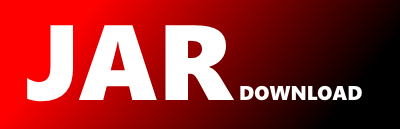
com.google.api.services.cloudchannel.v1.Cloudchannel Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudchannel.v1;
/**
* Service definition for Cloudchannel (v1).
*
*
* The Cloud Channel API enables Google Cloud partners to have a single unified resale platform and APIs across all of Google Cloud including GCP, Workspace, Maps and Chrome.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudchannelRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Cloudchannel extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Channel API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://cloudchannel.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://cloudchannel.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Cloudchannel(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Cloudchannel(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Accounts collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Accounts.List request = cloudchannel.accounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounts accounts() {
return new Accounts();
}
/**
* The "accounts" collection of methods.
*/
public class Accounts {
/**
* Confirms the existence of Cloud Identity accounts based on the domain and if the Cloud Identity
* accounts are owned by the reseller. Possible error codes: * PERMISSION_DENIED: The reseller
* account making the request is different from the reseller account in the API request. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * INVALID_VALUE: Invalid
* domain value in the request. Return value: A list of CloudIdentityCustomerAccount resources for
* the domain (may be empty) Note: in the v1alpha1 version of the API, a NOT_FOUND error returns if
* no CloudIdentityCustomerAccount resources match the domain.
*
* Create a request for the method "accounts.checkCloudIdentityAccountsExist".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link CheckCloudIdentityAccountsExist#execute()} method to invoke the
* remote operation.
*
* @param parent Required. The reseller account's resource name. Parent uses the format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CheckCloudIdentityAccountsExistRequest}
* @return the request
*/
public CheckCloudIdentityAccountsExist checkCloudIdentityAccountsExist(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CheckCloudIdentityAccountsExistRequest content) throws java.io.IOException {
CheckCloudIdentityAccountsExist result = new CheckCloudIdentityAccountsExist(parent, content);
initialize(result);
return result;
}
public class CheckCloudIdentityAccountsExist extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}:checkCloudIdentityAccountsExist";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Confirms the existence of Cloud Identity accounts based on the domain and if the Cloud Identity
* accounts are owned by the reseller. Possible error codes: * PERMISSION_DENIED: The reseller
* account making the request is different from the reseller account in the API request. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * INVALID_VALUE: Invalid
* domain value in the request. Return value: A list of CloudIdentityCustomerAccount resources for
* the domain (may be empty) Note: in the v1alpha1 version of the API, a NOT_FOUND error returns
* if no CloudIdentityCustomerAccount resources match the domain.
*
* Create a request for the method "accounts.checkCloudIdentityAccountsExist".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link CheckCloudIdentityAccountsExist#execute()} method to
* invoke the remote operation. {@link CheckCloudIdentityAccountsExist#initialize(com.google.a
* pi.client.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this
* instance immediately after invoking the constructor.
*
* @param parent Required. The reseller account's resource name. Parent uses the format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CheckCloudIdentityAccountsExistRequest}
* @since 1.13
*/
protected CheckCloudIdentityAccountsExist(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CheckCloudIdentityAccountsExistRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CheckCloudIdentityAccountsExistResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public CheckCloudIdentityAccountsExist set$Xgafv(java.lang.String $Xgafv) {
return (CheckCloudIdentityAccountsExist) super.set$Xgafv($Xgafv);
}
@Override
public CheckCloudIdentityAccountsExist setAccessToken(java.lang.String accessToken) {
return (CheckCloudIdentityAccountsExist) super.setAccessToken(accessToken);
}
@Override
public CheckCloudIdentityAccountsExist setAlt(java.lang.String alt) {
return (CheckCloudIdentityAccountsExist) super.setAlt(alt);
}
@Override
public CheckCloudIdentityAccountsExist setCallback(java.lang.String callback) {
return (CheckCloudIdentityAccountsExist) super.setCallback(callback);
}
@Override
public CheckCloudIdentityAccountsExist setFields(java.lang.String fields) {
return (CheckCloudIdentityAccountsExist) super.setFields(fields);
}
@Override
public CheckCloudIdentityAccountsExist setKey(java.lang.String key) {
return (CheckCloudIdentityAccountsExist) super.setKey(key);
}
@Override
public CheckCloudIdentityAccountsExist setOauthToken(java.lang.String oauthToken) {
return (CheckCloudIdentityAccountsExist) super.setOauthToken(oauthToken);
}
@Override
public CheckCloudIdentityAccountsExist setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CheckCloudIdentityAccountsExist) super.setPrettyPrint(prettyPrint);
}
@Override
public CheckCloudIdentityAccountsExist setQuotaUser(java.lang.String quotaUser) {
return (CheckCloudIdentityAccountsExist) super.setQuotaUser(quotaUser);
}
@Override
public CheckCloudIdentityAccountsExist setUploadType(java.lang.String uploadType) {
return (CheckCloudIdentityAccountsExist) super.setUploadType(uploadType);
}
@Override
public CheckCloudIdentityAccountsExist setUploadProtocol(java.lang.String uploadProtocol) {
return (CheckCloudIdentityAccountsExist) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The reseller account's resource name. Parent uses the format:
* accounts/{account_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The reseller account's resource name. Parent uses the format: accounts/{account_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The reseller account's resource name. Parent uses the format:
* accounts/{account_id}
*/
public CheckCloudIdentityAccountsExist setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public CheckCloudIdentityAccountsExist set(String parameterName, Object value) {
return (CheckCloudIdentityAccountsExist) super.set(parameterName, value);
}
}
/**
* Lists service accounts with subscriber privileges on the Pub/Sub topic created for this Channel
* Services account or integrator. Possible error codes: * PERMISSION_DENIED: The reseller account
* making the request and the provided reseller account are different, or the impersonated user is
* not a super admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* NOT_FOUND: The topic resource doesn't exist. * INTERNAL: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. Return value: A list
* of service email addresses.
*
* Create a request for the method "accounts.listSubscribers".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ListSubscribers#execute()} method to invoke the remote operation.
*
* @param account Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
* this field empty/unset.
* @return the request
*/
public ListSubscribers listSubscribers(java.lang.String account) throws java.io.IOException {
ListSubscribers result = new ListSubscribers(account);
initialize(result);
return result;
}
public class ListSubscribers extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+account}:listSubscribers";
private final java.util.regex.Pattern ACCOUNT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists service accounts with subscriber privileges on the Pub/Sub topic created for this Channel
* Services account or integrator. Possible error codes: * PERMISSION_DENIED: The reseller account
* making the request and the provided reseller account are different, or the impersonated user is
* not a super admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* NOT_FOUND: The topic resource doesn't exist. * INTERNAL: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. Return value: A
* list of service email addresses.
*
* Create a request for the method "accounts.listSubscribers".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ListSubscribers#execute()} method to invoke the remote
* operation. {@link ListSubscribers#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param account Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
* this field empty/unset.
* @since 1.13
*/
protected ListSubscribers(java.lang.String account) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListSubscribersResponse.class);
this.account = com.google.api.client.util.Preconditions.checkNotNull(account, "Required parameter account must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListSubscribers set$Xgafv(java.lang.String $Xgafv) {
return (ListSubscribers) super.set$Xgafv($Xgafv);
}
@Override
public ListSubscribers setAccessToken(java.lang.String accessToken) {
return (ListSubscribers) super.setAccessToken(accessToken);
}
@Override
public ListSubscribers setAlt(java.lang.String alt) {
return (ListSubscribers) super.setAlt(alt);
}
@Override
public ListSubscribers setCallback(java.lang.String callback) {
return (ListSubscribers) super.setCallback(callback);
}
@Override
public ListSubscribers setFields(java.lang.String fields) {
return (ListSubscribers) super.setFields(fields);
}
@Override
public ListSubscribers setKey(java.lang.String key) {
return (ListSubscribers) super.setKey(key);
}
@Override
public ListSubscribers setOauthToken(java.lang.String oauthToken) {
return (ListSubscribers) super.setOauthToken(oauthToken);
}
@Override
public ListSubscribers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListSubscribers) super.setPrettyPrint(prettyPrint);
}
@Override
public ListSubscribers setQuotaUser(java.lang.String quotaUser) {
return (ListSubscribers) super.setQuotaUser(quotaUser);
}
@Override
public ListSubscribers setUploadType(java.lang.String uploadType) {
return (ListSubscribers) super.setUploadType(uploadType);
}
@Override
public ListSubscribers setUploadProtocol(java.lang.String uploadProtocol) {
return (ListSubscribers) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String account;
/** Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getAccount() {
return account;
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
public ListSubscribers setAccount(java.lang.String account) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
this.account = account;
return this;
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String integrator;
/** Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getIntegrator() {
return integrator;
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
public ListSubscribers setIntegrator(java.lang.String integrator) {
this.integrator = integrator;
return this;
}
/**
* Optional. The maximum number of service accounts to return. The service may return fewer
* than this value. If unspecified, returns at most 100 service accounts. The maximum value is
* 1000; the server will coerce values above 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of service accounts to return. The service may return fewer than this
value. If unspecified, returns at most 100 service accounts. The maximum value is 1000; the server
will coerce values above 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of service accounts to return. The service may return fewer
* than this value. If unspecified, returns at most 100 service accounts. The maximum value is
* 1000; the server will coerce values above 1000.
*/
public ListSubscribers setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListSubscribers` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSubscribers` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListSubscribers` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListSubscribers` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListSubscribers` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSubscribers` must match the call that provided the page token.
*/
public ListSubscribers setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListSubscribers set(String parameterName, Object value) {
return (ListSubscribers) super.set(parameterName, value);
}
}
/**
* List TransferableOffers of a customer based on Cloud Identity ID or Customer Name in the request.
* Use this method when a reseller gets the entitlement information of an unowned customer. The
* reseller should provide the customer's Cloud Identity ID or Customer Name. Possible error codes:
* * PERMISSION_DENIED: * The customer doesn't belong to the reseller and has no auth token. * The
* customer provided incorrect reseller information when generating auth token. * The reseller
* account making the request is different from the reseller account in the query. * The reseller is
* not authorized to transact on this Product. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: Required request
* parameters are missing or invalid. Return value: List of TransferableOffer for the given customer
* and SKU.
*
* Create a request for the method "accounts.listTransferableOffers".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ListTransferableOffers#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The resource name of the reseller's account.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableOffersRequest}
* @return the request
*/
public ListTransferableOffers listTransferableOffers(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableOffersRequest content) throws java.io.IOException {
ListTransferableOffers result = new ListTransferableOffers(parent, content);
initialize(result);
return result;
}
public class ListTransferableOffers extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}:listTransferableOffers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* List TransferableOffers of a customer based on Cloud Identity ID or Customer Name in the
* request. Use this method when a reseller gets the entitlement information of an unowned
* customer. The reseller should provide the customer's Cloud Identity ID or Customer Name.
* Possible error codes: * PERMISSION_DENIED: * The customer doesn't belong to the reseller and
* has no auth token. * The customer provided incorrect reseller information when generating auth
* token. * The reseller account making the request is different from the reseller account in the
* query. * The reseller is not authorized to transact on this Product. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: Required request
* parameters are missing or invalid. Return value: List of TransferableOffer for the given
* customer and SKU.
*
* Create a request for the method "accounts.listTransferableOffers".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ListTransferableOffers#execute()} method to invoke the
* remote operation. {@link ListTransferableOffers#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent Required. The resource name of the reseller's account.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableOffersRequest}
* @since 1.13
*/
protected ListTransferableOffers(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableOffersRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableOffersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public ListTransferableOffers set$Xgafv(java.lang.String $Xgafv) {
return (ListTransferableOffers) super.set$Xgafv($Xgafv);
}
@Override
public ListTransferableOffers setAccessToken(java.lang.String accessToken) {
return (ListTransferableOffers) super.setAccessToken(accessToken);
}
@Override
public ListTransferableOffers setAlt(java.lang.String alt) {
return (ListTransferableOffers) super.setAlt(alt);
}
@Override
public ListTransferableOffers setCallback(java.lang.String callback) {
return (ListTransferableOffers) super.setCallback(callback);
}
@Override
public ListTransferableOffers setFields(java.lang.String fields) {
return (ListTransferableOffers) super.setFields(fields);
}
@Override
public ListTransferableOffers setKey(java.lang.String key) {
return (ListTransferableOffers) super.setKey(key);
}
@Override
public ListTransferableOffers setOauthToken(java.lang.String oauthToken) {
return (ListTransferableOffers) super.setOauthToken(oauthToken);
}
@Override
public ListTransferableOffers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListTransferableOffers) super.setPrettyPrint(prettyPrint);
}
@Override
public ListTransferableOffers setQuotaUser(java.lang.String quotaUser) {
return (ListTransferableOffers) super.setQuotaUser(quotaUser);
}
@Override
public ListTransferableOffers setUploadType(java.lang.String uploadType) {
return (ListTransferableOffers) super.setUploadType(uploadType);
}
@Override
public ListTransferableOffers setUploadProtocol(java.lang.String uploadProtocol) {
return (ListTransferableOffers) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource name of the reseller's account. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller's account.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The resource name of the reseller's account. */
public ListTransferableOffers setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public ListTransferableOffers set(String parameterName, Object value) {
return (ListTransferableOffers) super.set(parameterName, value);
}
}
/**
* List TransferableSkus of a customer based on the Cloud Identity ID or Customer Name in the
* request. Use this method to list the entitlements information of an unowned customer. You should
* provide the customer's Cloud Identity ID or Customer Name. Possible error codes: *
* PERMISSION_DENIED: * The customer doesn't belong to the reseller and has no auth token. * The
* supplied auth token is invalid. * The reseller account making the request is different from the
* reseller account in the query. * INVALID_ARGUMENT: Required request parameters are missing or
* invalid. Return value: A list of the customer's TransferableSku.
*
* Create a request for the method "accounts.listTransferableSkus".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ListTransferableSkus#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The reseller account's resource name. Parent uses the format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableSkusRequest}
* @return the request
*/
public ListTransferableSkus listTransferableSkus(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableSkusRequest content) throws java.io.IOException {
ListTransferableSkus result = new ListTransferableSkus(parent, content);
initialize(result);
return result;
}
public class ListTransferableSkus extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}:listTransferableSkus";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* List TransferableSkus of a customer based on the Cloud Identity ID or Customer Name in the
* request. Use this method to list the entitlements information of an unowned customer. You
* should provide the customer's Cloud Identity ID or Customer Name. Possible error codes: *
* PERMISSION_DENIED: * The customer doesn't belong to the reseller and has no auth token. * The
* supplied auth token is invalid. * The reseller account making the request is different from the
* reseller account in the query. * INVALID_ARGUMENT: Required request parameters are missing or
* invalid. Return value: A list of the customer's TransferableSku.
*
* Create a request for the method "accounts.listTransferableSkus".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ListTransferableSkus#execute()} method to invoke the
* remote operation. {@link ListTransferableSkus#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent Required. The reseller account's resource name. Parent uses the format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableSkusRequest}
* @since 1.13
*/
protected ListTransferableSkus(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableSkusRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListTransferableSkusResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public ListTransferableSkus set$Xgafv(java.lang.String $Xgafv) {
return (ListTransferableSkus) super.set$Xgafv($Xgafv);
}
@Override
public ListTransferableSkus setAccessToken(java.lang.String accessToken) {
return (ListTransferableSkus) super.setAccessToken(accessToken);
}
@Override
public ListTransferableSkus setAlt(java.lang.String alt) {
return (ListTransferableSkus) super.setAlt(alt);
}
@Override
public ListTransferableSkus setCallback(java.lang.String callback) {
return (ListTransferableSkus) super.setCallback(callback);
}
@Override
public ListTransferableSkus setFields(java.lang.String fields) {
return (ListTransferableSkus) super.setFields(fields);
}
@Override
public ListTransferableSkus setKey(java.lang.String key) {
return (ListTransferableSkus) super.setKey(key);
}
@Override
public ListTransferableSkus setOauthToken(java.lang.String oauthToken) {
return (ListTransferableSkus) super.setOauthToken(oauthToken);
}
@Override
public ListTransferableSkus setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListTransferableSkus) super.setPrettyPrint(prettyPrint);
}
@Override
public ListTransferableSkus setQuotaUser(java.lang.String quotaUser) {
return (ListTransferableSkus) super.setQuotaUser(quotaUser);
}
@Override
public ListTransferableSkus setUploadType(java.lang.String uploadType) {
return (ListTransferableSkus) super.setUploadType(uploadType);
}
@Override
public ListTransferableSkus setUploadProtocol(java.lang.String uploadProtocol) {
return (ListTransferableSkus) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The reseller account's resource name. Parent uses the format:
* accounts/{account_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The reseller account's resource name. Parent uses the format: accounts/{account_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The reseller account's resource name. Parent uses the format:
* accounts/{account_id}
*/
public ListTransferableSkus setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public ListTransferableSkus set(String parameterName, Object value) {
return (ListTransferableSkus) super.set(parameterName, value);
}
}
/**
* Registers a service account with subscriber privileges on the Pub/Sub topic for this Channel
* Services account or integrator. After you create a subscriber, you get the events through
* SubscriberEvent Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request and the provided reseller account are different, or the impersonated user is not a super
* admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. * INTERNAL: Any
* non-user error related to a technical issue in the backend. Contact Cloud Channel support. *
* UNKNOWN: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. Return value: The topic name with the registered service email address.
*
* Create a request for the method "accounts.register".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Register#execute()} method to invoke the remote operation.
*
* @param account Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest}
* @return the request
*/
public Register register(java.lang.String account, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest content) throws java.io.IOException {
Register result = new Register(account, content);
initialize(result);
return result;
}
public class Register extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+account}:register";
private final java.util.regex.Pattern ACCOUNT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Registers a service account with subscriber privileges on the Pub/Sub topic for this Channel
* Services account or integrator. After you create a subscriber, you get the events through
* SubscriberEvent Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request and the provided reseller account are different, or the impersonated user is not a
* super admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* INTERNAL: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. * UNKNOWN: Any non-user error related to a technical issue in the backend. Contact
* Cloud Channel support. Return value: The topic name with the registered service email address.
*
* Create a request for the method "accounts.register".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Register#execute()} method to invoke the remote operation.
* {@link
* Register#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param account Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest}
* @since 1.13
*/
protected Register(java.lang.String account, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberResponse.class);
this.account = com.google.api.client.util.Preconditions.checkNotNull(account, "Required parameter account must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public Register set$Xgafv(java.lang.String $Xgafv) {
return (Register) super.set$Xgafv($Xgafv);
}
@Override
public Register setAccessToken(java.lang.String accessToken) {
return (Register) super.setAccessToken(accessToken);
}
@Override
public Register setAlt(java.lang.String alt) {
return (Register) super.setAlt(alt);
}
@Override
public Register setCallback(java.lang.String callback) {
return (Register) super.setCallback(callback);
}
@Override
public Register setFields(java.lang.String fields) {
return (Register) super.setFields(fields);
}
@Override
public Register setKey(java.lang.String key) {
return (Register) super.setKey(key);
}
@Override
public Register setOauthToken(java.lang.String oauthToken) {
return (Register) super.setOauthToken(oauthToken);
}
@Override
public Register setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Register) super.setPrettyPrint(prettyPrint);
}
@Override
public Register setQuotaUser(java.lang.String quotaUser) {
return (Register) super.setQuotaUser(quotaUser);
}
@Override
public Register setUploadType(java.lang.String uploadType) {
return (Register) super.setUploadType(uploadType);
}
@Override
public Register setUploadProtocol(java.lang.String uploadProtocol) {
return (Register) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String account;
/** Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getAccount() {
return account;
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
public Register setAccount(java.lang.String account) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
this.account = account;
return this;
}
@Override
public Register set(String parameterName, Object value) {
return (Register) super.set(parameterName, value);
}
}
/**
* Unregisters a service account with subscriber privileges on the Pub/Sub topic created for this
* Channel Services account or integrator. If there are no service accounts left with subscriber
* privileges, this deletes the topic. You can call ListSubscribers to check for these accounts.
* Possible error codes: * PERMISSION_DENIED: The reseller account making the request and the
* provided reseller account are different, or the impersonated user is not a super admin. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The topic
* resource doesn't exist. * INTERNAL: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The topic name that
* unregistered the service email address. Returns a success response if the service email address
* wasn't registered with the topic.
*
* Create a request for the method "accounts.unregister".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Unregister#execute()} method to invoke the remote operation.
*
* @param account Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest}
* @return the request
*/
public Unregister unregister(java.lang.String account, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest content) throws java.io.IOException {
Unregister result = new Unregister(account, content);
initialize(result);
return result;
}
public class Unregister extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+account}:unregister";
private final java.util.regex.Pattern ACCOUNT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Unregisters a service account with subscriber privileges on the Pub/Sub topic created for this
* Channel Services account or integrator. If there are no service accounts left with subscriber
* privileges, this deletes the topic. You can call ListSubscribers to check for these accounts.
* Possible error codes: * PERMISSION_DENIED: The reseller account making the request and the
* provided reseller account are different, or the impersonated user is not a super admin. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The topic
* resource doesn't exist. * INTERNAL: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The topic name that
* unregistered the service email address. Returns a success response if the service email address
* wasn't registered with the topic.
*
* Create a request for the method "accounts.unregister".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Unregister#execute()} method to invoke the remote
* operation. {@link
* Unregister#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param account Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest}
* @since 1.13
*/
protected Unregister(java.lang.String account, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberResponse.class);
this.account = com.google.api.client.util.Preconditions.checkNotNull(account, "Required parameter account must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public Unregister set$Xgafv(java.lang.String $Xgafv) {
return (Unregister) super.set$Xgafv($Xgafv);
}
@Override
public Unregister setAccessToken(java.lang.String accessToken) {
return (Unregister) super.setAccessToken(accessToken);
}
@Override
public Unregister setAlt(java.lang.String alt) {
return (Unregister) super.setAlt(alt);
}
@Override
public Unregister setCallback(java.lang.String callback) {
return (Unregister) super.setCallback(callback);
}
@Override
public Unregister setFields(java.lang.String fields) {
return (Unregister) super.setFields(fields);
}
@Override
public Unregister setKey(java.lang.String key) {
return (Unregister) super.setKey(key);
}
@Override
public Unregister setOauthToken(java.lang.String oauthToken) {
return (Unregister) super.setOauthToken(oauthToken);
}
@Override
public Unregister setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unregister) super.setPrettyPrint(prettyPrint);
}
@Override
public Unregister setQuotaUser(java.lang.String quotaUser) {
return (Unregister) super.setQuotaUser(quotaUser);
}
@Override
public Unregister setUploadType(java.lang.String uploadType) {
return (Unregister) super.setUploadType(uploadType);
}
@Override
public Unregister setUploadProtocol(java.lang.String uploadProtocol) {
return (Unregister) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String account;
/** Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getAccount() {
return account;
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
public Unregister setAccount(java.lang.String account) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
this.account = account;
return this;
}
@Override
public Unregister set(String parameterName, Object value) {
return (Unregister) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ChannelPartnerLinks collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.ChannelPartnerLinks.List request = cloudchannel.channelPartnerLinks().list(parameters ...)}
*
*
* @return the resource collection
*/
public ChannelPartnerLinks channelPartnerLinks() {
return new ChannelPartnerLinks();
}
/**
* The "channelPartnerLinks" collection of methods.
*/
public class ChannelPartnerLinks {
/**
* Initiates a channel partner link between a distributor and a reseller, or between resellers in an
* n-tier reseller channel. Invited partners need to follow the invite_link_uri provided in the
* response to accept. After accepting the invitation, a link is set up between the two parties. You
* must be a distributor to call this method. Possible error codes: * PERMISSION_DENIED: The
* reseller account making the request is different from the reseller account in the API request. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * ALREADY_EXISTS: The
* ChannelPartnerLink sent in the request already exists. * NOT_FOUND: No Cloud Identity customer
* exists for provided domain. * INTERNAL: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The new ChannelPartnerLink
* resource.
*
* Create a request for the method "channelPartnerLinks.create".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Create a channel partner link for the provided reseller account's resource name. Parent
* uses the format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerLink}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerLink content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/channelPartnerLinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Initiates a channel partner link between a distributor and a reseller, or between resellers in
* an n-tier reseller channel. Invited partners need to follow the invite_link_uri provided in the
* response to accept. After accepting the invitation, a link is set up between the two parties.
* You must be a distributor to call this method. Possible error codes: * PERMISSION_DENIED: The
* reseller account making the request is different from the reseller account in the API request.
* * INVALID_ARGUMENT: Required request parameters are missing or invalid. * ALREADY_EXISTS: The
* ChannelPartnerLink sent in the request already exists. * NOT_FOUND: No Cloud Identity customer
* exists for provided domain. * INTERNAL: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The new ChannelPartnerLink
* resource.
*
* Create a request for the method "channelPartnerLinks.create".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Create a channel partner link for the provided reseller account's resource name. Parent
* uses the format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerLink}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerLink content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerLink.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Create a channel partner link for the provided reseller account's resource
* name. Parent uses the format: accounts/{account_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Create a channel partner link for the provided reseller account's resource name. Parent
uses the format: accounts/{account_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Create a channel partner link for the provided reseller account's resource
* name. Parent uses the format: accounts/{account_id}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Returns the requested ChannelPartnerLink resource. You must be a distributor to call this method.
* Possible error codes: * PERMISSION_DENIED: The reseller account making the request is different
* from the reseller account in the API request. * INVALID_ARGUMENT: Required request parameters are
* missing or invalid. * NOT_FOUND: ChannelPartnerLink resource not found because of an invalid
* channel partner link name. Return value: The ChannelPartnerLink resource.
*
* Create a request for the method "channelPartnerLinks.get".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the channel partner link to retrieve. Name uses the format:
* accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the
* partner.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+$");
/**
* Returns the requested ChannelPartnerLink resource. You must be a distributor to call this
* method. Possible error codes: * PERMISSION_DENIED: The reseller account making the request is
* different from the reseller account in the API request. * INVALID_ARGUMENT: Required request
* parameters are missing or invalid. * NOT_FOUND: ChannelPartnerLink resource not found because
* of an invalid channel partner link name. Return value: The ChannelPartnerLink resource.
*
* Create a request for the method "channelPartnerLinks.get".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the channel partner link to retrieve. Name uses the format:
* accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the
* partner.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerLink.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the channel partner link to retrieve. Name uses the
* format: accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity
* ID of the partner.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the channel partner link to retrieve. Name uses the format:
accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the partner.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the channel partner link to retrieve. Name uses the
* format: accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity
* ID of the partner.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
this.name = name;
return this;
}
/** Optional. The level of granularity the ChannelPartnerLink will display. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Optional. The level of granularity the ChannelPartnerLink will display.
*/
public java.lang.String getView() {
return view;
}
/** Optional. The level of granularity the ChannelPartnerLink will display. */
public Get setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List ChannelPartnerLinks belonging to a distributor. You must be a distributor to call this
* method. Possible error codes: * PERMISSION_DENIED: The reseller account making the request is
* different from the reseller account in the API request. * INVALID_ARGUMENT: Required request
* parameters are missing or invalid. Return value: The list of the distributor account's
* ChannelPartnerLink resources.
*
* Create a request for the method "channelPartnerLinks.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller account for listing channel partner links. Parent uses
* the format: accounts/{account_id}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/channelPartnerLinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* List ChannelPartnerLinks belonging to a distributor. You must be a distributor to call this
* method. Possible error codes: * PERMISSION_DENIED: The reseller account making the request is
* different from the reseller account in the API request. * INVALID_ARGUMENT: Required request
* parameters are missing or invalid. Return value: The list of the distributor account's
* ChannelPartnerLink resources.
*
* Create a request for the method "channelPartnerLinks.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the reseller account for listing channel partner links. Parent uses
* the format: accounts/{account_id}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListChannelPartnerLinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller account for listing channel partner links.
* Parent uses the format: accounts/{account_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller account for listing channel partner links. Parent uses
the format: accounts/{account_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller account for listing channel partner links.
* Parent uses the format: accounts/{account_id}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, server will pick a default size (25). The maximum value is 200; the server
* will coerce values above 200.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server might return fewer results than requested. If unspecified,
server will pick a default size (25). The maximum value is 200; the server will coerce values above
200.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, server will pick a default size (25). The maximum value is 200; the server
* will coerce values above 200.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token for a page of results other than the first page. Obtained using
* ListChannelPartnerLinksResponse.next_page_token of the previous
* CloudChannelService.ListChannelPartnerLinks call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token for a page of results other than the first page. Obtained using
ListChannelPartnerLinksResponse.next_page_token of the previous
CloudChannelService.ListChannelPartnerLinks call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token for a page of results other than the first page. Obtained using
* ListChannelPartnerLinksResponse.next_page_token of the previous
* CloudChannelService.ListChannelPartnerLinks call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Optional. The level of granularity the ChannelPartnerLink will display. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Optional. The level of granularity the ChannelPartnerLink will display.
*/
public java.lang.String getView() {
return view;
}
/** Optional. The level of granularity the ChannelPartnerLink will display. */
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a channel partner link. Distributors call this method to change a link's status. For
* example, to suspend a partner link. You must be a distributor to call this method. Possible error
* codes: * PERMISSION_DENIED: The reseller account making the request is different from the
* reseller account in the API request. * INVALID_ARGUMENT: * Required request parameters are
* missing or invalid. * Link state cannot change from invited to active or suspended. * Cannot send
* reseller_cloud_identity_id, invite_url, or name in update mask. * NOT_FOUND: ChannelPartnerLink
* resource not found. * INTERNAL: Any non-user error related to a technical issue in the backend.
* Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. Return value: The updated ChannelPartnerLink resource.
*
* Create a request for the method "channelPartnerLinks.patch".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the channel partner link to cancel. Name uses the format:
* accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the
* partner.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UpdateChannelPartnerLinkRequest}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UpdateChannelPartnerLinkRequest content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+$");
/**
* Updates a channel partner link. Distributors call this method to change a link's status. For
* example, to suspend a partner link. You must be a distributor to call this method. Possible
* error codes: * PERMISSION_DENIED: The reseller account making the request is different from the
* reseller account in the API request. * INVALID_ARGUMENT: * Required request parameters are
* missing or invalid. * Link state cannot change from invited to active or suspended. * Cannot
* send reseller_cloud_identity_id, invite_url, or name in update mask. * NOT_FOUND:
* ChannelPartnerLink resource not found. * INTERNAL: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. Return value: The updated
* ChannelPartnerLink resource.
*
* Create a request for the method "channelPartnerLinks.patch".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the channel partner link to cancel. Name uses the format:
* accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the
* partner.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UpdateChannelPartnerLinkRequest}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UpdateChannelPartnerLinkRequest content) {
super(Cloudchannel.this, "PATCH", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerLink.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the channel partner link to cancel. Name uses the format:
* accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the
* partner.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the channel partner link to cancel. Name uses the format:
accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the partner.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the channel partner link to cancel. Name uses the format:
* accounts/{account_id}/channelPartnerLinks/{id} where {id} is the Cloud Identity ID of the
* partner.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ChannelPartnerRepricingConfigs collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.ChannelPartnerRepricingConfigs.List request = cloudchannel.channelPartnerRepricingConfigs().list(parameters ...)}
*
*
* @return the resource collection
*/
public ChannelPartnerRepricingConfigs channelPartnerRepricingConfigs() {
return new ChannelPartnerRepricingConfigs();
}
/**
* The "channelPartnerRepricingConfigs" collection of methods.
*/
public class ChannelPartnerRepricingConfigs {
/**
* Creates a ChannelPartnerRepricingConfig. Call this method to set modifications for a specific
* ChannelPartner's bill. You can only create configs if the RepricingConfig.effective_invoice_month
* is a future month. If needed, you can create a config for the current month, with some
* restrictions. When creating a config for a future month, make sure there are no existing configs
* for that RepricingConfig.effective_invoice_month. The following restrictions are for creating
* configs in the current month. * This functionality is reserved for recovering from an erroneous
* config, and should not be used for regular business cases. * The new config will not modify
* exports used with other configs. Changes to the config may be immediate, but may take up to 24
* hours. * There is a limit of ten configs for any ChannelPartner or
* RepricingConfig.EntitlementGranularity.entitlement, for any
* RepricingConfig.effective_invoice_month. * The contained
* ChannelPartnerRepricingConfig.repricing_config value must be different from the value used in the
* current config for a ChannelPartner. Possible Error Codes: * PERMISSION_DENIED: If the account
* making the request and the account being queried are different. * INVALID_ARGUMENT: Missing or
* invalid required parameters in the request. Also displays if the updated config is for the
* current month or past months. * NOT_FOUND: The ChannelPartnerRepricingConfig specified does not
* exist or is not associated with the given account. * INTERNAL: Any non-user error related to
* technical issues in the backend. In this case, contact Cloud Channel support. Return Value: If
* successful, the updated ChannelPartnerRepricingConfig resource, otherwise returns an error.
*
* Create a request for the method "channelPartnerRepricingConfigs.create".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the ChannelPartner that will receive the repricing config. Parent
* uses the format: accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/channelPartnerRepricingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+$");
/**
* Creates a ChannelPartnerRepricingConfig. Call this method to set modifications for a specific
* ChannelPartner's bill. You can only create configs if the
* RepricingConfig.effective_invoice_month is a future month. If needed, you can create a config
* for the current month, with some restrictions. When creating a config for a future month, make
* sure there are no existing configs for that RepricingConfig.effective_invoice_month. The
* following restrictions are for creating configs in the current month. * This functionality is
* reserved for recovering from an erroneous config, and should not be used for regular business
* cases. * The new config will not modify exports used with other configs. Changes to the config
* may be immediate, but may take up to 24 hours. * There is a limit of ten configs for any
* ChannelPartner or RepricingConfig.EntitlementGranularity.entitlement, for any
* RepricingConfig.effective_invoice_month. * The contained
* ChannelPartnerRepricingConfig.repricing_config value must be different from the value used in
* the current config for a ChannelPartner. Possible Error Codes: * PERMISSION_DENIED: If the
* account making the request and the account being queried are different. * INVALID_ARGUMENT:
* Missing or invalid required parameters in the request. Also displays if the updated config is
* for the current month or past months. * NOT_FOUND: The ChannelPartnerRepricingConfig specified
* does not exist or is not associated with the given account. * INTERNAL: Any non-user error
* related to technical issues in the backend. In this case, contact Cloud Channel support. Return
* Value: If successful, the updated ChannelPartnerRepricingConfig resource, otherwise returns an
* error.
*
* Create a request for the method "channelPartnerRepricingConfigs.create".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the ChannelPartner that will receive the repricing config. Parent
* uses the format: accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the ChannelPartner that will receive the repricing
* config. Parent uses the format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the ChannelPartner that will receive the repricing config. Parent
uses the format: accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the ChannelPartner that will receive the repricing
* config. Parent uses the format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the given ChannelPartnerRepricingConfig permanently. You can only delete configs if their
* RepricingConfig.effective_invoice_month is set to a date after the current month. Possible error
* codes: * PERMISSION_DENIED: The account making the request does not own this customer. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * FAILED_PRECONDITION: The
* ChannelPartnerRepricingConfig is active or in the past. * NOT_FOUND: No
* ChannelPartnerRepricingConfig found for the name in the request.
*
* Create a request for the method "channelPartnerRepricingConfigs.delete".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the channel partner repricing config rule to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
/**
* Deletes the given ChannelPartnerRepricingConfig permanently. You can only delete configs if
* their RepricingConfig.effective_invoice_month is set to a date after the current month.
* Possible error codes: * PERMISSION_DENIED: The account making the request does not own this
* customer. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* FAILED_PRECONDITION: The ChannelPartnerRepricingConfig is active or in the past. * NOT_FOUND:
* No ChannelPartnerRepricingConfig found for the name in the request.
*
* Create a request for the method "channelPartnerRepricingConfigs.delete".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the channel partner repricing config rule to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Cloudchannel.this, "DELETE", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the channel partner repricing config rule to delete.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the channel partner repricing config rule to delete.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the channel partner repricing config rule to delete.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about how a Distributor modifies their bill before sending it to a
* ChannelPartner. Possible Error Codes: * PERMISSION_DENIED: If the account making the request and
* the account being queried are different. * NOT_FOUND: The ChannelPartnerRepricingConfig was not
* found. * INTERNAL: Any non-user error related to technical issues in the backend. In this case,
* contact Cloud Channel support. Return Value: If successful, the ChannelPartnerRepricingConfig
* resource, otherwise returns an error.
*
* Create a request for the method "channelPartnerRepricingConfigs.get".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the ChannelPartnerRepricingConfig Format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConf
* igs/{id}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
/**
* Gets information about how a Distributor modifies their bill before sending it to a
* ChannelPartner. Possible Error Codes: * PERMISSION_DENIED: If the account making the request
* and the account being queried are different. * NOT_FOUND: The ChannelPartnerRepricingConfig was
* not found. * INTERNAL: Any non-user error related to technical issues in the backend. In this
* case, contact Cloud Channel support. Return Value: If successful, the
* ChannelPartnerRepricingConfig resource, otherwise returns an error.
*
* Create a request for the method "channelPartnerRepricingConfigs.get".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the ChannelPartnerRepricingConfig Format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConf
* igs/{id}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the ChannelPartnerRepricingConfig Format: accounts/{acco
* unt_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConfigs/{id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the ChannelPartnerRepricingConfig Format:
accounts/{account_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConfigs/{id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the ChannelPartnerRepricingConfig Format: accounts/{acco
* unt_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConfigs/{id}.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about how a Reseller modifies their bill before sending it to a ChannelPartner.
* Possible Error Codes: * PERMISSION_DENIED: If the account making the request and the account
* being queried are different. * NOT_FOUND: The ChannelPartnerRepricingConfig specified does not
* exist or is not associated with the given account. * INTERNAL: Any non-user error related to
* technical issues in the backend. In this case, contact Cloud Channel support. Return Value: If
* successful, the ChannelPartnerRepricingConfig resources. The data for each resource is displayed
* in the ascending order of: * Channel Partner ID * RepricingConfig.effective_invoice_month *
* ChannelPartnerRepricingConfig.update_time If unsuccessful, returns an error.
*
* Create a request for the method "channelPartnerRepricingConfigs.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the account's ChannelPartnerLink. Parent uses the format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}. Supports
* accounts/{account_id}/channelPartnerLinks/- to retrieve configs for all channel partners.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/channelPartnerRepricingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+$");
/**
* Lists information about how a Reseller modifies their bill before sending it to a
* ChannelPartner. Possible Error Codes: * PERMISSION_DENIED: If the account making the request
* and the account being queried are different. * NOT_FOUND: The ChannelPartnerRepricingConfig
* specified does not exist or is not associated with the given account. * INTERNAL: Any non-user
* error related to technical issues in the backend. In this case, contact Cloud Channel support.
* Return Value: If successful, the ChannelPartnerRepricingConfig resources. The data for each
* resource is displayed in the ascending order of: * Channel Partner ID *
* RepricingConfig.effective_invoice_month * ChannelPartnerRepricingConfig.update_time If
* unsuccessful, returns an error.
*
* Create a request for the method "channelPartnerRepricingConfigs.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the account's ChannelPartnerLink. Parent uses the format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}. Supports
* accounts/{account_id}/channelPartnerLinks/- to retrieve configs for all channel partners.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListChannelPartnerRepricingConfigsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the account's ChannelPartnerLink. Parent uses the
* format: accounts/{account_id}/channelPartnerLinks/{channel_partner_id}. Supports
* accounts/{account_id}/channelPartnerLinks/- to retrieve configs for all channel
* partners.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the account's ChannelPartnerLink. Parent uses the format:
accounts/{account_id}/channelPartnerLinks/{channel_partner_id}. Supports
accounts/{account_id}/channelPartnerLinks/- to retrieve configs for all channel partners.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the account's ChannelPartnerLink. Parent uses the
* format: accounts/{account_id}/channelPartnerLinks/{channel_partner_id}. Supports
* accounts/{account_id}/channelPartnerLinks/- to retrieve configs for all channel
* partners.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter for [CloudChannelService.ListChannelPartnerRepricingConfigs] results
* (channel_partner_link only). You can use this filter when you support a BatchGet-like
* query. To use the filter, you must set
* `parent=accounts/{account_id}/channelPartnerLinks/-`. Example: `channel_partner_link =
* accounts/account_id/channelPartnerLinks/c1` OR `channel_partner_link =
* accounts/account_id/channelPartnerLinks/c2`.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter for [CloudChannelService.ListChannelPartnerRepricingConfigs] results
(channel_partner_link only). You can use this filter when you support a BatchGet-like query. To use
the filter, you must set `parent=accounts/{account_id}/channelPartnerLinks/-`. Example:
`channel_partner_link = accounts/account_id/channelPartnerLinks/c1` OR `channel_partner_link =
accounts/account_id/channelPartnerLinks/c2`.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter for [CloudChannelService.ListChannelPartnerRepricingConfigs] results
* (channel_partner_link only). You can use this filter when you support a BatchGet-like
* query. To use the filter, you must set
* `parent=accounts/{account_id}/channelPartnerLinks/-`. Example: `channel_partner_link =
* accounts/account_id/channelPartnerLinks/c1` OR `channel_partner_link =
* accounts/account_id/channelPartnerLinks/c2`.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of repricing configs to return. The service may return
* fewer than this value. If unspecified, returns a maximum of 50 rules. The maximum value
* is 100; values above 100 will be coerced to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of repricing configs to return. The service may return fewer than this
value. If unspecified, returns a maximum of 50 rules. The maximum value is 100; values above 100
will be coerced to 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of repricing configs to return. The service may return
* fewer than this value. If unspecified, returns a maximum of 50 rules. The maximum value
* is 100; values above 100 will be coerced to 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListChannelPartnerRepricingConfigsResponse.next_page_token of the previous
* CloudChannelService.ListChannelPartnerRepricingConfigs call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results beyond the first page. Obtained through
ListChannelPartnerRepricingConfigsResponse.next_page_token of the previous
CloudChannelService.ListChannelPartnerRepricingConfigs call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListChannelPartnerRepricingConfigsResponse.next_page_token of the previous
* CloudChannelService.ListChannelPartnerRepricingConfigs call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a ChannelPartnerRepricingConfig. Call this method to set modifications for a specific
* ChannelPartner's bill. This method overwrites the existing CustomerRepricingConfig. You can only
* update configs if the RepricingConfig.effective_invoice_month is a future month. To make changes
* to configs for the current month, use CreateChannelPartnerRepricingConfig, taking note of its
* restrictions. You cannot update the RepricingConfig.effective_invoice_month. When updating a
* config in the future: * This config must already exist. Possible Error Codes: *
* PERMISSION_DENIED: If the account making the request and the account being queried are different.
* * INVALID_ARGUMENT: Missing or invalid required parameters in the request. Also displays if the
* updated config is for the current month or past months. * NOT_FOUND: The
* ChannelPartnerRepricingConfig specified does not exist or is not associated with the given
* account. * INTERNAL: Any non-user error related to technical issues in the backend. In this case,
* contact Cloud Channel support. Return Value: If successful, the updated
* ChannelPartnerRepricingConfig resource, otherwise returns an error.
*
* Create a request for the method "channelPartnerRepricingConfigs.patch".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Resource name of the ChannelPartnerRepricingConfig. Format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConf
* igs/{id}.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
/**
* Updates a ChannelPartnerRepricingConfig. Call this method to set modifications for a specific
* ChannelPartner's bill. This method overwrites the existing CustomerRepricingConfig. You can
* only update configs if the RepricingConfig.effective_invoice_month is a future month. To make
* changes to configs for the current month, use CreateChannelPartnerRepricingConfig, taking note
* of its restrictions. You cannot update the RepricingConfig.effective_invoice_month. When
* updating a config in the future: * This config must already exist. Possible Error Codes: *
* PERMISSION_DENIED: If the account making the request and the account being queried are
* different. * INVALID_ARGUMENT: Missing or invalid required parameters in the request. Also
* displays if the updated config is for the current month or past months. * NOT_FOUND: The
* ChannelPartnerRepricingConfig specified does not exist or is not associated with the given
* account. * INTERNAL: Any non-user error related to technical issues in the backend. In this
* case, contact Cloud Channel support. Return Value: If successful, the updated
* ChannelPartnerRepricingConfig resource, otherwise returns an error.
*
* Create a request for the method "channelPartnerRepricingConfigs.patch".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Resource name of the ChannelPartnerRepricingConfig. Format:
* accounts/{account_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConf
* igs/{id}.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig content) {
super(Cloudchannel.this, "PATCH", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChannelPartnerRepricingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Resource name of the ChannelPartnerRepricingConfig. Format: accounts/{acco
* unt_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConfigs/{id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Resource name of the ChannelPartnerRepricingConfig. Format:
accounts/{account_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConfigs/{id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the ChannelPartnerRepricingConfig. Format: accounts/{acco
* unt_id}/channelPartnerLinks/{channel_partner_id}/channelPartnerRepricingConfigs/{id}.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/channelPartnerRepricingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Customers collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Customers.List request = cloudchannel.customers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Customers customers() {
return new Customers();
}
/**
* The "customers" collection of methods.
*/
public class Customers {
/**
* Creates a new Customer resource under the reseller or distributor account. Possible error codes:
* * PERMISSION_DENIED: * The reseller account making the request is different from the reseller
* account in the API request. * You are not authorized to create a customer. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: * Required request
* parameters are missing or invalid. * Domain field value doesn't match the primary email domain.
* Return value: The newly created Customer resource.
*
* Create a request for the method "customers.create".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of reseller account in which to create the customer. Parent uses the
* format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+$");
/**
* Creates a new Customer resource under the reseller or distributor account. Possible error
* codes: * PERMISSION_DENIED: * The reseller account making the request is different from the
* reseller account in the API request. * You are not authorized to create a customer. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: * Required
* request parameters are missing or invalid. * Domain field value doesn't match the primary email
* domain. Return value: The newly created Customer resource.
*
* Create a request for the method "customers.create".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of reseller account in which to create the customer. Parent uses the
* format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of reseller account in which to create the customer. Parent
* uses the format: accounts/{account_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of reseller account in which to create the customer. Parent uses the
format: accounts/{account_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of reseller account in which to create the customer. Parent
* uses the format: accounts/{account_id}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the given Customer permanently. Possible error codes: * PERMISSION_DENIED: The account
* making the request does not own this customer. * INVALID_ARGUMENT: Required request parameters
* are missing or invalid. * FAILED_PRECONDITION: The customer has existing entitlements. *
* NOT_FOUND: No Customer resource found for the name in the request.
*
* Create a request for the method "customers.delete".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the customer to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
/**
* Deletes the given Customer permanently. Possible error codes: * PERMISSION_DENIED: The account
* making the request does not own this customer. * INVALID_ARGUMENT: Required request parameters
* are missing or invalid. * FAILED_PRECONDITION: The customer has existing entitlements. *
* NOT_FOUND: No Customer resource found for the name in the request.
*
* Create a request for the method "customers.delete".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the customer to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Cloudchannel.this, "DELETE", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource name of the customer to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the customer to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The resource name of the customer to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns the requested Customer resource. Possible error codes: * PERMISSION_DENIED: The reseller
* account making the request is different from the reseller account in the API request. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The customer
* resource doesn't exist. Usually the result of an invalid name parameter. Return value: The
* Customer resource.
*
* Create a request for the method "customers.get".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
/**
* Returns the requested Customer resource. Possible error codes: * PERMISSION_DENIED: The
* reseller account making the request is different from the reseller account in the API request.
* * INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The
* customer resource doesn't exist. Usually the result of an invalid name parameter. Return value:
* The Customer resource.
*
* Create a request for the method "customers.get".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the customer to retrieve. Name uses the format:
accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports a Customer from the Cloud Identity associated with the provided Cloud Identity ID or
* domain before a TransferEntitlements call. If a linked Customer already exists and
* overwrite_if_exists is true, it will update that Customer's data. Possible error codes: *
* PERMISSION_DENIED: * The reseller account making the request is different from the reseller
* account in the API request. * You are not authorized to import the customer. See
* https://support.google.com/channelservices/answer/9759265 * NOT_FOUND: Cloud Identity doesn't
* exist or was deleted. * INVALID_ARGUMENT: Required parameters are missing, or the auth_token is
* expired or invalid. * ALREADY_EXISTS: A customer already exists and has conflicting critical
* fields. Requires an overwrite. Return value: The Customer.
*
* Create a request for the method "customers.import".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link CloudchannelImport#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest}
* @return the request
*/
public CloudchannelImport cloudchannelImport(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest content) throws java.io.IOException {
CloudchannelImport result = new CloudchannelImport(parent, content);
initialize(result);
return result;
}
public class CloudchannelImport extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customers:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+$");
/**
* Imports a Customer from the Cloud Identity associated with the provided Cloud Identity ID or
* domain before a TransferEntitlements call. If a linked Customer already exists and
* overwrite_if_exists is true, it will update that Customer's data. Possible error codes: *
* PERMISSION_DENIED: * The reseller account making the request is different from the reseller
* account in the API request. * You are not authorized to import the customer. See
* https://support.google.com/channelservices/answer/9759265 * NOT_FOUND: Cloud Identity doesn't
* exist or was deleted. * INVALID_ARGUMENT: Required parameters are missing, or the auth_token is
* expired or invalid. * ALREADY_EXISTS: A customer already exists and has conflicting critical
* fields. Requires an overwrite. Return value: The Customer.
*
* Create a request for the method "customers.import".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link CloudchannelImport#execute()} method to invoke the remote
* operation. {@link CloudchannelImport#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest}
* @since 1.13
*/
protected CloudchannelImport(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
}
@Override
public CloudchannelImport set$Xgafv(java.lang.String $Xgafv) {
return (CloudchannelImport) super.set$Xgafv($Xgafv);
}
@Override
public CloudchannelImport setAccessToken(java.lang.String accessToken) {
return (CloudchannelImport) super.setAccessToken(accessToken);
}
@Override
public CloudchannelImport setAlt(java.lang.String alt) {
return (CloudchannelImport) super.setAlt(alt);
}
@Override
public CloudchannelImport setCallback(java.lang.String callback) {
return (CloudchannelImport) super.setCallback(callback);
}
@Override
public CloudchannelImport setFields(java.lang.String fields) {
return (CloudchannelImport) super.setFields(fields);
}
@Override
public CloudchannelImport setKey(java.lang.String key) {
return (CloudchannelImport) super.setKey(key);
}
@Override
public CloudchannelImport setOauthToken(java.lang.String oauthToken) {
return (CloudchannelImport) super.setOauthToken(oauthToken);
}
@Override
public CloudchannelImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CloudchannelImport) super.setPrettyPrint(prettyPrint);
}
@Override
public CloudchannelImport setQuotaUser(java.lang.String quotaUser) {
return (CloudchannelImport) super.setQuotaUser(quotaUser);
}
@Override
public CloudchannelImport setUploadType(java.lang.String uploadType) {
return (CloudchannelImport) super.setUploadType(uploadType);
}
@Override
public CloudchannelImport setUploadProtocol(java.lang.String uploadProtocol) {
return (CloudchannelImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller's account. Parent takes the format:
accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
public CloudchannelImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public CloudchannelImport set(String parameterName, Object value) {
return (CloudchannelImport) super.set(parameterName, value);
}
}
/**
* List Customers. Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request is different from the reseller account in the API request. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. Return value: List of Customers, or an empty list if
* there are no customers.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller account to list customers from. Parent uses the format:
* accounts/{account_id}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+$");
/**
* List Customers. Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request is different from the reseller account in the API request. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. Return value: List of Customers, or an empty list if
* there are no customers.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the reseller account to list customers from. Parent uses the format:
* accounts/{account_id}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListCustomersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller account to list customers from. Parent uses
* the format: accounts/{account_id}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller account to list customers from. Parent uses the format:
accounts/{account_id}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller account to list customers from. Parent uses
* the format: accounts/{account_id}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Filters applied to the [CloudChannelService.ListCustomers] results. See
* https://cloud.google.com/channel/docs/concepts/google-cloud/filter-customers for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filters applied to the [CloudChannelService.ListCustomers] results. See
https://cloud.google.com/channel/docs/concepts/google-cloud/filter-customers for more information.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Filters applied to the [CloudChannelService.ListCustomers] results. See
* https://cloud.google.com/channel/docs/concepts/google-cloud/filter-customers for more
* information.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of customers to return. The service may return fewer than
* this value. If unspecified, returns at most 10 customers. The maximum value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of customers to return. The service may return fewer than this value.
If unspecified, returns at most 10 customers. The maximum value is 50.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of customers to return. The service may return fewer than
* this value. If unspecified, returns at most 10 customers. The maximum value is 50.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token identifying a page of results other than the first page. Obtained
* through ListCustomersResponse.next_page_token of the previous
* CloudChannelService.ListCustomers call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results other than the first page. Obtained through
ListCustomersResponse.next_page_token of the previous CloudChannelService.ListCustomers call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token identifying a page of results other than the first page. Obtained
* through ListCustomersResponse.next_page_token of the previous
* CloudChannelService.ListCustomers call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing Customer resource for the reseller or distributor. Possible error codes: *
* PERMISSION_DENIED: The reseller account making the request is different from the reseller account
* in the API request. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* NOT_FOUND: No Customer resource found for the name in the request. Return value: The updated
* Customer resource.
*
* Create a request for the method "customers.patch".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
/**
* Updates an existing Customer resource for the reseller or distributor. Possible error codes: *
* PERMISSION_DENIED: The reseller account making the request is different from the reseller
* account in the API request. * INVALID_ARGUMENT: Required request parameters are missing or
* invalid. * NOT_FOUND: No Customer resource found for the name in the request. Return value: The
* updated Customer resource.
*
* Create a request for the method "customers.patch".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) {
super(Cloudchannel.this, "PATCH", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Resource name of the customer. Format:
* accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the customer. Format:
* accounts/{account_id}/customers/{customer_id}
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/channelPartnerLinks/[^/]+/customers/[^/]+$");
}
this.name = name;
return this;
}
/** The update mask that applies to the resource. Optional. */
@com.google.api.client.util.Key
private String updateMask;
/** The update mask that applies to the resource. Optional.
*/
public String getUpdateMask() {
return updateMask;
}
/** The update mask that applies to the resource. Optional. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Customers collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Customers.List request = cloudchannel.customers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Customers customers() {
return new Customers();
}
/**
* The "customers" collection of methods.
*/
public class Customers {
/**
* Creates a new Customer resource under the reseller or distributor account. Possible error codes:
* * PERMISSION_DENIED: * The reseller account making the request is different from the reseller
* account in the API request. * You are not authorized to create a customer. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: * Required request
* parameters are missing or invalid. * Domain field value doesn't match the primary email domain.
* Return value: The newly created Customer resource.
*
* Create a request for the method "customers.create".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of reseller account in which to create the customer. Parent uses the
* format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Creates a new Customer resource under the reseller or distributor account. Possible error
* codes: * PERMISSION_DENIED: * The reseller account making the request is different from the
* reseller account in the API request. * You are not authorized to create a customer. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: * Required
* request parameters are missing or invalid. * Domain field value doesn't match the primary email
* domain. Return value: The newly created Customer resource.
*
* Create a request for the method "customers.create".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of reseller account in which to create the customer. Parent uses the
* format: accounts/{account_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of reseller account in which to create the customer. Parent
* uses the format: accounts/{account_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of reseller account in which to create the customer. Parent uses the
format: accounts/{account_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of reseller account in which to create the customer. Parent
* uses the format: accounts/{account_id}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the given Customer permanently. Possible error codes: * PERMISSION_DENIED: The account
* making the request does not own this customer. * INVALID_ARGUMENT: Required request parameters
* are missing or invalid. * FAILED_PRECONDITION: The customer has existing entitlements. *
* NOT_FOUND: No Customer resource found for the name in the request.
*
* Create a request for the method "customers.delete".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the customer to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Deletes the given Customer permanently. Possible error codes: * PERMISSION_DENIED: The account
* making the request does not own this customer. * INVALID_ARGUMENT: Required request parameters
* are missing or invalid. * FAILED_PRECONDITION: The customer has existing entitlements. *
* NOT_FOUND: No Customer resource found for the name in the request.
*
* Create a request for the method "customers.delete".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the customer to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Cloudchannel.this, "DELETE", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource name of the customer to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the customer to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The resource name of the customer to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns the requested Customer resource. Possible error codes: * PERMISSION_DENIED: The reseller
* account making the request is different from the reseller account in the API request. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The customer
* resource doesn't exist. Usually the result of an invalid name parameter. Return value: The
* Customer resource.
*
* Create a request for the method "customers.get".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Returns the requested Customer resource. Possible error codes: * PERMISSION_DENIED: The
* reseller account making the request is different from the reseller account in the API request.
* * INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The
* customer resource doesn't exist. Usually the result of an invalid name parameter. Return value:
* The Customer resource.
*
* Create a request for the method "customers.get".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the customer to retrieve. Name uses the format:
accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the customer to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports a Customer from the Cloud Identity associated with the provided Cloud Identity ID or
* domain before a TransferEntitlements call. If a linked Customer already exists and
* overwrite_if_exists is true, it will update that Customer's data. Possible error codes: *
* PERMISSION_DENIED: * The reseller account making the request is different from the reseller
* account in the API request. * You are not authorized to import the customer. See
* https://support.google.com/channelservices/answer/9759265 * NOT_FOUND: Cloud Identity doesn't
* exist or was deleted. * INVALID_ARGUMENT: Required parameters are missing, or the auth_token is
* expired or invalid. * ALREADY_EXISTS: A customer already exists and has conflicting critical
* fields. Requires an overwrite. Return value: The Customer.
*
* Create a request for the method "customers.import".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link CloudchannelImport#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest}
* @return the request
*/
public CloudchannelImport cloudchannelImport(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest content) throws java.io.IOException {
CloudchannelImport result = new CloudchannelImport(parent, content);
initialize(result);
return result;
}
public class CloudchannelImport extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customers:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Imports a Customer from the Cloud Identity associated with the provided Cloud Identity ID or
* domain before a TransferEntitlements call. If a linked Customer already exists and
* overwrite_if_exists is true, it will update that Customer's data. Possible error codes: *
* PERMISSION_DENIED: * The reseller account making the request is different from the reseller
* account in the API request. * You are not authorized to import the customer. See
* https://support.google.com/channelservices/answer/9759265 * NOT_FOUND: Cloud Identity doesn't
* exist or was deleted. * INVALID_ARGUMENT: Required parameters are missing, or the auth_token is
* expired or invalid. * ALREADY_EXISTS: A customer already exists and has conflicting critical
* fields. Requires an overwrite. Return value: The Customer.
*
* Create a request for the method "customers.import".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link CloudchannelImport#execute()} method to invoke the remote
* operation. {@link CloudchannelImport#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest}
* @since 1.13
*/
protected CloudchannelImport(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ImportCustomerRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public CloudchannelImport set$Xgafv(java.lang.String $Xgafv) {
return (CloudchannelImport) super.set$Xgafv($Xgafv);
}
@Override
public CloudchannelImport setAccessToken(java.lang.String accessToken) {
return (CloudchannelImport) super.setAccessToken(accessToken);
}
@Override
public CloudchannelImport setAlt(java.lang.String alt) {
return (CloudchannelImport) super.setAlt(alt);
}
@Override
public CloudchannelImport setCallback(java.lang.String callback) {
return (CloudchannelImport) super.setCallback(callback);
}
@Override
public CloudchannelImport setFields(java.lang.String fields) {
return (CloudchannelImport) super.setFields(fields);
}
@Override
public CloudchannelImport setKey(java.lang.String key) {
return (CloudchannelImport) super.setKey(key);
}
@Override
public CloudchannelImport setOauthToken(java.lang.String oauthToken) {
return (CloudchannelImport) super.setOauthToken(oauthToken);
}
@Override
public CloudchannelImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CloudchannelImport) super.setPrettyPrint(prettyPrint);
}
@Override
public CloudchannelImport setQuotaUser(java.lang.String quotaUser) {
return (CloudchannelImport) super.setQuotaUser(quotaUser);
}
@Override
public CloudchannelImport setUploadType(java.lang.String uploadType) {
return (CloudchannelImport) super.setUploadType(uploadType);
}
@Override
public CloudchannelImport setUploadProtocol(java.lang.String uploadProtocol) {
return (CloudchannelImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller's account. Parent takes the format:
accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller's account. Parent takes the format:
* accounts/{account_id} or accounts/{account_id}/channelPartnerLinks/{channel_partner_id}
*/
public CloudchannelImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public CloudchannelImport set(String parameterName, Object value) {
return (CloudchannelImport) super.set(parameterName, value);
}
}
/**
* List Customers. Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request is different from the reseller account in the API request. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. Return value: List of Customers, or an empty list if
* there are no customers.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller account to list customers from. Parent uses the format:
* accounts/{account_id}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* List Customers. Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request is different from the reseller account in the API request. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. Return value: List of Customers, or an empty list if
* there are no customers.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the reseller account to list customers from. Parent uses the format:
* accounts/{account_id}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListCustomersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller account to list customers from. Parent uses
* the format: accounts/{account_id}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller account to list customers from. Parent uses the format:
accounts/{account_id}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller account to list customers from. Parent uses
* the format: accounts/{account_id}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Filters applied to the [CloudChannelService.ListCustomers] results. See
* https://cloud.google.com/channel/docs/concepts/google-cloud/filter-customers for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filters applied to the [CloudChannelService.ListCustomers] results. See
https://cloud.google.com/channel/docs/concepts/google-cloud/filter-customers for more information.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Filters applied to the [CloudChannelService.ListCustomers] results. See
* https://cloud.google.com/channel/docs/concepts/google-cloud/filter-customers for more
* information.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of customers to return. The service may return fewer than
* this value. If unspecified, returns at most 10 customers. The maximum value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of customers to return. The service may return fewer than this value.
If unspecified, returns at most 10 customers. The maximum value is 50.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of customers to return. The service may return fewer than
* this value. If unspecified, returns at most 10 customers. The maximum value is 50.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token identifying a page of results other than the first page. Obtained
* through ListCustomersResponse.next_page_token of the previous
* CloudChannelService.ListCustomers call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results other than the first page. Obtained through
ListCustomersResponse.next_page_token of the previous CloudChannelService.ListCustomers call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token identifying a page of results other than the first page. Obtained
* through ListCustomersResponse.next_page_token of the previous
* CloudChannelService.ListCustomers call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists the following: * Offers that you can purchase for a customer. * Offers that you can change
* for an entitlement. Possible error codes: * PERMISSION_DENIED: * The customer doesn't belong to
* the reseller * The reseller is not authorized to transact on this Product. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: Required request
* parameters are missing or invalid.
*
* Create a request for the method "customers.listPurchasableOffers".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ListPurchasableOffers#execute()} method to invoke the remote
* operation.
*
* @param customer Required. The resource name of the customer to list Offers for. Format:
* accounts/{account_id}/customers/{customer_id}.
* @return the request
*/
public ListPurchasableOffers listPurchasableOffers(java.lang.String customer) throws java.io.IOException {
ListPurchasableOffers result = new ListPurchasableOffers(customer);
initialize(result);
return result;
}
public class ListPurchasableOffers extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+customer}:listPurchasableOffers";
private final java.util.regex.Pattern CUSTOMER_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Lists the following: * Offers that you can purchase for a customer. * Offers that you can
* change for an entitlement. Possible error codes: * PERMISSION_DENIED: * The customer doesn't
* belong to the reseller * The reseller is not authorized to transact on this Product. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: Required request
* parameters are missing or invalid.
*
* Create a request for the method "customers.listPurchasableOffers".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ListPurchasableOffers#execute()} method to invoke the
* remote operation. {@link ListPurchasableOffers#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param customer Required. The resource name of the customer to list Offers for. Format:
* accounts/{account_id}/customers/{customer_id}.
* @since 1.13
*/
protected ListPurchasableOffers(java.lang.String customer) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListPurchasableOffersResponse.class);
this.customer = com.google.api.client.util.Preconditions.checkNotNull(customer, "Required parameter customer must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListPurchasableOffers set$Xgafv(java.lang.String $Xgafv) {
return (ListPurchasableOffers) super.set$Xgafv($Xgafv);
}
@Override
public ListPurchasableOffers setAccessToken(java.lang.String accessToken) {
return (ListPurchasableOffers) super.setAccessToken(accessToken);
}
@Override
public ListPurchasableOffers setAlt(java.lang.String alt) {
return (ListPurchasableOffers) super.setAlt(alt);
}
@Override
public ListPurchasableOffers setCallback(java.lang.String callback) {
return (ListPurchasableOffers) super.setCallback(callback);
}
@Override
public ListPurchasableOffers setFields(java.lang.String fields) {
return (ListPurchasableOffers) super.setFields(fields);
}
@Override
public ListPurchasableOffers setKey(java.lang.String key) {
return (ListPurchasableOffers) super.setKey(key);
}
@Override
public ListPurchasableOffers setOauthToken(java.lang.String oauthToken) {
return (ListPurchasableOffers) super.setOauthToken(oauthToken);
}
@Override
public ListPurchasableOffers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListPurchasableOffers) super.setPrettyPrint(prettyPrint);
}
@Override
public ListPurchasableOffers setQuotaUser(java.lang.String quotaUser) {
return (ListPurchasableOffers) super.setQuotaUser(quotaUser);
}
@Override
public ListPurchasableOffers setUploadType(java.lang.String uploadType) {
return (ListPurchasableOffers) super.setUploadType(uploadType);
}
@Override
public ListPurchasableOffers setUploadProtocol(java.lang.String uploadProtocol) {
return (ListPurchasableOffers) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer to list Offers for. Format:
* accounts/{account_id}/customers/{customer_id}.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Required. The resource name of the customer to list Offers for. Format:
accounts/{account_id}/customers/{customer_id}.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Required. The resource name of the customer to list Offers for. Format:
* accounts/{account_id}/customers/{customer_id}.
*/
public ListPurchasableOffers setCustomer(java.lang.String customer) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.customer = customer;
return this;
}
/**
* Optional. Resource name of the new target Billing Account. Provide this Billing Account
* when setting up billing for a trial subscription. Format:
* accounts/{account_id}/billingAccounts/{billing_account_id}. This field is only relevant
* for multi-currency accounts. It should be left empty for single currency accounts.
*/
@com.google.api.client.util.Key("changeOfferPurchase.billingAccount")
private java.lang.String changeOfferPurchaseBillingAccount;
/** Optional. Resource name of the new target Billing Account. Provide this Billing Account when
setting up billing for a trial subscription. Format:
accounts/{account_id}/billingAccounts/{billing_account_id}. This field is only relevant for multi-
currency accounts. It should be left empty for single currency accounts.
*/
public java.lang.String getChangeOfferPurchaseBillingAccount() {
return changeOfferPurchaseBillingAccount;
}
/**
* Optional. Resource name of the new target Billing Account. Provide this Billing Account
* when setting up billing for a trial subscription. Format:
* accounts/{account_id}/billingAccounts/{billing_account_id}. This field is only relevant
* for multi-currency accounts. It should be left empty for single currency accounts.
*/
public ListPurchasableOffers setChangeOfferPurchaseBillingAccount(java.lang.String changeOfferPurchaseBillingAccount) {
this.changeOfferPurchaseBillingAccount = changeOfferPurchaseBillingAccount;
return this;
}
/**
* Required. Resource name of the entitlement. Format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key("changeOfferPurchase.entitlement")
private java.lang.String changeOfferPurchaseEntitlement;
/** Required. Resource name of the entitlement. Format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getChangeOfferPurchaseEntitlement() {
return changeOfferPurchaseEntitlement;
}
/**
* Required. Resource name of the entitlement. Format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public ListPurchasableOffers setChangeOfferPurchaseEntitlement(java.lang.String changeOfferPurchaseEntitlement) {
this.changeOfferPurchaseEntitlement = changeOfferPurchaseEntitlement;
return this;
}
/**
* Optional. Resource name of the new target SKU. Provide this SKU when upgrading or
* downgrading an entitlement. Format: products/{product_id}/skus/{sku_id}
*/
@com.google.api.client.util.Key("changeOfferPurchase.newSku")
private java.lang.String changeOfferPurchaseNewSku;
/** Optional. Resource name of the new target SKU. Provide this SKU when upgrading or downgrading an
entitlement. Format: products/{product_id}/skus/{sku_id}
*/
public java.lang.String getChangeOfferPurchaseNewSku() {
return changeOfferPurchaseNewSku;
}
/**
* Optional. Resource name of the new target SKU. Provide this SKU when upgrading or
* downgrading an entitlement. Format: products/{product_id}/skus/{sku_id}
*/
public ListPurchasableOffers setChangeOfferPurchaseNewSku(java.lang.String changeOfferPurchaseNewSku) {
this.changeOfferPurchaseNewSku = changeOfferPurchaseNewSku;
return this;
}
/**
* Optional. Billing account that the result should be restricted to. Format:
* accounts/{account_id}/billingAccounts/{billing_account_id}.
*/
@com.google.api.client.util.Key("createEntitlementPurchase.billingAccount")
private java.lang.String createEntitlementPurchaseBillingAccount;
/** Optional. Billing account that the result should be restricted to. Format:
accounts/{account_id}/billingAccounts/{billing_account_id}.
*/
public java.lang.String getCreateEntitlementPurchaseBillingAccount() {
return createEntitlementPurchaseBillingAccount;
}
/**
* Optional. Billing account that the result should be restricted to. Format:
* accounts/{account_id}/billingAccounts/{billing_account_id}.
*/
public ListPurchasableOffers setCreateEntitlementPurchaseBillingAccount(java.lang.String createEntitlementPurchaseBillingAccount) {
this.createEntitlementPurchaseBillingAccount = createEntitlementPurchaseBillingAccount;
return this;
}
/**
* Required. SKU that the result should be restricted to. Format:
* products/{product_id}/skus/{sku_id}.
*/
@com.google.api.client.util.Key("createEntitlementPurchase.sku")
private java.lang.String createEntitlementPurchaseSku;
/** Required. SKU that the result should be restricted to. Format: products/{product_id}/skus/{sku_id}.
*/
public java.lang.String getCreateEntitlementPurchaseSku() {
return createEntitlementPurchaseSku;
}
/**
* Required. SKU that the result should be restricted to. Format:
* products/{product_id}/skus/{sku_id}.
*/
public ListPurchasableOffers setCreateEntitlementPurchaseSku(java.lang.String createEntitlementPurchaseSku) {
this.createEntitlementPurchaseSku = createEntitlementPurchaseSku;
return this;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** Optional. The BCP-47 language code. For example, "en-US". The response will localize in the
corresponding language code, if specified. The default value is "en-US".
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
public ListPurchasableOffers setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 Offers. The maximum value is 1000; the server will
* coerce values above 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server might return fewer results than requested. If unspecified,
returns at most 100 Offers. The maximum value is 1000; the server will coerce values above 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 Offers. The maximum value is 1000; the server will
* coerce values above 1000.
*/
public ListPurchasableOffers setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token for a page of results other than the first page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token for a page of results other than the first page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token for a page of results other than the first page. */
public ListPurchasableOffers setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListPurchasableOffers set(String parameterName, Object value) {
return (ListPurchasableOffers) super.set(parameterName, value);
}
}
/**
* Lists the following: * SKUs that you can purchase for a customer * SKUs that you can upgrade or
* downgrade for an entitlement. Possible error codes: * PERMISSION_DENIED: The customer doesn't
* belong to the reseller. * INVALID_ARGUMENT: Required request parameters are missing or invalid.
*
* Create a request for the method "customers.listPurchasableSkus".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ListPurchasableSkus#execute()} method to invoke the remote operation.
*
* @param customer Required. The resource name of the customer to list SKUs for. Format:
* accounts/{account_id}/customers/{customer_id}.
* @return the request
*/
public ListPurchasableSkus listPurchasableSkus(java.lang.String customer) throws java.io.IOException {
ListPurchasableSkus result = new ListPurchasableSkus(customer);
initialize(result);
return result;
}
public class ListPurchasableSkus extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+customer}:listPurchasableSkus";
private final java.util.regex.Pattern CUSTOMER_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Lists the following: * SKUs that you can purchase for a customer * SKUs that you can upgrade or
* downgrade for an entitlement. Possible error codes: * PERMISSION_DENIED: The customer doesn't
* belong to the reseller. * INVALID_ARGUMENT: Required request parameters are missing or invalid.
*
* Create a request for the method "customers.listPurchasableSkus".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ListPurchasableSkus#execute()} method to invoke the remote
* operation. {@link ListPurchasableSkus#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param customer Required. The resource name of the customer to list SKUs for. Format:
* accounts/{account_id}/customers/{customer_id}.
* @since 1.13
*/
protected ListPurchasableSkus(java.lang.String customer) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListPurchasableSkusResponse.class);
this.customer = com.google.api.client.util.Preconditions.checkNotNull(customer, "Required parameter customer must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListPurchasableSkus set$Xgafv(java.lang.String $Xgafv) {
return (ListPurchasableSkus) super.set$Xgafv($Xgafv);
}
@Override
public ListPurchasableSkus setAccessToken(java.lang.String accessToken) {
return (ListPurchasableSkus) super.setAccessToken(accessToken);
}
@Override
public ListPurchasableSkus setAlt(java.lang.String alt) {
return (ListPurchasableSkus) super.setAlt(alt);
}
@Override
public ListPurchasableSkus setCallback(java.lang.String callback) {
return (ListPurchasableSkus) super.setCallback(callback);
}
@Override
public ListPurchasableSkus setFields(java.lang.String fields) {
return (ListPurchasableSkus) super.setFields(fields);
}
@Override
public ListPurchasableSkus setKey(java.lang.String key) {
return (ListPurchasableSkus) super.setKey(key);
}
@Override
public ListPurchasableSkus setOauthToken(java.lang.String oauthToken) {
return (ListPurchasableSkus) super.setOauthToken(oauthToken);
}
@Override
public ListPurchasableSkus setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListPurchasableSkus) super.setPrettyPrint(prettyPrint);
}
@Override
public ListPurchasableSkus setQuotaUser(java.lang.String quotaUser) {
return (ListPurchasableSkus) super.setQuotaUser(quotaUser);
}
@Override
public ListPurchasableSkus setUploadType(java.lang.String uploadType) {
return (ListPurchasableSkus) super.setUploadType(uploadType);
}
@Override
public ListPurchasableSkus setUploadProtocol(java.lang.String uploadProtocol) {
return (ListPurchasableSkus) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer to list SKUs for. Format:
* accounts/{account_id}/customers/{customer_id}.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Required. The resource name of the customer to list SKUs for. Format:
accounts/{account_id}/customers/{customer_id}.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Required. The resource name of the customer to list SKUs for. Format:
* accounts/{account_id}/customers/{customer_id}.
*/
public ListPurchasableSkus setCustomer(java.lang.String customer) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.customer = customer;
return this;
}
/** Required. Change Type for the entitlement. */
@com.google.api.client.util.Key("changeOfferPurchase.changeType")
private java.lang.String changeOfferPurchaseChangeType;
/** Required. Change Type for the entitlement.
*/
public java.lang.String getChangeOfferPurchaseChangeType() {
return changeOfferPurchaseChangeType;
}
/** Required. Change Type for the entitlement. */
public ListPurchasableSkus setChangeOfferPurchaseChangeType(java.lang.String changeOfferPurchaseChangeType) {
this.changeOfferPurchaseChangeType = changeOfferPurchaseChangeType;
return this;
}
/**
* Required. Resource name of the entitlement. Format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key("changeOfferPurchase.entitlement")
private java.lang.String changeOfferPurchaseEntitlement;
/** Required. Resource name of the entitlement. Format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getChangeOfferPurchaseEntitlement() {
return changeOfferPurchaseEntitlement;
}
/**
* Required. Resource name of the entitlement. Format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public ListPurchasableSkus setChangeOfferPurchaseEntitlement(java.lang.String changeOfferPurchaseEntitlement) {
this.changeOfferPurchaseEntitlement = changeOfferPurchaseEntitlement;
return this;
}
/**
* Required. List SKUs belonging to this Product. Format: products/{product_id}. Supports
* products/- to retrieve SKUs for all products.
*/
@com.google.api.client.util.Key("createEntitlementPurchase.product")
private java.lang.String createEntitlementPurchaseProduct;
/** Required. List SKUs belonging to this Product. Format: products/{product_id}. Supports products/-
to retrieve SKUs for all products.
*/
public java.lang.String getCreateEntitlementPurchaseProduct() {
return createEntitlementPurchaseProduct;
}
/**
* Required. List SKUs belonging to this Product. Format: products/{product_id}. Supports
* products/- to retrieve SKUs for all products.
*/
public ListPurchasableSkus setCreateEntitlementPurchaseProduct(java.lang.String createEntitlementPurchaseProduct) {
this.createEntitlementPurchaseProduct = createEntitlementPurchaseProduct;
return this;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** Optional. The BCP-47 language code. For example, "en-US". The response will localize in the
corresponding language code, if specified. The default value is "en-US".
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
public ListPurchasableSkus setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 SKUs. The maximum value is 1000; the server will coerce
* values above 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server might return fewer results than requested. If unspecified,
returns at most 100 SKUs. The maximum value is 1000; the server will coerce values above 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 SKUs. The maximum value is 1000; the server will coerce
* values above 1000.
*/
public ListPurchasableSkus setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token for a page of results other than the first page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token for a page of results other than the first page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token for a page of results other than the first page. */
public ListPurchasableSkus setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListPurchasableSkus set(String parameterName, Object value) {
return (ListPurchasableSkus) super.set(parameterName, value);
}
}
/**
* Updates an existing Customer resource for the reseller or distributor. Possible error codes: *
* PERMISSION_DENIED: The reseller account making the request is different from the reseller account
* in the API request. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* NOT_FOUND: No Customer resource found for the name in the request. Return value: The updated
* Customer resource.
*
* Create a request for the method "customers.patch".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Updates an existing Customer resource for the reseller or distributor. Possible error codes: *
* PERMISSION_DENIED: The reseller account making the request is different from the reseller
* account in the API request. * INVALID_ARGUMENT: Required request parameters are missing or
* invalid. * NOT_FOUND: No Customer resource found for the name in the request. Return value: The
* updated Customer resource.
*
* Create a request for the method "customers.patch".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer content) {
super(Cloudchannel.this, "PATCH", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Customer.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Resource name of the customer. Format:
* accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the customer. Format:
* accounts/{account_id}/customers/{customer_id}
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.name = name;
return this;
}
/** The update mask that applies to the resource. Optional. */
@com.google.api.client.util.Key
private String updateMask;
/** The update mask that applies to the resource. Optional.
*/
public String getUpdateMask() {
return updateMask;
}
/** The update mask that applies to the resource. Optional. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Creates a Cloud Identity for the given customer using the customer's information, or the
* information provided here. Possible error codes: * PERMISSION_DENIED: * The customer doesn't
* belong to the reseller. * You are not authorized to provision cloud identity id. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: Required request
* parameters are missing or invalid. * NOT_FOUND: The customer was not found. * ALREADY_EXISTS: The
* customer's primary email already exists. Retry after changing the customer's primary contact
* email. * INTERNAL: Any non-user error related to a technical issue in the backend. Contact Cloud
* Channel support. * UNKNOWN: Any non-user error related to a technical issue in the backend.
* Contact Cloud Channel support. Return value: The ID of a long-running operation. To get the
* results of the operation, call the GetOperation method of CloudChannelOperationsService. The
* Operation metadata contains an instance of OperationMetadata.
*
* Create a request for the method "customers.provisionCloudIdentity".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ProvisionCloudIdentity#execute()} method to invoke the remote
* operation.
*
* @param customer Required. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ProvisionCloudIdentityRequest}
* @return the request
*/
public ProvisionCloudIdentity provisionCloudIdentity(java.lang.String customer, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ProvisionCloudIdentityRequest content) throws java.io.IOException {
ProvisionCloudIdentity result = new ProvisionCloudIdentity(customer, content);
initialize(result);
return result;
}
public class ProvisionCloudIdentity extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+customer}:provisionCloudIdentity";
private final java.util.regex.Pattern CUSTOMER_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Creates a Cloud Identity for the given customer using the customer's information, or the
* information provided here. Possible error codes: * PERMISSION_DENIED: * The customer doesn't
* belong to the reseller. * You are not authorized to provision cloud identity id. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: Required request
* parameters are missing or invalid. * NOT_FOUND: The customer was not found. * ALREADY_EXISTS:
* The customer's primary email already exists. Retry after changing the customer's primary
* contact email. * INTERNAL: Any non-user error related to a technical issue in the backend.
* Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical issue in
* the backend. Contact Cloud Channel support. Return value: The ID of a long-running operation.
* To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata contains an instance of
* OperationMetadata.
*
* Create a request for the method "customers.provisionCloudIdentity".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ProvisionCloudIdentity#execute()} method to invoke the
* remote operation. {@link ProvisionCloudIdentity#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param customer Required. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ProvisionCloudIdentityRequest}
* @since 1.13
*/
protected ProvisionCloudIdentity(java.lang.String customer, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ProvisionCloudIdentityRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.customer = com.google.api.client.util.Preconditions.checkNotNull(customer, "Required parameter customer must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public ProvisionCloudIdentity set$Xgafv(java.lang.String $Xgafv) {
return (ProvisionCloudIdentity) super.set$Xgafv($Xgafv);
}
@Override
public ProvisionCloudIdentity setAccessToken(java.lang.String accessToken) {
return (ProvisionCloudIdentity) super.setAccessToken(accessToken);
}
@Override
public ProvisionCloudIdentity setAlt(java.lang.String alt) {
return (ProvisionCloudIdentity) super.setAlt(alt);
}
@Override
public ProvisionCloudIdentity setCallback(java.lang.String callback) {
return (ProvisionCloudIdentity) super.setCallback(callback);
}
@Override
public ProvisionCloudIdentity setFields(java.lang.String fields) {
return (ProvisionCloudIdentity) super.setFields(fields);
}
@Override
public ProvisionCloudIdentity setKey(java.lang.String key) {
return (ProvisionCloudIdentity) super.setKey(key);
}
@Override
public ProvisionCloudIdentity setOauthToken(java.lang.String oauthToken) {
return (ProvisionCloudIdentity) super.setOauthToken(oauthToken);
}
@Override
public ProvisionCloudIdentity setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ProvisionCloudIdentity) super.setPrettyPrint(prettyPrint);
}
@Override
public ProvisionCloudIdentity setQuotaUser(java.lang.String quotaUser) {
return (ProvisionCloudIdentity) super.setQuotaUser(quotaUser);
}
@Override
public ProvisionCloudIdentity setUploadType(java.lang.String uploadType) {
return (ProvisionCloudIdentity) super.setUploadType(uploadType);
}
@Override
public ProvisionCloudIdentity setUploadProtocol(java.lang.String uploadProtocol) {
return (ProvisionCloudIdentity) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the customer. Format:
* accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Required. Resource name of the customer. Format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Required. Resource name of the customer. Format:
* accounts/{account_id}/customers/{customer_id}
*/
public ProvisionCloudIdentity setCustomer(java.lang.String customer) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.customer = customer;
return this;
}
@Override
public ProvisionCloudIdentity set(String parameterName, Object value) {
return (ProvisionCloudIdentity) super.set(parameterName, value);
}
}
/**
* Lists the billing accounts that are eligible to purchase particular SKUs for a given customer.
* Possible error codes: * PERMISSION_DENIED: The customer doesn't belong to the reseller. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. Return value: Based on the
* provided list of SKUs, returns a list of SKU groups that must be purchased using the same billing
* account and the billing accounts eligible to purchase each SKU group.
*
* Create a request for the method "customers.queryEligibleBillingAccounts".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link QueryEligibleBillingAccounts#execute()} method to invoke the remote
* operation.
*
* @param customer Required. The resource name of the customer to list eligible billing accounts for. Format:
* accounts/{account_id}/customers/{customer_id}.
* @return the request
*/
public QueryEligibleBillingAccounts queryEligibleBillingAccounts(java.lang.String customer) throws java.io.IOException {
QueryEligibleBillingAccounts result = new QueryEligibleBillingAccounts(customer);
initialize(result);
return result;
}
public class QueryEligibleBillingAccounts extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+customer}:queryEligibleBillingAccounts";
private final java.util.regex.Pattern CUSTOMER_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Lists the billing accounts that are eligible to purchase particular SKUs for a given customer.
* Possible error codes: * PERMISSION_DENIED: The customer doesn't belong to the reseller. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. Return value: Based on
* the provided list of SKUs, returns a list of SKU groups that must be purchased using the same
* billing account and the billing accounts eligible to purchase each SKU group.
*
* Create a request for the method "customers.queryEligibleBillingAccounts".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link QueryEligibleBillingAccounts#execute()} method to invoke
* the remote operation. {@link QueryEligibleBillingAccounts#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param customer Required. The resource name of the customer to list eligible billing accounts for. Format:
* accounts/{account_id}/customers/{customer_id}.
* @since 1.13
*/
protected QueryEligibleBillingAccounts(java.lang.String customer) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1QueryEligibleBillingAccountsResponse.class);
this.customer = com.google.api.client.util.Preconditions.checkNotNull(customer, "Required parameter customer must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public QueryEligibleBillingAccounts set$Xgafv(java.lang.String $Xgafv) {
return (QueryEligibleBillingAccounts) super.set$Xgafv($Xgafv);
}
@Override
public QueryEligibleBillingAccounts setAccessToken(java.lang.String accessToken) {
return (QueryEligibleBillingAccounts) super.setAccessToken(accessToken);
}
@Override
public QueryEligibleBillingAccounts setAlt(java.lang.String alt) {
return (QueryEligibleBillingAccounts) super.setAlt(alt);
}
@Override
public QueryEligibleBillingAccounts setCallback(java.lang.String callback) {
return (QueryEligibleBillingAccounts) super.setCallback(callback);
}
@Override
public QueryEligibleBillingAccounts setFields(java.lang.String fields) {
return (QueryEligibleBillingAccounts) super.setFields(fields);
}
@Override
public QueryEligibleBillingAccounts setKey(java.lang.String key) {
return (QueryEligibleBillingAccounts) super.setKey(key);
}
@Override
public QueryEligibleBillingAccounts setOauthToken(java.lang.String oauthToken) {
return (QueryEligibleBillingAccounts) super.setOauthToken(oauthToken);
}
@Override
public QueryEligibleBillingAccounts setPrettyPrint(java.lang.Boolean prettyPrint) {
return (QueryEligibleBillingAccounts) super.setPrettyPrint(prettyPrint);
}
@Override
public QueryEligibleBillingAccounts setQuotaUser(java.lang.String quotaUser) {
return (QueryEligibleBillingAccounts) super.setQuotaUser(quotaUser);
}
@Override
public QueryEligibleBillingAccounts setUploadType(java.lang.String uploadType) {
return (QueryEligibleBillingAccounts) super.setUploadType(uploadType);
}
@Override
public QueryEligibleBillingAccounts setUploadProtocol(java.lang.String uploadProtocol) {
return (QueryEligibleBillingAccounts) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer to list eligible billing accounts for.
* Format: accounts/{account_id}/customers/{customer_id}.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Required. The resource name of the customer to list eligible billing accounts for. Format:
accounts/{account_id}/customers/{customer_id}.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Required. The resource name of the customer to list eligible billing accounts for.
* Format: accounts/{account_id}/customers/{customer_id}.
*/
public QueryEligibleBillingAccounts setCustomer(java.lang.String customer) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CUSTOMER_PATTERN.matcher(customer).matches(),
"Parameter customer must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.customer = customer;
return this;
}
/**
* Required. List of SKUs to list eligible billing accounts for. At least one SKU is
* required. Format: products/{product_id}/skus/{sku_id}.
*/
@com.google.api.client.util.Key
private java.util.List skus;
/** Required. List of SKUs to list eligible billing accounts for. At least one SKU is required. Format:
products/{product_id}/skus/{sku_id}.
*/
public java.util.List getSkus() {
return skus;
}
/**
* Required. List of SKUs to list eligible billing accounts for. At least one SKU is
* required. Format: products/{product_id}/skus/{sku_id}.
*/
public QueryEligibleBillingAccounts setSkus(java.util.List skus) {
this.skus = skus;
return this;
}
@Override
public QueryEligibleBillingAccounts set(String parameterName, Object value) {
return (QueryEligibleBillingAccounts) super.set(parameterName, value);
}
}
/**
* Transfers customer entitlements to new reseller. Possible error codes: * PERMISSION_DENIED: * The
* customer doesn't belong to the reseller. * The reseller is not authorized to transact on this
* Product. See https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT:
* Required request parameters are missing or invalid. * NOT_FOUND: The customer or offer resource
* was not found. * ALREADY_EXISTS: The SKU was already transferred for the customer. *
* CONDITION_NOT_MET or FAILED_PRECONDITION: * The SKU requires domain verification to transfer, but
* the domain is not verified. * An Add-On SKU (example, Vault or Drive) is missing the pre-
* requisite SKU (example, G Suite Basic). * (Developer accounts only) Reseller and resold domain
* must meet the following naming requirements: * Domain names must start with goog-test. * Domain
* names must include the reseller domain. * Specify all transferring entitlements. * INTERNAL: Any
* non-user error related to a technical issue in the backend. Contact Cloud Channel support. *
* UNKNOWN: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. Return value: The ID of a long-running operation. To get the results of the operation,
* call the GetOperation method of CloudChannelOperationsService. The Operation metadata will
* contain an instance of OperationMetadata.
*
* Create a request for the method "customers.transferEntitlements".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link TransferEntitlements#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The resource name of the reseller's customer account that will receive transferred
* entitlements. Parent uses the format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsRequest}
* @return the request
*/
public TransferEntitlements transferEntitlements(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsRequest content) throws java.io.IOException {
TransferEntitlements result = new TransferEntitlements(parent, content);
initialize(result);
return result;
}
public class TransferEntitlements extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}:transferEntitlements";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Transfers customer entitlements to new reseller. Possible error codes: * PERMISSION_DENIED: *
* The customer doesn't belong to the reseller. * The reseller is not authorized to transact on
* this Product. See https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT:
* Required request parameters are missing or invalid. * NOT_FOUND: The customer or offer resource
* was not found. * ALREADY_EXISTS: The SKU was already transferred for the customer. *
* CONDITION_NOT_MET or FAILED_PRECONDITION: * The SKU requires domain verification to transfer,
* but the domain is not verified. * An Add-On SKU (example, Vault or Drive) is missing the pre-
* requisite SKU (example, G Suite Basic). * (Developer accounts only) Reseller and resold domain
* must meet the following naming requirements: * Domain names must start with goog-test. * Domain
* names must include the reseller domain. * Specify all transferring entitlements. * INTERNAL:
* Any non-user error related to a technical issue in the backend. Contact Cloud Channel support.
* * UNKNOWN: Any non-user error related to a technical issue in the backend. Contact Cloud
* Channel support. Return value: The ID of a long-running operation. To get the results of the
* operation, call the GetOperation method of CloudChannelOperationsService. The Operation
* metadata will contain an instance of OperationMetadata.
*
* Create a request for the method "customers.transferEntitlements".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link TransferEntitlements#execute()} method to invoke the
* remote operation. {@link TransferEntitlements#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent Required. The resource name of the reseller's customer account that will receive transferred
* entitlements. Parent uses the format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsRequest}
* @since 1.13
*/
protected TransferEntitlements(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public TransferEntitlements set$Xgafv(java.lang.String $Xgafv) {
return (TransferEntitlements) super.set$Xgafv($Xgafv);
}
@Override
public TransferEntitlements setAccessToken(java.lang.String accessToken) {
return (TransferEntitlements) super.setAccessToken(accessToken);
}
@Override
public TransferEntitlements setAlt(java.lang.String alt) {
return (TransferEntitlements) super.setAlt(alt);
}
@Override
public TransferEntitlements setCallback(java.lang.String callback) {
return (TransferEntitlements) super.setCallback(callback);
}
@Override
public TransferEntitlements setFields(java.lang.String fields) {
return (TransferEntitlements) super.setFields(fields);
}
@Override
public TransferEntitlements setKey(java.lang.String key) {
return (TransferEntitlements) super.setKey(key);
}
@Override
public TransferEntitlements setOauthToken(java.lang.String oauthToken) {
return (TransferEntitlements) super.setOauthToken(oauthToken);
}
@Override
public TransferEntitlements setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TransferEntitlements) super.setPrettyPrint(prettyPrint);
}
@Override
public TransferEntitlements setQuotaUser(java.lang.String quotaUser) {
return (TransferEntitlements) super.setQuotaUser(quotaUser);
}
@Override
public TransferEntitlements setUploadType(java.lang.String uploadType) {
return (TransferEntitlements) super.setUploadType(uploadType);
}
@Override
public TransferEntitlements setUploadProtocol(java.lang.String uploadProtocol) {
return (TransferEntitlements) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller's customer account that will receive
* transferred entitlements. Parent uses the format:
* accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller's customer account that will receive transferred
entitlements. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller's customer account that will receive
* transferred entitlements. Parent uses the format:
* accounts/{account_id}/customers/{customer_id}
*/
public TransferEntitlements setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public TransferEntitlements set(String parameterName, Object value) {
return (TransferEntitlements) super.set(parameterName, value);
}
}
/**
* Transfers customer entitlements from their current reseller to Google. Possible error codes: *
* PERMISSION_DENIED: The customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. * NOT_FOUND: The customer or offer resource was not
* found. * ALREADY_EXISTS: The SKU was already transferred for the customer. * CONDITION_NOT_MET or
* FAILED_PRECONDITION: * The SKU requires domain verification to transfer, but the domain is not
* verified. * An Add-On SKU (example, Vault or Drive) is missing the pre-requisite SKU (example, G
* Suite Basic). * (Developer accounts only) Reseller and resold domain must meet the following
* naming requirements: * Domain names must start with goog-test. * Domain names must include the
* reseller domain. * INTERNAL: Any non-user error related to a technical issue in the backend.
* Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. Return value: The ID of a long-running operation. To get
* the results of the operation, call the GetOperation method of CloudChannelOperationsService. The
* response will contain google.protobuf.Empty on success. The Operation metadata will contain an
* instance of OperationMetadata.
*
* Create a request for the method "customers.transferEntitlementsToGoogle".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link TransferEntitlementsToGoogle#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The resource name of the reseller's customer account where the entitlements transfer from.
* Parent uses the format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsToGoogleRequest}
* @return the request
*/
public TransferEntitlementsToGoogle transferEntitlementsToGoogle(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsToGoogleRequest content) throws java.io.IOException {
TransferEntitlementsToGoogle result = new TransferEntitlementsToGoogle(parent, content);
initialize(result);
return result;
}
public class TransferEntitlementsToGoogle extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}:transferEntitlementsToGoogle";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Transfers customer entitlements from their current reseller to Google. Possible error codes: *
* PERMISSION_DENIED: The customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. * NOT_FOUND: The customer or offer resource was not
* found. * ALREADY_EXISTS: The SKU was already transferred for the customer. * CONDITION_NOT_MET
* or FAILED_PRECONDITION: * The SKU requires domain verification to transfer, but the domain is
* not verified. * An Add-On SKU (example, Vault or Drive) is missing the pre-requisite SKU
* (example, G Suite Basic). * (Developer accounts only) Reseller and resold domain must meet the
* following naming requirements: * Domain names must start with goog-test. * Domain names must
* include the reseller domain. * INTERNAL: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The ID of a long-running
* operation. To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The response will contain google.protobuf.Empty on success. The
* Operation metadata will contain an instance of OperationMetadata.
*
* Create a request for the method "customers.transferEntitlementsToGoogle".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link TransferEntitlementsToGoogle#execute()} method to invoke
* the remote operation. {@link TransferEntitlementsToGoogle#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param parent Required. The resource name of the reseller's customer account where the entitlements transfer from.
* Parent uses the format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsToGoogleRequest}
* @since 1.13
*/
protected TransferEntitlementsToGoogle(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1TransferEntitlementsToGoogleRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public TransferEntitlementsToGoogle set$Xgafv(java.lang.String $Xgafv) {
return (TransferEntitlementsToGoogle) super.set$Xgafv($Xgafv);
}
@Override
public TransferEntitlementsToGoogle setAccessToken(java.lang.String accessToken) {
return (TransferEntitlementsToGoogle) super.setAccessToken(accessToken);
}
@Override
public TransferEntitlementsToGoogle setAlt(java.lang.String alt) {
return (TransferEntitlementsToGoogle) super.setAlt(alt);
}
@Override
public TransferEntitlementsToGoogle setCallback(java.lang.String callback) {
return (TransferEntitlementsToGoogle) super.setCallback(callback);
}
@Override
public TransferEntitlementsToGoogle setFields(java.lang.String fields) {
return (TransferEntitlementsToGoogle) super.setFields(fields);
}
@Override
public TransferEntitlementsToGoogle setKey(java.lang.String key) {
return (TransferEntitlementsToGoogle) super.setKey(key);
}
@Override
public TransferEntitlementsToGoogle setOauthToken(java.lang.String oauthToken) {
return (TransferEntitlementsToGoogle) super.setOauthToken(oauthToken);
}
@Override
public TransferEntitlementsToGoogle setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TransferEntitlementsToGoogle) super.setPrettyPrint(prettyPrint);
}
@Override
public TransferEntitlementsToGoogle setQuotaUser(java.lang.String quotaUser) {
return (TransferEntitlementsToGoogle) super.setQuotaUser(quotaUser);
}
@Override
public TransferEntitlementsToGoogle setUploadType(java.lang.String uploadType) {
return (TransferEntitlementsToGoogle) super.setUploadType(uploadType);
}
@Override
public TransferEntitlementsToGoogle setUploadProtocol(java.lang.String uploadProtocol) {
return (TransferEntitlementsToGoogle) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller's customer account where the entitlements
* transfer from. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller's customer account where the entitlements transfer
from. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller's customer account where the entitlements
* transfer from. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
public TransferEntitlementsToGoogle setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public TransferEntitlementsToGoogle set(String parameterName, Object value) {
return (TransferEntitlementsToGoogle) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the CustomerRepricingConfigs collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.CustomerRepricingConfigs.List request = cloudchannel.customerRepricingConfigs().list(parameters ...)}
*
*
* @return the resource collection
*/
public CustomerRepricingConfigs customerRepricingConfigs() {
return new CustomerRepricingConfigs();
}
/**
* The "customerRepricingConfigs" collection of methods.
*/
public class CustomerRepricingConfigs {
/**
* Creates a CustomerRepricingConfig. Call this method to set modifications for a specific
* customer's bill. You can only create configs if the RepricingConfig.effective_invoice_month is a
* future month. If needed, you can create a config for the current month, with some restrictions.
* When creating a config for a future month, make sure there are no existing configs for that
* RepricingConfig.effective_invoice_month. The following restrictions are for creating configs in
* the current month. * This functionality is reserved for recovering from an erroneous config, and
* should not be used for regular business cases. * The new config will not modify exports used with
* other configs. Changes to the config may be immediate, but may take up to 24 hours. * There is a
* limit of ten configs for any RepricingConfig.EntitlementGranularity.entitlement, for any
* RepricingConfig.effective_invoice_month. * The contained CustomerRepricingConfig.repricing_config
* value must be different from the value used in the current config for a
* RepricingConfig.EntitlementGranularity.entitlement. Possible Error Codes: * PERMISSION_DENIED: If
* the account making the request and the account being queried are different. * INVALID_ARGUMENT:
* Missing or invalid required parameters in the request. Also displays if the updated config is for
* the current month or past months. * NOT_FOUND: The CustomerRepricingConfig specified does not
* exist or is not associated with the given account. * INTERNAL: Any non-user error related to
* technical issues in the backend. In this case, contact Cloud Channel support. Return Value: If
* successful, the updated CustomerRepricingConfig resource, otherwise returns an error.
*
* Create a request for the method "customerRepricingConfigs.create".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the customer that will receive this repricing config. Parent uses the
* format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customerRepricingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Creates a CustomerRepricingConfig. Call this method to set modifications for a specific
* customer's bill. You can only create configs if the RepricingConfig.effective_invoice_month is
* a future month. If needed, you can create a config for the current month, with some
* restrictions. When creating a config for a future month, make sure there are no existing
* configs for that RepricingConfig.effective_invoice_month. The following restrictions are for
* creating configs in the current month. * This functionality is reserved for recovering from an
* erroneous config, and should not be used for regular business cases. * The new config will not
* modify exports used with other configs. Changes to the config may be immediate, but may take up
* to 24 hours. * There is a limit of ten configs for any
* RepricingConfig.EntitlementGranularity.entitlement, for any
* RepricingConfig.effective_invoice_month. * The contained
* CustomerRepricingConfig.repricing_config value must be different from the value used in the
* current config for a RepricingConfig.EntitlementGranularity.entitlement. Possible Error Codes:
* * PERMISSION_DENIED: If the account making the request and the account being queried are
* different. * INVALID_ARGUMENT: Missing or invalid required parameters in the request. Also
* displays if the updated config is for the current month or past months. * NOT_FOUND: The
* CustomerRepricingConfig specified does not exist or is not associated with the given account. *
* INTERNAL: Any non-user error related to technical issues in the backend. In this case, contact
* Cloud Channel support. Return Value: If successful, the updated CustomerRepricingConfig
* resource, otherwise returns an error.
*
* Create a request for the method "customerRepricingConfigs.create".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the customer that will receive this repricing config. Parent uses the
* format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer that will receive this repricing config.
* Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the customer that will receive this repricing config. Parent uses
the format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the customer that will receive this repricing config.
* Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the given CustomerRepricingConfig permanently. You can only delete configs if their
* RepricingConfig.effective_invoice_month is set to a date after the current month. Possible error
* codes: * PERMISSION_DENIED: The account making the request does not own this customer. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * FAILED_PRECONDITION: The
* CustomerRepricingConfig is active or in the past. * NOT_FOUND: No CustomerRepricingConfig found
* for the name in the request.
*
* Create a request for the method "customerRepricingConfigs.delete".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the customer repricing config rule to delete. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
/**
* Deletes the given CustomerRepricingConfig permanently. You can only delete configs if their
* RepricingConfig.effective_invoice_month is set to a date after the current month. Possible
* error codes: * PERMISSION_DENIED: The account making the request does not own this customer. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * FAILED_PRECONDITION:
* The CustomerRepricingConfig is active or in the past. * NOT_FOUND: No CustomerRepricingConfig
* found for the name in the request.
*
* Create a request for the method "customerRepricingConfigs.delete".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the customer repricing config rule to delete. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Cloudchannel.this, "DELETE", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer repricing config rule to delete. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the customer repricing config rule to delete. Format:
accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the customer repricing config rule to delete. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about how a Reseller modifies their bill before sending it to a Customer.
* Possible Error Codes: * PERMISSION_DENIED: If the account making the request and the account
* being queried are different. * NOT_FOUND: The CustomerRepricingConfig was not found. * INTERNAL:
* Any non-user error related to technical issues in the backend. In this case, contact Cloud
* Channel support. Return Value: If successful, the CustomerRepricingConfig resource, otherwise
* returns an error.
*
* Create a request for the method "customerRepricingConfigs.get".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
/**
* Gets information about how a Reseller modifies their bill before sending it to a Customer.
* Possible Error Codes: * PERMISSION_DENIED: If the account making the request and the account
* being queried are different. * NOT_FOUND: The CustomerRepricingConfig was not found. *
* INTERNAL: Any non-user error related to technical issues in the backend. In this case, contact
* Cloud Channel support. Return Value: If successful, the CustomerRepricingConfig resource,
* otherwise returns an error.
*
* Create a request for the method "customerRepricingConfigs.get".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the CustomerRepricingConfig. Format:
accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about how a Reseller modifies their bill before sending it to a Customer.
* Possible Error Codes: * PERMISSION_DENIED: If the account making the request and the account
* being queried are different. * NOT_FOUND: The CustomerRepricingConfig specified does not exist or
* is not associated with the given account. * INTERNAL: Any non-user error related to technical
* issues in the backend. In this case, contact Cloud Channel support. Return Value: If successful,
* the CustomerRepricingConfig resources. The data for each resource is displayed in the ascending
* order of: * Customer ID * RepricingConfig.EntitlementGranularity.entitlement *
* RepricingConfig.effective_invoice_month * CustomerRepricingConfig.update_time If unsuccessful,
* returns an error.
*
* Create a request for the method "customerRepricingConfigs.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the customer. Parent uses the format:
* accounts/{account_id}/customers/{customer_id}. Supports accounts/{account_id}/customers/-
* to retrieve configs for all customers.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/customerRepricingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Lists information about how a Reseller modifies their bill before sending it to a Customer.
* Possible Error Codes: * PERMISSION_DENIED: If the account making the request and the account
* being queried are different. * NOT_FOUND: The CustomerRepricingConfig specified does not exist
* or is not associated with the given account. * INTERNAL: Any non-user error related to
* technical issues in the backend. In this case, contact Cloud Channel support. Return Value: If
* successful, the CustomerRepricingConfig resources. The data for each resource is displayed in
* the ascending order of: * Customer ID * RepricingConfig.EntitlementGranularity.entitlement *
* RepricingConfig.effective_invoice_month * CustomerRepricingConfig.update_time If unsuccessful,
* returns an error.
*
* Create a request for the method "customerRepricingConfigs.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the customer. Parent uses the format:
* accounts/{account_id}/customers/{customer_id}. Supports accounts/{account_id}/customers/-
* to retrieve configs for all customers.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListCustomerRepricingConfigsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the customer. Parent uses the format:
* accounts/{account_id}/customers/{customer_id}. Supports
* accounts/{account_id}/customers/- to retrieve configs for all customers.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the customer. Parent uses the format:
accounts/{account_id}/customers/{customer_id}. Supports accounts/{account_id}/customers/- to
retrieve configs for all customers.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the customer. Parent uses the format:
* accounts/{account_id}/customers/{customer_id}. Supports
* accounts/{account_id}/customers/- to retrieve configs for all customers.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter for [CloudChannelService.ListCustomerRepricingConfigs] results
* (customer only). You can use this filter when you support a BatchGet-like query. To use
* the filter, you must set `parent=accounts/{account_id}/customers/-`. Example: customer
* = accounts/account_id/customers/c1 OR customer = accounts/account_id/customers/c2.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter for [CloudChannelService.ListCustomerRepricingConfigs] results (customer only).
You can use this filter when you support a BatchGet-like query. To use the filter, you must set
`parent=accounts/{account_id}/customers/-`. Example: customer = accounts/account_id/customers/c1 OR
customer = accounts/account_id/customers/c2.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter for [CloudChannelService.ListCustomerRepricingConfigs] results
* (customer only). You can use this filter when you support a BatchGet-like query. To use
* the filter, you must set `parent=accounts/{account_id}/customers/-`. Example: customer
* = accounts/account_id/customers/c1 OR customer = accounts/account_id/customers/c2.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of repricing configs to return. The service may return
* fewer than this value. If unspecified, returns a maximum of 50 rules. The maximum value
* is 100; values above 100 will be coerced to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of repricing configs to return. The service may return fewer than this
value. If unspecified, returns a maximum of 50 rules. The maximum value is 100; values above 100
will be coerced to 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of repricing configs to return. The service may return
* fewer than this value. If unspecified, returns a maximum of 50 rules. The maximum value
* is 100; values above 100 will be coerced to 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListCustomerRepricingConfigsResponse.next_page_token of the previous
* CloudChannelService.ListCustomerRepricingConfigs call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results beyond the first page. Obtained through
ListCustomerRepricingConfigsResponse.next_page_token of the previous
CloudChannelService.ListCustomerRepricingConfigs call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListCustomerRepricingConfigsResponse.next_page_token of the previous
* CloudChannelService.ListCustomerRepricingConfigs call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a CustomerRepricingConfig. Call this method to set modifications for a specific
* customer's bill. This method overwrites the existing CustomerRepricingConfig. You can only update
* configs if the RepricingConfig.effective_invoice_month is a future month. To make changes to
* configs for the current month, use CreateCustomerRepricingConfig, taking note of its
* restrictions. You cannot update the RepricingConfig.effective_invoice_month. When updating a
* config in the future: * This config must already exist. Possible Error Codes: *
* PERMISSION_DENIED: If the account making the request and the account being queried are different.
* * INVALID_ARGUMENT: Missing or invalid required parameters in the request. Also displays if the
* updated config is for the current month or past months. * NOT_FOUND: The CustomerRepricingConfig
* specified does not exist or is not associated with the given account. * INTERNAL: Any non-user
* error related to technical issues in the backend. In this case, contact Cloud Channel support.
* Return Value: If successful, the updated CustomerRepricingConfig resource, otherwise returns an
* error.
*
* Create a request for the method "customerRepricingConfigs.patch".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
/**
* Updates a CustomerRepricingConfig. Call this method to set modifications for a specific
* customer's bill. This method overwrites the existing CustomerRepricingConfig. You can only
* update configs if the RepricingConfig.effective_invoice_month is a future month. To make
* changes to configs for the current month, use CreateCustomerRepricingConfig, taking note of its
* restrictions. You cannot update the RepricingConfig.effective_invoice_month. When updating a
* config in the future: * This config must already exist. Possible Error Codes: *
* PERMISSION_DENIED: If the account making the request and the account being queried are
* different. * INVALID_ARGUMENT: Missing or invalid required parameters in the request. Also
* displays if the updated config is for the current month or past months. * NOT_FOUND: The
* CustomerRepricingConfig specified does not exist or is not associated with the given account. *
* INTERNAL: Any non-user error related to technical issues in the backend. In this case, contact
* Cloud Channel support. Return Value: If successful, the updated CustomerRepricingConfig
* resource, otherwise returns an error.
*
* Create a request for the method "customerRepricingConfigs.patch".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig content) {
super(Cloudchannel.this, "PATCH", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CustomerRepricingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Resource name of the CustomerRepricingConfig. Format:
accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the CustomerRepricingConfig. Format:
* accounts/{account_id}/customers/{customer_id}/customerRepricingConfigs/{id}.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/customerRepricingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Entitlements collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Entitlements.List request = cloudchannel.entitlements().list(parameters ...)}
*
*
* @return the resource collection
*/
public Entitlements entitlements() {
return new Entitlements();
}
/**
* The "entitlements" collection of methods.
*/
public class Entitlements {
/**
* Activates a previously suspended entitlement. Entitlements suspended for pending ToS acceptance
* can't be activated using this method. An entitlement activation is a long-running operation and
* it updates the state of the customer entitlement. Possible error codes: * PERMISSION_DENIED: The
* reseller account making the request is different from the reseller account in the API request. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: Entitlement
* resource not found. * SUSPENSION_NOT_RESELLER_INITIATED: Can only activate reseller-initiated
* suspensions and entitlements that have accepted the TOS. * NOT_SUSPENDED: Can only activate
* suspended entitlements not in an ACTIVE state. * INTERNAL: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. Return value: The ID
* of a long-running operation. To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.activate".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Activate#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the entitlement to activate. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ActivateEntitlementRequest}
* @return the request
*/
public Activate activate(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ActivateEntitlementRequest content) throws java.io.IOException {
Activate result = new Activate(name, content);
initialize(result);
return result;
}
public class Activate extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:activate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Activates a previously suspended entitlement. Entitlements suspended for pending ToS acceptance
* can't be activated using this method. An entitlement activation is a long-running operation and
* it updates the state of the customer entitlement. Possible error codes: * PERMISSION_DENIED:
* The reseller account making the request is different from the reseller account in the API
* request. * INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND:
* Entitlement resource not found. * SUSPENSION_NOT_RESELLER_INITIATED: Can only activate
* reseller-initiated suspensions and entitlements that have accepted the TOS. * NOT_SUSPENDED:
* Can only activate suspended entitlements not in an ACTIVE state. * INTERNAL: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-
* user error related to a technical issue in the backend. Contact Cloud Channel support. Return
* value: The ID of a long-running operation. To get the results of the operation, call the
* GetOperation method of CloudChannelOperationsService. The Operation metadata will contain an
* instance of OperationMetadata.
*
* Create a request for the method "entitlements.activate".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Activate#execute()} method to invoke the remote operation.
* {@link
* Activate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the entitlement to activate. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ActivateEntitlementRequest}
* @since 1.13
*/
protected Activate(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ActivateEntitlementRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public Activate set$Xgafv(java.lang.String $Xgafv) {
return (Activate) super.set$Xgafv($Xgafv);
}
@Override
public Activate setAccessToken(java.lang.String accessToken) {
return (Activate) super.setAccessToken(accessToken);
}
@Override
public Activate setAlt(java.lang.String alt) {
return (Activate) super.setAlt(alt);
}
@Override
public Activate setCallback(java.lang.String callback) {
return (Activate) super.setCallback(callback);
}
@Override
public Activate setFields(java.lang.String fields) {
return (Activate) super.setFields(fields);
}
@Override
public Activate setKey(java.lang.String key) {
return (Activate) super.setKey(key);
}
@Override
public Activate setOauthToken(java.lang.String oauthToken) {
return (Activate) super.setOauthToken(oauthToken);
}
@Override
public Activate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Activate) super.setPrettyPrint(prettyPrint);
}
@Override
public Activate setQuotaUser(java.lang.String quotaUser) {
return (Activate) super.setQuotaUser(quotaUser);
}
@Override
public Activate setUploadType(java.lang.String uploadType) {
return (Activate) super.setUploadType(uploadType);
}
@Override
public Activate setUploadProtocol(java.lang.String uploadProtocol) {
return (Activate) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the entitlement to activate. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the entitlement to activate. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the entitlement to activate. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public Activate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Activate set(String parameterName, Object value) {
return (Activate) super.set(parameterName, value);
}
}
/**
* Cancels a previously fulfilled entitlement. An entitlement cancellation is a long-running
* operation. Possible error codes: * PERMISSION_DENIED: The reseller account making the request is
* different from the reseller account in the API request. * FAILED_PRECONDITION: There are Google
* Cloud projects linked to the Google Cloud entitlement's Cloud Billing subaccount. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: Entitlement
* resource not found. * DELETION_TYPE_NOT_ALLOWED: Cancel is only allowed for Google Workspace add-
* ons, or entitlements for Google Cloud's development platform. * INTERNAL: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-
* user error related to a technical issue in the backend. Contact Cloud Channel support. Return
* value: The ID of a long-running operation. To get the results of the operation, call the
* GetOperation method of CloudChannelOperationsService. The response will contain
* google.protobuf.Empty on success. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.cancel".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the entitlement to cancel. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CancelEntitlementRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CancelEntitlementRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Cancels a previously fulfilled entitlement. An entitlement cancellation is a long-running
* operation. Possible error codes: * PERMISSION_DENIED: The reseller account making the request
* is different from the reseller account in the API request. * FAILED_PRECONDITION: There are
* Google Cloud projects linked to the Google Cloud entitlement's Cloud Billing subaccount. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: Entitlement
* resource not found. * DELETION_TYPE_NOT_ALLOWED: Cancel is only allowed for Google Workspace
* add-ons, or entitlements for Google Cloud's development platform. * INTERNAL: Any non-user
* error related to a technical issue in the backend. Contact Cloud Channel support. * UNKNOWN:
* Any non-user error related to a technical issue in the backend. Contact Cloud Channel support.
* Return value: The ID of a long-running operation. To get the results of the operation, call the
* GetOperation method of CloudChannelOperationsService. The response will contain
* google.protobuf.Empty on success. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.cancel".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the entitlement to cancel. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CancelEntitlementRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CancelEntitlementRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the entitlement to cancel. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the entitlement to cancel. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the entitlement to cancel. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Updates the Offer for an existing customer entitlement. An entitlement update is a long-running
* operation and it updates the entitlement as a result of fulfillment. Possible error codes: *
* PERMISSION_DENIED: The customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. * NOT_FOUND: Offer or Entitlement resource not found.
* * INTERNAL: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. * UNKNOWN: Any non-user error related to a technical issue in the backend. Contact Cloud
* Channel support. Return value: The ID of a long-running operation. To get the results of the
* operation, call the GetOperation method of CloudChannelOperationsService. The Operation metadata
* will contain an instance of OperationMetadata.
*
* Create a request for the method "entitlements.changeOffer".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ChangeOffer#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeOfferRequest}
* @return the request
*/
public ChangeOffer changeOffer(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeOfferRequest content) throws java.io.IOException {
ChangeOffer result = new ChangeOffer(name, content);
initialize(result);
return result;
}
public class ChangeOffer extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:changeOffer";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Updates the Offer for an existing customer entitlement. An entitlement update is a long-running
* operation and it updates the entitlement as a result of fulfillment. Possible error codes: *
* PERMISSION_DENIED: The customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required
* request parameters are missing or invalid. * NOT_FOUND: Offer or Entitlement resource not
* found. * INTERNAL: Any non-user error related to a technical issue in the backend. Contact
* Cloud Channel support. * UNKNOWN: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. Return value: The ID of a long-running operation. To
* get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.changeOffer".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ChangeOffer#execute()} method to invoke the remote
* operation. {@link
* ChangeOffer#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeOfferRequest}
* @since 1.13
*/
protected ChangeOffer(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeOfferRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public ChangeOffer set$Xgafv(java.lang.String $Xgafv) {
return (ChangeOffer) super.set$Xgafv($Xgafv);
}
@Override
public ChangeOffer setAccessToken(java.lang.String accessToken) {
return (ChangeOffer) super.setAccessToken(accessToken);
}
@Override
public ChangeOffer setAlt(java.lang.String alt) {
return (ChangeOffer) super.setAlt(alt);
}
@Override
public ChangeOffer setCallback(java.lang.String callback) {
return (ChangeOffer) super.setCallback(callback);
}
@Override
public ChangeOffer setFields(java.lang.String fields) {
return (ChangeOffer) super.setFields(fields);
}
@Override
public ChangeOffer setKey(java.lang.String key) {
return (ChangeOffer) super.setKey(key);
}
@Override
public ChangeOffer setOauthToken(java.lang.String oauthToken) {
return (ChangeOffer) super.setOauthToken(oauthToken);
}
@Override
public ChangeOffer setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ChangeOffer) super.setPrettyPrint(prettyPrint);
}
@Override
public ChangeOffer setQuotaUser(java.lang.String quotaUser) {
return (ChangeOffer) super.setQuotaUser(quotaUser);
}
@Override
public ChangeOffer setUploadType(java.lang.String uploadType) {
return (ChangeOffer) super.setUploadType(uploadType);
}
@Override
public ChangeOffer setUploadProtocol(java.lang.String uploadProtocol) {
return (ChangeOffer) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the entitlement to update. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public ChangeOffer setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ChangeOffer set(String parameterName, Object value) {
return (ChangeOffer) super.set(parameterName, value);
}
}
/**
* Change parameters of the entitlement. An entitlement update is a long-running operation and it
* updates the entitlement as a result of fulfillment. Possible error codes: * PERMISSION_DENIED:
* The customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters are
* missing or invalid. For example, the number of seats being changed is greater than the allowed
* number of max seats, or decreasing seats for a commitment based plan. * NOT_FOUND: Entitlement
* resource not found. * INTERNAL: Any non-user error related to a technical issue in the backend.
* Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. Return value: The ID of a long-running operation. To get
* the results of the operation, call the GetOperation method of CloudChannelOperationsService. The
* Operation metadata will contain an instance of OperationMetadata.
*
* Create a request for the method "entitlements.changeParameters".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ChangeParameters#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeParametersRequest}
* @return the request
*/
public ChangeParameters changeParameters(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeParametersRequest content) throws java.io.IOException {
ChangeParameters result = new ChangeParameters(name, content);
initialize(result);
return result;
}
public class ChangeParameters extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:changeParameters";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Change parameters of the entitlement. An entitlement update is a long-running operation and it
* updates the entitlement as a result of fulfillment. Possible error codes: * PERMISSION_DENIED:
* The customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters
* are missing or invalid. For example, the number of seats being changed is greater than the
* allowed number of max seats, or decreasing seats for a commitment based plan. * NOT_FOUND:
* Entitlement resource not found. * INTERNAL: Any non-user error related to a technical issue in
* the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. Return value: The ID of a long-
* running operation. To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.changeParameters".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ChangeParameters#execute()} method to invoke the remote
* operation. {@link ChangeParameters#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeParametersRequest}
* @since 1.13
*/
protected ChangeParameters(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeParametersRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public ChangeParameters set$Xgafv(java.lang.String $Xgafv) {
return (ChangeParameters) super.set$Xgafv($Xgafv);
}
@Override
public ChangeParameters setAccessToken(java.lang.String accessToken) {
return (ChangeParameters) super.setAccessToken(accessToken);
}
@Override
public ChangeParameters setAlt(java.lang.String alt) {
return (ChangeParameters) super.setAlt(alt);
}
@Override
public ChangeParameters setCallback(java.lang.String callback) {
return (ChangeParameters) super.setCallback(callback);
}
@Override
public ChangeParameters setFields(java.lang.String fields) {
return (ChangeParameters) super.setFields(fields);
}
@Override
public ChangeParameters setKey(java.lang.String key) {
return (ChangeParameters) super.setKey(key);
}
@Override
public ChangeParameters setOauthToken(java.lang.String oauthToken) {
return (ChangeParameters) super.setOauthToken(oauthToken);
}
@Override
public ChangeParameters setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ChangeParameters) super.setPrettyPrint(prettyPrint);
}
@Override
public ChangeParameters setQuotaUser(java.lang.String quotaUser) {
return (ChangeParameters) super.setQuotaUser(quotaUser);
}
@Override
public ChangeParameters setUploadType(java.lang.String uploadType) {
return (ChangeParameters) super.setUploadType(uploadType);
}
@Override
public ChangeParameters setUploadProtocol(java.lang.String uploadProtocol) {
return (ChangeParameters) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entitlement to update. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public ChangeParameters setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ChangeParameters set(String parameterName, Object value) {
return (ChangeParameters) super.set(parameterName, value);
}
}
/**
* Updates the renewal settings for an existing customer entitlement. An entitlement update is a
* long-running operation and it updates the entitlement as a result of fulfillment. Possible error
* codes: * PERMISSION_DENIED: The customer doesn't belong to the reseller. * INVALID_ARGUMENT:
* Required request parameters are missing or invalid. * NOT_FOUND: Entitlement resource not found.
* * NOT_COMMITMENT_PLAN: Renewal Settings are only applicable for a commitment plan. Can't enable
* or disable renewals for non-commitment plans. * INTERNAL: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. Return value: The ID
* of a long-running operation. To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.changeRenewalSettings".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ChangeRenewalSettings#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeRenewalSettingsRequest}
* @return the request
*/
public ChangeRenewalSettings changeRenewalSettings(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeRenewalSettingsRequest content) throws java.io.IOException {
ChangeRenewalSettings result = new ChangeRenewalSettings(name, content);
initialize(result);
return result;
}
public class ChangeRenewalSettings extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:changeRenewalSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Updates the renewal settings for an existing customer entitlement. An entitlement update is a
* long-running operation and it updates the entitlement as a result of fulfillment. Possible
* error codes: * PERMISSION_DENIED: The customer doesn't belong to the reseller. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: Entitlement
* resource not found. * NOT_COMMITMENT_PLAN: Renewal Settings are only applicable for a
* commitment plan. Can't enable or disable renewals for non-commitment plans. * INTERNAL: Any
* non-user error related to a technical issue in the backend. Contact Cloud Channel support. *
* UNKNOWN: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. Return value: The ID of a long-running operation. To get the results of the operation,
* call the GetOperation method of CloudChannelOperationsService. The Operation metadata will
* contain an instance of OperationMetadata.
*
* Create a request for the method "entitlements.changeRenewalSettings".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ChangeRenewalSettings#execute()} method to invoke the
* remote operation. {@link ChangeRenewalSettings#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeRenewalSettingsRequest}
* @since 1.13
*/
protected ChangeRenewalSettings(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ChangeRenewalSettingsRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public ChangeRenewalSettings set$Xgafv(java.lang.String $Xgafv) {
return (ChangeRenewalSettings) super.set$Xgafv($Xgafv);
}
@Override
public ChangeRenewalSettings setAccessToken(java.lang.String accessToken) {
return (ChangeRenewalSettings) super.setAccessToken(accessToken);
}
@Override
public ChangeRenewalSettings setAlt(java.lang.String alt) {
return (ChangeRenewalSettings) super.setAlt(alt);
}
@Override
public ChangeRenewalSettings setCallback(java.lang.String callback) {
return (ChangeRenewalSettings) super.setCallback(callback);
}
@Override
public ChangeRenewalSettings setFields(java.lang.String fields) {
return (ChangeRenewalSettings) super.setFields(fields);
}
@Override
public ChangeRenewalSettings setKey(java.lang.String key) {
return (ChangeRenewalSettings) super.setKey(key);
}
@Override
public ChangeRenewalSettings setOauthToken(java.lang.String oauthToken) {
return (ChangeRenewalSettings) super.setOauthToken(oauthToken);
}
@Override
public ChangeRenewalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ChangeRenewalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public ChangeRenewalSettings setQuotaUser(java.lang.String quotaUser) {
return (ChangeRenewalSettings) super.setQuotaUser(quotaUser);
}
@Override
public ChangeRenewalSettings setUploadType(java.lang.String uploadType) {
return (ChangeRenewalSettings) super.setUploadType(uploadType);
}
@Override
public ChangeRenewalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (ChangeRenewalSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entitlement to update. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the entitlement to update. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public ChangeRenewalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ChangeRenewalSettings set(String parameterName, Object value) {
return (ChangeRenewalSettings) super.set(parameterName, value);
}
}
/**
* Creates an entitlement for a customer. Possible error codes: * PERMISSION_DENIED: * The customer
* doesn't belong to the reseller. * The reseller is not authorized to transact on this Product. See
* https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: * Required request
* parameters are missing or invalid. * There is already a customer entitlement for a SKU from the
* same product family. * INVALID_VALUE: Make sure the OfferId is valid. If it is, contact Google
* Channel support for further troubleshooting. * NOT_FOUND: The customer or offer resource was not
* found. * ALREADY_EXISTS: * The SKU was already purchased for the customer. * The customer's
* primary email already exists. Retry after changing the customer's primary contact email. *
* CONDITION_NOT_MET or FAILED_PRECONDITION: * The domain required for purchasing a SKU has not been
* verified. * A pre-requisite SKU required to purchase an Add-On SKU is missing. For example,
* Google Workspace Business Starter is required to purchase Vault or Drive. * (Developer accounts
* only) Reseller and resold domain must meet the following naming requirements: * Domain names must
* start with goog-test. * Domain names must include the reseller domain. * INTERNAL: Any non-user
* error related to a technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any
* non-user error related to a technical issue in the backend. Contact Cloud Channel support. Return
* value: The ID of a long-running operation. To get the results of the operation, call the
* GetOperation method of CloudChannelOperationsService. The Operation metadata will contain an
* instance of OperationMetadata.
*
* Create a request for the method "entitlements.create".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller's customer account in which to create the entitlement.
* Parent uses the format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CreateEntitlementRequest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CreateEntitlementRequest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/entitlements";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Creates an entitlement for a customer. Possible error codes: * PERMISSION_DENIED: * The
* customer doesn't belong to the reseller. * The reseller is not authorized to transact on this
* Product. See https://support.google.com/channelservices/answer/9759265 * INVALID_ARGUMENT: *
* Required request parameters are missing or invalid. * There is already a customer entitlement
* for a SKU from the same product family. * INVALID_VALUE: Make sure the OfferId is valid. If it
* is, contact Google Channel support for further troubleshooting. * NOT_FOUND: The customer or
* offer resource was not found. * ALREADY_EXISTS: * The SKU was already purchased for the
* customer. * The customer's primary email already exists. Retry after changing the customer's
* primary contact email. * CONDITION_NOT_MET or FAILED_PRECONDITION: * The domain required for
* purchasing a SKU has not been verified. * A pre-requisite SKU required to purchase an Add-On
* SKU is missing. For example, Google Workspace Business Starter is required to purchase Vault or
* Drive. * (Developer accounts only) Reseller and resold domain must meet the following naming
* requirements: * Domain names must start with goog-test. * Domain names must include the
* reseller domain. * INTERNAL: Any non-user error related to a technical issue in the backend.
* Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical issue in
* the backend. Contact Cloud Channel support. Return value: The ID of a long-running operation.
* To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.create".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the reseller's customer account in which to create the entitlement.
* Parent uses the format: accounts/{account_id}/customers/{customer_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CreateEntitlementRequest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CreateEntitlementRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller's customer account in which to create the
* entitlement. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller's customer account in which to create the entitlement.
Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller's customer account in which to create the
* entitlement. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Returns the requested Entitlement resource. Possible error codes: * PERMISSION_DENIED: The
* customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters are
* missing or invalid. * NOT_FOUND: The customer entitlement was not found. Return value: The
* requested Entitlement resource.
*
* Create a request for the method "entitlements.get".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the entitlement to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Returns the requested Entitlement resource. Possible error codes: * PERMISSION_DENIED: The
* customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters are
* missing or invalid. * NOT_FOUND: The customer entitlement was not found. Return value: The
* requested Entitlement resource.
*
* Create a request for the method "entitlements.get".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the entitlement to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Entitlement.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the entitlement to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the entitlement to retrieve. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the entitlement to retrieve. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Entitlements belonging to a customer. Possible error codes: * PERMISSION_DENIED: The
* customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters are
* missing or invalid. Return value: A list of the customer's Entitlements.
*
* Create a request for the method "entitlements.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller's customer account to list entitlements for. Parent uses
* the format: accounts/{account_id}/customers/{customer_id}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/entitlements";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+$");
/**
* Lists Entitlements belonging to a customer. Possible error codes: * PERMISSION_DENIED: The
* customer doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters are
* missing or invalid. Return value: A list of the customer's Entitlements.
*
* Create a request for the method "entitlements.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the reseller's customer account to list entitlements for. Parent uses
* the format: accounts/{account_id}/customers/{customer_id}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListEntitlementsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller's customer account to list entitlements
* for. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller's customer account to list entitlements for. Parent
uses the format: accounts/{account_id}/customers/{customer_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller's customer account to list entitlements
* for. Parent uses the format: accounts/{account_id}/customers/{customer_id}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, return at most 50 entitlements. The maximum value is 100; the server will
* coerce values above 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server might return fewer results than requested. If unspecified,
return at most 50 entitlements. The maximum value is 100; the server will coerce values above 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, return at most 50 entitlements. The maximum value is 100; the server will
* coerce values above 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token for a page of results other than the first page. Obtained using
* ListEntitlementsResponse.next_page_token of the previous
* CloudChannelService.ListEntitlements call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token for a page of results other than the first page. Obtained using
ListEntitlementsResponse.next_page_token of the previous CloudChannelService.ListEntitlements call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token for a page of results other than the first page. Obtained using
* ListEntitlementsResponse.next_page_token of the previous
* CloudChannelService.ListEntitlements call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* List entitlement history. Possible error codes: * PERMISSION_DENIED: The reseller account making
* the request and the provided reseller account are different. * INVALID_ARGUMENT: Missing or
* invalid required fields in the request. * NOT_FOUND: The parent resource doesn't exist. Usually
* the result of an invalid name parameter. * INTERNAL: Any non-user error related to a technical
* issue in the backend. In this case, contact CloudChannel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. In this case, contact Cloud Channel support. Return
* value: List of EntitlementChanges.
*
* Create a request for the method "entitlements.listEntitlementChanges".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ListEntitlementChanges#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The resource name of the entitlement for which to list entitlement changes. The `-`
* wildcard may be used to match entitlements across a customer. Formats: *
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id} *
* accounts/{account_id}/customers/{customer_id}/entitlements/-
* @return the request
*/
public ListEntitlementChanges listEntitlementChanges(java.lang.String parent) throws java.io.IOException {
ListEntitlementChanges result = new ListEntitlementChanges(parent);
initialize(result);
return result;
}
public class ListEntitlementChanges extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}:listEntitlementChanges";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* List entitlement history. Possible error codes: * PERMISSION_DENIED: The reseller account
* making the request and the provided reseller account are different. * INVALID_ARGUMENT: Missing
* or invalid required fields in the request. * NOT_FOUND: The parent resource doesn't exist.
* Usually the result of an invalid name parameter. * INTERNAL: Any non-user error related to a
* technical issue in the backend. In this case, contact CloudChannel support. * UNKNOWN: Any non-
* user error related to a technical issue in the backend. In this case, contact Cloud Channel
* support. Return value: List of EntitlementChanges.
*
* Create a request for the method "entitlements.listEntitlementChanges".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ListEntitlementChanges#execute()} method to invoke the
* remote operation. {@link ListEntitlementChanges#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent Required. The resource name of the entitlement for which to list entitlement changes. The `-`
* wildcard may be used to match entitlements across a customer. Formats: *
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id} *
* accounts/{account_id}/customers/{customer_id}/entitlements/-
* @since 1.13
*/
protected ListEntitlementChanges(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListEntitlementChangesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListEntitlementChanges set$Xgafv(java.lang.String $Xgafv) {
return (ListEntitlementChanges) super.set$Xgafv($Xgafv);
}
@Override
public ListEntitlementChanges setAccessToken(java.lang.String accessToken) {
return (ListEntitlementChanges) super.setAccessToken(accessToken);
}
@Override
public ListEntitlementChanges setAlt(java.lang.String alt) {
return (ListEntitlementChanges) super.setAlt(alt);
}
@Override
public ListEntitlementChanges setCallback(java.lang.String callback) {
return (ListEntitlementChanges) super.setCallback(callback);
}
@Override
public ListEntitlementChanges setFields(java.lang.String fields) {
return (ListEntitlementChanges) super.setFields(fields);
}
@Override
public ListEntitlementChanges setKey(java.lang.String key) {
return (ListEntitlementChanges) super.setKey(key);
}
@Override
public ListEntitlementChanges setOauthToken(java.lang.String oauthToken) {
return (ListEntitlementChanges) super.setOauthToken(oauthToken);
}
@Override
public ListEntitlementChanges setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListEntitlementChanges) super.setPrettyPrint(prettyPrint);
}
@Override
public ListEntitlementChanges setQuotaUser(java.lang.String quotaUser) {
return (ListEntitlementChanges) super.setQuotaUser(quotaUser);
}
@Override
public ListEntitlementChanges setUploadType(java.lang.String uploadType) {
return (ListEntitlementChanges) super.setUploadType(uploadType);
}
@Override
public ListEntitlementChanges setUploadProtocol(java.lang.String uploadProtocol) {
return (ListEntitlementChanges) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the entitlement for which to list entitlement changes.
* The `-` wildcard may be used to match entitlements across a customer. Formats: *
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id} *
* accounts/{account_id}/customers/{customer_id}/entitlements/-
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the entitlement for which to list entitlement changes. The `-`
wildcard may be used to match entitlements across a customer. Formats: *
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id} *
accounts/{account_id}/customers/{customer_id}/entitlements/-
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the entitlement for which to list entitlement changes.
* The `-` wildcard may be used to match entitlements across a customer. Formats: *
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id} *
* accounts/{account_id}/customers/{customer_id}/entitlements/-
*/
public ListEntitlementChanges setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filters applied to the list results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filters applied to the list results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filters applied to the list results. */
public ListEntitlementChanges setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of entitlement changes to return. The service may return
* fewer than this value. If unspecified, returns at most 10 entitlement changes. The
* maximum value is 50; the server will coerce values above 50.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of entitlement changes to return. The service may return fewer than
this value. If unspecified, returns at most 10 entitlement changes. The maximum value is 50; the
server will coerce values above 50.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of entitlement changes to return. The service may return
* fewer than this value. If unspecified, returns at most 10 entitlement changes. The
* maximum value is 50; the server will coerce values above 50.
*/
public ListEntitlementChanges setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous
* CloudChannelService.ListEntitlementChanges call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to
* CloudChannelService.ListEntitlementChanges must match the call that provided the page
* token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous CloudChannelService.ListEntitlementChanges call.
Provide this to retrieve the subsequent page. When paginating, all other parameters provided to
CloudChannelService.ListEntitlementChanges must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous
* CloudChannelService.ListEntitlementChanges call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to
* CloudChannelService.ListEntitlementChanges must match the call that provided the page
* token.
*/
public ListEntitlementChanges setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListEntitlementChanges set(String parameterName, Object value) {
return (ListEntitlementChanges) super.set(parameterName, value);
}
}
/**
* Returns the requested Offer resource. Possible error codes: * PERMISSION_DENIED: The entitlement
* doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters are missing or
* invalid. * NOT_FOUND: Entitlement or offer was not found. Return value: The Offer resource.
*
* Create a request for the method "entitlements.lookupOffer".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link LookupOffer#execute()} method to invoke the remote operation.
*
* @param entitlement Required. The resource name of the entitlement to retrieve the Offer. Entitlement uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @return the request
*/
public LookupOffer lookupOffer(java.lang.String entitlement) throws java.io.IOException {
LookupOffer result = new LookupOffer(entitlement);
initialize(result);
return result;
}
public class LookupOffer extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+entitlement}:lookupOffer";
private final java.util.regex.Pattern ENTITLEMENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Returns the requested Offer resource. Possible error codes: * PERMISSION_DENIED: The
* entitlement doesn't belong to the reseller. * INVALID_ARGUMENT: Required request parameters are
* missing or invalid. * NOT_FOUND: Entitlement or offer was not found. Return value: The Offer
* resource.
*
* Create a request for the method "entitlements.lookupOffer".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link LookupOffer#execute()} method to invoke the remote
* operation. {@link
* LookupOffer#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param entitlement Required. The resource name of the entitlement to retrieve the Offer. Entitlement uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @since 1.13
*/
protected LookupOffer(java.lang.String entitlement) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1Offer.class);
this.entitlement = com.google.api.client.util.Preconditions.checkNotNull(entitlement, "Required parameter entitlement must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ENTITLEMENT_PATTERN.matcher(entitlement).matches(),
"Parameter entitlement must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public LookupOffer set$Xgafv(java.lang.String $Xgafv) {
return (LookupOffer) super.set$Xgafv($Xgafv);
}
@Override
public LookupOffer setAccessToken(java.lang.String accessToken) {
return (LookupOffer) super.setAccessToken(accessToken);
}
@Override
public LookupOffer setAlt(java.lang.String alt) {
return (LookupOffer) super.setAlt(alt);
}
@Override
public LookupOffer setCallback(java.lang.String callback) {
return (LookupOffer) super.setCallback(callback);
}
@Override
public LookupOffer setFields(java.lang.String fields) {
return (LookupOffer) super.setFields(fields);
}
@Override
public LookupOffer setKey(java.lang.String key) {
return (LookupOffer) super.setKey(key);
}
@Override
public LookupOffer setOauthToken(java.lang.String oauthToken) {
return (LookupOffer) super.setOauthToken(oauthToken);
}
@Override
public LookupOffer setPrettyPrint(java.lang.Boolean prettyPrint) {
return (LookupOffer) super.setPrettyPrint(prettyPrint);
}
@Override
public LookupOffer setQuotaUser(java.lang.String quotaUser) {
return (LookupOffer) super.setQuotaUser(quotaUser);
}
@Override
public LookupOffer setUploadType(java.lang.String uploadType) {
return (LookupOffer) super.setUploadType(uploadType);
}
@Override
public LookupOffer setUploadProtocol(java.lang.String uploadProtocol) {
return (LookupOffer) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the entitlement to retrieve the Offer. Entitlement uses
* the format: accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String entitlement;
/** Required. The resource name of the entitlement to retrieve the Offer. Entitlement uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getEntitlement() {
return entitlement;
}
/**
* Required. The resource name of the entitlement to retrieve the Offer. Entitlement uses
* the format: accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public LookupOffer setEntitlement(java.lang.String entitlement) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ENTITLEMENT_PATTERN.matcher(entitlement).matches(),
"Parameter entitlement must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.entitlement = entitlement;
return this;
}
@Override
public LookupOffer set(String parameterName, Object value) {
return (LookupOffer) super.set(parameterName, value);
}
}
/**
* Starts paid service for a trial entitlement. Starts paid service for a trial entitlement
* immediately. This method is only applicable if a plan is set up for a trial entitlement but has
* some trial days remaining. Possible error codes: * PERMISSION_DENIED: The customer doesn't belong
* to the reseller. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* NOT_FOUND: Entitlement resource not found. * FAILED_PRECONDITION/NOT_IN_TRIAL: This method only
* works for entitlement on trial plans. * INTERNAL: Any non-user error related to a technical issue
* in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. Return value: The ID of a long-
* running operation. To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.startPaidService".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link StartPaidService#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entitlement to start a paid service for. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1StartPaidServiceRequest}
* @return the request
*/
public StartPaidService startPaidService(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1StartPaidServiceRequest content) throws java.io.IOException {
StartPaidService result = new StartPaidService(name, content);
initialize(result);
return result;
}
public class StartPaidService extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:startPaidService";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Starts paid service for a trial entitlement. Starts paid service for a trial entitlement
* immediately. This method is only applicable if a plan is set up for a trial entitlement but has
* some trial days remaining. Possible error codes: * PERMISSION_DENIED: The customer doesn't
* belong to the reseller. * INVALID_ARGUMENT: Required request parameters are missing or invalid.
* * NOT_FOUND: Entitlement resource not found. * FAILED_PRECONDITION/NOT_IN_TRIAL: This method
* only works for entitlement on trial plans. * INTERNAL: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. Return value: The
* ID of a long-running operation. To get the results of the operation, call the GetOperation
* method of CloudChannelOperationsService. The Operation metadata will contain an instance of
* OperationMetadata.
*
* Create a request for the method "entitlements.startPaidService".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link StartPaidService#execute()} method to invoke the remote
* operation. {@link StartPaidService#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The name of the entitlement to start a paid service for. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1StartPaidServiceRequest}
* @since 1.13
*/
protected StartPaidService(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1StartPaidServiceRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public StartPaidService set$Xgafv(java.lang.String $Xgafv) {
return (StartPaidService) super.set$Xgafv($Xgafv);
}
@Override
public StartPaidService setAccessToken(java.lang.String accessToken) {
return (StartPaidService) super.setAccessToken(accessToken);
}
@Override
public StartPaidService setAlt(java.lang.String alt) {
return (StartPaidService) super.setAlt(alt);
}
@Override
public StartPaidService setCallback(java.lang.String callback) {
return (StartPaidService) super.setCallback(callback);
}
@Override
public StartPaidService setFields(java.lang.String fields) {
return (StartPaidService) super.setFields(fields);
}
@Override
public StartPaidService setKey(java.lang.String key) {
return (StartPaidService) super.setKey(key);
}
@Override
public StartPaidService setOauthToken(java.lang.String oauthToken) {
return (StartPaidService) super.setOauthToken(oauthToken);
}
@Override
public StartPaidService setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StartPaidService) super.setPrettyPrint(prettyPrint);
}
@Override
public StartPaidService setQuotaUser(java.lang.String quotaUser) {
return (StartPaidService) super.setQuotaUser(quotaUser);
}
@Override
public StartPaidService setUploadType(java.lang.String uploadType) {
return (StartPaidService) super.setUploadType(uploadType);
}
@Override
public StartPaidService setUploadProtocol(java.lang.String uploadProtocol) {
return (StartPaidService) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the entitlement to start a paid service for. Name uses the
* format: accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entitlement to start a paid service for. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the entitlement to start a paid service for. Name uses the
* format: accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public StartPaidService setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public StartPaidService set(String parameterName, Object value) {
return (StartPaidService) super.set(parameterName, value);
}
}
/**
* Suspends a previously fulfilled entitlement. An entitlement suspension is a long-running
* operation. Possible error codes: * PERMISSION_DENIED: The customer doesn't belong to the
* reseller. * INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND:
* Entitlement resource not found. * NOT_ACTIVE: Entitlement is not active. * INTERNAL: Any non-user
* error related to a technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any
* non-user error related to a technical issue in the backend. Contact Cloud Channel support. Return
* value: The ID of a long-running operation. To get the results of the operation, call the
* GetOperation method of CloudChannelOperationsService. The Operation metadata will contain an
* instance of OperationMetadata.
*
* Create a request for the method "entitlements.suspend".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Suspend#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the entitlement to suspend. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1SuspendEntitlementRequest}
* @return the request
*/
public Suspend suspend(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1SuspendEntitlementRequest content) throws java.io.IOException {
Suspend result = new Suspend(name, content);
initialize(result);
return result;
}
public class Suspend extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:suspend";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
/**
* Suspends a previously fulfilled entitlement. An entitlement suspension is a long-running
* operation. Possible error codes: * PERMISSION_DENIED: The customer doesn't belong to the
* reseller. * INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND:
* Entitlement resource not found. * NOT_ACTIVE: Entitlement is not active. * INTERNAL: Any non-
* user error related to a technical issue in the backend. Contact Cloud Channel support. *
* UNKNOWN: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. Return value: The ID of a long-running operation. To get the results of the operation,
* call the GetOperation method of CloudChannelOperationsService. The Operation metadata will
* contain an instance of OperationMetadata.
*
* Create a request for the method "entitlements.suspend".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Suspend#execute()} method to invoke the remote operation.
* {@link
* Suspend#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the entitlement to suspend. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1SuspendEntitlementRequest}
* @since 1.13
*/
protected Suspend(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1SuspendEntitlementRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
}
@Override
public Suspend set$Xgafv(java.lang.String $Xgafv) {
return (Suspend) super.set$Xgafv($Xgafv);
}
@Override
public Suspend setAccessToken(java.lang.String accessToken) {
return (Suspend) super.setAccessToken(accessToken);
}
@Override
public Suspend setAlt(java.lang.String alt) {
return (Suspend) super.setAlt(alt);
}
@Override
public Suspend setCallback(java.lang.String callback) {
return (Suspend) super.setCallback(callback);
}
@Override
public Suspend setFields(java.lang.String fields) {
return (Suspend) super.setFields(fields);
}
@Override
public Suspend setKey(java.lang.String key) {
return (Suspend) super.setKey(key);
}
@Override
public Suspend setOauthToken(java.lang.String oauthToken) {
return (Suspend) super.setOauthToken(oauthToken);
}
@Override
public Suspend setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Suspend) super.setPrettyPrint(prettyPrint);
}
@Override
public Suspend setQuotaUser(java.lang.String quotaUser) {
return (Suspend) super.setQuotaUser(quotaUser);
}
@Override
public Suspend setUploadType(java.lang.String uploadType) {
return (Suspend) super.setUploadType(uploadType);
}
@Override
public Suspend setUploadProtocol(java.lang.String uploadProtocol) {
return (Suspend) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the entitlement to suspend. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the entitlement to suspend. Name uses the format:
accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the entitlement to suspend. Name uses the format:
* accounts/{account_id}/customers/{customer_id}/entitlements/{entitlement_id}
*/
public Suspend setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/customers/[^/]+/entitlements/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Suspend set(String parameterName, Object value) {
return (Suspend) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Offers collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Offers.List request = cloudchannel.offers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Offers offers() {
return new Offers();
}
/**
* The "offers" collection of methods.
*/
public class Offers {
/**
* Lists the Offers the reseller can sell. Possible error codes: * INVALID_ARGUMENT: Required
* request parameters are missing or invalid.
*
* Create a request for the method "offers.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the reseller account from which to list Offers. Parent uses the
* format: accounts/{account_id}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/offers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists the Offers the reseller can sell. Possible error codes: * INVALID_ARGUMENT: Required
* request parameters are missing or invalid.
*
* Create a request for the method "offers.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the reseller account from which to list Offers. Parent uses the
* format: accounts/{account_id}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListOffersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the reseller account from which to list Offers. Parent
* uses the format: accounts/{account_id}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the reseller account from which to list Offers. Parent uses the
format: accounts/{account_id}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the reseller account from which to list Offers. Parent
* uses the format: accounts/{account_id}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The expression to filter results by name (name of the Offer), sku.name (name of
* the SKU), or sku.product.name (name of the Product). Example 1:
* sku.product.name=products/p1 AND sku.name!=products/p1/skus/s1 Example 2:
* name=accounts/a1/offers/o1
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. The expression to filter results by name (name of the Offer), sku.name (name of the SKU),
or sku.product.name (name of the Product). Example 1: sku.product.name=products/p1 AND
sku.name!=products/p1/skus/s1 Example 2: name=accounts/a1/offers/o1
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. The expression to filter results by name (name of the Offer), sku.name (name of
* the SKU), or sku.product.name (name of the Product). Example 1:
* sku.product.name=products/p1 AND sku.name!=products/p1/skus/s1 Example 2:
* name=accounts/a1/offers/o1
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** Optional. The BCP-47 language code. For example, "en-US". The response will localize in the
corresponding language code, if specified. The default value is "en-US".
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 500 Offers. The maximum value is 1000; the server will
* coerce values above 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server might return fewer results than requested. If unspecified,
returns at most 500 Offers. The maximum value is 1000; the server will coerce values above 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 500 Offers. The maximum value is 1000; the server will
* coerce values above 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token for a page of results other than the first page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token for a page of results other than the first page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token for a page of results other than the first page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. A boolean flag that determines if a response returns future offers 30 days from
* now. If the show_future_offers is true, the response will only contain offers that are
* scheduled to be available 30 days from now.
*/
@com.google.api.client.util.Key
private java.lang.Boolean showFutureOffers;
/** Optional. A boolean flag that determines if a response returns future offers 30 days from now. If
the show_future_offers is true, the response will only contain offers that are scheduled to be
available 30 days from now.
*/
public java.lang.Boolean getShowFutureOffers() {
return showFutureOffers;
}
/**
* Optional. A boolean flag that determines if a response returns future offers 30 days from
* now. If the show_future_offers is true, the response will only contain offers that are
* scheduled to be available 30 days from now.
*/
public List setShowFutureOffers(java.lang.Boolean showFutureOffers) {
this.showFutureOffers = showFutureOffers;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ReportJobs collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.ReportJobs.List request = cloudchannel.reportJobs().list(parameters ...)}
*
*
* @return the resource collection
*/
public ReportJobs reportJobs() {
return new ReportJobs();
}
/**
* The "reportJobs" collection of methods.
*/
public class ReportJobs {
/**
* Retrieves data generated by CloudChannelReportsService.RunReportJob. Deprecated: Please use
* [Export Channel Services data to
* BigQuery](https://cloud.google.com/channel/docs/rebilling/export-data-to-bigquery) instead.
*
* Create a request for the method "reportJobs.fetchReportResults".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link FetchReportResults#execute()} method to invoke the remote operation.
*
* @param reportJob Required. The report job created by CloudChannelReportsService.RunReportJob. Report_job uses the
* format: accounts/{account_id}/reportJobs/{report_job_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1FetchReportResultsRequest}
* @return the request
*/
public FetchReportResults fetchReportResults(java.lang.String reportJob, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1FetchReportResultsRequest content) throws java.io.IOException {
FetchReportResults result = new FetchReportResults(reportJob, content);
initialize(result);
return result;
}
public class FetchReportResults extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+reportJob}:fetchReportResults";
private final java.util.regex.Pattern REPORT_JOB_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/reportJobs/[^/]+$");
/**
* Retrieves data generated by CloudChannelReportsService.RunReportJob. Deprecated: Please use
* [Export Channel Services data to
* BigQuery](https://cloud.google.com/channel/docs/rebilling/export-data-to-bigquery) instead.
*
* Create a request for the method "reportJobs.fetchReportResults".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link FetchReportResults#execute()} method to invoke the remote
* operation. {@link FetchReportResults#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param reportJob Required. The report job created by CloudChannelReportsService.RunReportJob. Report_job uses the
* format: accounts/{account_id}/reportJobs/{report_job_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1FetchReportResultsRequest}
* @since 1.13
*/
protected FetchReportResults(java.lang.String reportJob, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1FetchReportResultsRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1FetchReportResultsResponse.class);
this.reportJob = com.google.api.client.util.Preconditions.checkNotNull(reportJob, "Required parameter reportJob must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(REPORT_JOB_PATTERN.matcher(reportJob).matches(),
"Parameter reportJob must conform to the pattern " +
"^accounts/[^/]+/reportJobs/[^/]+$");
}
}
@Override
public FetchReportResults set$Xgafv(java.lang.String $Xgafv) {
return (FetchReportResults) super.set$Xgafv($Xgafv);
}
@Override
public FetchReportResults setAccessToken(java.lang.String accessToken) {
return (FetchReportResults) super.setAccessToken(accessToken);
}
@Override
public FetchReportResults setAlt(java.lang.String alt) {
return (FetchReportResults) super.setAlt(alt);
}
@Override
public FetchReportResults setCallback(java.lang.String callback) {
return (FetchReportResults) super.setCallback(callback);
}
@Override
public FetchReportResults setFields(java.lang.String fields) {
return (FetchReportResults) super.setFields(fields);
}
@Override
public FetchReportResults setKey(java.lang.String key) {
return (FetchReportResults) super.setKey(key);
}
@Override
public FetchReportResults setOauthToken(java.lang.String oauthToken) {
return (FetchReportResults) super.setOauthToken(oauthToken);
}
@Override
public FetchReportResults setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FetchReportResults) super.setPrettyPrint(prettyPrint);
}
@Override
public FetchReportResults setQuotaUser(java.lang.String quotaUser) {
return (FetchReportResults) super.setQuotaUser(quotaUser);
}
@Override
public FetchReportResults setUploadType(java.lang.String uploadType) {
return (FetchReportResults) super.setUploadType(uploadType);
}
@Override
public FetchReportResults setUploadProtocol(java.lang.String uploadProtocol) {
return (FetchReportResults) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The report job created by CloudChannelReportsService.RunReportJob. Report_job
* uses the format: accounts/{account_id}/reportJobs/{report_job_id}
*/
@com.google.api.client.util.Key
private java.lang.String reportJob;
/** Required. The report job created by CloudChannelReportsService.RunReportJob. Report_job uses the
format: accounts/{account_id}/reportJobs/{report_job_id}
*/
public java.lang.String getReportJob() {
return reportJob;
}
/**
* Required. The report job created by CloudChannelReportsService.RunReportJob. Report_job
* uses the format: accounts/{account_id}/reportJobs/{report_job_id}
*/
public FetchReportResults setReportJob(java.lang.String reportJob) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(REPORT_JOB_PATTERN.matcher(reportJob).matches(),
"Parameter reportJob must conform to the pattern " +
"^accounts/[^/]+/reportJobs/[^/]+$");
}
this.reportJob = reportJob;
return this;
}
@Override
public FetchReportResults set(String parameterName, Object value) {
return (FetchReportResults) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Reports collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Reports.List request = cloudchannel.reports().list(parameters ...)}
*
*
* @return the resource collection
*/
public Reports reports() {
return new Reports();
}
/**
* The "reports" collection of methods.
*/
public class Reports {
/**
* Lists the reports that RunReportJob can run. These reports include an ID, a description, and the
* list of columns that will be in the result. Deprecated: Please use [Export Channel Services data
* to BigQuery](https://cloud.google.com/channel/docs/rebilling/export-data-to-bigquery) instead.
*
* Create a request for the method "reports.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the partner account to list available reports for. Parent uses the
* format: accounts/{account_id}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/reports";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists the reports that RunReportJob can run. These reports include an ID, a description, and
* the list of columns that will be in the result. Deprecated: Please use [Export Channel Services
* data to BigQuery](https://cloud.google.com/channel/docs/rebilling/export-data-to-bigquery)
* instead.
*
* Create a request for the method "reports.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the partner account to list available reports for. Parent uses the
* format: accounts/{account_id}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListReportsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the partner account to list available reports for. Parent
* uses the format: accounts/{account_id}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the partner account to list available reports for. Parent uses the
format: accounts/{account_id}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the partner account to list available reports for. Parent
* uses the format: accounts/{account_id}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The BCP-47 language code, such as "en-US". If specified, the response is
* localized to the corresponding language code if the original data sources support it.
* Default is "en-US".
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** Optional. The BCP-47 language code, such as "en-US". If specified, the response is localized to the
corresponding language code if the original data sources support it. Default is "en-US".
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Optional. The BCP-47 language code, such as "en-US". If specified, the response is
* localized to the corresponding language code if the original data sources support it.
* Default is "en-US".
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Optional. Requested page size of the report. The server might return fewer results than
* requested. If unspecified, returns 20 reports. The maximum value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size of the report. The server might return fewer results than requested.
If unspecified, returns 20 reports. The maximum value is 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size of the report. The server might return fewer results than
* requested. If unspecified, returns 20 reports. The maximum value is 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token that specifies a page of results beyond the first page. Obtained
* through ListReportsResponse.next_page_token of the previous
* CloudChannelReportsService.ListReports call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token that specifies a page of results beyond the first page. Obtained through
ListReportsResponse.next_page_token of the previous CloudChannelReportsService.ListReports call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token that specifies a page of results beyond the first page. Obtained
* through ListReportsResponse.next_page_token of the previous
* CloudChannelReportsService.ListReports call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Begins generation of data for a given report. The report identifier is a UID (for example,
* `613bf59q`). Possible error codes: * PERMISSION_DENIED: The user doesn't have access to this
* report. * INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The
* report identifier was not found. * INTERNAL: Any non-user error related to a technical issue in
* the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The ID of a long-running
* operation. To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata contains an instance of OperationMetadata.
* To get the results of report generation, call CloudChannelReportsService.FetchReportResults with
* the RunReportJobResponse.report_job. Deprecated: Please use [Export Channel Services data to
* BigQuery](https://cloud.google.com/channel/docs/rebilling/export-data-to-bigquery) instead.
*
* Create a request for the method "reports.run".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Run#execute()} method to invoke the remote operation.
*
* @param name Required. The report's resource name. Specifies the account and report used to generate report data.
* The report_id identifier is a UID (for example, `613bf59q`). Name uses the format:
* accounts/{account_id}/reports/{report_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RunReportJobRequest}
* @return the request
*/
public Run run(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RunReportJobRequest content) throws java.io.IOException {
Run result = new Run(name, content);
initialize(result);
return result;
}
public class Run extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:run";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/reports/[^/]+$");
/**
* Begins generation of data for a given report. The report identifier is a UID (for example,
* `613bf59q`). Possible error codes: * PERMISSION_DENIED: The user doesn't have access to this
* report. * INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND:
* The report identifier was not found. * INTERNAL: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. Return value: The ID of a long-
* running operation. To get the results of the operation, call the GetOperation method of
* CloudChannelOperationsService. The Operation metadata contains an instance of
* OperationMetadata. To get the results of report generation, call
* CloudChannelReportsService.FetchReportResults with the RunReportJobResponse.report_job.
* Deprecated: Please use [Export Channel Services data to
* BigQuery](https://cloud.google.com/channel/docs/rebilling/export-data-to-bigquery) instead.
*
* Create a request for the method "reports.run".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Run#execute()} method to invoke the remote operation.
* {@link Run#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The report's resource name. Specifies the account and report used to generate report data.
* The report_id identifier is a UID (for example, `613bf59q`). Name uses the format:
* accounts/{account_id}/reports/{report_id}
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RunReportJobRequest}
* @since 1.13
*/
protected Run(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RunReportJobRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
}
@Override
public Run set$Xgafv(java.lang.String $Xgafv) {
return (Run) super.set$Xgafv($Xgafv);
}
@Override
public Run setAccessToken(java.lang.String accessToken) {
return (Run) super.setAccessToken(accessToken);
}
@Override
public Run setAlt(java.lang.String alt) {
return (Run) super.setAlt(alt);
}
@Override
public Run setCallback(java.lang.String callback) {
return (Run) super.setCallback(callback);
}
@Override
public Run setFields(java.lang.String fields) {
return (Run) super.setFields(fields);
}
@Override
public Run setKey(java.lang.String key) {
return (Run) super.setKey(key);
}
@Override
public Run setOauthToken(java.lang.String oauthToken) {
return (Run) super.setOauthToken(oauthToken);
}
@Override
public Run setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Run) super.setPrettyPrint(prettyPrint);
}
@Override
public Run setQuotaUser(java.lang.String quotaUser) {
return (Run) super.setQuotaUser(quotaUser);
}
@Override
public Run setUploadType(java.lang.String uploadType) {
return (Run) super.setUploadType(uploadType);
}
@Override
public Run setUploadProtocol(java.lang.String uploadProtocol) {
return (Run) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The report's resource name. Specifies the account and report used to generate
* report data. The report_id identifier is a UID (for example, `613bf59q`). Name uses the
* format: accounts/{account_id}/reports/{report_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The report's resource name. Specifies the account and report used to generate report
data. The report_id identifier is a UID (for example, `613bf59q`). Name uses the format:
accounts/{account_id}/reports/{report_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The report's resource name. Specifies the account and report used to generate
* report data. The report_id identifier is a UID (for example, `613bf59q`). Name uses the
* format: accounts/{account_id}/reports/{report_id}
*/
public Run setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Run set(String parameterName, Object value) {
return (Run) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SkuGroups collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.SkuGroups.List request = cloudchannel.skuGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public SkuGroups skuGroups() {
return new SkuGroups();
}
/**
* The "skuGroups" collection of methods.
*/
public class SkuGroups {
/**
* Lists the Rebilling supported SKU groups the account is authorized to sell. Reference:
* https://cloud.google.com/skus/sku-groups Possible Error Codes: * PERMISSION_DENIED: If the
* account making the request and the account being queried are different, or the account doesn't
* exist. * INTERNAL: Any non-user error related to technical issues in the backend. In this case,
* contact Cloud Channel support. Return Value: If successful, the SkuGroup resources. The data for
* each resource is displayed in the alphabetical order of SKU group display name. The data for each
* resource is displayed in the ascending order of SkuGroup.display_name If unsuccessful, returns an
* error.
*
* Create a request for the method "skuGroups.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the account from which to list SKU groups. Parent uses the format:
* accounts/{account}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/skuGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists the Rebilling supported SKU groups the account is authorized to sell. Reference:
* https://cloud.google.com/skus/sku-groups Possible Error Codes: * PERMISSION_DENIED: If the
* account making the request and the account being queried are different, or the account doesn't
* exist. * INTERNAL: Any non-user error related to technical issues in the backend. In this case,
* contact Cloud Channel support. Return Value: If successful, the SkuGroup resources. The data
* for each resource is displayed in the alphabetical order of SKU group display name. The data
* for each resource is displayed in the ascending order of SkuGroup.display_name If unsuccessful,
* returns an error.
*
* Create a request for the method "skuGroups.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the account from which to list SKU groups. Parent uses the format:
* accounts/{account}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListSkuGroupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the account from which to list SKU groups. Parent uses the
* format: accounts/{account}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the account from which to list SKU groups. Parent uses the format:
accounts/{account}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the account from which to list SKU groups. Parent uses the
* format: accounts/{account}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of SKU groups to return. The service may return fewer than
* this value. If unspecified, returns a maximum of 1000 SKU groups. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of SKU groups to return. The service may return fewer than this value.
If unspecified, returns a maximum of 1000 SKU groups. The maximum value is 1000; values above 1000
will be coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of SKU groups to return. The service may return fewer than
* this value. If unspecified, returns a maximum of 1000 SKU groups. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListSkuGroupsResponse.next_page_token of the previous CloudChannelService.ListSkuGroups
* call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results beyond the first page. Obtained through
ListSkuGroupsResponse.next_page_token of the previous CloudChannelService.ListSkuGroups call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListSkuGroupsResponse.next_page_token of the previous CloudChannelService.ListSkuGroups
* call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the BillableSkus collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.BillableSkus.List request = cloudchannel.billableSkus().list(parameters ...)}
*
*
* @return the resource collection
*/
public BillableSkus billableSkus() {
return new BillableSkus();
}
/**
* The "billableSkus" collection of methods.
*/
public class BillableSkus {
/**
* Lists the Billable SKUs in a given SKU group. Possible error codes: PERMISSION_DENIED: If the
* account making the request and the account being queried for are different, or the account
* doesn't exist. INVALID_ARGUMENT: Missing or invalid required parameters in the request. INTERNAL:
* Any non-user error related to technical issue in the backend. In this case, contact cloud channel
* support. Return Value: If successful, the BillableSku resources. The data for each resource is
* displayed in the ascending order of: * BillableSku.service_display_name *
* BillableSku.sku_display_name If unsuccessful, returns an error.
*
* Create a request for the method "billableSkus.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the SKU group. Format: accounts/{account}/skuGroups/{sku_group}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/billableSkus";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/skuGroups/[^/]+$");
/**
* Lists the Billable SKUs in a given SKU group. Possible error codes: PERMISSION_DENIED: If the
* account making the request and the account being queried for are different, or the account
* doesn't exist. INVALID_ARGUMENT: Missing or invalid required parameters in the request.
* INTERNAL: Any non-user error related to technical issue in the backend. In this case, contact
* cloud channel support. Return Value: If successful, the BillableSku resources. The data for
* each resource is displayed in the ascending order of: * BillableSku.service_display_name *
* BillableSku.sku_display_name If unsuccessful, returns an error.
*
* Create a request for the method "billableSkus.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the SKU group. Format: accounts/{account}/skuGroups/{sku_group}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListSkuGroupBillableSkusResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/skuGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the SKU group. Format:
* accounts/{account}/skuGroups/{sku_group}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the SKU group. Format: accounts/{account}/skuGroups/{sku_group}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the SKU group. Format:
* accounts/{account}/skuGroups/{sku_group}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/skuGroups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of SKUs to return. The service may return fewer than this
* value. If unspecified, returns a maximum of 100000 SKUs. The maximum value is 100000;
* values above 100000 will be coerced to 100000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of SKUs to return. The service may return fewer than this value. If
unspecified, returns a maximum of 100000 SKUs. The maximum value is 100000; values above 100000
will be coerced to 100000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of SKUs to return. The service may return fewer than this
* value. If unspecified, returns a maximum of 100000 SKUs. The maximum value is 100000;
* values above 100000 will be coerced to 100000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListSkuGroupBillableSkusResponse.next_page_token of the previous
* CloudChannelService.ListSkuGroupBillableSkus call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results beyond the first page. Obtained through
ListSkuGroupBillableSkusResponse.next_page_token of the previous
CloudChannelService.ListSkuGroupBillableSkus call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A token identifying a page of results beyond the first page. Obtained through
* ListSkuGroupBillableSkusResponse.next_page_token of the previous
* CloudChannelService.ListSkuGroupBillableSkus call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Integrators collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Integrators.List request = cloudchannel.integrators().list(parameters ...)}
*
*
* @return the resource collection
*/
public Integrators integrators() {
return new Integrators();
}
/**
* The "integrators" collection of methods.
*/
public class Integrators {
/**
* Lists service accounts with subscriber privileges on the Pub/Sub topic created for this Channel
* Services account or integrator. Possible error codes: * PERMISSION_DENIED: The reseller account
* making the request and the provided reseller account are different, or the impersonated user is
* not a super admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* NOT_FOUND: The topic resource doesn't exist. * INTERNAL: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. Return value: A list
* of service email addresses.
*
* Create a request for the method "integrators.listSubscribers".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link ListSubscribers#execute()} method to invoke the remote operation.
*
* @param integrator Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
* this field empty/unset.
* @return the request
*/
public ListSubscribers listSubscribers(java.lang.String integrator) throws java.io.IOException {
ListSubscribers result = new ListSubscribers(integrator);
initialize(result);
return result;
}
public class ListSubscribers extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+integrator}:listSubscribers";
private final java.util.regex.Pattern INTEGRATOR_PATTERN =
java.util.regex.Pattern.compile("^integrators/[^/]+$");
/**
* Lists service accounts with subscriber privileges on the Pub/Sub topic created for this Channel
* Services account or integrator. Possible error codes: * PERMISSION_DENIED: The reseller account
* making the request and the provided reseller account are different, or the impersonated user is
* not a super admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* NOT_FOUND: The topic resource doesn't exist. * INTERNAL: Any non-user error related to a
* technical issue in the backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error
* related to a technical issue in the backend. Contact Cloud Channel support. Return value: A
* list of service email addresses.
*
* Create a request for the method "integrators.listSubscribers".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link ListSubscribers#execute()} method to invoke the remote
* operation. {@link ListSubscribers#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param integrator Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
* this field empty/unset.
* @since 1.13
*/
protected ListSubscribers(java.lang.String integrator) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListSubscribersResponse.class);
this.integrator = com.google.api.client.util.Preconditions.checkNotNull(integrator, "Required parameter integrator must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(INTEGRATOR_PATTERN.matcher(integrator).matches(),
"Parameter integrator must conform to the pattern " +
"^integrators/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListSubscribers set$Xgafv(java.lang.String $Xgafv) {
return (ListSubscribers) super.set$Xgafv($Xgafv);
}
@Override
public ListSubscribers setAccessToken(java.lang.String accessToken) {
return (ListSubscribers) super.setAccessToken(accessToken);
}
@Override
public ListSubscribers setAlt(java.lang.String alt) {
return (ListSubscribers) super.setAlt(alt);
}
@Override
public ListSubscribers setCallback(java.lang.String callback) {
return (ListSubscribers) super.setCallback(callback);
}
@Override
public ListSubscribers setFields(java.lang.String fields) {
return (ListSubscribers) super.setFields(fields);
}
@Override
public ListSubscribers setKey(java.lang.String key) {
return (ListSubscribers) super.setKey(key);
}
@Override
public ListSubscribers setOauthToken(java.lang.String oauthToken) {
return (ListSubscribers) super.setOauthToken(oauthToken);
}
@Override
public ListSubscribers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListSubscribers) super.setPrettyPrint(prettyPrint);
}
@Override
public ListSubscribers setQuotaUser(java.lang.String quotaUser) {
return (ListSubscribers) super.setQuotaUser(quotaUser);
}
@Override
public ListSubscribers setUploadType(java.lang.String uploadType) {
return (ListSubscribers) super.setUploadType(uploadType);
}
@Override
public ListSubscribers setUploadProtocol(java.lang.String uploadProtocol) {
return (ListSubscribers) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String integrator;
/** Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getIntegrator() {
return integrator;
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
public ListSubscribers setIntegrator(java.lang.String integrator) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(INTEGRATOR_PATTERN.matcher(integrator).matches(),
"Parameter integrator must conform to the pattern " +
"^integrators/[^/]+$");
}
this.integrator = integrator;
return this;
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String account;
/** Optional. Resource name of the account. Required if integrator is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getAccount() {
return account;
}
/**
* Optional. Resource name of the account. Required if integrator is not provided. Otherwise,
* leave this field empty/unset.
*/
public ListSubscribers setAccount(java.lang.String account) {
this.account = account;
return this;
}
/**
* Optional. The maximum number of service accounts to return. The service may return fewer
* than this value. If unspecified, returns at most 100 service accounts. The maximum value is
* 1000; the server will coerce values above 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of service accounts to return. The service may return fewer than this
value. If unspecified, returns at most 100 service accounts. The maximum value is 1000; the server
will coerce values above 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of service accounts to return. The service may return fewer
* than this value. If unspecified, returns at most 100 service accounts. The maximum value is
* 1000; the server will coerce values above 1000.
*/
public ListSubscribers setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListSubscribers` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSubscribers` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListSubscribers` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListSubscribers` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListSubscribers` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSubscribers` must match the call that provided the page token.
*/
public ListSubscribers setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListSubscribers set(String parameterName, Object value) {
return (ListSubscribers) super.set(parameterName, value);
}
}
/**
* Registers a service account with subscriber privileges on the Pub/Sub topic for this Channel
* Services account or integrator. After you create a subscriber, you get the events through
* SubscriberEvent Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request and the provided reseller account are different, or the impersonated user is not a super
* admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. * INTERNAL: Any
* non-user error related to a technical issue in the backend. Contact Cloud Channel support. *
* UNKNOWN: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. Return value: The topic name with the registered service email address.
*
* Create a request for the method "integrators.registerSubscriber".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link RegisterSubscriber#execute()} method to invoke the remote operation.
*
* @param integrator Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest}
* @return the request
*/
public RegisterSubscriber registerSubscriber(java.lang.String integrator, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest content) throws java.io.IOException {
RegisterSubscriber result = new RegisterSubscriber(integrator, content);
initialize(result);
return result;
}
public class RegisterSubscriber extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+integrator}:registerSubscriber";
private final java.util.regex.Pattern INTEGRATOR_PATTERN =
java.util.regex.Pattern.compile("^integrators/[^/]+$");
/**
* Registers a service account with subscriber privileges on the Pub/Sub topic for this Channel
* Services account or integrator. After you create a subscriber, you get the events through
* SubscriberEvent Possible error codes: * PERMISSION_DENIED: The reseller account making the
* request and the provided reseller account are different, or the impersonated user is not a
* super admin. * INVALID_ARGUMENT: Required request parameters are missing or invalid. *
* INTERNAL: Any non-user error related to a technical issue in the backend. Contact Cloud Channel
* support. * UNKNOWN: Any non-user error related to a technical issue in the backend. Contact
* Cloud Channel support. Return value: The topic name with the registered service email address.
*
* Create a request for the method "integrators.registerSubscriber".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link RegisterSubscriber#execute()} method to invoke the remote
* operation. {@link RegisterSubscriber#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param integrator Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest}
* @since 1.13
*/
protected RegisterSubscriber(java.lang.String integrator, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RegisterSubscriberResponse.class);
this.integrator = com.google.api.client.util.Preconditions.checkNotNull(integrator, "Required parameter integrator must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(INTEGRATOR_PATTERN.matcher(integrator).matches(),
"Parameter integrator must conform to the pattern " +
"^integrators/[^/]+$");
}
}
@Override
public RegisterSubscriber set$Xgafv(java.lang.String $Xgafv) {
return (RegisterSubscriber) super.set$Xgafv($Xgafv);
}
@Override
public RegisterSubscriber setAccessToken(java.lang.String accessToken) {
return (RegisterSubscriber) super.setAccessToken(accessToken);
}
@Override
public RegisterSubscriber setAlt(java.lang.String alt) {
return (RegisterSubscriber) super.setAlt(alt);
}
@Override
public RegisterSubscriber setCallback(java.lang.String callback) {
return (RegisterSubscriber) super.setCallback(callback);
}
@Override
public RegisterSubscriber setFields(java.lang.String fields) {
return (RegisterSubscriber) super.setFields(fields);
}
@Override
public RegisterSubscriber setKey(java.lang.String key) {
return (RegisterSubscriber) super.setKey(key);
}
@Override
public RegisterSubscriber setOauthToken(java.lang.String oauthToken) {
return (RegisterSubscriber) super.setOauthToken(oauthToken);
}
@Override
public RegisterSubscriber setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RegisterSubscriber) super.setPrettyPrint(prettyPrint);
}
@Override
public RegisterSubscriber setQuotaUser(java.lang.String quotaUser) {
return (RegisterSubscriber) super.setQuotaUser(quotaUser);
}
@Override
public RegisterSubscriber setUploadType(java.lang.String uploadType) {
return (RegisterSubscriber) super.setUploadType(uploadType);
}
@Override
public RegisterSubscriber setUploadProtocol(java.lang.String uploadProtocol) {
return (RegisterSubscriber) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String integrator;
/** Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getIntegrator() {
return integrator;
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
public RegisterSubscriber setIntegrator(java.lang.String integrator) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(INTEGRATOR_PATTERN.matcher(integrator).matches(),
"Parameter integrator must conform to the pattern " +
"^integrators/[^/]+$");
}
this.integrator = integrator;
return this;
}
@Override
public RegisterSubscriber set(String parameterName, Object value) {
return (RegisterSubscriber) super.set(parameterName, value);
}
}
/**
* Unregisters a service account with subscriber privileges on the Pub/Sub topic created for this
* Channel Services account or integrator. If there are no service accounts left with subscriber
* privileges, this deletes the topic. You can call ListSubscribers to check for these accounts.
* Possible error codes: * PERMISSION_DENIED: The reseller account making the request and the
* provided reseller account are different, or the impersonated user is not a super admin. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The topic
* resource doesn't exist. * INTERNAL: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The topic name that
* unregistered the service email address. Returns a success response if the service email address
* wasn't registered with the topic.
*
* Create a request for the method "integrators.unregisterSubscriber".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link UnregisterSubscriber#execute()} method to invoke the remote
* operation.
*
* @param integrator Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest}
* @return the request
*/
public UnregisterSubscriber unregisterSubscriber(java.lang.String integrator, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest content) throws java.io.IOException {
UnregisterSubscriber result = new UnregisterSubscriber(integrator, content);
initialize(result);
return result;
}
public class UnregisterSubscriber extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+integrator}:unregisterSubscriber";
private final java.util.regex.Pattern INTEGRATOR_PATTERN =
java.util.regex.Pattern.compile("^integrators/[^/]+$");
/**
* Unregisters a service account with subscriber privileges on the Pub/Sub topic created for this
* Channel Services account or integrator. If there are no service accounts left with subscriber
* privileges, this deletes the topic. You can call ListSubscribers to check for these accounts.
* Possible error codes: * PERMISSION_DENIED: The reseller account making the request and the
* provided reseller account are different, or the impersonated user is not a super admin. *
* INVALID_ARGUMENT: Required request parameters are missing or invalid. * NOT_FOUND: The topic
* resource doesn't exist. * INTERNAL: Any non-user error related to a technical issue in the
* backend. Contact Cloud Channel support. * UNKNOWN: Any non-user error related to a technical
* issue in the backend. Contact Cloud Channel support. Return value: The topic name that
* unregistered the service email address. Returns a success response if the service email address
* wasn't registered with the topic.
*
* Create a request for the method "integrators.unregisterSubscriber".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link UnregisterSubscriber#execute()} method to invoke the
* remote operation. {@link UnregisterSubscriber#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param integrator Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
* this field empty/unset.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest}
* @since 1.13
*/
protected UnregisterSubscriber(java.lang.String integrator, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1UnregisterSubscriberResponse.class);
this.integrator = com.google.api.client.util.Preconditions.checkNotNull(integrator, "Required parameter integrator must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(INTEGRATOR_PATTERN.matcher(integrator).matches(),
"Parameter integrator must conform to the pattern " +
"^integrators/[^/]+$");
}
}
@Override
public UnregisterSubscriber set$Xgafv(java.lang.String $Xgafv) {
return (UnregisterSubscriber) super.set$Xgafv($Xgafv);
}
@Override
public UnregisterSubscriber setAccessToken(java.lang.String accessToken) {
return (UnregisterSubscriber) super.setAccessToken(accessToken);
}
@Override
public UnregisterSubscriber setAlt(java.lang.String alt) {
return (UnregisterSubscriber) super.setAlt(alt);
}
@Override
public UnregisterSubscriber setCallback(java.lang.String callback) {
return (UnregisterSubscriber) super.setCallback(callback);
}
@Override
public UnregisterSubscriber setFields(java.lang.String fields) {
return (UnregisterSubscriber) super.setFields(fields);
}
@Override
public UnregisterSubscriber setKey(java.lang.String key) {
return (UnregisterSubscriber) super.setKey(key);
}
@Override
public UnregisterSubscriber setOauthToken(java.lang.String oauthToken) {
return (UnregisterSubscriber) super.setOauthToken(oauthToken);
}
@Override
public UnregisterSubscriber setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UnregisterSubscriber) super.setPrettyPrint(prettyPrint);
}
@Override
public UnregisterSubscriber setQuotaUser(java.lang.String quotaUser) {
return (UnregisterSubscriber) super.setQuotaUser(quotaUser);
}
@Override
public UnregisterSubscriber setUploadType(java.lang.String uploadType) {
return (UnregisterSubscriber) super.setUploadType(uploadType);
}
@Override
public UnregisterSubscriber setUploadProtocol(java.lang.String uploadProtocol) {
return (UnregisterSubscriber) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
@com.google.api.client.util.Key
private java.lang.String integrator;
/** Optional. Resource name of the integrator. Required if account is not provided. Otherwise, leave
this field empty/unset.
*/
public java.lang.String getIntegrator() {
return integrator;
}
/**
* Optional. Resource name of the integrator. Required if account is not provided. Otherwise,
* leave this field empty/unset.
*/
public UnregisterSubscriber setIntegrator(java.lang.String integrator) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(INTEGRATOR_PATTERN.matcher(integrator).matches(),
"Parameter integrator must conform to the pattern " +
"^integrators/[^/]+$");
}
this.integrator = integrator;
return this;
}
@Override
public UnregisterSubscriber set(String parameterName, Object value) {
return (UnregisterSubscriber) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Operations.List request = cloudchannel.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleLongrunningCancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningCancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.cloudchannel.v1.model.GoogleLongrunningCancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningCancelOperationRequest content) {
super(Cloudchannel.this, "POST", REST_PATH, content, com.google.api.services.cloudchannel.v1.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Cloudchannel.this, "DELETE", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleLongrunningListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Products collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Products.List request = cloudchannel.products().list(parameters ...)}
*
*
* @return the resource collection
*/
public Products products() {
return new Products();
}
/**
* The "products" collection of methods.
*/
public class Products {
/**
* Lists the Products the reseller is authorized to sell. Possible error codes: * INVALID_ARGUMENT:
* Required request parameters are missing or invalid.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/products";
/**
* Lists the Products the reseller is authorized to sell. Possible error codes: *
* INVALID_ARGUMENT: Required request parameters are missing or invalid.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListProductsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource name of the reseller account. Format: accounts/{account_id}. */
@com.google.api.client.util.Key
private java.lang.String account;
/** Required. The resource name of the reseller account. Format: accounts/{account_id}.
*/
public java.lang.String getAccount() {
return account;
}
/** Required. The resource name of the reseller account. Format: accounts/{account_id}. */
public List setAccount(java.lang.String account) {
this.account = account;
return this;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in the
* corresponding language code, if specified. The default value is "en-US".
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** Optional. The BCP-47 language code. For example, "en-US". The response will localize in the
corresponding language code, if specified. The default value is "en-US".
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in the
* corresponding language code, if specified. The default value is "en-US".
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 Products. The maximum value is 1000; the server will
* coerce values above 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server might return fewer results than requested. If unspecified,
returns at most 100 Products. The maximum value is 1000; the server will coerce values above 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 Products. The maximum value is 1000; the server will
* coerce values above 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token for a page of results other than the first page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token for a page of results other than the first page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token for a page of results other than the first page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Skus collection.
*
* The typical use is:
*
* {@code Cloudchannel cloudchannel = new Cloudchannel(...);}
* {@code Cloudchannel.Skus.List request = cloudchannel.skus().list(parameters ...)}
*
*
* @return the resource collection
*/
public Skus skus() {
return new Skus();
}
/**
* The "skus" collection of methods.
*/
public class Skus {
/**
* Lists the SKUs for a product the reseller is authorized to sell. Possible error codes: *
* INVALID_ARGUMENT: Required request parameters are missing or invalid.
*
* Create a request for the method "skus.list".
*
* This request holds the parameters needed by the cloudchannel server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the Product to list SKUs for. Parent uses the format:
* products/{product_id}. Supports products/- to retrieve SKUs for all products.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudchannelRequest {
private static final String REST_PATH = "v1/{+parent}/skus";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^products/[^/]+$");
/**
* Lists the SKUs for a product the reseller is authorized to sell. Possible error codes: *
* INVALID_ARGUMENT: Required request parameters are missing or invalid.
*
* Create a request for the method "skus.list".
*
* This request holds the parameters needed by the the cloudchannel server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the Product to list SKUs for. Parent uses the format:
* products/{product_id}. Supports products/- to retrieve SKUs for all products.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Cloudchannel.this, "GET", REST_PATH, null, com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1ListSkusResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^products/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Product to list SKUs for. Parent uses the format:
* products/{product_id}. Supports products/- to retrieve SKUs for all products.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the Product to list SKUs for. Parent uses the format:
products/{product_id}. Supports products/- to retrieve SKUs for all products.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the Product to list SKUs for. Parent uses the format:
* products/{product_id}. Supports products/- to retrieve SKUs for all products.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^products/[^/]+$");
}
this.parent = parent;
return this;
}
/** Required. Resource name of the reseller. Format: accounts/{account_id}. */
@com.google.api.client.util.Key
private java.lang.String account;
/** Required. Resource name of the reseller. Format: accounts/{account_id}.
*/
public java.lang.String getAccount() {
return account;
}
/** Required. Resource name of the reseller. Format: accounts/{account_id}. */
public List setAccount(java.lang.String account) {
this.account = account;
return this;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** Optional. The BCP-47 language code. For example, "en-US". The response will localize in the
corresponding language code, if specified. The default value is "en-US".
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Optional. The BCP-47 language code. For example, "en-US". The response will localize in
* the corresponding language code, if specified. The default value is "en-US".
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 SKUs. The maximum value is 1000; the server will coerce
* values above 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server might return fewer results than requested. If unspecified,
returns at most 100 SKUs. The maximum value is 1000; the server will coerce values above 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server might return fewer results than requested. If
* unspecified, returns at most 100 SKUs. The maximum value is 1000; the server will coerce
* values above 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token for a page of results other than the first page. Optional. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token for a page of results other than the first page. Optional.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token for a page of results other than the first page. Optional. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link Cloudchannel}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Cloudchannel}. */
@Override
public Cloudchannel build() {
return new Cloudchannel(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudchannelRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudchannelRequestInitializer(
CloudchannelRequestInitializer cloudchannelRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudchannelRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}