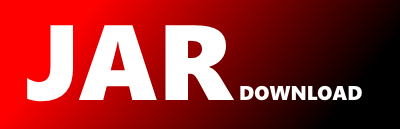
com.google.api.services.cloudasset.v1.model.AnalyzerOrgPolicy Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudasset.v1.model;
/**
* This organization policy message is a modified version of the one defined in the Organization
* Policy system. This message contains several fields defined in the original organization policy
* with some new fields for analysis purpose.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Asset API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AnalyzerOrgPolicy extends com.google.api.client.json.GenericJson {
/**
* The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-name-format)
* of an organization/folder/project resource where this organization policy applies to. For any
* user defined org policies, this field has the same value as the [attached_resource] field. Only
* for default policy, this field has the different value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String appliedResource;
/**
* The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-name-format)
* of an organization/folder/project resource where this organization policy is set. Notice that
* some type of constraints are defined with default policy. This field will be empty for them.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String attachedResource;
/**
* If `inherit_from_parent` is true, Rules set higher up in the hierarchy (up to the closest root)
* are inherited and present in the effective policy. If it is false, then no rules are inherited,
* and this policy becomes the effective root for evaluation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean inheritFromParent;
/**
* Ignores policies set above this resource and restores the default behavior of the constraint at
* this resource. This field can be set in policies for either list or boolean constraints. If
* set, `rules` must be empty and `inherit_from_parent` must be set to false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean reset;
/**
* List of rules for this organization policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List rules;
/**
* The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-name-format)
* of an organization/folder/project resource where this organization policy applies to. For any
* user defined org policies, this field has the same value as the [attached_resource] field. Only
* for default policy, this field has the different value.
* @return value or {@code null} for none
*/
public java.lang.String getAppliedResource() {
return appliedResource;
}
/**
* The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-name-format)
* of an organization/folder/project resource where this organization policy applies to. For any
* user defined org policies, this field has the same value as the [attached_resource] field. Only
* for default policy, this field has the different value.
* @param appliedResource appliedResource or {@code null} for none
*/
public AnalyzerOrgPolicy setAppliedResource(java.lang.String appliedResource) {
this.appliedResource = appliedResource;
return this;
}
/**
* The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-name-format)
* of an organization/folder/project resource where this organization policy is set. Notice that
* some type of constraints are defined with default policy. This field will be empty for them.
* @return value or {@code null} for none
*/
public java.lang.String getAttachedResource() {
return attachedResource;
}
/**
* The [full resource name] (https://cloud.google.com/asset-inventory/docs/resource-name-format)
* of an organization/folder/project resource where this organization policy is set. Notice that
* some type of constraints are defined with default policy. This field will be empty for them.
* @param attachedResource attachedResource or {@code null} for none
*/
public AnalyzerOrgPolicy setAttachedResource(java.lang.String attachedResource) {
this.attachedResource = attachedResource;
return this;
}
/**
* If `inherit_from_parent` is true, Rules set higher up in the hierarchy (up to the closest root)
* are inherited and present in the effective policy. If it is false, then no rules are inherited,
* and this policy becomes the effective root for evaluation.
* @return value or {@code null} for none
*/
public java.lang.Boolean getInheritFromParent() {
return inheritFromParent;
}
/**
* If `inherit_from_parent` is true, Rules set higher up in the hierarchy (up to the closest root)
* are inherited and present in the effective policy. If it is false, then no rules are inherited,
* and this policy becomes the effective root for evaluation.
* @param inheritFromParent inheritFromParent or {@code null} for none
*/
public AnalyzerOrgPolicy setInheritFromParent(java.lang.Boolean inheritFromParent) {
this.inheritFromParent = inheritFromParent;
return this;
}
/**
* Ignores policies set above this resource and restores the default behavior of the constraint at
* this resource. This field can be set in policies for either list or boolean constraints. If
* set, `rules` must be empty and `inherit_from_parent` must be set to false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getReset() {
return reset;
}
/**
* Ignores policies set above this resource and restores the default behavior of the constraint at
* this resource. This field can be set in policies for either list or boolean constraints. If
* set, `rules` must be empty and `inherit_from_parent` must be set to false.
* @param reset reset or {@code null} for none
*/
public AnalyzerOrgPolicy setReset(java.lang.Boolean reset) {
this.reset = reset;
return this;
}
/**
* List of rules for this organization policy.
* @return value or {@code null} for none
*/
public java.util.List getRules() {
return rules;
}
/**
* List of rules for this organization policy.
* @param rules rules or {@code null} for none
*/
public AnalyzerOrgPolicy setRules(java.util.List rules) {
this.rules = rules;
return this;
}
@Override
public AnalyzerOrgPolicy set(String fieldName, Object value) {
return (AnalyzerOrgPolicy) super.set(fieldName, value);
}
@Override
public AnalyzerOrgPolicy clone() {
return (AnalyzerOrgPolicy) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy