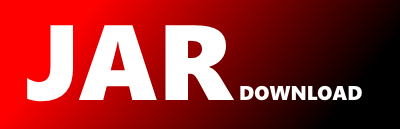
com.google.api.services.clouddebugger.v2.model.GerritSourceContext Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-01-11 18:31:16 UTC)
* on 2017-02-04 at 01:52:59 UTC
* Modify at your own risk.
*/
package com.google.api.services.clouddebugger.v2.model;
/**
* A SourceContext referring to a Gerrit project.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Stackdriver Debugger API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GerritSourceContext extends com.google.api.client.json.GenericJson {
/**
* An alias, which may be a branch or tag.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AliasContext aliasContext;
/**
* The name of an alias (branch, tag, etc.).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String aliasName;
/**
* The full project name within the host. Projects may be nested, so "project/subproject" is a
* valid project name. The "repo name" is hostURI/project.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gerritProject;
/**
* The URI of a running Gerrit instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hostUri;
/**
* A revision (commit) ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String revisionId;
/**
* An alias, which may be a branch or tag.
* @return value or {@code null} for none
*/
public AliasContext getAliasContext() {
return aliasContext;
}
/**
* An alias, which may be a branch or tag.
* @param aliasContext aliasContext or {@code null} for none
*/
public GerritSourceContext setAliasContext(AliasContext aliasContext) {
this.aliasContext = aliasContext;
return this;
}
/**
* The name of an alias (branch, tag, etc.).
* @return value or {@code null} for none
*/
public java.lang.String getAliasName() {
return aliasName;
}
/**
* The name of an alias (branch, tag, etc.).
* @param aliasName aliasName or {@code null} for none
*/
public GerritSourceContext setAliasName(java.lang.String aliasName) {
this.aliasName = aliasName;
return this;
}
/**
* The full project name within the host. Projects may be nested, so "project/subproject" is a
* valid project name. The "repo name" is hostURI/project.
* @return value or {@code null} for none
*/
public java.lang.String getGerritProject() {
return gerritProject;
}
/**
* The full project name within the host. Projects may be nested, so "project/subproject" is a
* valid project name. The "repo name" is hostURI/project.
* @param gerritProject gerritProject or {@code null} for none
*/
public GerritSourceContext setGerritProject(java.lang.String gerritProject) {
this.gerritProject = gerritProject;
return this;
}
/**
* The URI of a running Gerrit instance.
* @return value or {@code null} for none
*/
public java.lang.String getHostUri() {
return hostUri;
}
/**
* The URI of a running Gerrit instance.
* @param hostUri hostUri or {@code null} for none
*/
public GerritSourceContext setHostUri(java.lang.String hostUri) {
this.hostUri = hostUri;
return this;
}
/**
* A revision (commit) ID.
* @return value or {@code null} for none
*/
public java.lang.String getRevisionId() {
return revisionId;
}
/**
* A revision (commit) ID.
* @param revisionId revisionId or {@code null} for none
*/
public GerritSourceContext setRevisionId(java.lang.String revisionId) {
this.revisionId = revisionId;
return this;
}
@Override
public GerritSourceContext set(String fieldName, Object value) {
return (GerritSourceContext) super.set(fieldName, value);
}
@Override
public GerritSourceContext clone() {
return (GerritSourceContext) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy