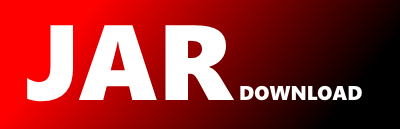
com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1CloudIdentityInfo Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudchannel.v1.model;
/**
* Cloud Identity information for the Cloud Channel Customer.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Channel API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudChannelV1CloudIdentityInfo extends com.google.api.client.json.GenericJson {
/**
* Output only. URI of Customer's Admin console dashboard.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adminConsoleUri;
/**
* The alternate email.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String alternateEmail;
/**
* CustomerType indicates verification type needed for using services.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customerType;
/**
* Edu information about the customer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudChannelV1EduData eduData;
/**
* Output only. Whether the domain is verified. This field is not returned for a Customer's
* cloud_identity_info resource. Partners can use the domains.get() method of the Workspace SDK's
* Directory API, or listen to the PRIMARY_DOMAIN_VERIFIED Pub/Sub event in to track domain
* verification of their resolve Workspace customers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isDomainVerified;
/**
* Language code.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/**
* Phone number associated with the Cloud Identity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String phoneNumber;
/**
* Output only. The primary domain name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String primaryDomain;
/**
* Output only. URI of Customer's Admin console dashboard.
* @return value or {@code null} for none
*/
public java.lang.String getAdminConsoleUri() {
return adminConsoleUri;
}
/**
* Output only. URI of Customer's Admin console dashboard.
* @param adminConsoleUri adminConsoleUri or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setAdminConsoleUri(java.lang.String adminConsoleUri) {
this.adminConsoleUri = adminConsoleUri;
return this;
}
/**
* The alternate email.
* @return value or {@code null} for none
*/
public java.lang.String getAlternateEmail() {
return alternateEmail;
}
/**
* The alternate email.
* @param alternateEmail alternateEmail or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setAlternateEmail(java.lang.String alternateEmail) {
this.alternateEmail = alternateEmail;
return this;
}
/**
* CustomerType indicates verification type needed for using services.
* @return value or {@code null} for none
*/
public java.lang.String getCustomerType() {
return customerType;
}
/**
* CustomerType indicates verification type needed for using services.
* @param customerType customerType or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setCustomerType(java.lang.String customerType) {
this.customerType = customerType;
return this;
}
/**
* Edu information about the customer.
* @return value or {@code null} for none
*/
public GoogleCloudChannelV1EduData getEduData() {
return eduData;
}
/**
* Edu information about the customer.
* @param eduData eduData or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setEduData(GoogleCloudChannelV1EduData eduData) {
this.eduData = eduData;
return this;
}
/**
* Output only. Whether the domain is verified. This field is not returned for a Customer's
* cloud_identity_info resource. Partners can use the domains.get() method of the Workspace SDK's
* Directory API, or listen to the PRIMARY_DOMAIN_VERIFIED Pub/Sub event in to track domain
* verification of their resolve Workspace customers.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsDomainVerified() {
return isDomainVerified;
}
/**
* Output only. Whether the domain is verified. This field is not returned for a Customer's
* cloud_identity_info resource. Partners can use the domains.get() method of the Workspace SDK's
* Directory API, or listen to the PRIMARY_DOMAIN_VERIFIED Pub/Sub event in to track domain
* verification of their resolve Workspace customers.
* @param isDomainVerified isDomainVerified or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setIsDomainVerified(java.lang.Boolean isDomainVerified) {
this.isDomainVerified = isDomainVerified;
return this;
}
/**
* Language code.
* @return value or {@code null} for none
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Language code.
* @param languageCode languageCode or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Phone number associated with the Cloud Identity.
* @return value or {@code null} for none
*/
public java.lang.String getPhoneNumber() {
return phoneNumber;
}
/**
* Phone number associated with the Cloud Identity.
* @param phoneNumber phoneNumber or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setPhoneNumber(java.lang.String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* Output only. The primary domain name.
* @return value or {@code null} for none
*/
public java.lang.String getPrimaryDomain() {
return primaryDomain;
}
/**
* Output only. The primary domain name.
* @param primaryDomain primaryDomain or {@code null} for none
*/
public GoogleCloudChannelV1CloudIdentityInfo setPrimaryDomain(java.lang.String primaryDomain) {
this.primaryDomain = primaryDomain;
return this;
}
@Override
public GoogleCloudChannelV1CloudIdentityInfo set(String fieldName, Object value) {
return (GoogleCloudChannelV1CloudIdentityInfo) super.set(fieldName, value);
}
@Override
public GoogleCloudChannelV1CloudIdentityInfo clone() {
return (GoogleCloudChannelV1CloudIdentityInfo) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy