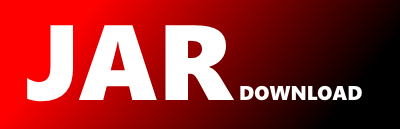
com.google.api.services.cloudidentity.v1beta1.CloudIdentity Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudidentity.v1beta1;
/**
* Service definition for CloudIdentity (v1beta1).
*
*
* API for provisioning and managing identity resources.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudIdentityRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudIdentity extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.30.9 of the Cloud Identity API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://cloudidentity.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudIdentity(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudIdentity(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Groups collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.Groups.List request = cloudidentity.groups().list(parameters ...)}
*
*
* @return the resource collection
*/
public Groups groups() {
return new Groups();
}
/**
* The "groups" collection of methods.
*/
public class Groups {
/**
* Creates a `Group`.
*
* Create a request for the method "groups.create".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.Group}
* @return the request
*/
public Create create(com.google.api.services.cloudidentity.v1beta1.model.Group content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/groups";
/**
* Creates a `Group`.
*
* Create a request for the method "groups.create".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.Group}
* @since 1.13
*/
protected Create(com.google.api.services.cloudidentity.v1beta1.model.Group content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1beta1.model.Operation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The initial configuration option for the `Group`. */
@com.google.api.client.util.Key
private java.lang.String initialGroupConfig;
/** Required. The initial configuration option for the `Group`.
*/
public java.lang.String getInitialGroupConfig() {
return initialGroupConfig;
}
/** Required. The initial configuration option for the `Group`. */
public Create setInitialGroupConfig(java.lang.String initialGroupConfig) {
this.initialGroupConfig = initialGroupConfig;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `Group`.
*
* Create a request for the method "groups.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the `Group` to
* retrieve.
Must be of the form `groups/{group_id}`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Deletes a `Group`.
*
* Create a request for the method "groups.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the `Group` to
* retrieve.
Must be of the form `groups/{group_id}`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve.
*
* Must be of the form `groups/{group_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group`
to retrieve.
Must be of the form `groups/{group_id}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve.
*
* Must be of the form `groups/{group_id}`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `Group`.
*
* Create a request for the method "groups.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the `Group` to
* retrieve.
Must be of the form `groups/{group_id}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Retrieves a `Group`.
*
* Create a request for the method "groups.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the `Group` to
* retrieve.
Must be of the form `groups/{group_id}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.Group.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve.
*
* Must be of the form `groups/{group_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group`
to retrieve.
Must be of the form `groups/{group_id}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Group` to retrieve.
*
* Must be of the form `groups/{group_id}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the `Group`s under a customer or namespace.
*
* Create a request for the method "groups.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/groups";
/**
* Lists the `Group`s under a customer or namespace.
*
* Create a request for the method "groups.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.ListGroupsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of results to return.
*
* Note that the number of results returned may be less than this value even if there are more
* available results. To fetch all results, clients must continue calling this method
* repeatedly until the response no longer contains a `next_page_token`.
*
* If unspecified, defaults to 200 for `View.BASIC` and to 50 for `View.FULL`.
*
* Must not be greater than 1000 for `View.BASIC` or 500 for `View.FULL`.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return.
Note that the number of results returned may be less than this value even if there are more
available results. To fetch all results, clients must continue calling this method repeatedly until
the response no longer contains a `next_page_token`.
If unspecified, defaults to 200 for `View.BASIC` and to 50 for `View.FULL`.
Must not be greater than 1000 for `View.BASIC` or 500 for `View.FULL`.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return.
*
* Note that the number of results returned may be less than this value even if there are more
* available results. To fetch all results, clients must continue calling this method
* repeatedly until the response no longer contains a `next_page_token`.
*
* If unspecified, defaults to 200 for `View.BASIC` and to 50 for `View.FULL`.
*
* Must not be greater than 1000 for `View.BASIC` or 500 for `View.FULL`.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The `next_page_token` value returned from a previous list request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous list request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The `next_page_token` value returned from a previous list request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. The parent resource under which to list all `Group`s.
*
* Must be of the form `identitysources/{identity_source_id}` for external- identity-mapped
* groups or `customers/{customer_id}` for Google Groups.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource under which to list all `Group`s.
Must be of the form `identitysources/{identity_source_id}` for external- identity-mapped groups or
`customers/{customer_id}` for Google Groups.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource under which to list all `Group`s.
*
* Must be of the form `identitysources/{identity_source_id}` for external- identity-mapped
* groups or `customers/{customer_id}` for Google Groups.
*/
public List setParent(java.lang.String parent) {
this.parent = parent;
return this;
}
/**
* The level of detail to be returned.
*
* If unspecified, defaults to `View.BASIC`.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** The level of detail to be returned.
If unspecified, defaults to `View.BASIC`.
*/
public java.lang.String getView() {
return view;
}
/**
* The level of detail to be returned.
*
* If unspecified, defaults to `View.BASIC`.
*/
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a `Group` by
* its `EntityKey`.
*
* Create a request for the method "groups.lookup".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Lookup lookup() throws java.io.IOException {
Lookup result = new Lookup();
initialize(result);
return result;
}
public class Lookup extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/groups:lookup";
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a `Group`
* by its `EntityKey`.
*
* Create a request for the method "groups.lookup".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
* {@link
* Lookup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Lookup() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.LookupGroupNameResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Lookup set$Xgafv(java.lang.String $Xgafv) {
return (Lookup) super.set$Xgafv($Xgafv);
}
@Override
public Lookup setAccessToken(java.lang.String accessToken) {
return (Lookup) super.setAccessToken(accessToken);
}
@Override
public Lookup setAlt(java.lang.String alt) {
return (Lookup) super.setAlt(alt);
}
@Override
public Lookup setCallback(java.lang.String callback) {
return (Lookup) super.setCallback(callback);
}
@Override
public Lookup setFields(java.lang.String fields) {
return (Lookup) super.setFields(fields);
}
@Override
public Lookup setKey(java.lang.String key) {
return (Lookup) super.setKey(key);
}
@Override
public Lookup setOauthToken(java.lang.String oauthToken) {
return (Lookup) super.setOauthToken(oauthToken);
}
@Override
public Lookup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Lookup) super.setPrettyPrint(prettyPrint);
}
@Override
public Lookup setQuotaUser(java.lang.String quotaUser) {
return (Lookup) super.setQuotaUser(quotaUser);
}
@Override
public Lookup setUploadType(java.lang.String uploadType) {
return (Lookup) super.setUploadType(uploadType);
}
@Override
public Lookup setUploadProtocol(java.lang.String uploadProtocol) {
return (Lookup) super.setUploadProtocol(uploadProtocol);
}
/**
* The ID of the entity.
*
* For Google-managed entities, the `id` must be the email address of an existing group or
* user.
*
* For external-identity-mapped entities, the `id` must be a string conforming to the Identity
* Source's requirements.
*
* Must be unique within a `namespace`.
*/
@com.google.api.client.util.Key("groupKey.id")
private java.lang.String groupKeyId;
/** The ID of the entity.
For Google-managed entities, the `id` must be the email address of an existing group or user.
For external-identity-mapped entities, the `id` must be a string conforming to the Identity
Source's requirements.
Must be unique within a `namespace`.
*/
public java.lang.String getGroupKeyId() {
return groupKeyId;
}
/**
* The ID of the entity.
*
* For Google-managed entities, the `id` must be the email address of an existing group or
* user.
*
* For external-identity-mapped entities, the `id` must be a string conforming to the Identity
* Source's requirements.
*
* Must be unique within a `namespace`.
*/
public Lookup setGroupKeyId(java.lang.String groupKeyId) {
this.groupKeyId = groupKeyId;
return this;
}
/**
* The namespace in which the entity exists.
*
* If not specified, the `EntityKey` represents a Google-managed entity such as a Google user
* or a Google Group.
*
* If specified, the `EntityKey` represents an external-identity-mapped group. The namespace
* must correspond to an identity source created in Admin Console. Must be of the form
* `identitysources/{identity_source_id}.
*/
@com.google.api.client.util.Key("groupKey.namespace")
private java.lang.String groupKeyNamespace;
/** The namespace in which the entity exists.
If not specified, the `EntityKey` represents a Google-managed entity such as a Google user or a
Google Group.
If specified, the `EntityKey` represents an external-identity-mapped group. The namespace must
correspond to an identity source created in Admin Console. Must be of the form
`identitysources/{identity_source_id}.
*/
public java.lang.String getGroupKeyNamespace() {
return groupKeyNamespace;
}
/**
* The namespace in which the entity exists.
*
* If not specified, the `EntityKey` represents a Google-managed entity such as a Google user
* or a Google Group.
*
* If specified, the `EntityKey` represents an external-identity-mapped group. The namespace
* must correspond to an identity source created in Admin Console. Must be of the form
* `identitysources/{identity_source_id}.
*/
public Lookup setGroupKeyNamespace(java.lang.String groupKeyNamespace) {
this.groupKeyNamespace = groupKeyNamespace;
return this;
}
@Override
public Lookup set(String parameterName, Object value) {
return (Lookup) super.set(parameterName, value);
}
}
/**
* Updates a `Group`.
*
* Create a request for the method "groups.patch".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Group`.
Shall be of the form `groups/{group_id}`.
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.Group}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudidentity.v1beta1.model.Group content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Updates a `Group`.
*
* Create a request for the method "groups.patch".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Group`.
Shall be of the form `groups/{group_id}`.
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.Group}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudidentity.v1beta1.model.Group content) {
super(CloudIdentity.this, "PATCH", REST_PATH, content, com.google.api.services.cloudidentity.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of
* the `Group`.
*
* Shall be of the form `groups/{group_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Group`.
Shall be of the form `groups/{group_id}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The [resource name](https://cloud.google.com/apis/design/resource_names) of
* the `Group`.
*
* Shall be of the form `groups/{group_id}`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The fully-qualified names of fields to update.
*
* May only contain the following fields: `display_name`, `description`.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The fully-qualified names of fields to update.
May only contain the following fields: `display_name`, `description`.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The fully-qualified names of fields to update.
*
* May only contain the following fields: `display_name`, `description`.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Searches for `Group`s matching a specified query.
*
* Create a request for the method "groups.search".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Search search() throws java.io.IOException {
Search result = new Search();
initialize(result);
return result;
}
public class Search extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/groups:search";
/**
* Searches for `Group`s matching a specified query.
*
* Create a request for the method "groups.search".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Search() {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.SearchGroupsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of results to return.
*
* Note that the number of results returned may be less than this value even if there are more
* available results. To fetch all results, clients must continue calling this method
* repeatedly until the response no longer contains a `next_page_token`.
*
* If unspecified, defaults to 200 for `GroupView.BASIC` and to 50 for `GroupView.FULL`.
*
* Must not be greater than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return.
Note that the number of results returned may be less than this value even if there are more
available results. To fetch all results, clients must continue calling this method repeatedly until
the response no longer contains a `next_page_token`.
If unspecified, defaults to 200 for `GroupView.BASIC` and to 50 for `GroupView.FULL`.
Must not be greater than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return.
*
* Note that the number of results returned may be less than this value even if there are more
* available results. To fetch all results, clients must continue calling this method
* repeatedly until the response no longer contains a `next_page_token`.
*
* If unspecified, defaults to 200 for `GroupView.BASIC` and to 50 for `GroupView.FULL`.
*
* Must not be greater than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
public Search setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The `next_page_token` value returned from a previous search request, if any.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous search request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The `next_page_token` value returned from a previous search request, if any.
*/
public Search setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. The search query.
*
* Must be specified in [Common Expression Language](https://opensource.google/projects/cel).
* May only contain equality operators on the parent and inclusion operators on labels (e.g.,
* `parent == 'customers/{customer_id}' &&
* 'cloudidentity.googleapis.com/groups.discussion_forum' in labels`).
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. The search query.
Must be specified in [Common Expression Language](https://opensource.google/projects/cel). May only
contain equality operators on the parent and inclusion operators on labels (e.g., `parent ==
'customers/{customer_id}' && 'cloudidentity.googleapis.com/groups.discussion_forum' in labels`).
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. The search query.
*
* Must be specified in [Common Expression Language](https://opensource.google/projects/cel).
* May only contain equality operators on the parent and inclusion operators on labels (e.g.,
* `parent == 'customers/{customer_id}' &&
* 'cloudidentity.googleapis.com/groups.discussion_forum' in labels`).
*/
public Search setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* The level of detail to be returned.
*
* If unspecified, defaults to `View.BASIC`.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** The level of detail to be returned.
If unspecified, defaults to `View.BASIC`.
*/
public java.lang.String getView() {
return view;
}
/**
* The level of detail to be returned.
*
* If unspecified, defaults to `View.BASIC`.
*/
public Search setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Memberships collection.
*
* The typical use is:
*
* {@code CloudIdentity cloudidentity = new CloudIdentity(...);}
* {@code CloudIdentity.Memberships.List request = cloudidentity.memberships().list(parameters ...)}
*
*
* @return the resource collection
*/
public Memberships memberships() {
return new Memberships();
}
/**
* The "memberships" collection of methods.
*/
public class Memberships {
/**
* Creates a `Membership`.
*
* Create a request for the method "memberships.create".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent `Group` resource under which to create the `Membership`.
Must be of the form
* `groups/{group_id}`.
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.Membership}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudidentity.v1beta1.model.Membership content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+parent}/memberships";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Creates a `Membership`.
*
* Create a request for the method "memberships.create".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent `Group` resource under which to create the `Membership`.
Must be of the form
* `groups/{group_id}`.
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.Membership}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudidentity.v1beta1.model.Membership content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1beta1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent `Group` resource under which to create the `Membership`.
*
* Must be of the form `groups/{group_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent `Group` resource under which to create the `Membership`.
Must be of the form `groups/{group_id}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent `Group` resource under which to create the `Membership`.
*
* Must be of the form `groups/{group_id}`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `Membership`.
*
* Create a request for the method "memberships.delete".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Membership` to delete.
Must be of the form
* `groups/{group_id}/memberships/{membership_id}`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/memberships/[^/]+$");
/**
* Deletes a `Membership`.
*
* Create a request for the method "memberships.delete".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Membership` to delete.
Must be of the form
* `groups/{group_id}/memberships/{membership_id}`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIdentity.this, "DELETE", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to delete.
*
* Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Membership` to delete.
Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to delete.
*
* Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `Membership`.
*
* Create a request for the method "memberships.get".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Membership` to retrieve.
Must be of the form
* `groups/{group_id}/memberships/{membership_id}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/memberships/[^/]+$");
/**
* Retrieves a `Membership`.
*
* Create a request for the method "memberships.get".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Membership` to retrieve.
Must be of the form
* `groups/{group_id}/memberships/{membership_id}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.Membership.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to retrieve.
*
* Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Membership` to retrieve.
Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` to retrieve.
*
* Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the `Membership`s within a `Group`.
*
* Create a request for the method "memberships.list".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name.
Must be of the
* form `groups/{group_id}`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+parent}/memberships";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Lists the `Membership`s within a `Group`.
*
* Create a request for the method "memberships.list".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name.
Must be of the
* form `groups/{group_id}`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.ListMembershipsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name.
*
* Must be of the form `groups/{group_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent `Group` resource under which to lookup the `Membership` name.
Must be of the form `groups/{group_id}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name.
*
* Must be of the form `groups/{group_id}`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of results to return.
*
* Note that the number of results returned may be less than this value even if there are
* more available results. To fetch all results, clients must continue calling this method
* repeatedly until the response no longer contains a `next_page_token`.
*
* If unspecified, defaults to 200 for `GroupView.BASIC` and to 50 for `GroupView.FULL`.
*
* Must not be greater than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return.
Note that the number of results returned may be less than this value even if there are more
available results. To fetch all results, clients must continue calling this method repeatedly until
the response no longer contains a `next_page_token`.
If unspecified, defaults to 200 for `GroupView.BASIC` and to 50 for `GroupView.FULL`.
Must not be greater than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return.
*
* Note that the number of results returned may be less than this value even if there are
* more available results. To fetch all results, clients must continue calling this method
* repeatedly until the response no longer contains a `next_page_token`.
*
* If unspecified, defaults to 200 for `GroupView.BASIC` and to 50 for `GroupView.FULL`.
*
* Must not be greater than 1000 for `GroupView.BASIC` or 500 for `GroupView.FULL`.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The `next_page_token` value returned from a previous search request, if any.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous search request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The `next_page_token` value returned from a previous search request, if any.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The level of detail to be returned.
*
* If unspecified, defaults to `MembershipView.BASIC`.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** The level of detail to be returned.
If unspecified, defaults to `MembershipView.BASIC`.
*/
public java.lang.String getView() {
return view;
}
/**
* The level of detail to be returned.
*
* If unspecified, defaults to `MembershipView.BASIC`.
*/
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a
* `Membership` by its `EntityKey`.
*
* Create a request for the method "memberships.lookup".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name.
Must be of the
* form `groups/{group_id}`.
* @return the request
*/
public Lookup lookup(java.lang.String parent) throws java.io.IOException {
Lookup result = new Lookup(parent);
initialize(result);
return result;
}
public class Lookup extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+parent}/memberships:lookup";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+$");
/**
* Looks up the [resource name](https://cloud.google.com/apis/design/resource_names) of a
* `Membership` by its `EntityKey`.
*
* Create a request for the method "memberships.lookup".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
* {@link
* Lookup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent `Group` resource under which to lookup the `Membership` name.
Must be of the
* form `groups/{group_id}`.
* @since 1.13
*/
protected Lookup(java.lang.String parent) {
super(CloudIdentity.this, "GET", REST_PATH, null, com.google.api.services.cloudidentity.v1beta1.model.LookupMembershipNameResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Lookup set$Xgafv(java.lang.String $Xgafv) {
return (Lookup) super.set$Xgafv($Xgafv);
}
@Override
public Lookup setAccessToken(java.lang.String accessToken) {
return (Lookup) super.setAccessToken(accessToken);
}
@Override
public Lookup setAlt(java.lang.String alt) {
return (Lookup) super.setAlt(alt);
}
@Override
public Lookup setCallback(java.lang.String callback) {
return (Lookup) super.setCallback(callback);
}
@Override
public Lookup setFields(java.lang.String fields) {
return (Lookup) super.setFields(fields);
}
@Override
public Lookup setKey(java.lang.String key) {
return (Lookup) super.setKey(key);
}
@Override
public Lookup setOauthToken(java.lang.String oauthToken) {
return (Lookup) super.setOauthToken(oauthToken);
}
@Override
public Lookup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Lookup) super.setPrettyPrint(prettyPrint);
}
@Override
public Lookup setQuotaUser(java.lang.String quotaUser) {
return (Lookup) super.setQuotaUser(quotaUser);
}
@Override
public Lookup setUploadType(java.lang.String uploadType) {
return (Lookup) super.setUploadType(uploadType);
}
@Override
public Lookup setUploadProtocol(java.lang.String uploadProtocol) {
return (Lookup) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name.
*
* Must be of the form `groups/{group_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent `Group` resource under which to lookup the `Membership` name.
Must be of the form `groups/{group_id}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent `Group` resource under which to lookup the `Membership` name.
*
* Must be of the form `groups/{group_id}`.
*/
public Lookup setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^groups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The ID of the entity.
*
* For Google-managed entities, the `id` must be the email address of an existing group or
* user.
*
* For external-identity-mapped entities, the `id` must be a string conforming to the
* Identity Source's requirements.
*
* Must be unique within a `namespace`.
*/
@com.google.api.client.util.Key("memberKey.id")
private java.lang.String memberKeyId;
/** The ID of the entity.
For Google-managed entities, the `id` must be the email address of an existing group or user.
For external-identity-mapped entities, the `id` must be a string conforming to the Identity
Source's requirements.
Must be unique within a `namespace`.
*/
public java.lang.String getMemberKeyId() {
return memberKeyId;
}
/**
* The ID of the entity.
*
* For Google-managed entities, the `id` must be the email address of an existing group or
* user.
*
* For external-identity-mapped entities, the `id` must be a string conforming to the
* Identity Source's requirements.
*
* Must be unique within a `namespace`.
*/
public Lookup setMemberKeyId(java.lang.String memberKeyId) {
this.memberKeyId = memberKeyId;
return this;
}
/**
* The namespace in which the entity exists.
*
* If not specified, the `EntityKey` represents a Google-managed entity such as a Google
* user or a Google Group.
*
* If specified, the `EntityKey` represents an external-identity-mapped group. The namespace
* must correspond to an identity source created in Admin Console. Must be of the form
* `identitysources/{identity_source_id}.
*/
@com.google.api.client.util.Key("memberKey.namespace")
private java.lang.String memberKeyNamespace;
/** The namespace in which the entity exists.
If not specified, the `EntityKey` represents a Google-managed entity such as a Google user or a
Google Group.
If specified, the `EntityKey` represents an external-identity-mapped group. The namespace must
correspond to an identity source created in Admin Console. Must be of the form
`identitysources/{identity_source_id}.
*/
public java.lang.String getMemberKeyNamespace() {
return memberKeyNamespace;
}
/**
* The namespace in which the entity exists.
*
* If not specified, the `EntityKey` represents a Google-managed entity such as a Google
* user or a Google Group.
*
* If specified, the `EntityKey` represents an external-identity-mapped group. The namespace
* must correspond to an identity source created in Admin Console. Must be of the form
* `identitysources/{identity_source_id}.
*/
public Lookup setMemberKeyNamespace(java.lang.String memberKeyNamespace) {
this.memberKeyNamespace = memberKeyNamespace;
return this;
}
@Override
public Lookup set(String parameterName, Object value) {
return (Lookup) super.set(parameterName, value);
}
}
/**
* Modifies the `MembershipRole`s of a `Membership`.
*
* Create a request for the method "memberships.modifyMembershipRoles".
*
* This request holds the parameters needed by the cloudidentity server. After setting any optional
* parameters, call the {@link ModifyMembershipRoles#execute()} method to invoke the remote
* operation.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Membership` whose roles are to be modified.
Must be of the form
* `groups/{group_id}/memberships/{membership_id}`.
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.ModifyMembershipRolesRequest}
* @return the request
*/
public ModifyMembershipRoles modifyMembershipRoles(java.lang.String name, com.google.api.services.cloudidentity.v1beta1.model.ModifyMembershipRolesRequest content) throws java.io.IOException {
ModifyMembershipRoles result = new ModifyMembershipRoles(name, content);
initialize(result);
return result;
}
public class ModifyMembershipRoles extends CloudIdentityRequest {
private static final String REST_PATH = "v1beta1/{+name}:modifyMembershipRoles";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^groups/[^/]+/memberships/[^/]+$");
/**
* Modifies the `MembershipRole`s of a `Membership`.
*
* Create a request for the method "memberships.modifyMembershipRoles".
*
* This request holds the parameters needed by the the cloudidentity server. After setting any
* optional parameters, call the {@link ModifyMembershipRoles#execute()} method to invoke the
* remote operation. {@link ModifyMembershipRoles#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of
the
* `Membership` whose roles are to be modified.
Must be of the form
* `groups/{group_id}/memberships/{membership_id}`.
* @param content the {@link com.google.api.services.cloudidentity.v1beta1.model.ModifyMembershipRolesRequest}
* @since 1.13
*/
protected ModifyMembershipRoles(java.lang.String name, com.google.api.services.cloudidentity.v1beta1.model.ModifyMembershipRolesRequest content) {
super(CloudIdentity.this, "POST", REST_PATH, content, com.google.api.services.cloudidentity.v1beta1.model.ModifyMembershipRolesResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
}
@Override
public ModifyMembershipRoles set$Xgafv(java.lang.String $Xgafv) {
return (ModifyMembershipRoles) super.set$Xgafv($Xgafv);
}
@Override
public ModifyMembershipRoles setAccessToken(java.lang.String accessToken) {
return (ModifyMembershipRoles) super.setAccessToken(accessToken);
}
@Override
public ModifyMembershipRoles setAlt(java.lang.String alt) {
return (ModifyMembershipRoles) super.setAlt(alt);
}
@Override
public ModifyMembershipRoles setCallback(java.lang.String callback) {
return (ModifyMembershipRoles) super.setCallback(callback);
}
@Override
public ModifyMembershipRoles setFields(java.lang.String fields) {
return (ModifyMembershipRoles) super.setFields(fields);
}
@Override
public ModifyMembershipRoles setKey(java.lang.String key) {
return (ModifyMembershipRoles) super.setKey(key);
}
@Override
public ModifyMembershipRoles setOauthToken(java.lang.String oauthToken) {
return (ModifyMembershipRoles) super.setOauthToken(oauthToken);
}
@Override
public ModifyMembershipRoles setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ModifyMembershipRoles) super.setPrettyPrint(prettyPrint);
}
@Override
public ModifyMembershipRoles setQuotaUser(java.lang.String quotaUser) {
return (ModifyMembershipRoles) super.setQuotaUser(quotaUser);
}
@Override
public ModifyMembershipRoles setUploadType(java.lang.String uploadType) {
return (ModifyMembershipRoles) super.setUploadType(uploadType);
}
@Override
public ModifyMembershipRoles setUploadProtocol(java.lang.String uploadProtocol) {
return (ModifyMembershipRoles) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` whose roles are to be modified.
*
* Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
`Membership` whose roles are to be modified.
Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The [resource name](https://cloud.google.com/apis/design/resource_names) of the
* `Membership` whose roles are to be modified.
*
* Must be of the form `groups/{group_id}/memberships/{membership_id}`.
*/
public ModifyMembershipRoles setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^groups/[^/]+/memberships/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ModifyMembershipRoles set(String parameterName, Object value) {
return (ModifyMembershipRoles) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link CloudIdentity}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudIdentity}. */
@Override
public CloudIdentity build() {
return new CloudIdentity(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudIdentityRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudIdentityRequestInitializer(
CloudIdentityRequestInitializer cloudidentityRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudidentityRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}