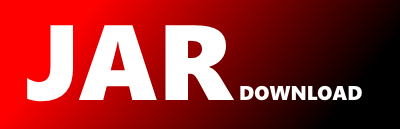
com.google.api.services.cloudasset.v1.model.ResourceSearchResult Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudasset.v1.model;
/**
* A result of Resource Search, containing information of a cloud resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Asset API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ResourceSearchResult extends com.google.api.client.json.GenericJson {
/**
* The additional searchable attributes of this resource. The attributes may vary from one
* resource type to another. Examples: `projectId` for Project, `dnsName` for DNS ManagedZone.
* This field contains a subset of the resource metadata fields that are returned by the List or
* Get APIs provided by the corresponding Google Cloud service (e.g., Compute Engine). see [API
* references and supported searchable attributes](https://cloud.google.com/asset-
* inventory/docs/supported-asset-types) to see which fields are included. You can search values
* of these fields through free text search. However, you should not consume the field
* programically as the field names and values may change as the Google Cloud service updates to a
* new incompatible API version. To search against the `additional_attributes`: * Use a free text
* query to match the attributes values. Example: to search `additional_attributes = { dnsName:
* "foobar" }`, you can issue a query `foobar`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map additionalAttributes;
/**
* The type of this resource. Example: `compute.googleapis.com/Disk`. To search against the
* `asset_type`: * Specify the `asset_type` field in your search request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String assetType;
/**
* Attached resources of this resource. For example, an OSConfig Inventory is an attached resource
* of a Compute Instance. This field is repeated because a resource could have multiple attached
* resources. This `attached_resources` field is not searchable. Some attributes of the attached
* resources are exposed in `additional_attributes` field, so as to allow users to search on them.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List attachedResources;
static {
// hack to force ProGuard to consider AttachedResource used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AttachedResource.class);
}
/**
* The create timestamp of this resource, at which the resource was created. The granularity is in
* seconds. Timestamp.nanos will always be 0. This field is available only when the resource's
* Protobuf contains it. To search against `create_time`: * Use a field query. - value in seconds
* since unix epoch. Example: `createTime > 1609459200` - value in date string. Example:
* `createTime > 2021-01-01` - value in date-time string (must be quoted). Example: `createTime >
* "2021-01-01T00:00:00"`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* One or more paragraphs of text description of this resource. Maximum length could be up to 1M
* bytes. This field is available only when the resource's Protobuf contains it. To search against
* the `description`: * Use a field query. Example: `description:"important instance"` * Use a
* free text query. Example: `"important instance"`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The display name of this resource. This field is available only when the resource's Protobuf
* contains it. To search against the `display_name`: * Use a field query. Example:
* `displayName:"My Instance"` * Use a free text query. Example: `"My Instance"`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* The effective tags on this resource. All of the tags that are both attached to and inherited by
* a resource are collectively called the effective tags. For more information, see [tag
* inheritance](https://cloud.google.com/resource-manager/docs/tags/tags-overview#inheritance). To
* search against the `effective_tags`: * Use a field query. Example: -
* `effectiveTagKeys:"123456789/env*"` - `effectiveTagKeys="123456789/env"` -
* `effectiveTagKeys:"env"` - `effectiveTagKeyIds="tagKeys/123"` - `effectiveTagValues:"env"` -
* `effectiveTagValues:"env/prod"` - `effectiveTagValues:"123456789/env/prod*"` -
* `effectiveTagValues="123456789/env/prod"` - `effectiveTagValueIds="tagValues/456"`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List effectiveTags;
static {
// hack to force ProGuard to consider EffectiveTagDetails used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(EffectiveTagDetails.class);
}
/**
* Enrichments of the asset. Currently supported enrichment types with SearchAllResources API: *
* RESOURCE_OWNERS The corresponding read masks in order to get the enrichment: *
* enrichments.resource_owners The corresponding required permissions: *
* cloudasset.assets.searchEnrichmentResourceOwners Example query to get resource owner
* enrichment: ``` scope: "projects/my-project" query: "name: my-project" assetTypes:
* "cloudresourcemanager.googleapis.com/Project" readMask: { paths: "asset_type" paths: "name"
* paths: "enrichments.resource_owners" } ```
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List enrichments;
static {
// hack to force ProGuard to consider AssetEnrichment used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AssetEnrichment.class);
}
/**
* The folder(s) that this resource belongs to, in the form of folders/{FOLDER_NUMBER}. This field
* is available when the resource belongs to one or more folders. To search against `folders`: *
* Use a field query. Example: `folders:(123 OR 456)` * Use a free text query. Example: `123` *
* Specify the `scope` field as this folder in your search request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List folders;
/**
* The Cloud KMS [CryptoKey](https://cloud.google.com/kms/docs/reference/rest/v1/projects.location
* s.keyRings.cryptoKeys) name or [CryptoKeyVersion](https://cloud.google.com/kms/docs/reference/r
* est/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions) name. This field only presents
* for the purpose of backward compatibility. Use the `kms_keys` field to retrieve Cloud KMS key
* information. This field is available only when the resource's Protobuf contains it and will
* only be populated for [these resource types](https://cloud.google.com/asset-
* inventory/docs/legacy-field-names#resource_types_with_the_to_be_deprecated_kmskey_field) for
* backward compatible purposes. To search against the `kms_key`: * Use a field query. Example:
* `kmsKey:key` * Use a free text query. Example: `key`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kmsKey;
/**
* The Cloud KMS [CryptoKey](https://cloud.google.com/kms/docs/reference/rest/v1/projects.location
* s.keyRings.cryptoKeys) names or [CryptoKeyVersion](https://cloud.google.com/kms/docs/reference/
* rest/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions) names. This field is
* available only when the resource's Protobuf contains it. To search against the `kms_keys`: *
* Use a field query. Example: `kmsKeys:key` * Use a free text query. Example: `key`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List kmsKeys;
/**
* User labels associated with this resource. See [Labelling and grouping Google Cloud
* resources](https://cloud.google.com/blog/products/gcp/labelling-and-grouping-your-google-cloud-
* platform-resources) for more information. This field is available only when the resource's
* Protobuf contains it. To search against the `labels`: * Use a field query: - query on any
* label's key or value. Example: `labels:prod` - query by a given label. Example:
* `labels.env:prod` - query by a given label's existence. Example: `labels.env:*` * Use a free
* text query. Example: `prod`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Location can be `global`, regional like `us-east1`, or zonal like `us-west1-b`. This field is
* available only when the resource's Protobuf contains it. To search against the `location`: *
* Use a field query. Example: `location:us-west*` * Use a free text query. Example: `us-west*`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String location;
/**
* The full resource name of this resource. Example:
* `//compute.googleapis.com/projects/my_project_123/zones/zone1/instances/instance1`. See [Cloud
* Asset Inventory Resource Name Format](https://cloud.google.com/asset-inventory/docs/resource-
* name-format) for more information. To search against the `name`: * Use a field query. Example:
* `name:instance1` * Use a free text query. Example: `instance1`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Network tags associated with this resource. Like labels, network tags are a type of annotations
* used to group Google Cloud resources. See [Labelling Google Cloud
* resources](https://cloud.google.com/blog/products/gcp/labelling-and-grouping-your-google-cloud-
* platform-resources) for more information. This field is available only when the resource's
* Protobuf contains it. To search against the `network_tags`: * Use a field query. Example:
* `networkTags:internal` * Use a free text query. Example: `internal`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List networkTags;
/**
* The organization that this resource belongs to, in the form of
* organizations/{ORGANIZATION_NUMBER}. This field is available when the resource belongs to an
* organization. To search against `organization`: * Use a field query. Example:
* `organization:123` * Use a free text query. Example: `123` * Specify the `scope` field as this
* organization in your search request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String organization;
/**
* The type of this resource's immediate parent, if there is one. To search against the
* `parent_asset_type`: * Use a field query. Example:
* `parentAssetType:"cloudresourcemanager.googleapis.com/Project"` * Use a free text query.
* Example: `cloudresourcemanager.googleapis.com/Project`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String parentAssetType;
/**
* The full resource name of this resource's parent, if it has one. To search against the
* `parent_full_resource_name`: * Use a field query. Example: `parentFullResourceName:"project-
* name"` * Use a free text query. Example: `project-name`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String parentFullResourceName;
/**
* The project that this resource belongs to, in the form of projects/{PROJECT_NUMBER}. This field
* is available when the resource belongs to a project. To search against `project`: * Use a field
* query. Example: `project:12345` * Use a free text query. Example: `12345` * Specify the `scope`
* field as this project in your search request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String project;
/**
* A map of related resources of this resource, keyed by the relationship type. A relationship
* type is in the format of {SourceType}_{ACTION}_{DestType}. Example: `DISK_TO_INSTANCE`,
* `DISK_TO_NETWORK`, `INSTANCE_TO_INSTANCEGROUP`. See [supported relationship
* types](https://cloud.google.com/asset-inventory/docs/supported-asset-
* types#supported_relationship_types).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map relationships;
static {
// hack to force ProGuard to consider RelatedResources used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(RelatedResources.class);
}
/**
* The actual content of Security Command Center security marks associated with the asset. To
* search against SCC SecurityMarks field: * Use a field query: - query by a given key value pair.
* Example: `sccSecurityMarks.foo=bar` - query by a given key's existence. Example:
* `sccSecurityMarks.foo:*`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map sccSecurityMarks;
/**
* The state of this resource. Different resources types have different state definitions that are
* mapped from various fields of different resource types. This field is available only when the
* resource's Protobuf contains it. Example: If the resource is an instance provided by Compute
* Engine, its state will include PROVISIONING, STAGING, RUNNING, STOPPING, SUSPENDING, SUSPENDED,
* REPAIRING, and TERMINATED. See `status` definition in [API
* Reference](https://cloud.google.com/compute/docs/reference/rest/v1/instances). If the resource
* is a project provided by Resource Manager, its state will include LIFECYCLE_STATE_UNSPECIFIED,
* ACTIVE, DELETE_REQUESTED and DELETE_IN_PROGRESS. See `lifecycleState` definition in [API
* Reference](https://cloud.google.com/resource-manager/reference/rest/v1/projects). To search
* against the `state`: * Use a field query. Example: `state:RUNNING` * Use a free text query.
* Example: `RUNNING`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagKey namespaced names, in the format of {ORG_ID}/{TAG_KEY_SHORT_NAME}. To search
* against the `tagKeys`: * Use a field query. Example: - `tagKeys:"123456789/env*"` -
* `tagKeys="123456789/env"` - `tagKeys:"env"` * Use a free text query. Example: - `env`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tagKeys;
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagValue IDs, in the format of tagValues/{TAG_VALUE_ID}. To search against the
* `tagValueIds`: * Use a field query. Example: - `tagValueIds="tagValues/456"` * Use a free text
* query. Example: - `456`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tagValueIds;
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagValue namespaced names, in the format of
* {ORG_ID}/{TAG_KEY_SHORT_NAME}/{TAG_VALUE_SHORT_NAME}. To search against the `tagValues`: * Use
* a field query. Example: - `tagValues:"env"` - `tagValues:"env/prod"` -
* `tagValues:"123456789/env/prod*"` - `tagValues="123456789/env/prod"` * Use a free text query.
* Example: - `prod`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tagValues;
/**
* The tags directly attached to this resource. To search against the `tags`: * Use a field query.
* Example: - `tagKeys:"123456789/env*"` - `tagKeys="123456789/env"` - `tagKeys:"env"` -
* `tagKeyIds="tagKeys/123"` - `tagValues:"env"` - `tagValues:"env/prod"` -
* `tagValues:"123456789/env/prod*"` - `tagValues="123456789/env/prod"` -
* `tagValueIds="tagValues/456"` * Use a free text query. Example: - `env/prod`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tags;
/**
* The last update timestamp of this resource, at which the resource was last modified or deleted.
* The granularity is in seconds. Timestamp.nanos will always be 0. This field is available only
* when the resource's Protobuf contains it. To search against `update_time`: * Use a field query.
* - value in seconds since unix epoch. Example: `updateTime < 1609459200` - value in date string.
* Example: `updateTime < 2021-01-01` - value in date-time string (must be quoted). Example:
* `updateTime < "2021-01-01T00:00:00"`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Versioned resource representations of this resource. This is repeated because there could be
* multiple versions of resource representations during version migration. This
* `versioned_resources` field is not searchable. Some attributes of the resource representations
* are exposed in `additional_attributes` field, so as to allow users to search on them.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List versionedResources;
/**
* The additional searchable attributes of this resource. The attributes may vary from one
* resource type to another. Examples: `projectId` for Project, `dnsName` for DNS ManagedZone.
* This field contains a subset of the resource metadata fields that are returned by the List or
* Get APIs provided by the corresponding Google Cloud service (e.g., Compute Engine). see [API
* references and supported searchable attributes](https://cloud.google.com/asset-
* inventory/docs/supported-asset-types) to see which fields are included. You can search values
* of these fields through free text search. However, you should not consume the field
* programically as the field names and values may change as the Google Cloud service updates to a
* new incompatible API version. To search against the `additional_attributes`: * Use a free text
* query to match the attributes values. Example: to search `additional_attributes = { dnsName:
* "foobar" }`, you can issue a query `foobar`.
* @return value or {@code null} for none
*/
public java.util.Map getAdditionalAttributes() {
return additionalAttributes;
}
/**
* The additional searchable attributes of this resource. The attributes may vary from one
* resource type to another. Examples: `projectId` for Project, `dnsName` for DNS ManagedZone.
* This field contains a subset of the resource metadata fields that are returned by the List or
* Get APIs provided by the corresponding Google Cloud service (e.g., Compute Engine). see [API
* references and supported searchable attributes](https://cloud.google.com/asset-
* inventory/docs/supported-asset-types) to see which fields are included. You can search values
* of these fields through free text search. However, you should not consume the field
* programically as the field names and values may change as the Google Cloud service updates to a
* new incompatible API version. To search against the `additional_attributes`: * Use a free text
* query to match the attributes values. Example: to search `additional_attributes = { dnsName:
* "foobar" }`, you can issue a query `foobar`.
* @param additionalAttributes additionalAttributes or {@code null} for none
*/
public ResourceSearchResult setAdditionalAttributes(java.util.Map additionalAttributes) {
this.additionalAttributes = additionalAttributes;
return this;
}
/**
* The type of this resource. Example: `compute.googleapis.com/Disk`. To search against the
* `asset_type`: * Specify the `asset_type` field in your search request.
* @return value or {@code null} for none
*/
public java.lang.String getAssetType() {
return assetType;
}
/**
* The type of this resource. Example: `compute.googleapis.com/Disk`. To search against the
* `asset_type`: * Specify the `asset_type` field in your search request.
* @param assetType assetType or {@code null} for none
*/
public ResourceSearchResult setAssetType(java.lang.String assetType) {
this.assetType = assetType;
return this;
}
/**
* Attached resources of this resource. For example, an OSConfig Inventory is an attached resource
* of a Compute Instance. This field is repeated because a resource could have multiple attached
* resources. This `attached_resources` field is not searchable. Some attributes of the attached
* resources are exposed in `additional_attributes` field, so as to allow users to search on them.
* @return value or {@code null} for none
*/
public java.util.List getAttachedResources() {
return attachedResources;
}
/**
* Attached resources of this resource. For example, an OSConfig Inventory is an attached resource
* of a Compute Instance. This field is repeated because a resource could have multiple attached
* resources. This `attached_resources` field is not searchable. Some attributes of the attached
* resources are exposed in `additional_attributes` field, so as to allow users to search on them.
* @param attachedResources attachedResources or {@code null} for none
*/
public ResourceSearchResult setAttachedResources(java.util.List attachedResources) {
this.attachedResources = attachedResources;
return this;
}
/**
* The create timestamp of this resource, at which the resource was created. The granularity is in
* seconds. Timestamp.nanos will always be 0. This field is available only when the resource's
* Protobuf contains it. To search against `create_time`: * Use a field query. - value in seconds
* since unix epoch. Example: `createTime > 1609459200` - value in date string. Example:
* `createTime > 2021-01-01` - value in date-time string (must be quoted). Example: `createTime >
* "2021-01-01T00:00:00"`
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* The create timestamp of this resource, at which the resource was created. The granularity is in
* seconds. Timestamp.nanos will always be 0. This field is available only when the resource's
* Protobuf contains it. To search against `create_time`: * Use a field query. - value in seconds
* since unix epoch. Example: `createTime > 1609459200` - value in date string. Example:
* `createTime > 2021-01-01` - value in date-time string (must be quoted). Example: `createTime >
* "2021-01-01T00:00:00"`
* @param createTime createTime or {@code null} for none
*/
public ResourceSearchResult setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* One or more paragraphs of text description of this resource. Maximum length could be up to 1M
* bytes. This field is available only when the resource's Protobuf contains it. To search against
* the `description`: * Use a field query. Example: `description:"important instance"` * Use a
* free text query. Example: `"important instance"`
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* One or more paragraphs of text description of this resource. Maximum length could be up to 1M
* bytes. This field is available only when the resource's Protobuf contains it. To search against
* the `description`: * Use a field query. Example: `description:"important instance"` * Use a
* free text query. Example: `"important instance"`
* @param description description or {@code null} for none
*/
public ResourceSearchResult setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The display name of this resource. This field is available only when the resource's Protobuf
* contains it. To search against the `display_name`: * Use a field query. Example:
* `displayName:"My Instance"` * Use a free text query. Example: `"My Instance"`
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The display name of this resource. This field is available only when the resource's Protobuf
* contains it. To search against the `display_name`: * Use a field query. Example:
* `displayName:"My Instance"` * Use a free text query. Example: `"My Instance"`
* @param displayName displayName or {@code null} for none
*/
public ResourceSearchResult setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* The effective tags on this resource. All of the tags that are both attached to and inherited by
* a resource are collectively called the effective tags. For more information, see [tag
* inheritance](https://cloud.google.com/resource-manager/docs/tags/tags-overview#inheritance). To
* search against the `effective_tags`: * Use a field query. Example: -
* `effectiveTagKeys:"123456789/env*"` - `effectiveTagKeys="123456789/env"` -
* `effectiveTagKeys:"env"` - `effectiveTagKeyIds="tagKeys/123"` - `effectiveTagValues:"env"` -
* `effectiveTagValues:"env/prod"` - `effectiveTagValues:"123456789/env/prod*"` -
* `effectiveTagValues="123456789/env/prod"` - `effectiveTagValueIds="tagValues/456"`
* @return value or {@code null} for none
*/
public java.util.List getEffectiveTags() {
return effectiveTags;
}
/**
* The effective tags on this resource. All of the tags that are both attached to and inherited by
* a resource are collectively called the effective tags. For more information, see [tag
* inheritance](https://cloud.google.com/resource-manager/docs/tags/tags-overview#inheritance). To
* search against the `effective_tags`: * Use a field query. Example: -
* `effectiveTagKeys:"123456789/env*"` - `effectiveTagKeys="123456789/env"` -
* `effectiveTagKeys:"env"` - `effectiveTagKeyIds="tagKeys/123"` - `effectiveTagValues:"env"` -
* `effectiveTagValues:"env/prod"` - `effectiveTagValues:"123456789/env/prod*"` -
* `effectiveTagValues="123456789/env/prod"` - `effectiveTagValueIds="tagValues/456"`
* @param effectiveTags effectiveTags or {@code null} for none
*/
public ResourceSearchResult setEffectiveTags(java.util.List effectiveTags) {
this.effectiveTags = effectiveTags;
return this;
}
/**
* Enrichments of the asset. Currently supported enrichment types with SearchAllResources API: *
* RESOURCE_OWNERS The corresponding read masks in order to get the enrichment: *
* enrichments.resource_owners The corresponding required permissions: *
* cloudasset.assets.searchEnrichmentResourceOwners Example query to get resource owner
* enrichment: ``` scope: "projects/my-project" query: "name: my-project" assetTypes:
* "cloudresourcemanager.googleapis.com/Project" readMask: { paths: "asset_type" paths: "name"
* paths: "enrichments.resource_owners" } ```
* @return value or {@code null} for none
*/
public java.util.List getEnrichments() {
return enrichments;
}
/**
* Enrichments of the asset. Currently supported enrichment types with SearchAllResources API: *
* RESOURCE_OWNERS The corresponding read masks in order to get the enrichment: *
* enrichments.resource_owners The corresponding required permissions: *
* cloudasset.assets.searchEnrichmentResourceOwners Example query to get resource owner
* enrichment: ``` scope: "projects/my-project" query: "name: my-project" assetTypes:
* "cloudresourcemanager.googleapis.com/Project" readMask: { paths: "asset_type" paths: "name"
* paths: "enrichments.resource_owners" } ```
* @param enrichments enrichments or {@code null} for none
*/
public ResourceSearchResult setEnrichments(java.util.List enrichments) {
this.enrichments = enrichments;
return this;
}
/**
* The folder(s) that this resource belongs to, in the form of folders/{FOLDER_NUMBER}. This field
* is available when the resource belongs to one or more folders. To search against `folders`: *
* Use a field query. Example: `folders:(123 OR 456)` * Use a free text query. Example: `123` *
* Specify the `scope` field as this folder in your search request.
* @return value or {@code null} for none
*/
public java.util.List getFolders() {
return folders;
}
/**
* The folder(s) that this resource belongs to, in the form of folders/{FOLDER_NUMBER}. This field
* is available when the resource belongs to one or more folders. To search against `folders`: *
* Use a field query. Example: `folders:(123 OR 456)` * Use a free text query. Example: `123` *
* Specify the `scope` field as this folder in your search request.
* @param folders folders or {@code null} for none
*/
public ResourceSearchResult setFolders(java.util.List folders) {
this.folders = folders;
return this;
}
/**
* The Cloud KMS [CryptoKey](https://cloud.google.com/kms/docs/reference/rest/v1/projects.location
* s.keyRings.cryptoKeys) name or [CryptoKeyVersion](https://cloud.google.com/kms/docs/reference/r
* est/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions) name. This field only presents
* for the purpose of backward compatibility. Use the `kms_keys` field to retrieve Cloud KMS key
* information. This field is available only when the resource's Protobuf contains it and will
* only be populated for [these resource types](https://cloud.google.com/asset-
* inventory/docs/legacy-field-names#resource_types_with_the_to_be_deprecated_kmskey_field) for
* backward compatible purposes. To search against the `kms_key`: * Use a field query. Example:
* `kmsKey:key` * Use a free text query. Example: `key`
* @return value or {@code null} for none
*/
public java.lang.String getKmsKey() {
return kmsKey;
}
/**
* The Cloud KMS [CryptoKey](https://cloud.google.com/kms/docs/reference/rest/v1/projects.location
* s.keyRings.cryptoKeys) name or [CryptoKeyVersion](https://cloud.google.com/kms/docs/reference/r
* est/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions) name. This field only presents
* for the purpose of backward compatibility. Use the `kms_keys` field to retrieve Cloud KMS key
* information. This field is available only when the resource's Protobuf contains it and will
* only be populated for [these resource types](https://cloud.google.com/asset-
* inventory/docs/legacy-field-names#resource_types_with_the_to_be_deprecated_kmskey_field) for
* backward compatible purposes. To search against the `kms_key`: * Use a field query. Example:
* `kmsKey:key` * Use a free text query. Example: `key`
* @param kmsKey kmsKey or {@code null} for none
*/
public ResourceSearchResult setKmsKey(java.lang.String kmsKey) {
this.kmsKey = kmsKey;
return this;
}
/**
* The Cloud KMS [CryptoKey](https://cloud.google.com/kms/docs/reference/rest/v1/projects.location
* s.keyRings.cryptoKeys) names or [CryptoKeyVersion](https://cloud.google.com/kms/docs/reference/
* rest/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions) names. This field is
* available only when the resource's Protobuf contains it. To search against the `kms_keys`: *
* Use a field query. Example: `kmsKeys:key` * Use a free text query. Example: `key`
* @return value or {@code null} for none
*/
public java.util.List getKmsKeys() {
return kmsKeys;
}
/**
* The Cloud KMS [CryptoKey](https://cloud.google.com/kms/docs/reference/rest/v1/projects.location
* s.keyRings.cryptoKeys) names or [CryptoKeyVersion](https://cloud.google.com/kms/docs/reference/
* rest/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions) names. This field is
* available only when the resource's Protobuf contains it. To search against the `kms_keys`: *
* Use a field query. Example: `kmsKeys:key` * Use a free text query. Example: `key`
* @param kmsKeys kmsKeys or {@code null} for none
*/
public ResourceSearchResult setKmsKeys(java.util.List kmsKeys) {
this.kmsKeys = kmsKeys;
return this;
}
/**
* User labels associated with this resource. See [Labelling and grouping Google Cloud
* resources](https://cloud.google.com/blog/products/gcp/labelling-and-grouping-your-google-cloud-
* platform-resources) for more information. This field is available only when the resource's
* Protobuf contains it. To search against the `labels`: * Use a field query: - query on any
* label's key or value. Example: `labels:prod` - query by a given label. Example:
* `labels.env:prod` - query by a given label's existence. Example: `labels.env:*` * Use a free
* text query. Example: `prod`
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* User labels associated with this resource. See [Labelling and grouping Google Cloud
* resources](https://cloud.google.com/blog/products/gcp/labelling-and-grouping-your-google-cloud-
* platform-resources) for more information. This field is available only when the resource's
* Protobuf contains it. To search against the `labels`: * Use a field query: - query on any
* label's key or value. Example: `labels:prod` - query by a given label. Example:
* `labels.env:prod` - query by a given label's existence. Example: `labels.env:*` * Use a free
* text query. Example: `prod`
* @param labels labels or {@code null} for none
*/
public ResourceSearchResult setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Location can be `global`, regional like `us-east1`, or zonal like `us-west1-b`. This field is
* available only when the resource's Protobuf contains it. To search against the `location`: *
* Use a field query. Example: `location:us-west*` * Use a free text query. Example: `us-west*`
* @return value or {@code null} for none
*/
public java.lang.String getLocation() {
return location;
}
/**
* Location can be `global`, regional like `us-east1`, or zonal like `us-west1-b`. This field is
* available only when the resource's Protobuf contains it. To search against the `location`: *
* Use a field query. Example: `location:us-west*` * Use a free text query. Example: `us-west*`
* @param location location or {@code null} for none
*/
public ResourceSearchResult setLocation(java.lang.String location) {
this.location = location;
return this;
}
/**
* The full resource name of this resource. Example:
* `//compute.googleapis.com/projects/my_project_123/zones/zone1/instances/instance1`. See [Cloud
* Asset Inventory Resource Name Format](https://cloud.google.com/asset-inventory/docs/resource-
* name-format) for more information. To search against the `name`: * Use a field query. Example:
* `name:instance1` * Use a free text query. Example: `instance1`
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The full resource name of this resource. Example:
* `//compute.googleapis.com/projects/my_project_123/zones/zone1/instances/instance1`. See [Cloud
* Asset Inventory Resource Name Format](https://cloud.google.com/asset-inventory/docs/resource-
* name-format) for more information. To search against the `name`: * Use a field query. Example:
* `name:instance1` * Use a free text query. Example: `instance1`
* @param name name or {@code null} for none
*/
public ResourceSearchResult setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Network tags associated with this resource. Like labels, network tags are a type of annotations
* used to group Google Cloud resources. See [Labelling Google Cloud
* resources](https://cloud.google.com/blog/products/gcp/labelling-and-grouping-your-google-cloud-
* platform-resources) for more information. This field is available only when the resource's
* Protobuf contains it. To search against the `network_tags`: * Use a field query. Example:
* `networkTags:internal` * Use a free text query. Example: `internal`
* @return value or {@code null} for none
*/
public java.util.List getNetworkTags() {
return networkTags;
}
/**
* Network tags associated with this resource. Like labels, network tags are a type of annotations
* used to group Google Cloud resources. See [Labelling Google Cloud
* resources](https://cloud.google.com/blog/products/gcp/labelling-and-grouping-your-google-cloud-
* platform-resources) for more information. This field is available only when the resource's
* Protobuf contains it. To search against the `network_tags`: * Use a field query. Example:
* `networkTags:internal` * Use a free text query. Example: `internal`
* @param networkTags networkTags or {@code null} for none
*/
public ResourceSearchResult setNetworkTags(java.util.List networkTags) {
this.networkTags = networkTags;
return this;
}
/**
* The organization that this resource belongs to, in the form of
* organizations/{ORGANIZATION_NUMBER}. This field is available when the resource belongs to an
* organization. To search against `organization`: * Use a field query. Example:
* `organization:123` * Use a free text query. Example: `123` * Specify the `scope` field as this
* organization in your search request.
* @return value or {@code null} for none
*/
public java.lang.String getOrganization() {
return organization;
}
/**
* The organization that this resource belongs to, in the form of
* organizations/{ORGANIZATION_NUMBER}. This field is available when the resource belongs to an
* organization. To search against `organization`: * Use a field query. Example:
* `organization:123` * Use a free text query. Example: `123` * Specify the `scope` field as this
* organization in your search request.
* @param organization organization or {@code null} for none
*/
public ResourceSearchResult setOrganization(java.lang.String organization) {
this.organization = organization;
return this;
}
/**
* The type of this resource's immediate parent, if there is one. To search against the
* `parent_asset_type`: * Use a field query. Example:
* `parentAssetType:"cloudresourcemanager.googleapis.com/Project"` * Use a free text query.
* Example: `cloudresourcemanager.googleapis.com/Project`
* @return value or {@code null} for none
*/
public java.lang.String getParentAssetType() {
return parentAssetType;
}
/**
* The type of this resource's immediate parent, if there is one. To search against the
* `parent_asset_type`: * Use a field query. Example:
* `parentAssetType:"cloudresourcemanager.googleapis.com/Project"` * Use a free text query.
* Example: `cloudresourcemanager.googleapis.com/Project`
* @param parentAssetType parentAssetType or {@code null} for none
*/
public ResourceSearchResult setParentAssetType(java.lang.String parentAssetType) {
this.parentAssetType = parentAssetType;
return this;
}
/**
* The full resource name of this resource's parent, if it has one. To search against the
* `parent_full_resource_name`: * Use a field query. Example: `parentFullResourceName:"project-
* name"` * Use a free text query. Example: `project-name`
* @return value or {@code null} for none
*/
public java.lang.String getParentFullResourceName() {
return parentFullResourceName;
}
/**
* The full resource name of this resource's parent, if it has one. To search against the
* `parent_full_resource_name`: * Use a field query. Example: `parentFullResourceName:"project-
* name"` * Use a free text query. Example: `project-name`
* @param parentFullResourceName parentFullResourceName or {@code null} for none
*/
public ResourceSearchResult setParentFullResourceName(java.lang.String parentFullResourceName) {
this.parentFullResourceName = parentFullResourceName;
return this;
}
/**
* The project that this resource belongs to, in the form of projects/{PROJECT_NUMBER}. This field
* is available when the resource belongs to a project. To search against `project`: * Use a field
* query. Example: `project:12345` * Use a free text query. Example: `12345` * Specify the `scope`
* field as this project in your search request.
* @return value or {@code null} for none
*/
public java.lang.String getProject() {
return project;
}
/**
* The project that this resource belongs to, in the form of projects/{PROJECT_NUMBER}. This field
* is available when the resource belongs to a project. To search against `project`: * Use a field
* query. Example: `project:12345` * Use a free text query. Example: `12345` * Specify the `scope`
* field as this project in your search request.
* @param project project or {@code null} for none
*/
public ResourceSearchResult setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* A map of related resources of this resource, keyed by the relationship type. A relationship
* type is in the format of {SourceType}_{ACTION}_{DestType}. Example: `DISK_TO_INSTANCE`,
* `DISK_TO_NETWORK`, `INSTANCE_TO_INSTANCEGROUP`. See [supported relationship
* types](https://cloud.google.com/asset-inventory/docs/supported-asset-
* types#supported_relationship_types).
* @return value or {@code null} for none
*/
public java.util.Map getRelationships() {
return relationships;
}
/**
* A map of related resources of this resource, keyed by the relationship type. A relationship
* type is in the format of {SourceType}_{ACTION}_{DestType}. Example: `DISK_TO_INSTANCE`,
* `DISK_TO_NETWORK`, `INSTANCE_TO_INSTANCEGROUP`. See [supported relationship
* types](https://cloud.google.com/asset-inventory/docs/supported-asset-
* types#supported_relationship_types).
* @param relationships relationships or {@code null} for none
*/
public ResourceSearchResult setRelationships(java.util.Map relationships) {
this.relationships = relationships;
return this;
}
/**
* The actual content of Security Command Center security marks associated with the asset. To
* search against SCC SecurityMarks field: * Use a field query: - query by a given key value pair.
* Example: `sccSecurityMarks.foo=bar` - query by a given key's existence. Example:
* `sccSecurityMarks.foo:*`
* @return value or {@code null} for none
*/
public java.util.Map getSccSecurityMarks() {
return sccSecurityMarks;
}
/**
* The actual content of Security Command Center security marks associated with the asset. To
* search against SCC SecurityMarks field: * Use a field query: - query by a given key value pair.
* Example: `sccSecurityMarks.foo=bar` - query by a given key's existence. Example:
* `sccSecurityMarks.foo:*`
* @param sccSecurityMarks sccSecurityMarks or {@code null} for none
*/
public ResourceSearchResult setSccSecurityMarks(java.util.Map sccSecurityMarks) {
this.sccSecurityMarks = sccSecurityMarks;
return this;
}
/**
* The state of this resource. Different resources types have different state definitions that are
* mapped from various fields of different resource types. This field is available only when the
* resource's Protobuf contains it. Example: If the resource is an instance provided by Compute
* Engine, its state will include PROVISIONING, STAGING, RUNNING, STOPPING, SUSPENDING, SUSPENDED,
* REPAIRING, and TERMINATED. See `status` definition in [API
* Reference](https://cloud.google.com/compute/docs/reference/rest/v1/instances). If the resource
* is a project provided by Resource Manager, its state will include LIFECYCLE_STATE_UNSPECIFIED,
* ACTIVE, DELETE_REQUESTED and DELETE_IN_PROGRESS. See `lifecycleState` definition in [API
* Reference](https://cloud.google.com/resource-manager/reference/rest/v1/projects). To search
* against the `state`: * Use a field query. Example: `state:RUNNING` * Use a free text query.
* Example: `RUNNING`
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* The state of this resource. Different resources types have different state definitions that are
* mapped from various fields of different resource types. This field is available only when the
* resource's Protobuf contains it. Example: If the resource is an instance provided by Compute
* Engine, its state will include PROVISIONING, STAGING, RUNNING, STOPPING, SUSPENDING, SUSPENDED,
* REPAIRING, and TERMINATED. See `status` definition in [API
* Reference](https://cloud.google.com/compute/docs/reference/rest/v1/instances). If the resource
* is a project provided by Resource Manager, its state will include LIFECYCLE_STATE_UNSPECIFIED,
* ACTIVE, DELETE_REQUESTED and DELETE_IN_PROGRESS. See `lifecycleState` definition in [API
* Reference](https://cloud.google.com/resource-manager/reference/rest/v1/projects). To search
* against the `state`: * Use a field query. Example: `state:RUNNING` * Use a free text query.
* Example: `RUNNING`
* @param state state or {@code null} for none
*/
public ResourceSearchResult setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagKey namespaced names, in the format of {ORG_ID}/{TAG_KEY_SHORT_NAME}. To search
* against the `tagKeys`: * Use a field query. Example: - `tagKeys:"123456789/env*"` -
* `tagKeys="123456789/env"` - `tagKeys:"env"` * Use a free text query. Example: - `env`
* @return value or {@code null} for none
*/
public java.util.List getTagKeys() {
return tagKeys;
}
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagKey namespaced names, in the format of {ORG_ID}/{TAG_KEY_SHORT_NAME}. To search
* against the `tagKeys`: * Use a field query. Example: - `tagKeys:"123456789/env*"` -
* `tagKeys="123456789/env"` - `tagKeys:"env"` * Use a free text query. Example: - `env`
* @param tagKeys tagKeys or {@code null} for none
*/
public ResourceSearchResult setTagKeys(java.util.List tagKeys) {
this.tagKeys = tagKeys;
return this;
}
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagValue IDs, in the format of tagValues/{TAG_VALUE_ID}. To search against the
* `tagValueIds`: * Use a field query. Example: - `tagValueIds="tagValues/456"` * Use a free text
* query. Example: - `456`
* @return value or {@code null} for none
*/
public java.util.List getTagValueIds() {
return tagValueIds;
}
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagValue IDs, in the format of tagValues/{TAG_VALUE_ID}. To search against the
* `tagValueIds`: * Use a field query. Example: - `tagValueIds="tagValues/456"` * Use a free text
* query. Example: - `456`
* @param tagValueIds tagValueIds or {@code null} for none
*/
public ResourceSearchResult setTagValueIds(java.util.List tagValueIds) {
this.tagValueIds = tagValueIds;
return this;
}
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagValue namespaced names, in the format of
* {ORG_ID}/{TAG_KEY_SHORT_NAME}/{TAG_VALUE_SHORT_NAME}. To search against the `tagValues`: * Use
* a field query. Example: - `tagValues:"env"` - `tagValues:"env/prod"` -
* `tagValues:"123456789/env/prod*"` - `tagValues="123456789/env/prod"` * Use a free text query.
* Example: - `prod`
* @return value or {@code null} for none
*/
public java.util.List getTagValues() {
return tagValues;
}
/**
* This field is only present for the purpose of backward compatibility. Use the `tags` field
* instead. TagValue namespaced names, in the format of
* {ORG_ID}/{TAG_KEY_SHORT_NAME}/{TAG_VALUE_SHORT_NAME}. To search against the `tagValues`: * Use
* a field query. Example: - `tagValues:"env"` - `tagValues:"env/prod"` -
* `tagValues:"123456789/env/prod*"` - `tagValues="123456789/env/prod"` * Use a free text query.
* Example: - `prod`
* @param tagValues tagValues or {@code null} for none
*/
public ResourceSearchResult setTagValues(java.util.List tagValues) {
this.tagValues = tagValues;
return this;
}
/**
* The tags directly attached to this resource. To search against the `tags`: * Use a field query.
* Example: - `tagKeys:"123456789/env*"` - `tagKeys="123456789/env"` - `tagKeys:"env"` -
* `tagKeyIds="tagKeys/123"` - `tagValues:"env"` - `tagValues:"env/prod"` -
* `tagValues:"123456789/env/prod*"` - `tagValues="123456789/env/prod"` -
* `tagValueIds="tagValues/456"` * Use a free text query. Example: - `env/prod`
* @return value or {@code null} for none
*/
public java.util.List getTags() {
return tags;
}
/**
* The tags directly attached to this resource. To search against the `tags`: * Use a field query.
* Example: - `tagKeys:"123456789/env*"` - `tagKeys="123456789/env"` - `tagKeys:"env"` -
* `tagKeyIds="tagKeys/123"` - `tagValues:"env"` - `tagValues:"env/prod"` -
* `tagValues:"123456789/env/prod*"` - `tagValues="123456789/env/prod"` -
* `tagValueIds="tagValues/456"` * Use a free text query. Example: - `env/prod`
* @param tags tags or {@code null} for none
*/
public ResourceSearchResult setTags(java.util.List tags) {
this.tags = tags;
return this;
}
/**
* The last update timestamp of this resource, at which the resource was last modified or deleted.
* The granularity is in seconds. Timestamp.nanos will always be 0. This field is available only
* when the resource's Protobuf contains it. To search against `update_time`: * Use a field query.
* - value in seconds since unix epoch. Example: `updateTime < 1609459200` - value in date string.
* Example: `updateTime < 2021-01-01` - value in date-time string (must be quoted). Example:
* `updateTime < "2021-01-01T00:00:00"`
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* The last update timestamp of this resource, at which the resource was last modified or deleted.
* The granularity is in seconds. Timestamp.nanos will always be 0. This field is available only
* when the resource's Protobuf contains it. To search against `update_time`: * Use a field query.
* - value in seconds since unix epoch. Example: `updateTime < 1609459200` - value in date string.
* Example: `updateTime < 2021-01-01` - value in date-time string (must be quoted). Example:
* `updateTime < "2021-01-01T00:00:00"`
* @param updateTime updateTime or {@code null} for none
*/
public ResourceSearchResult setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* Versioned resource representations of this resource. This is repeated because there could be
* multiple versions of resource representations during version migration. This
* `versioned_resources` field is not searchable. Some attributes of the resource representations
* are exposed in `additional_attributes` field, so as to allow users to search on them.
* @return value or {@code null} for none
*/
public java.util.List getVersionedResources() {
return versionedResources;
}
/**
* Versioned resource representations of this resource. This is repeated because there could be
* multiple versions of resource representations during version migration. This
* `versioned_resources` field is not searchable. Some attributes of the resource representations
* are exposed in `additional_attributes` field, so as to allow users to search on them.
* @param versionedResources versionedResources or {@code null} for none
*/
public ResourceSearchResult setVersionedResources(java.util.List versionedResources) {
this.versionedResources = versionedResources;
return this;
}
@Override
public ResourceSearchResult set(String fieldName, Object value) {
return (ResourceSearchResult) super.set(fieldName, value);
}
@Override
public ResourceSearchResult clone() {
return (ResourceSearchResult) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy