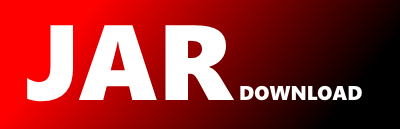
com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1AndroidAttributes Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudidentity.v1.model;
/**
* Resource representing the Android specific attributes of a Device.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Identity API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleAppsCloudidentityDevicesV1AndroidAttributes extends com.google.api.client.json.GenericJson {
/**
* Whether the device passes Android CTS compliance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean ctsProfileMatch;
/**
* Whether applications from unknown sources can be installed on device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enabledUnknownSources;
/**
* Whether any potentially harmful apps were detected on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasPotentiallyHarmfulApps;
/**
* Whether this account is on an owner/primary profile. For phones, only true for owner profiles.
* Android 4+ devices can have secondary or restricted user profiles.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean ownerProfileAccount;
/**
* Ownership privileges on device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ownershipPrivilege;
/**
* Whether device supports Android work profiles. If false, this service will not block access to
* corp data even if an administrator turns on the "Enforce Work Profile" policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean supportsWorkProfile;
/**
* Whether Android verified boot status is GREEN.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean verifiedBoot;
/**
* Whether Google Play Protect Verify Apps is enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean verifyAppsEnabled;
/**
* Whether the device passes Android CTS compliance.
* @return value or {@code null} for none
*/
public java.lang.Boolean getCtsProfileMatch() {
return ctsProfileMatch;
}
/**
* Whether the device passes Android CTS compliance.
* @param ctsProfileMatch ctsProfileMatch or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setCtsProfileMatch(java.lang.Boolean ctsProfileMatch) {
this.ctsProfileMatch = ctsProfileMatch;
return this;
}
/**
* Whether applications from unknown sources can be installed on device.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnabledUnknownSources() {
return enabledUnknownSources;
}
/**
* Whether applications from unknown sources can be installed on device.
* @param enabledUnknownSources enabledUnknownSources or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setEnabledUnknownSources(java.lang.Boolean enabledUnknownSources) {
this.enabledUnknownSources = enabledUnknownSources;
return this;
}
/**
* Whether any potentially harmful apps were detected on the device.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasPotentiallyHarmfulApps() {
return hasPotentiallyHarmfulApps;
}
/**
* Whether any potentially harmful apps were detected on the device.
* @param hasPotentiallyHarmfulApps hasPotentiallyHarmfulApps or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setHasPotentiallyHarmfulApps(java.lang.Boolean hasPotentiallyHarmfulApps) {
this.hasPotentiallyHarmfulApps = hasPotentiallyHarmfulApps;
return this;
}
/**
* Whether this account is on an owner/primary profile. For phones, only true for owner profiles.
* Android 4+ devices can have secondary or restricted user profiles.
* @return value or {@code null} for none
*/
public java.lang.Boolean getOwnerProfileAccount() {
return ownerProfileAccount;
}
/**
* Whether this account is on an owner/primary profile. For phones, only true for owner profiles.
* Android 4+ devices can have secondary or restricted user profiles.
* @param ownerProfileAccount ownerProfileAccount or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setOwnerProfileAccount(java.lang.Boolean ownerProfileAccount) {
this.ownerProfileAccount = ownerProfileAccount;
return this;
}
/**
* Ownership privileges on device.
* @return value or {@code null} for none
*/
public java.lang.String getOwnershipPrivilege() {
return ownershipPrivilege;
}
/**
* Ownership privileges on device.
* @param ownershipPrivilege ownershipPrivilege or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setOwnershipPrivilege(java.lang.String ownershipPrivilege) {
this.ownershipPrivilege = ownershipPrivilege;
return this;
}
/**
* Whether device supports Android work profiles. If false, this service will not block access to
* corp data even if an administrator turns on the "Enforce Work Profile" policy.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSupportsWorkProfile() {
return supportsWorkProfile;
}
/**
* Whether device supports Android work profiles. If false, this service will not block access to
* corp data even if an administrator turns on the "Enforce Work Profile" policy.
* @param supportsWorkProfile supportsWorkProfile or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setSupportsWorkProfile(java.lang.Boolean supportsWorkProfile) {
this.supportsWorkProfile = supportsWorkProfile;
return this;
}
/**
* Whether Android verified boot status is GREEN.
* @return value or {@code null} for none
*/
public java.lang.Boolean getVerifiedBoot() {
return verifiedBoot;
}
/**
* Whether Android verified boot status is GREEN.
* @param verifiedBoot verifiedBoot or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setVerifiedBoot(java.lang.Boolean verifiedBoot) {
this.verifiedBoot = verifiedBoot;
return this;
}
/**
* Whether Google Play Protect Verify Apps is enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getVerifyAppsEnabled() {
return verifyAppsEnabled;
}
/**
* Whether Google Play Protect Verify Apps is enabled.
* @param verifyAppsEnabled verifyAppsEnabled or {@code null} for none
*/
public GoogleAppsCloudidentityDevicesV1AndroidAttributes setVerifyAppsEnabled(java.lang.Boolean verifyAppsEnabled) {
this.verifyAppsEnabled = verifyAppsEnabled;
return this;
}
@Override
public GoogleAppsCloudidentityDevicesV1AndroidAttributes set(String fieldName, Object value) {
return (GoogleAppsCloudidentityDevicesV1AndroidAttributes) super.set(fieldName, value);
}
@Override
public GoogleAppsCloudidentityDevicesV1AndroidAttributes clone() {
return (GoogleAppsCloudidentityDevicesV1AndroidAttributes) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy