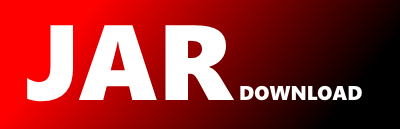
com.google.api.services.cloudcontrolspartner.v1.model.Violation Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudcontrolspartner.v1.model;
/**
* Details of resource Violation
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Controls Partner API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Violation extends com.google.api.client.json.GenericJson {
/**
* Output only. Time of the event which triggered the Violation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String beginTime;
/**
* Output only. Category under which this violation is mapped. e.g. Location, Service Usage,
* Access, Encryption, etc.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String category;
/**
* Output only. Description for the Violation. e.g. OrgPolicy gcp.resourceLocations has non
* compliant value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The folder_id of the violation
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long folderId;
/**
* Identifier. Format: `organizations/{organization}/locations/{location}/customers/{customer}/wor
* kloads/{workload}/violations/{violation}`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. Immutable. Name of the OrgPolicy which was modified with non-compliant change and
* resulted this violation. Format: `projects/{project_number}/policies/{constraint_name}`
* `folders/{folder_id}/policies/{constraint_name}`
* `organizations/{organization_id}/policies/{constraint_name}`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String nonCompliantOrgPolicy;
/**
* Output only. Compliance violation remediation
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Remediation remediation;
/**
* Output only. Time of the event which fixed the Violation. If the violation is ACTIVE this will
* be empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String resolveTime;
/**
* Output only. State of the violation
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Output only. The last time when the Violation record was updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Output only. Time of the event which triggered the Violation.
* @return value or {@code null} for none
*/
public String getBeginTime() {
return beginTime;
}
/**
* Output only. Time of the event which triggered the Violation.
* @param beginTime beginTime or {@code null} for none
*/
public Violation setBeginTime(String beginTime) {
this.beginTime = beginTime;
return this;
}
/**
* Output only. Category under which this violation is mapped. e.g. Location, Service Usage,
* Access, Encryption, etc.
* @return value or {@code null} for none
*/
public java.lang.String getCategory() {
return category;
}
/**
* Output only. Category under which this violation is mapped. e.g. Location, Service Usage,
* Access, Encryption, etc.
* @param category category or {@code null} for none
*/
public Violation setCategory(java.lang.String category) {
this.category = category;
return this;
}
/**
* Output only. Description for the Violation. e.g. OrgPolicy gcp.resourceLocations has non
* compliant value.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Output only. Description for the Violation. e.g. OrgPolicy gcp.resourceLocations has non
* compliant value.
* @param description description or {@code null} for none
*/
public Violation setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The folder_id of the violation
* @return value or {@code null} for none
*/
public java.lang.Long getFolderId() {
return folderId;
}
/**
* The folder_id of the violation
* @param folderId folderId or {@code null} for none
*/
public Violation setFolderId(java.lang.Long folderId) {
this.folderId = folderId;
return this;
}
/**
* Identifier. Format: `organizations/{organization}/locations/{location}/customers/{customer}/wor
* kloads/{workload}/violations/{violation}`
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Format: `organizations/{organization}/locations/{location}/customers/{customer}/wor
* kloads/{workload}/violations/{violation}`
* @param name name or {@code null} for none
*/
public Violation setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. Immutable. Name of the OrgPolicy which was modified with non-compliant change and
* resulted this violation. Format: `projects/{project_number}/policies/{constraint_name}`
* `folders/{folder_id}/policies/{constraint_name}`
* `organizations/{organization_id}/policies/{constraint_name}`
* @return value or {@code null} for none
*/
public java.lang.String getNonCompliantOrgPolicy() {
return nonCompliantOrgPolicy;
}
/**
* Output only. Immutable. Name of the OrgPolicy which was modified with non-compliant change and
* resulted this violation. Format: `projects/{project_number}/policies/{constraint_name}`
* `folders/{folder_id}/policies/{constraint_name}`
* `organizations/{organization_id}/policies/{constraint_name}`
* @param nonCompliantOrgPolicy nonCompliantOrgPolicy or {@code null} for none
*/
public Violation setNonCompliantOrgPolicy(java.lang.String nonCompliantOrgPolicy) {
this.nonCompliantOrgPolicy = nonCompliantOrgPolicy;
return this;
}
/**
* Output only. Compliance violation remediation
* @return value or {@code null} for none
*/
public Remediation getRemediation() {
return remediation;
}
/**
* Output only. Compliance violation remediation
* @param remediation remediation or {@code null} for none
*/
public Violation setRemediation(Remediation remediation) {
this.remediation = remediation;
return this;
}
/**
* Output only. Time of the event which fixed the Violation. If the violation is ACTIVE this will
* be empty.
* @return value or {@code null} for none
*/
public String getResolveTime() {
return resolveTime;
}
/**
* Output only. Time of the event which fixed the Violation. If the violation is ACTIVE this will
* be empty.
* @param resolveTime resolveTime or {@code null} for none
*/
public Violation setResolveTime(String resolveTime) {
this.resolveTime = resolveTime;
return this;
}
/**
* Output only. State of the violation
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. State of the violation
* @param state state or {@code null} for none
*/
public Violation setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* Output only. The last time when the Violation record was updated.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The last time when the Violation record was updated.
* @param updateTime updateTime or {@code null} for none
*/
public Violation setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public Violation set(String fieldName, Object value) {
return (Violation) super.set(fieldName, value);
}
@Override
public Violation clone() {
return (Violation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy