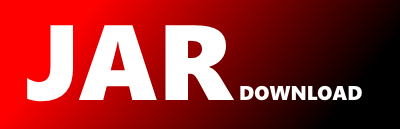
com.google.api.services.cloudasset.v1.model.BigQueryDestination Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudasset.v1.model;
/**
* A BigQuery destination for exporting assets to.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Asset API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class BigQueryDestination extends com.google.api.client.json.GenericJson {
/**
* Required. The BigQuery dataset in format "projects/projectId/datasets/datasetId", to which the
* snapshot result should be exported. If this dataset does not exist, the export call returns an
* INVALID_ARGUMENT error. Setting the `contentType` for `exportAssets` determines the
* [schema](/asset-inventory/docs/exporting-to-bigquery#bigquery-schema) of the BigQuery table.
* Setting `separateTablesPerAssetType` to `TRUE` also influences the schema.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dataset;
/**
* If the destination table already exists and this flag is `TRUE`, the table will be overwritten
* by the contents of assets snapshot. If the flag is `FALSE` or unset and the destination table
* already exists, the export call returns an INVALID_ARGUMEMT error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/**
* [partition_spec] determines whether to export to partitioned table(s) and how to partition the
* data. If [partition_spec] is unset or [partition_spec.partition_key] is unset or
* `PARTITION_KEY_UNSPECIFIED`, the snapshot results will be exported to non-partitioned table(s).
* [force] will decide whether to overwrite existing table(s). If [partition_spec] is specified.
* First, the snapshot results will be written to partitioned table(s) with two additional
* timestamp columns, readTime and requestTime, one of which will be the partition key. Secondly,
* in the case when any destination table already exists, it will first try to update existing
* table's schema as necessary by appending additional columns. Then, if [force] is `TRUE`, the
* corresponding partition will be overwritten by the snapshot results (data in different
* partitions will remain intact); if [force] is unset or `FALSE`, it will append the data. An
* error will be returned if the schema update or data appension fails.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PartitionSpec partitionSpec;
/**
* If this flag is `TRUE`, the snapshot results will be written to one or multiple tables, each of
* which contains results of one asset type. The [force] and [partition_spec] fields will apply to
* each of them. Field [table] will be concatenated with "_" and the asset type names (see
* https://cloud.google.com/asset-inventory/docs/supported-asset-types for supported asset types)
* to construct per-asset-type table names, in which all non-alphanumeric characters like "." and
* "/" will be substituted by "_". Example: if field [table] is "mytable" and snapshot results
* contain "storage.googleapis.com/Bucket" assets, the corresponding table name will be
* "mytable_storage_googleapis_com_Bucket". If any of these tables does not exist, a new table
* with the concatenated name will be created. When [content_type] in the ExportAssetsRequest is
* `RESOURCE`, the schema of each table will include RECORD-type columns mapped to the nested
* fields in the Asset.resource.data field of that asset type (up to the 15 nested level BigQuery
* supports (https://cloud.google.com/bigquery/docs/nested-repeated#limitations)). The fields in
* >15 nested levels will be stored in JSON format string as a child column of its parent RECORD
* column. If error occurs when exporting to any table, the whole export call will return an error
* but the export results that already succeed will persist. Example: if exporting to table_type_A
* succeeds when exporting to table_type_B fails during one export call, the results in
* table_type_A will persist and there will not be partial results persisting in a table.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean separateTablesPerAssetType;
/**
* Required. The BigQuery table to which the snapshot result should be written. If this table does
* not exist, a new table with the given name will be created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String table;
/**
* Required. The BigQuery dataset in format "projects/projectId/datasets/datasetId", to which the
* snapshot result should be exported. If this dataset does not exist, the export call returns an
* INVALID_ARGUMENT error. Setting the `contentType` for `exportAssets` determines the
* [schema](/asset-inventory/docs/exporting-to-bigquery#bigquery-schema) of the BigQuery table.
* Setting `separateTablesPerAssetType` to `TRUE` also influences the schema.
* @return value or {@code null} for none
*/
public java.lang.String getDataset() {
return dataset;
}
/**
* Required. The BigQuery dataset in format "projects/projectId/datasets/datasetId", to which the
* snapshot result should be exported. If this dataset does not exist, the export call returns an
* INVALID_ARGUMENT error. Setting the `contentType` for `exportAssets` determines the
* [schema](/asset-inventory/docs/exporting-to-bigquery#bigquery-schema) of the BigQuery table.
* Setting `separateTablesPerAssetType` to `TRUE` also influences the schema.
* @param dataset dataset or {@code null} for none
*/
public BigQueryDestination setDataset(java.lang.String dataset) {
this.dataset = dataset;
return this;
}
/**
* If the destination table already exists and this flag is `TRUE`, the table will be overwritten
* by the contents of assets snapshot. If the flag is `FALSE` or unset and the destination table
* already exists, the export call returns an INVALID_ARGUMEMT error.
* @return value or {@code null} for none
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* If the destination table already exists and this flag is `TRUE`, the table will be overwritten
* by the contents of assets snapshot. If the flag is `FALSE` or unset and the destination table
* already exists, the export call returns an INVALID_ARGUMEMT error.
* @param force force or {@code null} for none
*/
public BigQueryDestination setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* [partition_spec] determines whether to export to partitioned table(s) and how to partition the
* data. If [partition_spec] is unset or [partition_spec.partition_key] is unset or
* `PARTITION_KEY_UNSPECIFIED`, the snapshot results will be exported to non-partitioned table(s).
* [force] will decide whether to overwrite existing table(s). If [partition_spec] is specified.
* First, the snapshot results will be written to partitioned table(s) with two additional
* timestamp columns, readTime and requestTime, one of which will be the partition key. Secondly,
* in the case when any destination table already exists, it will first try to update existing
* table's schema as necessary by appending additional columns. Then, if [force] is `TRUE`, the
* corresponding partition will be overwritten by the snapshot results (data in different
* partitions will remain intact); if [force] is unset or `FALSE`, it will append the data. An
* error will be returned if the schema update or data appension fails.
* @return value or {@code null} for none
*/
public PartitionSpec getPartitionSpec() {
return partitionSpec;
}
/**
* [partition_spec] determines whether to export to partitioned table(s) and how to partition the
* data. If [partition_spec] is unset or [partition_spec.partition_key] is unset or
* `PARTITION_KEY_UNSPECIFIED`, the snapshot results will be exported to non-partitioned table(s).
* [force] will decide whether to overwrite existing table(s). If [partition_spec] is specified.
* First, the snapshot results will be written to partitioned table(s) with two additional
* timestamp columns, readTime and requestTime, one of which will be the partition key. Secondly,
* in the case when any destination table already exists, it will first try to update existing
* table's schema as necessary by appending additional columns. Then, if [force] is `TRUE`, the
* corresponding partition will be overwritten by the snapshot results (data in different
* partitions will remain intact); if [force] is unset or `FALSE`, it will append the data. An
* error will be returned if the schema update or data appension fails.
* @param partitionSpec partitionSpec or {@code null} for none
*/
public BigQueryDestination setPartitionSpec(PartitionSpec partitionSpec) {
this.partitionSpec = partitionSpec;
return this;
}
/**
* If this flag is `TRUE`, the snapshot results will be written to one or multiple tables, each of
* which contains results of one asset type. The [force] and [partition_spec] fields will apply to
* each of them. Field [table] will be concatenated with "_" and the asset type names (see
* https://cloud.google.com/asset-inventory/docs/supported-asset-types for supported asset types)
* to construct per-asset-type table names, in which all non-alphanumeric characters like "." and
* "/" will be substituted by "_". Example: if field [table] is "mytable" and snapshot results
* contain "storage.googleapis.com/Bucket" assets, the corresponding table name will be
* "mytable_storage_googleapis_com_Bucket". If any of these tables does not exist, a new table
* with the concatenated name will be created. When [content_type] in the ExportAssetsRequest is
* `RESOURCE`, the schema of each table will include RECORD-type columns mapped to the nested
* fields in the Asset.resource.data field of that asset type (up to the 15 nested level BigQuery
* supports (https://cloud.google.com/bigquery/docs/nested-repeated#limitations)). The fields in
* >15 nested levels will be stored in JSON format string as a child column of its parent RECORD
* column. If error occurs when exporting to any table, the whole export call will return an error
* but the export results that already succeed will persist. Example: if exporting to table_type_A
* succeeds when exporting to table_type_B fails during one export call, the results in
* table_type_A will persist and there will not be partial results persisting in a table.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSeparateTablesPerAssetType() {
return separateTablesPerAssetType;
}
/**
* If this flag is `TRUE`, the snapshot results will be written to one or multiple tables, each of
* which contains results of one asset type. The [force] and [partition_spec] fields will apply to
* each of them. Field [table] will be concatenated with "_" and the asset type names (see
* https://cloud.google.com/asset-inventory/docs/supported-asset-types for supported asset types)
* to construct per-asset-type table names, in which all non-alphanumeric characters like "." and
* "/" will be substituted by "_". Example: if field [table] is "mytable" and snapshot results
* contain "storage.googleapis.com/Bucket" assets, the corresponding table name will be
* "mytable_storage_googleapis_com_Bucket". If any of these tables does not exist, a new table
* with the concatenated name will be created. When [content_type] in the ExportAssetsRequest is
* `RESOURCE`, the schema of each table will include RECORD-type columns mapped to the nested
* fields in the Asset.resource.data field of that asset type (up to the 15 nested level BigQuery
* supports (https://cloud.google.com/bigquery/docs/nested-repeated#limitations)). The fields in
* >15 nested levels will be stored in JSON format string as a child column of its parent RECORD
* column. If error occurs when exporting to any table, the whole export call will return an error
* but the export results that already succeed will persist. Example: if exporting to table_type_A
* succeeds when exporting to table_type_B fails during one export call, the results in
* table_type_A will persist and there will not be partial results persisting in a table.
* @param separateTablesPerAssetType separateTablesPerAssetType or {@code null} for none
*/
public BigQueryDestination setSeparateTablesPerAssetType(java.lang.Boolean separateTablesPerAssetType) {
this.separateTablesPerAssetType = separateTablesPerAssetType;
return this;
}
/**
* Required. The BigQuery table to which the snapshot result should be written. If this table does
* not exist, a new table with the given name will be created.
* @return value or {@code null} for none
*/
public java.lang.String getTable() {
return table;
}
/**
* Required. The BigQuery table to which the snapshot result should be written. If this table does
* not exist, a new table with the given name will be created.
* @param table table or {@code null} for none
*/
public BigQueryDestination setTable(java.lang.String table) {
this.table = table;
return this;
}
@Override
public BigQueryDestination set(String fieldName, Object value) {
return (BigQueryDestination) super.set(fieldName, value);
}
@Override
public BigQueryDestination clone() {
return (BigQueryDestination) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy