeyExpiryHours * constraints/resourcemanager.accessBoundaries *
* constraints/resourcemanager.allowedExportDestinations *
* constraints/sql.restrictAuthorizedNetworks *
* constraints/sql.restrictNoncompliantDiagnosticDataAccess *
* constraints/sql.restrictNoncompliantResourceCreation * constraints/sql.restrictPublicIp *
* constraints/storage.publicAccessPrevention * constraints/storage.restrictAuthTypes *
* constraints/storage.uniformBucketLevelAccess This RPC only returns either resources of types
* [supported by search APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types)
* or IAM policies.
*
* Create a request for the method "v1.analyzeOrgPolicyGovernedAssets".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link AnalyzeOrgPolicyGovernedAssets#execute()} method to invoke
* the remote operation. {@link AnalyzeOrgPolicyGovernedAssets#initialize(com.google.api.clien
* t.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param scope Required. The organization to scope the request. Only organization policies within the scope will be
* analyzed. The output assets will also be limited to the ones governed by those in-scope
* organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
* @since 1.13
*/
protected AnalyzeOrgPolicyGovernedAssets(java.lang.String scope) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.AnalyzeOrgPolicyGovernedAssetsResponse.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public AnalyzeOrgPolicyGovernedAssets set$Xgafv(java.lang.String $Xgafv) {
return (AnalyzeOrgPolicyGovernedAssets) super.set$Xgafv($Xgafv);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setAccessToken(java.lang.String accessToken) {
return (AnalyzeOrgPolicyGovernedAssets) super.setAccessToken(accessToken);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setAlt(java.lang.String alt) {
return (AnalyzeOrgPolicyGovernedAssets) super.setAlt(alt);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setCallback(java.lang.String callback) {
return (AnalyzeOrgPolicyGovernedAssets) super.setCallback(callback);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setFields(java.lang.String fields) {
return (AnalyzeOrgPolicyGovernedAssets) super.setFields(fields);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setKey(java.lang.String key) {
return (AnalyzeOrgPolicyGovernedAssets) super.setKey(key);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setOauthToken(java.lang.String oauthToken) {
return (AnalyzeOrgPolicyGovernedAssets) super.setOauthToken(oauthToken);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AnalyzeOrgPolicyGovernedAssets) super.setPrettyPrint(prettyPrint);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setQuotaUser(java.lang.String quotaUser) {
return (AnalyzeOrgPolicyGovernedAssets) super.setQuotaUser(quotaUser);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setUploadType(java.lang.String uploadType) {
return (AnalyzeOrgPolicyGovernedAssets) super.setUploadType(uploadType);
}
@Override
public AnalyzeOrgPolicyGovernedAssets setUploadProtocol(java.lang.String uploadProtocol) {
return (AnalyzeOrgPolicyGovernedAssets) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The organization to scope the request. Only organization policies within the
* scope will be analyzed. The output assets will also be limited to the ones governed by
* those in-scope organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. The organization to scope the request. Only organization policies within the scope will
be analyzed. The output assets will also be limited to the ones governed by those in-scope
organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. The organization to scope the request. Only organization policies within the
* scope will be analyzed. The output assets will also be limited to the ones governed by
* those in-scope organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
*/
public AnalyzeOrgPolicyGovernedAssets setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
/**
* Required. The name of the constraint to analyze governed assets for. The analysis only
* contains analyzed organization policies for the provided constraint.
*/
@com.google.api.client.util.Key
private java.lang.String constraint;
/** Required. The name of the constraint to analyze governed assets for. The analysis only contains
analyzed organization policies for the provided constraint.
*/
public java.lang.String getConstraint() {
return constraint;
}
/**
* Required. The name of the constraint to analyze governed assets for. The analysis only
* contains analyzed organization policies for the provided constraint.
*/
public AnalyzeOrgPolicyGovernedAssets setConstraint(java.lang.String constraint) {
this.constraint = constraint;
return this;
}
/**
* The expression to filter AnalyzeOrgPolicyGovernedAssetsResponse.governed_assets. For
* governed resources, filtering is currently available for bare literal values and the
* following fields: * governed_resource.project * governed_resource.folders *
* consolidated_policy.rules.enforce When filtering by `governed_resource.project` or
* `consolidated_policy.rules.enforce`, the only supported operator is `=`. When filtering by
* `governed_resource.folders`, the supported operators are `=` and `:`. For example,
* filtering by `governed_resource.project="projects/12345678"` will return all the governed
* resources under "projects/12345678", including the project itself if applicable. For
* governed IAM policies, filtering is currently available for bare literal values and the
* following fields: * governed_iam_policy.project * governed_iam_policy.folders *
* consolidated_policy.rules.enforce When filtering by `governed_iam_policy.project` or
* `consolidated_policy.rules.enforce`, the only supported operator is `=`. When filtering by
* `governed_iam_policy.folders`, the supported operators are `=` and `:`. For example,
* filtering by `governed_iam_policy.folders:"folders/12345678"` will return all the governed
* IAM policies under "folders/001".
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** The expression to filter AnalyzeOrgPolicyGovernedAssetsResponse.governed_assets. For governed
resources, filtering is currently available for bare literal values and the following fields: *
governed_resource.project * governed_resource.folders * consolidated_policy.rules.enforce When
filtering by `governed_resource.project` or `consolidated_policy.rules.enforce`, the only supported
operator is `=`. When filtering by `governed_resource.folders`, the supported operators are `=` and
`:`. For example, filtering by `governed_resource.project="projects/12345678"` will return all the
governed resources under "projects/12345678", including the project itself if applicable. For
governed IAM policies, filtering is currently available for bare literal values and the following
fields: * governed_iam_policy.project * governed_iam_policy.folders *
consolidated_policy.rules.enforce When filtering by `governed_iam_policy.project` or
`consolidated_policy.rules.enforce`, the only supported operator is `=`. When filtering by
`governed_iam_policy.folders`, the supported operators are `=` and `:`. For example, filtering by
`governed_iam_policy.folders:"folders/12345678"` will return all the governed IAM policies under
"folders/001".
*/
public java.lang.String getFilter() {
return filter;
}
/**
* The expression to filter AnalyzeOrgPolicyGovernedAssetsResponse.governed_assets. For
* governed resources, filtering is currently available for bare literal values and the
* following fields: * governed_resource.project * governed_resource.folders *
* consolidated_policy.rules.enforce When filtering by `governed_resource.project` or
* `consolidated_policy.rules.enforce`, the only supported operator is `=`. When filtering by
* `governed_resource.folders`, the supported operators are `=` and `:`. For example,
* filtering by `governed_resource.project="projects/12345678"` will return all the governed
* resources under "projects/12345678", including the project itself if applicable. For
* governed IAM policies, filtering is currently available for bare literal values and the
* following fields: * governed_iam_policy.project * governed_iam_policy.folders *
* consolidated_policy.rules.enforce When filtering by `governed_iam_policy.project` or
* `consolidated_policy.rules.enforce`, the only supported operator is `=`. When filtering by
* `governed_iam_policy.folders`, the supported operators are `=` and `:`. For example,
* filtering by `governed_iam_policy.folders:"folders/12345678"` will return all the governed
* IAM policies under "folders/001".
*/
public AnalyzeOrgPolicyGovernedAssets setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of items to return per page. If unspecified,
* AnalyzeOrgPolicyGovernedAssetsResponse.governed_assets will contain 100 items with a
* maximum of 200.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return per page. If unspecified,
AnalyzeOrgPolicyGovernedAssetsResponse.governed_assets will contain 100 items with a maximum of
200.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return per page. If unspecified,
* AnalyzeOrgPolicyGovernedAssetsResponse.governed_assets will contain 100 items with a
* maximum of 200.
*/
public AnalyzeOrgPolicyGovernedAssets setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The pagination token to retrieve the next page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The pagination token to retrieve the next page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The pagination token to retrieve the next page. */
public AnalyzeOrgPolicyGovernedAssets setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public AnalyzeOrgPolicyGovernedAssets set(String parameterName, Object value) {
return (AnalyzeOrgPolicyGovernedAssets) super.set(parameterName, value);
}
}
/**
* Analyzes organization policies governed containers (projects, folders or organization) under a
* scope.
*
* Create a request for the method "v1.analyzeOrgPolicyGovernedContainers".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link AnalyzeOrgPolicyGovernedContainers#execute()} method to invoke the
* remote operation.
*
* @param scope Required. The organization to scope the request. Only organization policies within the scope will be
* analyzed. The output containers will also be limited to the ones governed by those in-
* scope organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
* @return the request
*/
public AnalyzeOrgPolicyGovernedContainers analyzeOrgPolicyGovernedContainers(java.lang.String scope) throws java.io.IOException {
AnalyzeOrgPolicyGovernedContainers result = new AnalyzeOrgPolicyGovernedContainers(scope);
initialize(result);
return result;
}
public class AnalyzeOrgPolicyGovernedContainers extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}:analyzeOrgPolicyGovernedContainers";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Analyzes organization policies governed containers (projects, folders or organization) under a
* scope.
*
* Create a request for the method "v1.analyzeOrgPolicyGovernedContainers".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link AnalyzeOrgPolicyGovernedContainers#execute()} method to
* invoke the remote operation. {@link AnalyzeOrgPolicyGovernedContainers#initialize(com.googl
* e.api.client.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize
* this instance immediately after invoking the constructor.
*
* @param scope Required. The organization to scope the request. Only organization policies within the scope will be
* analyzed. The output containers will also be limited to the ones governed by those in-
* scope organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
* @since 1.13
*/
protected AnalyzeOrgPolicyGovernedContainers(java.lang.String scope) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.AnalyzeOrgPolicyGovernedContainersResponse.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public AnalyzeOrgPolicyGovernedContainers set$Xgafv(java.lang.String $Xgafv) {
return (AnalyzeOrgPolicyGovernedContainers) super.set$Xgafv($Xgafv);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setAccessToken(java.lang.String accessToken) {
return (AnalyzeOrgPolicyGovernedContainers) super.setAccessToken(accessToken);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setAlt(java.lang.String alt) {
return (AnalyzeOrgPolicyGovernedContainers) super.setAlt(alt);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setCallback(java.lang.String callback) {
return (AnalyzeOrgPolicyGovernedContainers) super.setCallback(callback);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setFields(java.lang.String fields) {
return (AnalyzeOrgPolicyGovernedContainers) super.setFields(fields);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setKey(java.lang.String key) {
return (AnalyzeOrgPolicyGovernedContainers) super.setKey(key);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setOauthToken(java.lang.String oauthToken) {
return (AnalyzeOrgPolicyGovernedContainers) super.setOauthToken(oauthToken);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AnalyzeOrgPolicyGovernedContainers) super.setPrettyPrint(prettyPrint);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setQuotaUser(java.lang.String quotaUser) {
return (AnalyzeOrgPolicyGovernedContainers) super.setQuotaUser(quotaUser);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setUploadType(java.lang.String uploadType) {
return (AnalyzeOrgPolicyGovernedContainers) super.setUploadType(uploadType);
}
@Override
public AnalyzeOrgPolicyGovernedContainers setUploadProtocol(java.lang.String uploadProtocol) {
return (AnalyzeOrgPolicyGovernedContainers) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The organization to scope the request. Only organization policies within the
* scope will be analyzed. The output containers will also be limited to the ones governed by
* those in-scope organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. The organization to scope the request. Only organization policies within the scope will
be analyzed. The output containers will also be limited to the ones governed by those in-scope
organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. The organization to scope the request. Only organization policies within the
* scope will be analyzed. The output containers will also be limited to the ones governed by
* those in-scope organization policies. * organizations/{ORGANIZATION_NUMBER} (e.g.,
* "organizations/123456")
*/
public AnalyzeOrgPolicyGovernedContainers setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
/**
* Required. The name of the constraint to analyze governed containers for. The analysis only
* contains organization policies for the provided constraint.
*/
@com.google.api.client.util.Key
private java.lang.String constraint;
/** Required. The name of the constraint to analyze governed containers for. The analysis only contains
organization policies for the provided constraint.
*/
public java.lang.String getConstraint() {
return constraint;
}
/**
* Required. The name of the constraint to analyze governed containers for. The analysis only
* contains organization policies for the provided constraint.
*/
public AnalyzeOrgPolicyGovernedContainers setConstraint(java.lang.String constraint) {
this.constraint = constraint;
return this;
}
/**
* The expression to filter AnalyzeOrgPolicyGovernedContainersResponse.governed_containers.
* Filtering is currently available for bare literal values and the following fields: * parent
* * consolidated_policy.rules.enforce When filtering by a specific field, the only supported
* operator is `=`. For example, filtering by
* parent="//cloudresourcemanager.googleapis.com/folders/001" will return all the containers
* under "folders/001".
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** The expression to filter AnalyzeOrgPolicyGovernedContainersResponse.governed_containers. Filtering
is currently available for bare literal values and the following fields: * parent *
consolidated_policy.rules.enforce When filtering by a specific field, the only supported operator
is `=`. For example, filtering by parent="//cloudresourcemanager.googleapis.com/folders/001" will
return all the containers under "folders/001".
*/
public java.lang.String getFilter() {
return filter;
}
/**
* The expression to filter AnalyzeOrgPolicyGovernedContainersResponse.governed_containers.
* Filtering is currently available for bare literal values and the following fields: * parent
* * consolidated_policy.rules.enforce When filtering by a specific field, the only supported
* operator is `=`. For example, filtering by
* parent="//cloudresourcemanager.googleapis.com/folders/001" will return all the containers
* under "folders/001".
*/
public AnalyzeOrgPolicyGovernedContainers setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of items to return per page. If unspecified,
* AnalyzeOrgPolicyGovernedContainersResponse.governed_containers will contain 100 items with
* a maximum of 200.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return per page. If unspecified,
AnalyzeOrgPolicyGovernedContainersResponse.governed_containers will contain 100 items with a
maximum of 200.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return per page. If unspecified,
* AnalyzeOrgPolicyGovernedContainersResponse.governed_containers will contain 100 items with
* a maximum of 200.
*/
public AnalyzeOrgPolicyGovernedContainers setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The pagination token to retrieve the next page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The pagination token to retrieve the next page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The pagination token to retrieve the next page. */
public AnalyzeOrgPolicyGovernedContainers setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public AnalyzeOrgPolicyGovernedContainers set(String parameterName, Object value) {
return (AnalyzeOrgPolicyGovernedContainers) super.set(parameterName, value);
}
}
/**
* Batch gets the update history of assets that overlap a time window. For IAM_POLICY content, this
* API outputs history when the asset and its attached IAM POLICY both exist. This can create gaps
* in the output history. Otherwise, this API outputs history with asset in both non-delete or
* deleted status. If a specified asset does not exist, this API returns an INVALID_ARGUMENT error.
*
* Create a request for the method "v1.batchGetAssetsHistory".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link BatchGetAssetsHistory#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The relative name of the root asset. It can only be an organization number (such as
* "organizations/123"), a project ID (such as "projects/my-project-id")", or a project
* number (such as "projects/12345").
* @return the request
*/
public BatchGetAssetsHistory batchGetAssetsHistory(java.lang.String parent) throws java.io.IOException {
BatchGetAssetsHistory result = new BatchGetAssetsHistory(parent);
initialize(result);
return result;
}
public class BatchGetAssetsHistory extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}:batchGetAssetsHistory";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Batch gets the update history of assets that overlap a time window. For IAM_POLICY content,
* this API outputs history when the asset and its attached IAM POLICY both exist. This can create
* gaps in the output history. Otherwise, this API outputs history with asset in both non-delete
* or deleted status. If a specified asset does not exist, this API returns an INVALID_ARGUMENT
* error.
*
* Create a request for the method "v1.batchGetAssetsHistory".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link BatchGetAssetsHistory#execute()} method to invoke the
* remote operation. {@link BatchGetAssetsHistory#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent Required. The relative name of the root asset. It can only be an organization number (such as
* "organizations/123"), a project ID (such as "projects/my-project-id")", or a project
* number (such as "projects/12345").
* @since 1.13
*/
protected BatchGetAssetsHistory(java.lang.String parent) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.BatchGetAssetsHistoryResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public BatchGetAssetsHistory set$Xgafv(java.lang.String $Xgafv) {
return (BatchGetAssetsHistory) super.set$Xgafv($Xgafv);
}
@Override
public BatchGetAssetsHistory setAccessToken(java.lang.String accessToken) {
return (BatchGetAssetsHistory) super.setAccessToken(accessToken);
}
@Override
public BatchGetAssetsHistory setAlt(java.lang.String alt) {
return (BatchGetAssetsHistory) super.setAlt(alt);
}
@Override
public BatchGetAssetsHistory setCallback(java.lang.String callback) {
return (BatchGetAssetsHistory) super.setCallback(callback);
}
@Override
public BatchGetAssetsHistory setFields(java.lang.String fields) {
return (BatchGetAssetsHistory) super.setFields(fields);
}
@Override
public BatchGetAssetsHistory setKey(java.lang.String key) {
return (BatchGetAssetsHistory) super.setKey(key);
}
@Override
public BatchGetAssetsHistory setOauthToken(java.lang.String oauthToken) {
return (BatchGetAssetsHistory) super.setOauthToken(oauthToken);
}
@Override
public BatchGetAssetsHistory setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchGetAssetsHistory) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchGetAssetsHistory setQuotaUser(java.lang.String quotaUser) {
return (BatchGetAssetsHistory) super.setQuotaUser(quotaUser);
}
@Override
public BatchGetAssetsHistory setUploadType(java.lang.String uploadType) {
return (BatchGetAssetsHistory) super.setUploadType(uploadType);
}
@Override
public BatchGetAssetsHistory setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchGetAssetsHistory) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The relative name of the root asset. It can only be an organization number (such
* as "organizations/123"), a project ID (such as "projects/my-project-id")", or a project
* number (such as "projects/12345").
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The relative name of the root asset. It can only be an organization number (such as
"organizations/123"), a project ID (such as "projects/my-project-id")", or a project number (such
as "projects/12345").
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The relative name of the root asset. It can only be an organization number (such
* as "organizations/123"), a project ID (such as "projects/my-project-id")", or a project
* number (such as "projects/12345").
*/
public BatchGetAssetsHistory setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A list of the full names of the assets. See: https://cloud.google.com/asset-
* inventory/docs/resource-name-format Example:
* `//compute.googleapis.com/projects/my_project_123/zones/zone1/instances/instance1`. The
* request becomes a no-op if the asset name list is empty, and the max size of the asset name
* list is 100 in one request.
*/
@com.google.api.client.util.Key
private java.util.List assetNames;
/** A list of the full names of the assets. See: https://cloud.google.com/asset-
inventory/docs/resource-name-format Example:
`//compute.googleapis.com/projects/my_project_123/zones/zone1/instances/instance1`. The request
becomes a no-op if the asset name list is empty, and the max size of the asset name list is 100 in
one request.
*/
public java.util.List getAssetNames() {
return assetNames;
}
/**
* A list of the full names of the assets. See: https://cloud.google.com/asset-
* inventory/docs/resource-name-format Example:
* `//compute.googleapis.com/projects/my_project_123/zones/zone1/instances/instance1`. The
* request becomes a no-op if the asset name list is empty, and the max size of the asset name
* list is 100 in one request.
*/
public BatchGetAssetsHistory setAssetNames(java.util.List assetNames) {
this.assetNames = assetNames;
return this;
}
/** Optional. The content type. */
@com.google.api.client.util.Key
private java.lang.String contentType;
/** Optional. The content type.
*/
public java.lang.String getContentType() {
return contentType;
}
/** Optional. The content type. */
public BatchGetAssetsHistory setContentType(java.lang.String contentType) {
this.contentType = contentType;
return this;
}
/**
* End time of the time window (inclusive). If not specified, the current timestamp is used
* instead.
*/
@com.google.api.client.util.Key("readTimeWindow.endTime")
private String readTimeWindowEndTime;
/** End time of the time window (inclusive). If not specified, the current timestamp is used instead.
*/
public String getReadTimeWindowEndTime() {
return readTimeWindowEndTime;
}
/**
* End time of the time window (inclusive). If not specified, the current timestamp is used
* instead.
*/
public BatchGetAssetsHistory setReadTimeWindowEndTime(String readTimeWindowEndTime) {
this.readTimeWindowEndTime = readTimeWindowEndTime;
return this;
}
/** Start time of the time window (exclusive). */
@com.google.api.client.util.Key("readTimeWindow.startTime")
private String readTimeWindowStartTime;
/** Start time of the time window (exclusive).
*/
public String getReadTimeWindowStartTime() {
return readTimeWindowStartTime;
}
/** Start time of the time window (exclusive). */
public BatchGetAssetsHistory setReadTimeWindowStartTime(String readTimeWindowStartTime) {
this.readTimeWindowStartTime = readTimeWindowStartTime;
return this;
}
/**
* Optional. A list of relationship types to output, for example: `INSTANCE_TO_INSTANCEGROUP`.
* This field should only be specified if content_type=RELATIONSHIP. * If specified: it
* outputs specified relationships' history on the [asset_names]. It returns an error if any
* of the [relationship_types] doesn't belong to the supported relationship types of the
* [asset_names] or if any of the [asset_names]'s types doesn't belong to the source types of
* the [relationship_types]. * Otherwise: it outputs the supported relationships' history on
* the [asset_names] or returns an error if any of the [asset_names]'s types has no
* relationship support. See [Introduction to Cloud Asset
* Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset
* types and relationship types.
*/
@com.google.api.client.util.Key
private java.util.List relationshipTypes;
/** Optional. A list of relationship types to output, for example: `INSTANCE_TO_INSTANCEGROUP`. This
field should only be specified if content_type=RELATIONSHIP. * If specified: it outputs specified
relationships' history on the [asset_names]. It returns an error if any of the [relationship_types]
doesn't belong to the supported relationship types of the [asset_names] or if any of the
[asset_names]'s types doesn't belong to the source types of the [relationship_types]. * Otherwise:
it outputs the supported relationships' history on the [asset_names] or returns an error if any of
the [asset_names]'s types has no relationship support. See [Introduction to Cloud Asset
Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset types
and relationship types.
*/
public java.util.List getRelationshipTypes() {
return relationshipTypes;
}
/**
* Optional. A list of relationship types to output, for example: `INSTANCE_TO_INSTANCEGROUP`.
* This field should only be specified if content_type=RELATIONSHIP. * If specified: it
* outputs specified relationships' history on the [asset_names]. It returns an error if any
* of the [relationship_types] doesn't belong to the supported relationship types of the
* [asset_names] or if any of the [asset_names]'s types doesn't belong to the source types of
* the [relationship_types]. * Otherwise: it outputs the supported relationships' history on
* the [asset_names] or returns an error if any of the [asset_names]'s types has no
* relationship support. See [Introduction to Cloud Asset
* Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset
* types and relationship types.
*/
public BatchGetAssetsHistory setRelationshipTypes(java.util.List relationshipTypes) {
this.relationshipTypes = relationshipTypes;
return this;
}
@Override
public BatchGetAssetsHistory set(String parameterName, Object value) {
return (BatchGetAssetsHistory) super.set(parameterName, value);
}
}
/**
* Exports assets with time and resource types to a given Cloud Storage location/BigQuery table. For
* Cloud Storage location destinations, the output format is newline-delimited JSON. Each line
* represents a google.cloud.asset.v1.Asset in the JSON format; for BigQuery table destinations, the
* output table stores the fields in asset Protobuf as columns. This API implements the
* google.longrunning.Operation API, which allows you to keep track of the export. We recommend
* intervals of at least 2 seconds with exponential retry to poll the export operation result. For
* regular-size resource parent, the export operation usually finishes within 5 minutes.
*
* Create a request for the method "v1.exportAssets".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link ExportAssets#execute()} method to invoke the remote operation.
*
* @param parent Required. The relative name of the root asset. This can only be an organization number (such as
* "organizations/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345"), or a folder number (such as "folders/123").
* @param content the {@link com.google.api.services.cloudasset.v1.model.ExportAssetsRequest}
* @return the request
*/
public ExportAssets exportAssets(java.lang.String parent, com.google.api.services.cloudasset.v1.model.ExportAssetsRequest content) throws java.io.IOException {
ExportAssets result = new ExportAssets(parent, content);
initialize(result);
return result;
}
public class ExportAssets extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}:exportAssets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Exports assets with time and resource types to a given Cloud Storage location/BigQuery table.
* For Cloud Storage location destinations, the output format is newline-delimited JSON. Each line
* represents a google.cloud.asset.v1.Asset in the JSON format; for BigQuery table destinations,
* the output table stores the fields in asset Protobuf as columns. This API implements the
* google.longrunning.Operation API, which allows you to keep track of the export. We recommend
* intervals of at least 2 seconds with exponential retry to poll the export operation result. For
* regular-size resource parent, the export operation usually finishes within 5 minutes.
*
* Create a request for the method "v1.exportAssets".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link ExportAssets#execute()} method to invoke the remote
* operation. {@link
* ExportAssets#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The relative name of the root asset. This can only be an organization number (such as
* "organizations/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345"), or a folder number (such as "folders/123").
* @param content the {@link com.google.api.services.cloudasset.v1.model.ExportAssetsRequest}
* @since 1.13
*/
protected ExportAssets(java.lang.String parent, com.google.api.services.cloudasset.v1.model.ExportAssetsRequest content) {
super(CloudAsset.this, "POST", REST_PATH, content, com.google.api.services.cloudasset.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public ExportAssets set$Xgafv(java.lang.String $Xgafv) {
return (ExportAssets) super.set$Xgafv($Xgafv);
}
@Override
public ExportAssets setAccessToken(java.lang.String accessToken) {
return (ExportAssets) super.setAccessToken(accessToken);
}
@Override
public ExportAssets setAlt(java.lang.String alt) {
return (ExportAssets) super.setAlt(alt);
}
@Override
public ExportAssets setCallback(java.lang.String callback) {
return (ExportAssets) super.setCallback(callback);
}
@Override
public ExportAssets setFields(java.lang.String fields) {
return (ExportAssets) super.setFields(fields);
}
@Override
public ExportAssets setKey(java.lang.String key) {
return (ExportAssets) super.setKey(key);
}
@Override
public ExportAssets setOauthToken(java.lang.String oauthToken) {
return (ExportAssets) super.setOauthToken(oauthToken);
}
@Override
public ExportAssets setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportAssets) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportAssets setQuotaUser(java.lang.String quotaUser) {
return (ExportAssets) super.setQuotaUser(quotaUser);
}
@Override
public ExportAssets setUploadType(java.lang.String uploadType) {
return (ExportAssets) super.setUploadType(uploadType);
}
@Override
public ExportAssets setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportAssets) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The relative name of the root asset. This can only be an organization number
* (such as "organizations/123"), a project ID (such as "projects/my-project-id"), or a
* project number (such as "projects/12345"), or a folder number (such as "folders/123").
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The relative name of the root asset. This can only be an organization number (such as
"organizations/123"), a project ID (such as "projects/my-project-id"), or a project number (such as
"projects/12345"), or a folder number (such as "folders/123").
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The relative name of the root asset. This can only be an organization number
* (such as "organizations/123"), a project ID (such as "projects/my-project-id"), or a
* project number (such as "projects/12345"), or a folder number (such as "folders/123").
*/
public ExportAssets setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public ExportAssets set(String parameterName, Object value) {
return (ExportAssets) super.set(parameterName, value);
}
}
/**
* Issue a job that queries assets using a SQL statement compatible with [BigQuery
* SQL](https://cloud.google.com/bigquery/docs/introduction-sql). If the query execution finishes
* within timeout and there's no pagination, the full query results will be returned in the
* `QueryAssetsResponse`. Otherwise, full query results can be obtained by issuing extra requests
* with the `job_reference` from the a previous `QueryAssets` call. Note, the query result has
* approximately 10 GB limitation enforced by
* [BigQuery](https://cloud.google.com/bigquery/docs/best-practices-performance-output). Queries
* return larger results will result in errors.
*
* Create a request for the method "v1.queryAssets".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link QueryAssets#execute()} method to invoke the remote operation.
*
* @param parent Required. The relative name of the root asset. This can only be an organization number (such as
* "organizations/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345"), or a folder number (such as "folders/123"). Only assets
* belonging to the `parent` will be returned.
* @param content the {@link com.google.api.services.cloudasset.v1.model.QueryAssetsRequest}
* @return the request
*/
public QueryAssets queryAssets(java.lang.String parent, com.google.api.services.cloudasset.v1.model.QueryAssetsRequest content) throws java.io.IOException {
QueryAssets result = new QueryAssets(parent, content);
initialize(result);
return result;
}
public class QueryAssets extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+parent}:queryAssets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Issue a job that queries assets using a SQL statement compatible with [BigQuery
* SQL](https://cloud.google.com/bigquery/docs/introduction-sql). If the query execution finishes
* within timeout and there's no pagination, the full query results will be returned in the
* `QueryAssetsResponse`. Otherwise, full query results can be obtained by issuing extra requests
* with the `job_reference` from the a previous `QueryAssets` call. Note, the query result has
* approximately 10 GB limitation enforced by
* [BigQuery](https://cloud.google.com/bigquery/docs/best-practices-performance-output). Queries
* return larger results will result in errors.
*
* Create a request for the method "v1.queryAssets".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link QueryAssets#execute()} method to invoke the remote
* operation. {@link
* QueryAssets#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The relative name of the root asset. This can only be an organization number (such as
* "organizations/123"), a project ID (such as "projects/my-project-id"), or a project number
* (such as "projects/12345"), or a folder number (such as "folders/123"). Only assets
* belonging to the `parent` will be returned.
* @param content the {@link com.google.api.services.cloudasset.v1.model.QueryAssetsRequest}
* @since 1.13
*/
protected QueryAssets(java.lang.String parent, com.google.api.services.cloudasset.v1.model.QueryAssetsRequest content) {
super(CloudAsset.this, "POST", REST_PATH, content, com.google.api.services.cloudasset.v1.model.QueryAssetsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public QueryAssets set$Xgafv(java.lang.String $Xgafv) {
return (QueryAssets) super.set$Xgafv($Xgafv);
}
@Override
public QueryAssets setAccessToken(java.lang.String accessToken) {
return (QueryAssets) super.setAccessToken(accessToken);
}
@Override
public QueryAssets setAlt(java.lang.String alt) {
return (QueryAssets) super.setAlt(alt);
}
@Override
public QueryAssets setCallback(java.lang.String callback) {
return (QueryAssets) super.setCallback(callback);
}
@Override
public QueryAssets setFields(java.lang.String fields) {
return (QueryAssets) super.setFields(fields);
}
@Override
public QueryAssets setKey(java.lang.String key) {
return (QueryAssets) super.setKey(key);
}
@Override
public QueryAssets setOauthToken(java.lang.String oauthToken) {
return (QueryAssets) super.setOauthToken(oauthToken);
}
@Override
public QueryAssets setPrettyPrint(java.lang.Boolean prettyPrint) {
return (QueryAssets) super.setPrettyPrint(prettyPrint);
}
@Override
public QueryAssets setQuotaUser(java.lang.String quotaUser) {
return (QueryAssets) super.setQuotaUser(quotaUser);
}
@Override
public QueryAssets setUploadType(java.lang.String uploadType) {
return (QueryAssets) super.setUploadType(uploadType);
}
@Override
public QueryAssets setUploadProtocol(java.lang.String uploadProtocol) {
return (QueryAssets) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The relative name of the root asset. This can only be an organization number
* (such as "organizations/123"), a project ID (such as "projects/my-project-id"), or a
* project number (such as "projects/12345"), or a folder number (such as "folders/123"). Only
* assets belonging to the `parent` will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The relative name of the root asset. This can only be an organization number (such as
"organizations/123"), a project ID (such as "projects/my-project-id"), or a project number (such as
"projects/12345"), or a folder number (such as "folders/123"). Only assets belonging to the
`parent` will be returned.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The relative name of the root asset. This can only be an organization number
* (such as "organizations/123"), a project ID (such as "projects/my-project-id"), or a
* project number (such as "projects/12345"), or a folder number (such as "folders/123"). Only
* assets belonging to the `parent` will be returned.
*/
public QueryAssets setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public QueryAssets set(String parameterName, Object value) {
return (QueryAssets) super.set(parameterName, value);
}
}
/**
* Searches all IAM policies within the specified scope, such as a project, folder, or organization.
* The caller must be granted the `cloudasset.assets.searchAllIamPolicies` permission on the desired
* scope, otherwise the request will be rejected.
*
* Create a request for the method "v1.searchAllIamPolicies".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link SearchAllIamPolicies#execute()} method to invoke the remote
* operation.
*
* @param scope Required. A scope can be a project, a folder, or an organization. The search is limited to the IAM
* policies within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllIamPolicies`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
* @return the request
*/
public SearchAllIamPolicies searchAllIamPolicies(java.lang.String scope) throws java.io.IOException {
SearchAllIamPolicies result = new SearchAllIamPolicies(scope);
initialize(result);
return result;
}
public class SearchAllIamPolicies extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}:searchAllIamPolicies";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Searches all IAM policies within the specified scope, such as a project, folder, or
* organization. The caller must be granted the `cloudasset.assets.searchAllIamPolicies`
* permission on the desired scope, otherwise the request will be rejected.
*
* Create a request for the method "v1.searchAllIamPolicies".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link SearchAllIamPolicies#execute()} method to invoke the
* remote operation. {@link SearchAllIamPolicies#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param scope Required. A scope can be a project, a folder, or an organization. The search is limited to the IAM
* policies within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllIamPolicies`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
* @since 1.13
*/
protected SearchAllIamPolicies(java.lang.String scope) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.SearchAllIamPoliciesResponse.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchAllIamPolicies set$Xgafv(java.lang.String $Xgafv) {
return (SearchAllIamPolicies) super.set$Xgafv($Xgafv);
}
@Override
public SearchAllIamPolicies setAccessToken(java.lang.String accessToken) {
return (SearchAllIamPolicies) super.setAccessToken(accessToken);
}
@Override
public SearchAllIamPolicies setAlt(java.lang.String alt) {
return (SearchAllIamPolicies) super.setAlt(alt);
}
@Override
public SearchAllIamPolicies setCallback(java.lang.String callback) {
return (SearchAllIamPolicies) super.setCallback(callback);
}
@Override
public SearchAllIamPolicies setFields(java.lang.String fields) {
return (SearchAllIamPolicies) super.setFields(fields);
}
@Override
public SearchAllIamPolicies setKey(java.lang.String key) {
return (SearchAllIamPolicies) super.setKey(key);
}
@Override
public SearchAllIamPolicies setOauthToken(java.lang.String oauthToken) {
return (SearchAllIamPolicies) super.setOauthToken(oauthToken);
}
@Override
public SearchAllIamPolicies setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchAllIamPolicies) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchAllIamPolicies setQuotaUser(java.lang.String quotaUser) {
return (SearchAllIamPolicies) super.setQuotaUser(quotaUser);
}
@Override
public SearchAllIamPolicies setUploadType(java.lang.String uploadType) {
return (SearchAllIamPolicies) super.setUploadType(uploadType);
}
@Override
public SearchAllIamPolicies setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchAllIamPolicies) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. A scope can be a project, a folder, or an organization. The search is limited to
* the IAM policies within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllIamPolicies`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. A scope can be a project, a folder, or an organization. The search is limited to the IAM
policies within the `scope`. The caller must be granted the
[`cloudasset.assets.searchAllIamPolicies`](https://cloud.google.com/asset-inventory/docs/access-
control#required_permissions) permission on the desired scope. The allowed values are: *
projects/{PROJECT_ID} (e.g., "projects/foo-bar") * projects/{PROJECT_NUMBER} (e.g.,
"projects/12345678") * folders/{FOLDER_NUMBER} (e.g., "folders/1234567") *
organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. A scope can be a project, a folder, or an organization. The search is limited to
* the IAM policies within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllIamPolicies`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
public SearchAllIamPolicies setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
/**
* Optional. A list of asset types that the IAM policies are attached to. If empty, it will
* search the IAM policies that are attached to all the asset types [supported by search
* APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types) Regular
* expressions are also supported. For example: * "compute.googleapis.com.*" snapshots IAM
* policies attached to asset type starts with "compute.googleapis.com". * ".*Instance"
* snapshots IAM policies attached to asset type ends with "Instance". * ".*Instance.*"
* snapshots IAM policies attached to asset type contains "Instance". See
* [RE2](https://github.com/google/re2/wiki/Syntax) for all supported regular expression
* syntax. If the regular expression does not match any supported asset type, an
* INVALID_ARGUMENT error will be returned.
*/
@com.google.api.client.util.Key
private java.util.List assetTypes;
/** Optional. A list of asset types that the IAM policies are attached to. If empty, it will search the
IAM policies that are attached to all the asset types [supported by search
APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types) Regular expressions are
also supported. For example: * "compute.googleapis.com.*" snapshots IAM policies attached to asset
type starts with "compute.googleapis.com". * ".*Instance" snapshots IAM policies attached to asset
type ends with "Instance". * ".*Instance.*" snapshots IAM policies attached to asset type contains
"Instance". See [RE2](https://github.com/google/re2/wiki/Syntax) for all supported regular
expression syntax. If the regular expression does not match any supported asset type, an
INVALID_ARGUMENT error will be returned.
*/
public java.util.List getAssetTypes() {
return assetTypes;
}
/**
* Optional. A list of asset types that the IAM policies are attached to. If empty, it will
* search the IAM policies that are attached to all the asset types [supported by search
* APIs](https://cloud.google.com/asset-inventory/docs/supported-asset-types) Regular
* expressions are also supported. For example: * "compute.googleapis.com.*" snapshots IAM
* policies attached to asset type starts with "compute.googleapis.com". * ".*Instance"
* snapshots IAM policies attached to asset type ends with "Instance". * ".*Instance.*"
* snapshots IAM policies attached to asset type contains "Instance". See
* [RE2](https://github.com/google/re2/wiki/Syntax) for all supported regular expression
* syntax. If the regular expression does not match any supported asset type, an
* INVALID_ARGUMENT error will be returned.
*/
public SearchAllIamPolicies setAssetTypes(java.util.List assetTypes) {
this.assetTypes = assetTypes;
return this;
}
/**
* Optional. A comma-separated list of fields specifying the sorting order of the results. The
* default order is ascending. Add " DESC" after the field name to indicate descending order.
* Redundant space characters are ignored. Example: "assetType DESC, resource". Only singular
* primitive fields in the response are sortable: * resource * assetType * project All the
* other fields such as repeated fields (e.g., `folders`) and non-primitive fields (e.g.,
* `policy`) are not supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. A comma-separated list of fields specifying the sorting order of the results. The default
order is ascending. Add " DESC" after the field name to indicate descending order. Redundant space
characters are ignored. Example: "assetType DESC, resource". Only singular primitive fields in the
response are sortable: * resource * assetType * project All the other fields such as repeated
fields (e.g., `folders`) and non-primitive fields (e.g., `policy`) are not supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. A comma-separated list of fields specifying the sorting order of the results. The
* default order is ascending. Add " DESC" after the field name to indicate descending order.
* Redundant space characters are ignored. Example: "assetType DESC, resource". Only singular
* primitive fields in the response are sortable: * resource * assetType * project All the
* other fields such as repeated fields (e.g., `folders`) and non-primitive fields (e.g.,
* `policy`) are not supported.
*/
public SearchAllIamPolicies setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The page size for search result pagination. Page size is capped at 500 even if a
* larger value is given. If set to zero or a negative value, server will pick an appropriate
* default. Returned results may be fewer than requested. When this happens, there could be
* more results as long as `next_page_token` is returned.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The page size for search result pagination. Page size is capped at 500 even if a larger
value is given. If set to zero or a negative value, server will pick an appropriate default.
Returned results may be fewer than requested. When this happens, there could be more results as
long as `next_page_token` is returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The page size for search result pagination. Page size is capped at 500 even if a
* larger value is given. If set to zero or a negative value, server will pick an appropriate
* default. Returned results may be fewer than requested. When this happens, there could be
* more results as long as `next_page_token` is returned.
*/
public SearchAllIamPolicies setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, retrieve the next batch of results from the preceding call to this
* method. `page_token` must be the value of `next_page_token` from the previous response. The
* values of all other method parameters must be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, retrieve the next batch of results from the preceding call to this method.
`page_token` must be the value of `next_page_token` from the previous response. The values of all
other method parameters must be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, retrieve the next batch of results from the preceding call to this
* method. `page_token` must be the value of `next_page_token` from the previous response. The
* values of all other method parameters must be identical to those in the previous call.
*/
public SearchAllIamPolicies setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. The query statement. See [how to construct a
* query](https://cloud.google.com/asset-inventory/docs/searching-iam-
* policies#how_to_construct_a_query) for more information. If not specified or empty, it will
* search all the IAM policies within the specified `scope`. Note that the query string is
* compared against each IAM policy binding, including its principals, roles, and IAM
* conditions. The returned IAM policies will only contain the bindings that match your query.
* To learn more about the IAM policy structure, see the [IAM policy
* documentation](https://cloud.google.com/iam/help/allow-policies/structure). Examples: *
* `policy:[email protected]` to find IAM policy bindings that specify user "[email protected]". *
* `policy:roles/compute.admin` to find IAM policy bindings that specify the Compute Admin
* role. * `policy:comp*` to find IAM policy bindings that contain "comp" as a prefix of any
* word in the binding. * `policy.role.permissions:storage.buckets.update` to find IAM policy
* bindings that specify a role containing "storage.buckets.update" permission. Note that if
* callers don't have `iam.roles.get` access to a role's included permissions, policy bindings
* that specify this role will be dropped from the search results. *
* `policy.role.permissions:upd*` to find IAM policy bindings that specify a role containing
* "upd" as a prefix of any word in the role permission. Note that if callers don't have
* `iam.roles.get` access to a role's included permissions, policy bindings that specify this
* role will be dropped from the search results. * `resource:organizations/123456` to find IAM
* policy bindings that are set on "organizations/123456". *
* `resource=//cloudresourcemanager.googleapis.com/projects/myproject` to find IAM policy
* bindings that are set on the project named "myproject". * `Important` to find IAM policy
* bindings that contain "Important" as a word in any of the searchable fields (except for the
* included permissions). * `resource:(instance1 OR instance2) policy:amy` to find IAM policy
* bindings that are set on resources "instance1" or "instance2" and also specify user "amy".
* * `roles:roles/compute.admin` to find IAM policy bindings that specify the Compute Admin
* role. * `memberTypes:user` to find IAM policy bindings that contain the principal type
* "user".
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Optional. The query statement. See [how to construct a query](https://cloud.google.com/asset-
inventory/docs/searching-iam-policies#how_to_construct_a_query) for more information. If not
specified or empty, it will search all the IAM policies within the specified `scope`. Note that the
query string is compared against each IAM policy binding, including its principals, roles, and IAM
conditions. The returned IAM policies will only contain the bindings that match your query. To
learn more about the IAM policy structure, see the [IAM policy
documentation](https://cloud.google.com/iam/help/allow-policies/structure). Examples: *
`policy:[email protected]` to find IAM policy bindings that specify user "[email protected]". *
`policy:roles/compute.admin` to find IAM policy bindings that specify the Compute Admin role. *
`policy:comp*` to find IAM policy bindings that contain "comp" as a prefix of any word in the
binding. * `policy.role.permissions:storage.buckets.update` to find IAM policy bindings that
specify a role containing "storage.buckets.update" permission. Note that if callers don't have
`iam.roles.get` access to a role's included permissions, policy bindings that specify this role
will be dropped from the search results. * `policy.role.permissions:upd*` to find IAM policy
bindings that specify a role containing "upd" as a prefix of any word in the role permission. Note
that if callers don't have `iam.roles.get` access to a role's included permissions, policy bindings
that specify this role will be dropped from the search results. * `resource:organizations/123456`
to find IAM policy bindings that are set on "organizations/123456". *
`resource=//cloudresourcemanager.googleapis.com/projects/myproject` to find IAM policy bindings
that are set on the project named "myproject". * `Important` to find IAM policy bindings that
contain "Important" as a word in any of the searchable fields (except for the included
permissions). * `resource:(instance1 OR instance2) policy:amy` to find IAM policy bindings that are
set on resources "instance1" or "instance2" and also specify user "amy". *
`roles:roles/compute.admin` to find IAM policy bindings that specify the Compute Admin role. *
`memberTypes:user` to find IAM policy bindings that contain the principal type "user".
*/
public java.lang.String getQuery() {
return query;
}
/**
* Optional. The query statement. See [how to construct a
* query](https://cloud.google.com/asset-inventory/docs/searching-iam-
* policies#how_to_construct_a_query) for more information. If not specified or empty, it will
* search all the IAM policies within the specified `scope`. Note that the query string is
* compared against each IAM policy binding, including its principals, roles, and IAM
* conditions. The returned IAM policies will only contain the bindings that match your query.
* To learn more about the IAM policy structure, see the [IAM policy
* documentation](https://cloud.google.com/iam/help/allow-policies/structure). Examples: *
* `policy:[email protected]` to find IAM policy bindings that specify user "[email protected]". *
* `policy:roles/compute.admin` to find IAM policy bindings that specify the Compute Admin
* role. * `policy:comp*` to find IAM policy bindings that contain "comp" as a prefix of any
* word in the binding. * `policy.role.permissions:storage.buckets.update` to find IAM policy
* bindings that specify a role containing "storage.buckets.update" permission. Note that if
* callers don't have `iam.roles.get` access to a role's included permissions, policy bindings
* that specify this role will be dropped from the search results. *
* `policy.role.permissions:upd*` to find IAM policy bindings that specify a role containing
* "upd" as a prefix of any word in the role permission. Note that if callers don't have
* `iam.roles.get` access to a role's included permissions, policy bindings that specify this
* role will be dropped from the search results. * `resource:organizations/123456` to find IAM
* policy bindings that are set on "organizations/123456". *
* `resource=//cloudresourcemanager.googleapis.com/projects/myproject` to find IAM policy
* bindings that are set on the project named "myproject". * `Important` to find IAM policy
* bindings that contain "Important" as a word in any of the searchable fields (except for the
* included permissions). * `resource:(instance1 OR instance2) policy:amy` to find IAM policy
* bindings that are set on resources "instance1" or "instance2" and also specify user "amy".
* * `roles:roles/compute.admin` to find IAM policy bindings that specify the Compute Admin
* role. * `memberTypes:user` to find IAM policy bindings that contain the principal type
* "user".
*/
public SearchAllIamPolicies setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public SearchAllIamPolicies set(String parameterName, Object value) {
return (SearchAllIamPolicies) super.set(parameterName, value);
}
}
/**
* Searches all Google Cloud resources within the specified scope, such as a project, folder, or
* organization. The caller must be granted the `cloudasset.assets.searchAllResources` permission on
* the desired scope, otherwise the request will be rejected.
*
* Create a request for the method "v1.searchAllResources".
*
* This request holds the parameters needed by the cloudasset server. After setting any optional
* parameters, call the {@link SearchAllResources#execute()} method to invoke the remote operation.
*
* @param scope Required. A scope can be a project, a folder, or an organization. The search is limited to the
* resources within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllResources`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
* @return the request
*/
public SearchAllResources searchAllResources(java.lang.String scope) throws java.io.IOException {
SearchAllResources result = new SearchAllResources(scope);
initialize(result);
return result;
}
public class SearchAllResources extends CloudAssetRequest {
private static final String REST_PATH = "v1/{+scope}:searchAllResources";
private final java.util.regex.Pattern SCOPE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Searches all Google Cloud resources within the specified scope, such as a project, folder, or
* organization. The caller must be granted the `cloudasset.assets.searchAllResources` permission
* on the desired scope, otherwise the request will be rejected.
*
* Create a request for the method "v1.searchAllResources".
*
* This request holds the parameters needed by the the cloudasset server. After setting any
* optional parameters, call the {@link SearchAllResources#execute()} method to invoke the remote
* operation. {@link SearchAllResources#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param scope Required. A scope can be a project, a folder, or an organization. The search is limited to the
* resources within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllResources`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
* @since 1.13
*/
protected SearchAllResources(java.lang.String scope) {
super(CloudAsset.this, "GET", REST_PATH, null, com.google.api.services.cloudasset.v1.model.SearchAllResourcesResponse.class);
this.scope = com.google.api.client.util.Preconditions.checkNotNull(scope, "Required parameter scope must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchAllResources set$Xgafv(java.lang.String $Xgafv) {
return (SearchAllResources) super.set$Xgafv($Xgafv);
}
@Override
public SearchAllResources setAccessToken(java.lang.String accessToken) {
return (SearchAllResources) super.setAccessToken(accessToken);
}
@Override
public SearchAllResources setAlt(java.lang.String alt) {
return (SearchAllResources) super.setAlt(alt);
}
@Override
public SearchAllResources setCallback(java.lang.String callback) {
return (SearchAllResources) super.setCallback(callback);
}
@Override
public SearchAllResources setFields(java.lang.String fields) {
return (SearchAllResources) super.setFields(fields);
}
@Override
public SearchAllResources setKey(java.lang.String key) {
return (SearchAllResources) super.setKey(key);
}
@Override
public SearchAllResources setOauthToken(java.lang.String oauthToken) {
return (SearchAllResources) super.setOauthToken(oauthToken);
}
@Override
public SearchAllResources setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchAllResources) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchAllResources setQuotaUser(java.lang.String quotaUser) {
return (SearchAllResources) super.setQuotaUser(quotaUser);
}
@Override
public SearchAllResources setUploadType(java.lang.String uploadType) {
return (SearchAllResources) super.setUploadType(uploadType);
}
@Override
public SearchAllResources setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchAllResources) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. A scope can be a project, a folder, or an organization. The search is limited to
* the resources within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllResources`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/** Required. A scope can be a project, a folder, or an organization. The search is limited to the
resources within the `scope`. The caller must be granted the
[`cloudasset.assets.searchAllResources`](https://cloud.google.com/asset-inventory/docs/access-
control#required_permissions) permission on the desired scope. The allowed values are: *
projects/{PROJECT_ID} (e.g., "projects/foo-bar") * projects/{PROJECT_NUMBER} (e.g.,
"projects/12345678") * folders/{FOLDER_NUMBER} (e.g., "folders/1234567") *
organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
public java.lang.String getScope() {
return scope;
}
/**
* Required. A scope can be a project, a folder, or an organization. The search is limited to
* the resources within the `scope`. The caller must be granted the
* [`cloudasset.assets.searchAllResources`](https://cloud.google.com/asset-
* inventory/docs/access-control#required_permissions) permission on the desired scope. The
* allowed values are: * projects/{PROJECT_ID} (e.g., "projects/foo-bar") *
* projects/{PROJECT_NUMBER} (e.g., "projects/12345678") * folders/{FOLDER_NUMBER} (e.g.,
* "folders/1234567") * organizations/{ORGANIZATION_NUMBER} (e.g., "organizations/123456")
*/
public SearchAllResources setScope(java.lang.String scope) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCOPE_PATTERN.matcher(scope).matches(),
"Parameter scope must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.scope = scope;
return this;
}
/**
* Optional. A list of asset types that this request searches for. If empty, it will search
* all the asset types [supported by search APIs](https://cloud.google.com/asset-
* inventory/docs/supported-asset-types). Regular expressions are also supported. For example:
* * "compute.googleapis.com.*" snapshots resources whose asset type starts with
* "compute.googleapis.com". * ".*Instance" snapshots resources whose asset type ends with
* "Instance". * ".*Instance.*" snapshots resources whose asset type contains "Instance". See
* [RE2](https://github.com/google/re2/wiki/Syntax) for all supported regular expression
* syntax. If the regular expression does not match any supported asset type, an
* INVALID_ARGUMENT error will be returned.
*/
@com.google.api.client.util.Key
private java.util.List assetTypes;
/** Optional. A list of asset types that this request searches for. If empty, it will search all the
asset types [supported by search APIs](https://cloud.google.com/asset-inventory/docs/supported-
asset-types). Regular expressions are also supported. For example: * "compute.googleapis.com.*"
snapshots resources whose asset type starts with "compute.googleapis.com". * ".*Instance" snapshots
resources whose asset type ends with "Instance". * ".*Instance.*" snapshots resources whose asset
type contains "Instance". See [RE2](https://github.com/google/re2/wiki/Syntax) for all supported
regular expression syntax. If the regular expression does not match any supported asset type, an
INVALID_ARGUMENT error will be returned.
*/
public java.util.List getAssetTypes() {
return assetTypes;
}
/**
* Optional. A list of asset types that this request searches for. If empty, it will search
* all the asset types [supported by search APIs](https://cloud.google.com/asset-
* inventory/docs/supported-asset-types). Regular expressions are also supported. For example:
* * "compute.googleapis.com.*" snapshots resources whose asset type starts with
* "compute.googleapis.com". * ".*Instance" snapshots resources whose asset type ends with
* "Instance". * ".*Instance.*" snapshots resources whose asset type contains "Instance". See
* [RE2](https://github.com/google/re2/wiki/Syntax) for all supported regular expression
* syntax. If the regular expression does not match any supported asset type, an
* INVALID_ARGUMENT error will be returned.
*/
public SearchAllResources setAssetTypes(java.util.List assetTypes) {
this.assetTypes = assetTypes;
return this;
}
/**
* Optional. A comma-separated list of fields specifying the sorting order of the results. The
* default order is ascending. Add " DESC" after the field name to indicate descending order.
* Redundant space characters are ignored. Example: "location DESC, name". Only the following
* fields in the response are sortable: * name * assetType * project * displayName *
* description * location * createTime * updateTime * state * parentFullResourceName *
* parentAssetType
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. A comma-separated list of fields specifying the sorting order of the results. The default
order is ascending. Add " DESC" after the field name to indicate descending order. Redundant space
characters are ignored. Example: "location DESC, name". Only the following fields in the response
are sortable: * name * assetType * project * displayName * description * location * createTime *
updateTime * state * parentFullResourceName * parentAssetType
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. A comma-separated list of fields specifying the sorting order of the results. The
* default order is ascending. Add " DESC" after the field name to indicate descending order.
* Redundant space characters are ignored. Example: "location DESC, name". Only the following
* fields in the response are sortable: * name * assetType * project * displayName *
* description * location * createTime * updateTime * state * parentFullResourceName *
* parentAssetType
*/
public SearchAllResources setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The page size for search result pagination. Page size is capped at 500 even if a
* larger value is given. If set to zero or a negative value, server will pick an appropriate
* default. Returned results may be fewer than requested. When this happens, there could be
* more results as long as `next_page_token` is returned.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The page size for search result pagination. Page size is capped at 500 even if a larger
value is given. If set to zero or a negative value, server will pick an appropriate default.
Returned results may be fewer than requested. When this happens, there could be more results as
long as `next_page_token` is returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The page size for search result pagination. Page size is capped at 500 even if a
* larger value is given. If set to zero or a negative value, server will pick an appropriate
* default. Returned results may be fewer than requested. When this happens, there could be
* more results as long as `next_page_token` is returned.
*/
public SearchAllResources setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. `page_token` must be the value of `next_page_token` from the previous
* response. The values of all other method parameters, must be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. `page_token` must be the value of `next_page_token` from the previous response. The values
of all other method parameters, must be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. `page_token` must be the value of `next_page_token` from the previous
* response. The values of all other method parameters, must be identical to those in the
* previous call.
*/
public SearchAllResources setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. The query statement. See [how to construct a
* query](https://cloud.google.com/asset-inventory/docs/searching-
* resources#how_to_construct_a_query) for more information. If not specified or empty, it
* will search all the resources within the specified `scope`. Examples: * `name:Important` to
* find Google Cloud resources whose name contains `Important` as a word. * `name=Important`
* to find the Google Cloud resource whose name is exactly `Important`. * `displayName:Impor*`
* to find Google Cloud resources whose display name contains `Impor` as a prefix of any word
* in the field. * `location:us-west*` to find Google Cloud resources whose location contains
* both `us` and `west` as prefixes. * `labels:prod` to find Google Cloud resources whose
* labels contain `prod` as a key or value. * `labels.env:prod` to find Google Cloud resources
* that have a label `env` and its value is `prod`. * `labels.env:*` to find Google Cloud
* resources that have a label `env`. * `tagKeys:env` to find Google Cloud resources that have
* directly attached tags where the
* [`TagKey.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagKeys#resource:-tagkey) contains `env`. * `tagValues:prod*` to
* find Google Cloud resources that have directly attached tags where the
* [`TagValue.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) contains a word prefixed by `prod`.
* * `tagValueIds=tagValues/123` to find Google Cloud resources that have directly attached
* tags where the [`TagValue.name`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) is exactly `tagValues/123`. *
* `effectiveTagKeys:env` to find Google Cloud resources that have directly attached or
* inherited tags where the [`TagKey.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagKeys#resource:-tagkey) contains `env`. *
* `effectiveTagValues:prod*` to find Google Cloud resources that have directly attached or
* inherited tags where the [`TagValue.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) contains a word prefixed by `prod`.
* * `effectiveTagValueIds=tagValues/123` to find Google Cloud resources that have directly
* attached or inherited tags where the [`TagValue.name`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) is exactly `tagValues/123`. *
* `kmsKey:key` to find Google Cloud resources encrypted with a customer-managed encryption
* key whose name contains `key` as a word. This field is deprecated. Use the `kmsKeys` field
* to retrieve Cloud KMS key information. * `kmsKeys:key` to find Google Cloud resources
* encrypted with customer-managed encryption keys whose name contains the word `key`. *
* `relationships:instance-group-1` to find Google Cloud resources that have relationships
* with `instance-group-1` in the related resource name. *
* `relationships:INSTANCE_TO_INSTANCEGROUP` to find Compute Engine instances that have
* relationships of type `INSTANCE_TO_INSTANCEGROUP`. *
* `relationships.INSTANCE_TO_INSTANCEGROUP:instance-group-1` to find Compute Engine instances
* that have relationships with `instance-group-1` in the Compute Engine instance group
* resource name, for relationship type `INSTANCE_TO_INSTANCEGROUP`. *
* `sccSecurityMarks.key=value` to find Cloud resources that are attached with security marks
* whose key is `key` and value is `value`. * `sccSecurityMarks.key:*` to find Cloud resources
* that are attached with security marks whose key is `key`. * `state:ACTIVE` to find Google
* Cloud resources whose state contains `ACTIVE` as a word. * `NOT state:ACTIVE` to find
* Google Cloud resources whose state doesn't contain `ACTIVE` as a word. *
* `createTime<1609459200` to find Google Cloud resources that were created before `2021-01-01
* 00:00:00 UTC`. `1609459200` is the epoch timestamp of `2021-01-01 00:00:00 UTC` in seconds.
* * `updateTime>1609459200` to find Google Cloud resources that were updated after
* `2021-01-01 00:00:00 UTC`. `1609459200` is the epoch timestamp of `2021-01-01 00:00:00 UTC`
* in seconds. * `Important` to find Google Cloud resources that contain `Important` as a word
* in any of the searchable fields. * `Impor*` to find Google Cloud resources that contain
* `Impor` as a prefix of any word in any of the searchable fields. * `Important location:(us-
* west1 OR global)` to find Google Cloud resources that contain `Important` as a word in any
* of the searchable fields and are also located in the `us-west1` region or the `global`
* location.
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Optional. The query statement. See [how to construct a query](https://cloud.google.com/asset-
inventory/docs/searching-resources#how_to_construct_a_query) for more information. If not specified
or empty, it will search all the resources within the specified `scope`. Examples: *
`name:Important` to find Google Cloud resources whose name contains `Important` as a word. *
`name=Important` to find the Google Cloud resource whose name is exactly `Important`. *
`displayName:Impor*` to find Google Cloud resources whose display name contains `Impor` as a prefix
of any word in the field. * `location:us-west*` to find Google Cloud resources whose location
contains both `us` and `west` as prefixes. * `labels:prod` to find Google Cloud resources whose
labels contain `prod` as a key or value. * `labels.env:prod` to find Google Cloud resources that
have a label `env` and its value is `prod`. * `labels.env:*` to find Google Cloud resources that
have a label `env`. * `tagKeys:env` to find Google Cloud resources that have directly attached tags
where the [`TagKey.namespacedName`](https://cloud.google.com/resource-
manager/reference/rest/v3/tagKeys#resource:-tagkey) contains `env`. * `tagValues:prod*` to find
Google Cloud resources that have directly attached tags where the
[`TagValue.namespacedName`](https://cloud.google.com/resource-
manager/reference/rest/v3/tagValues#resource:-tagvalue) contains a word prefixed by `prod`. *
`tagValueIds=tagValues/123` to find Google Cloud resources that have directly attached tags where
the [`TagValue.name`](https://cloud.google.com/resource-
manager/reference/rest/v3/tagValues#resource:-tagvalue) is exactly `tagValues/123`. *
`effectiveTagKeys:env` to find Google Cloud resources that have directly attached or inherited tags
where the [`TagKey.namespacedName`](https://cloud.google.com/resource-
manager/reference/rest/v3/tagKeys#resource:-tagkey) contains `env`. * `effectiveTagValues:prod*` to
find Google Cloud resources that have directly attached or inherited tags where the
[`TagValue.namespacedName`](https://cloud.google.com/resource-
manager/reference/rest/v3/tagValues#resource:-tagvalue) contains a word prefixed by `prod`. *
`effectiveTagValueIds=tagValues/123` to find Google Cloud resources that have directly attached or
inherited tags where the [`TagValue.name`](https://cloud.google.com/resource-
manager/reference/rest/v3/tagValues#resource:-tagvalue) is exactly `tagValues/123`. * `kmsKey:key`
to find Google Cloud resources encrypted with a customer-managed encryption key whose name contains
`key` as a word. This field is deprecated. Use the `kmsKeys` field to retrieve Cloud KMS key
information. * `kmsKeys:key` to find Google Cloud resources encrypted with customer-managed
encryption keys whose name contains the word `key`. * `relationships:instance-group-1` to find
Google Cloud resources that have relationships with `instance-group-1` in the related resource
name. * `relationships:INSTANCE_TO_INSTANCEGROUP` to find Compute Engine instances that have
relationships of type `INSTANCE_TO_INSTANCEGROUP`. *
`relationships.INSTANCE_TO_INSTANCEGROUP:instance-group-1` to find Compute Engine instances that
have relationships with `instance-group-1` in the Compute Engine instance group resource name, for
relationship type `INSTANCE_TO_INSTANCEGROUP`. * `sccSecurityMarks.key=value` to find Cloud
resources that are attached with security marks whose key is `key` and value is `value`. *
`sccSecurityMarks.key:*` to find Cloud resources that are attached with security marks whose key is
`key`. * `state:ACTIVE` to find Google Cloud resources whose state contains `ACTIVE` as a word. *
`NOT state:ACTIVE` to find Google Cloud resources whose state doesn't contain `ACTIVE` as a word. *
`createTime<1609459200` to find Google Cloud resources that were created before `2021-01-01
00:00:00 UTC`. `1609459200` is the epoch timestamp of `2021-01-01 00:00:00 UTC` in seconds. *
`updateTime>1609459200` to find Google Cloud resources that were updated after `2021-01-01 00:00:00
UTC`. `1609459200` is the epoch timestamp of `2021-01-01 00:00:00 UTC` in seconds. * `Important` to
find Google Cloud resources that contain `Important` as a word in any of the searchable fields. *
`Impor*` to find Google Cloud resources that contain `Impor` as a prefix of any word in any of the
searchable fields. * `Important location:(us-west1 OR global)` to find Google Cloud resources that
contain `Important` as a word in any of the searchable fields and are also located in the `us-
west1` region or the `global` location.
*/
public java.lang.String getQuery() {
return query;
}
/**
* Optional. The query statement. See [how to construct a
* query](https://cloud.google.com/asset-inventory/docs/searching-
* resources#how_to_construct_a_query) for more information. If not specified or empty, it
* will search all the resources within the specified `scope`. Examples: * `name:Important` to
* find Google Cloud resources whose name contains `Important` as a word. * `name=Important`
* to find the Google Cloud resource whose name is exactly `Important`. * `displayName:Impor*`
* to find Google Cloud resources whose display name contains `Impor` as a prefix of any word
* in the field. * `location:us-west*` to find Google Cloud resources whose location contains
* both `us` and `west` as prefixes. * `labels:prod` to find Google Cloud resources whose
* labels contain `prod` as a key or value. * `labels.env:prod` to find Google Cloud resources
* that have a label `env` and its value is `prod`. * `labels.env:*` to find Google Cloud
* resources that have a label `env`. * `tagKeys:env` to find Google Cloud resources that have
* directly attached tags where the
* [`TagKey.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagKeys#resource:-tagkey) contains `env`. * `tagValues:prod*` to
* find Google Cloud resources that have directly attached tags where the
* [`TagValue.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) contains a word prefixed by `prod`.
* * `tagValueIds=tagValues/123` to find Google Cloud resources that have directly attached
* tags where the [`TagValue.name`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) is exactly `tagValues/123`. *
* `effectiveTagKeys:env` to find Google Cloud resources that have directly attached or
* inherited tags where the [`TagKey.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagKeys#resource:-tagkey) contains `env`. *
* `effectiveTagValues:prod*` to find Google Cloud resources that have directly attached or
* inherited tags where the [`TagValue.namespacedName`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) contains a word prefixed by `prod`.
* * `effectiveTagValueIds=tagValues/123` to find Google Cloud resources that have directly
* attached or inherited tags where the [`TagValue.name`](https://cloud.google.com/resource-
* manager/reference/rest/v3/tagValues#resource:-tagvalue) is exactly `tagValues/123`. *
* `kmsKey:key` to find Google Cloud resources encrypted with a customer-managed encryption
* key whose name contains `key` as a word. This field is deprecated. Use the `kmsKeys` field
* to retrieve Cloud KMS key information. * `kmsKeys:key` to find Google Cloud resources
* encrypted with customer-managed encryption keys whose name contains the word `key`. *
* `relationships:instance-group-1` to find Google Cloud resources that have relationships
* with `instance-group-1` in the related resource name. *
* `relationships:INSTANCE_TO_INSTANCEGROUP` to find Compute Engine instances that have
* relationships of type `INSTANCE_TO_INSTANCEGROUP`. *
* `relationships.INSTANCE_TO_INSTANCEGROUP:instance-group-1` to find Compute Engine instances
* that have relationships with `instance-group-1` in the Compute Engine instance group
* resource name, for relationship type `INSTANCE_TO_INSTANCEGROUP`. *
* `sccSecurityMarks.key=value` to find Cloud resources that are attached with security marks
* whose key is `key` and value is `value`. * `sccSecurityMarks.key:*` to find Cloud resources
* that are attached with security marks whose key is `key`. * `state:ACTIVE` to find Google
* Cloud resources whose state contains `ACTIVE` as a word. * `NOT state:ACTIVE` to find
* Google Cloud resources whose state doesn't contain `ACTIVE` as a word. *
* `createTime<1609459200` to find Google Cloud resources that were created before `2021-01-01
* 00:00:00 UTC`. `1609459200` is the epoch timestamp of `2021-01-01 00:00:00 UTC` in seconds.
* * `updateTime>1609459200` to find Google Cloud resources that were updated after
* `2021-01-01 00:00:00 UTC`. `1609459200` is the epoch timestamp of `2021-01-01 00:00:00 UTC`
* in seconds. * `Important` to find Google Cloud resources that contain `Important` as a word
* in any of the searchable fields. * `Impor*` to find Google Cloud resources that contain
* `Impor` as a prefix of any word in any of the searchable fields. * `Important location:(us-
* west1 OR global)` to find Google Cloud resources that contain `Important` as a word in any
* of the searchable fields and are also located in the `us-west1` region or the `global`
* location.
*/
public SearchAllResources setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* Optional. A comma-separated list of fields that you want returned in the results. The
* following fields are returned by default if not specified: * `name` * `assetType` *
* `project` * `folders` * `organization` * `displayName` * `description` * `location` *
* `labels` * `tags` * `effectiveTags` * `networkTags` * `kmsKeys` * `createTime` *
* `updateTime` * `state` * `additionalAttributes` * `parentFullResourceName` *
* `parentAssetType` Some fields of large size, such as `versionedResources`,
* `attachedResources`, `effectiveTags` etc., are not returned by default, but you can specify
* them in the `read_mask` parameter if you want to include them. If `"*"` is specified, all
* [available fields](https://cloud.google.com/asset-
* inventory/docs/reference/rest/v1/TopLevel/searchAllResources#resourcesearchresult) are
* returned. Examples: `"name,location"`, `"name,versionedResources"`, `"*"`. Any invalid
* field path will trigger INVALID_ARGUMENT error.
*/
@com.google.api.client.util.Key
private String readMask;
/** Optional. A comma-separated list of fields that you want returned in the results. The following
fields are returned by default if not specified: * `name` * `assetType` * `project` * `folders` *
`organization` * `displayName` * `description` * `location` * `labels` * `tags` * `effectiveTags` *
`networkTags` * `kmsKeys` * `createTime` * `updateTime` * `state` * `additionalAttributes` *
`parentFullResourceName` * `parentAssetType` Some fields of large size, such as
`versionedResources`, `attachedResources`, `effectiveTags` etc., are not returned by default, but
you can specify them in the `read_mask` parameter if you want to include them. If `"*"` is
specified, all [available fields](https://cloud.google.com/asset-
inventory/docs/reference/rest/v1/TopLevel/searchAllResources#resourcesearchresult) are returned.
Examples: `"name,location"`, `"name,versionedResources"`, `"*"`. Any invalid field path will
trigger INVALID_ARGUMENT error.
*/
public String getReadMask() {
return readMask;
}
/**
* Optional. A comma-separated list of fields that you want returned in the results. The
* following fields are returned by default if not specified: * `name` * `assetType` *
* `project` * `folders` * `organization` * `displayName` * `description` * `location` *
* `labels` * `tags` * `effectiveTags` * `networkTags` * `kmsKeys` * `createTime` *
* `updateTime` * `state` * `additionalAttributes` * `parentFullResourceName` *
* `parentAssetType` Some fields of large size, such as `versionedResources`,
* `attachedResources`, `effectiveTags` etc., are not returned by default, but you can specify
* them in the `read_mask` parameter if you want to include them. If `"*"` is specified, all
* [available fields](https://cloud.google.com/asset-
* inventory/docs/reference/rest/v1/TopLevel/searchAllResources#resourcesearchresult) are
* returned. Examples: `"name,location"`, `"name,versionedResources"`, `"*"`. Any invalid
* field path will trigger INVALID_ARGUMENT error.
*/
public SearchAllResources setReadMask(String readMask) {
this.readMask = readMask;
return this;
}
@Override
public SearchAllResources set(String parameterName, Object value) {
return (SearchAllResources) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link CloudAsset}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudAsset}. */
@Override
public CloudAsset build() {
return new CloudAsset(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudAssetRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudAssetRequestInitializer(
CloudAssetRequestInitializer cloudassetRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudassetRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}