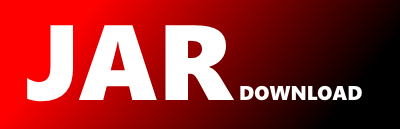
com.google.api.services.cloudchannel.v1.model.GoogleCloudChannelV1RepricingConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudchannel.v1.model;
/**
* Configuration for repricing a Google bill over a period of time.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Channel API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudChannelV1RepricingConfig extends com.google.api.client.json.GenericJson {
/**
* Required. Information about the adjustment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudChannelV1RepricingAdjustment adjustment;
/**
* Applies the repricing configuration at the channel partner level. Only
* ChannelPartnerRepricingConfig supports this value. Deprecated: This is no longer supported. Use
* RepricingConfig.entitlement_granularity instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudChannelV1RepricingConfigChannelPartnerGranularity channelPartnerGranularity;
/**
* The conditional overrides to apply for this configuration. If you list multiple overrides, only
* the first valid override is used. If you don't list any overrides, the API uses the normal
* adjustment and rebilling basis.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List conditionalOverrides;
static {
// hack to force ProGuard to consider GoogleCloudChannelV1ConditionalOverride used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudChannelV1ConditionalOverride.class);
}
/**
* Required. The YearMonth when these adjustments activate. The Day field needs to be "0" since we
* only accept YearMonth repricing boundaries.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleTypeDate effectiveInvoiceMonth;
/**
* Applies the repricing configuration at the entitlement level. Note: If a
* ChannelPartnerRepricingConfig using RepricingConfig.EntitlementGranularity becomes effective,
* then no existing or future RepricingConfig.ChannelPartnerGranularity will apply to the
* RepricingConfig.EntitlementGranularity.entitlement. This is the recommended value for both
* CustomerRepricingConfig and ChannelPartnerRepricingConfig.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudChannelV1RepricingConfigEntitlementGranularity entitlementGranularity;
/**
* Required. The RebillingBasis to use for this bill. Specifies the relative cost based on
* repricing costs you will apply.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rebillingBasis;
/**
* Required. Information about the adjustment.
* @return value or {@code null} for none
*/
public GoogleCloudChannelV1RepricingAdjustment getAdjustment() {
return adjustment;
}
/**
* Required. Information about the adjustment.
* @param adjustment adjustment or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfig setAdjustment(GoogleCloudChannelV1RepricingAdjustment adjustment) {
this.adjustment = adjustment;
return this;
}
/**
* Applies the repricing configuration at the channel partner level. Only
* ChannelPartnerRepricingConfig supports this value. Deprecated: This is no longer supported. Use
* RepricingConfig.entitlement_granularity instead.
* @return value or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfigChannelPartnerGranularity getChannelPartnerGranularity() {
return channelPartnerGranularity;
}
/**
* Applies the repricing configuration at the channel partner level. Only
* ChannelPartnerRepricingConfig supports this value. Deprecated: This is no longer supported. Use
* RepricingConfig.entitlement_granularity instead.
* @param channelPartnerGranularity channelPartnerGranularity or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfig setChannelPartnerGranularity(GoogleCloudChannelV1RepricingConfigChannelPartnerGranularity channelPartnerGranularity) {
this.channelPartnerGranularity = channelPartnerGranularity;
return this;
}
/**
* The conditional overrides to apply for this configuration. If you list multiple overrides, only
* the first valid override is used. If you don't list any overrides, the API uses the normal
* adjustment and rebilling basis.
* @return value or {@code null} for none
*/
public java.util.List getConditionalOverrides() {
return conditionalOverrides;
}
/**
* The conditional overrides to apply for this configuration. If you list multiple overrides, only
* the first valid override is used. If you don't list any overrides, the API uses the normal
* adjustment and rebilling basis.
* @param conditionalOverrides conditionalOverrides or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfig setConditionalOverrides(java.util.List conditionalOverrides) {
this.conditionalOverrides = conditionalOverrides;
return this;
}
/**
* Required. The YearMonth when these adjustments activate. The Day field needs to be "0" since we
* only accept YearMonth repricing boundaries.
* @return value or {@code null} for none
*/
public GoogleTypeDate getEffectiveInvoiceMonth() {
return effectiveInvoiceMonth;
}
/**
* Required. The YearMonth when these adjustments activate. The Day field needs to be "0" since we
* only accept YearMonth repricing boundaries.
* @param effectiveInvoiceMonth effectiveInvoiceMonth or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfig setEffectiveInvoiceMonth(GoogleTypeDate effectiveInvoiceMonth) {
this.effectiveInvoiceMonth = effectiveInvoiceMonth;
return this;
}
/**
* Applies the repricing configuration at the entitlement level. Note: If a
* ChannelPartnerRepricingConfig using RepricingConfig.EntitlementGranularity becomes effective,
* then no existing or future RepricingConfig.ChannelPartnerGranularity will apply to the
* RepricingConfig.EntitlementGranularity.entitlement. This is the recommended value for both
* CustomerRepricingConfig and ChannelPartnerRepricingConfig.
* @return value or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfigEntitlementGranularity getEntitlementGranularity() {
return entitlementGranularity;
}
/**
* Applies the repricing configuration at the entitlement level. Note: If a
* ChannelPartnerRepricingConfig using RepricingConfig.EntitlementGranularity becomes effective,
* then no existing or future RepricingConfig.ChannelPartnerGranularity will apply to the
* RepricingConfig.EntitlementGranularity.entitlement. This is the recommended value for both
* CustomerRepricingConfig and ChannelPartnerRepricingConfig.
* @param entitlementGranularity entitlementGranularity or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfig setEntitlementGranularity(GoogleCloudChannelV1RepricingConfigEntitlementGranularity entitlementGranularity) {
this.entitlementGranularity = entitlementGranularity;
return this;
}
/**
* Required. The RebillingBasis to use for this bill. Specifies the relative cost based on
* repricing costs you will apply.
* @return value or {@code null} for none
*/
public java.lang.String getRebillingBasis() {
return rebillingBasis;
}
/**
* Required. The RebillingBasis to use for this bill. Specifies the relative cost based on
* repricing costs you will apply.
* @param rebillingBasis rebillingBasis or {@code null} for none
*/
public GoogleCloudChannelV1RepricingConfig setRebillingBasis(java.lang.String rebillingBasis) {
this.rebillingBasis = rebillingBasis;
return this;
}
@Override
public GoogleCloudChannelV1RepricingConfig set(String fieldName, Object value) {
return (GoogleCloudChannelV1RepricingConfig) super.set(fieldName, value);
}
@Override
public GoogleCloudChannelV1RepricingConfig clone() {
return (GoogleCloudChannelV1RepricingConfig) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy