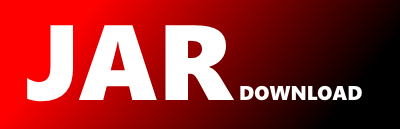
com.google.api.services.cloudidentity.v1.CloudIdentity Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudidentity.v1;
/**
* Service definition for CloudIdentity (v1).
*
*
* API for provisioning and managing identity resources.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudIdentityRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudIdentity extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Identity API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://cloudidentity.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://cloudidentity.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudIdentity(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudIdentity(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Customers collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.Customers.List request = cloudidentity.customers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Customers customers() {
return new Customers();
}
/**
* The "customers" collection of methods.
*/
public class Customers {
/**
* An accessor for creating requests from the Userinvitations collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.Userinvitations.List request = cloudidentity.userinvitations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Userinvitations userinvitations() {
return new Userinvitations();
}
/**
* The "userinvitations" collection of methods.
*/
public class Userinvitations {
/**
* Cancels a UserInvitation that was already sent.
*
* Create a request for the method "userinvitations.cancel".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @param content the {@link com.google.api.services.cloudidentity.v1.model.CancelUserInvitationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.cloudidentity.v1.model.CancelUserInvitationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/userinvitations/[^/]+$");
/**
* Cancels a UserInvitation that was already sent.
*
* Create a request for the method "userinvitations.cancel".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @param content the {@link com.google.api.services.cloudidentity.v1.model.CancelUserInvitationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.cloudidentity.v1.model.CancelUserInvitationRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. `UserInvitation` name in the format
`customers/{customer}/userinvitations/{user_email_address}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Retrieves a UserInvitation resource. **Note:** New consumer accounts with the customer's verified
* domain created within the previous 48 hours will not appear in the result. This delay also
* applies to newly-verified domains.
*
* Create a request for the method "userinvitations.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/userinvitations/[^/]+$");
/**
* Retrieves a UserInvitation resource. **Note:** New consumer accounts with the customer's
* verified domain created within the previous 48 hours will not appear in the result. This delay
* also applies to newly-verified domains.
*
* Create a request for the method "userinvitations.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.UserInvitation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. `UserInvitation` name in the format
`customers/{customer}/userinvitations/{user_email_address}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Verifies whether a user account is eligible to receive a UserInvitation (is an unmanaged
* account). Eligibility is based on the following criteria: * the email address is a consumer
* account and it's the primary email address of the account, and * the domain of the email address
* matches an existing verified Google Workspace or Cloud Identity domain If both conditions are
* met, the user is eligible. **Note:** This method is not supported for Workspace Essentials
* customers.
*
* Create a request for the method "userinvitations.isInvitableUser".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link IsInvitableUser#execute()} method to invoke the remote operation.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @return the request
*/
public IsInvitableUser isInvitableUser(java.lang.String name) throws java.io.IOException {
IsInvitableUser result = new IsInvitableUser(name);
initialize(result);
return result;
}
public class IsInvitableUser extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:isInvitableUser";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/userinvitations/[^/]+$");
/**
* Verifies whether a user account is eligible to receive a UserInvitation (is an unmanaged
* account). Eligibility is based on the following criteria: * the email address is a consumer
* account and it's the primary email address of the account, and * the domain of the email
* address matches an existing verified Google Workspace or Cloud Identity domain If both
* conditions are met, the user is eligible. **Note:** This method is not supported for Workspace
* Essentials customers.
*
* Create a request for the method "userinvitations.isInvitableUser".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link IsInvitableUser#execute()} method to invoke the remote
* operation. {@link IsInvitableUser#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @since 1.13
*/
protected IsInvitableUser(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.IsInvitableUserResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public IsInvitableUser set$Xgafv(java.lang.String $Xgafv) {
return (IsInvitableUser) super.set$Xgafv($Xgafv);
}
@Override
public IsInvitableUser setAccessToken(java.lang.String accessToken) {
return (IsInvitableUser) super.setAccessToken(accessToken);
}
@Override
public IsInvitableUser setAlt(java.lang.String alt) {
return (IsInvitableUser) super.setAlt(alt);
}
@Override
public IsInvitableUser setCallback(java.lang.String callback) {
return (IsInvitableUser) super.setCallback(callback);
}
@Override
public IsInvitableUser setFields(java.lang.String fields) {
return (IsInvitableUser) super.setFields(fields);
}
@Override
public IsInvitableUser setKey(java.lang.String key) {
return (IsInvitableUser) super.setKey(key);
}
@Override
public IsInvitableUser setOauthToken(java.lang.String oauthToken) {
return (IsInvitableUser) super.setOauthToken(oauthToken);
}
@Override
public IsInvitableUser setPrettyPrint(java.lang.Boolean prettyPrint) {
return (IsInvitableUser) super.setPrettyPrint(prettyPrint);
}
@Override
public IsInvitableUser setQuotaUser(java.lang.String quotaUser) {
return (IsInvitableUser) super.setQuotaUser(quotaUser);
}
@Override
public IsInvitableUser setUploadType(java.lang.String uploadType) {
return (IsInvitableUser) super.setUploadType(uploadType);
}
@Override
public IsInvitableUser setUploadProtocol(java.lang.String uploadProtocol) {
return (IsInvitableUser) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. `UserInvitation` name in the format
`customers/{customer}/userinvitations/{user_email_address}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
public IsInvitableUser setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public IsInvitableUser set(String parameterName, Object value) {
return (IsInvitableUser) super.set(parameterName, value);
}
}
/**
* Retrieves a list of UserInvitation resources. **Note:** New consumer accounts with the customer's
* verified domain created within the previous 48 hours will not appear in the result. This delay
* also applies to newly-verified domains.
*
* Create a request for the method "userinvitations.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The customer ID of the Google Workspace or Cloud Identity account the UserInvitation
* resources are associated with.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/userinvitations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Retrieves a list of UserInvitation resources. **Note:** New consumer accounts with the
* customer's verified domain created within the previous 48 hours will not appear in the result.
* This delay also applies to newly-verified domains.
*
* Create a request for the method "userinvitations.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The customer ID of the Google Workspace or Cloud Identity account the UserInvitation
* resources are associated with.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.ListUserInvitationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The customer ID of the Google Workspace or Cloud Identity account the
* UserInvitation resources are associated with.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer ID of the Google Workspace or Cloud Identity account the UserInvitation
resources are associated with.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The customer ID of the Google Workspace or Cloud Identity account the
* UserInvitation resources are associated with.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A query string for filtering `UserInvitation` results by their current state,
* in the format: `"state=='invited'"`.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A query string for filtering `UserInvitation` results by their current state, in the
format: `"state=='invited'"`.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A query string for filtering `UserInvitation` results by their current state,
* in the format: `"state=='invited'"`.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The sort order of the list results. You can sort the results in descending
* order based on either email or last update timestamp but not both, using `order_by="email
* desc"`. Currently, sorting is supported for `update_time asc`, `update_time desc`, `email
* asc`, and `email desc`. If not specified, results will be returned based on `email asc`
* order.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. The sort order of the list results. You can sort the results in descending order based on
either email or last update timestamp but not both, using `order_by="email desc"`. Currently,
sorting is supported for `update_time asc`, `update_time desc`, `email asc`, and `email desc`. If
not specified, results will be returned based on `email asc` order.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. The sort order of the list results. You can sort the results in descending
* order based on either email or last update timestamp but not both, using `order_by="email
* desc"`. Currently, sorting is supported for `update_time asc`, `update_time desc`, `email
* asc`, and `email desc`. If not specified, results will be returned based on `email asc`
* order.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of UserInvitation resources to return. If unspecified, at
* most 100 resources will be returned. The maximum value is 200; values above 200 will be
* set to 200.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of UserInvitation resources to return. If unspecified, at most 100
resources will be returned. The maximum value is 200; values above 200 will be set to 200.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of UserInvitation resources to return. If unspecified, at
* most 100 resources will be returned. The maximum value is 200; values above 200 will be
* set to 200.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListUserInvitations` call. Provide this
* to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListBooks` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListUserInvitations` call. Provide this to
retrieve the subsequent page. When paginating, all other parameters provided to `ListBooks` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListUserInvitations` call. Provide this
* to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListBooks` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sends a UserInvitation to email. If the `UserInvitation` does not exist for this request and it
* is a valid request, the request creates a `UserInvitation`. **Note:** The `get` and `list`
* methods have a 48-hour delay where newly-created consumer accounts will not appear in the
* results. You can still send a `UserInvitation` to those accounts if you know the unmanaged email
* address and IsInvitableUser==True.
*
* Create a request for the method "userinvitations.send".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @param content the {@link com.google.api.services.cloudidentity.v1.model.SendUserInvitationRequest}
* @return the request
*/
public Send send(java.lang.String name, com.google.api.services.cloudidentity.v1.model.SendUserInvitationRequest content) throws java.io.IOException {
Send result = new Send(name, content);
initialize(result);
return result;
}
public class Send extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:send";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/userinvitations/[^/]+$");
/**
* Sends a UserInvitation to email. If the `UserInvitation` does not exist for this request and it
* is a valid request, the request creates a `UserInvitation`. **Note:** The `get` and `list`
* methods have a 48-hour delay where newly-created consumer accounts will not appear in the
* results. You can still send a `UserInvitation` to those accounts if you know the unmanaged
* email address and IsInvitableUser==True.
*
* Create a request for the method "userinvitations.send".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Send#execute()} method to invoke the remote operation.
* {@link Send#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
* @param content the {@link com.google.api.services.cloudidentity.v1.model.SendUserInvitationRequest}
* @since 1.13
*/
protected Send(java.lang.String name, com.google.api.services.cloudidentity.v1.model.SendUserInvitationRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
}
@Override
public Send set$Xgafv(java.lang.String $Xgafv) {
return (Send) super.set$Xgafv($Xgafv);
}
@Override
public Send setAccessToken(java.lang.String accessToken) {
return (Send) super.setAccessToken(accessToken);
}
@Override
public Send setAlt(java.lang.String alt) {
return (Send) super.setAlt(alt);
}
@Override
public Send setCallback(java.lang.String callback) {
return (Send) super.setCallback(callback);
}
@Override
public Send setFields(java.lang.String fields) {
return (Send) super.setFields(fields);
}
@Override
public Send setKey(java.lang.String key) {
return (Send) super.setKey(key);
}
@Override
public Send setOauthToken(java.lang.String oauthToken) {
return (Send) super.setOauthToken(oauthToken);
}
@Override
public Send setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Send) super.setPrettyPrint(prettyPrint);
}
@Override
public Send setQuotaUser(java.lang.String quotaUser) {
return (Send) super.setQuotaUser(quotaUser);
}
@Override
public Send setUploadType(java.lang.String uploadType) {
return (Send) super.setUploadType(uploadType);
}
@Override
public Send setUploadProtocol(java.lang.String uploadProtocol) {
return (Send) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. `UserInvitation` name in the format
`customers/{customer}/userinvitations/{user_email_address}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. `UserInvitation` name in the format
* `customers/{customer}/userinvitations/{user_email_address}`
*/
public Send setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/userinvitations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Send set(String parameterName, Object value) {
return (Send) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Devices collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.Devices.List request = cloudidentity.devices().list(parameters ...)}
*
*
* @return the resource collection
*/
public Devices devices() {
return new Devices();
}
/**
* The "devices" collection of methods.
*/
public class Devices {
/**
* Cancels an unfinished device wipe. This operation can be used to cancel device wipe in the gap
* between the wipe operation returning success and the device being wiped. This operation is
* possible when the device is in a "pending wipe" state. The device enters the "pending wipe" state
* when a wipe device command is issued, but has not yet been sent to the device. The cancel wipe
* will fail if the wipe command has already been issued to the device.
*
* Create a request for the method "devices.cancelWipe".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link CancelWipe#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}`, where device is the unique ID assigned to the Device.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceRequest}
* @return the request
*/
public CancelWipe cancelWipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceRequest content) throws java.io.IOException {
CancelWipe result = new CancelWipe(name, content);
initialize(result);
return result;
}
public class CancelWipe extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:cancelWipe";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+$");
/**
* Cancels an unfinished device wipe. This operation can be used to cancel device wipe in the gap
* between the wipe operation returning success and the device being wiped. This operation is
* possible when the device is in a "pending wipe" state. The device enters the "pending wipe"
* state when a wipe device command is issued, but has not yet been sent to the device. The cancel
* wipe will fail if the wipe command has already been issued to the device.
*
* Create a request for the method "devices.cancelWipe".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link CancelWipe#execute()} method to invoke the remote
* operation. {@link
* CancelWipe#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}`, where device is the unique ID assigned to the Device.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceRequest}
* @since 1.13
*/
protected CancelWipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
}
@Override
public CancelWipe set$Xgafv(java.lang.String $Xgafv) {
return (CancelWipe) super.set$Xgafv($Xgafv);
}
@Override
public CancelWipe setAccessToken(java.lang.String accessToken) {
return (CancelWipe) super.setAccessToken(accessToken);
}
@Override
public CancelWipe setAlt(java.lang.String alt) {
return (CancelWipe) super.setAlt(alt);
}
@Override
public CancelWipe setCallback(java.lang.String callback) {
return (CancelWipe) super.setCallback(callback);
}
@Override
public CancelWipe setFields(java.lang.String fields) {
return (CancelWipe) super.setFields(fields);
}
@Override
public CancelWipe setKey(java.lang.String key) {
return (CancelWipe) super.setKey(key);
}
@Override
public CancelWipe setOauthToken(java.lang.String oauthToken) {
return (CancelWipe) super.setOauthToken(oauthToken);
}
@Override
public CancelWipe setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CancelWipe) super.setPrettyPrint(prettyPrint);
}
@Override
public CancelWipe setQuotaUser(java.lang.String quotaUser) {
return (CancelWipe) super.setQuotaUser(quotaUser);
}
@Override
public CancelWipe setUploadType(java.lang.String uploadType) {
return (CancelWipe) super.setUploadType(uploadType);
}
@Override
public CancelWipe setUploadProtocol(java.lang.String uploadProtocol) {
return (CancelWipe) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}`, where device is the unique ID assigned to the Device.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}`, where device is the unique ID assigned to the Device.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}`, where device is the unique ID assigned to the Device.
*/
public CancelWipe setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
this.name = name;
return this;
}
@Override
public CancelWipe set(String parameterName, Object value) {
return (CancelWipe) super.set(parameterName, value);
}
}
/**
* Creates a device. Only company-owned device may be created. **Note**: This method is available
* only to customers who have one of the following SKUs: Enterprise Standard, Enterprise Plus,
* Enterprise for Education, and Cloud Identity Premium
*
* Create a request for the method "devices.create".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1Device}
* @return the request
*/
public Create create(com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1Device content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends CloudIdentityRequest {
private static final String REST_PATH = "v1/devices";
/**
* Creates a device. Only company-owned device may be created. **Note**: This method is available
* only to customers who have one of the following SKUs: Enterprise Standard, Enterprise Plus,
* Enterprise for Education, and Cloud Identity Premium
*
* Create a request for the method "devices.create".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1Device}
* @since 1.13
*/
protected Create(com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1Device content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`, where
* customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`, where
* customer is the customer to whom the device belongs.
*/
public Create setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified device.
*
* Create a request for the method "devices.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}`, where device is the unique ID assigned to the Device.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+$");
/**
* Deletes the specified device.
*
* Create a request for the method "devices.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}`, where device is the unique ID assigned to the Device.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}`, where device is the unique ID assigned to the Device.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}`, where device is the unique ID assigned to the Device.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}`, where device is the unique ID assigned to the Device.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`, where
* customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`, where
* customer is the customer to whom the device belongs.
*/
public Delete setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in the
* format: `devices/{device}`, where device is the unique ID assigned to the Device.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+$");
/**
* Retrieves the specified device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in the
* format: `devices/{device}`, where device is the unique ID assigned to the Device.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1Device.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in the format: `devices/{device}`, where device is the unique ID assigned to the
* Device.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in the
format: `devices/{device}`, where device is the unique ID assigned to the Device.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in the format: `devices/{device}`, where device is the unique ID assigned to the
* Device.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Customer in the format: `customers/{customer}`, where customer is the customer to whom the
* device belongs. If you're using this API for your own organization, use
* `customers/my_customer`. If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Customer in
the format: `customers/{customer}`, where customer is the customer to whom the device belongs. If
you're using this API for your own organization, use `customers/my_customer`. If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Customer in the format: `customers/{customer}`, where customer is the customer to whom the
* device belongs. If you're using this API for your own organization, use
* `customers/my_customer`. If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
public Get setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists/Searches devices.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/devices";
/**
* Lists/Searches devices.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ListDevicesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer in the format: `customers/{customer}`, where customer is the customer to whom the
* device belongs. If you're using this API for your own organization, use
* `customers/my_customer`. If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer in
the format: `customers/{customer}`, where customer is the customer to whom the device belongs. If
you're using this API for your own organization, use `customers/my_customer`. If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer in the format: `customers/{customer}`, where customer is the customer to whom the
* device belongs. If you're using this API for your own organization, use
* `customers/my_customer`. If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
public List setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
/**
* Optional. Additional restrictions when fetching list of devices. For a list of search
* fields, refer to [Mobile device search fields](https://developers.google.com/admin-
* sdk/directory/v1/search-operators). Multiple search fields are separated by the space
* character.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Additional restrictions when fetching list of devices. For a list of search fields, refer
to [Mobile device search fields](https://developers.google.com/admin-sdk/directory/v1/search-
operators). Multiple search fields are separated by the space character.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Additional restrictions when fetching list of devices. For a list of search
* fields, refer to [Mobile device search fields](https://developers.google.com/admin-
* sdk/directory/v1/search-operators). Multiple search fields are separated by the space
* character.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Order specification for devices in the response. Only one of the following field
* names may be used to specify the order: `create_time`, `last_sync_time`, `model`,
* `os_version`, `device_type` and `serial_number`. `desc` may be specified optionally at the
* end to specify results to be sorted in descending order. Default order is ascending.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Order specification for devices in the response. Only one of the following field names
may be used to specify the order: `create_time`, `last_sync_time`, `model`, `os_version`,
`device_type` and `serial_number`. `desc` may be specified optionally at the end to specify results
to be sorted in descending order. Default order is ascending.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. Order specification for devices in the response. Only one of the following field
* names may be used to specify the order: `create_time`, `last_sync_time`, `model`,
* `os_version`, `device_type` and `serial_number`. `desc` may be specified optionally at the
* end to specify results to be sorted in descending order. Default order is ascending.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of Devices to return. If unspecified, at most 20 Devices will
* be returned. The maximum value is 100; values above 100 will be coerced to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of Devices to return. If unspecified, at most 20 Devices will be
returned. The maximum value is 100; values above 100 will be coerced to 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of Devices to return. If unspecified, at most 20 Devices will
* be returned. The maximum value is 100; values above 100 will be coerced to 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListDevices` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListDevices` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListDevices` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListDevices` must match the
call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListDevices` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListDevices` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Optional. The view to use for the List request. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Optional. The view to use for the List request.
*/
public java.lang.String getView() {
return view;
}
/** Optional. The view to use for the List request. */
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Wipes all data on the specified device.
*
* Create a request for the method "devices.wipe".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Wipe#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceRequest}
* @return the request
*/
public Wipe wipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceRequest content) throws java.io.IOException {
Wipe result = new Wipe(name, content);
initialize(result);
return result;
}
public class Wipe extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:wipe";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+$");
/**
* Wipes all data on the specified device.
*
* Create a request for the method "devices.wipe".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Wipe#execute()} method to invoke the remote operation.
* {@link Wipe#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceRequest}
* @since 1.13
*/
protected Wipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
}
@Override
public Wipe set$Xgafv(java.lang.String $Xgafv) {
return (Wipe) super.set$Xgafv($Xgafv);
}
@Override
public Wipe setAccessToken(java.lang.String accessToken) {
return (Wipe) super.setAccessToken(accessToken);
}
@Override
public Wipe setAlt(java.lang.String alt) {
return (Wipe) super.setAlt(alt);
}
@Override
public Wipe setCallback(java.lang.String callback) {
return (Wipe) super.setCallback(callback);
}
@Override
public Wipe setFields(java.lang.String fields) {
return (Wipe) super.setFields(fields);
}
@Override
public Wipe setKey(java.lang.String key) {
return (Wipe) super.setKey(key);
}
@Override
public Wipe setOauthToken(java.lang.String oauthToken) {
return (Wipe) super.setOauthToken(oauthToken);
}
@Override
public Wipe setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Wipe) super.setPrettyPrint(prettyPrint);
}
@Override
public Wipe setQuotaUser(java.lang.String quotaUser) {
return (Wipe) super.setQuotaUser(quotaUser);
}
@Override
public Wipe setUploadType(java.lang.String uploadType) {
return (Wipe) super.setUploadType(uploadType);
}
@Override
public Wipe setUploadProtocol(java.lang.String uploadProtocol) {
return (Wipe) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique
* ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID assigned to the
Device, and device_user is the unique ID assigned to the User.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique
* ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
public Wipe setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Wipe set(String parameterName, Object value) {
return (Wipe) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the DeviceUsers collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.DeviceUsers.List request = cloudidentity.deviceUsers().list(parameters ...)}
*
*
* @return the resource collection
*/
public DeviceUsers deviceUsers() {
return new DeviceUsers();
}
/**
* The "deviceUsers" collection of methods.
*/
public class DeviceUsers {
/**
* Approves device to access user data.
*
* Create a request for the method "deviceUsers.approve".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Approve#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ApproveDeviceUserRequest}
* @return the request
*/
public Approve approve(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ApproveDeviceUserRequest content) throws java.io.IOException {
Approve result = new Approve(name, content);
initialize(result);
return result;
}
public class Approve extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:approve";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+$");
/**
* Approves device to access user data.
*
* Create a request for the method "deviceUsers.approve".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
* {@link
* Approve#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ApproveDeviceUserRequest}
* @since 1.13
*/
protected Approve(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ApproveDeviceUserRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
}
@Override
public Approve set$Xgafv(java.lang.String $Xgafv) {
return (Approve) super.set$Xgafv($Xgafv);
}
@Override
public Approve setAccessToken(java.lang.String accessToken) {
return (Approve) super.setAccessToken(accessToken);
}
@Override
public Approve setAlt(java.lang.String alt) {
return (Approve) super.setAlt(alt);
}
@Override
public Approve setCallback(java.lang.String callback) {
return (Approve) super.setCallback(callback);
}
@Override
public Approve setFields(java.lang.String fields) {
return (Approve) super.setFields(fields);
}
@Override
public Approve setKey(java.lang.String key) {
return (Approve) super.setKey(key);
}
@Override
public Approve setOauthToken(java.lang.String oauthToken) {
return (Approve) super.setOauthToken(oauthToken);
}
@Override
public Approve setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Approve) super.setPrettyPrint(prettyPrint);
}
@Override
public Approve setQuotaUser(java.lang.String quotaUser) {
return (Approve) super.setQuotaUser(quotaUser);
}
@Override
public Approve setUploadType(java.lang.String uploadType) {
return (Approve) super.setUploadType(uploadType);
}
@Override
public Approve setUploadProtocol(java.lang.String uploadProtocol) {
return (Approve) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID assigned to the
Device, and device_user is the unique ID assigned to the User.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
public Approve setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Approve set(String parameterName, Object value) {
return (Approve) super.set(parameterName, value);
}
}
/**
* Blocks device from accessing user data
*
* Create a request for the method "deviceUsers.block".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Block#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1BlockDeviceUserRequest}
* @return the request
*/
public Block block(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1BlockDeviceUserRequest content) throws java.io.IOException {
Block result = new Block(name, content);
initialize(result);
return result;
}
public class Block extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:block";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+$");
/**
* Blocks device from accessing user data
*
* Create a request for the method "deviceUsers.block".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Block#execute()} method to invoke the remote operation.
* {@link
* Block#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1BlockDeviceUserRequest}
* @since 1.13
*/
protected Block(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1BlockDeviceUserRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
}
@Override
public Block set$Xgafv(java.lang.String $Xgafv) {
return (Block) super.set$Xgafv($Xgafv);
}
@Override
public Block setAccessToken(java.lang.String accessToken) {
return (Block) super.setAccessToken(accessToken);
}
@Override
public Block setAlt(java.lang.String alt) {
return (Block) super.setAlt(alt);
}
@Override
public Block setCallback(java.lang.String callback) {
return (Block) super.setCallback(callback);
}
@Override
public Block setFields(java.lang.String fields) {
return (Block) super.setFields(fields);
}
@Override
public Block setKey(java.lang.String key) {
return (Block) super.setKey(key);
}
@Override
public Block setOauthToken(java.lang.String oauthToken) {
return (Block) super.setOauthToken(oauthToken);
}
@Override
public Block setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Block) super.setPrettyPrint(prettyPrint);
}
@Override
public Block setQuotaUser(java.lang.String quotaUser) {
return (Block) super.setQuotaUser(quotaUser);
}
@Override
public Block setUploadType(java.lang.String uploadType) {
return (Block) super.setUploadType(uploadType);
}
@Override
public Block setUploadProtocol(java.lang.String uploadProtocol) {
return (Block) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID assigned to the
Device, and device_user is the unique ID assigned to the User.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
public Block setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Block set(String parameterName, Object value) {
return (Block) super.set(parameterName, value);
}
}
/**
* Cancels an unfinished user account wipe. This operation can be used to cancel device wipe in the
* gap between the wipe operation returning success and the device being wiped.
*
* Create a request for the method "deviceUsers.cancelWipe".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link CancelWipe#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceUserRequest}
* @return the request
*/
public CancelWipe cancelWipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceUserRequest content) throws java.io.IOException {
CancelWipe result = new CancelWipe(name, content);
initialize(result);
return result;
}
public class CancelWipe extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:cancelWipe";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+$");
/**
* Cancels an unfinished user account wipe. This operation can be used to cancel device wipe in
* the gap between the wipe operation returning success and the device being wiped.
*
* Create a request for the method "deviceUsers.cancelWipe".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link CancelWipe#execute()} method to invoke the remote
* operation. {@link
* CancelWipe#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceUserRequest}
* @since 1.13
*/
protected CancelWipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1CancelWipeDeviceUserRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
}
@Override
public CancelWipe set$Xgafv(java.lang.String $Xgafv) {
return (CancelWipe) super.set$Xgafv($Xgafv);
}
@Override
public CancelWipe setAccessToken(java.lang.String accessToken) {
return (CancelWipe) super.setAccessToken(accessToken);
}
@Override
public CancelWipe setAlt(java.lang.String alt) {
return (CancelWipe) super.setAlt(alt);
}
@Override
public CancelWipe setCallback(java.lang.String callback) {
return (CancelWipe) super.setCallback(callback);
}
@Override
public CancelWipe setFields(java.lang.String fields) {
return (CancelWipe) super.setFields(fields);
}
@Override
public CancelWipe setKey(java.lang.String key) {
return (CancelWipe) super.setKey(key);
}
@Override
public CancelWipe setOauthToken(java.lang.String oauthToken) {
return (CancelWipe) super.setOauthToken(oauthToken);
}
@Override
public CancelWipe setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CancelWipe) super.setPrettyPrint(prettyPrint);
}
@Override
public CancelWipe setQuotaUser(java.lang.String quotaUser) {
return (CancelWipe) super.setQuotaUser(quotaUser);
}
@Override
public CancelWipe setUploadType(java.lang.String uploadType) {
return (CancelWipe) super.setUploadType(uploadType);
}
@Override
public CancelWipe setUploadProtocol(java.lang.String uploadProtocol) {
return (CancelWipe) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID assigned to the
Device, and device_user is the unique ID assigned to the User.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
public CancelWipe setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public CancelWipe set(String parameterName, Object value) {
return (CancelWipe) super.set(parameterName, value);
}
}
/**
* Deletes the specified DeviceUser. This also revokes the user's access to device data.
*
* Create a request for the method "deviceUsers.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+$");
/**
* Deletes the specified DeviceUser. This also revokes the user's access to device data.
*
* Create a request for the method "deviceUsers.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID assigned to the
Device, and device_user is the unique ID assigned to the User.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`,
* where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`,
* where customer is the customer to whom the device belongs.
*/
public Delete setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified DeviceUser
*
* Create a request for the method "deviceUsers.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+$");
/**
* Retrieves the specified DeviceUser
*
* Create a request for the method "deviceUsers.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1DeviceUser.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID assigned to the
Device, and device_user is the unique ID assigned to the User.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`,
* where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`,
* where customer is the customer to whom the device belongs.
*/
public Get setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists/Searches DeviceUsers.
*
* Create a request for the method "deviceUsers.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. To list all DeviceUsers, set this to "devices/-". To list all DeviceUsers owned by a
* device, set this to the resource name of the device. Format: devices/{device}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/deviceUsers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+$");
/**
* Lists/Searches DeviceUsers.
*
* Create a request for the method "deviceUsers.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. To list all DeviceUsers, set this to "devices/-". To list all DeviceUsers owned by a
* device, set this to the resource name of the device. Format: devices/{device}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ListDeviceUsersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^devices/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. To list all DeviceUsers, set this to "devices/-". To list all DeviceUsers owned
* by a device, set this to the resource name of the device. Format: devices/{device}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. To list all DeviceUsers, set this to "devices/-". To list all DeviceUsers owned by a
device, set this to the resource name of the device. Format: devices/{device}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. To list all DeviceUsers, set this to "devices/-". To list all DeviceUsers owned
* by a device, set this to the resource name of the device. Format: devices/{device}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^devices/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`,
* where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use `customers/my_customer`
* If you're using this API to manage another organization, use `customers/{customer}`,
* where customer is the customer to whom the device belongs.
*/
public List setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
/**
* Optional. Additional restrictions when fetching list of devices. For a list of search
* fields, refer to [Mobile device search fields](https://developers.google.com/admin-
* sdk/directory/v1/search-operators). Multiple search fields are separated by the space
* character.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Additional restrictions when fetching list of devices. For a list of search fields, refer
to [Mobile device search fields](https://developers.google.com/admin-sdk/directory/v1/search-
operators). Multiple search fields are separated by the space character.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Additional restrictions when fetching list of devices. For a list of search
* fields, refer to [Mobile device search fields](https://developers.google.com/admin-
* sdk/directory/v1/search-operators). Multiple search fields are separated by the space
* character.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Order specification for devices in the response. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Order specification for devices in the response.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Order specification for devices in the response. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of DeviceUsers to return. If unspecified, at most 5
* DeviceUsers will be returned. The maximum value is 20; values above 20 will be coerced to
* 20.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of DeviceUsers to return. If unspecified, at most 5 DeviceUsers will
be returned. The maximum value is 20; values above 20 will be coerced to 20.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of DeviceUsers to return. If unspecified, at most 5
* DeviceUsers will be returned. The maximum value is 20; values above 20 will be coerced to
* 20.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListDeviceUsers` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListBooks` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListDeviceUsers` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListBooks` must match the
call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListDeviceUsers` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListBooks` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Looks up resource names of the DeviceUsers associated with the caller's credentials, as well as
* the properties provided in the request. This method must be called with end-user credentials with
* the scope: https://www.googleapis.com/auth/cloud-identity.devices.lookup If multiple properties
* are provided, only DeviceUsers having all of these properties are considered as matches - i.e.
* the query behaves like an AND. Different platforms require different amounts of information from
* the caller to ensure that the DeviceUser is uniquely identified. - iOS: No properties need to be
* passed, the caller's credentials are sufficient to identify the corresponding DeviceUser. -
* Android: Specifying the 'android_id' field is required. - Desktop: Specifying the
* 'raw_resource_id' field is required.
*
* Create a request for the method "deviceUsers.lookup".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
*
* @param parent Must be set to "devices/-/deviceUsers" to search across all DeviceUser belonging to the user.
* @return the request
*/
public Lookup lookup(java.lang.String parent) throws java.io.IOException {
Lookup result = new Lookup(parent);
initialize(result);
return result;
}
public class Lookup extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}:lookup";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers$");
/**
* Looks up resource names of the DeviceUsers associated with the caller's credentials, as well as
* the properties provided in the request. This method must be called with end-user credentials
* with the scope: https://www.googleapis.com/auth/cloud-identity.devices.lookup If multiple
* properties are provided, only DeviceUsers having all of these properties are considered as
* matches - i.e. the query behaves like an AND. Different platforms require different amounts of
* information from the caller to ensure that the DeviceUser is uniquely identified. - iOS: No
* properties need to be passed, the caller's credentials are sufficient to identify the
* corresponding DeviceUser. - Android: Specifying the 'android_id' field is required. - Desktop:
* Specifying the 'raw_resource_id' field is required.
*
* Create a request for the method "deviceUsers.lookup".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
* {@link
* Lookup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Must be set to "devices/-/deviceUsers" to search across all DeviceUser belonging to the user.
* @since 1.13
*/
protected Lookup(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1LookupSelfDeviceUsersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^devices/[^/]+/deviceUsers$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Lookup set$Xgafv(java.lang.String $Xgafv) {
return (Lookup) super.set$Xgafv($Xgafv);
}
@Override
public Lookup setAccessToken(java.lang.String accessToken) {
return (Lookup) super.setAccessToken(accessToken);
}
@Override
public Lookup setAlt(java.lang.String alt) {
return (Lookup) super.setAlt(alt);
}
@Override
public Lookup setCallback(java.lang.String callback) {
return (Lookup) super.setCallback(callback);
}
@Override
public Lookup setFields(java.lang.String fields) {
return (Lookup) super.setFields(fields);
}
@Override
public Lookup setKey(java.lang.String key) {
return (Lookup) super.setKey(key);
}
@Override
public Lookup setOauthToken(java.lang.String oauthToken) {
return (Lookup) super.setOauthToken(oauthToken);
}
@Override
public Lookup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Lookup) super.setPrettyPrint(prettyPrint);
}
@Override
public Lookup setQuotaUser(java.lang.String quotaUser) {
return (Lookup) super.setQuotaUser(quotaUser);
}
@Override
public Lookup setUploadType(java.lang.String uploadType) {
return (Lookup) super.setUploadType(uploadType);
}
@Override
public Lookup setUploadProtocol(java.lang.String uploadProtocol) {
return (Lookup) super.setUploadProtocol(uploadProtocol);
}
/**
* Must be set to "devices/-/deviceUsers" to search across all DeviceUser belonging to the
* user.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Must be set to "devices/-/deviceUsers" to search across all DeviceUser belonging to the user.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Must be set to "devices/-/deviceUsers" to search across all DeviceUser belonging to the
* user.
*/
public Lookup setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^devices/[^/]+/deviceUsers$");
}
this.parent = parent;
return this;
}
/**
* Android Id returned by [Settings.Secure#ANDROID_ID](https://developer.android.com/referen
* ce/android/provider/Settings.Secure.html#ANDROID_ID).
*/
@com.google.api.client.util.Key
private java.lang.String androidId;
/** Android Id returned by [Settings.Secure#ANDROID_ID](https://developer.android.com/reference/android
/provider/Settings.Secure.html#ANDROID_ID).
*/
public java.lang.String getAndroidId() {
return androidId;
}
/**
* Android Id returned by [Settings.Secure#ANDROID_ID](https://developer.android.com/referen
* ce/android/provider/Settings.Secure.html#ANDROID_ID).
*/
public Lookup setAndroidId(java.lang.String androidId) {
this.androidId = androidId;
return this;
}
/**
* The maximum number of DeviceUsers to return. If unspecified, at most 20 DeviceUsers will
* be returned. The maximum value is 20; values above 20 will be coerced to 20.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of DeviceUsers to return. If unspecified, at most 20 DeviceUsers will be
returned. The maximum value is 20; values above 20 will be coerced to 20.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of DeviceUsers to return. If unspecified, at most 20 DeviceUsers will
* be returned. The maximum value is 20; values above 20 will be coerced to 20.
*/
public Lookup setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `LookupDeviceUsers` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to
* `LookupDeviceUsers` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `LookupDeviceUsers` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `LookupDeviceUsers` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `LookupDeviceUsers` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to
* `LookupDeviceUsers` must match the call that provided the page token.
*/
public Lookup setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Raw Resource Id used by Google Endpoint Verification. If the user is enrolled into Google
* Endpoint Verification, this id will be saved as the 'device_resource_id' field in the
* following platform dependent files. * macOS: ~/.secureConnect/context_aware_config.json *
* Windows: %USERPROFILE%\AppData\Local\Google\Endpoint Verification\accounts.json * Linux:
* ~/.secureConnect/context_aware_config.json
*/
@com.google.api.client.util.Key
private java.lang.String rawResourceId;
/** Raw Resource Id used by Google Endpoint Verification. If the user is enrolled into Google Endpoint
Verification, this id will be saved as the 'device_resource_id' field in the following platform
dependent files. * macOS: ~/.secureConnect/context_aware_config.json * Windows:
%USERPROFILE%\AppData\Local\Google\Endpoint Verification\accounts.json * Linux:
~/.secureConnect/context_aware_config.json
*/
public java.lang.String getRawResourceId() {
return rawResourceId;
}
/**
* Raw Resource Id used by Google Endpoint Verification. If the user is enrolled into Google
* Endpoint Verification, this id will be saved as the 'device_resource_id' field in the
* following platform dependent files. * macOS: ~/.secureConnect/context_aware_config.json *
* Windows: %USERPROFILE%\AppData\Local\Google\Endpoint Verification\accounts.json * Linux:
* ~/.secureConnect/context_aware_config.json
*/
public Lookup setRawResourceId(java.lang.String rawResourceId) {
this.rawResourceId = rawResourceId;
return this;
}
/**
* The user whose DeviceUser's resource name will be fetched. Must be set to 'me' to fetch
* the DeviceUser's resource name for the calling user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user whose DeviceUser's resource name will be fetched. Must be set to 'me' to fetch the
DeviceUser's resource name for the calling user.
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user whose DeviceUser's resource name will be fetched. Must be set to 'me' to fetch
* the DeviceUser's resource name for the calling user.
*/
public Lookup setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Lookup set(String parameterName, Object value) {
return (Lookup) super.set(parameterName, value);
}
}
/**
* Wipes the user's account on a device. Other data on the device that is not associated with the
* user's work account is not affected. For example, if a Gmail app is installed on a device that is
* used for personal and work purposes, and the user is logged in to the Gmail app with their
* personal account as well as their work account, wiping the "deviceUser" by their work
* administrator will not affect their personal account within Gmail or other apps such as Photos.
*
* Create a request for the method "deviceUsers.wipe".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Wipe#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceUserRequest}
* @return the request
*/
public Wipe wipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceUserRequest content) throws java.io.IOException {
Wipe result = new Wipe(name, content);
initialize(result);
return result;
}
public class Wipe extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:wipe";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+$");
/**
* Wipes the user's account on a device. Other data on the device that is not associated with the
* user's work account is not affected. For example, if a Gmail app is installed on a device that
* is used for personal and work purposes, and the user is logged in to the Gmail app with their
* personal account as well as their work account, wiping the "deviceUser" by their work
* administrator will not affect their personal account within Gmail or other apps such as Photos.
*
* Create a request for the method "deviceUsers.wipe".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Wipe#execute()} method to invoke the remote operation.
* {@link Wipe#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
* format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID
* assigned to the Device, and device_user is the unique ID assigned to the User.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceUserRequest}
* @since 1.13
*/
protected Wipe(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1WipeDeviceUserRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
}
@Override
public Wipe set$Xgafv(java.lang.String $Xgafv) {
return (Wipe) super.set$Xgafv($Xgafv);
}
@Override
public Wipe setAccessToken(java.lang.String accessToken) {
return (Wipe) super.setAccessToken(accessToken);
}
@Override
public Wipe setAlt(java.lang.String alt) {
return (Wipe) super.setAlt(alt);
}
@Override
public Wipe setCallback(java.lang.String callback) {
return (Wipe) super.setCallback(callback);
}
@Override
public Wipe setFields(java.lang.String fields) {
return (Wipe) super.setFields(fields);
}
@Override
public Wipe setKey(java.lang.String key) {
return (Wipe) super.setKey(key);
}
@Override
public Wipe setOauthToken(java.lang.String oauthToken) {
return (Wipe) super.setOauthToken(oauthToken);
}
@Override
public Wipe setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Wipe) super.setPrettyPrint(prettyPrint);
}
@Override
public Wipe setQuotaUser(java.lang.String quotaUser) {
return (Wipe) super.setQuotaUser(quotaUser);
}
@Override
public Wipe setUploadType(java.lang.String uploadType) {
return (Wipe) super.setUploadType(uploadType);
}
@Override
public Wipe setUploadProtocol(java.lang.String uploadProtocol) {
return (Wipe) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Device in
format: `devices/{device}/deviceUsers/{device_user}`, where device is the unique ID assigned to the
Device, and device_user is the unique ID assigned to the User.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Device in format: `devices/{device}/deviceUsers/{device_user}`, where device is the
* unique ID assigned to the Device, and device_user is the unique ID assigned to the User.
*/
public Wipe setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Wipe set(String parameterName, Object value) {
return (Wipe) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ClientStates collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.ClientStates.List request = cloudidentity.clientStates().list(parameters ...)}
*
*
* @return the resource collection
*/
public ClientStates clientStates() {
return new ClientStates();
}
/**
* The "clientStates" collection of methods.
*/
public class ClientStates {
/**
* Gets the client state for the device user
*
* Create a request for the method "clientStates.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the ClientState in
* format: `devices/{device}/deviceUsers/{device_user}/clientStates/{partner}`, where
* `device` is the unique ID assigned to the Device, `device_user` is the unique ID assigned
* to the User and `partner` identifies the partner storing the data. To get the client state
* for devices belonging to your own organization, the `partnerId` is in the format:
* `customerId-*anystring*`. Where the `customerId` is your organization's customer ID and
* `anystring` is any suffix. This suffix is used in setting up Custom Access Levels in
* Context-Aware Access. You may use `my_customer` instead of the customer ID for devices
* managed by your own organization. You may specify `-` in place of the `{device}`, so the
* ClientState resource name can be:
* `devices/-/deviceUsers/{device_user_resource}/clientStates/{partner}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+/clientStates/[^/]+$");
/**
* Gets the client state for the device user
*
* Create a request for the method "clientStates.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the ClientState in
* format: `devices/{device}/deviceUsers/{device_user}/clientStates/{partner}`, where
* `device` is the unique ID assigned to the Device, `device_user` is the unique ID assigned
* to the User and `partner` identifies the partner storing the data. To get the client state
* for devices belonging to your own organization, the `partnerId` is in the format:
* `customerId-*anystring*`. Where the `customerId` is your organization's customer ID and
* `anystring` is any suffix. This suffix is used in setting up Custom Access Levels in
* Context-Aware Access. You may use `my_customer` instead of the customer ID for devices
* managed by your own organization. You may specify `-` in place of the `{device}`, so the
* ClientState resource name can be:
* `devices/-/deviceUsers/{device_user_resource}/clientStates/{partner}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ClientState.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+/clientStates/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* ClientState in format:
* `devices/{device}/deviceUsers/{device_user}/clientStates/{partner}`, where `device` is
* the unique ID assigned to the Device, `device_user` is the unique ID assigned to the
* User and `partner` identifies the partner storing the data. To get the client state for
* devices belonging to your own organization, the `partnerId` is in the format:
* `customerId-*anystring*`. Where the `customerId` is your organization's customer ID and
* `anystring` is any suffix. This suffix is used in setting up Custom Access Levels in
* Context-Aware Access. You may use `my_customer` instead of the customer ID for devices
* managed by your own organization. You may specify `-` in place of the `{device}`, so
* the ClientState resource name can be:
* `devices/-/deviceUsers/{device_user_resource}/clientStates/{partner}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the ClientState
in format: `devices/{device}/deviceUsers/{device_user}/clientStates/{partner}`, where `device` is
the unique ID assigned to the Device, `device_user` is the unique ID assigned to the User and
`partner` identifies the partner storing the data. To get the client state for devices belonging to
your own organization, the `partnerId` is in the format: `customerId-*anystring*`. Where the
`customerId` is your organization's customer ID and `anystring` is any suffix. This suffix is used
in setting up Custom Access Levels in Context-Aware Access. You may use `my_customer` instead of
the customer ID for devices managed by your own organization. You may specify `-` in place of the
`{device}`, so the ClientState resource name can be:
`devices/-/deviceUsers/{device_user_resource}/clientStates/{partner}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* ClientState in format:
* `devices/{device}/deviceUsers/{device_user}/clientStates/{partner}`, where `device` is
* the unique ID assigned to the Device, `device_user` is the unique ID assigned to the
* User and `partner` identifies the partner storing the data. To get the client state for
* devices belonging to your own organization, the `partnerId` is in the format:
* `customerId-*anystring*`. Where the `customerId` is your organization's customer ID and
* `anystring` is any suffix. This suffix is used in setting up Custom Access Levels in
* Context-Aware Access. You may use `my_customer` instead of the customer ID for devices
* managed by your own organization. You may specify `-` in place of the `{device}`, so
* the ClientState resource name can be:
* `devices/-/deviceUsers/{device_user_resource}/clientStates/{partner}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+/clientStates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use
* `customers/my_customer` If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use
* `customers/my_customer` If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
public Get setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the client states for the given search query.
*
* Create a request for the method "clientStates.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. To list all ClientStates, set this to "devices/-/deviceUsers/-". To list all ClientStates
* owned by a DeviceUser, set this to the resource name of the DeviceUser. Format:
* devices/{device}/deviceUsers/{deviceUser}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/clientStates";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+$");
/**
* Lists the client states for the given search query.
*
* Create a request for the method "clientStates.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. To list all ClientStates, set this to "devices/-/deviceUsers/-". To list all ClientStates
* owned by a DeviceUser, set this to the resource name of the DeviceUser. Format:
* devices/{device}/deviceUsers/{deviceUser}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ListClientStatesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. To list all ClientStates, set this to "devices/-/deviceUsers/-". To list all
* ClientStates owned by a DeviceUser, set this to the resource name of the DeviceUser.
* Format: devices/{device}/deviceUsers/{deviceUser}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. To list all ClientStates, set this to "devices/-/deviceUsers/-". To list all ClientStates
owned by a DeviceUser, set this to the resource name of the DeviceUser. Format:
devices/{device}/deviceUsers/{deviceUser}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. To list all ClientStates, set this to "devices/-/deviceUsers/-". To list all
* ClientStates owned by a DeviceUser, set this to the resource name of the DeviceUser.
* Format: devices/{device}/deviceUsers/{deviceUser}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use
* `customers/my_customer` If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use
* `customers/my_customer` If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
public List setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
/** Optional. Additional restrictions when fetching list of client states. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Additional restrictions when fetching list of client states.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Additional restrictions when fetching list of client states. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Order specification for client states in the response. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Order specification for client states in the response.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Order specification for client states in the response. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. A page token, received from a previous `ListClientStates` call. Provide this
* to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListClientStates` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListClientStates` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListClientStates` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListClientStates` call. Provide this
* to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListClientStates` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the client state for the device user **Note**: This method is available only to customers
* who have one of the following SKUs: Enterprise Standard, Enterprise Plus, Enterprise for
* Education, and Cloud Identity Premium
*
* Create a request for the method "clientStates.patch".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the ClientState
* in format: `devices/{device}/deviceUsers/{device_user}/clientState/{partner}`, where
* partner corresponds to the partner storing the data. For partners belonging to the
* "BeyondCorp Alliance", this is the partner ID specified to you by Google. For all other
* callers, this is a string of the form: `{customer}-suffix`, where `customer` is your
* customer ID. The *suffix* is any string the caller specifies. This string will be
* displayed verbatim in the administration console. This suffix is used in setting up Custom
* Access Levels in Context-Aware Access. Your organization's customer ID can be obtained
* from the URL: `GET https://www.googleapis.com/admin/directory/v1/customers/my_customer`
* The `id` field in the response contains the customer ID starting with the letter 'C'. The
* customer ID to be used in this API is the string after the letter 'C' (not including 'C')
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ClientState}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ClientState content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^devices/[^/]+/deviceUsers/[^/]+/clientStates/[^/]+$");
/**
* Updates the client state for the device user **Note**: This method is available only to
* customers who have one of the following SKUs: Enterprise Standard, Enterprise Plus, Enterprise
* for Education, and Cloud Identity Premium
*
* Create a request for the method "clientStates.patch".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the ClientState
* in format: `devices/{device}/deviceUsers/{device_user}/clientState/{partner}`, where
* partner corresponds to the partner storing the data. For partners belonging to the
* "BeyondCorp Alliance", this is the partner ID specified to you by Google. For all other
* callers, this is a string of the form: `{customer}-suffix`, where `customer` is your
* customer ID. The *suffix* is any string the caller specifies. This string will be
* displayed verbatim in the administration console. This suffix is used in setting up Custom
* Access Levels in Context-Aware Access. Your organization's customer ID can be obtained
* from the URL: `GET https://www.googleapis.com/admin/directory/v1/customers/my_customer`
* The `id` field in the response contains the customer ID starting with the letter 'C'. The
* customer ID to be used in this API is the string after the letter 'C' (not including 'C')
* @param content the {@link com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ClientState}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.GoogleAppsCloudidentityDevicesV1ClientState content) {
super(CloudIdentity.this, "PATCH", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+/clientStates/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of
* the ClientState in format:
* `devices/{device}/deviceUsers/{device_user}/clientState/{partner}`, where partner
* corresponds to the partner storing the data. For partners belonging to the "BeyondCorp
* Alliance", this is the partner ID specified to you by Google. For all other callers,
* this is a string of the form: `{customer}-suffix`, where `customer` is your customer
* ID. The *suffix* is any string the caller specifies. This string will be displayed
* verbatim in the administration console. This suffix is used in setting up Custom Access
* Levels in Context-Aware Access. Your organization's customer ID can be obtained from
* the URL: `GET https://www.googleapis.com/admin/directory/v1/customers/my_customer` The
* `id` field in the response contains the customer ID starting with the letter 'C'. The
* customer ID to be used in this API is the string after the letter 'C' (not including
* 'C')
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
ClientState in format: `devices/{device}/deviceUsers/{device_user}/clientState/{partner}`, where
partner corresponds to the partner storing the data. For partners belonging to the "BeyondCorp
Alliance", this is the partner ID specified to you by Google. For all other callers, this is a
string of the form: `{customer}-suffix`, where `customer` is your customer ID. The *suffix* is any
string the caller specifies. This string will be displayed verbatim in the administration console.
This suffix is used in setting up Custom Access Levels in Context-Aware Access. Your organization's
customer ID can be obtained from the URL: `GET
https://www.googleapis.com/admin/directory/v1/customers/my_customer` The `id` field in the response
contains the customer ID starting with the letter 'C'. The customer ID to be used in this API is
the string after the letter 'C' (not including 'C')
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of
* the ClientState in format:
* `devices/{device}/deviceUsers/{device_user}/clientState/{partner}`, where partner
* corresponds to the partner storing the data. For partners belonging to the "BeyondCorp
* Alliance", this is the partner ID specified to you by Google. For all other callers,
* this is a string of the form: `{customer}-suffix`, where `customer` is your customer
* ID. The *suffix* is any string the caller specifies. This string will be displayed
* verbatim in the administration console. This suffix is used in setting up Custom Access
* Levels in Context-Aware Access. Your organization's customer ID can be obtained from
* the URL: `GET https://www.googleapis.com/admin/directory/v1/customers/my_customer` The
* `id` field in the response contains the customer ID starting with the letter 'C'. The
* customer ID to be used in this API is the string after the letter 'C' (not including
* 'C')
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^devices/[^/]+/deviceUsers/[^/]+/clientStates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use
* `customers/my_customer` If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
@com.google.api.client.util.Key
private java.lang.String customer;
/** Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the customer. If
you're using this API for your own organization, use `customers/my_customer` If you're using this
API to manage another organization, use `customers/{customer}`, where customer is the customer to
whom the device belongs.
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Optional. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* customer. If you're using this API for your own organization, use
* `customers/my_customer` If you're using this API to manage another organization, use
* `customers/{customer}`, where customer is the customer to whom the device belongs.
*/
public Patch setCustomer(java.lang.String customer) {
this.customer = customer;
return this;
}
/**
* Optional. Comma-separated list of fully qualified names of fields to be updated. If not
* specified, all updatable fields in ClientState are updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Comma-separated list of fully qualified names of fields to be updated. If not specified,
all updatable fields in ClientState are updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Comma-separated list of fully qualified names of fields to be updated. If not
* specified, all updatable fields in ClientState are updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Groups collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.Groups.List request = cloudidentity.groups().list(parameters ...)}
*
*
* @return the resource collection
*/
public Groups groups() {
return new Groups();
}
/**
* The "groups" collection of methods.
*/
public class Groups {
/**
* Creates a Group.
*
* Create a request for the method "groups.create".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.Group}
* @return the request
*/
public Create create(com.google.api.services.cloudidentity.v1.model.Group content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends CloudIdentityRequest {
private static final String REST_PATH = "v1/groups";
/**
* Creates a Group.
*
* Create a request for the method "groups.create".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.Group}
* @since 1.13
*/
protected Create(com.google.api.services.cloudidentity.v1.model.Group content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Optional. The initial configuration option for the `Group`. */
@com.google.api.client.util.Key
private java.lang.String initialGroupConfig;
/** Optional. The initial configuration option for the `Group`.
*/
public java.lang.String getInitialGroupConfig() {
return initialGroupConfig;
}
/** Optional. The initial configuration option for the `Group`. */
public Create setInitialGroupConfig(java.lang.String initialGroupConfig) {
this.initialGroupConfig = initialGroupConfig;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `Group`.
*
* Create a request for the method "groups.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group` to
* retrieve. Must be of the form `groups/{group}`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Deletes a `Group`.
*
* Create a request for the method "groups.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group` to
* retrieve. Must be of the form `groups/{group}`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve. Must be of the form `groups/{group}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group`
to retrieve. Must be of the form `groups/{group}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve. Must be of the form `groups/{group}`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `Group`.
*
* Create a request for the method "groups.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group` to
* retrieve. Must be of the form `groups/{group}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Retrieves a `Group`.
*
* Create a request for the method "groups.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group` to
* retrieve. Must be of the form `groups/{group}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Group.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve. Must be of the form `groups/{group}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group`
to retrieve. Must be of the form `groups/{group}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve. Must be of the form `groups/{group}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Get Security Settings
*
* Create a request for the method "groups.getSecuritySettings".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link GetSecuritySettings#execute()} method to invoke the remote operation.
*
* @param name Required. The security settings to retrieve. Format: `groups/{group_id}/securitySettings`
* @return the request
*/
public GetSecuritySettings getSecuritySettings(java.lang.String name) throws java.io.IOException {
GetSecuritySettings result = new GetSecuritySettings(name);
initialize(result);
return result;
}
public class GetSecuritySettings extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/securitySettings$");
/**
* Get Security Settings
*
* Create a request for the method "groups.getSecuritySettings".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link GetSecuritySettings#execute()} method to invoke the remote
* operation. {@link GetSecuritySettings#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The security settings to retrieve. Format: `groups/{group_id}/securitySettings`
* @since 1.13
*/
protected GetSecuritySettings(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.SecuritySettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/securitySettings$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSecuritySettings set$Xgafv(java.lang.String $Xgafv) {
return (GetSecuritySettings) super.set$Xgafv($Xgafv);
}
@Override
public GetSecuritySettings setAccessToken(java.lang.String accessToken) {
return (GetSecuritySettings) super.setAccessToken(accessToken);
}
@Override
public GetSecuritySettings setAlt(java.lang.String alt) {
return (GetSecuritySettings) super.setAlt(alt);
}
@Override
public GetSecuritySettings setCallback(java.lang.String callback) {
return (GetSecuritySettings) super.setCallback(callback);
}
@Override
public GetSecuritySettings setFields(java.lang.String fields) {
return (GetSecuritySettings) super.setFields(fields);
}
@Override
public GetSecuritySettings setKey(java.lang.String key) {
return (GetSecuritySettings) super.setKey(key);
}
@Override
public GetSecuritySettings setOauthToken(java.lang.String oauthToken) {
return (GetSecuritySettings) super.setOauthToken(oauthToken);
}
@Override
public GetSecuritySettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSecuritySettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSecuritySettings setQuotaUser(java.lang.String quotaUser) {
return (GetSecuritySettings) super.setQuotaUser(quotaUser);
}
@Override
public GetSecuritySettings setUploadType(java.lang.String uploadType) {
return (GetSecuritySettings) super.setUploadType(uploadType);
}
@Override
public GetSecuritySettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSecuritySettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The security settings to retrieve. Format: `groups/{group_id}/securitySettings`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The security settings to retrieve. Format: `groups/{group_id}/securitySettings`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The security settings to retrieve. Format: `groups/{group_id}/securitySettings`
*/
public GetSecuritySettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/securitySettings$");
}
this.name = name;
return this;
}
/**
* Field-level read mask of which fields to return. "*" returns all fields. If not specified,
* all fields will be returned. May only contain the following field: `member_restriction`.
*/
@com.google.api.client.util.Key
private String readMask;
/** Field-level read mask of which fields to return. "*" returns all fields. If not specified, all
fields will be returned. May only contain the following field: `member_restriction`.
*/
public String getReadMask() {
return readMask;
}
/**
* Field-level read mask of which fields to return. "*" returns all fields. If not specified,
* all fields will be returned. May only contain the following field: `member_restriction`.
*/
public GetSecuritySettings setReadMask(String readMask) {
this.readMask = readMask;
return this;
}
@Override
public GetSecuritySettings set(String parameterName, Object value) {
return (GetSecuritySettings) super.set(parameterName, value);
}
}
/**
* Lists the `Group` resources under a customer or namespace.
*
* Create a request for the method "groups.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/groups";
/**
* Lists the `Group` resources under a customer or namespace.
*
* Create a request for the method "groups.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.ListGroupsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of results to return. Note that the number of results returned may be
* less than this value even if there are more available results. To fetch all results,
* clients must continue calling this method repeatedly until the response no longer contains
* a `next_page_token`. If unspecified, defaults to 200 for `View.BASIC` and to 50 for
* `View.FULL`. Must not be greater than 1000 for `View.BASIC` or 500 for `View.FULL`.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. Note that the number of results returned may be less than
this value even if there are more available results. To fetch all results, clients must continue
calling this method repeatedly until the response no longer contains a `next_page_token`. If
unspecified, defaults to 200 for `View.BASIC` and to 50 for `View.FULL`. Must not be greater than
1000 for `View.BASIC` or 500 for `View.FULL`.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return. Note that the number of results returned may be
* less than this value even if there are more available results. To fetch all results,
* clients must continue calling this method repeatedly until the response no longer contains
* a `next_page_token`. If unspecified, defaults to 200 for `View.BASIC` and to 50 for
* `View.FULL`. Must not be greater than 1000 for `View.BASIC` or 500 for `View.FULL`.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The `next_page_token` value returned from a previous list request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous list request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The `next_page_token` value returned from a previous list request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. The parent resource under which to list all `Group` resources. Must be of the
* form `identitysources/{identity_source}` for external- identity-mapped groups or
* `customers/{customer_id}` for Google Groups. The `customer_id` must begin with "C" (for
* example, 'C046psxkn'). [Find your customer ID.]
* (https://support.google.com/cloudidentity/answer/10070793)
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource under which to list all `Group` resources. Must be of the form
`identitysources/{identity_source}` for external- identity-mapped groups or
`customers/{customer_id}` for Google Groups. The `customer_id` must begin with "C" (for example,
'C046psxkn'). [Find your customer ID.] (https://support.google.com/cloudidentity/answer/10070793)
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource under which to list all `Group` resources. Must be of the
* form `identitysources/{identity_source}` for external- identity-mapped groups or
* `customers/{customer_id}` for Google Groups. The `customer_id` must begin with "C" (for
* example, 'C046psxkn'). [Find your customer ID.]
* (https://support.google.com/cloudidentity/answer/10070793)
*/
public List setParent(java.lang.String parent) {
this.parent = parent;
return this;
}
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`. */
@com.google.api.client.util.Key
private java.lang.String view;
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`.
*/
public java.lang.String getView() {
return view;
}
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`. */
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a `Group` by
* its `EntityKey`.
*
* Create a request for the method "groups.lookup".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Lookup lookup() throws java.io.IOException {
Lookup result = new Lookup();
initialize(result);
return result;
}
public class Lookup extends CloudIdentityRequest {
private static final String REST_PATH = "v1/groups:lookup";
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a `Group`
* by its `EntityKey`.
*
* Create a request for the method "groups.lookup".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
* {@link
* Lookup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Lookup() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.LookupGroupNameResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Lookup set$Xgafv(java.lang.String $Xgafv) {
return (Lookup) super.set$Xgafv($Xgafv);
}
@Override
public Lookup setAccessToken(java.lang.String accessToken) {
return (Lookup) super.setAccessToken(accessToken);
}
@Override
public Lookup setAlt(java.lang.String alt) {
return (Lookup) super.setAlt(alt);
}
@Override
public Lookup setCallback(java.lang.String callback) {
return (Lookup) super.setCallback(callback);
}
@Override
public Lookup setFields(java.lang.String fields) {
return (Lookup) super.setFields(fields);
}
@Override
public Lookup setKey(java.lang.String key) {
return (Lookup) super.setKey(key);
}
@Override
public Lookup setOauthToken(java.lang.String oauthToken) {
return (Lookup) super.setOauthToken(oauthToken);
}
@Override
public Lookup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Lookup) super.setPrettyPrint(prettyPrint);
}
@Override
public Lookup setQuotaUser(java.lang.String quotaUser) {
return (Lookup) super.setQuotaUser(quotaUser);
}
@Override
public Lookup setUploadType(java.lang.String uploadType) {
return (Lookup) super.setUploadType(uploadType);
}
@Override
public Lookup setUploadProtocol(java.lang.String uploadProtocol) {
return (Lookup) super.setUploadProtocol(uploadProtocol);
}
/**
* The ID of the entity. For Google-managed entities, the `id` should be the email address of
* an existing group or user. Email addresses need to adhere to [name guidelines for users and
* groups](https://support.google.com/a/answer/9193374). For external-identity-mapped
* entities, the `id` must be a string conforming to the Identity Source's requirements. Must
* be unique within a `namespace`.
*/
@com.google.api.client.util.Key("groupKey.id")
private java.lang.String groupKeyId;
/** The ID of the entity. For Google-managed entities, the `id` should be the email address of an
existing group or user. Email addresses need to adhere to [name guidelines for users and
groups](https://support.google.com/a/answer/9193374). For external-identity-mapped entities, the
`id` must be a string conforming to the Identity Source's requirements. Must be unique within a
`namespace`.
*/
public java.lang.String getGroupKeyId() {
return groupKeyId;
}
/**
* The ID of the entity. For Google-managed entities, the `id` should be the email address of
* an existing group or user. Email addresses need to adhere to [name guidelines for users and
* groups](https://support.google.com/a/answer/9193374). For external-identity-mapped
* entities, the `id` must be a string conforming to the Identity Source's requirements. Must
* be unique within a `namespace`.
*/
public Lookup setGroupKeyId(java.lang.String groupKeyId) {
this.groupKeyId = groupKeyId;
return this;
}
/**
* The namespace in which the entity exists. If not specified, the `EntityKey` represents a
* Google-managed entity such as a Google user or a Google Group. If specified, the
* `EntityKey` represents an external-identity-mapped group. The namespace must correspond to
* an identity source created in Admin Console and must be in the form of
* `identitysources/{identity_source}`.
*/
@com.google.api.client.util.Key("groupKey.namespace")
private java.lang.String groupKeyNamespace;
/** The namespace in which the entity exists. If not specified, the `EntityKey` represents a Google-
managed entity such as a Google user or a Google Group. If specified, the `EntityKey` represents an
external-identity-mapped group. The namespace must correspond to an identity source created in
Admin Console and must be in the form of `identitysources/{identity_source}`.
*/
public java.lang.String getGroupKeyNamespace() {
return groupKeyNamespace;
}
/**
* The namespace in which the entity exists. If not specified, the `EntityKey` represents a
* Google-managed entity such as a Google user or a Google Group. If specified, the
* `EntityKey` represents an external-identity-mapped group. The namespace must correspond to
* an identity source created in Admin Console and must be in the form of
* `identitysources/{identity_source}`.
*/
public Lookup setGroupKeyNamespace(java.lang.String groupKeyNamespace) {
this.groupKeyNamespace = groupKeyNamespace;
return this;
}
@Override
public Lookup set(String parameterName, Object value) {
return (Lookup) super.set(parameterName, value);
}
}
/**
* Updates a `Group`.
*
* Create a request for the method "groups.patch".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group`. Shall be of the form `groups/{group}`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.Group}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.Group content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Updates a `Group`.
*
* Create a request for the method "groups.patch".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group`. Shall be of the form `groups/{group}`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.Group}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.Group content) {
super(CloudIdentity.this, "PATCH", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of
* the `Group`. Shall be of the form `groups/{group}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Group`. Shall be of the form `groups/{group}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of
* the `Group`. Shall be of the form `groups/{group}`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The names of fields to update. May only contain the following field names:
* `display_name`, `description`, `labels`.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The names of fields to update. May only contain the following field names:
`display_name`, `description`, `labels`.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The names of fields to update. May only contain the following field names:
* `display_name`, `description`, `labels`.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Searches for `Group` resources matching a specified query.
*
* Create a request for the method "groups.search".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Search search() throws java.io.IOException {
Search result = new Search();
initialize(result);
return result;
}
public class Search extends CloudIdentityRequest {
private static final String REST_PATH = "v1/groups:search";
/**
* Searches for `Group` resources matching a specified query.
*
* Create a request for the method "groups.search".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Search() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.SearchGroupsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of results to return. Note that the number of results returned may be
* less than this value even if there are more available results. To fetch all results,
* clients must continue calling this method repeatedly until the response no longer contains
* a `next_page_token`. If unspecified, defaults to 200 for `GroupView.BASIC` and 50 for
* `GroupView.FULL`. Must not be greater than 1000 for `GroupView.BASIC` or 500 for
* `GroupView.FULL`.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. Note that the number of results returned may be less than
this value even if there are more available results. To fetch all results, clients must continue
calling this method repeatedly until the response no longer contains a `next_page_token`. If
unspecified, defaults to 200 for `GroupView.BASIC` and 50 for `GroupView.FULL`. Must not be greater
than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return. Note that the number of results returned may be
* less than this value even if there are more available results. To fetch all results,
* clients must continue calling this method repeatedly until the response no longer contains
* a `next_page_token`. If unspecified, defaults to 200 for `GroupView.BASIC` and 50 for
* `GroupView.FULL`. Must not be greater than 1000 for `GroupView.BASIC` or 500 for
* `GroupView.FULL`.
*/
public Search setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The `next_page_token` value returned from a previous search request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous search request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The `next_page_token` value returned from a previous search request, if any. */
public Search setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. The search query. * Must be specified in [Common Expression
* Language](https://opensource.google/projects/cel). * Must contain equality operators on the
* parent, e.g. `parent == 'customers/{customer_id}'`. The `customer_id` must begin with "C"
* (for example, 'C046psxkn'). [Find your customer ID.]
* (https://support.google.com/cloudidentity/answer/10070793) * Can contain optional inclusion
* operators on `labels` such as `'cloudidentity.googleapis.com/groups.discussion_forum' in
* labels`). * Can contain an optional equality operator on `domain_name`. e.g. `domain_name
* == 'examplepetstore.com'` * Can contain optional `startsWith/contains/equality` operators
* on `group_key`, e.g. `group_key.startsWith('dev')`, `group_key.contains('dev'), group_key
* == '[email protected]'` * Can contain optional `startsWith/contains/equality`
* operators on `display_name`, such as `display_name.startsWith('dev')` ,
* `display_name.contains('dev')`, `display_name == 'dev'`
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. The search query. * Must be specified in [Common Expression
Language](https://opensource.google/projects/cel). * Must contain equality operators on the parent,
e.g. `parent == 'customers/{customer_id}'`. The `customer_id` must begin with "C" (for example,
'C046psxkn'). [Find your customer ID.] (https://support.google.com/cloudidentity/answer/10070793) *
Can contain optional inclusion operators on `labels` such as
`'cloudidentity.googleapis.com/groups.discussion_forum' in labels`). * Can contain an optional
equality operator on `domain_name`. e.g. `domain_name == 'examplepetstore.com'` * Can contain
optional `startsWith/contains/equality` operators on `group_key`, e.g.
`group_key.startsWith('dev')`, `group_key.contains('dev'), group_key == '[email protected]'`
* Can contain optional `startsWith/contains/equality` operators on `display_name`, such as
`display_name.startsWith('dev')` , `display_name.contains('dev')`, `display_name == 'dev'`
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. The search query. * Must be specified in [Common Expression
* Language](https://opensource.google/projects/cel). * Must contain equality operators on the
* parent, e.g. `parent == 'customers/{customer_id}'`. The `customer_id` must begin with "C"
* (for example, 'C046psxkn'). [Find your customer ID.]
* (https://support.google.com/cloudidentity/answer/10070793) * Can contain optional inclusion
* operators on `labels` such as `'cloudidentity.googleapis.com/groups.discussion_forum' in
* labels`). * Can contain an optional equality operator on `domain_name`. e.g. `domain_name
* == 'examplepetstore.com'` * Can contain optional `startsWith/contains/equality` operators
* on `group_key`, e.g. `group_key.startsWith('dev')`, `group_key.contains('dev'), group_key
* == '[email protected]'` * Can contain optional `startsWith/contains/equality`
* operators on `display_name`, such as `display_name.startsWith('dev')` ,
* `display_name.contains('dev')`, `display_name == 'dev'`
*/
public Search setQuery(java.lang.String query) {
this.query = query;
return this;
}
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`. */
@com.google.api.client.util.Key
private java.lang.String view;
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`.
*/
public java.lang.String getView() {
return view;
}
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`. */
public Search setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Update Security Settings
*
* Create a request for the method "groups.updateSecuritySettings".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link UpdateSecuritySettings#execute()} method to invoke the remote
* operation.
*
* @param name Output only. The resource name of the security settings. Shall be of the form
* `groups/{group_id}/securitySettings`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.SecuritySettings}
* @return the request
*/
public UpdateSecuritySettings updateSecuritySettings(java.lang.String name, com.google.api.services.cloudidentity.v1.model.SecuritySettings content) throws java.io.IOException {
UpdateSecuritySettings result = new UpdateSecuritySettings(name, content);
initialize(result);
return result;
}
public class UpdateSecuritySettings extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/securitySettings$");
/**
* Update Security Settings
*
* Create a request for the method "groups.updateSecuritySettings".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link UpdateSecuritySettings#execute()} method to invoke the
* remote operation. {@link UpdateSecuritySettings#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Output only. The resource name of the security settings. Shall be of the form
* `groups/{group_id}/securitySettings`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.SecuritySettings}
* @since 1.13
*/
protected UpdateSecuritySettings(java.lang.String name, com.google.api.services.cloudidentity.v1.model.SecuritySettings content) {
super(CloudIdentity.this, "PATCH", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/securitySettings$");
}
}
@Override
public UpdateSecuritySettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateSecuritySettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateSecuritySettings setAccessToken(java.lang.String accessToken) {
return (UpdateSecuritySettings) super.setAccessToken(accessToken);
}
@Override
public UpdateSecuritySettings setAlt(java.lang.String alt) {
return (UpdateSecuritySettings) super.setAlt(alt);
}
@Override
public UpdateSecuritySettings setCallback(java.lang.String callback) {
return (UpdateSecuritySettings) super.setCallback(callback);
}
@Override
public UpdateSecuritySettings setFields(java.lang.String fields) {
return (UpdateSecuritySettings) super.setFields(fields);
}
@Override
public UpdateSecuritySettings setKey(java.lang.String key) {
return (UpdateSecuritySettings) super.setKey(key);
}
@Override
public UpdateSecuritySettings setOauthToken(java.lang.String oauthToken) {
return (UpdateSecuritySettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateSecuritySettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateSecuritySettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateSecuritySettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateSecuritySettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateSecuritySettings setUploadType(java.lang.String uploadType) {
return (UpdateSecuritySettings) super.setUploadType(uploadType);
}
@Override
public UpdateSecuritySettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateSecuritySettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of the security settings. Shall be of the form
* `groups/{group_id}/securitySettings`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of the security settings. Shall be of the form
`groups/{group_id}/securitySettings`.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the security settings. Shall be of the form
* `groups/{group_id}/securitySettings`.
*/
public UpdateSecuritySettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/securitySettings$");
}
this.name = name;
return this;
}
/**
* Required. The fully-qualified names of fields to update. May only contain the following
* field: `member_restriction.query`.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The fully-qualified names of fields to update. May only contain the following field:
`member_restriction.query`.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The fully-qualified names of fields to update. May only contain the following
* field: `member_restriction.query`.
*/
public UpdateSecuritySettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateSecuritySettings set(String parameterName, Object value) {
return (UpdateSecuritySettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Memberships collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.Memberships.List request = cloudidentity.memberships().list(parameters ...)}
*
*
* @return the resource collection
*/
public Memberships memberships() {
return new Memberships();
}
/**
* The "memberships" collection of methods.
*/
public class Memberships {
/**
* Check a potential member for membership in a group. **Note:** This feature is only available to
* Google Workspace Enterprise Standard, Enterprise Plus, and Enterprise for Education; and Cloud
* Identity Premium accounts. If the account of the member is not one of these, a 403
* (PERMISSION_DENIED) HTTP status code will be returned. A member has membership to a group as long
* as there is a single viewable transitive membership between the group and the member. The actor
* must have view permissions to at least one transitive membership between the member and group.
*
* Create a request for the method "memberships.checkTransitiveMembership".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link CheckTransitiveMembership#execute()} method to invoke the remote
* operation.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to check the
* transitive membership in. Format: `groups/{group}`, where `group` is the unique id
* assigned to the Group to which the Membership belongs to.
* @return the request
*/
public CheckTransitiveMembership checkTransitiveMembership(java.lang.String parent) throws java.io.IOException {
CheckTransitiveMembership result = new CheckTransitiveMembership(parent);
initialize(result);
return result;
}
public class CheckTransitiveMembership extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships:checkTransitiveMembership";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Check a potential member for membership in a group. **Note:** This feature is only available to
* Google Workspace Enterprise Standard, Enterprise Plus, and Enterprise for Education; and Cloud
* Identity Premium accounts. If the account of the member is not one of these, a 403
* (PERMISSION_DENIED) HTTP status code will be returned. A member has membership to a group as
* long as there is a single viewable transitive membership between the group and the member. The
* actor must have view permissions to at least one transitive membership between the member and
* group.
*
* Create a request for the method "memberships.checkTransitiveMembership".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link CheckTransitiveMembership#execute()} method to invoke the
* remote operation. {@link CheckTransitiveMembership#initialize(com.google.api.client.googlea
* pis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to check the
* transitive membership in. Format: `groups/{group}`, where `group` is the unique id
* assigned to the Group to which the Membership belongs to.
* @since 1.13
*/
protected CheckTransitiveMembership(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.CheckTransitiveMembershipResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public CheckTransitiveMembership set$Xgafv(java.lang.String $Xgafv) {
return (CheckTransitiveMembership) super.set$Xgafv($Xgafv);
}
@Override
public CheckTransitiveMembership setAccessToken(java.lang.String accessToken) {
return (CheckTransitiveMembership) super.setAccessToken(accessToken);
}
@Override
public CheckTransitiveMembership setAlt(java.lang.String alt) {
return (CheckTransitiveMembership) super.setAlt(alt);
}
@Override
public CheckTransitiveMembership setCallback(java.lang.String callback) {
return (CheckTransitiveMembership) super.setCallback(callback);
}
@Override
public CheckTransitiveMembership setFields(java.lang.String fields) {
return (CheckTransitiveMembership) super.setFields(fields);
}
@Override
public CheckTransitiveMembership setKey(java.lang.String key) {
return (CheckTransitiveMembership) super.setKey(key);
}
@Override
public CheckTransitiveMembership setOauthToken(java.lang.String oauthToken) {
return (CheckTransitiveMembership) super.setOauthToken(oauthToken);
}
@Override
public CheckTransitiveMembership setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CheckTransitiveMembership) super.setPrettyPrint(prettyPrint);
}
@Override
public CheckTransitiveMembership setQuotaUser(java.lang.String quotaUser) {
return (CheckTransitiveMembership) super.setQuotaUser(quotaUser);
}
@Override
public CheckTransitiveMembership setUploadType(java.lang.String uploadType) {
return (CheckTransitiveMembership) super.setUploadType(uploadType);
}
@Override
public CheckTransitiveMembership setUploadProtocol(java.lang.String uploadProtocol) {
return (CheckTransitiveMembership) super.setUploadProtocol(uploadProtocol);
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* check the transitive membership in. Format: `groups/{group}`, where `group` is the unique
* id assigned to the Group to which the Membership belongs to.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/**[ Resource name](https://cloud.google.com/apis/design/resource_names) of the group to check the
[ transitive membership in. Format: `groups/{group}`, where `group` is the unique id assigned to the
[ Group to which the Membership belongs to.
[
*/
public java.lang.String getParent() {
return parent;
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* check the transitive membership in. Format: `groups/{group}`, where `group` is the unique
* id assigned to the Group to which the Membership belongs to.
*/
public CheckTransitiveMembership setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A CEL expression that MUST include member specification. This is a `required`
* field. Certain groups are uniquely identified by both a 'member_key_id' and a
* 'member_key_namespace', which requires an additional query input: 'member_key_namespace'.
* Example query: `member_key_id == 'member_key_id_value'`
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. A CEL expression that MUST include member specification. This is a `required` field.
Certain groups are uniquely identified by both a 'member_key_id' and a 'member_key_namespace',
which requires an additional query input: 'member_key_namespace'. Example query: `member_key_id ==
'member_key_id_value'`
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. A CEL expression that MUST include member specification. This is a `required`
* field. Certain groups are uniquely identified by both a 'member_key_id' and a
* 'member_key_namespace', which requires an additional query input: 'member_key_namespace'.
* Example query: `member_key_id == 'member_key_id_value'`
*/
public CheckTransitiveMembership setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public CheckTransitiveMembership set(String parameterName, Object value) {
return (CheckTransitiveMembership) super.set(parameterName, value);
}
}
/**
* Creates a `Membership`.
*
* Create a request for the method "memberships.create".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent `Group` resource under which to create the `Membership`. Must be of the form
* `groups/{group}`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.Membership}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudidentity.v1.model.Membership content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Creates a `Membership`.
*
* Create a request for the method "memberships.create".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent `Group` resource under which to create the `Membership`. Must be of the form
* `groups/{group}`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.Membership}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudidentity.v1.model.Membership content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent `Group` resource under which to create the `Membership`. Must be of
* the form `groups/{group}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent `Group` resource under which to create the `Membership`. Must be of the form
`groups/{group}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent `Group` resource under which to create the `Membership`. Must be of
* the form `groups/{group}`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `Membership`.
*
* Create a request for the method "memberships.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to delete. Must be of the form `groups/{group}/memberships/{membership}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/memberships/[^/]+$");
/**
* Deletes a `Membership`.
*
* Create a request for the method "memberships.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to delete. Must be of the form `groups/{group}/memberships/{membership}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to delete. Must be of the form `groups/{group}/memberships/{membership}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Membership` to delete. Must be of the form `groups/{group}/memberships/{membership}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to delete. Must be of the form `groups/{group}/memberships/{membership}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `Membership`.
*
* Create a request for the method "memberships.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to retrieve. Must be of the form `groups/{group}/memberships/{membership}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/memberships/[^/]+$");
/**
* Retrieves a `Membership`.
*
* Create a request for the method "memberships.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to retrieve. Must be of the form `groups/{group}/memberships/{membership}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Membership.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to retrieve. Must be of the form `groups/{group}/memberships/{membership}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Membership` to retrieve. Must be of the form `groups/{group}/memberships/{membership}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to retrieve. Must be of the form `groups/{group}/memberships/{membership}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Get a membership graph of just a member or both a member and a group. **Note:** This feature is
* only available to Google Workspace Enterprise Standard, Enterprise Plus, and Enterprise for
* Education; and Cloud Identity Premium accounts. If the account of the member is not one of these,
* a 403 (PERMISSION_DENIED) HTTP status code will be returned. Given a member, the response will
* contain all membership paths from the member. Given both a group and a member, the response will
* contain all membership paths between the group and the member.
*
* Create a request for the method "memberships.getMembershipGraph".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link GetMembershipGraph#execute()} method to invoke the remote operation.
*
* @param parent Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: `groups/{group}`, where `group` is the unique ID
* assigned to the Group to which the Membership belongs to. group can be a wildcard
* collection id "-". When a group is specified, the membership graph will be constrained to
* paths between the member (defined in the query) and the parent. If a wildcard collection
* is provided, all membership paths connected to the member will be returned.
* @return the request
*/
public GetMembershipGraph getMembershipGraph(java.lang.String parent) throws java.io.IOException {
GetMembershipGraph result = new GetMembershipGraph(parent);
initialize(result);
return result;
}
public class GetMembershipGraph extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships:getMembershipGraph";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Get a membership graph of just a member or both a member and a group. **Note:** This feature is
* only available to Google Workspace Enterprise Standard, Enterprise Plus, and Enterprise for
* Education; and Cloud Identity Premium accounts. If the account of the member is not one of
* these, a 403 (PERMISSION_DENIED) HTTP status code will be returned. Given a member, the
* response will contain all membership paths from the member. Given both a group and a member,
* the response will contain all membership paths between the group and the member.
*
* Create a request for the method "memberships.getMembershipGraph".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link GetMembershipGraph#execute()} method to invoke the remote
* operation. {@link GetMembershipGraph#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: `groups/{group}`, where `group` is the unique ID
* assigned to the Group to which the Membership belongs to. group can be a wildcard
* collection id "-". When a group is specified, the membership graph will be constrained to
* paths between the member (defined in the query) and the parent. If a wildcard collection
* is provided, all membership paths connected to the member will be returned.
* @since 1.13
*/
protected GetMembershipGraph(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetMembershipGraph set$Xgafv(java.lang.String $Xgafv) {
return (GetMembershipGraph) super.set$Xgafv($Xgafv);
}
@Override
public GetMembershipGraph setAccessToken(java.lang.String accessToken) {
return (GetMembershipGraph) super.setAccessToken(accessToken);
}
@Override
public GetMembershipGraph setAlt(java.lang.String alt) {
return (GetMembershipGraph) super.setAlt(alt);
}
@Override
public GetMembershipGraph setCallback(java.lang.String callback) {
return (GetMembershipGraph) super.setCallback(callback);
}
@Override
public GetMembershipGraph setFields(java.lang.String fields) {
return (GetMembershipGraph) super.setFields(fields);
}
@Override
public GetMembershipGraph setKey(java.lang.String key) {
return (GetMembershipGraph) super.setKey(key);
}
@Override
public GetMembershipGraph setOauthToken(java.lang.String oauthToken) {
return (GetMembershipGraph) super.setOauthToken(oauthToken);
}
@Override
public GetMembershipGraph setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetMembershipGraph) super.setPrettyPrint(prettyPrint);
}
@Override
public GetMembershipGraph setQuotaUser(java.lang.String quotaUser) {
return (GetMembershipGraph) super.setQuotaUser(quotaUser);
}
@Override
public GetMembershipGraph setUploadType(java.lang.String uploadType) {
return (GetMembershipGraph) super.setUploadType(uploadType);
}
@Override
public GetMembershipGraph setUploadProtocol(java.lang.String uploadProtocol) {
return (GetMembershipGraph) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* group to search transitive memberships in. Format: `groups/{group}`, where `group` is the
* unique ID assigned to the Group to which the Membership belongs to. group can be a
* wildcard collection id "-". When a group is specified, the membership graph will be
* constrained to paths between the member (defined in the query) and the parent. If a
* wildcard collection is provided, all membership paths connected to the member will be
* returned.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
search transitive memberships in. Format: `groups/{group}`, where `group` is the unique ID assigned
to the Group to which the Membership belongs to. group can be a wildcard collection id "-". When a
group is specified, the membership graph will be constrained to paths between the member (defined
in the query) and the parent. If a wildcard collection is provided, all membership paths connected
to the member will be returned.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* group to search transitive memberships in. Format: `groups/{group}`, where `group` is the
* unique ID assigned to the Group to which the Membership belongs to. group can be a
* wildcard collection id "-". When a group is specified, the membership graph will be
* constrained to paths between the member (defined in the query) and the parent. If a
* wildcard collection is provided, all membership paths connected to the member will be
* returned.
*/
public GetMembershipGraph setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A CEL expression that MUST include member specification AND label(s). Certain
* groups are uniquely identified by both a 'member_key_id' and a 'member_key_namespace',
* which requires an additional query input: 'member_key_namespace'. Example query:
* `member_key_id == 'member_key_id_value' && in labels`
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. A CEL expression that MUST include member specification AND label(s). Certain groups are
uniquely identified by both a 'member_key_id' and a 'member_key_namespace', which requires an
additional query input: 'member_key_namespace'. Example query: `member_key_id ==
'member_key_id_value' && in labels`
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. A CEL expression that MUST include member specification AND label(s). Certain
* groups are uniquely identified by both a 'member_key_id' and a 'member_key_namespace',
* which requires an additional query input: 'member_key_namespace'. Example query:
* `member_key_id == 'member_key_id_value' && in labels`
*/
public GetMembershipGraph setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public GetMembershipGraph set(String parameterName, Object value) {
return (GetMembershipGraph) super.set(parameterName, value);
}
}
/**
* Lists the `Membership`s within a `Group`.
*
* Create a request for the method "memberships.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name. Must be of the
* form `groups/{group}`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Lists the `Membership`s within a `Group`.
*
* Create a request for the method "memberships.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name. Must be of the
* form `groups/{group}`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.ListMembershipsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name. Must
* be of the form `groups/{group}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent `Group` resource under which to lookup the `Membership` name. Must be of the
form `groups/{group}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name. Must
* be of the form `groups/{group}`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of results to return. Note that the number of results returned may be
* less than this value even if there are more available results. To fetch all results,
* clients must continue calling this method repeatedly until the response no longer
* contains a `next_page_token`. If unspecified, defaults to 200 for `GroupView.BASIC` and
* to 50 for `GroupView.FULL`. Must not be greater than 1000 for `GroupView.BASIC` or 500
* for `GroupView.FULL`.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. Note that the number of results returned may be less than
this value even if there are more available results. To fetch all results, clients must continue
calling this method repeatedly until the response no longer contains a `next_page_token`. If
unspecified, defaults to 200 for `GroupView.BASIC` and to 50 for `GroupView.FULL`. Must not be
greater than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return. Note that the number of results returned may be
* less than this value even if there are more available results. To fetch all results,
* clients must continue calling this method repeatedly until the response no longer
* contains a `next_page_token`. If unspecified, defaults to 200 for `GroupView.BASIC` and
* to 50 for `GroupView.FULL`. Must not be greater than 1000 for `GroupView.BASIC` or 500
* for `GroupView.FULL`.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The `next_page_token` value returned from a previous search request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous search request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The `next_page_token` value returned from a previous search request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`. */
@com.google.api.client.util.Key
private java.lang.String view;
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`.
*/
public java.lang.String getView() {
return view;
}
/** The level of detail to be returned. If unspecified, defaults to `View.BASIC`. */
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a
* `Membership` by its `EntityKey`.
*
* Create a request for the method "memberships.lookup".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name. Must be of the
* form `groups/{group}`.
* @return the request
*/
public Lookup lookup(java.lang.String parent) throws java.io.IOException {
Lookup result = new Lookup(parent);
initialize(result);
return result;
}
public class Lookup extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships:lookup";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a
* `Membership` by its `EntityKey`.
*
* Create a request for the method "memberships.lookup".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
* {@link
* Lookup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name. Must be of the
* form `groups/{group}`.
* @since 1.13
*/
protected Lookup(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.LookupMembershipNameResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Lookup set$Xgafv(java.lang.String $Xgafv) {
return (Lookup) super.set$Xgafv($Xgafv);
}
@Override
public Lookup setAccessToken(java.lang.String accessToken) {
return (Lookup) super.setAccessToken(accessToken);
}
@Override
public Lookup setAlt(java.lang.String alt) {
return (Lookup) super.setAlt(alt);
}
@Override
public Lookup setCallback(java.lang.String callback) {
return (Lookup) super.setCallback(callback);
}
@Override
public Lookup setFields(java.lang.String fields) {
return (Lookup) super.setFields(fields);
}
@Override
public Lookup setKey(java.lang.String key) {
return (Lookup) super.setKey(key);
}
@Override
public Lookup setOauthToken(java.lang.String oauthToken) {
return (Lookup) super.setOauthToken(oauthToken);
}
@Override
public Lookup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Lookup) super.setPrettyPrint(prettyPrint);
}
@Override
public Lookup setQuotaUser(java.lang.String quotaUser) {
return (Lookup) super.setQuotaUser(quotaUser);
}
@Override
public Lookup setUploadType(java.lang.String uploadType) {
return (Lookup) super.setUploadType(uploadType);
}
@Override
public Lookup setUploadProtocol(java.lang.String uploadProtocol) {
return (Lookup) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name. Must
* be of the form `groups/{group}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent `Group` resource under which to lookup the `Membership` name. Must be of the
form `groups/{group}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name. Must
* be of the form `groups/{group}`.
*/
public Lookup setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The ID of the entity. For Google-managed entities, the `id` should be the email address
* of an existing group or user. Email addresses need to adhere to [name guidelines for
* users and groups](https://support.google.com/a/answer/9193374). For external-identity-
* mapped entities, the `id` must be a string conforming to the Identity Source's
* requirements. Must be unique within a `namespace`.
*/
@com.google.api.client.util.Key("memberKey.id")
private java.lang.String memberKeyId;
/** The ID of the entity. For Google-managed entities, the `id` should be the email address of an
existing group or user. Email addresses need to adhere to [name guidelines for users and
groups](https://support.google.com/a/answer/9193374). For external-identity-mapped entities, the
`id` must be a string conforming to the Identity Source's requirements. Must be unique within a
`namespace`.
*/
public java.lang.String getMemberKeyId() {
return memberKeyId;
}
/**
* The ID of the entity. For Google-managed entities, the `id` should be the email address
* of an existing group or user. Email addresses need to adhere to [name guidelines for
* users and groups](https://support.google.com/a/answer/9193374). For external-identity-
* mapped entities, the `id` must be a string conforming to the Identity Source's
* requirements. Must be unique within a `namespace`.
*/
public Lookup setMemberKeyId(java.lang.String memberKeyId) {
this.memberKeyId = memberKeyId;
return this;
}
/**
* The namespace in which the entity exists. If not specified, the `EntityKey` represents a
* Google-managed entity such as a Google user or a Google Group. If specified, the
* `EntityKey` represents an external-identity-mapped group. The namespace must correspond
* to an identity source created in Admin Console and must be in the form of
* `identitysources/{identity_source}`.
*/
@com.google.api.client.util.Key("memberKey.namespace")
private java.lang.String memberKeyNamespace;
/** The namespace in which the entity exists. If not specified, the `EntityKey` represents a Google-
managed entity such as a Google user or a Google Group. If specified, the `EntityKey` represents an
external-identity-mapped group. The namespace must correspond to an identity source created in
Admin Console and must be in the form of `identitysources/{identity_source}`.
*/
public java.lang.String getMemberKeyNamespace() {
return memberKeyNamespace;
}
/**
* The namespace in which the entity exists. If not specified, the `EntityKey` represents a
* Google-managed entity such as a Google user or a Google Group. If specified, the
* `EntityKey` represents an external-identity-mapped group. The namespace must correspond
* to an identity source created in Admin Console and must be in the form of
* `identitysources/{identity_source}`.
*/
public Lookup setMemberKeyNamespace(java.lang.String memberKeyNamespace) {
this.memberKeyNamespace = memberKeyNamespace;
return this;
}
@Override
public Lookup set(String parameterName, Object value) {
return (Lookup) super.set(parameterName, value);
}
}
/**
* Modifies the `MembershipRole`s of a `Membership`.
*
* Create a request for the method "memberships.modifyMembershipRoles".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link ModifyMembershipRoles#execute()} method to invoke the remote
* operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` whose roles are to be modified. Must be of the form
* `groups/{group}/memberships/{membership}`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.ModifyMembershipRolesRequest}
* @return the request
*/
public ModifyMembershipRoles modifyMembershipRoles(java.lang.String name, com.google.api.services.cloudidentity.v1.model.ModifyMembershipRolesRequest content) throws java.io.IOException {
ModifyMembershipRoles result = new ModifyMembershipRoles(name, content);
initialize(result);
return result;
}
public class ModifyMembershipRoles extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}:modifyMembershipRoles";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/memberships/[^/]+$");
/**
* Modifies the `MembershipRole`s of a `Membership`.
*
* Create a request for the method "memberships.modifyMembershipRoles".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link ModifyMembershipRoles#execute()} method to invoke the
* remote operation. {@link ModifyMembershipRoles#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` whose roles are to be modified. Must be of the form
* `groups/{group}/memberships/{membership}`.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.ModifyMembershipRolesRequest}
* @since 1.13
*/
protected ModifyMembershipRoles(java.lang.String name, com.google.api.services.cloudidentity.v1.model.ModifyMembershipRolesRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.ModifyMembershipRolesResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
}
@Override
public ModifyMembershipRoles set$Xgafv(java.lang.String $Xgafv) {
return (ModifyMembershipRoles) super.set$Xgafv($Xgafv);
}
@Override
public ModifyMembershipRoles setAccessToken(java.lang.String accessToken) {
return (ModifyMembershipRoles) super.setAccessToken(accessToken);
}
@Override
public ModifyMembershipRoles setAlt(java.lang.String alt) {
return (ModifyMembershipRoles) super.setAlt(alt);
}
@Override
public ModifyMembershipRoles setCallback(java.lang.String callback) {
return (ModifyMembershipRoles) super.setCallback(callback);
}
@Override
public ModifyMembershipRoles setFields(java.lang.String fields) {
return (ModifyMembershipRoles) super.setFields(fields);
}
@Override
public ModifyMembershipRoles setKey(java.lang.String key) {
return (ModifyMembershipRoles) super.setKey(key);
}
@Override
public ModifyMembershipRoles setOauthToken(java.lang.String oauthToken) {
return (ModifyMembershipRoles) super.setOauthToken(oauthToken);
}
@Override
public ModifyMembershipRoles setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ModifyMembershipRoles) super.setPrettyPrint(prettyPrint);
}
@Override
public ModifyMembershipRoles setQuotaUser(java.lang.String quotaUser) {
return (ModifyMembershipRoles) super.setQuotaUser(quotaUser);
}
@Override
public ModifyMembershipRoles setUploadType(java.lang.String uploadType) {
return (ModifyMembershipRoles) super.setUploadType(uploadType);
}
@Override
public ModifyMembershipRoles setUploadProtocol(java.lang.String uploadProtocol) {
return (ModifyMembershipRoles) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` whose roles are to be modified. Must be of the form
* `groups/{group}/memberships/{membership}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Membership` whose roles are to be modified. Must be of the form
`groups/{group}/memberships/{membership}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` whose roles are to be modified. Must be of the form
* `groups/{group}/memberships/{membership}`.
*/
public ModifyMembershipRoles setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ModifyMembershipRoles set(String parameterName, Object value) {
return (ModifyMembershipRoles) super.set(parameterName, value);
}
}
/**
* Searches direct groups of a member.
*
* Create a request for the method "memberships.searchDirectGroups".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link SearchDirectGroups#execute()} method to invoke the remote operation.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
* transitive memberships in. Format: groups/{group_id}, where group_id is always '-' as this
* API will search across all groups for a given member.
* @return the request
*/
public SearchDirectGroups searchDirectGroups(java.lang.String parent) throws java.io.IOException {
SearchDirectGroups result = new SearchDirectGroups(parent);
initialize(result);
return result;
}
public class SearchDirectGroups extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships:searchDirectGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Searches direct groups of a member.
*
* Create a request for the method "memberships.searchDirectGroups".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link SearchDirectGroups#execute()} method to invoke the remote
* operation. {@link SearchDirectGroups#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
* transitive memberships in. Format: groups/{group_id}, where group_id is always '-' as this
* API will search across all groups for a given member.
* @since 1.13
*/
protected SearchDirectGroups(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.SearchDirectGroupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchDirectGroups set$Xgafv(java.lang.String $Xgafv) {
return (SearchDirectGroups) super.set$Xgafv($Xgafv);
}
@Override
public SearchDirectGroups setAccessToken(java.lang.String accessToken) {
return (SearchDirectGroups) super.setAccessToken(accessToken);
}
@Override
public SearchDirectGroups setAlt(java.lang.String alt) {
return (SearchDirectGroups) super.setAlt(alt);
}
@Override
public SearchDirectGroups setCallback(java.lang.String callback) {
return (SearchDirectGroups) super.setCallback(callback);
}
@Override
public SearchDirectGroups setFields(java.lang.String fields) {
return (SearchDirectGroups) super.setFields(fields);
}
@Override
public SearchDirectGroups setKey(java.lang.String key) {
return (SearchDirectGroups) super.setKey(key);
}
@Override
public SearchDirectGroups setOauthToken(java.lang.String oauthToken) {
return (SearchDirectGroups) super.setOauthToken(oauthToken);
}
@Override
public SearchDirectGroups setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchDirectGroups) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchDirectGroups setQuotaUser(java.lang.String quotaUser) {
return (SearchDirectGroups) super.setQuotaUser(quotaUser);
}
@Override
public SearchDirectGroups setUploadType(java.lang.String uploadType) {
return (SearchDirectGroups) super.setUploadType(uploadType);
}
@Override
public SearchDirectGroups setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchDirectGroups) super.setUploadProtocol(uploadProtocol);
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: groups/{group_id}, where group_id is always '-'
* as this API will search across all groups for a given member.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/**[ Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
[ transitive memberships in. Format: groups/{group_id}, where group_id is always '-' as this API
[ will search across all groups for a given member.
[
*/
public java.lang.String getParent() {
return parent;
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: groups/{group_id}, where group_id is always '-'
* as this API will search across all groups for a given member.
*/
public SearchDirectGroups setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The ordering of membership relation for the display name or email in the response. The
* syntax for this field can be found at
* https://cloud.google.com/apis/design/design_patterns#sorting_order. Example: Sort by the
* ascending display name: order_by="group_name" or order_by="group_name asc". Sort by the
* descending display name: order_by="group_name desc". Sort by the ascending group key:
* order_by="group_key" or order_by="group_key asc". Sort by the descending group key:
* order_by="group_key desc".
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** The ordering of membership relation for the display name or email in the response. The syntax for
this field can be found at https://cloud.google.com/apis/design/design_patterns#sorting_order.
Example: Sort by the ascending display name: order_by="group_name" or order_by="group_name asc".
Sort by the descending display name: order_by="group_name desc". Sort by the ascending group key:
order_by="group_key" or order_by="group_key asc". Sort by the descending group key:
order_by="group_key desc".
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* The ordering of membership relation for the display name or email in the response. The
* syntax for this field can be found at
* https://cloud.google.com/apis/design/design_patterns#sorting_order. Example: Sort by the
* ascending display name: order_by="group_name" or order_by="group_name asc". Sort by the
* descending display name: order_by="group_name desc". Sort by the ascending group key:
* order_by="group_key" or order_by="group_key asc". Sort by the descending group key:
* order_by="group_key desc".
*/
public SearchDirectGroups setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** The default page size is 200 (max 1000). */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The default page size is 200 (max 1000).
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The default page size is 200 (max 1000). */
public SearchDirectGroups setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request, if any */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request, if any
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request, if any */
public SearchDirectGroups setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. A CEL expression that MUST include member specification AND label(s). Users can
* search on label attributes of groups. CONTAINS match ('in') is supported on labels.
* Identity-mapped groups are uniquely identified by both a `member_key_id` and a
* `member_key_namespace`, which requires an additional query input: `member_key_namespace`.
* Example query: `member_key_id == 'member_key_id_value' && 'label_value' in labels`
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. A CEL expression that MUST include member specification AND label(s). Users can search on
label attributes of groups. CONTAINS match ('in') is supported on labels. Identity-mapped groups
are uniquely identified by both a `member_key_id` and a `member_key_namespace`, which requires an
additional query input: `member_key_namespace`. Example query: `member_key_id ==
'member_key_id_value' && 'label_value' in labels`
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. A CEL expression that MUST include member specification AND label(s). Users can
* search on label attributes of groups. CONTAINS match ('in') is supported on labels.
* Identity-mapped groups are uniquely identified by both a `member_key_id` and a
* `member_key_namespace`, which requires an additional query input: `member_key_namespace`.
* Example query: `member_key_id == 'member_key_id_value' && 'label_value' in labels`
*/
public SearchDirectGroups setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public SearchDirectGroups set(String parameterName, Object value) {
return (SearchDirectGroups) super.set(parameterName, value);
}
}
/**
* Search transitive groups of a member. **Note:** This feature is only available to Google
* Workspace Enterprise Standard, Enterprise Plus, and Enterprise for Education; and Cloud Identity
* Premium accounts. If the account of the member is not one of these, a 403 (PERMISSION_DENIED)
* HTTP status code will be returned. A transitive group is any group that has a direct or indirect
* membership to the member. Actor must have view permissions all transitive groups.
*
* Create a request for the method "memberships.searchTransitiveGroups".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link SearchTransitiveGroups#execute()} method to invoke the remote
* operation.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
* transitive memberships in. Format: `groups/{group}`, where `group` is always '-' as this
* API will search across all groups for a given member.
* @return the request
*/
public SearchTransitiveGroups searchTransitiveGroups(java.lang.String parent) throws java.io.IOException {
SearchTransitiveGroups result = new SearchTransitiveGroups(parent);
initialize(result);
return result;
}
public class SearchTransitiveGroups extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships:searchTransitiveGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Search transitive groups of a member. **Note:** This feature is only available to Google
* Workspace Enterprise Standard, Enterprise Plus, and Enterprise for Education; and Cloud
* Identity Premium accounts. If the account of the member is not one of these, a 403
* (PERMISSION_DENIED) HTTP status code will be returned. A transitive group is any group that has
* a direct or indirect membership to the member. Actor must have view permissions all transitive
* groups.
*
* Create a request for the method "memberships.searchTransitiveGroups".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link SearchTransitiveGroups#execute()} method to invoke the
* remote operation. {@link SearchTransitiveGroups#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
* transitive memberships in. Format: `groups/{group}`, where `group` is always '-' as this
* API will search across all groups for a given member.
* @since 1.13
*/
protected SearchTransitiveGroups(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.SearchTransitiveGroupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchTransitiveGroups set$Xgafv(java.lang.String $Xgafv) {
return (SearchTransitiveGroups) super.set$Xgafv($Xgafv);
}
@Override
public SearchTransitiveGroups setAccessToken(java.lang.String accessToken) {
return (SearchTransitiveGroups) super.setAccessToken(accessToken);
}
@Override
public SearchTransitiveGroups setAlt(java.lang.String alt) {
return (SearchTransitiveGroups) super.setAlt(alt);
}
@Override
public SearchTransitiveGroups setCallback(java.lang.String callback) {
return (SearchTransitiveGroups) super.setCallback(callback);
}
@Override
public SearchTransitiveGroups setFields(java.lang.String fields) {
return (SearchTransitiveGroups) super.setFields(fields);
}
@Override
public SearchTransitiveGroups setKey(java.lang.String key) {
return (SearchTransitiveGroups) super.setKey(key);
}
@Override
public SearchTransitiveGroups setOauthToken(java.lang.String oauthToken) {
return (SearchTransitiveGroups) super.setOauthToken(oauthToken);
}
@Override
public SearchTransitiveGroups setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchTransitiveGroups) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchTransitiveGroups setQuotaUser(java.lang.String quotaUser) {
return (SearchTransitiveGroups) super.setQuotaUser(quotaUser);
}
@Override
public SearchTransitiveGroups setUploadType(java.lang.String uploadType) {
return (SearchTransitiveGroups) super.setUploadType(uploadType);
}
@Override
public SearchTransitiveGroups setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchTransitiveGroups) super.setUploadProtocol(uploadProtocol);
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: `groups/{group}`, where `group` is always '-'
* as this API will search across all groups for a given member.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/**[ Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
[ transitive memberships in. Format: `groups/{group}`, where `group` is always '-' as this API will
[ search across all groups for a given member.
[
*/
public java.lang.String getParent() {
return parent;
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: `groups/{group}`, where `group` is always '-'
* as this API will search across all groups for a given member.
*/
public SearchTransitiveGroups setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/** The default page size is 200 (max 1000). */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The default page size is 200 (max 1000).
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The default page size is 200 (max 1000). */
public SearchTransitiveGroups setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request, if any. */
public SearchTransitiveGroups setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. A CEL expression that MUST include member specification AND label(s). This is a
* `required` field. Users can search on label attributes of groups. CONTAINS match ('in')
* is supported on labels. Identity-mapped groups are uniquely identified by both a
* `member_key_id` and a `member_key_namespace`, which requires an additional query input:
* `member_key_namespace`. Example query: `member_key_id == 'member_key_id_value' && in
* labels` Query may optionally contain equality operators on the parent of the group
* restricting the search within a particular customer, e.g. `parent ==
* 'customers/{customer_id}'`. The `customer_id` must begin with "C" (for example,
* 'C046psxkn'). This filtering is only supported for Admins with groups read permissons on
* the input customer. Example query: `member_key_id == 'member_key_id_value' && in labels
* && parent == 'customers/C046psxkn'`
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. A CEL expression that MUST include member specification AND label(s). This is a
`required` field. Users can search on label attributes of groups. CONTAINS match ('in') is
supported on labels. Identity-mapped groups are uniquely identified by both a `member_key_id` and a
`member_key_namespace`, which requires an additional query input: `member_key_namespace`. Example
query: `member_key_id == 'member_key_id_value' && in labels` Query may optionally contain equality
operators on the parent of the group restricting the search within a particular customer, e.g.
`parent == 'customers/{customer_id}'`. The `customer_id` must begin with "C" (for example,
'C046psxkn'). This filtering is only supported for Admins with groups read permissons on the input
customer. Example query: `member_key_id == 'member_key_id_value' && in labels && parent ==
'customers/C046psxkn'`
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. A CEL expression that MUST include member specification AND label(s). This is a
* `required` field. Users can search on label attributes of groups. CONTAINS match ('in')
* is supported on labels. Identity-mapped groups are uniquely identified by both a
* `member_key_id` and a `member_key_namespace`, which requires an additional query input:
* `member_key_namespace`. Example query: `member_key_id == 'member_key_id_value' && in
* labels` Query may optionally contain equality operators on the parent of the group
* restricting the search within a particular customer, e.g. `parent ==
* 'customers/{customer_id}'`. The `customer_id` must begin with "C" (for example,
* 'C046psxkn'). This filtering is only supported for Admins with groups read permissons on
* the input customer. Example query: `member_key_id == 'member_key_id_value' && in labels
* && parent == 'customers/C046psxkn'`
*/
public SearchTransitiveGroups setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public SearchTransitiveGroups set(String parameterName, Object value) {
return (SearchTransitiveGroups) super.set(parameterName, value);
}
}
/**
* Search transitive memberships of a group. **Note:** This feature is only available to Google
* Workspace Enterprise Standard, Enterprise Plus, and Enterprise for Education; and Cloud Identity
* Premium accounts. If the account of the group is not one of these, a 403 (PERMISSION_DENIED) HTTP
* status code will be returned. A transitive membership is any direct or indirect membership of a
* group. Actor must have view permissions to all transitive memberships.
*
* Create a request for the method "memberships.searchTransitiveMemberships".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link SearchTransitiveMemberships#execute()} method to invoke the remote
* operation.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
* transitive memberships in. Format: `groups/{group}`, where `group` is the unique ID
* assigned to the Group.
* @return the request
*/
public SearchTransitiveMemberships searchTransitiveMemberships(java.lang.String parent) throws java.io.IOException {
SearchTransitiveMemberships result = new SearchTransitiveMemberships(parent);
initialize(result);
return result;
}
public class SearchTransitiveMemberships extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/memberships:searchTransitiveMemberships";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Search transitive memberships of a group. **Note:** This feature is only available to Google
* Workspace Enterprise Standard, Enterprise Plus, and Enterprise for Education; and Cloud
* Identity Premium accounts. If the account of the group is not one of these, a 403
* (PERMISSION_DENIED) HTTP status code will be returned. A transitive membership is any direct or
* indirect membership of a group. Actor must have view permissions to all transitive memberships.
*
* Create a request for the method "memberships.searchTransitiveMemberships".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link SearchTransitiveMemberships#execute()} method to invoke
* the remote operation. {@link SearchTransitiveMemberships#initialize(com.google.api.client.g
* oogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param parent [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
* transitive memberships in. Format: `groups/{group}`, where `group` is the unique ID
* assigned to the Group.
* @since 1.13
*/
protected SearchTransitiveMemberships(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.SearchTransitiveMembershipsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchTransitiveMemberships set$Xgafv(java.lang.String $Xgafv) {
return (SearchTransitiveMemberships) super.set$Xgafv($Xgafv);
}
@Override
public SearchTransitiveMemberships setAccessToken(java.lang.String accessToken) {
return (SearchTransitiveMemberships) super.setAccessToken(accessToken);
}
@Override
public SearchTransitiveMemberships setAlt(java.lang.String alt) {
return (SearchTransitiveMemberships) super.setAlt(alt);
}
@Override
public SearchTransitiveMemberships setCallback(java.lang.String callback) {
return (SearchTransitiveMemberships) super.setCallback(callback);
}
@Override
public SearchTransitiveMemberships setFields(java.lang.String fields) {
return (SearchTransitiveMemberships) super.setFields(fields);
}
@Override
public SearchTransitiveMemberships setKey(java.lang.String key) {
return (SearchTransitiveMemberships) super.setKey(key);
}
@Override
public SearchTransitiveMemberships setOauthToken(java.lang.String oauthToken) {
return (SearchTransitiveMemberships) super.setOauthToken(oauthToken);
}
@Override
public SearchTransitiveMemberships setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchTransitiveMemberships) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchTransitiveMemberships setQuotaUser(java.lang.String quotaUser) {
return (SearchTransitiveMemberships) super.setQuotaUser(quotaUser);
}
@Override
public SearchTransitiveMemberships setUploadType(java.lang.String uploadType) {
return (SearchTransitiveMemberships) super.setUploadType(uploadType);
}
@Override
public SearchTransitiveMemberships setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchTransitiveMemberships) super.setUploadProtocol(uploadProtocol);
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: `groups/{group}`, where `group` is the unique
* ID assigned to the Group.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/**[ Resource name](https://cloud.google.com/apis/design/resource_names) of the group to search
[ transitive memberships in. Format: `groups/{group}`, where `group` is the unique ID assigned to
[ the Group.
[
*/
public java.lang.String getParent() {
return parent;
}
/**
* [Resource name](https://cloud.google.com/apis/design/resource_names) of the group to
* search transitive memberships in. Format: `groups/{group}`, where `group` is the unique
* ID assigned to the Group.
*/
public SearchTransitiveMemberships setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/** The default page size is 200 (max 1000). */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The default page size is 200 (max 1000).
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The default page size is 200 (max 1000). */
public SearchTransitiveMemberships setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request, if any. */
public SearchTransitiveMemberships setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public SearchTransitiveMemberships set(String parameterName, Object value) {
return (SearchTransitiveMemberships) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the InboundSamlSsoProfiles collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.InboundSamlSsoProfiles.List request = cloudidentity.inboundSamlSsoProfiles().list(parameters ...)}
*
*
* @return the resource collection
*/
public InboundSamlSsoProfiles inboundSamlSsoProfiles() {
return new InboundSamlSsoProfiles();
}
/**
* The "inboundSamlSsoProfiles" collection of methods.
*/
public class InboundSamlSsoProfiles {
/**
* Creates an InboundSamlSsoProfile for a customer. When the target customer has enabled [Multi-
* party approval for sensitive actions](https://support.google.com/a/answer/13790448), the
* `Operation` in the response will have `"done": false`, it will not have a response, and the
* metadata will have `"state": "awaiting-multi-party-approval"`.
*
* Create a request for the method "inboundSamlSsoProfiles.create".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile}
* @return the request
*/
public Create create(com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends CloudIdentityRequest {
private static final String REST_PATH = "v1/inboundSamlSsoProfiles";
/**
* Creates an InboundSamlSsoProfile for a customer. When the target customer has enabled [Multi-
* party approval for sensitive actions](https://support.google.com/a/answer/13790448), the
* `Operation` in the response will have `"done": false`, it will not have a response, and the
* metadata will have `"state": "awaiting-multi-party-approval"`.
*
* Create a request for the method "inboundSamlSsoProfiles.create".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile}
* @since 1.13
*/
protected Create(com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an InboundSamlSsoProfile.
*
* Create a request for the method "inboundSamlSsoProfiles.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to delete. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSamlSsoProfiles/[^/]+$");
/**
* Deletes an InboundSamlSsoProfile.
*
* Create a request for the method "inboundSamlSsoProfiles.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to delete. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to delete. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
InboundSamlSsoProfile to delete. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to delete. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an InboundSamlSsoProfile.
*
* Create a request for the method "inboundSamlSsoProfiles.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to get. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSamlSsoProfiles/[^/]+$");
/**
* Gets an InboundSamlSsoProfile.
*
* Create a request for the method "inboundSamlSsoProfiles.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to get. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to get. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
InboundSamlSsoProfile to get. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSamlSsoProfile to get. Format: `inboundSamlSsoProfiles/{sso_profile_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists InboundSamlSsoProfiles for a customer.
*
* Create a request for the method "inboundSamlSsoProfiles.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/inboundSamlSsoProfiles";
/**
* Lists InboundSamlSsoProfiles for a customer.
*
* Create a request for the method "inboundSamlSsoProfiles.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.ListInboundSamlSsoProfilesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* A [Common Expression Language](https://github.com/google/cel-spec) expression to filter the
* results. The only supported filter is filtering by customer. For example:
* `customer=="customers/C0123abc"`. Omitting the filter or specifying a filter of
* `customer=="customers/my_customer"` will return the profiles for the customer that the
* caller (authenticated user) belongs to.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A [Common Expression Language](https://github.com/google/cel-spec) expression to filter the
results. The only supported filter is filtering by customer. For example:
`customer=="customers/C0123abc"`. Omitting the filter or specifying a filter of
`customer=="customers/my_customer"` will return the profiles for the customer that the caller
(authenticated user) belongs to.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A [Common Expression Language](https://github.com/google/cel-spec) expression to filter the
* results. The only supported filter is filtering by customer. For example:
* `customer=="customers/C0123abc"`. Omitting the filter or specifying a filter of
* `customer=="customers/my_customer"` will return the profiles for the customer that the
* caller (authenticated user) belongs to.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of InboundSamlSsoProfiles to return. The service may return fewer than
* this value. If omitted (or defaulted to zero) the server will use a sensible default. This
* default may change over time. The maximum allowed value is 100. Requests with page_size
* greater than that will be silently interpreted as having this maximum value.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of InboundSamlSsoProfiles to return. The service may return fewer than this
value. If omitted (or defaulted to zero) the server will use a sensible default. This default may
change over time. The maximum allowed value is 100. Requests with page_size greater than that will
be silently interpreted as having this maximum value.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of InboundSamlSsoProfiles to return. The service may return fewer than
* this value. If omitted (or defaulted to zero) the server will use a sensible default. This
* default may change over time. The maximum allowed value is 100. Requests with page_size
* greater than that will be silently interpreted as having this maximum value.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListInboundSamlSsoProfiles` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListInboundSamlSsoProfiles` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListInboundSamlSsoProfiles` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListInboundSamlSsoProfiles`
must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListInboundSamlSsoProfiles` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListInboundSamlSsoProfiles` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an InboundSamlSsoProfile. When the target customer has enabled [Multi-party approval for
* sensitive actions](https://support.google.com/a/answer/13790448), the `Operation` in the response
* will have `"done": false`, it will not have a response, and the metadata will have `"state":
* "awaiting-multi-party-approval"`.
*
* Create a request for the method "inboundSamlSsoProfiles.patch".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the SAML SSO
* profile.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSamlSsoProfiles/[^/]+$");
/**
* Updates an InboundSamlSsoProfile. When the target customer has enabled [Multi-party approval
* for sensitive actions](https://support.google.com/a/answer/13790448), the `Operation` in the
* response will have `"done": false`, it will not have a response, and the metadata will have
* `"state": "awaiting-multi-party-approval"`.
*
* Create a request for the method "inboundSamlSsoProfiles.patch".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the SAML SSO
* profile.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.InboundSamlSsoProfile content) {
super(CloudIdentity.this, "PATCH", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* SAML SSO profile.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the SAML SSO
profile.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* SAML SSO profile.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
this.name = name;
return this;
}
/** Required. The list of fields to be updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. The list of fields to be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. The list of fields to be updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the IdpCredentials collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.IdpCredentials.List request = cloudidentity.idpCredentials().list(parameters ...)}
*
*
* @return the resource collection
*/
public IdpCredentials idpCredentials() {
return new IdpCredentials();
}
/**
* The "idpCredentials" collection of methods.
*/
public class IdpCredentials {
/**
* Adds an IdpCredential. Up to 2 credentials are allowed. When the target customer has enabled
* [Multi-party approval for sensitive actions](https://support.google.com/a/answer/13790448), the
* `Operation` in the response will have `"done": false`, it will not have a response, and the
* metadata will have `"state": "awaiting-multi-party-approval"`.
*
* Create a request for the method "idpCredentials.add".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Add#execute()} method to invoke the remote operation.
*
* @param parent Required. The InboundSamlSsoProfile that owns the IdpCredential. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
* @param content the {@link com.google.api.services.cloudidentity.v1.model.AddIdpCredentialRequest}
* @return the request
*/
public Add add(java.lang.String parent, com.google.api.services.cloudidentity.v1.model.AddIdpCredentialRequest content) throws java.io.IOException {
Add result = new Add(parent, content);
initialize(result);
return result;
}
public class Add extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/idpCredentials:add";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^inboundSamlSsoProfiles/[^/]+$");
/**
* Adds an IdpCredential. Up to 2 credentials are allowed. When the target customer has enabled
* [Multi-party approval for sensitive actions](https://support.google.com/a/answer/13790448), the
* `Operation` in the response will have `"done": false`, it will not have a response, and the
* metadata will have `"state": "awaiting-multi-party-approval"`.
*
* Create a request for the method "idpCredentials.add".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Add#execute()} method to invoke the remote operation.
* {@link Add#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The InboundSamlSsoProfile that owns the IdpCredential. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
* @param content the {@link com.google.api.services.cloudidentity.v1.model.AddIdpCredentialRequest}
* @since 1.13
*/
protected Add(java.lang.String parent, com.google.api.services.cloudidentity.v1.model.AddIdpCredentialRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
}
@Override
public Add set$Xgafv(java.lang.String $Xgafv) {
return (Add) super.set$Xgafv($Xgafv);
}
@Override
public Add setAccessToken(java.lang.String accessToken) {
return (Add) super.setAccessToken(accessToken);
}
@Override
public Add setAlt(java.lang.String alt) {
return (Add) super.setAlt(alt);
}
@Override
public Add setCallback(java.lang.String callback) {
return (Add) super.setCallback(callback);
}
@Override
public Add setFields(java.lang.String fields) {
return (Add) super.setFields(fields);
}
@Override
public Add setKey(java.lang.String key) {
return (Add) super.setKey(key);
}
@Override
public Add setOauthToken(java.lang.String oauthToken) {
return (Add) super.setOauthToken(oauthToken);
}
@Override
public Add setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Add) super.setPrettyPrint(prettyPrint);
}
@Override
public Add setQuotaUser(java.lang.String quotaUser) {
return (Add) super.setQuotaUser(quotaUser);
}
@Override
public Add setUploadType(java.lang.String uploadType) {
return (Add) super.setUploadType(uploadType);
}
@Override
public Add setUploadProtocol(java.lang.String uploadProtocol) {
return (Add) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The InboundSamlSsoProfile that owns the IdpCredential. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The InboundSamlSsoProfile that owns the IdpCredential. Format:
`inboundSamlSsoProfiles/{sso_profile_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The InboundSamlSsoProfile that owns the IdpCredential. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
*/
public Add setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Add set(String parameterName, Object value) {
return (Add) super.set(parameterName, value);
}
}
/**
* Deletes an IdpCredential.
*
* Create a request for the method "idpCredentials.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to delete. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSamlSsoProfiles/[^/]+/idpCredentials/[^/]+$");
/**
* Deletes an IdpCredential.
*
* Create a request for the method "idpCredentials.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to delete. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+/idpCredentials/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to delete. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
IdpCredential to delete. Format:
`inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to delete. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+/idpCredentials/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an IdpCredential.
*
* Create a request for the method "idpCredentials.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to retrieve. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSamlSsoProfiles/[^/]+/idpCredentials/[^/]+$");
/**
* Gets an IdpCredential.
*
* Create a request for the method "idpCredentials.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to retrieve. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.IdpCredential.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+/idpCredentials/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to retrieve. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
IdpCredential to retrieve. Format:
`inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* IdpCredential to retrieve. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}/idpCredentials/{idp_credential_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+/idpCredentials/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns a list of IdpCredentials in an InboundSamlSsoProfile.
*
* Create a request for the method "idpCredentials.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent, which owns this collection of `IdpCredential`s. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+parent}/idpCredentials";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^inboundSamlSsoProfiles/[^/]+$");
/**
* Returns a list of IdpCredentials in an InboundSamlSsoProfile.
*
* Create a request for the method "idpCredentials.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent, which owns this collection of `IdpCredential`s. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.ListIdpCredentialsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent, which owns this collection of `IdpCredential`s. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent, which owns this collection of `IdpCredential`s. Format:
`inboundSamlSsoProfiles/{sso_profile_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent, which owns this collection of `IdpCredential`s. Format:
* `inboundSamlSsoProfiles/{sso_profile_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^inboundSamlSsoProfiles/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of `IdpCredential`s to return. The service may return fewer than this
* value.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of `IdpCredential`s to return. The service may return fewer than this value.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of `IdpCredential`s to return. The service may return fewer than this
* value.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListIdpCredentials` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListIdpCredentials` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListIdpCredentials` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListIdpCredentials` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListIdpCredentials` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListIdpCredentials` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the InboundSsoAssignments collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.InboundSsoAssignments.List request = cloudidentity.inboundSsoAssignments().list(parameters ...)}
*
*
* @return the resource collection
*/
public InboundSsoAssignments inboundSsoAssignments() {
return new InboundSsoAssignments();
}
/**
* The "inboundSsoAssignments" collection of methods.
*/
public class InboundSsoAssignments {
/**
* Creates an InboundSsoAssignment for users and devices in a `Customer` under a given `Group` or
* `OrgUnit`.
*
* Create a request for the method "inboundSsoAssignments.create".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment}
* @return the request
*/
public Create create(com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends CloudIdentityRequest {
private static final String REST_PATH = "v1/inboundSsoAssignments";
/**
* Creates an InboundSsoAssignment for users and devices in a `Customer` under a given `Group` or
* `OrgUnit`.
*
* Create a request for the method "inboundSsoAssignments.create".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment}
* @since 1.13
*/
protected Create(com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an InboundSsoAssignment. To disable SSO, Create (or Update) an assignment that has
* `sso_mode` == `SSO_OFF`.
*
* Create a request for the method "inboundSsoAssignments.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to delete. Format: `inboundSsoAssignments/{assignment}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSsoAssignments/[^/]+$");
/**
* Deletes an InboundSsoAssignment. To disable SSO, Create (or Update) an assignment that has
* `sso_mode` == `SSO_OFF`.
*
* Create a request for the method "inboundSsoAssignments.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to delete. Format: `inboundSsoAssignments/{assignment}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSsoAssignments/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to delete. Format: `inboundSsoAssignments/{assignment}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
InboundSsoAssignment to delete. Format: `inboundSsoAssignments/{assignment}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to delete. Format: `inboundSsoAssignments/{assignment}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSsoAssignments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an InboundSsoAssignment.
*
* Create a request for the method "inboundSsoAssignments.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to fetch. Format: `inboundSsoAssignments/{assignment}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSsoAssignments/[^/]+$");
/**
* Gets an InboundSsoAssignment.
*
* Create a request for the method "inboundSsoAssignments.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to fetch. Format: `inboundSsoAssignments/{assignment}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSsoAssignments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to fetch. Format: `inboundSsoAssignments/{assignment}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
InboundSsoAssignment to fetch. Format: `inboundSsoAssignments/{assignment}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* InboundSsoAssignment to fetch. Format: `inboundSsoAssignments/{assignment}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSsoAssignments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the InboundSsoAssignments for a `Customer`.
*
* Create a request for the method "inboundSsoAssignments.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1/inboundSsoAssignments";
/**
* Lists the InboundSsoAssignments for a `Customer`.
*
* Create a request for the method "inboundSsoAssignments.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1.model.ListInboundSsoAssignmentsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* A CEL expression to filter the results. The only supported filter is filtering by customer.
* For example: `customer==customers/C0123abc`. Omitting the filter or specifying a filter of
* `customer==customers/my_customer` will return the assignments for the customer that the
* caller (authenticated user) belongs to.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A CEL expression to filter the results. The only supported filter is filtering by customer. For
example: `customer==customers/C0123abc`. Omitting the filter or specifying a filter of
`customer==customers/my_customer` will return the assignments for the customer that the caller
(authenticated user) belongs to.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A CEL expression to filter the results. The only supported filter is filtering by customer.
* For example: `customer==customers/C0123abc`. Omitting the filter or specifying a filter of
* `customer==customers/my_customer` will return the assignments for the customer that the
* caller (authenticated user) belongs to.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of assignments to return. The service may return fewer than this value.
* If omitted (or defaulted to zero) the server will use a sensible default. This default may
* change over time. The maximum allowed value is 100, though requests with page_size greater
* than that will be silently interpreted as having this maximum value. This may increase in
* the futue.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of assignments to return. The service may return fewer than this value. If
omitted (or defaulted to zero) the server will use a sensible default. This default may change over
time. The maximum allowed value is 100, though requests with page_size greater than that will be
silently interpreted as having this maximum value. This may increase in the futue.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of assignments to return. The service may return fewer than this value.
* If omitted (or defaulted to zero) the server will use a sensible default. This default may
* change over time. The maximum allowed value is 100, though requests with page_size greater
* than that will be silently interpreted as having this maximum value. This may increase in
* the futue.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListInboundSsoAssignments` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListInboundSsoAssignments` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListInboundSsoAssignments` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListInboundSsoAssignments`
must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListInboundSsoAssignments` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListInboundSsoAssignments` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an InboundSsoAssignment. The body of this request is the `inbound_sso_assignment` field
* and the `update_mask` is relative to that. For example: a PATCH to
* `/v1/inboundSsoAssignments/0abcdefg1234567&update_mask=rank` with a body of `{ "rank": 1 }` moves
* that (presumably group-targeted) SSO assignment to the highest priority and shifts any other
* group-targeted assignments down in priority.
*
* Create a request for the method "inboundSsoAssignments.patch".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Inbound SSO
* Assignment.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudIdentityRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^inboundSsoAssignments/[^/]+$");
/**
* Updates an InboundSsoAssignment. The body of this request is the `inbound_sso_assignment` field
* and the `update_mask` is relative to that. For example: a PATCH to
* `/v1/inboundSsoAssignments/0abcdefg1234567&update_mask=rank` with a body of `{ "rank": 1 }`
* moves that (presumably group-targeted) SSO assignment to the highest priority and shifts any
* other group-targeted assignments down in priority.
*
* Create a request for the method "inboundSsoAssignments.patch".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Inbound SSO
* Assignment.
* @param content the {@link com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudidentity.v1.model.InboundSsoAssignment content) {
super(CloudIdentity.this, "PATCH", REST_PATH, content, com.google.api.services.cloudidentity.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSsoAssignments/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Inbound SSO Assignment.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the Inbound
SSO Assignment.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. [Resource name](https://cloud.google.com/apis/design/resource_names) of the
* Inbound SSO Assignment.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^inboundSsoAssignments/[^/]+$");
}
this.name = name;
return this;
}
/** Required. The list of fields to be updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. The list of fields to be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. The list of fields to be updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link CloudIdentity}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudIdentity}. */
@Override
public CloudIdentity build() {
return new CloudIdentity(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudIdentityRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudIdentityRequestInitializer(
CloudIdentityRequestInitializer cloudidentityRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudidentityRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}