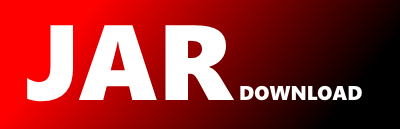
com.google.api.services.clouddebugger.model.Variable Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-08-03 17:34:38 UTC)
* on 2015-08-26 at 02:57:03 UTC
* Modify at your own risk.
*/
package com.google.api.services.clouddebugger.model;
/**
* Represents a variable or an argument possibly of a compound object type. 1. A simple variable
* such as, int x = 5 is represented as: { name: "x", value: "5" } 2. A compound object such as,
* struct T { int m1; int m2; }; T x = { 3, 7 }; is represented as: { name: "x", members { name:
* "m1", value: "3" }, members { name: "m2", value: "7" } } 3. A pointer where the pointee was
* captured such as, T x = { 3, 7 }; T* p = is represented as: { name: "p", value: "0x00500500",
* members { name: "m1", value: "3" }, members { name: "m2", value: "7" } } 4. A pointer where the
* pointee was not captured or is inaccessible such as, T* p = new T; is represented as: { name:
* "p", value: "0x00400400", members { value: "" } } the value text should decribe the reason for
* the missing value. such as , , . note that a null pointer should not have members. 5. An unnamed
* value such as, int* p = new int(7); is represented as, { name: "p", value: "0x00500500", members
* { value: "7" } } 6. An unnamed pointer where the pointee was not captured such as, int* p = new
* int(7); int** pp = is represented as: { name: "pp", value: "0x00500500", members { value:
* "0x00400400", members { value: "" } } } To optimize computation, memory and network traffic,
* variables that repeat in the output multiple times can be stored once in a shared variable table
* and be referenced using the var_index field. The variables stored in the shared table are
* nameless and are essentially a partition of the complete variable. To reconstruct the complete
* variable merge the referencing variable with the referenced variable. When using the shared
* variable table, variables can be represented as: T x = { 3, 7 }; T* p = T& r = x; are
* represented as, { name: "x", var_index: 3 } { name: "p", value "0x00500500", var_index: 3 } {
* name: "r", var_index: 3 } with shared variable table entry #3: { members { name: "m1", value: "3"
* }, members { name: "m2", value: "7" } } Note that the pointer address is stored with the
* referencing variable and not with the referenced variable, to allow the referenced variable to be
* shared between pointer and references.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Cloud Debugger API. For a detailed explanation
* see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Variable extends com.google.api.client.json.GenericJson {
/**
* The members contained or pointed to by the variable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List members;
/**
* The name of the variable, if any.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Status associated with the variable. This field will usually stay unset. A status of a single
* variable only applies to that variable or expression. The rest of breakpoint data still remains
* valid. Variables might be reported in error state even when breakpoint is not in final state.
* The message may refer to variable name with "refers_to" set to "VARIABLE_NAME". Alternatively
* "refers_to" will be set to "VARIABLE_VALUE". In either case variable value and members will be
* unset. Example of error message applied to name: "Invalid expression syntax". Example of
* information message applied to value: "Not captured". Examples of error message applied to
* value: "Malformed string", "Field f not found in class C", "Null pointer dereference".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StatusMessage status;
/**
* The simple value of the variable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* This is a reference to a variable in the shared variable table. More than one variable can
* reference the same variable in the table. The var_index field is an index into variable_table
* in Breakpoint.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer varTableIndex;
/**
* The members contained or pointed to by the variable.
* @return value or {@code null} for none
*/
public java.util.List getMembers() {
return members;
}
/**
* The members contained or pointed to by the variable.
* @param members members or {@code null} for none
*/
public Variable setMembers(java.util.List members) {
this.members = members;
return this;
}
/**
* The name of the variable, if any.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the variable, if any.
* @param name name or {@code null} for none
*/
public Variable setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Status associated with the variable. This field will usually stay unset. A status of a single
* variable only applies to that variable or expression. The rest of breakpoint data still remains
* valid. Variables might be reported in error state even when breakpoint is not in final state.
* The message may refer to variable name with "refers_to" set to "VARIABLE_NAME". Alternatively
* "refers_to" will be set to "VARIABLE_VALUE". In either case variable value and members will be
* unset. Example of error message applied to name: "Invalid expression syntax". Example of
* information message applied to value: "Not captured". Examples of error message applied to
* value: "Malformed string", "Field f not found in class C", "Null pointer dereference".
* @return value or {@code null} for none
*/
public StatusMessage getStatus() {
return status;
}
/**
* Status associated with the variable. This field will usually stay unset. A status of a single
* variable only applies to that variable or expression. The rest of breakpoint data still remains
* valid. Variables might be reported in error state even when breakpoint is not in final state.
* The message may refer to variable name with "refers_to" set to "VARIABLE_NAME". Alternatively
* "refers_to" will be set to "VARIABLE_VALUE". In either case variable value and members will be
* unset. Example of error message applied to name: "Invalid expression syntax". Example of
* information message applied to value: "Not captured". Examples of error message applied to
* value: "Malformed string", "Field f not found in class C", "Null pointer dereference".
* @param status status or {@code null} for none
*/
public Variable setStatus(StatusMessage status) {
this.status = status;
return this;
}
/**
* The simple value of the variable.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* The simple value of the variable.
* @param value value or {@code null} for none
*/
public Variable setValue(java.lang.String value) {
this.value = value;
return this;
}
/**
* This is a reference to a variable in the shared variable table. More than one variable can
* reference the same variable in the table. The var_index field is an index into variable_table
* in Breakpoint.
* @return value or {@code null} for none
*/
public java.lang.Integer getVarTableIndex() {
return varTableIndex;
}
/**
* This is a reference to a variable in the shared variable table. More than one variable can
* reference the same variable in the table. The var_index field is an index into variable_table
* in Breakpoint.
* @param varTableIndex varTableIndex or {@code null} for none
*/
public Variable setVarTableIndex(java.lang.Integer varTableIndex) {
this.varTableIndex = varTableIndex;
return this;
}
@Override
public Variable set(String fieldName, Object value) {
return (Variable) super.set(fieldName, value);
}
@Override
public Variable clone() {
return (Variable) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy