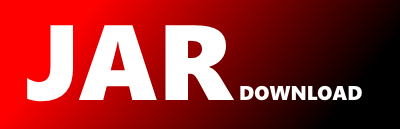
com.google.api.services.cloudidentity.v1.model.EntityKey Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudidentity.v1.model;
/**
* A unique identifier for an entity in the Cloud Identity Groups API. An entity can represent
* either a group with an optional `namespace` or a user without a `namespace`. The combination of
* `id` and `namespace` must be unique; however, the same `id` can be used with different
* `namespace`s.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Identity API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class EntityKey extends com.google.api.client.json.GenericJson {
/**
* The ID of the entity. For Google-managed entities, the `id` should be the email address of an
* existing group or user. Email addresses need to adhere to [name guidelines for users and
* groups](https://support.google.com/a/answer/9193374). For external-identity-mapped entities,
* the `id` must be a string conforming to the Identity Source's requirements. Must be unique
* within a `namespace`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The namespace in which the entity exists. If not specified, the `EntityKey` represents a
* Google-managed entity such as a Google user or a Google Group. If specified, the `EntityKey`
* represents an external-identity-mapped group. The namespace must correspond to an identity
* source created in Admin Console and must be in the form of `identitysources/{identity_source}`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String namespace;
/**
* The ID of the entity. For Google-managed entities, the `id` should be the email address of an
* existing group or user. Email addresses need to adhere to [name guidelines for users and
* groups](https://support.google.com/a/answer/9193374). For external-identity-mapped entities,
* the `id` must be a string conforming to the Identity Source's requirements. Must be unique
* within a `namespace`.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the entity. For Google-managed entities, the `id` should be the email address of an
* existing group or user. Email addresses need to adhere to [name guidelines for users and
* groups](https://support.google.com/a/answer/9193374). For external-identity-mapped entities,
* the `id` must be a string conforming to the Identity Source's requirements. Must be unique
* within a `namespace`.
* @param id id or {@code null} for none
*/
public EntityKey setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The namespace in which the entity exists. If not specified, the `EntityKey` represents a
* Google-managed entity such as a Google user or a Google Group. If specified, the `EntityKey`
* represents an external-identity-mapped group. The namespace must correspond to an identity
* source created in Admin Console and must be in the form of `identitysources/{identity_source}`.
* @return value or {@code null} for none
*/
public java.lang.String getNamespace() {
return namespace;
}
/**
* The namespace in which the entity exists. If not specified, the `EntityKey` represents a
* Google-managed entity such as a Google user or a Google Group. If specified, the `EntityKey`
* represents an external-identity-mapped group. The namespace must correspond to an identity
* source created in Admin Console and must be in the form of `identitysources/{identity_source}`.
* @param namespace namespace or {@code null} for none
*/
public EntityKey setNamespace(java.lang.String namespace) {
this.namespace = namespace;
return this;
}
@Override
public EntityKey set(String fieldName, Object value) {
return (EntityKey) super.set(fieldName, value);
}
@Override
public EntityKey clone() {
return (EntityKey) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy