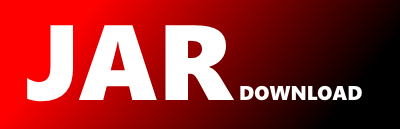
com.google.api.services.clouddebugger.v2.CloudDebugger Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-01-11 18:31:16 UTC)
* on 2017-01-22 at 02:07:42 UTC
* Modify at your own risk.
*/
package com.google.api.services.clouddebugger.v2;
/**
* Service definition for CloudDebugger (v2).
*
*
* Examines the call stack and variables of a running application without stopping or slowing it down.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudDebuggerRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudDebugger extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Stackdriver Debugger API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://clouddebugger.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudDebugger(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudDebugger(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Controller collection.
*
* The typical use is:
*
* {@code CloudDebugger clouddebugger = new CloudDebugger(...);}
* {@code CloudDebugger.Controller.List request = clouddebugger.controller().list(parameters ...)}
*
*
* @return the resource collection
*/
public Controller controller() {
return new Controller();
}
/**
* The "controller" collection of methods.
*/
public class Controller {
/**
* An accessor for creating requests from the Debuggees collection.
*
* The typical use is:
*
* {@code CloudDebugger clouddebugger = new CloudDebugger(...);}
* {@code CloudDebugger.Debuggees.List request = clouddebugger.debuggees().list(parameters ...)}
*
*
* @return the resource collection
*/
public Debuggees debuggees() {
return new Debuggees();
}
/**
* The "debuggees" collection of methods.
*/
public class Debuggees {
/**
* Registers the debuggee with the controller service.
*
* All agents attached to the same application should call this method with the same request content
* to get back the same stable `debuggee_id`. Agents should call this method again whenever
* `google.rpc.Code.NOT_FOUND` is returned from any controller method.
*
* This allows the controller service to disable the agent or recover from any data loss. If the
* debuggee is disabled by the server, the response will have `is_disabled` set to `true`.
*
* Create a request for the method "debuggees.register".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link Register#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.clouddebugger.v2.model.RegisterDebuggeeRequest}
* @return the request
*/
public Register register(com.google.api.services.clouddebugger.v2.model.RegisterDebuggeeRequest content) throws java.io.IOException {
Register result = new Register(content);
initialize(result);
return result;
}
public class Register extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/controller/debuggees/register";
/**
* Registers the debuggee with the controller service.
*
* All agents attached to the same application should call this method with the same request
* content to get back the same stable `debuggee_id`. Agents should call this method again
* whenever `google.rpc.Code.NOT_FOUND` is returned from any controller method.
*
* This allows the controller service to disable the agent or recover from any data loss. If the
* debuggee is disabled by the server, the response will have `is_disabled` set to `true`.
*
* Create a request for the method "debuggees.register".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link Register#execute()} method to invoke the remote operation.
* {@link
* Register#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.clouddebugger.v2.model.RegisterDebuggeeRequest}
* @since 1.13
*/
protected Register(com.google.api.services.clouddebugger.v2.model.RegisterDebuggeeRequest content) {
super(CloudDebugger.this, "POST", REST_PATH, content, com.google.api.services.clouddebugger.v2.model.RegisterDebuggeeResponse.class);
}
@Override
public Register set$Xgafv(java.lang.String $Xgafv) {
return (Register) super.set$Xgafv($Xgafv);
}
@Override
public Register setAccessToken(java.lang.String accessToken) {
return (Register) super.setAccessToken(accessToken);
}
@Override
public Register setAlt(java.lang.String alt) {
return (Register) super.setAlt(alt);
}
@Override
public Register setBearerToken(java.lang.String bearerToken) {
return (Register) super.setBearerToken(bearerToken);
}
@Override
public Register setCallback(java.lang.String callback) {
return (Register) super.setCallback(callback);
}
@Override
public Register setFields(java.lang.String fields) {
return (Register) super.setFields(fields);
}
@Override
public Register setKey(java.lang.String key) {
return (Register) super.setKey(key);
}
@Override
public Register setOauthToken(java.lang.String oauthToken) {
return (Register) super.setOauthToken(oauthToken);
}
@Override
public Register setPp(java.lang.Boolean pp) {
return (Register) super.setPp(pp);
}
@Override
public Register setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Register) super.setPrettyPrint(prettyPrint);
}
@Override
public Register setQuotaUser(java.lang.String quotaUser) {
return (Register) super.setQuotaUser(quotaUser);
}
@Override
public Register setUploadType(java.lang.String uploadType) {
return (Register) super.setUploadType(uploadType);
}
@Override
public Register setUploadProtocol(java.lang.String uploadProtocol) {
return (Register) super.setUploadProtocol(uploadProtocol);
}
@Override
public Register set(String parameterName, Object value) {
return (Register) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Breakpoints collection.
*
* The typical use is:
*
* {@code CloudDebugger clouddebugger = new CloudDebugger(...);}
* {@code CloudDebugger.Breakpoints.List request = clouddebugger.breakpoints().list(parameters ...)}
*
*
* @return the resource collection
*/
public Breakpoints breakpoints() {
return new Breakpoints();
}
/**
* The "breakpoints" collection of methods.
*/
public class Breakpoints {
/**
* Returns the list of all active breakpoints for the debuggee.
*
* The breakpoint specification (location, condition, and expression fields) is semantically
* immutable, although the field values may change. For example, an agent may update the location
* line number to reflect the actual line where the breakpoint was set, but this doesn't change the
* breakpoint semantics.
*
* This means that an agent does not need to check if a breakpoint has changed when it encounters
* the same breakpoint on a successive call. Moreover, an agent should remember the breakpoints that
* are completed until the controller removes them from the active list to avoid setting those
* breakpoints again.
*
* Create a request for the method "breakpoints.list".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param debuggeeId Identifies the debuggee.
* @return the request
*/
public List list(java.lang.String debuggeeId) throws java.io.IOException {
List result = new List(debuggeeId);
initialize(result);
return result;
}
public class List extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/controller/debuggees/{debuggeeId}/breakpoints";
/**
* Returns the list of all active breakpoints for the debuggee.
*
* The breakpoint specification (location, condition, and expression fields) is semantically
* immutable, although the field values may change. For example, an agent may update the location
* line number to reflect the actual line where the breakpoint was set, but this doesn't change
* the breakpoint semantics.
*
* This means that an agent does not need to check if a breakpoint has changed when it encounters
* the same breakpoint on a successive call. Moreover, an agent should remember the breakpoints
* that are completed until the controller removes them from the active list to avoid setting
* those breakpoints again.
*
* Create a request for the method "breakpoints.list".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param debuggeeId Identifies the debuggee.
* @since 1.13
*/
protected List(java.lang.String debuggeeId) {
super(CloudDebugger.this, "GET", REST_PATH, null, com.google.api.services.clouddebugger.v2.model.ListActiveBreakpointsResponse.class);
this.debuggeeId = com.google.api.client.util.Preconditions.checkNotNull(debuggeeId, "Required parameter debuggeeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Identifies the debuggee. */
@com.google.api.client.util.Key
private java.lang.String debuggeeId;
/** Identifies the debuggee.
*/
public java.lang.String getDebuggeeId() {
return debuggeeId;
}
/** Identifies the debuggee. */
public List setDebuggeeId(java.lang.String debuggeeId) {
this.debuggeeId = debuggeeId;
return this;
}
/**
* A wait token that, if specified, blocks the method call until the list of active
* breakpoints has changed, or a server selected timeout has expired. The value should be
* set from the last returned response.
*/
@com.google.api.client.util.Key
private java.lang.String waitToken;
/** A wait token that, if specified, blocks the method call until the list of active breakpoints has
changed, or a server selected timeout has expired. The value should be set from the last returned
response.
*/
public java.lang.String getWaitToken() {
return waitToken;
}
/**
* A wait token that, if specified, blocks the method call until the list of active
* breakpoints has changed, or a server selected timeout has expired. The value should be
* set from the last returned response.
*/
public List setWaitToken(java.lang.String waitToken) {
this.waitToken = waitToken;
return this;
}
/**
* If set to `true`, returns `google.rpc.Code.OK` status and sets the `wait_expired`
* response field to `true` when the server-selected timeout has expired (recommended).
*
* If set to `false`, returns `google.rpc.Code.ABORTED` status when the server-selected
* timeout has expired (deprecated).
*/
@com.google.api.client.util.Key
private java.lang.Boolean successOnTimeout;
/** If set to `true`, returns `google.rpc.Code.OK` status and sets the `wait_expired` response field to
`true` when the server-selected timeout has expired (recommended).
If set to `false`, returns `google.rpc.Code.ABORTED` status when the server-selected timeout has
expired (deprecated).
*/
public java.lang.Boolean getSuccessOnTimeout() {
return successOnTimeout;
}
/**
* If set to `true`, returns `google.rpc.Code.OK` status and sets the `wait_expired`
* response field to `true` when the server-selected timeout has expired (recommended).
*
* If set to `false`, returns `google.rpc.Code.ABORTED` status when the server-selected
* timeout has expired (deprecated).
*/
public List setSuccessOnTimeout(java.lang.Boolean successOnTimeout) {
this.successOnTimeout = successOnTimeout;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the breakpoint state or mutable fields. The entire Breakpoint message must be sent back
* to the controller service.
*
* Updates to active breakpoint fields are only allowed if the new value does not change the
* breakpoint specification. Updates to the `location`, `condition` and `expression` fields should
* not alter the breakpoint semantics. These may only make changes such as canonicalizing a value or
* snapping the location to the correct line of code.
*
* Create a request for the method "breakpoints.update".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param debuggeeId Identifies the debuggee being debugged.
* @param id Breakpoint identifier, unique in the scope of the debuggee.
* @param content the {@link com.google.api.services.clouddebugger.v2.model.UpdateActiveBreakpointRequest}
* @return the request
*/
public Update update(java.lang.String debuggeeId, java.lang.String id, com.google.api.services.clouddebugger.v2.model.UpdateActiveBreakpointRequest content) throws java.io.IOException {
Update result = new Update(debuggeeId, id, content);
initialize(result);
return result;
}
public class Update extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/controller/debuggees/{debuggeeId}/breakpoints/{id}";
/**
* Updates the breakpoint state or mutable fields. The entire Breakpoint message must be sent back
* to the controller service.
*
* Updates to active breakpoint fields are only allowed if the new value does not change the
* breakpoint specification. Updates to the `location`, `condition` and `expression` fields should
* not alter the breakpoint semantics. These may only make changes such as canonicalizing a value
* or snapping the location to the correct line of code.
*
* Create a request for the method "breakpoints.update".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param debuggeeId Identifies the debuggee being debugged.
* @param id Breakpoint identifier, unique in the scope of the debuggee.
* @param content the {@link com.google.api.services.clouddebugger.v2.model.UpdateActiveBreakpointRequest}
* @since 1.13
*/
protected Update(java.lang.String debuggeeId, java.lang.String id, com.google.api.services.clouddebugger.v2.model.UpdateActiveBreakpointRequest content) {
super(CloudDebugger.this, "PUT", REST_PATH, content, com.google.api.services.clouddebugger.v2.model.UpdateActiveBreakpointResponse.class);
this.debuggeeId = com.google.api.client.util.Preconditions.checkNotNull(debuggeeId, "Required parameter debuggeeId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setBearerToken(java.lang.String bearerToken) {
return (Update) super.setBearerToken(bearerToken);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPp(java.lang.Boolean pp) {
return (Update) super.setPp(pp);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Identifies the debuggee being debugged. */
@com.google.api.client.util.Key
private java.lang.String debuggeeId;
/** Identifies the debuggee being debugged.
*/
public java.lang.String getDebuggeeId() {
return debuggeeId;
}
/** Identifies the debuggee being debugged. */
public Update setDebuggeeId(java.lang.String debuggeeId) {
this.debuggeeId = debuggeeId;
return this;
}
/** Breakpoint identifier, unique in the scope of the debuggee. */
@com.google.api.client.util.Key
private java.lang.String id;
/** Breakpoint identifier, unique in the scope of the debuggee.
*/
public java.lang.String getId() {
return id;
}
/** Breakpoint identifier, unique in the scope of the debuggee. */
public Update setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Debugger collection.
*
* The typical use is:
*
* {@code CloudDebugger clouddebugger = new CloudDebugger(...);}
* {@code CloudDebugger.Debugger.List request = clouddebugger.debugger().list(parameters ...)}
*
*
* @return the resource collection
*/
public Debugger debugger() {
return new Debugger();
}
/**
* The "debugger" collection of methods.
*/
public class Debugger {
/**
* An accessor for creating requests from the Debuggees collection.
*
* The typical use is:
*
* {@code CloudDebugger clouddebugger = new CloudDebugger(...);}
* {@code CloudDebugger.Debuggees.List request = clouddebugger.debuggees().list(parameters ...)}
*
*
* @return the resource collection
*/
public Debuggees debuggees() {
return new Debuggees();
}
/**
* The "debuggees" collection of methods.
*/
public class Debuggees {
/**
* Lists all the debuggees that the user can set breakpoints to.
*
* Create a request for the method "debuggees.list".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/debugger/debuggees";
/**
* Lists all the debuggees that the user can set breakpoints to.
*
* Create a request for the method "debuggees.list".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudDebugger.this, "GET", REST_PATH, null, com.google.api.services.clouddebugger.v2.model.ListDebuggeesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* When set to `true`, the result includes all debuggees. Otherwise, the result includes
* only debuggees that are active.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeInactive;
/** When set to `true`, the result includes all debuggees. Otherwise, the result includes only
debuggees that are active.
*/
public java.lang.Boolean getIncludeInactive() {
return includeInactive;
}
/**
* When set to `true`, the result includes all debuggees. Otherwise, the result includes
* only debuggees that are active.
*/
public List setIncludeInactive(java.lang.Boolean includeInactive) {
this.includeInactive = includeInactive;
return this;
}
/** Project number of a Google Cloud project whose debuggees to list. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project number of a Google Cloud project whose debuggees to list.
*/
public java.lang.String getProject() {
return project;
}
/** Project number of a Google Cloud project whose debuggees to list. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
@com.google.api.client.util.Key
private java.lang.String clientVersion;
/** The client version making the call. Following: `domain/type/version` (e.g.,
`google.com/intellij/v1`).
*/
public java.lang.String getClientVersion() {
return clientVersion;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
public List setClientVersion(java.lang.String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Breakpoints collection.
*
* The typical use is:
*
* {@code CloudDebugger clouddebugger = new CloudDebugger(...);}
* {@code CloudDebugger.Breakpoints.List request = clouddebugger.breakpoints().list(parameters ...)}
*
*
* @return the resource collection
*/
public Breakpoints breakpoints() {
return new Breakpoints();
}
/**
* The "breakpoints" collection of methods.
*/
public class Breakpoints {
/**
* Deletes the breakpoint from the debuggee.
*
* Create a request for the method "breakpoints.delete".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param debuggeeId ID of the debuggee whose breakpoint to delete.
* @param breakpointId ID of the breakpoint to delete.
* @return the request
*/
public Delete delete(java.lang.String debuggeeId, java.lang.String breakpointId) throws java.io.IOException {
Delete result = new Delete(debuggeeId, breakpointId);
initialize(result);
return result;
}
public class Delete extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/debugger/debuggees/{debuggeeId}/breakpoints/{breakpointId}";
/**
* Deletes the breakpoint from the debuggee.
*
* Create a request for the method "breakpoints.delete".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param debuggeeId ID of the debuggee whose breakpoint to delete.
* @param breakpointId ID of the breakpoint to delete.
* @since 1.13
*/
protected Delete(java.lang.String debuggeeId, java.lang.String breakpointId) {
super(CloudDebugger.this, "DELETE", REST_PATH, null, com.google.api.services.clouddebugger.v2.model.Empty.class);
this.debuggeeId = com.google.api.client.util.Preconditions.checkNotNull(debuggeeId, "Required parameter debuggeeId must be specified.");
this.breakpointId = com.google.api.client.util.Preconditions.checkNotNull(breakpointId, "Required parameter breakpointId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** ID of the debuggee whose breakpoint to delete. */
@com.google.api.client.util.Key
private java.lang.String debuggeeId;
/** ID of the debuggee whose breakpoint to delete.
*/
public java.lang.String getDebuggeeId() {
return debuggeeId;
}
/** ID of the debuggee whose breakpoint to delete. */
public Delete setDebuggeeId(java.lang.String debuggeeId) {
this.debuggeeId = debuggeeId;
return this;
}
/** ID of the breakpoint to delete. */
@com.google.api.client.util.Key
private java.lang.String breakpointId;
/** ID of the breakpoint to delete.
*/
public java.lang.String getBreakpointId() {
return breakpointId;
}
/** ID of the breakpoint to delete. */
public Delete setBreakpointId(java.lang.String breakpointId) {
this.breakpointId = breakpointId;
return this;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
@com.google.api.client.util.Key
private java.lang.String clientVersion;
/** The client version making the call. Following: `domain/type/version` (e.g.,
`google.com/intellij/v1`).
*/
public java.lang.String getClientVersion() {
return clientVersion;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
public Delete setClientVersion(java.lang.String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets breakpoint information.
*
* Create a request for the method "breakpoints.get".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param debuggeeId ID of the debuggee whose breakpoint to get.
* @param breakpointId ID of the breakpoint to get.
* @return the request
*/
public Get get(java.lang.String debuggeeId, java.lang.String breakpointId) throws java.io.IOException {
Get result = new Get(debuggeeId, breakpointId);
initialize(result);
return result;
}
public class Get extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/debugger/debuggees/{debuggeeId}/breakpoints/{breakpointId}";
/**
* Gets breakpoint information.
*
* Create a request for the method "breakpoints.get".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param debuggeeId ID of the debuggee whose breakpoint to get.
* @param breakpointId ID of the breakpoint to get.
* @since 1.13
*/
protected Get(java.lang.String debuggeeId, java.lang.String breakpointId) {
super(CloudDebugger.this, "GET", REST_PATH, null, com.google.api.services.clouddebugger.v2.model.GetBreakpointResponse.class);
this.debuggeeId = com.google.api.client.util.Preconditions.checkNotNull(debuggeeId, "Required parameter debuggeeId must be specified.");
this.breakpointId = com.google.api.client.util.Preconditions.checkNotNull(breakpointId, "Required parameter breakpointId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** ID of the debuggee whose breakpoint to get. */
@com.google.api.client.util.Key
private java.lang.String debuggeeId;
/** ID of the debuggee whose breakpoint to get.
*/
public java.lang.String getDebuggeeId() {
return debuggeeId;
}
/** ID of the debuggee whose breakpoint to get. */
public Get setDebuggeeId(java.lang.String debuggeeId) {
this.debuggeeId = debuggeeId;
return this;
}
/** ID of the breakpoint to get. */
@com.google.api.client.util.Key
private java.lang.String breakpointId;
/** ID of the breakpoint to get.
*/
public java.lang.String getBreakpointId() {
return breakpointId;
}
/** ID of the breakpoint to get. */
public Get setBreakpointId(java.lang.String breakpointId) {
this.breakpointId = breakpointId;
return this;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
@com.google.api.client.util.Key
private java.lang.String clientVersion;
/** The client version making the call. Following: `domain/type/version` (e.g.,
`google.com/intellij/v1`).
*/
public java.lang.String getClientVersion() {
return clientVersion;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
public Get setClientVersion(java.lang.String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all breakpoints for the debuggee.
*
* Create a request for the method "breakpoints.list".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param debuggeeId ID of the debuggee whose breakpoints to list.
* @return the request
*/
public List list(java.lang.String debuggeeId) throws java.io.IOException {
List result = new List(debuggeeId);
initialize(result);
return result;
}
public class List extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/debugger/debuggees/{debuggeeId}/breakpoints";
/**
* Lists all breakpoints for the debuggee.
*
* Create a request for the method "breakpoints.list".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param debuggeeId ID of the debuggee whose breakpoints to list.
* @since 1.13
*/
protected List(java.lang.String debuggeeId) {
super(CloudDebugger.this, "GET", REST_PATH, null, com.google.api.services.clouddebugger.v2.model.ListBreakpointsResponse.class);
this.debuggeeId = com.google.api.client.util.Preconditions.checkNotNull(debuggeeId, "Required parameter debuggeeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** ID of the debuggee whose breakpoints to list. */
@com.google.api.client.util.Key
private java.lang.String debuggeeId;
/** ID of the debuggee whose breakpoints to list.
*/
public java.lang.String getDebuggeeId() {
return debuggeeId;
}
/** ID of the debuggee whose breakpoints to list. */
public List setDebuggeeId(java.lang.String debuggeeId) {
this.debuggeeId = debuggeeId;
return this;
}
/**
* A wait token that, if specified, blocks the call until the breakpoints list has
* changed, or a server selected timeout has expired. The value should be set from the
* last response. The error code `google.rpc.Code.ABORTED` (RPC) is returned on wait
* timeout, which should be called again with the same `wait_token`.
*/
@com.google.api.client.util.Key
private java.lang.String waitToken;
/** A wait token that, if specified, blocks the call until the breakpoints list has changed, or a
server selected timeout has expired. The value should be set from the last response. The error
code `google.rpc.Code.ABORTED` (RPC) is returned on wait timeout, which should be called again with
the same `wait_token`.
*/
public java.lang.String getWaitToken() {
return waitToken;
}
/**
* A wait token that, if specified, blocks the call until the breakpoints list has
* changed, or a server selected timeout has expired. The value should be set from the
* last response. The error code `google.rpc.Code.ABORTED` (RPC) is returned on wait
* timeout, which should be called again with the same `wait_token`.
*/
public List setWaitToken(java.lang.String waitToken) {
this.waitToken = waitToken;
return this;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
@com.google.api.client.util.Key
private java.lang.String clientVersion;
/** The client version making the call. Following: `domain/type/version` (e.g.,
`google.com/intellij/v1`).
*/
public java.lang.String getClientVersion() {
return clientVersion;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
public List setClientVersion(java.lang.String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
/** Only breakpoints with the specified action will pass the filter. */
@com.google.api.client.util.Key("action.value")
private java.lang.String actionValue;
/** Only breakpoints with the specified action will pass the filter.
*/
public java.lang.String getActionValue() {
return actionValue;
}
/** Only breakpoints with the specified action will pass the filter. */
public List setActionValue(java.lang.String actionValue) {
this.actionValue = actionValue;
return this;
}
/**
* When set to `true`, the response includes active and inactive breakpoints. Otherwise,
* it includes only active breakpoints.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeInactive;
/** When set to `true`, the response includes active and inactive breakpoints. Otherwise, it includes
only active breakpoints.
*/
public java.lang.Boolean getIncludeInactive() {
return includeInactive;
}
/**
* When set to `true`, the response includes active and inactive breakpoints. Otherwise,
* it includes only active breakpoints.
*/
public List setIncludeInactive(java.lang.Boolean includeInactive) {
this.includeInactive = includeInactive;
return this;
}
/**
* When set to `true`, the response includes the list of breakpoints set by any user.
* Otherwise, it includes only breakpoints set by the caller.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeAllUsers;
/** When set to `true`, the response includes the list of breakpoints set by any user. Otherwise, it
includes only breakpoints set by the caller.
*/
public java.lang.Boolean getIncludeAllUsers() {
return includeAllUsers;
}
/**
* When set to `true`, the response includes the list of breakpoints set by any user.
* Otherwise, it includes only breakpoints set by the caller.
*/
public List setIncludeAllUsers(java.lang.Boolean includeAllUsers) {
this.includeAllUsers = includeAllUsers;
return this;
}
/**
* This field is deprecated. The following fields are always stripped out of the result:
* `stack_frames`, `evaluated_expressions` and `variable_table`.
*/
@com.google.api.client.util.Key
private java.lang.Boolean stripResults;
/** This field is deprecated. The following fields are always stripped out of the result:
`stack_frames`, `evaluated_expressions` and `variable_table`.
*/
public java.lang.Boolean getStripResults() {
return stripResults;
}
/**
* This field is deprecated. The following fields are always stripped out of the result:
* `stack_frames`, `evaluated_expressions` and `variable_table`.
*/
public List setStripResults(java.lang.Boolean stripResults) {
this.stripResults = stripResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the breakpoint to the debuggee.
*
* Create a request for the method "breakpoints.set".
*
* This request holds the parameters needed by the clouddebugger server. After setting any optional
* parameters, call the {@link Set#execute()} method to invoke the remote operation.
*
* @param debuggeeId ID of the debuggee where the breakpoint is to be set.
* @param content the {@link com.google.api.services.clouddebugger.v2.model.Breakpoint}
* @return the request
*/
public Set set(java.lang.String debuggeeId, com.google.api.services.clouddebugger.v2.model.Breakpoint content) throws java.io.IOException {
Set result = new Set(debuggeeId, content);
initialize(result);
return result;
}
public class Set extends CloudDebuggerRequest {
private static final String REST_PATH = "v2/debugger/debuggees/{debuggeeId}/breakpoints/set";
/**
* Sets the breakpoint to the debuggee.
*
* Create a request for the method "breakpoints.set".
*
* This request holds the parameters needed by the the clouddebugger server. After setting any
* optional parameters, call the {@link Set#execute()} method to invoke the remote operation.
* {@link Set#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param debuggeeId ID of the debuggee where the breakpoint is to be set.
* @param content the {@link com.google.api.services.clouddebugger.v2.model.Breakpoint}
* @since 1.13
*/
protected Set(java.lang.String debuggeeId, com.google.api.services.clouddebugger.v2.model.Breakpoint content) {
super(CloudDebugger.this, "POST", REST_PATH, content, com.google.api.services.clouddebugger.v2.model.SetBreakpointResponse.class);
this.debuggeeId = com.google.api.client.util.Preconditions.checkNotNull(debuggeeId, "Required parameter debuggeeId must be specified.");
}
@Override
public Set set$Xgafv(java.lang.String $Xgafv) {
return (Set) super.set$Xgafv($Xgafv);
}
@Override
public Set setAccessToken(java.lang.String accessToken) {
return (Set) super.setAccessToken(accessToken);
}
@Override
public Set setAlt(java.lang.String alt) {
return (Set) super.setAlt(alt);
}
@Override
public Set setBearerToken(java.lang.String bearerToken) {
return (Set) super.setBearerToken(bearerToken);
}
@Override
public Set setCallback(java.lang.String callback) {
return (Set) super.setCallback(callback);
}
@Override
public Set setFields(java.lang.String fields) {
return (Set) super.setFields(fields);
}
@Override
public Set setKey(java.lang.String key) {
return (Set) super.setKey(key);
}
@Override
public Set setOauthToken(java.lang.String oauthToken) {
return (Set) super.setOauthToken(oauthToken);
}
@Override
public Set setPp(java.lang.Boolean pp) {
return (Set) super.setPp(pp);
}
@Override
public Set setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Set) super.setPrettyPrint(prettyPrint);
}
@Override
public Set setQuotaUser(java.lang.String quotaUser) {
return (Set) super.setQuotaUser(quotaUser);
}
@Override
public Set setUploadType(java.lang.String uploadType) {
return (Set) super.setUploadType(uploadType);
}
@Override
public Set setUploadProtocol(java.lang.String uploadProtocol) {
return (Set) super.setUploadProtocol(uploadProtocol);
}
/** ID of the debuggee where the breakpoint is to be set. */
@com.google.api.client.util.Key
private java.lang.String debuggeeId;
/** ID of the debuggee where the breakpoint is to be set.
*/
public java.lang.String getDebuggeeId() {
return debuggeeId;
}
/** ID of the debuggee where the breakpoint is to be set. */
public Set setDebuggeeId(java.lang.String debuggeeId) {
this.debuggeeId = debuggeeId;
return this;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
@com.google.api.client.util.Key
private java.lang.String clientVersion;
/** The client version making the call. Following: `domain/type/version` (e.g.,
`google.com/intellij/v1`).
*/
public java.lang.String getClientVersion() {
return clientVersion;
}
/**
* The client version making the call. Following: `domain/type/version` (e.g.,
* `google.com/intellij/v1`).
*/
public Set setClientVersion(java.lang.String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
@Override
public Set set(String parameterName, Object value) {
return (Set) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link CloudDebugger}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link CloudDebugger}. */
@Override
public CloudDebugger build() {
return new CloudDebugger(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudDebuggerRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudDebuggerRequestInitializer(
CloudDebuggerRequestInitializer clouddebuggerRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(clouddebuggerRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}