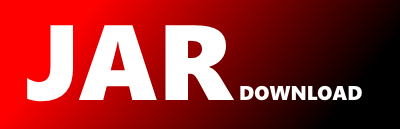
com.google.api.services.clouddebugger.v2.model.Variable Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-01-11 18:31:16 UTC)
* on 2017-02-04 at 01:52:59 UTC
* Modify at your own risk.
*/
package com.google.api.services.clouddebugger.v2.model;
/**
* Represents a variable or an argument possibly of a compound object type. Note how the following
* variables are represented:
*
* 1) A simple variable:
*
* int x = 5
*
* { name: "x", value: "5", type: "int" } // Captured variable
*
* 2) A compound object:
*
* struct T { int m1; int m2; }; T x = { 3, 7 };
*
* { // Captured variable name: "x", type: "T", members { name: "m1",
* value: "3", type: "int" }, members { name: "m2", value: "7", type: "int" } }
*
* 3) A pointer where the pointee was captured:
*
* T x = { 3, 7 }; T* p =
*
* { // Captured variable name: "p", type: "T*", value: "0x00500500",
* members { name: "m1", value: "3", type: "int" }, members { name: "m2", value: "7", type:
* "int" } }
*
* 4) A pointer where the pointee was not captured:
*
* T* p = new T;
*
* { // Captured variable name: "p", type: "T*", value: "0x00400400"
* status { is_error: true, description { format: "unavailable" } } }
*
* The status should describe the reason for the missing value, such as ``, ``, ``.
*
* Note that a null pointer should not have members.
*
* 5) An unnamed value:
*
* int* p = new int(7);
*
* { // Captured variable name: "p", value: "0x00500500", type:
* "int*", members { value: "7", type: "int" } }
*
* 6) An unnamed pointer where the pointee was not captured:
*
* int* p = new int(7); int** pp =
*
* { // Captured variable name: "pp", value: "0x00500500", type:
* "int**", members { value: "0x00400400", type: "int*"
* status { is_error: true, description: { format: "unavailable" } }
* } } }
*
* To optimize computation, memory and network traffic, variables that repeat in the output multiple
* times can be stored once in a shared variable table and be referenced using the `var_table_index`
* field. The variables stored in the shared table are nameless and are essentially a partition of
* the complete variable. To reconstruct the complete variable, merge the referencing variable with
* the referenced variable.
*
* When using the shared variable table, the following variables:
*
* T x = { 3, 7 }; T* p = T& r = x;
*
* { name: "x", var_table_index: 3, type: "T" } // Captured variables { name: "p", value
* "0x00500500", type="T*", var_table_index: 3 } { name: "r", type="T&", var_table_index: 3 }
*
* { // Shared variable table entry #3: members { name: "m1", value: "3", type: "int"
* }, members { name: "m2", value: "7", type: "int" } }
*
* Note that the pointer address is stored with the referencing variable and not with the referenced
* variable. This allows the referenced variable to be shared between pointers and references.
*
* The type field is optional. The debugger agent may or may not support it.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Stackdriver Debugger API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Variable extends com.google.api.client.json.GenericJson {
/**
* Members contained or pointed to by the variable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List members;
/**
* Name of the variable, if any.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Status associated with the variable. This field will usually stay unset. A status of a single
* variable only applies to that variable or expression. The rest of breakpoint data still remains
* valid. Variables might be reported in error state even when breakpoint is not in final state.
*
* The message may refer to variable name with `refers_to` set to `VARIABLE_NAME`. Alternatively
* `refers_to` will be set to `VARIABLE_VALUE`. In either case variable value and members will be
* unset.
*
* Example of error message applied to name: `Invalid expression syntax`.
*
* Example of information message applied to value: `Not captured`.
*
* Examples of error message applied to value:
*
* * `Malformed string`, * `Field f not found in class C` * `Null pointer dereference`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StatusMessage status;
/**
* Variable type (e.g. `MyClass`). If the variable is split with `var_table_index`, `type` goes
* next to `value`. The interpretation of a type is agent specific. It is recommended to include
* the dynamic type rather than a static type of an object.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* Simple value of the variable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* Reference to a variable in the shared variable table. More than one variable can reference the
* same variable in the table. The `var_table_index` field is an index into `variable_table` in
* Breakpoint.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer varTableIndex;
/**
* Members contained or pointed to by the variable.
* @return value or {@code null} for none
*/
public java.util.List getMembers() {
return members;
}
/**
* Members contained or pointed to by the variable.
* @param members members or {@code null} for none
*/
public Variable setMembers(java.util.List members) {
this.members = members;
return this;
}
/**
* Name of the variable, if any.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the variable, if any.
* @param name name or {@code null} for none
*/
public Variable setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Status associated with the variable. This field will usually stay unset. A status of a single
* variable only applies to that variable or expression. The rest of breakpoint data still remains
* valid. Variables might be reported in error state even when breakpoint is not in final state.
*
* The message may refer to variable name with `refers_to` set to `VARIABLE_NAME`. Alternatively
* `refers_to` will be set to `VARIABLE_VALUE`. In either case variable value and members will be
* unset.
*
* Example of error message applied to name: `Invalid expression syntax`.
*
* Example of information message applied to value: `Not captured`.
*
* Examples of error message applied to value:
*
* * `Malformed string`, * `Field f not found in class C` * `Null pointer dereference`
* @return value or {@code null} for none
*/
public StatusMessage getStatus() {
return status;
}
/**
* Status associated with the variable. This field will usually stay unset. A status of a single
* variable only applies to that variable or expression. The rest of breakpoint data still remains
* valid. Variables might be reported in error state even when breakpoint is not in final state.
*
* The message may refer to variable name with `refers_to` set to `VARIABLE_NAME`. Alternatively
* `refers_to` will be set to `VARIABLE_VALUE`. In either case variable value and members will be
* unset.
*
* Example of error message applied to name: `Invalid expression syntax`.
*
* Example of information message applied to value: `Not captured`.
*
* Examples of error message applied to value:
*
* * `Malformed string`, * `Field f not found in class C` * `Null pointer dereference`
* @param status status or {@code null} for none
*/
public Variable setStatus(StatusMessage status) {
this.status = status;
return this;
}
/**
* Variable type (e.g. `MyClass`). If the variable is split with `var_table_index`, `type` goes
* next to `value`. The interpretation of a type is agent specific. It is recommended to include
* the dynamic type rather than a static type of an object.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Variable type (e.g. `MyClass`). If the variable is split with `var_table_index`, `type` goes
* next to `value`. The interpretation of a type is agent specific. It is recommended to include
* the dynamic type rather than a static type of an object.
* @param type type or {@code null} for none
*/
public Variable setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* Simple value of the variable.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* Simple value of the variable.
* @param value value or {@code null} for none
*/
public Variable setValue(java.lang.String value) {
this.value = value;
return this;
}
/**
* Reference to a variable in the shared variable table. More than one variable can reference the
* same variable in the table. The `var_table_index` field is an index into `variable_table` in
* Breakpoint.
* @return value or {@code null} for none
*/
public java.lang.Integer getVarTableIndex() {
return varTableIndex;
}
/**
* Reference to a variable in the shared variable table. More than one variable can reference the
* same variable in the table. The `var_table_index` field is an index into `variable_table` in
* Breakpoint.
* @param varTableIndex varTableIndex or {@code null} for none
*/
public Variable setVarTableIndex(java.lang.Integer varTableIndex) {
this.varTableIndex = varTableIndex;
return this;
}
@Override
public Variable set(String fieldName, Object value) {
return (Variable) super.set(fieldName, value);
}
@Override
public Variable clone() {
return (Variable) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy